package jstarcraft.core;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Image;
import java.awt.Point;
import java.awt.Toolkit;
import java.awt.geom.AffineTransform;
import java.awt.image.BufferedImage;
import java.awt.image.DirectColorModel;
import java.awt.image.MemoryImageSource;
import java.awt.image.PixelGrabber;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.swing.ImageIcon;
import jstarcraft.icon.BaseIcon;
import jstarcraft.icon.HouseIcon;
import jstarcraft.icon.ScvIcon;
import jstarcraft.tile.Barrack;
import jstarcraft.tile.Headquarter;
import jstarcraft.tile.House;
import jstarcraft.tile.Marine;
import jstarcraft.tile.Mine;
import jstarcraft.tile.Scv;
import jstarcraft.tile.Sprite;
import jstarcraft.tile.Supply;
import jstarcraft.tile.Tile;
import jstarcraft.tile.Sprite.Animation;
/**
* 游戏中资源管理的类,包括图片资源和常量字符串等。
*
* @author Xewee.Zhiwei.Wang
* @time 2011-9-14 下午06:30:35
*/
public class ResourceManager {
private GridMap gridMap;
public static ResourceManager resourceManager;
//图片缓存的Map
//key为图片文件的文件名,value为图片文件的Image对象
private static Map<String,Image> IMAGE_CACHE = new HashMap<String, Image>();
/**
* 根据文件名从IMAGE_CACHE中得到图片的Image对象,
* 若不存在这个key,则新建并加入到IMAGE_CACHE。
*
* @author Xewee.Zhiwei.Wang
* @param fileName
* @return Image
*/
public static Image loadImage(String fileName) {
if(!IMAGE_CACHE.containsKey(fileName)){
// System.out.println(ResourceManager.class.getResource("/res/images/"+fileName));
Image image = new ImageIcon("res/images/"+fileName).getImage();
if (image != null) {
IMAGE_CACHE.put(fileName, image);
