const app = getApp()
const db = wx.cloud.database()
const dbQuestions = db.collection('tiku_exams')
const util = require("../../utils/util");
const _ = db.command
let titles = [] //题库
Page({
data: {
hour:'00',
minute:'00',
second:'00',
showScore:false,//是否展示成绩
testTime:0,//考试用时
errorOptions:[],
showAnswer:false,
percent: 0,
total: 0,
isSelect: false,
subject: null,
userSelect: '',
userScore: 0, //用户答对了几道题
totalScore: 0, //用户总得分
totalError: 0, //用户答错几道题
current: 1 //从第一道题开始
},
onUnload(){
console.log('执行了')
titles = []
},
getQuestionsList(type1,type2){
wx.setNavigationBarTitle({
title: type2 + '答题',
})
dbQuestions.where({
type: type1,
type2: type2
}).skip(titles.length).get()
.then(res => {
console.log("题库", res)
if(res.data.length!=0){
titles = titles.concat(res.data)
this.getQuestionsList(type1,type2)
}else{
let subject = titles[0]
console.log('subject', subject)
this.setData({
subject,
total: titles.length
})
//生成考试id
this.setData({
testId: util.formatTime(new Date()) + this.data.subject.type + '考试'
})
}
})
},
//一进入页面就会执行的生命周期
onLoad(e) {
//开始计时
this.time60()
console.log('答题页', e)
if (e.type1 && e.type2) { //按类型答题
this.getQuestionsList(e.type1,e.type2)
} else { //随机题库
wx.setNavigationBarTitle({
title: '随机答题',
})
dbQuestions.aggregate()
.sample({
size: app.globalData.randomNum //随机获取几道题,比如这里随机返回5道题
})
.end()
.then(res => {
console.log("随机题库", res)
titles = res.list
let subject = titles[0]
this.setData({
subject,
total: titles.length
})
//生成考试id
this.setData({
testId: util.formatTime(new Date()) + this.data.subject.type + '考试'
})
})
}
// 答题时提示用需要登陆注册才可以参加排名
// 发布之前先判断是否登录和注册
if (!app.globalData.userInfo || !app.globalData.userInfo.name) {
