package org.qhit.source.struts.action;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.util.List;
import javax.annotation.Resource;
import javax.imageio.ImageIO;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.apache.struts2.ServletActionContext;
import org.json.JSONObject;
import org.qhit.source.common.common;
import org.qhit.source.dao.IUploadDAO;
import org.qhit.source.service.commService;
import org.qhit.source.vo.Phototype;
import org.qhit.source.vo.Showimg;
import com.opensymphony.xwork2.Action;
import com.opensymphony.xwork2.ActionSupport;
@SuppressWarnings("serial")
public class photoAction extends ActionSupport {
//注入数据层的dao
@Resource private IUploadDAO iudao;
private HttpServletRequest request;
private HttpServletResponse response;
//查询相册类型
public void seacherType() throws Exception {
// TODO Auto-generated method stub
request = ServletActionContext.getRequest();
HttpSession session=request.getSession();
request.setCharacterEncoding("gbk");
List<Phototype> list = iudao.getTypeList();
session.setAttribute("photoType", list);
}
//查询所有相片
public void searchAllPhoto() throws Exception{
request = ServletActionContext.getRequest();
HttpSession session=request.getSession();
request.setCharacterEncoding("gbk");
List<Showimg> list=commService.ChangeDate(iudao.getPhotoAll());
session.setAttribute("photoAll", list);
}
//首页显示的控制类
public String showPhotoExecute() throws Exception{
this.seacherType();
this.searchAllPhoto();
return Action.SUCCESS;
}
//图片上传
private List<File> file;
private List<String> fileFileName;
