<?php
/*======================================================================*\
|| #################################################################### ||
|| # vBulletin 3.0.6
|| # ---------------------------------------------------------------- # ||
|| # Copyright ?000?005 Jelsoft Enterprises Ltd. All Rights Reserved. ||
|| # This file may not be redistributed in whole or significant part. # ||
|| # ---------------- VBULLETIN IS NOT FREE SOFTWARE ---------------- # ||
|| # http://www.vbulletin.com | http://www.vbulletin.com/license.html # ||
|| #################################################################### ||
\*======================================================================*/
error_reporting(E_ALL & ~E_NOTICE);
// predefine the phrasetypeids of a few phrase groups
define('PHRASETYPEID_HOLIDAY', 35);
define('PHRASETYPEID_ERROR', 1000);
define('PHRASETYPEID_REDIRECT', 2000);
define('PHRASETYPEID_MAILMSG', 3000);
define('PHRASETYPEID_MAILSUB', 4000);
define('PHRASETYPEID_SETTING', 5000);
define('PHRASETYPEID_ADMINHELP', 6000);
define('PHRASETYPEID_FAQTITLE', 7000);
define('PHRASETYPEID_FAQTEXT', 8000);
define('PHRASETYPEID_CPMESSAGE', 9000);
// this defines what adds the indent to forums in the forumjump menu
define('FORUM_PREPEND', ' ');
// ###################### Start construct phrase #######################
// this function is actually just a wrapper for sprintf
// but makes identification of phrase code easier
// and will not error if there are no additional arguments
function construct_phrase()
{
static $argpad;
$args = func_get_args();
$numargs = sizeof($args);
// if we have only one argument, just return the argument
if ($numargs < 2)
{
return $args[0];
}
else
{
// call sprintf() on the first argument of this function
$phrase = @call_user_func_array('sprintf', $args);
