Java的webservice入门(视频+代码)
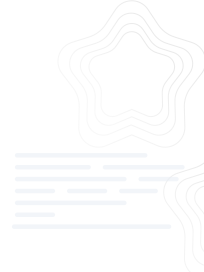


Java的Web服务(Web Service)是一种基于开放标准的、跨平台的数据交换技术,它允许不同系统之间通过网络进行通信和数据共享。本资源是针对Java的Web Service入门的学习材料,包含视频教程和配套代码,非常适合初学者入门学习。 在Java中实现Web Service,主要依赖于两种标准:SOAP(Simple Object Access Protocol)和WSDL(Web Services Description Language)。SOAP是一种基于XML的协议,用于在Web上交换结构化和类型化的信息;而WSDL则是一种XML格式的规范,用来描述Web Service接口及其绑定,使得服务消费者能够找到并调用服务。 1. **SOAP**:SOAP消息通常以HTTP作为传输协议,其消息结构包含头部(Header)、主体(Body),并且都是XML格式。SOAP允许开发者传递复杂的数据结构,并提供了错误处理机制。 2. **WSDL**:WSDL文档定义了Web Service的接口,包括服务提供的操作、消息格式、输入/输出参数、地址等信息。服务消费者通过读取WSDL可以知道如何与服务进行交互。 在Java中,有几种常见的Web Service实现框架: 1. **JAX-WS (Java API for XML Web Services)**:这是Java SE和EE平台的标准API,用于创建SOAP Web Service。JAX-WS通过注解(如`@WebService`)和XML配置文件(如wsdl)来定义服务接口和服务实现。 2. **JAX-RS (Java API for RESTful Web Services)**:RESTful Web Service是另一种常见的Web Service风格,它基于HTTP协议,使用URI(Uniform Resource Identifier)来定位资源,使用HTTP方法(GET、POST、PUT、DELETE等)来操作资源。JAX-RS简化了创建RESTful服务的过程,通过注解如`@Path`, `@GET`, `@POST`等即可定义服务。 3. **Apache CXF**:这是一个开源框架,支持JAX-WS和JAX-RS,提供丰富的工具和API,方便开发和调试Web Service。 4. **Spring Web Services**:Spring框架的一部分,专注于创建SOAP Web Service。它支持基于XML和基于契约优先的开发模式,也可以与Spring Boot结合使用。 本资源的"Java的webservice入门"视频教程很可能涵盖了以下内容: - Web Service的基本概念和工作原理 - 如何创建和发布一个简单的SOAP或RESTful Web Service - 使用JAX-WS或JAX-RS编写服务端代码 - 客户端如何调用Web Service - WSDL的生成和理解 - 测试工具(如SoapUI)的使用 配套代码可能包含了示例服务和客户端的实现,帮助初学者动手实践,加深理解。通过视频和代码的结合学习,你将能够掌握Java Web Service的基本开发流程,为进一步深入学习和应用打下坚实基础。在学习过程中,记得理论与实践相结合,不断尝试、调试和优化,以提升自己的技能。
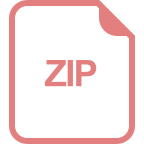
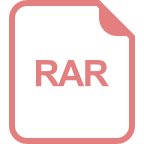
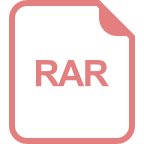
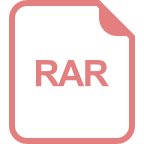
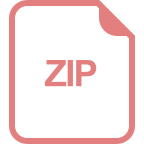
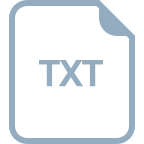
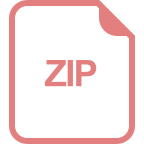
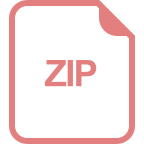
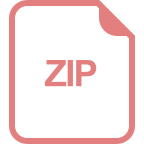
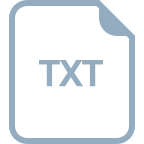
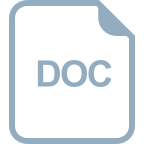
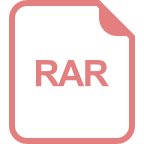
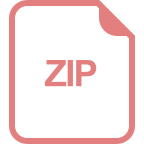
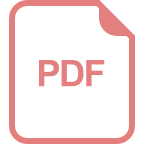
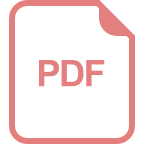
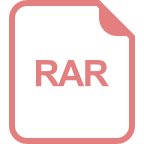
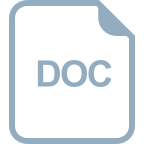
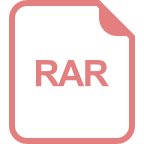
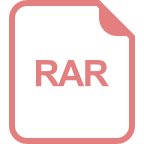
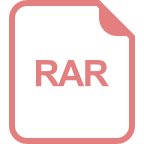