return image;
}
else {
return null;
}
}
return IMAGE_CACHE.get(fileName);
}
public static GridMapRender load(int type,List<Integer> types){
resourceManager = new ResourceManager(type,types);
return resourceManager.gridMap.getTileMapRender();
}
public GridMap getGridMap() {
return gridMap;
}
/**
* 初始化缩略图
* @return
*/
private Image initMapBg(){
BufferedImage buffer = new BufferedImage(128,128,BufferedImage.TYPE_INT_ARGB);
Graphics2D g2 = (Graphics2D) buffer.getGraphics();
for (int y = 0; y < gridMap.getHeight(); ++y) {
for (int x = 0; x < gridMap.getWidth(); ++x) {
AffineTransform affineTransform = new AffineTransform();
affineTransform.setToTranslation(x*10, y*5);
affineTransform.scale(0.2, 0.2);
g2.drawImage(Constant.IMAGE_BG, affineTransform, null);
}
}
return buffer;
}
private ResourceManager(int type,List<Integer> types) {
Constant.load();
gridMap = loadMap(type,types);
Constant.IMAGE_MAP_BG = initMapBg();
Constant.progress = Constant.TOTAL;
}
private GridMap loadMap(int type,List<Integer> types) {
List<String> list = new ArrayList<String>();
int width = readMap(list, "startmap1.map");
GridMap map = new GridMap(width, list.size());
GridMapRender mapRender = new GridMapRender(map);
mapRender.type = type;
map.setTileMapRender(mapRender);
map.setIconMap(Constant.ICON_MAP);
for (int y = 0; y < list.size(); ++y) {
// 读取每一行
String s = list.get(y);
for (int x = 0; x < s.length(); ++x) {
int code = (int)s.charAt(x)-40;
if(code<0||code>Constant.TILE_TABLE.length)
continue;
if(code<80&&!types.contains((code/20)))
continue;