if ($phrase !== false)
{
return $phrase;
}
else
{
// if that failed, add some extra arguments for debugging
for ($i = $numargs; $i < 10; $i++)
{
$args["$i"] = "[ARG:$i UNDEFINED]";
}
if ($phrase = @call_user_func_array('sprintf', $args))
{
return $phrase;
}
// if it still doesn't work, just return the un-parsed text
else
{
return $args[0];
}
}
}
}
// ##################### Start vbstrtolower ###########################
// Only converts A-Z to a-z, doesn't change any other characters
function vbstrtolower($string)
{
global $stylevar;
if (function_exists('mb_strtolower') AND $newstring = @mb_strtolower($string, $stylevar['charset']))
{
return $newstring;
}
else
{
$length = strlen($string);
for ($x = 0; $x < $length; $x++)
{
$decvalue = ord(substr($string, $x, 1));
if ($decvalue >= 65 AND $decvalue <= 90)
{
$newstring .= chr($decvalue + 32);
}
else
{
$newstring .= chr($decvalue);
}
}
return $newstring;
}
}
// ##################### Start vbstrlen ###########################
// Converts html entities to a regular character so strlen can be performed
function vbstrlen($string)
{
$string = preg_replace('#&\#([0-9]+);#', '_', $string);
return strlen($string);
}
// ####################### Start sanitize_pageresults #####################
function sanitize_pageresults($numresults, &$page, &$perpage, $maxperpage = 20, $defaultperpage = 20)
{
$perpage = intval($perpage);
if ($perpage < 1)
{
$perpage = $defaultperpage;
}
else if ($perpage > $maxperpage)
{
$perpage = $maxperpage;
}
$numpages = ceil($numresults / $perpage);
if ($page < 1)
{
$page = 1;
}
else if ($page > $numpages)
{
$page = $numpages;
}
}
// ###################### Start validemail #######################
function is_valid_email($email)
{
// checks for a valid email format
