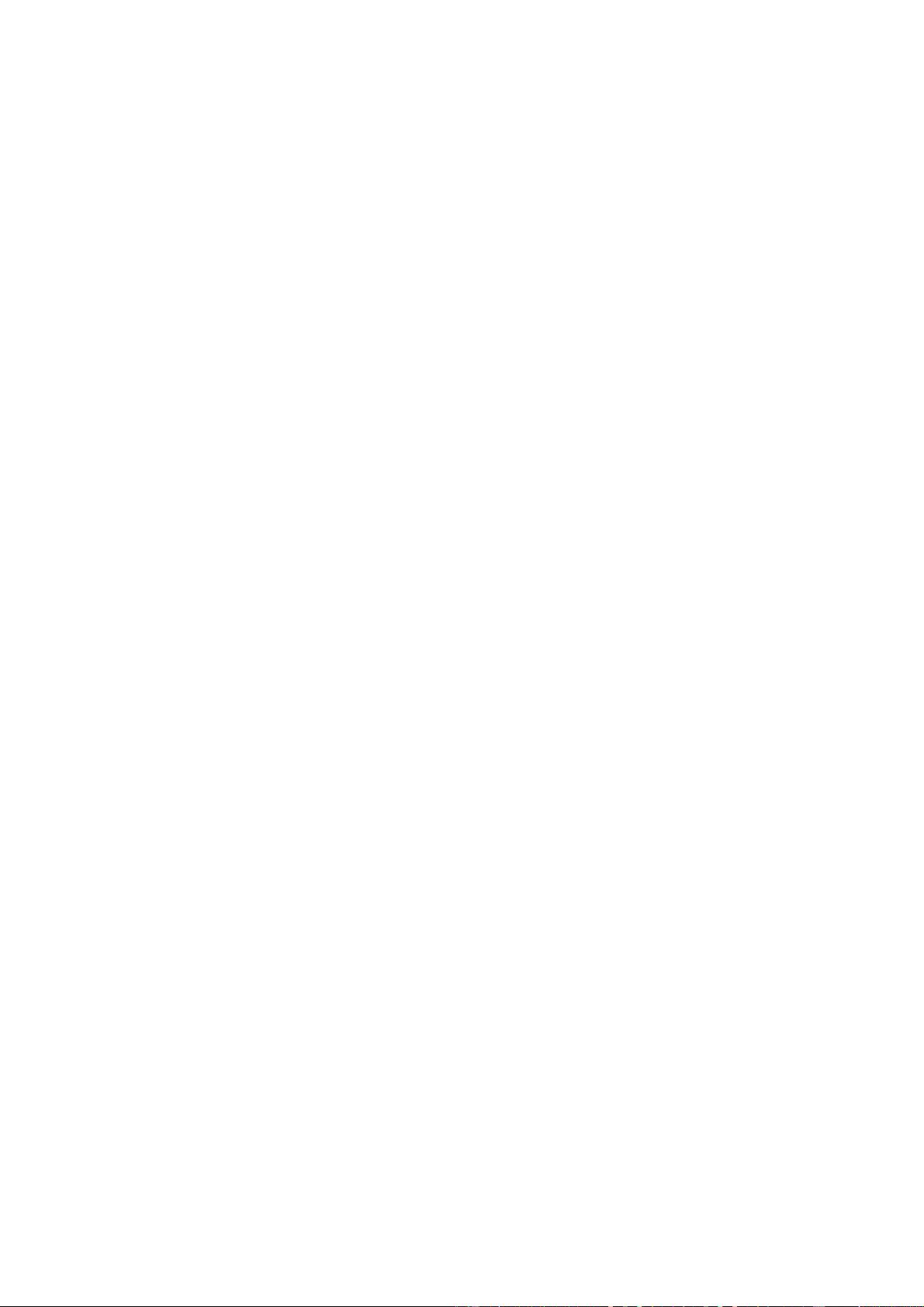
The C Book
Second Edition
by
Mike Banahan, Declan Brady and Mark Doran
Originally Published by Addison Wesley
In 1991
Not to Be Used for Any Business Purpose under Any Circumstance
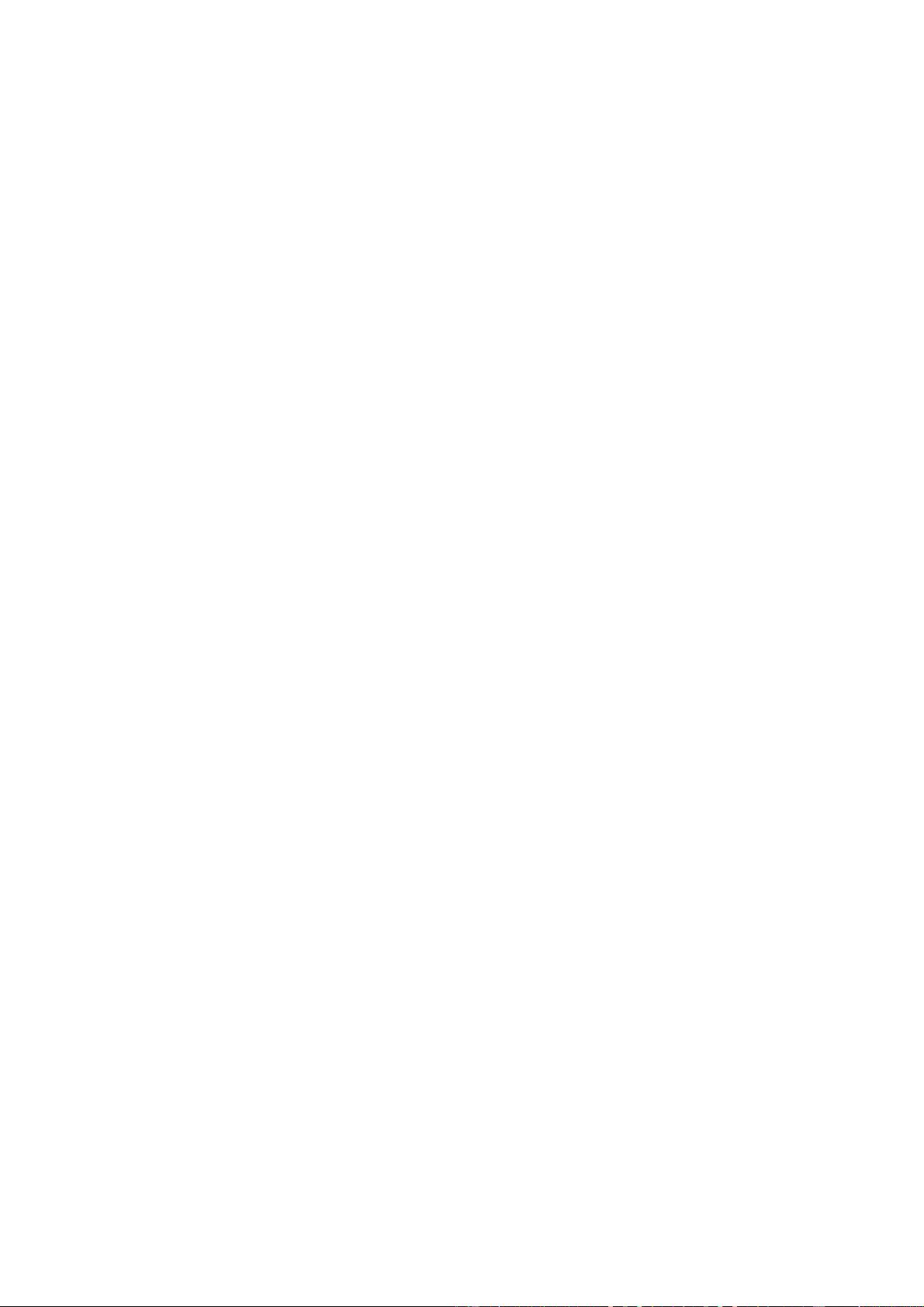
Table of Contents
•
Preface
o
About This Book
o
The Success of C
o
Standards
o
Hosted and Free-Standing Environments
o
Typographical conventions
o
Order of topics
o
Example programs
o
Deference to Higher Authority
o
Address for the Standard
•
Chapter 1. An Introduction to C
o
1.1. The form of a C program
o
1.2. Functions
o
1.3. A description of Example 1.1
o
1.4. Some more programs
o
1.5. Terminology
o
1.6. Summary
o
1.7. Exercises
•
Chapter 2. Variables and Arithmetic
o
2.1. Some fundamentals
o
2.2. The alphabet of C
o
2.3. The Textual Structure of Programs
o
2.4. Keywords and identifiers
o
2.5. Declaration of variables
o
2.6. Real types
o
2.7. Integral types
o
2.8. Expressions and arithmetic
o
2.9. Constants
o
2.10. Summary
o
2.11. Exercises
•