wx.showModal({
title: "需要参加积分排名吗?",
content: '只有授权登陆并注册用户后才可以参与积分排名,取消后本次答题不计入积分排行里',
success: res => {
if (res.confirm) {
wx.switchTab({
url: '/pages/me/me',
})
}
}
})
}
},
//用户选择
selectClick(e) {
console.log(e.detail.value)
this.setData({
userSelect: e.detail.value
})
},
//提交答题,并切换到下一题
submit() {
this.setData({
showAnswer:false
})
//1,获取用户选项并判空
let userSelect = this.data.userSelect
if (!userSelect || userSelect.length < 1) {
wx.showToast({
icon: 'none',
title: '请做选择',
})
return
}
//2,如果用户有选择,就更新进度条
let currentNum = this.data.current
//更新进度条
this.setData({
percent: (currentNum / titles.length * 100).toFixed(1)
})
//3,判断用户是否答对
console.log('用户选项', userSelect)
console.log('正确答案', this.data.subject.answer)
if (userSelect instanceof Array) { //多选的时候,把选项转字符串
console.log('是数组')
userSelect = userSelect.sort().toString()
}
if (this.data.subject.answer.sort().toString() == userSelect) {
console.log('用户答对了第' + currentNum + "道题")
this.setData({
userScore: this.data.userScore + 1
})
} else {
//4,记录用户答错的题,方便用户查漏补缺
let subjectNow = this.data.subject
subjectNow.userSelect = userSelect
this.data.errorOptions.push(subjectNow)
let temp = {}
Object.assign(temp, subjectNow)
delete temp._id
let userInfo = wx.getStorageSync('user') || {}
temp.nickName = userInfo && userInfo.nickName ? userInfo.nickName : '未登陆用户'
console.log('临时错题', temp)
//设置考试id
temp.testId = this.data.testId
// 添加用户错题到数据库
db.collection('tiku_test_errors').add({
data: temp
}).then(res => {
console.log('添加错题到数据库', res)
})
console.log('错题', subjectNow)
}
// 5,在答完最后一道题的时候,对用户进行打分
if (currentNum + 1 > titles.length) {
let totalScore = this.data.userScore
console.log('用户一共答对了' + totalScore + "道题")