return preg_match('#^[a-z0-9.!\#$%&\'*+-/=?^_`{|}~]+@([0-9.]+|([^\s]+\.+[a-z]{2,6}))$#si', $email);
}
// ###################### Start vb_number_format #######################
// format a number with user's own decimal and thousands chars
function vb_number_format($number, $decimals = 0, $bytesize = false)
{
global $bbuserinfo, $vbphrase;
if ($bytesize)
{
if ($number >= 1073741824)
{
$number = $number / 1073741824;
$decimals = 2;
$type = " $vbphrase[gigabytes]";
}
else if ($number >= 1048576)
{
$number = $number / 1048576;
$decimals = 2;
$type = " $vbphrase[megabytes]";
}
else if ($number >= 1024)
{
$number = $number / 1024;
$decimals = 1;
$type = " $vbphrase[kilobytes]";
}
else
{
$decimals = 0;
$type = " $vbphrase[bytes]";
}
}
return str_replace('_', ' ', number_format($number, $decimals, $bbuserinfo['lang_decimalsep'], $bbuserinfo['lang_thousandsep'])) . $type;
}
// ###################### Start getmembergroupids #######################
// returns an array of usergroupids from all the usergroups a user belongs to
function fetch_membergroupids_array($user, $getprimary = true)
{
if ($user['membergroupids'])
{
$membergroups = explode(',', str_replace(' ', '', $user['membergroupids']));
}
else
{
$membergroups = array();
}
if ($getprimary)
{
$membergroups[] = $user['usergroupid'];
}
return array_unique($membergroups);
}
// ###################### Start is member of #######################
// returns true/false if a $userinfo belongs to $usergroupid
// $userinfo must contain (userid, usergroupid, membergroupids)
function is_member_of($userinfo, $usergroupid)
{
static $user_memberships;
if ($userinfo['usergroupid'] == $usergroupid)
{
// user's primary usergroup is $usergroupid - return true
return true;
}
else if (!is_array($user_memberships["$userinfo[userid]"]))
{
// fetch membergroup ids