Tile tile = Constant.TILE_TABLE[code].clone(x, y,map);
tile.setHealth(1.0f);
map.add(tile);
//确定当前视觉范围
if(tile.getType()==type&&tile instanceof Headquarter){
//获取当前基地坐标
int hqX = GridMapRender.tileXToPx(tile.getTileX());
int hqY = GridMapRender.tileYToPx(tile.getTileY());
Point size = tile.getSize();
//获取屏幕中心坐标
int centerX = (800-GridMapRender.tileXToPx(size.x))/2;
int centerY = (600-GridMapRender.tileYToPx(size.y))/2;
mapRender.setOffset(Math.max(hqX-centerX, 0),Math.max(hqY-centerY, 0));
}
}
}
return map;
}
/**
* 解析地图文件,并将解析的字符串结果保存到list中
*
* @author Xewee.Zhiwei.Wang
* @time 2011-9-14 下午07:11:56
* @param list
* @param fileName
* @return
*/
private static int readMap(List<String> list, String fileName) {
BufferedReader br = null;
int width = 0;
try {
br = new BufferedReader(new InputStreamReader(new FileInputStream("res/map/" + fileName)));
String line = null;
while ((line = br.readLine()) != null) {
if (line.startsWith("#"))
continue;
list.add(line);
width = Math.max(width, line.length());
}
return width;
} catch (Exception e) {
e.printStackTrace();
return -1;
} finally {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public static class Constant {
public static String IP;
public static Color GREEN;
public static Color RED=Color.red;
//背景
public static Image IMAGE_BG;
//控制台
public static Image IMAGE_CONTROL;
//光照效果
public static Image SCV_SPARK;
//矿产
public static Image ICON_MINE;
//人口
public static Image ICON_MAN;
//矿产错误信息
public static String MINE_ERROR="没资源咯,还是多去采点矿吧";
//人口错误信息
public static String MAN_ERROR="太挤啦,快造点房子出来吧";
public static String BUILD_ERROR="地基不稳,不能修在这里";
