// stlTest.cpp : 定义控制台应用程序的入口点。
///////////////////////////////////////////////////////////////
//采用 STL中的list管理学生的信息
//对学生信息的操作用控制台输入
//并控制 find find_if insert erase sort count count_if操作
//考虑采用for_each循环遍历学生的信息
//
//作者:张浩
//日期:2008年4月29日
////////////////////////////////////////////////////////////////
//#include "stdafx.h"
#include <iostream>
#include <list>
#include <algorithm>
#include <math.h>
using namespace std;
//Student结构,存放学生信息
struct Student
{
int id;//学号
float score;//成绩
};
bool operator ==(const Student &stu1,const Student &stu2)
{
return (abs(stu1.score-stu2.score))<0.001;
}
bool operator >(const Student &stu1,const Student &stu2)
{
return (stu1.score > stu2.score);
}
bool operator <(const Student &stu1 ,const Student &stu2)
{
return (stu1.score < stu2.score);
}
Student myStu;//定义一个全局变量
////////////////////////
//输出学生信息
void PrintInfo(Student& stu)
{
cout << stu.id << " " << stu.score<< endl;
}
/////////////////////////
//查找学生
bool FindStu(Student &stu)
{
return (stu.id == myStu.id);
}
///////////////////////
//统计满足条件的学生的个数
bool CountStuNum(Student &stu)
{
return stu.score >= 60.0;//返回成绩大于60的学生的个数
}
//////////////////////////////////////////
void main()
{
list<Student> stuList;//
list<Student>::iterator stuIter;//迭代器
int i = 0;//计数
int pos = 0;//结点位置
int num = 0;//统计满足某个条件的学生个数
cout << "输入学生的学号,成绩(学号为零结束):" << endl;