Chapter 3. Control of Flow and Logical Expressions
o
3.1. The Task ahead
o
3.2. Control of flow
o
3.3. More logical expressions
o
3.4. Strange operators
o
3.5. Summary
o
3.6. Exercises
•
Chapter 4. Functions
o
4.1. Changes
o
4.2. The type of functions
o
4.3. Recursion and argument passing
i
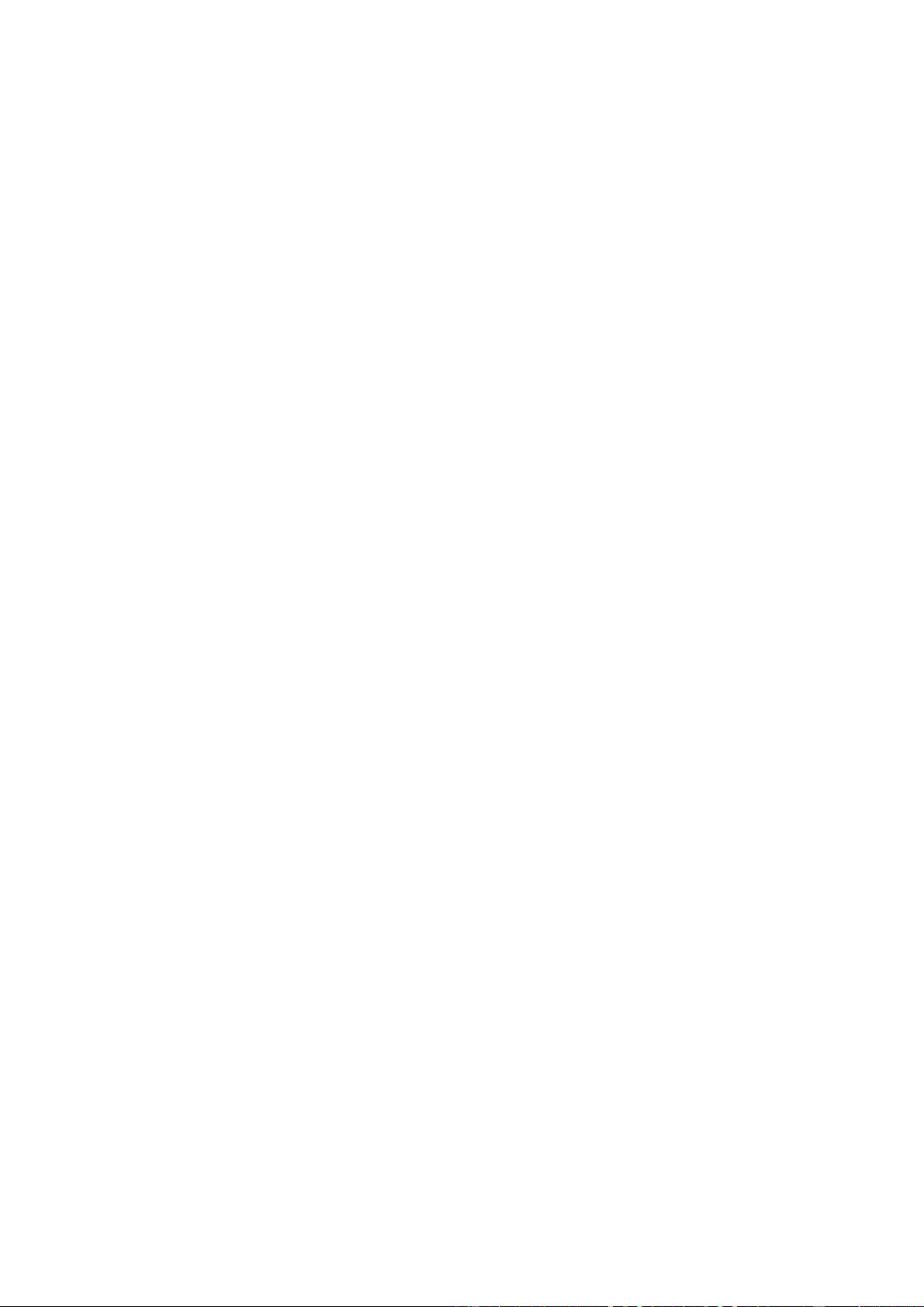
o
4.4. Linkage
o
4.5. Summary
o
4.6. Exercises
•
Chapter 5. Arrays and Pointers
o
5.1. Opening shots
o
5.2. Arrays
o
5.3. Pointers
o
5.4. Character handling
o
5.5. Sizeof and storage allocation
o
5.6. Pointers to functions
o
5.7. Expressions involving pointers
o
5.8. Arrays, the & operator and function declarations
o
5.9. Summary
o
5.10. Exercises
•
Chapter 6. Structured Data Types
o
6.1. History
o
6.2. Structures
o
6.3. Unions
o
6.4. Bitfields
o
6.5. Enums
o
6.6. Qualifiers and derived types
o
6.7. Initialization
o
6.8. Summary
o
6.9. Exercises
•
Chapter 7. The Preprocessor
o
7.1. Effect of the Standard
o
7.2. How the preprocessor works
o
7.3. Directives
o
7.4. Summary
o
7.5. Exercises
•
Chapter 8. Specialized Areas of C
o