public static Image IMAGE_MAP_BG;
//ICONS
public static final BaseIcon[][] SCV_ICONS = new BaseIcon[2][3];
public static final BaseIcon[][] HQ_ICONS = new BaseIcon[2][3];
public static final BaseIcon[][] BACK_ICONS = new BaseIcon[2][3];
//ICON资源表
public static final Map<Integer,BaseIcon[][]> ICON_TABLE = new HashMap<Integer, BaseIcon[][]>();
//Tile资源表
public static final Tile[] TILE_TABLE = new Tile[82];
private static final int TYPE = 4;
public static final int TYPE_SIZE = 20;
//进度控制
private static float progress;
private static final float TOTAL=19;
static void load(){
IMAGE_BG = loadImage("bg1.gif");
IMAGE_CONTROL = loadImage("panel/main.gif");
SCV_SPARK = loadImage("unit/0_scv_spark.gif");
ICON_MINE = loadImage("panel/mine.gif");
ICON_MAN = loadImage("panel/man.gif");
GREEN = new Color(16, 252, 24);
initTile();
}
private static final Map<String, BaseIcon> ICON_MAP = new HashMap<String, BaseIcon>();
private static int count = 0;
private static BaseIcon createIcon(BaseIcon icon){
ICON_MAP.put("icon"+(++count), icon);
return icon;
}
private static void initTile(){
//加载图片
Image scv = loadImage("unit/0_scv_red.gif");
Image marine = loadImage("unit/0_marine_red.gif");
Image marineFight = loadImage("unit/0_fight_marine_red.png");
// Image tank = loadImage("unit/0_tank.gif");
Image supply = loadImage("build/0_supply_red.gif");
Image barrack = loadImage("build/0_barrack_red.gif");
Image base = loadImage("build/0_hq_red.gif");
Image mine = loadImage("block/mine.gif");
//转换成buffer
BufferedImage scv_buffer=imageToBuffer(scv);
