package ljk.QRcode.swetake;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.geom.AffineTransform;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import jp.sourceforge.qrcode.QRCodeDecoder;
import jp.sourceforge.qrcode.exception.DecodingFailedException;
import com.swetake.util.Qrcode;
/**
* 二维码生成demo 使用 swetake开源工具
*
* link:http://blog.csdn.net/wojiao555555/article/details/36184705
*/
public class QRCodeSwetakeDemo {
public static void main(String args[]) throws Exception{
String sms = "http://www.wodsy.com";
String filePath = "C:\\Users\\Administrator\\Desktop\\QRcode124.png";
//QR码是正方形的,所以最好宽度和高度值相同
int width = 500;
int height = 500;
createrImage(sms, filePath, width, height);
String decodeMsg = decoderImage(filePath);
System.out.println("二维码内容为:"+decodeMsg);
}
/**
* 创建二维码
*
* @param sms_info 信息内容 如:www.wodsy.com
* @param filePath 输出路径 如:"C:\\Users\\Administrator\\Desktop\\QRcode124.png"
* @param width 宽度 生成二维码的宽度
* @param height 高度 生成二维码的高度
* @throws Exception
*/
public static void createrImage(String sms_info,String filePath,int width,int height) throws Exception {
try {
Qrcode testQrcode = new Qrcode();
testQrcode.setQrcodeErrorCorrect('M');//设置错误容错率 L(7%) M(15%) Q(25%) H(30%)
testQrcode.setQrcodeEncodeMode('B');//设置编码模式 N数字 A英文 其他为Binary (设置为A的时候生成的二维码异常)
testQrcode.setQrcodeVersion(7);//设置版本 (1~40)
String testString = sms_info;
byte[] d = testString.getBytes("UTF-8");
System.out.println("信息编码后长度:"+d.length);
// 限制最大字节数为120。容错率、编码模式、版本 这几个参数决定了这个二维码能存储多少字节。
if (d.length > 0 && d.length < 120) {
boolean[][] s = testQrcode.calQrcode(d);
BufferedImage bi = new BufferedImage(s.length, s[0].length,
BufferedImage.TYPE_BYTE_BINARY);
Graphics2D g = bi.createGraphics();
g.setBackground(Color.WHITE);
g.clearRect(0, 0, s.length, s[0].length);
g.setColor(Color.BLACK);
int mulriple=1; // 1像素
for (int i = 0; i < s.length; i++) {
for (int j = 0; j < s.length; j++) {
if (s[j][i]) {
g.fillRect(j * mulriple, i * mulriple, mulriple, mulriple);
}
}
}
g.dispose();//释放此图形的上下文以及它使用的所有系统资源。调用 dispose 之后,就不能再使用 Graphics 对象。
bi.flush();
File f = new File(filePath);
if (!f.exists()) {
f.createNewFile();
}
// 图像缩放
bi=resize(bi,width,height);
// 创建图片
ImageIO.write(bi, "png", f);
}else {
System.out.println("信息编码后长度过长,请升高版本值(比如 testQrcode.setQrcodeVersion(10)),或降低容错率(比如:testQrcode.setQrcodeErrorCorrect(L))");
}
}
catch (Exception e) {
e.printStackTrace();
}
}
/**
* 图像缩放
* @param source
* @param targetW
* @param targetH
* @return
*/
public static BufferedImage resize(BufferedImage source, int targetW,
int targetH) {
int type = source.getType();
BufferedImage target = null;
double sx = (double) targetW / source.getWidth();
double sy = (double) targetH / source.getHeight();
target = new BufferedImage(targetW, targetH, type);
Graphics2D g = target.createGraphics();
g.drawRenderedImage(source, AffineTransform.getScaleInstance(sx, sy));
g.dispose();
return target;
}
/**
* 解析二维码
* @param imgPath 二维码路径
* @return 二维码内容
*/
public static String decoderImage(String imgPath){
File imageFile = new File(imgPath);
BufferedImage bufImg = null;
String content = null;
try {
bufImg = ImageIO.read(imageFile);
QRCodeDecoder decoder = new QRCodeDecoder();
content = new String(decoder.decode(new TwoDimensionCodeImage(bufImg)), "UTF-8");
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
e.printStackTrace();
} catch (DecodingFailedException dfe) {
System.out.println("Error: " + dfe.getMessage());
dfe.printStackTrace();
}
return content;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
二维码创建与解析的demo,包含两种方式,swetake,Zxing。 该demo可直接运行创建二维码,也可直接解析二维码,两种方式都有demo。 在我的帖子:http://blog.csdn.net/wojiao555555/article/details/36184705 中有详细的解析说明。
资源推荐
资源详情
资源评论
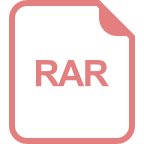
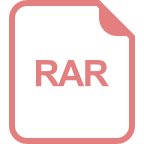
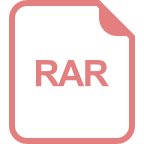
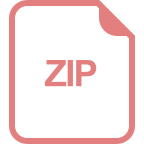
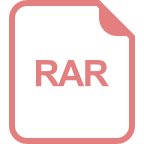
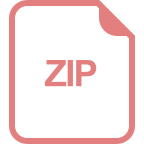
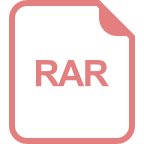
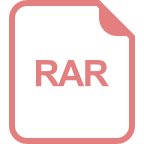
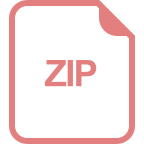
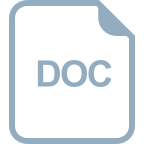
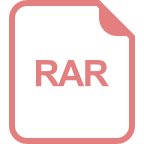
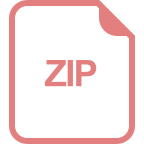
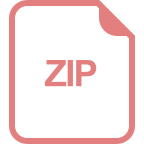
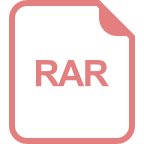
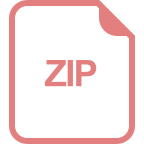
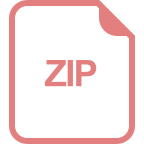
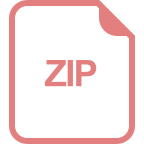
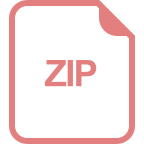
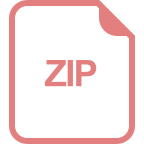
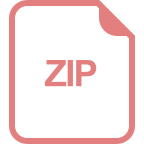
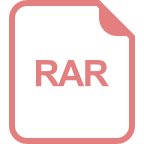
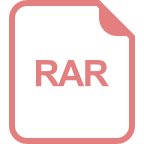
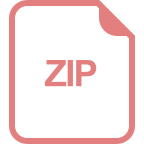
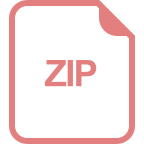
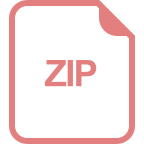
收起资源包目录