private List<String> fileContentType;
private int typeid; //上传的位置
//图片上传
public String uploadPhoto(){
InputStream in=null;
OutputStream out=null;
try{
String dir=ServletActionContext.getRequest().getRealPath("/upload");
for(int i=0; i<file.size(); i++){
in = new FileInputStream(file.get(i));
File upfile=new File(dir,fileFileName.get(i));
out = new FileOutputStream(upfile);
byte[] buff=new byte[1024];
int length=0;
while((length=in.read(buff))!=-1){
out.write(buff,0,length);
}
//见图片信息插入到数据库
savePhotoInfo(upfile);
}
}catch(Exception e){
e.printStackTrace();
}finally{
try{
in.close();
out.close();
}catch(IOException e){e.printStackTrace();}
}
return Action.SUCCESS;
}
//将上传的图片信息插入到数据库
public void savePhotoInfo(File file){
try{
BufferedImage buff = ImageIO.read(file);
Showimg img=new Showimg();
//得到图片的信息
int height=buff.getHeight();
int width=buff.getWidth();
if(width<700){
img.setWidth(width);
}else{
img.setWidth(600);
}
if(height<600){
img.setHeight(height);
}else{
img.setHeight(500);
}
img.setPhotoname(file.getName());
img.setPhototype((Phototype)iudao.load(typeid, Phototype.class));
iudao.addObject(img);
}catch(IOException e){
e.printStackTrace();
System.out.println("---- "+file.getName()+" -----文件上传失败。");
}
}
//创建相册类型
private Phototype ptype;
public String createTypeExecute() throws Exception{
iudao.addObject(ptype);
//跟新类型
this.seacherType();
return Action.SUCCESS;
}
//按相册类型查询图片
public String searchPartPhoto() throws Exception{
request = ServletActionContext.getRequest();