BufferedImage marine_buffer=imageToBuffer(marine);
BufferedImage marineFight_buffer=imageToBuffer(marineFight);
BufferedImage supply_buffe
没有合适的资源?快使用搜索试试~ 我知道了~
基于java的开发源码-即时战略游戏 StarCraft Ⅰ.zip
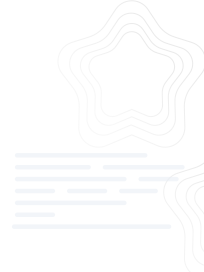
共792个文件
html:299个
svn-base:264个
java:90个

0 下载量 113 浏览量
2024-03-05
20:01:13
上传
评论
收藏 2.06MB ZIP 举报
温馨提示
基于java的开发源码-即时战略游戏 StarCraft Ⅰ.zip 基于java的开发源码-即时战略游戏 StarCraft Ⅰ.zip 基于java的开发源码-即时战略游戏 StarCraft Ⅰ.zip 基于java的开发源码-即时战略游戏 StarCraft Ⅰ.zip 基于java的开发源码-即时战略游戏 StarCraft Ⅰ.zip 基于java的开发源码-即时战略游戏 StarCraft Ⅰ.zip 基于java的开发源码-即时战略游戏 StarCraft Ⅰ.zip 基于java的开发源码-即时战略游戏 StarCraft Ⅰ.zip 基于java的开发源码-即时战略游戏 StarCraft Ⅰ.zip
资源推荐
资源详情
资源评论
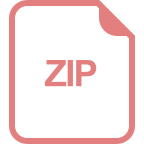
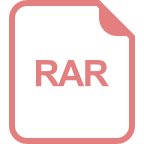
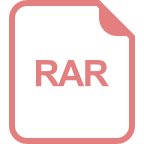
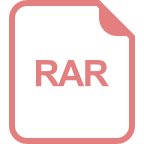
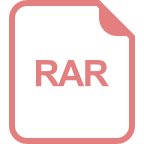
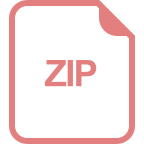
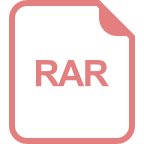
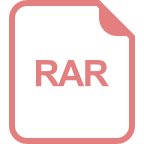
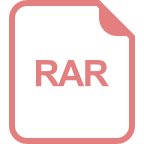
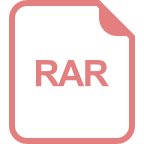
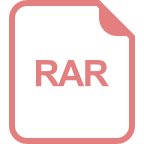
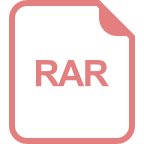
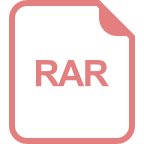
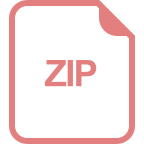
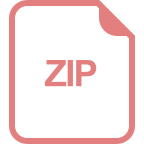
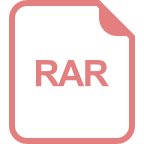
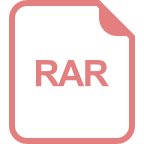
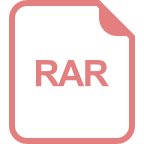
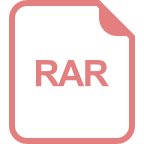
收起资源包目录

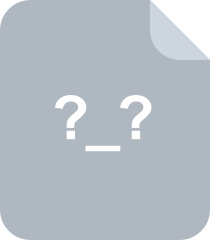
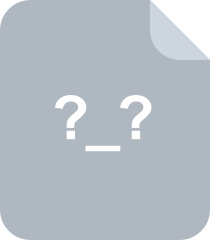