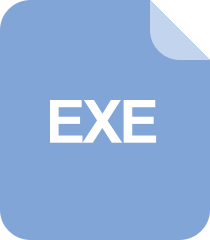

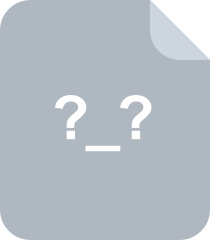

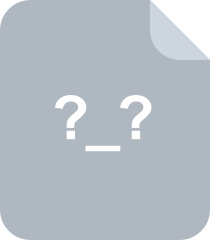
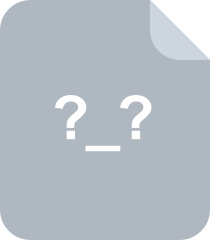


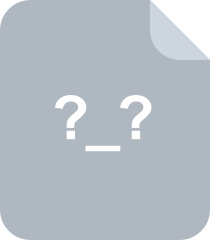
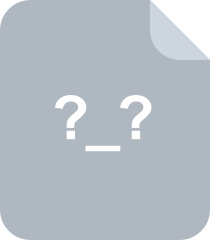
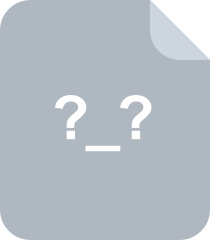
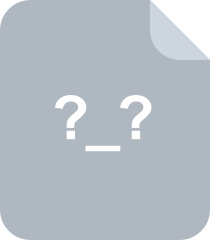
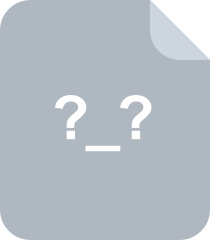
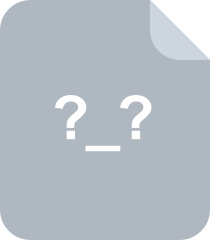
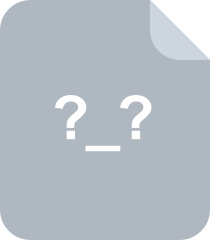
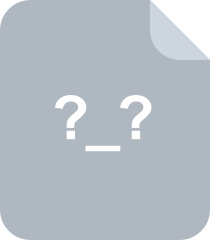
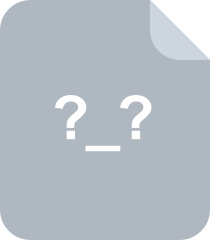

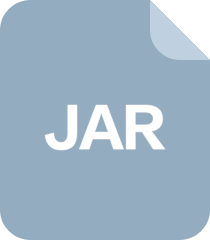
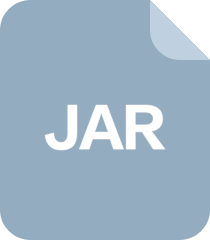
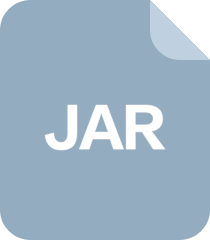
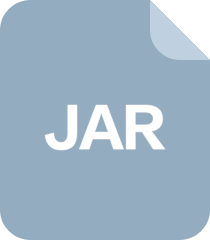
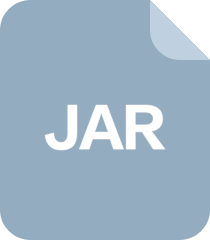
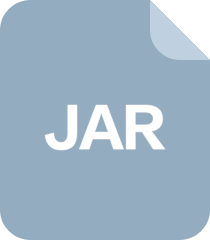
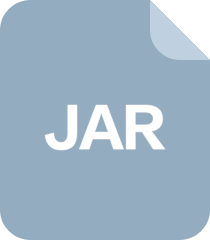
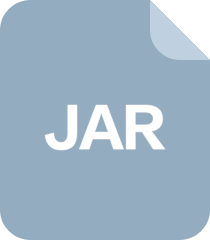
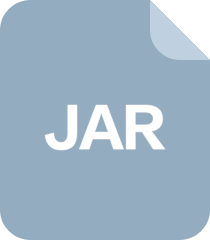