$user_memberships["$userinfo[userid]"] = fetch_membergroupids_array($userinfo);
}
// return true/false depending on membergroup ids
return in_array($usergroupid, $user_memberships["$userinfo[userid]"]);
}
// ###################### Start is_in_coventry #######################
function in_coventry($userid, $includeself = false)
{
global $vboptions, $bbuserinfo;
static $Coventry;
// if user is guest, or user is bbuser, user is NOT in Coventry.
if ($userid == 0 OR ($userid == $bbuserinfo['userid'] AND $includeself == false))
{
return false;
}
if (!is_array($Coventry))
{
if (trim($vboptions['globalignore']) != '')
{
$Coventry = preg_split('#\s+#s', $vboptions['globalignore'], -1, PREG_SPLIT_NO_EMPTY);
}
else
{
$Coventry = array();
}
}
// if Coventry is empty, user is not in Coventry
if (empty($Coventry))
{
return false;
}
// return whether or not user's id is in Coventry
return in_array($userid, $Coventry);
}
// ###################### Start queryphrase #######################
function fetch_phrase($phrasename, $phrasetypeid, $strreplace = '', $doquotes = true, $alllanguages = false, $languageid = -1)
{
// we need to do some caching in this function I believe
global $DB_site;
static $phrase_cache;
if (!empty($strreplace))
{
if (strpos("$phrasename", $strreplace) !== false)
{
$phrasename = substr($phrasename, strlen($strreplace));
}
}
$phrasetypeid = intval($phrasetypeid);
if (!isset($phrase_cache["{$phrasetypeid}-{$phrasename}"]))
{
$getphrases = $DB_site->query("
SELECT text, languageid
FROM " . TABLE_PREFIX . "phrase
WHERE phrasetypeid = $phrasetypeid
AND varname = '" . addslashes($phrasename) . "'"
. iif(!$alllanguages, "AND languageid IN (-1, 0, " . intval(LANGUAGEID) . ")")
);
while ($getphrase = $DB_site->fetch_array($getphrases))
{
$phrase_cache["{$phrasetypeid}-{$phrasename}"]["$getphrase[languageid]"] = $getphrase['text'];