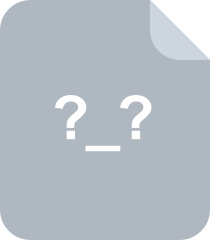
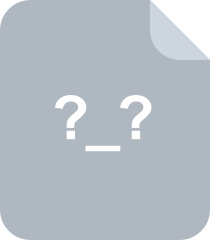
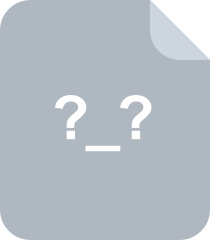
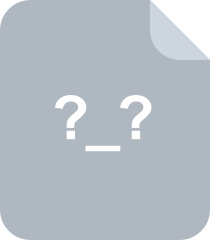
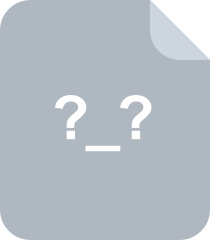
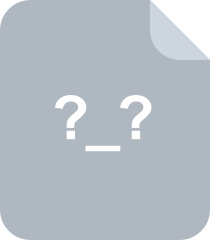
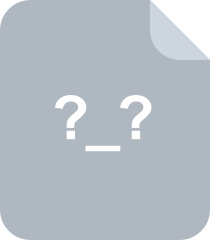
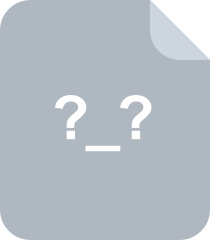
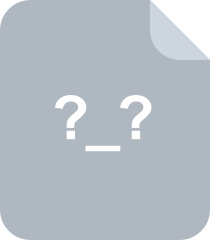
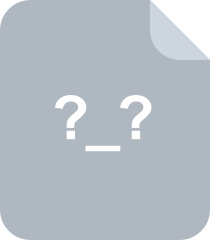
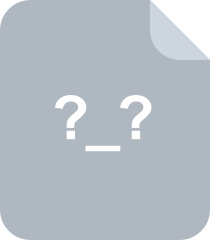
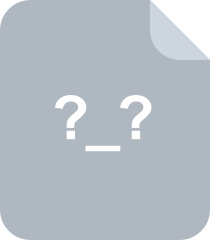
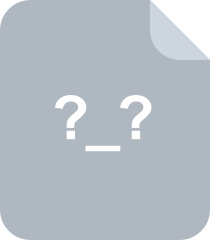
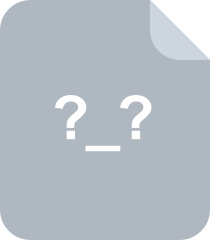
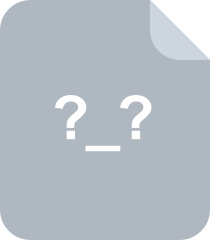
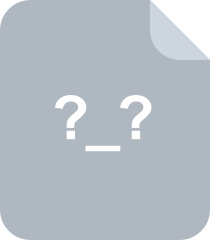
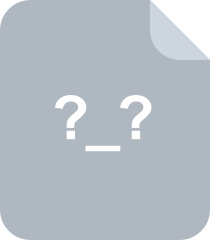
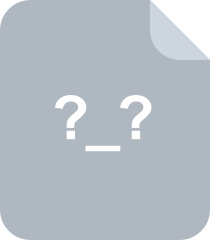
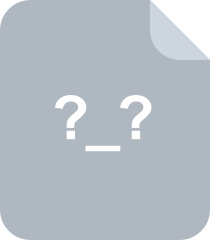
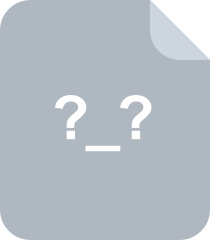
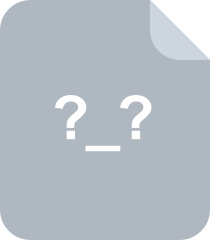
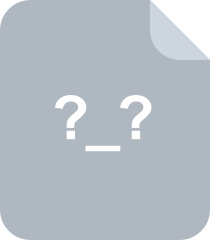
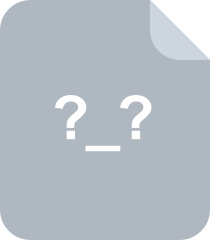
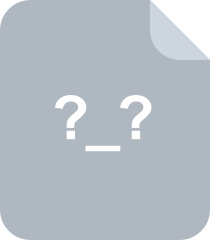
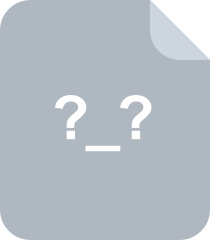
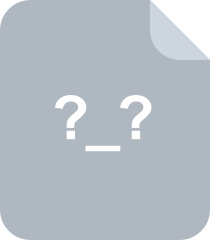
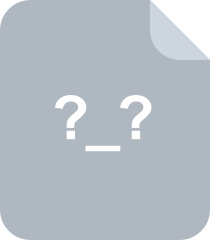
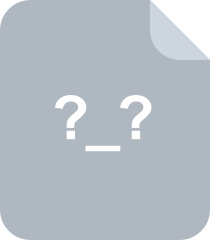
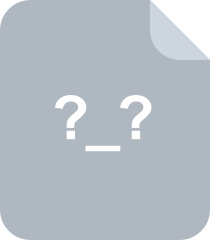
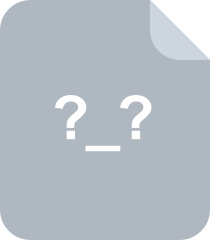
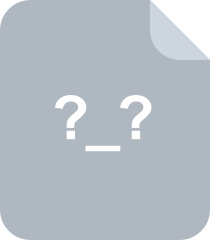
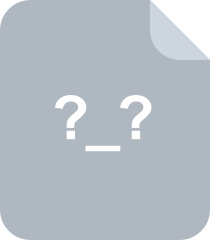
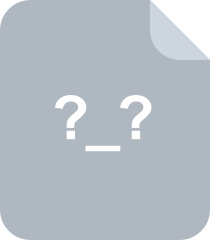
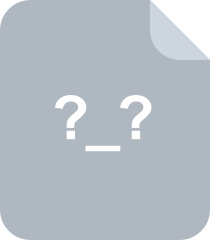
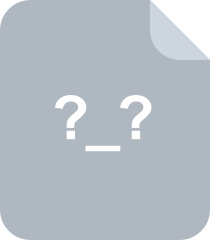
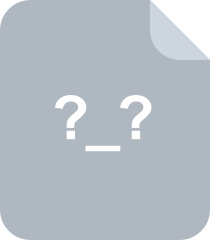
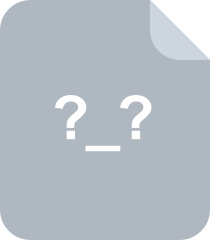
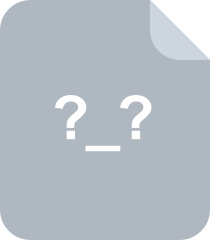
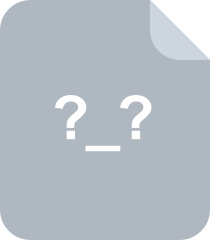