console.log('用户错题集', this.data.errorOptions)
this.setData({
totalScore: totalScore,
totalError: this.data.errorOptions.length,
hideButton: true //最后一题时隐藏按钮
})
wx.showToast({
icon: 'none',
title: '已经最后一道啦',
})
// this.addScore(totalScore)
return
}
let subject = titles[currentNum]
this.setData({
userSelect: '',
subject,
current: currentNum + 1,
isSelect: false,
})
},
//去查看错题集
seeError() {
console.log('点击了查看错题集')
//跳页
wx.switchTab({
url: '/pages/errorList/errorList'
})
},
//添加积分
addScore(score) {
// 发布之前先判断是否登录和注册,如果没有就不计分
if (!app.globalData.userInfo || !app.globalData.userInfo.name) {
return
}
db.collection('tiku_users').doc(app.globalData.openid).update({
data: {
score: _.inc(score)
}
}).then(res => {
wx.showToast({
title: '积分生效',
})
})
},
//查看答案
ok(){
//1,获取用户选项并判空
let userSelect = this.data.userSelect
if (!userSelect || userSelect.length < 1) {
wx.showToast({
icon: 'none',
title: '请做选择',
})
return
}
this.setData({
showAnswer:true
})
},
//提交考试
testEnd(){
//1,获取用户选项并判空
let userSelect = this.data.userSelect
if (!userSelect || userSelect.length < 1) {
wx.showToast({
icon: 'none',
title: '请做选择',
})
return
}
wx.showLoading({
title: '提交中',
mask:true
})
this.setData({
showScore: true, //最后一题时隐藏按钮
testTimeMin: this.data.testTimeMin,
testTimeSec: this.data.testTimeSec,
})
//添加积分
this.addScore(this.data.totalScore)
//停止倒计时
clearInterval(this.data.timeInterval)
wx.cloud.database().collection('tiku_test_results').add({
data:{
faceImg:app.globalData.userInfo.avatarUrl,
nickName:app.globalData.userInfo.nickName,
testId:this.data.testId,
time:this.data.testTimeMin + '分' + this.data.testTimeSec + '秒',
totalError:this.data.totalError,//答错xx道