while(1)
{
cout<<"输入第: "<<num+1<<"个学生的学号:";
cin >> myStu.id ;
if(myStu.id == 0)
{
break;
}
cout<<"输入第: "<<num+1<<"个学生的成绩:";
cin>> myStu.score;
num++;
stuList.push_back(myStu);
}
cout<<"输入完毕!"<<endl;
cout<<"(find测试)输入要查询学生的成绩:";
cin>>myStu.score;
stuIter = find(stuList.begin(), stuList.end(), myStu);//查找满足条件的第一个结点
if(abs((*stuIter).score-myStu.score)<0.01)
{
cout<< "第一个成绩为: " << myStu.score << " 的学生的信息如下:" << endl;
cout<< (*stuIter).id<< " " << (*stuIter).score << endl;
}
else
{
cout<<"不存在此学生!"<<endl;
}
cout<< "(find_if测试)输入要查询学生的学号:";
cin >> myStu.id;
stuIter = find_if(stuList.begin(), stuList.end(), FindStu);//查找满足条件的第一个结点
if((*stuIter).id==myStu.id)
{
cout << "学号为: " << myStu.id << " 的学生的信息如下:" << endl;
cout<< (*stuIter).id << " " << (*stuIter).score << endl;
}
else
{
cout<<"不存在此学生!"<<endl;
}
cout<< "(count测试)输入一个学生成绩(已经存在的)" << endl;
cin >> myStu.score;
num = count(stuList.begin(), stuList.end(), myStu);
cout << "成绩为: "<< myStu.score << "的学生的个数为;" << num << endl;
cout << "(count_if测试)计算及格人数:" << endl;
num = count_if(stuList.begin(), stuList.end(), CountStuNum);
cout << "及格人数为:" << num << endl;
cout << endl << "所有学生的信息如下:" << endl;
for_each(stuList.begin(), stuList.end(), PrintInfo);//用for_each遍历学生链
cout << "(insert测试)输入要插入的结点的位置以及学号 成绩;" << endl;
cin >> pos >> myStu.id >> myStu.score;//
stuIter = stuList.begin();
if(pos>=0)