}
unset($getphrase);
$DB_site->free_result($getphrases);
}
$phrase = &$phrase_cache["{$phrasetypeid}-{$phrasename}"];
if ($languageid == -1)
{ // didn't pass in a languageid as this is the default value so use the browsing user's languageid
$languageid = LANGUAGEID;
}
else if ($languageid == 0)
{ // the user is using forum default
global $vboptions;
$
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
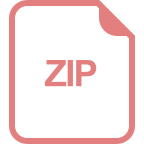
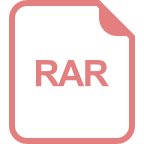
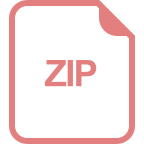
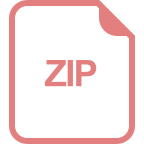
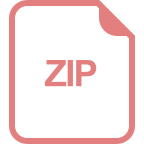
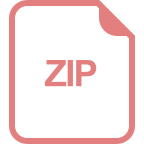
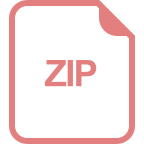
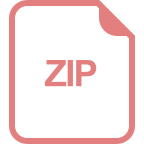
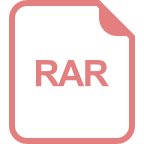
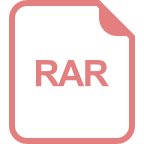
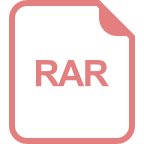
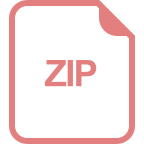
收起资源包目录

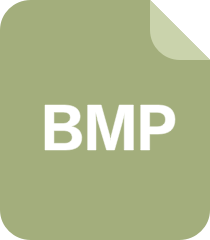
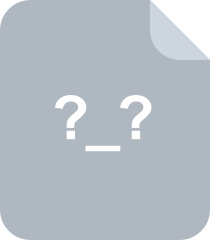
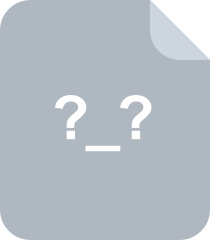
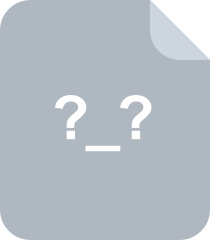
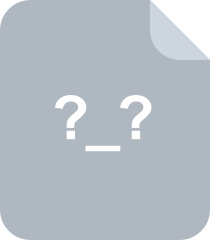
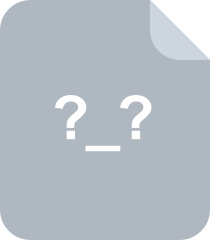
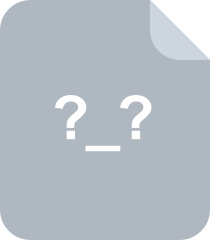
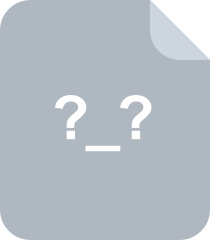
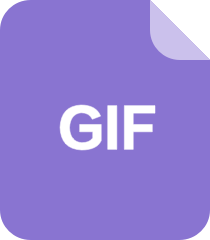
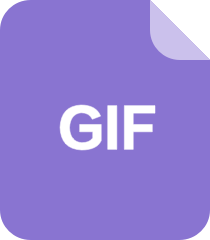
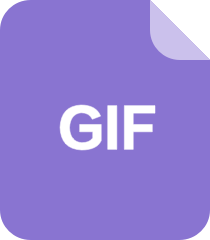
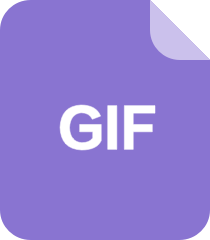
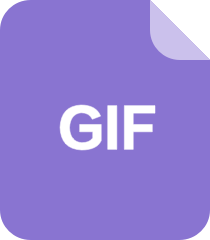
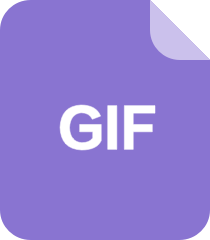
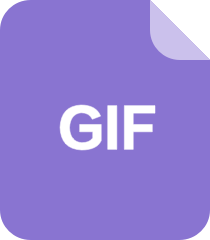
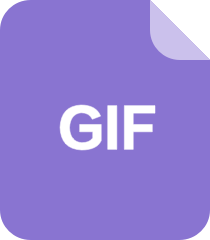
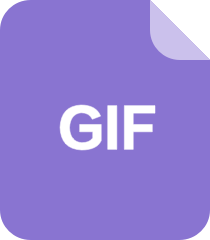
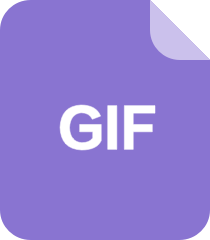
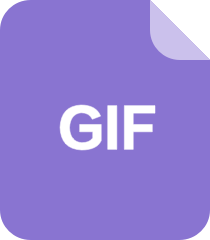
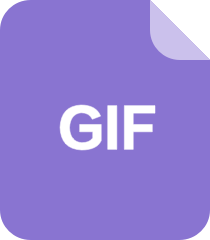
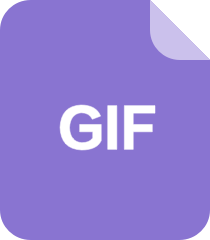