total:this.data.total//答错xx道
}
}).then(res=>{
wx.hideLoading({
success: (res) => {
wx.showToast({
title: '提交成功',
})
wx.redirectTo({
url: '/pages/test/testResult/testResult?totalError=' + this.data.totalError + '&testTimeMin=' + this.data.testTimeMin + '&testTimeSec=' + this.data.testTimeSec + '&totalScore=' + this.data.totalScore,
})
},
})
})
},
//倒计时1小时
time60(){
var that
没有合适的资源?快使用搜索试试~ 我知道了~
微信在线答题小程序,基于云开发搭建
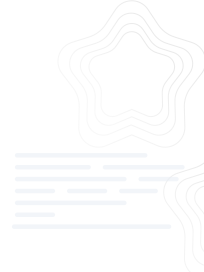
共275个文件
json:29个
js:25个
wxss:23个

需积分: 47 8 下载量 44 浏览量
2022-09-12
17:13:40
上传
评论 6
收藏 1.51MB RAR 举报
温馨提示
微信在线答题小程序,基于云开发搭建 const db = wx.cloud.database() const app = getApp() Page({ data: { }, //获取所有题目类型 onLoad() { this.getCurrentDate() this.getBannerList() const $ = db.command.aggregate db.collection('tiku_questions').aggregate() .group({ _id: '$type', num: $.sum(1) }).end().then(res => { console.log('题目类型', res) this.setData({ list: res.list }) }) }, getCurrentDate(){ var now = new Dat
资源详情
资源评论
资源推荐
收起资源包目录

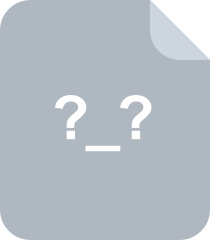
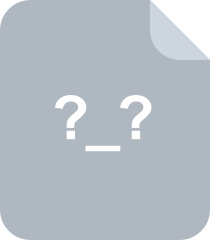
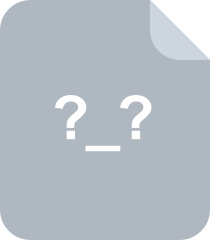
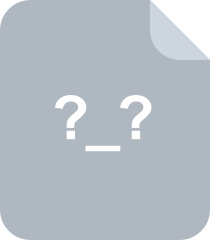
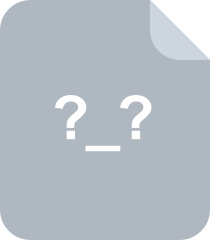
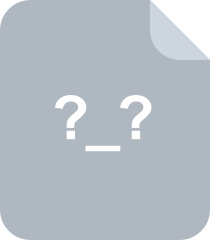
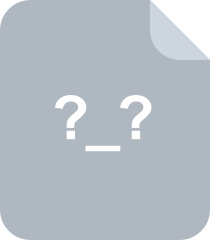
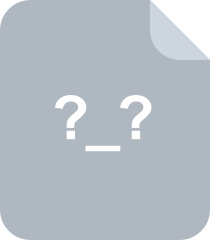
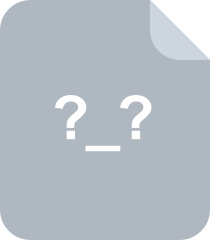
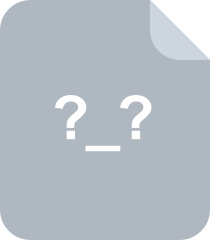
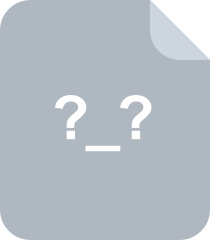
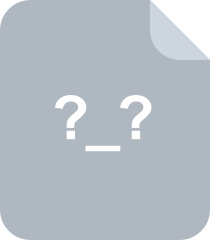
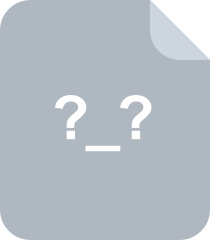
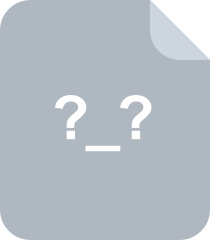
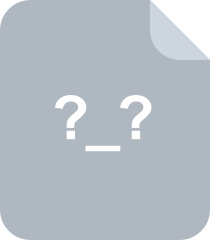
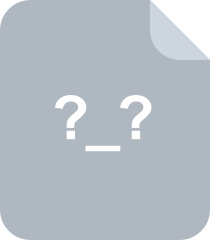
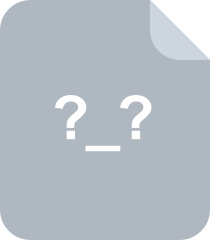