{
for( i = 0;i < pos&& stuIter!=stuList.end(); i++)
{
stuIter++;//找到插入的位置
}
if(stuIter!=stuList.end())
{
stuList.insert(stuIter, myStu);//插入结点(一个学生的信息)*/
cout << endl << "插入后所有学生的信息如下:" << endl;
for_each(stuList.begin(), stuList.end(), PrintInfo);//用for_each遍历学生链
}
else
{
cout<<"输入有误!"<<endl;
}
}
else
{
cout<<"输入有误!"<<endl;
}
cout << "(删除测试)输入要删除的结点的位置:" << endl;
cin >> pos;
stuIter = stuList.begin();
for( i = 0;i < pos&& stuIter!=stuList.end(); i++)
{
stuIter++;//找到插入的位置
}
if(stuIter!=stuList.end())
{
stuList.erase(stuIter);//删除在位置pos的结点
cout << endl << "删除后所有学生的信息如下:" << endl;
for_each(stuList.begin(), stuList.end(), PrintInfo);//用for_each遍历学生链
}
else
{
cout<<"输入有误!"<<endl;
}
cout << "按成绩排序后的学生信息如下:" << endl;
stuList.sort();
for_each(stuList.begin(), stuList.end(),PrintInfo);//用for_each遍历学生链表
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源详情
资源评论
资源推荐
收起资源包目录

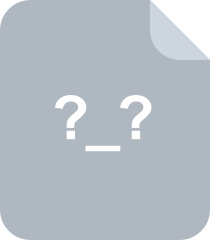
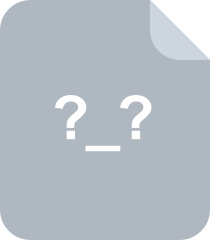
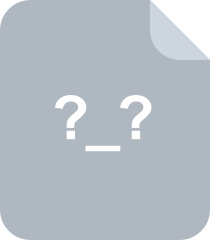
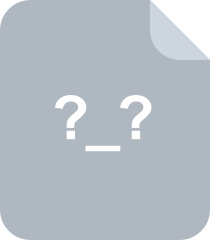
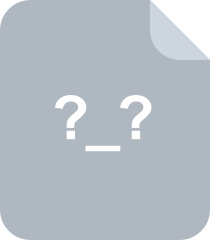
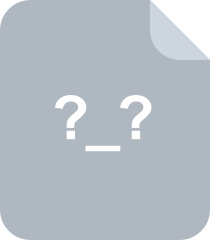
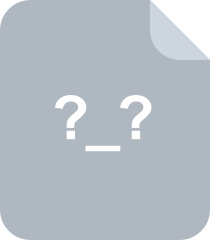
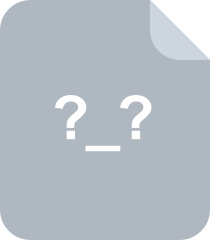
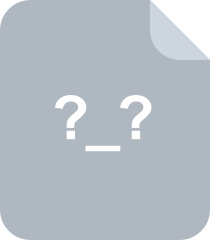
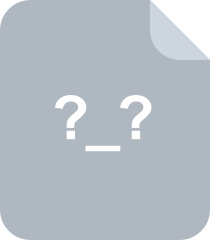
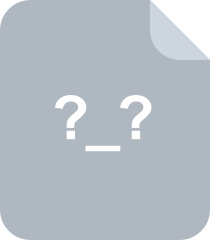