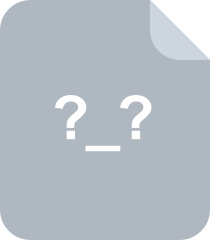
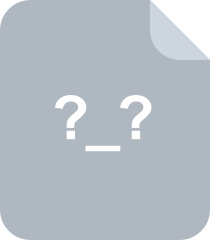
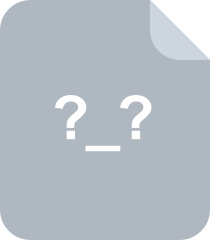
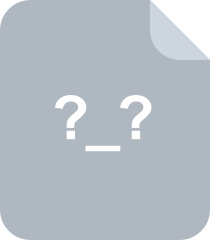
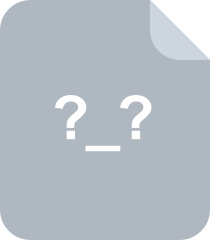
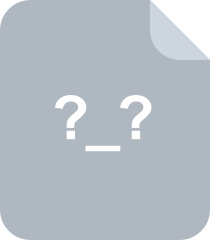
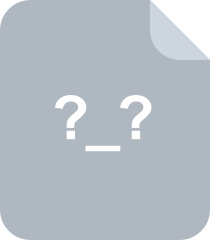
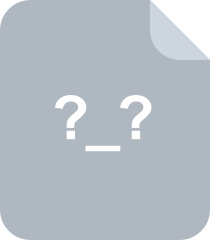
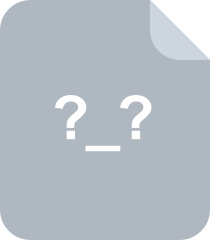
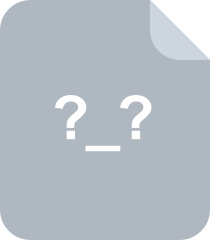
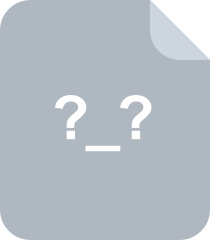
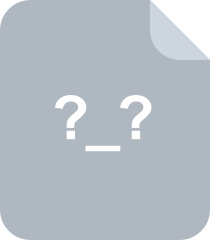
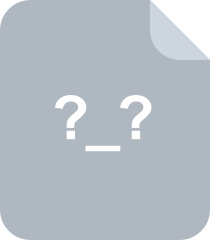
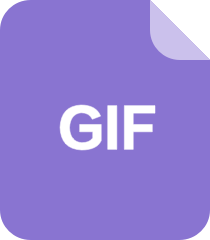
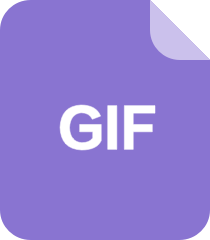
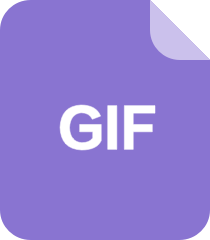
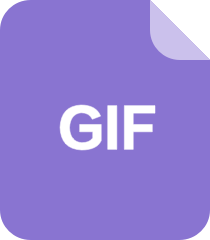
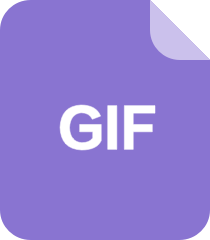
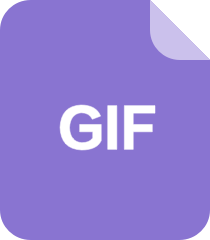
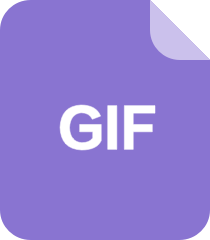
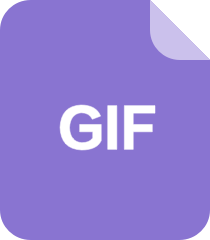
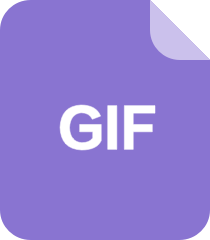
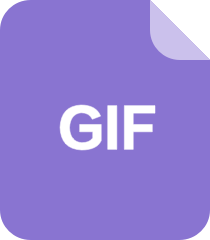
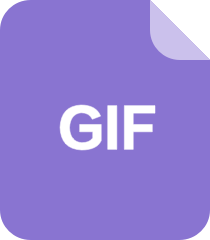
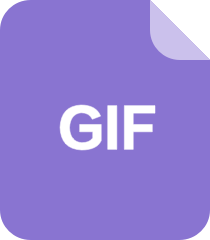
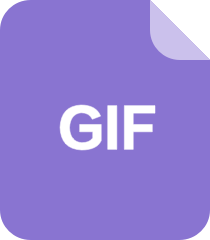
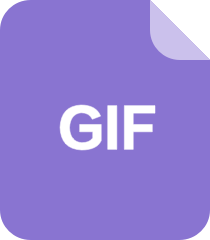
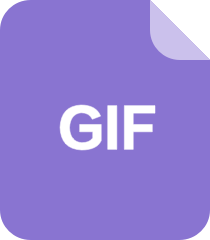
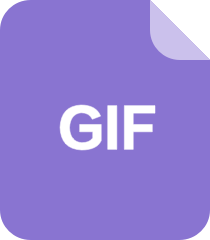
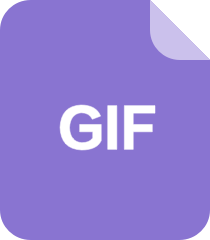
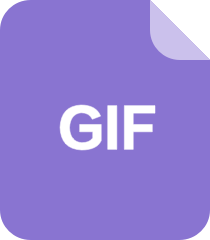
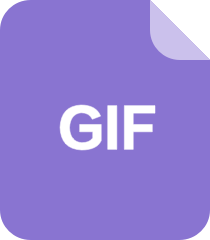
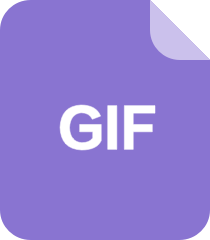
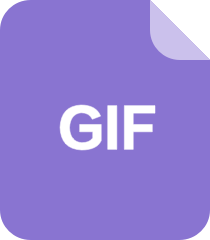
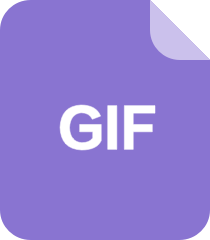
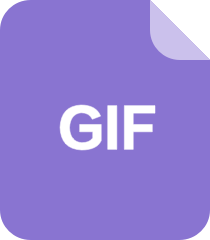
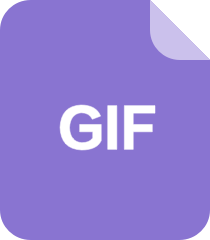
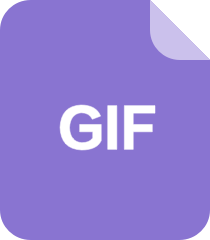
