import sys
import sqlite3
from PyQt5.QtCore import QCoreApplication,Qt, QRegExp, pyqtSlot, pyqtSignal, QDate
from PyQt5.QtWidgets import QApplication, QWidget, QMessageBox, QButtonGroup
from PyQt5.QtGui import QRegExpValidator
import prettytable as pt
from form_stu import *
from form_stu_info import *
import area
import basics as ba
class Form_StuInfo( QWidget, Ui_FormStuInfo):
def __init__(self):
super().__init__()
self.setupUi(self)
self.dateEdit_sbirth.setDate( QDate.currentDate())
self.all_validator()
self.choose_gender()
self.choose_native()
def all_validator(self):
regx1 = QRegExp("^[0-9]{8}$")
validator1 = QRegExpValidator(regx1, self.lineEdit_classno)
self.lineEdit_classno.setValidator(validator1)
regx2 = QRegExp("^[0-9]{10}$")
validator2 = QRegExpValidator(regx2, self.lineEdit_sno)
self.lineEdit_sno.setValidator(validator2)
def choose_gender(self):
"""选择性别"""
self.comboBox_sgender.clear()
self.dictSgender = {2: "男", 3: "女"}
for k, v in self.dictSgender.items():
self.comboBox_sgender.addItem(v, k)
def choose_native(self):
"""选择居住地"""
self.dictProvince = area.dictProvince
self.dictCity = area.dictCity
self.dictTown = area.dicTown
self.comboBox_province.clear() # 清空items
self.comboBox_province.addItem('请选择')
# 初始化省
for k, v in self.dictProvince.items():
self.comboBox_province.addItem(v, k) # 键、值反转
@pyqtSlot(int)
def on_comboBox_province_activated(self, index):
# 取省份名称
# value = self.comboBox_province.itemText(index)
# 取省份的键值
key = self.comboBox_province.itemData(index)
self.comboBox_town.clear() # 清空items
self.comboBox_city.clear() # 清空items
if key:
# self.lblResult.setText('未选择!')
self.comboBox_city.addItem('请选择')
# 初始化市
for k, v in self.dictCity[key].items():
self.comboBox_city.addItem(v, k) # 键、值反转
@pyqtSlot(int)
def on_comboBox_city_activated(self, index):
# 取市的名称
# value = self.comboBox_city.itemText(index)
# 取市的键值
key = self.comboBox_city.itemData(index)
self.comboBox_town.clear() # 清空items
if key:
self.comboBox_town.addItem('请选择')
# 初始化县区
for k, v in self.dictTown[key].items():
self.comboBox_town.addItem(v, k) # 键、值反转
def getsnative(self):
province_index = self.comboBox_province.currentIndex() #返回索引
city_index = self.comboBox_city.currentIndex()
province_name = self.comboBox_province.itemText(province_index) #通过索引查询文字
city_name = self.comboBox_city.itemText(city_index)
town_index = self.comboBox_town.currentIndex()
town_name = self.comboBox_town.itemText(town_index)
if town_name == "请选择":
snative = province_name + "省" + city_name + "市"
else:
snative = province_name + "省" + city_name + "市" + town_name
return snative
def confirm_sno(self):
"""确认学号是否为正确格式"""
self.sno_count = 1
sno_str = None
sno = self.lineEdit_sno.text()
if len(sno) == 0:
sno_str = "学号为空"
elif len(sno) < 10:
sno_str = "学号的长度低于10位"
elif not sno.isdigit():
sno_str = "学号不能包含字母"
if sno_str != None:
QMessageBox.warning(self, "警告", sno_str)
self.sno_count = 0
def confirm_classno(self):
"""确认班级是否为正确格式"""
self.classno_count = 1
classno_str = None
classno = self.lineEdit_classno.text()
if len(classno) == 0:
classno_str = "班级信息为空"
elif len(classno) < 8:
classno_str = "班号的长度低于8位"
elif not classno.isdigit():
classno_str = "班号不能包含字母"
if classno_str != None:
QMessageBox.warning(self, "警告", classno_str)
self.classno_count = 0
def confirm_sname(self):
"""确认姓名是否为正确格式"""
self.sname_count = 1
sname_str = None
sname = self.lineEdit_sname.text()
if len(sname) == 0:
sname_str = "姓名为空"
if sname_str != None:
QMessageBox.warning(self, "警告", sname_str)
self.sname_count = 0
def final_confirm(self):
""" 确保所有的参数都有数据"""
save_count = 0
self.confirm_sno()
if self.sno_count:
self.confirm_classno()
if self.classno_count:
self.confirm_sname()
if self.sname_count:
province_index = self.comboBox_province.currentIndex()
city_index = self.comboBox_city.currentIndex()
province_name = self.comboBox_province.itemText(province_index)
city_name = self.comboBox_city.itemText(city_index)
if province_name == "请选择" or city_name == "请选择":
QMessageBox.warning(self, "警告",
"居住地信息必须需要选择省份和城市")
else:
save_count = 1
#self.sinOut.emit(save_count)
return save_count
def clear_lineEdit(self):
"""重置清空的方法"""
self.lineEdit_sno.setText("")
self.lineEdit_classno.setText("")
self.lineEdit_sname.setText("")
self.comboBox_sgender.setCurrentIndex(0) # 用于设置当前的索引
self.comboBox_town.setCurrentIndex(-1) #- 1表示未设置当前项目的空组合框或组合框
self.comboBox_city.setCurrentIndex(-1)
#self.dateEdit_sbirth.setDateTime( QDateTime.currentDateTime())
self.dateEdit_sbirth.setDate( QDate.currentDate())
self.comboBox_province.setCurrentIndex(0) # 0是“请选择”
class StuPage(QWidget, Ui_FormStu):
def __init__( self, cn):
super().__init__()
self.setupUi(self)
self.cn = cn
# print( self.cn)
# 一样类型的框架,同样需要检查,区别在于最后是添加还是修改
self.form_stuinfo1 = Form_StuInfo()
self.verticalLayout_stuadd_1.addWidget( self.form_stuinfo1)
self.form_stuinfo2 = Form_StuInfo()
self.verticalLayout_stumod2_1.addWidget( self.form_stuinfo2)
self.all_validator()
self.buttons_group()
self.buttons_click()
def all_validator(self):
regx = QRegExp("^[0-9]{10}$")
validator1 = QRegExpValidator(regx, self.lineEdit_stumod_sno)
self.lineEdit_stumod_sno.setValidator(validator1)
validator2 = QRegExpValidator(regx, self.lineEdit_studel_sno)
self.lineEdit_studel_sno.setValidator(validator2)
def buttons_group(self):
self.stuquery_buttongroup = QButtonGroup()
self.stuquery_buttongroup.addButton( self.Button_stuquery_sno, 0)
self.stuquery_buttongroup.addButton( self.Button_stuquery_sname, 1)
self.stuquery_buttongroup.addButton( self.Button_stuquery_sgender, 2)
self.stuquery_buttongroup.addButton( self.Button_stuquery_classno, 3)
self.stuquery_buttongroup.addButton( self.Button_stuquery_snative, 4)
self.stusort_buttong
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
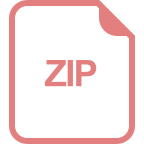
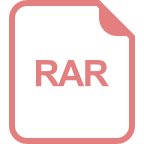
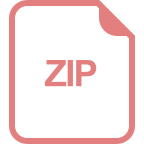
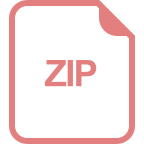
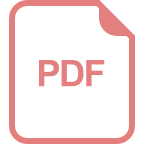
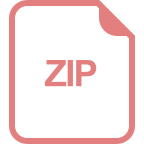
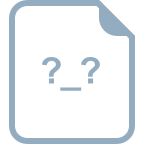
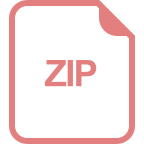
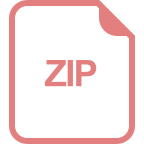
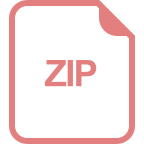
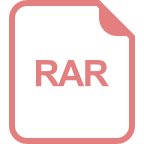
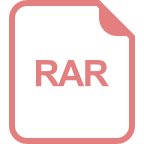
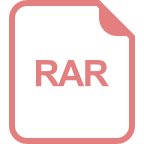
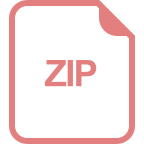
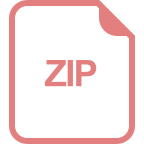
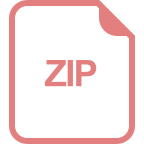
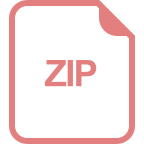
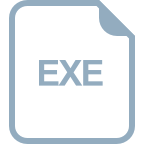
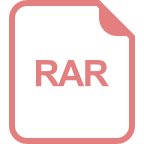
收起资源包目录