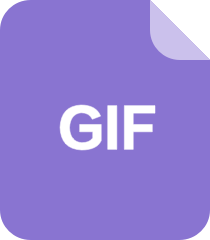
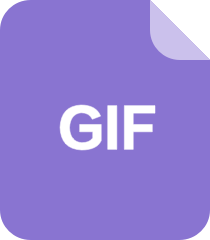
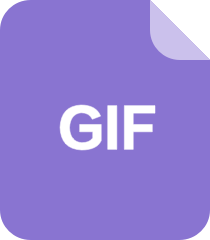
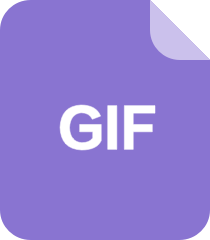
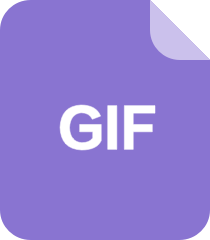
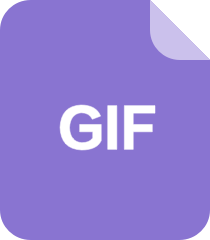
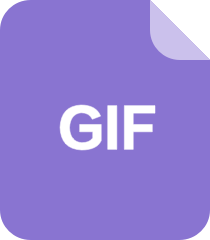
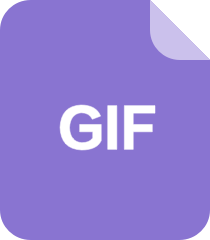
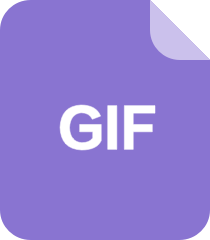
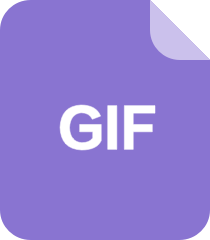
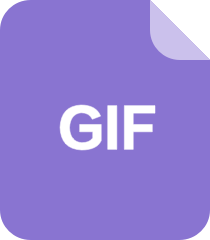
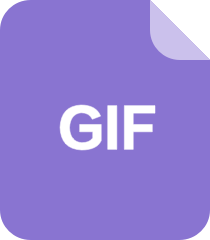
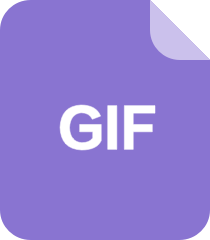
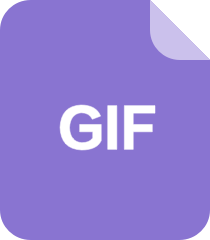
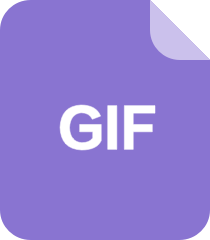
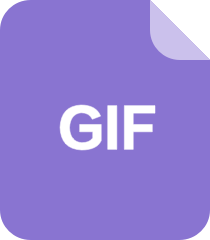
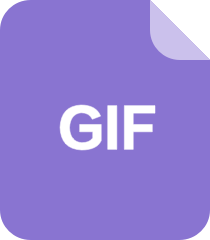
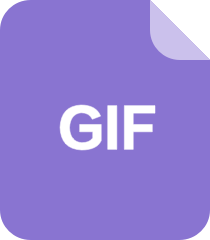
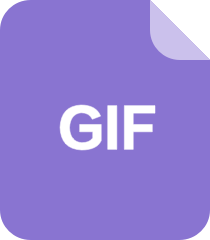
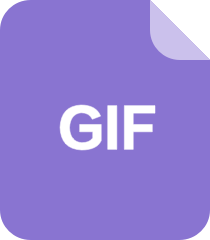
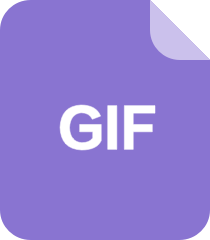
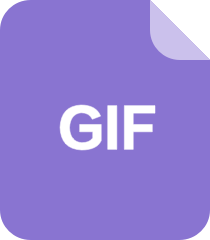
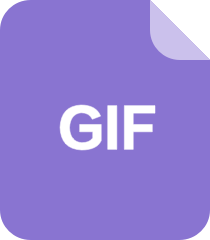
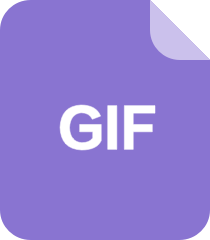
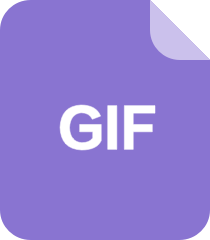
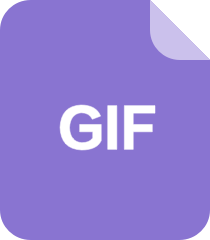
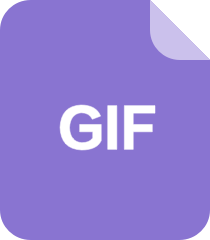
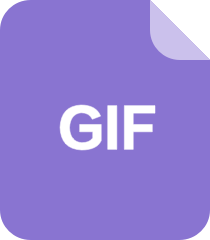
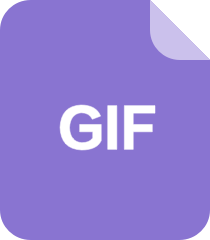
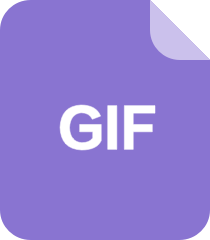
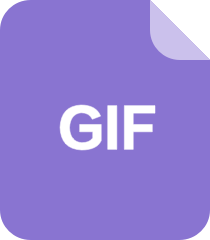
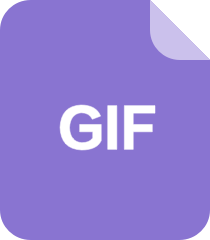
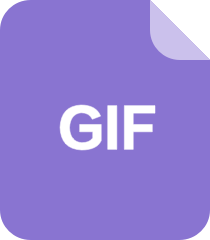
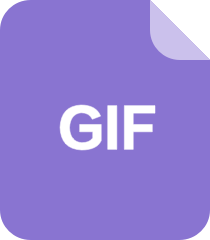
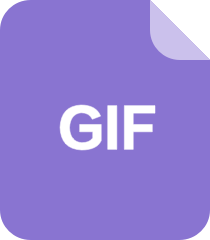
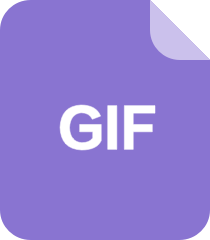
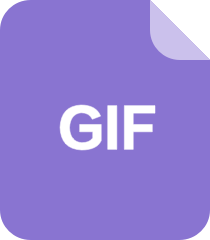
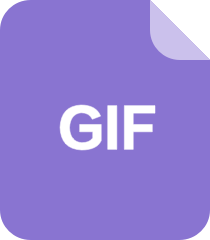
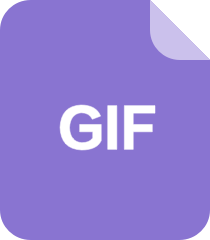
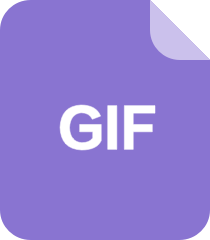
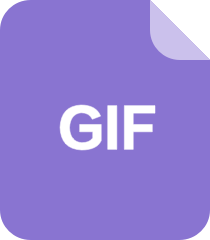
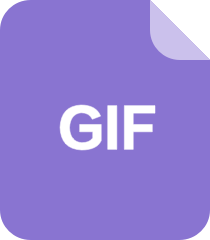
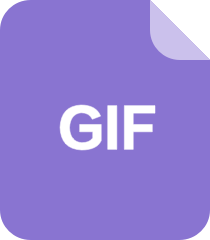
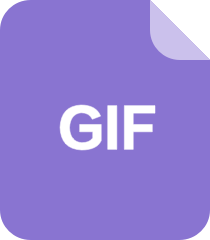