HttpSession session=request.getSession();
request.setCharacterEncoding("gbk");
int tid=Integer.parseInt(request.getParameter("typeid"));
if(tid==0){
this.searchAllPhoto();
}else{
List<Showimg> list = commService.ChangeDate(iudao.getPhoto(tid));
session.setAttribute("photoAll", list);
}
return Action.SUCCESS;
}
//上一张和下一张时输出图片信息
@SuppressWarnings("unchecked")
public void findPhoto()throws Exception{
request=ServletActionContext.getRequest();
response=ServletActionContext.getResponse();
request.setCharacterEncoding("gbk");
response.setContentType("text/html;charset=gbk");
PrintWriter out=response.getWriter();
String pname = request.getParameter("imgName");
String par=request.getParameter("parameter");
List<Showimg> list = (List<Showimg>)request.getSession().getAttribute("photoAll");
if(pname!=null){
for(int i=0; i<list.size(); i++){
Showimg img=(Showimg)list.get(i);
if(img.getPhotoname().equals(pname)){
JSONObject json=new JSONObject();
json.put("height", img.getHeight());
json.put("width", img.getWidth());
json.put("sdate", common.formatDate(img.getUdate()));
out.write(json.toString());
}
}
}
//输出层中第一张图片的信息
if(par!=null&&"first".equals(par)){
Showimg img=(Showimg)list.get(0);
JSONObject json=new JSONObject();
json.put("height", img.getHeight());
json.put("width", img.getWidth());
json.put("sdate", common.formatDate(img.getUdate()));
out.write(json.toString());
}
}
public Phototype getPtype() {
return ptype;
}
public void setPtype(Phototype ptype) {
this.ptype = ptype;
}
public int getTypeid() {
return typeid;
}