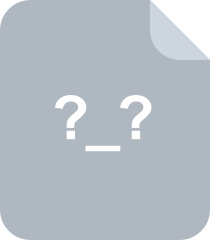
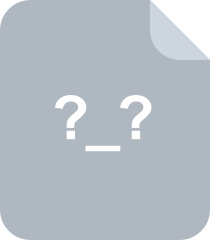
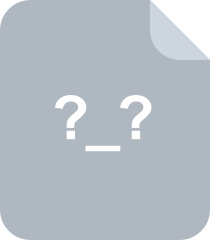
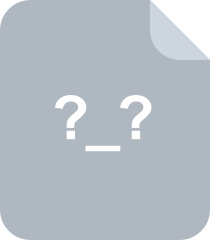
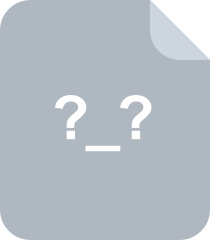
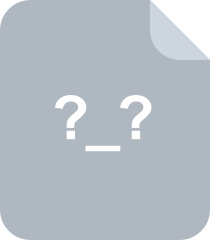
共 6 条
- 1
资源评论
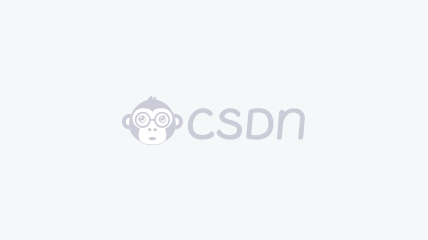
- Mo嘻哈2022-04-04没什么用,就是qt的注册登录ui界面转的.py代码

wujinxia
- 粉丝: 52
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

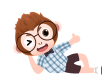
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


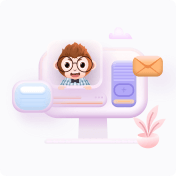
安全验证
文档复制为VIP权益,开通VIP直接复制