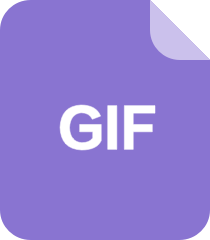
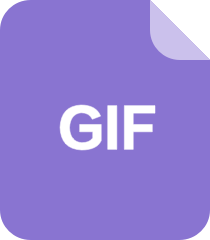
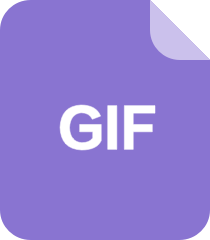
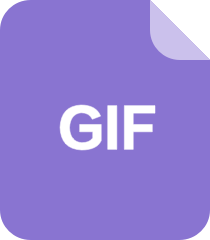
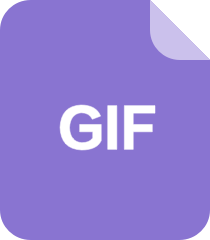
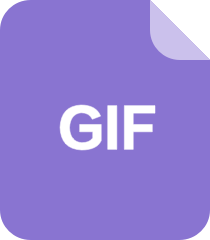
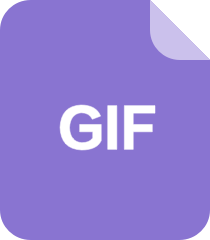
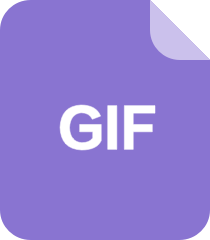
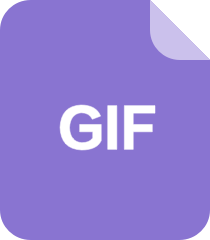
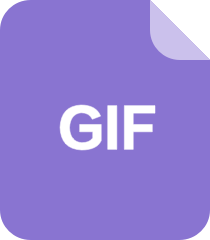
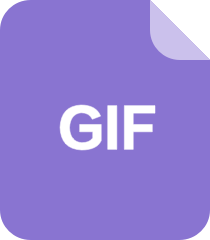
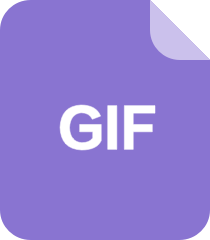
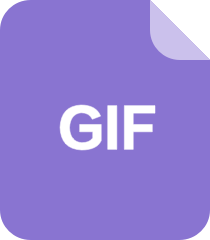
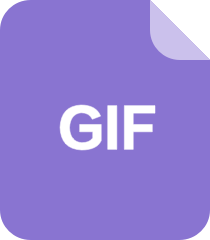
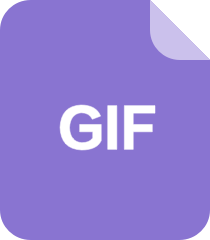
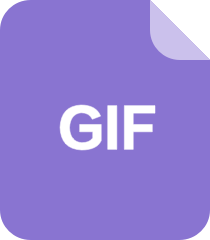
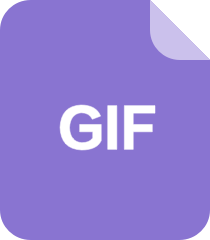
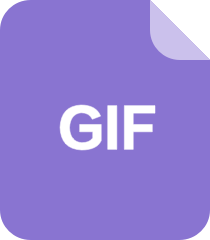
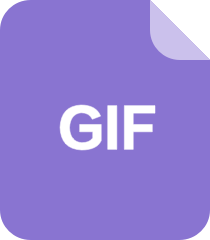
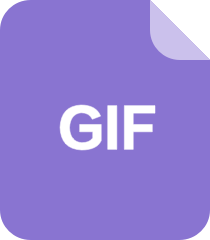
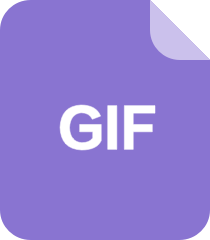
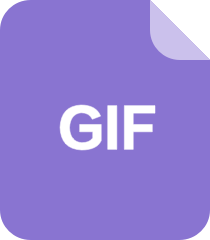
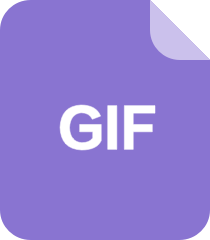
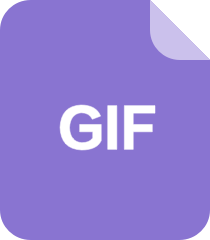
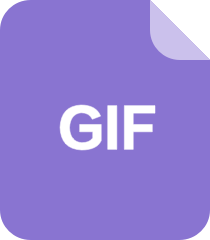
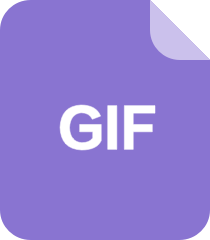
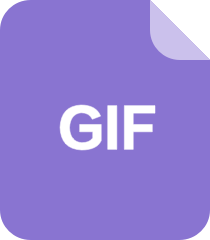
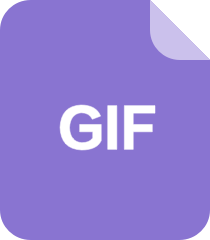
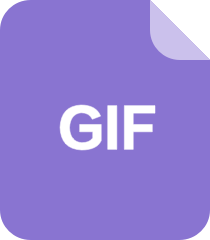
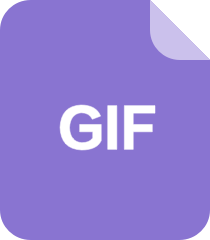
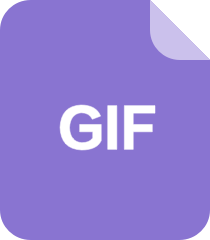
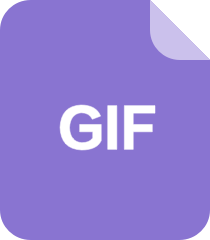
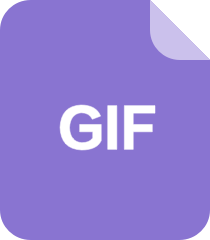
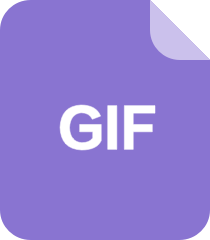
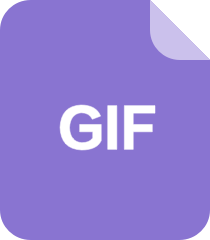
共 557 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
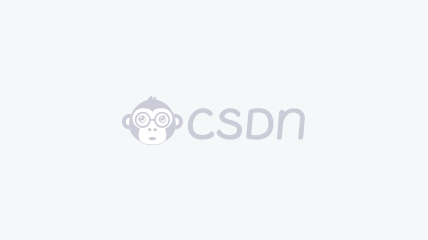


wsnbb_2023
- 粉丝: 17
- 资源: 6002
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

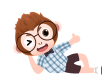
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


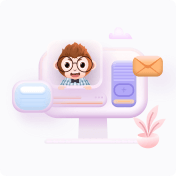
安全验证
文档复制为VIP权益,开通VIP直接复制