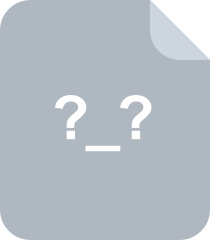
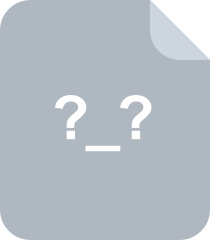
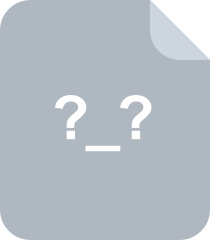
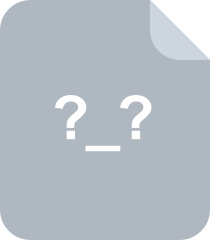
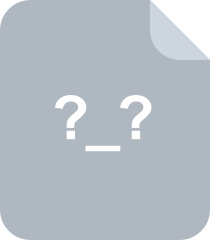
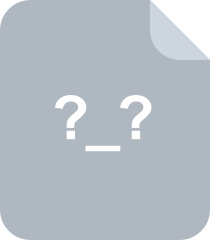
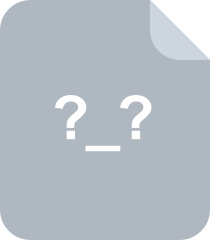
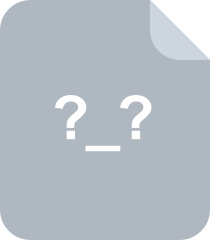
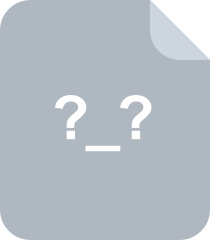
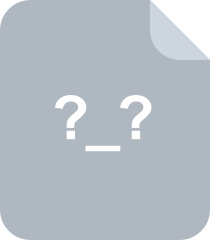
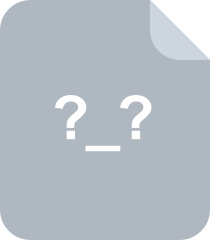
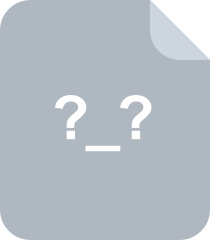
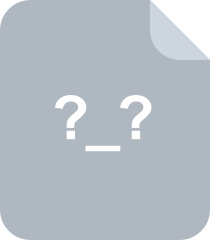
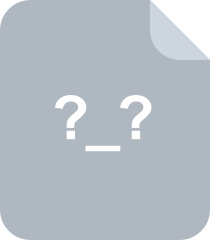
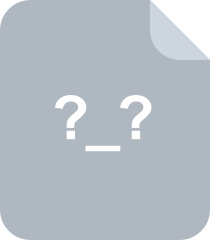
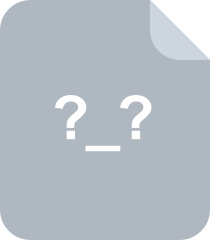
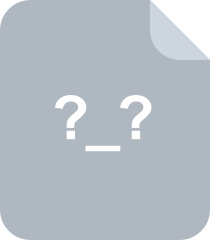
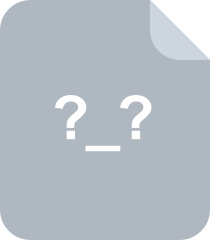
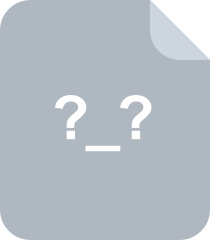
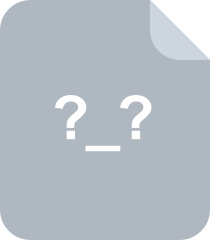
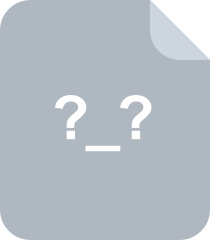
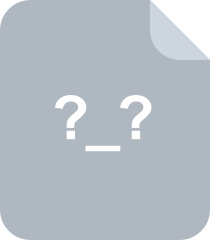
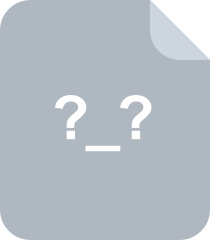
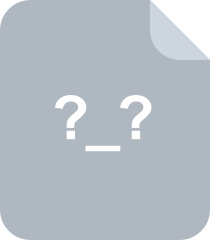
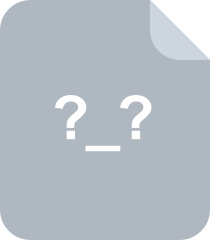
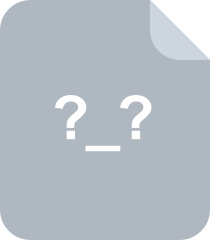
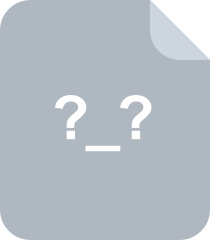
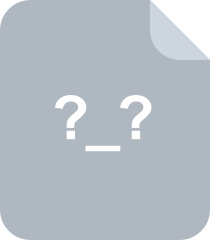
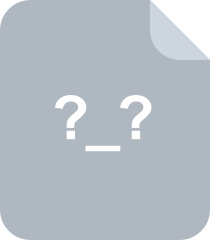
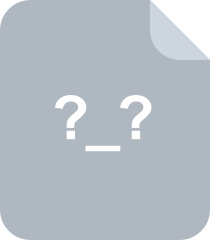
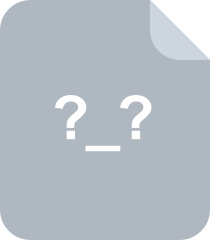
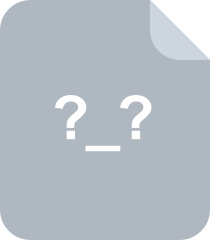
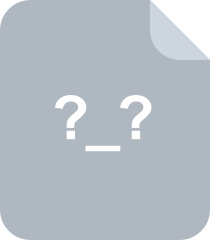