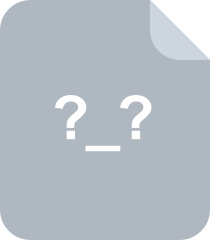




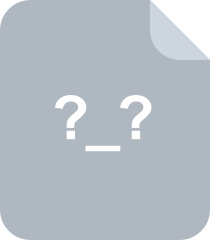
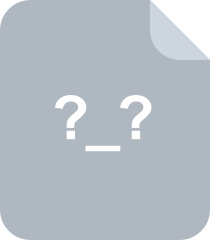
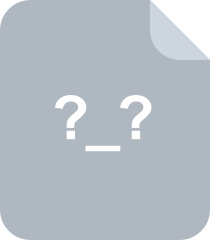

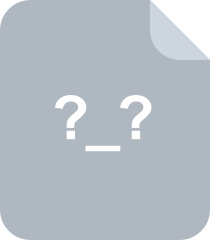
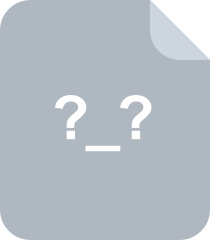
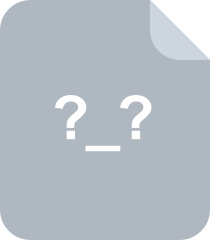

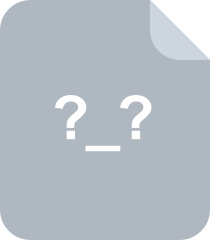
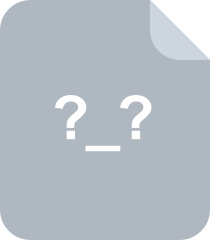




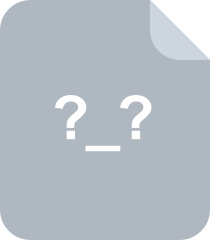
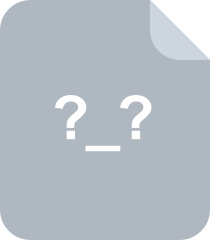
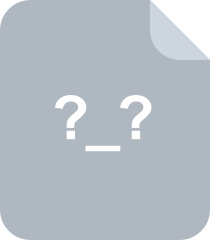

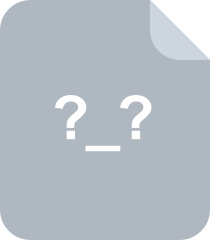
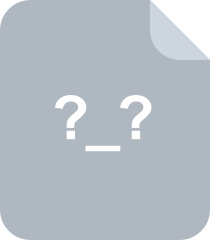
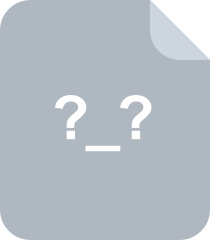
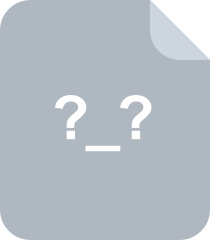

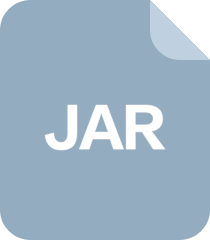
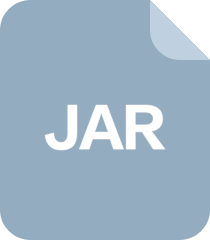
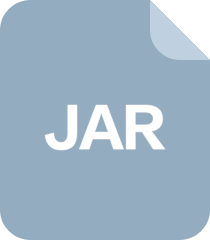
共 19 条
- 1
资源评论
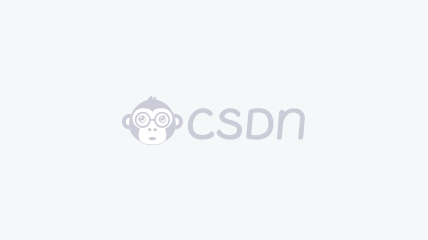

oO感悟人生Oo
- 粉丝: 10
- 资源: 15
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

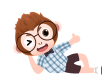
安全验证
文档复制为VIP权益,开通VIP直接复制