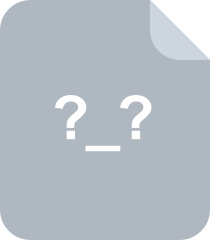
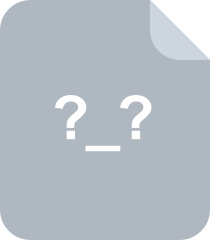


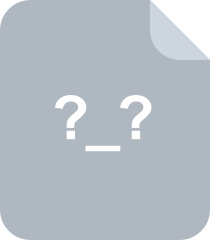
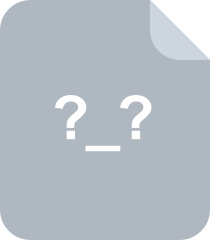
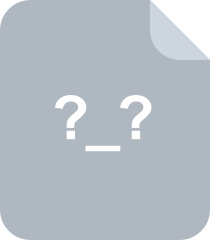
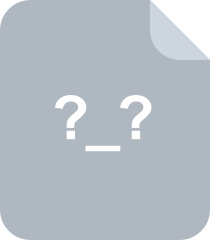
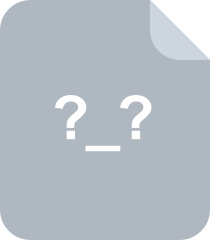
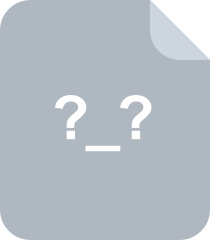
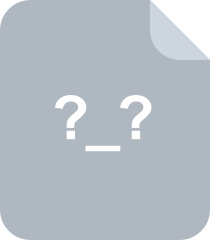

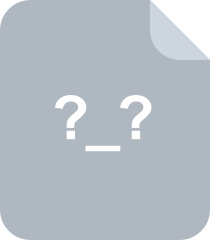





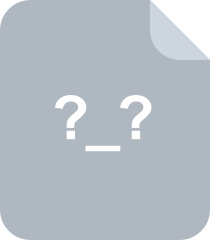
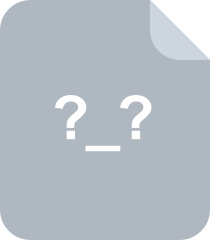


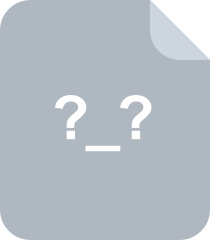
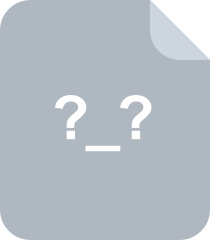
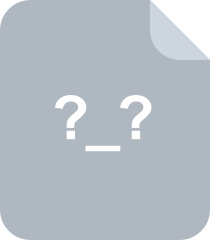
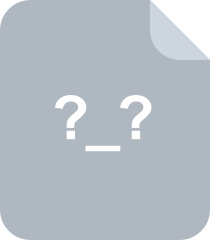
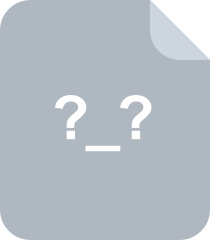
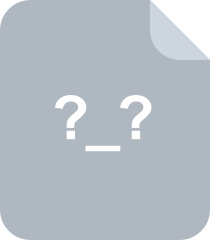
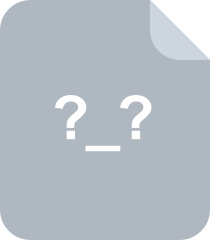
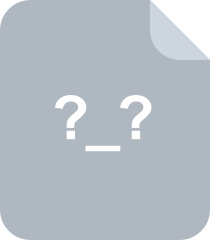
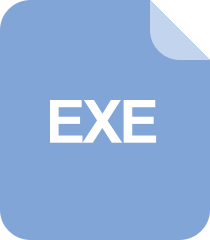