8.1. Government Health Warning
o
8.2. Declarations, Definitions and Accessibility
o
8.3. Typedef
o
8.4. Const and volatile
o
8.5. Sequence points
o
8.6. Summary
•
Chapter 9. Libraries
o
9.1. Introduction
o
9.2. Diagnostics
o
9.3. Character handling
o
9.4. Localization
o
9.5. Limits
o
9.6. Mathematical functions
ii
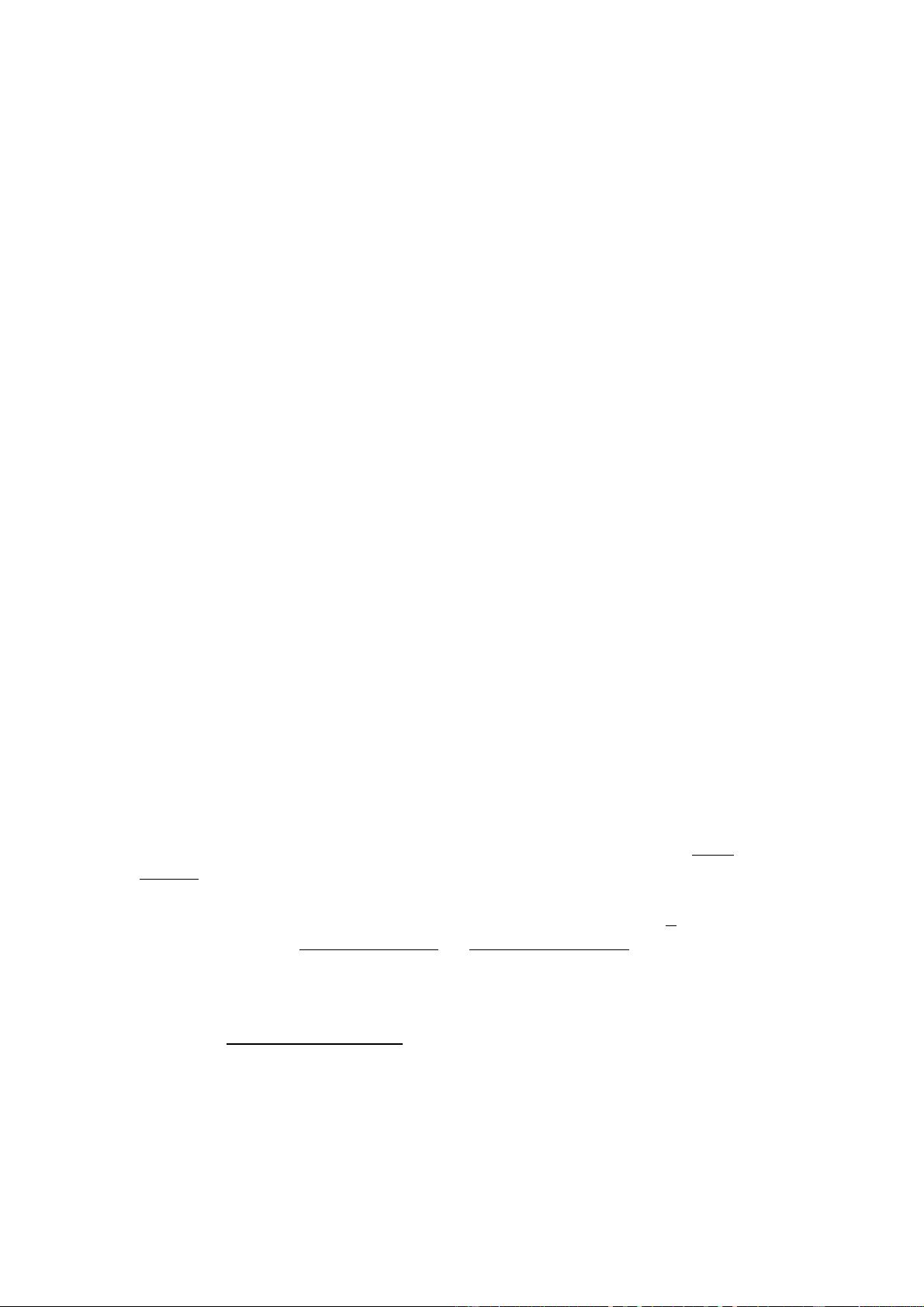
o
9.7. Non-local jumps
o
9.8. Signal handling
o
9.9. Variable numbers of arguments
o
9.10. Input and output
o
9.11. Formatted I/O
o
9.12. Character I/O
o
9.13. Unformatted I/O
o
9.14. Random access functions
o
9.15. General Utilities
o
9.16. String handling
o
9.17. Date and time
o
9.18. Summary
•
Chapter 10. Complete Programs in C
o
10.1. Putting it all together
o
10.2. Arguments to main
o
10.3. Interpreting program arguments
o
10.4. A pattern matching program
o
10.5. A more ambitious example
o
10.6. Afterword
•
Answers to Exercises
o
Chapter 1
o
Chapter 2
o
Chapter 3
o
Chapter 4
o
Chapter 5
o
Chapter 6
o
Chapter 7
•
Copyright and disclaimer