public void setTypeid(int typeid) {
this.typeid = typeid;
}
public List<File> getFile() {
return file;
}
public void setFile(List<File> file) {
this.file = file;
}
public List<String> getFileFileName() {
return fileFileName;
}
public void setFileFileName(List<String> fileFileName) {
this.fileFileName = fileFileName;
}
public List<String> getFileContentType() {
return fileContentType;
}
public void setFileContentType(List<String> fileContentType) {
this.fileContentType = fileContentType;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
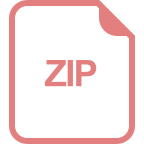
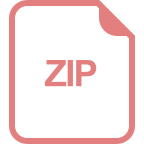
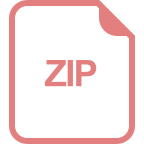
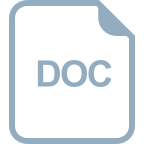
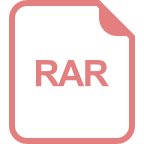
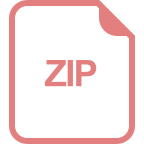
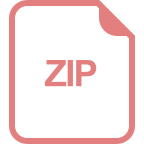
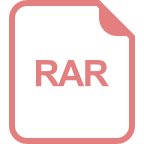
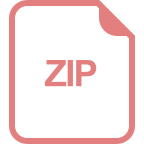
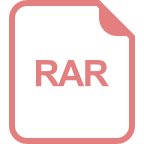
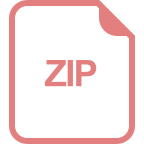
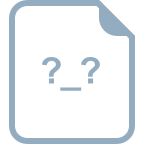
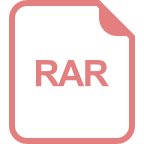
收起资源包目录

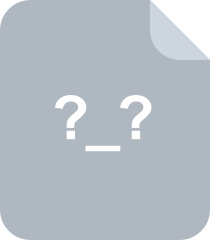
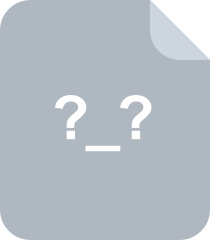
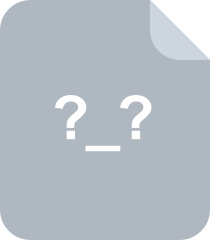
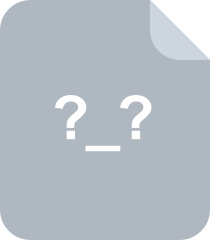
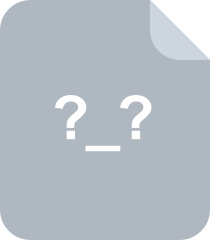
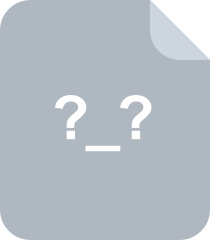
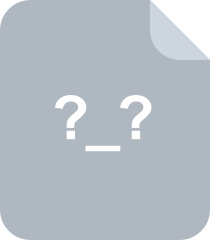
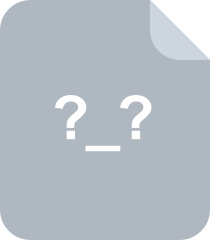
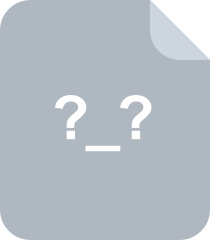
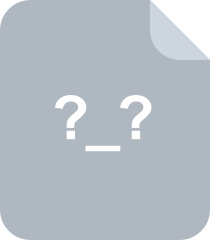
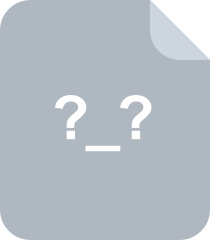