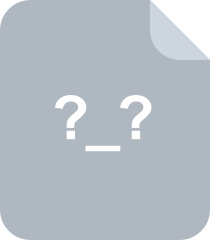

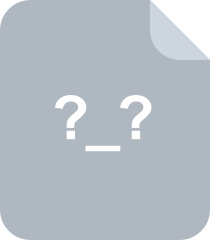
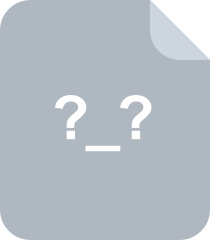
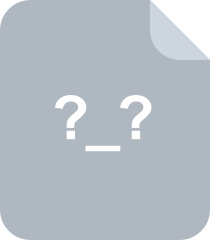


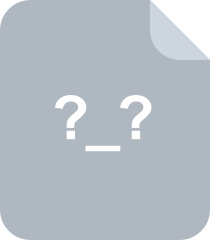




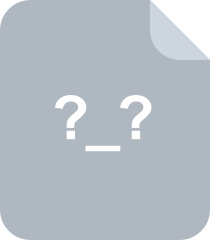



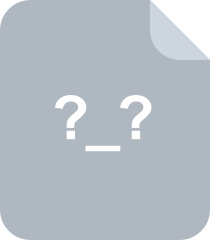
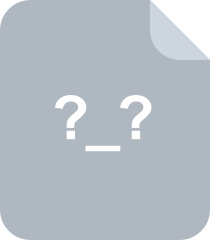
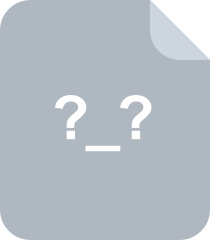

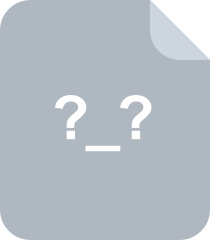





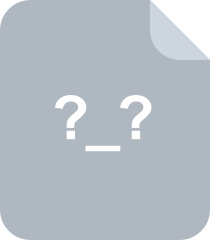
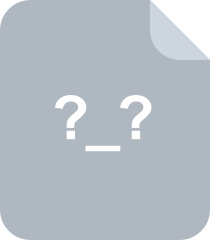

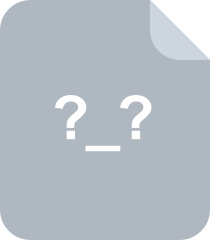
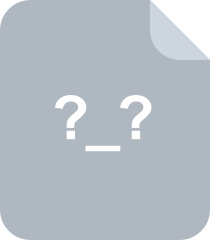
- 1

- 粉丝: 0
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

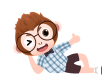
最新资源
- AllSort(直接插入排序,希尔排序,选择排序,堆排序,冒泡排序,快速排序,归并排序)
- 模拟qsort,改造冒泡排序使其能排序任意数据类型,即日常练习
- 数组经典习题之顺序排序和二分查找和冒泡排序
- 基于 Oops Framework 提供的游戏项目开发模板,项目中提供了最新版本 Cocos Creator 3.x 插件与游戏资源初始化通用逻辑
- live-ai这是一个深度学习的资料
- FeiQ.rar 局域网内通信服务软件
- 172.16.100.195
- 光储并网simulink仿真模型,直流微电网 光伏系统采用扰动观察法是实现mppt控制,储能可由单独蓄电池构成,也可由蓄电池和超级电容构成的混合储能系统,并采用lpf进行功率分配 并网采用pq控制
- python编写微信读取smart200plc的数据发送给微信联系人
- 光储并网VSG系统Matlab simulink仿真模型,附参考文献 系统前级直流部分包括光伏阵列、变器、储能系统和双向dcdc变器,后级交流子系统包括逆变器LC滤波器,交流负载 光储并网VSG系

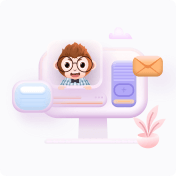

- 1
- 2
- 3
- 4
- 5
- 6
前往页