This is the online version of The C Book, second edition by Mike Banahan, Declan Brady and
Mark Doran, originally published by Addison Wesley in 1991. This version is made
freely
available.
While this book is no longer in print, it's content is still very relevant today. The
C language is still
popular, particularly for
open source software and embedded programming. We hope this book
will be useful, or at least interesting, to people who use C.
If you have any comments about this book, or if you find any bugs in its presentation, please send
a message to
consulting@gbdirect.co.uk.
iii
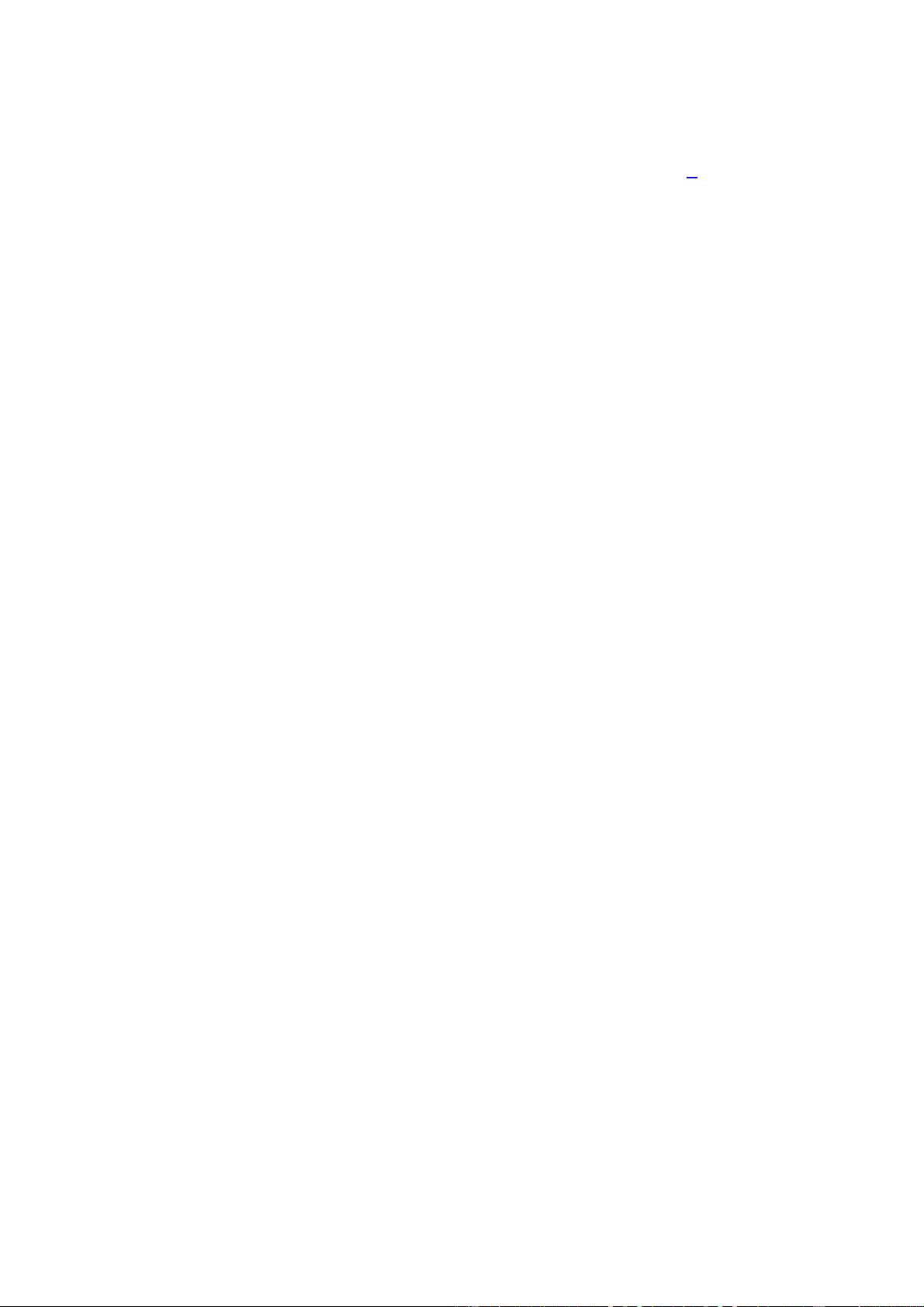
About This Book
This book was written with two groups of readers in mind. Whether you are new to C and want to
learn it, or already know the older version of the language but want to find out more about the new
standard, we hope that you will find what follows both instructive and at times entertaining too.
This is not a tutorial introduction to programming. The book is designed for programmers who
already have some experience of using a modern high-level procedural programming language. As