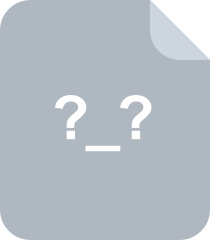
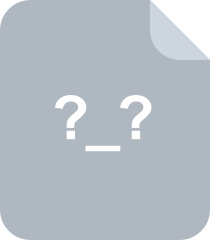
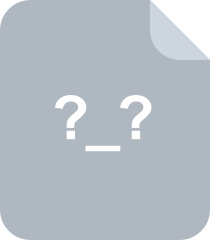
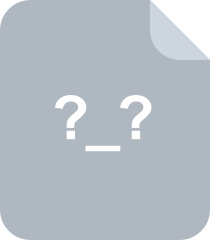
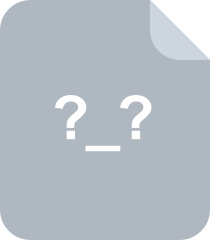
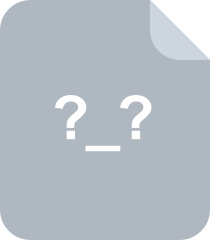
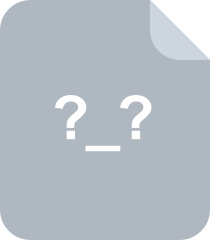
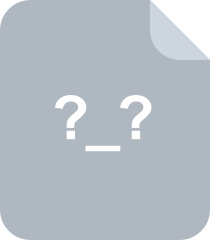
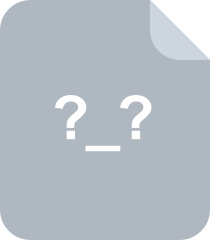
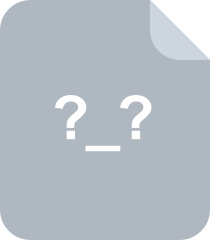
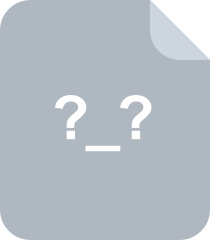
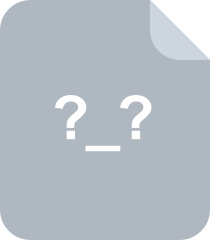
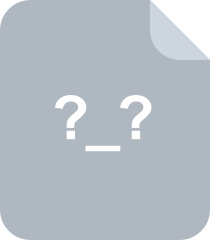
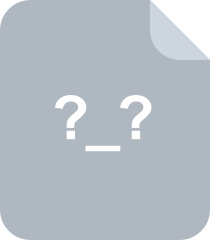
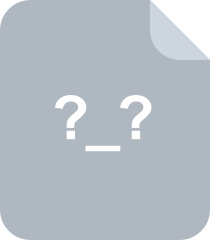
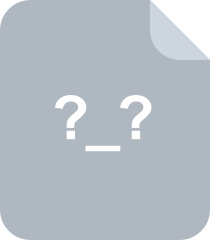
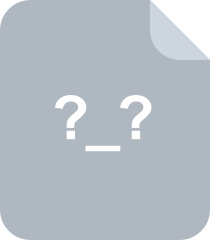
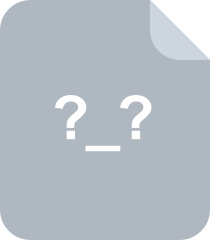
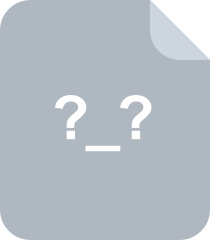
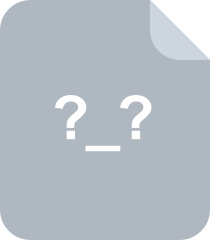
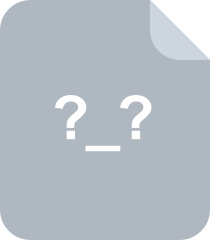
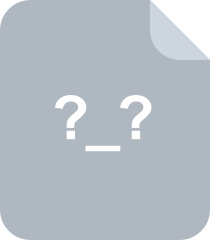
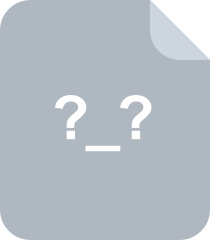
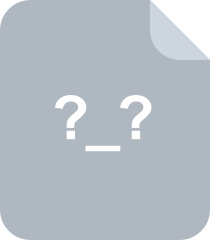
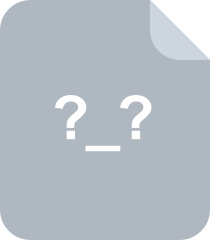
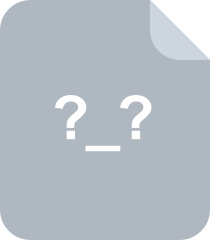
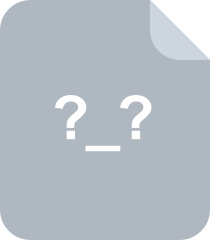
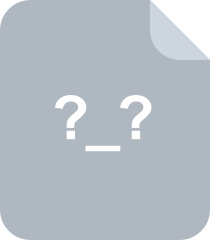
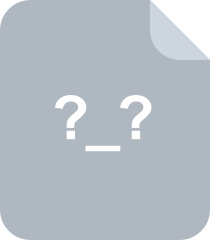
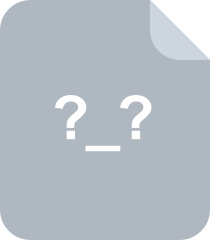
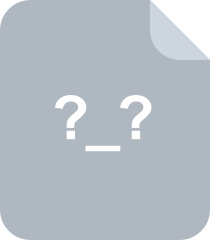
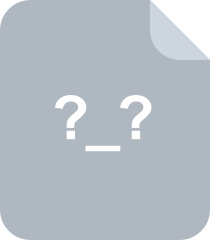
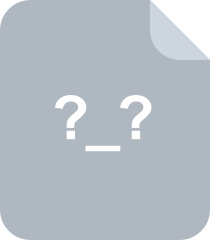