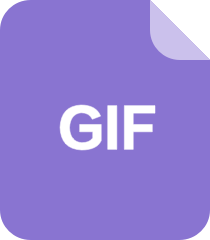
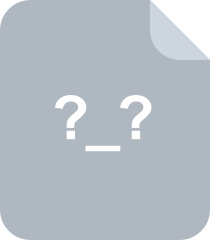
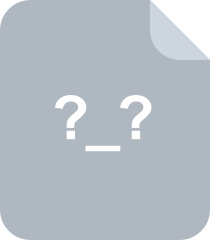
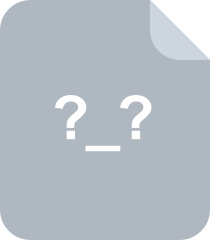
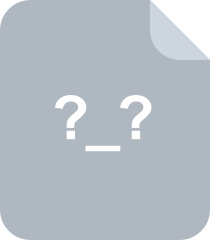
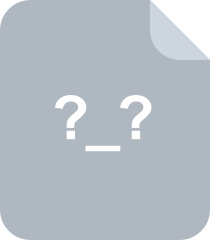
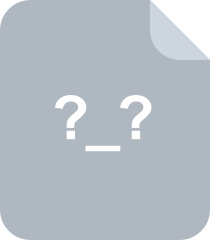
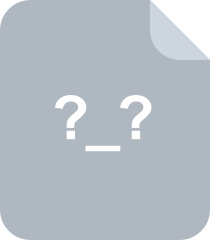
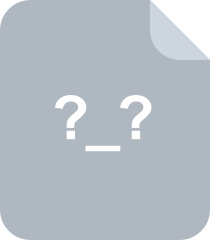
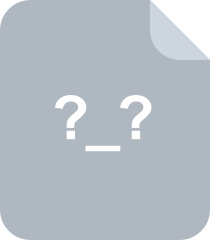
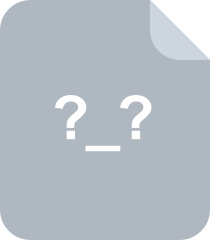
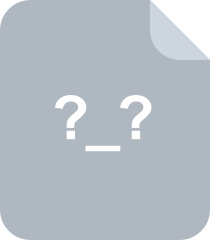
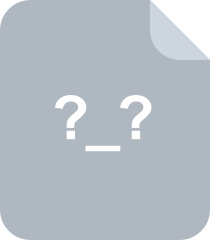
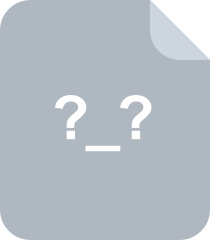
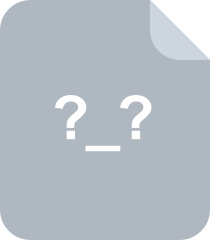
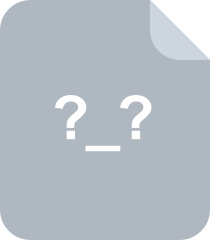
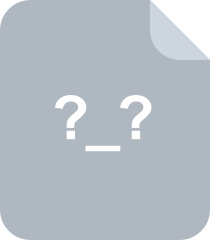
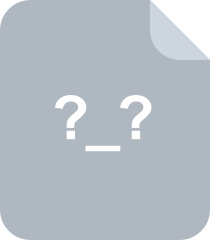
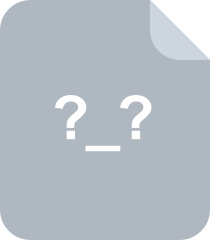
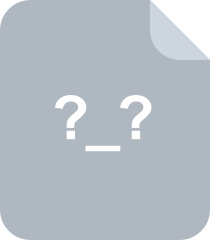
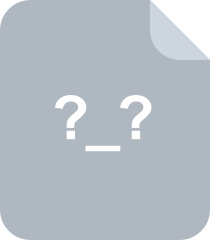
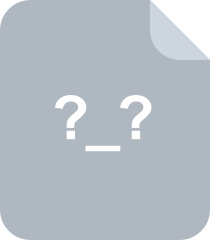
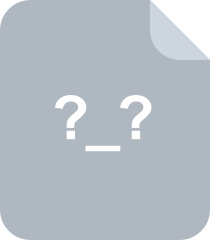
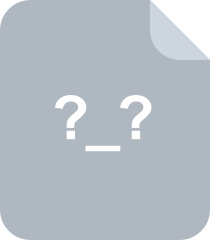
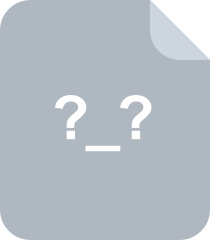
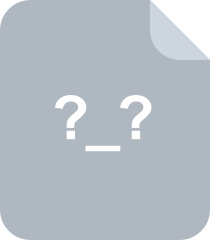
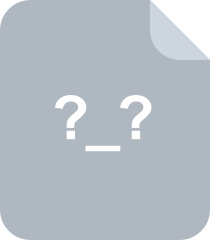
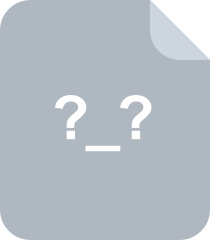
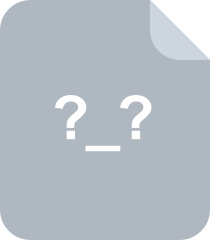
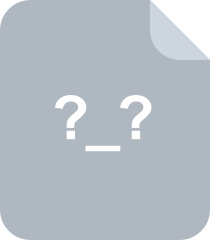
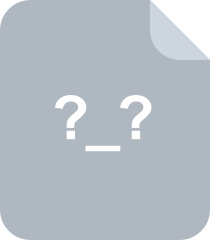
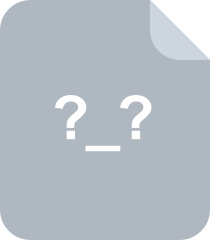
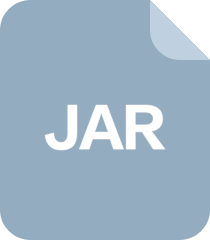
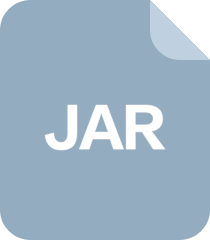
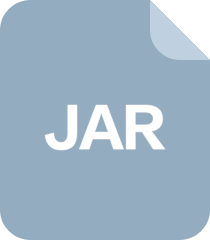
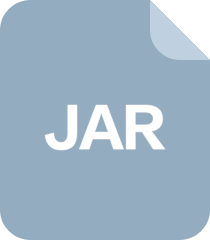
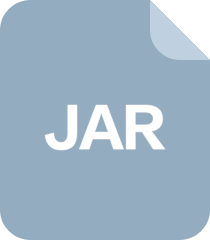