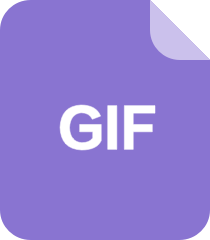
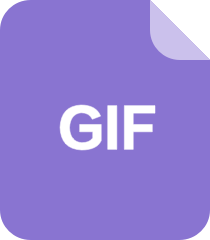
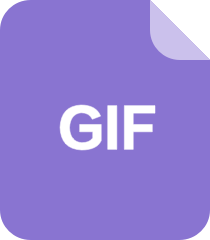
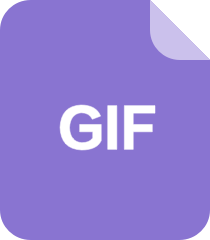
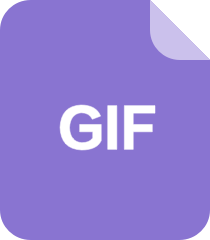
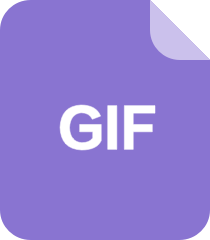
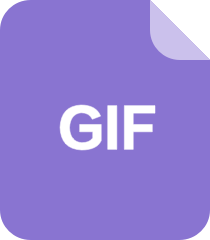
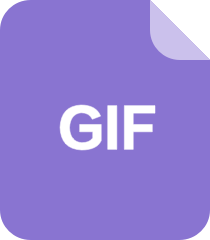
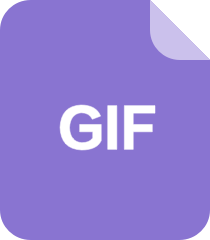
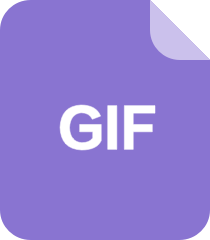
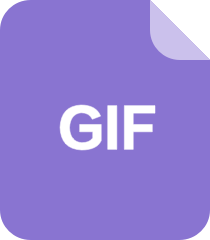
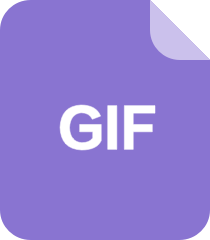
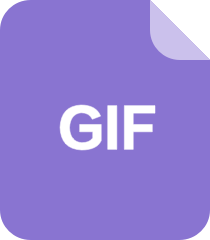
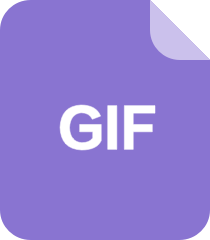
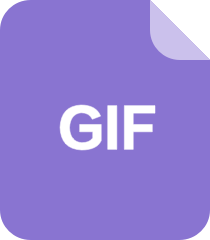
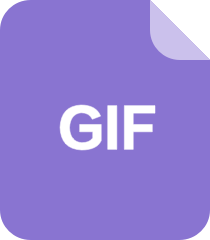
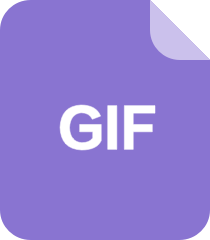
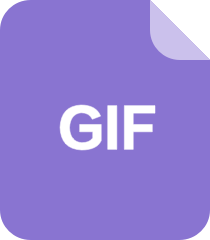
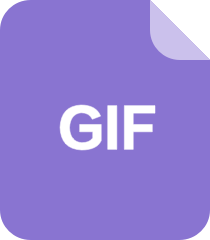
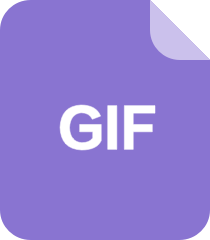
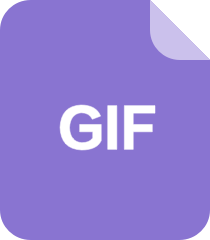
共 792 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
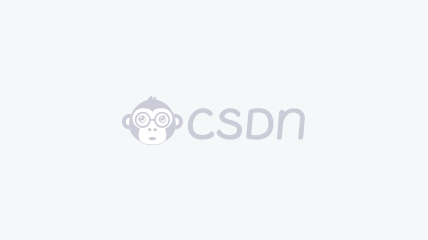

快乐无限出发
- 粉丝: 1200
- 资源: 7394
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

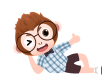
最新资源
- C#/WinForm演示退火算法(源码)
- 如何在 IntelliJ IDEA 中去掉 Java 方法注释后的空行.md
- 小程序官方组件库,内含各种组件实例,以及调用方式,多种UI可修改
- 2011年URL缩短服务JSON数据集
- Kaggle-Pokemon with stats(宠物小精灵数据)
- Harbor 最新v2.12.0的ARM64版离线安装包
- 【VUE网站静态模板】Uniapp 框架开发响应式网站,企业项目官网-APP,web网站,小程序快速生成 多语言:支持中文简体,中文繁体,英语
- 使用哈夫曼编码来对字符串进行编码HuffmanEncodingExample
- Ti芯片C2000内核手册
- c语言实现的花式爱心源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


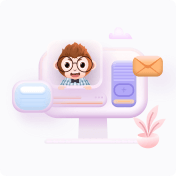
安全验证
文档复制为VIP权益,开通VIP直接复制