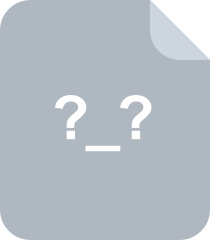
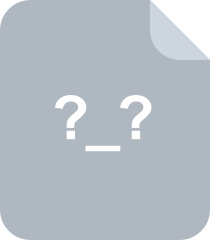
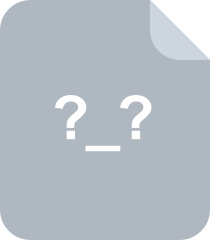
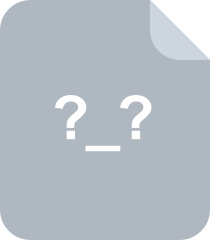
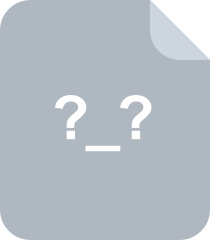
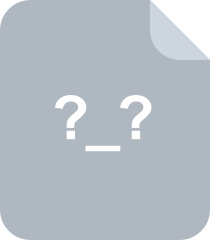
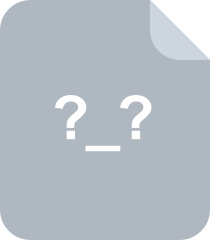
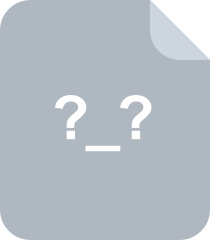
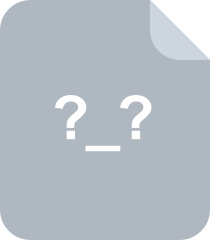
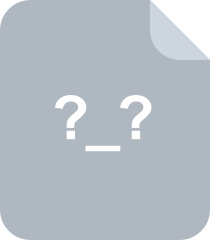
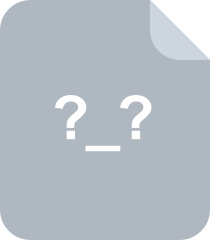
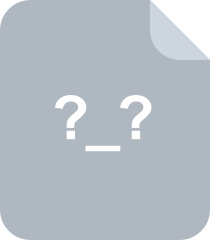
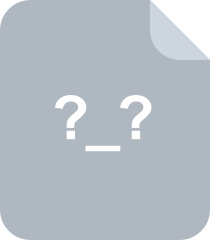
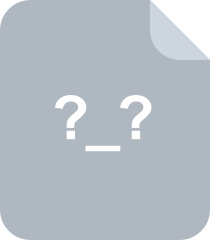
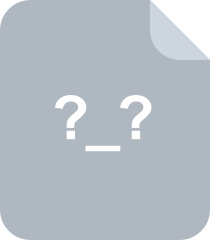
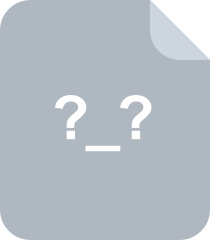
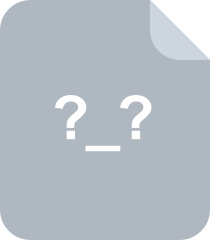
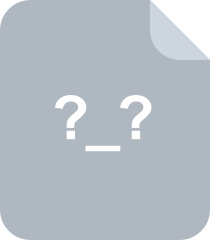
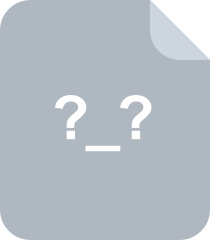
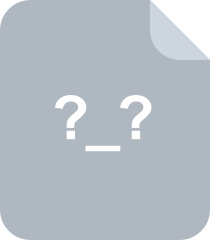
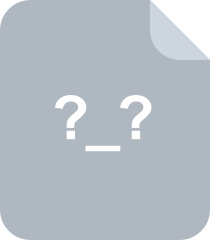
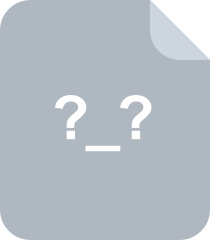
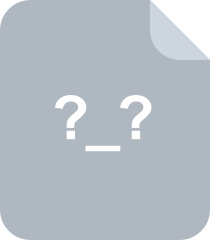
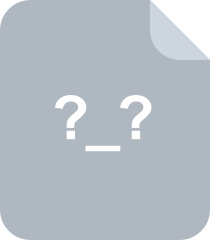
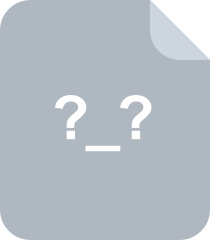
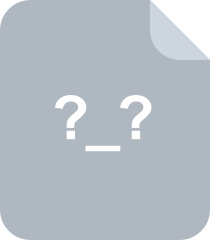
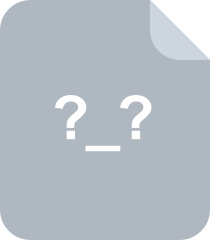
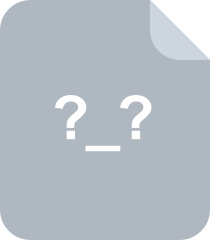
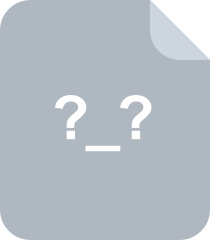
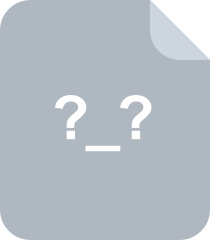
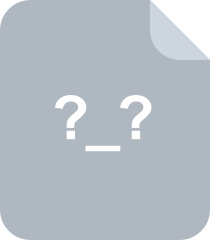
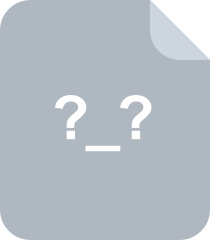
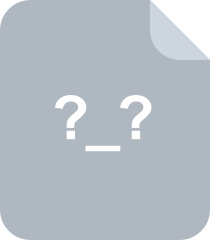
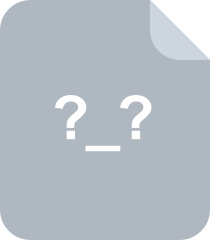
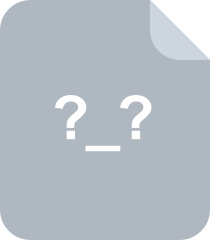
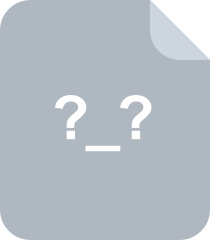
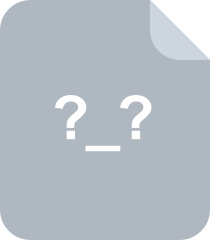
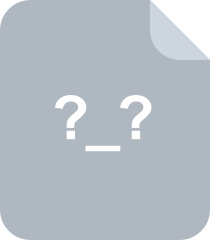
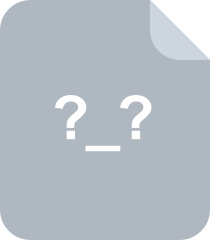
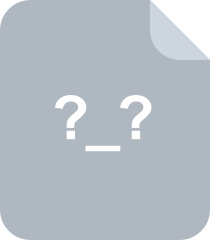