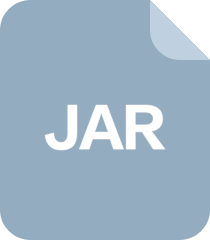
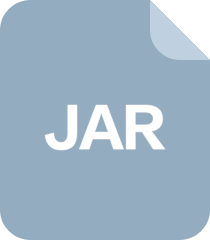
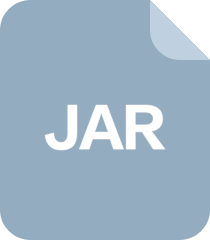
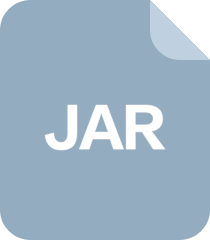
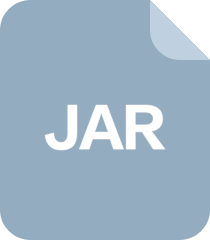
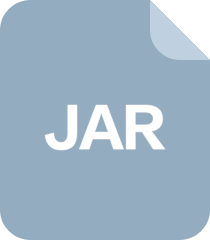
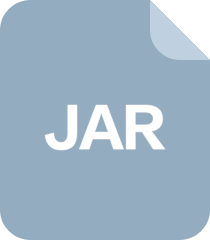
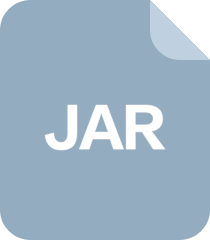
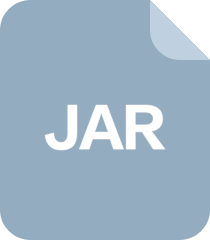
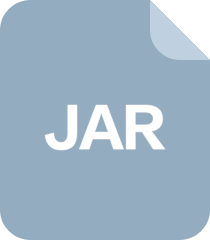
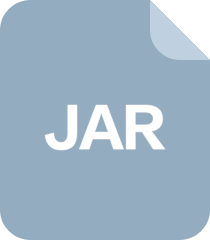
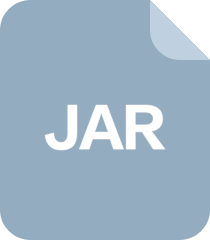
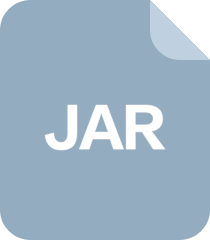
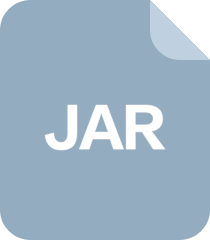
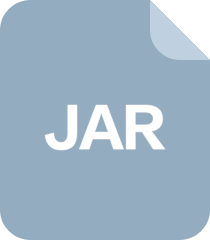
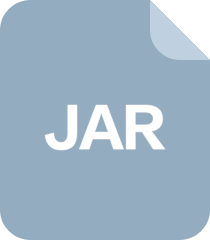
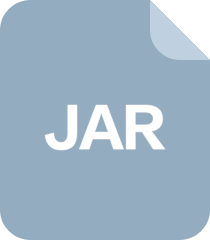
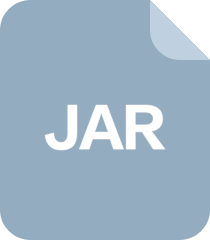
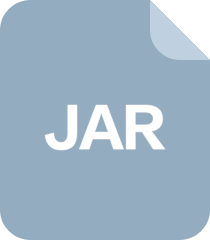
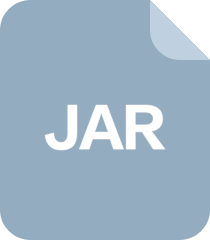
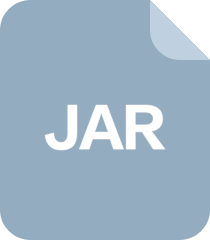
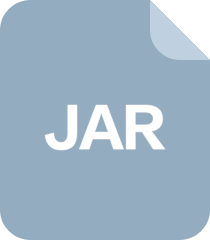
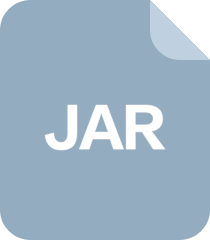
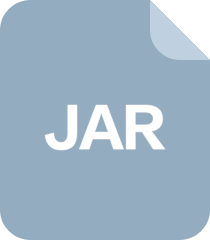
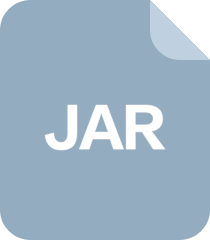
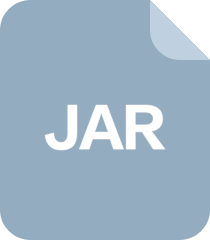
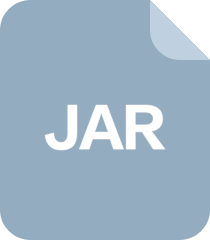
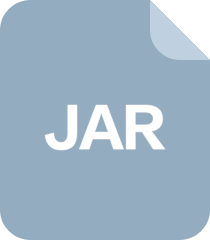
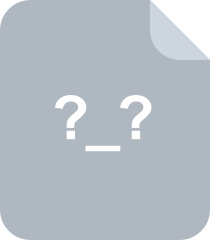
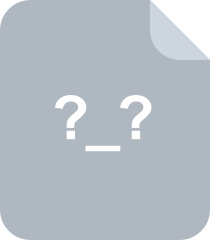
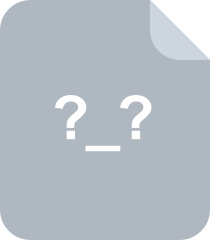
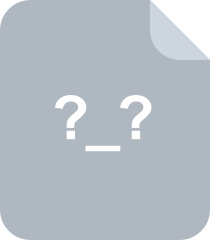
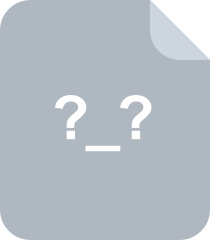
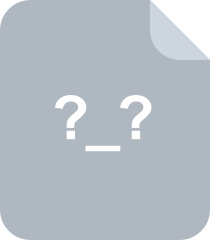
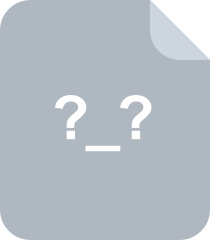
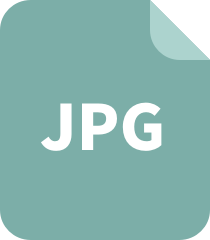
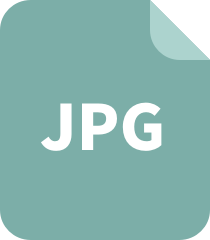
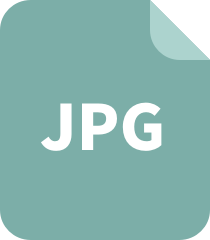