we explain later, C isn't really appropriate for complete beginners—though many have managed to
use it—so the book will assume that its readers have already done battle with the notions of
statements, variables, conditional execution, arrays, procedures (or subroutines) and so on. Instead
of wasting your time by ploughing through tedious descriptions of how to add two numbers
together and explaining that the symbol for multiplication is
*, the book concentrates on the things
that are special to C. In particular, it's the
way that C is used which is emphasized.
Those who already know C will be interested in the new Standard and how it affects existing C
programs. The effect on existing programs might not at first seem to be important to newcomers,
but in fact the ‘old’ and new versions of the language
are an issue for the beginner too. For some
years after the approval of the Standard, programmers will have to live in a world where they can
easily encounter a mixture of both the new and the old language, depending on the age of the
programs that they are working with. For that reason, the book highlights where the old and new
features differ significantly. Some of the old features are no ornament to the language and are well
worth avoiding; the Standard goes so far as to consider them obsolescent and recommends that
they should not be used. For that reason they are not described in detail, but only far enough to
allow a reader to understand what they mean. Anybody who intends to
write programs using these
old-style features should be reading a different book.
This is the second edition of the book, which has been revised to refer to the final, approved
version of the Standard. The first edition of the book was based on a draft of the Standard which
did contain some differences from the draft that was eventually approved. During the revision we
have taken the opportunity to include more summary material and an extra chapter illustrating the
use of C and the Standard Library to solve a number of small problems.
1