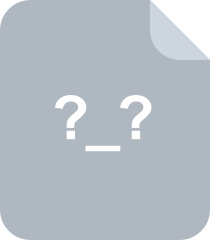
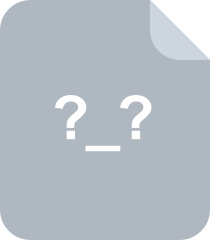
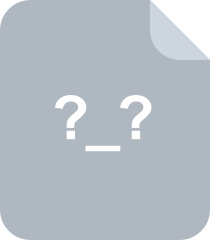
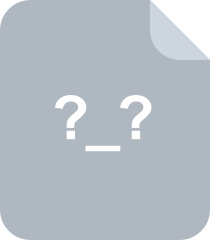
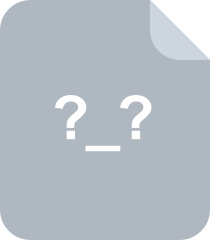
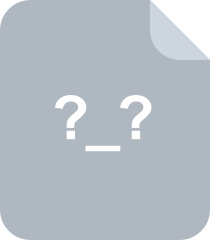
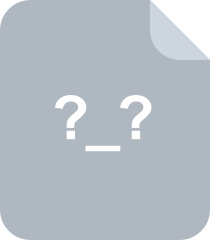
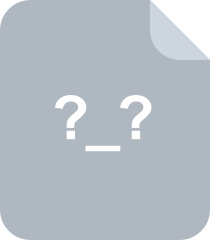
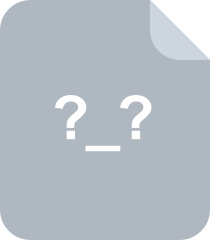
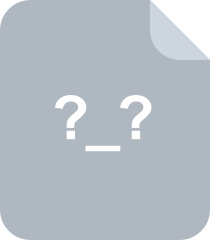
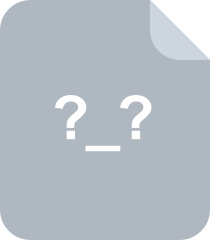
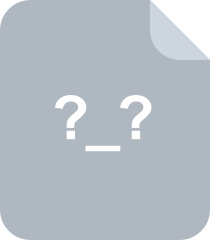
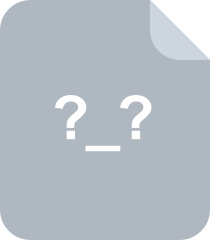
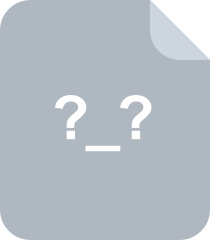
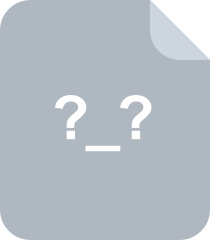
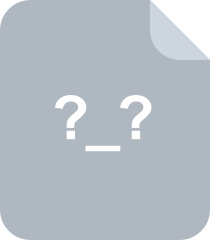
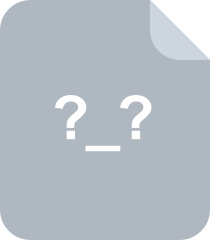
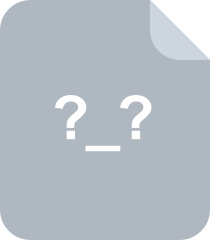
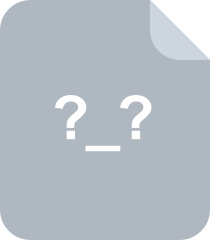
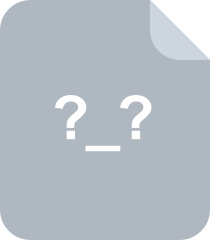
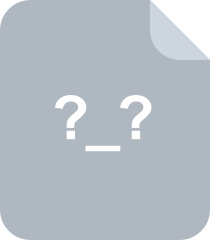
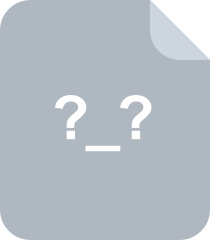
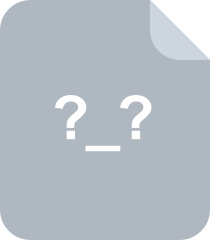
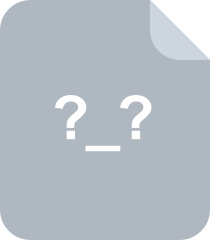
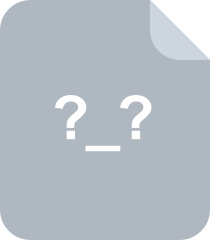
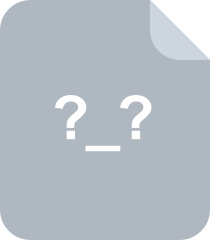
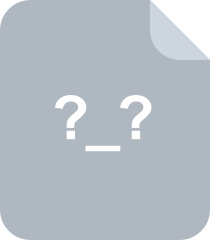
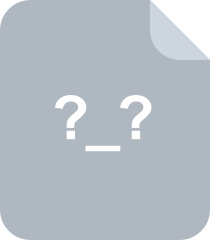
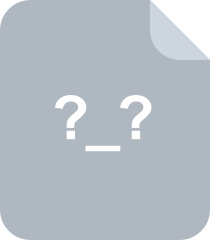
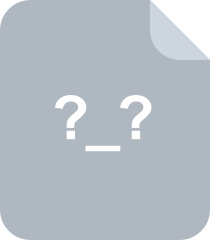
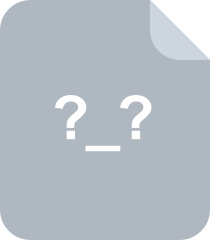
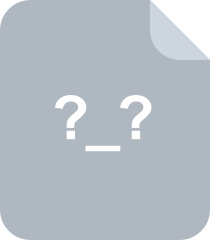
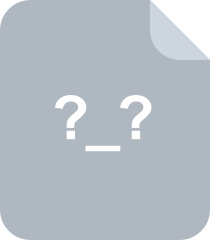
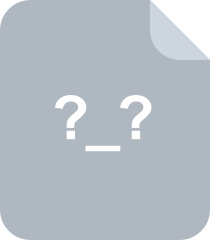
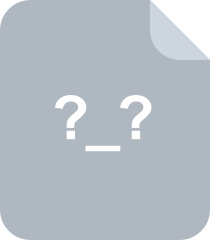
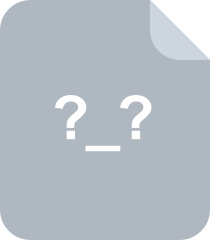
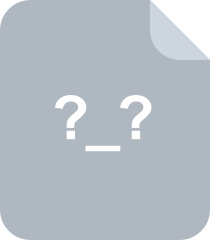
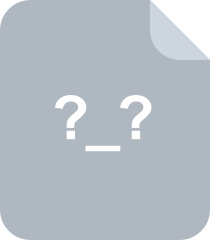
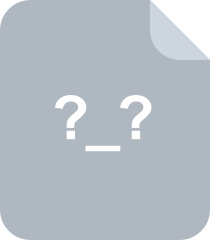
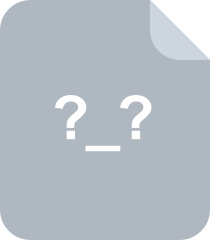
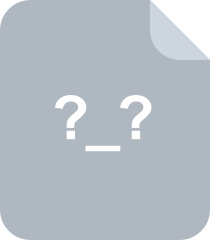