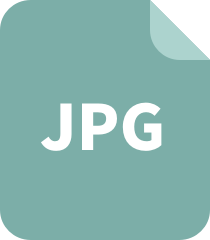
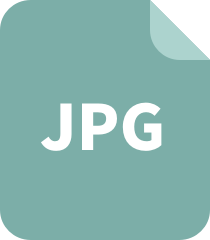
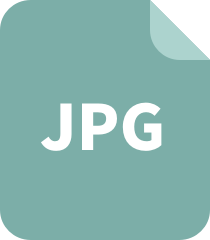
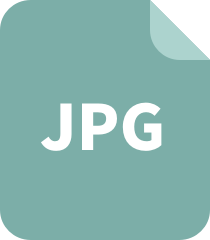
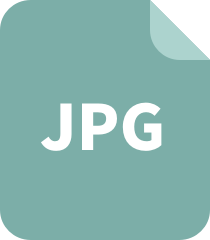
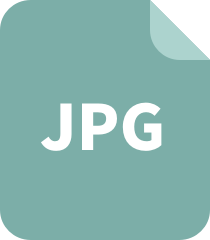
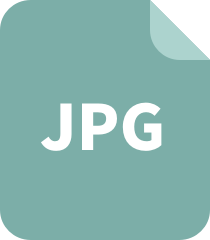
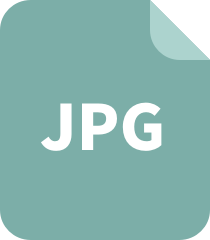
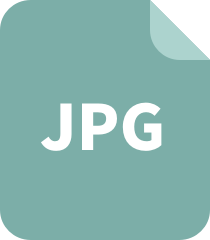
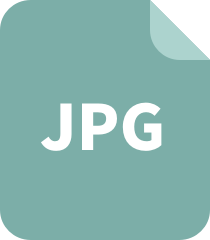
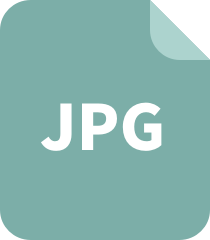
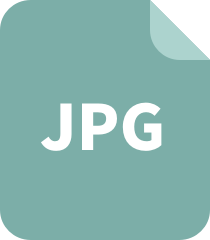
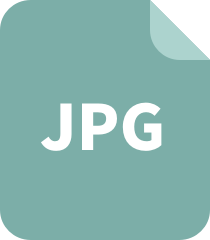
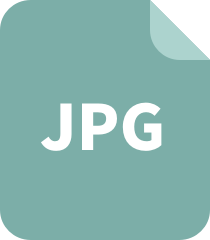
共 125 条
- 1
- 2
资源评论
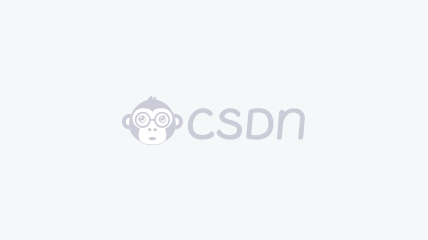
- shenjunstar2012-11-01是用SSH2做的哦,后面下载的人请注意,还可以的。
- qq1574028852012-11-07Struts、Hibernate、Spring都涉及到一些
- jisuanjixilaji2016-11-22到现在还在用,挺有帮助

wushiming
- 粉丝: 0
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

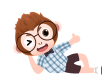
最新资源
- 实现了7种排序算法.三种复杂度排序.三种nlogn复杂度排序(堆排序,归并排序,快速排序)一种线性复杂度的排序.zip
- 冒泡排序 直接选择排序 直接插入排序 随机快速排序 归并排序 堆排序.zip
- 课设-内部排序算法比较 包括冒泡排序、直接插入排序、简单选择排序、快速排序、希尔排序、归并排序和堆排序.zip
- Python排序算法.zip
- C语言实现直接插入排序、希尔排序、选择排序、冒泡排序、堆排序、快速排序、归并排序、计数排序,并带图详解.zip
- 常用工具集参考用于图像等数据处理
- 音乐展示网页、基于Stenography的图像数字水印添加与提取,以及基于颜色矩和Tamura算法的图像相似度评估算法py源码
- 基于EmguCV(OpenCV .net封装),图像数字水印加解密算法的实现,其中包含最低有效位算法,离散傅里叶变换算法+文档书
- 基于matlab+DWT的图像水印项目,数字水印+源代码+文档说明+图片+报告pdf
- (优秀毕业设计)基于python实现的数字图像可视化水印系统的设计与实现,多种数字算法实现+源代码+文档说明+理论演示pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


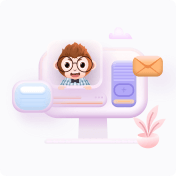
安全验证
文档复制为VIP权益,开通VIP直接复制