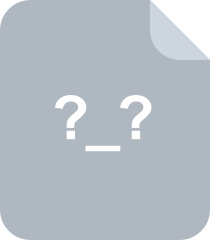
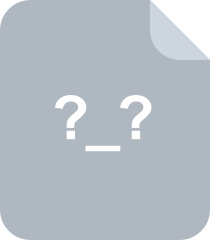
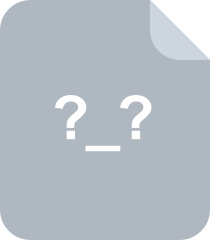
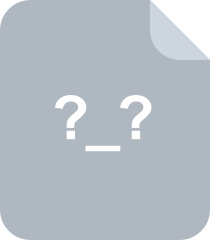
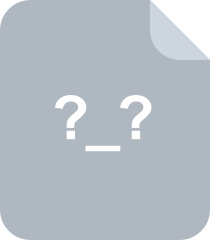
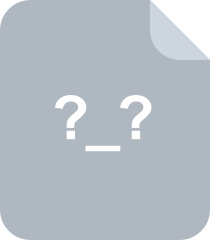
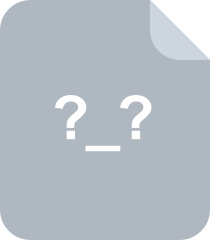
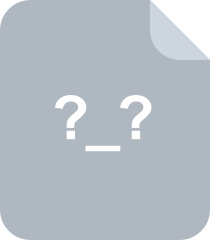
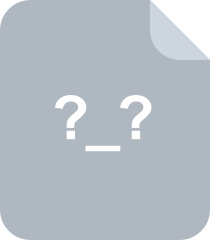
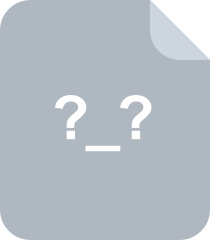
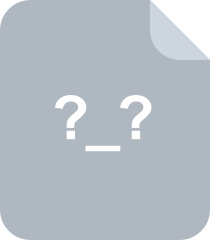
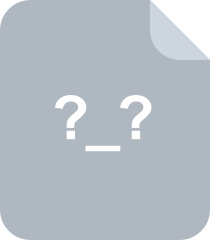
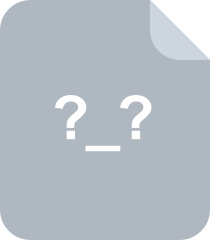
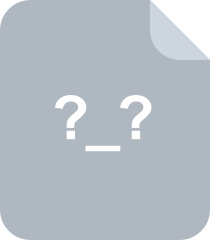
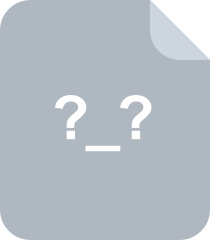
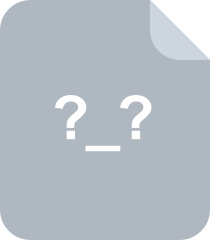
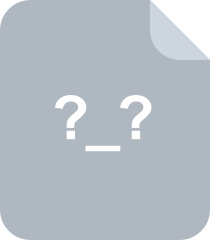
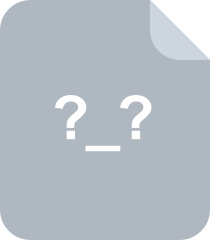
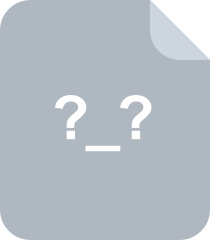
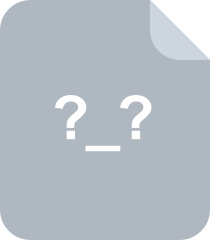
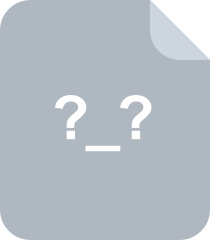
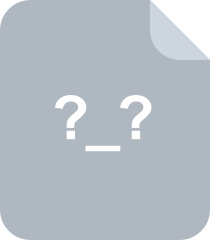
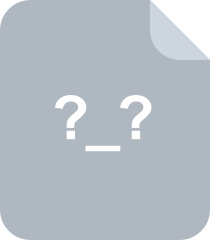
共 239 条
- 1
- 2
- 3
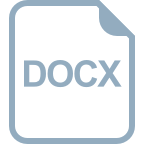
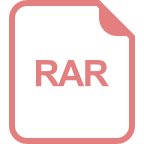
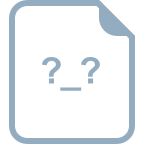
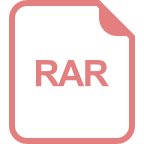
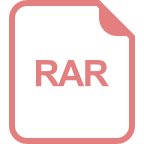

wudizuijimo
- 粉丝: 11
- 资源: 25
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

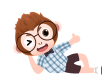
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0