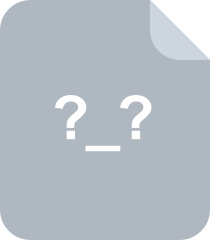
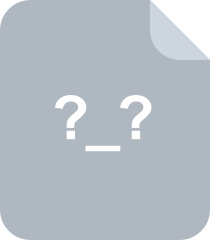
共 275 条
- 1
- 2
- 3
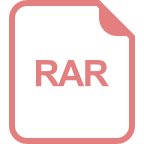
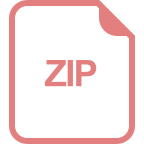
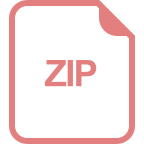
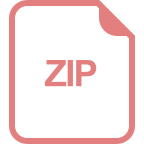
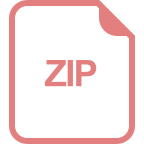
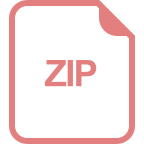
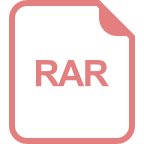
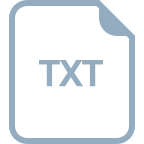
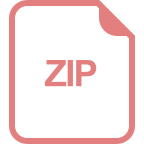
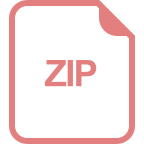
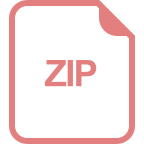
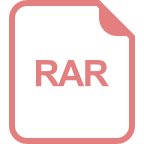
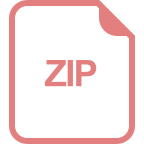
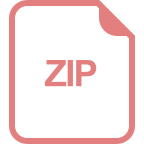
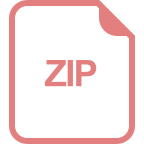
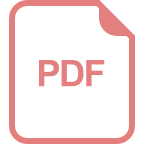
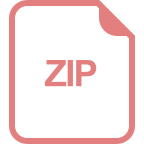
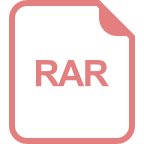

wujiequ
- 粉丝: 0
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

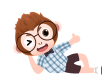
最新资源
- LabVIEW实现LoRa通信【LabVIEW物联网实战】
- CS-TY4-4WCN-转-公版-XP1-8B4WF-wifi8188
- 计算机网络期末复习资料(课后题答案+往年考试题+复习提纲+知识点总结)
- 从零学习自动驾驶Lattice规划算法(下) 轨迹采样 轨迹评估 碰撞检测 包含matlab代码实现和cpp代码实现,方便对照学习 cpp代码用vs2019编译 依赖qt5.15做可视化 更新:
- 风光储、风光储并网直流微电网simulink仿真模型 系统由光伏发电系统、风力发电系统、混合储能系统(可单独储能系统)、逆变器VSR+大电网构成 光伏系统采用扰动观察法实现mppt控
- (180014016)pycairo-1.18.2-cp35-cp35m-win32.whl.rar
- (180014046)pycairo-1.21.0-cp311-cp311-win32.whl.rar
- DS-7808-HS-HF / DS-7808-HW-E1
- (180014004)pycairo-1.20.0-cp36-cp36m-win32.whl.rar
- (178330212)基于Springboot+VUE的校园图书管理系统
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


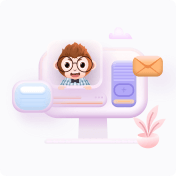
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0