/*
* Distributed as part of c3p0 v.0.9.2-pre3
*
* Copyright (C) 2012 Machinery For Change, Inc.
*
* Author: Steve Waldman <swaldman@mchange.com>
*
* This library is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License version 2.1, as
* published by the Free Software Foundation.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this software; see the file LICENSE. If not, write to the
* Free Software Foundation, Inc., 59 Temple Place, Suite 330,
* Boston, MA 02111-1307, USA.
*/
package com.mchange.v2.resourcepool;
import java.util.*;
import com.mchange.v2.async.*;
import com.mchange.v2.log.*;
import com.mchange.v2.lang.ThreadUtils;
import com.mchange.v2.util.ResourceClosedException;
class BasicResourcePool implements ResourcePool
{
private final static MLogger logger = MLog.getLogger( BasicResourcePool.class );
final static int AUTO_CULL_FREQUENCY_DIVISOR = 4;
final static int AUTO_MAX_CULL_FREQUENCY = (15 * 60 * 1000); //15 mins
final static int AUTO_MIN_CULL_FREQUENCY = (1 * 1000); //15 mins
//XXX: temporary -- for selecting between AcquireTask types
// remove soon, and use only ScatteredAcquireTask,
// presuming no problems appear
final static String USE_SCATTERED_ACQUIRE_TASK_KEY = "com.mchange.v2.resourcepool.experimental.useScatteredAcquireTask";
final static boolean USE_SCATTERED_ACQUIRE_TASK;
static
{
String checkScattered = com.mchange.v2.cfg.MultiPropertiesConfig.readVmConfig().getProperty(USE_SCATTERED_ACQUIRE_TASK_KEY);
if (checkScattered != null && checkScattered.trim().toLowerCase().equals("false"))
{
USE_SCATTERED_ACQUIRE_TASK = false;
if ( logger.isLoggable( MLevel.INFO ) )
logger.info(BasicResourcePool.class.getName() + " using traditional, Thread-blocking AcquireTask. Yuk. Why?");
}
else
USE_SCATTERED_ACQUIRE_TASK = true;
}
// end temporary switch between acquire task types
//MT: unchanged post c'tor
final Manager mgr;
final int start;
final int min;
final int max;
final int inc;
final int num_acq_attempts;
final int acq_attempt_delay;
final long check_idle_resources_delay; //milliseconds
final long max_resource_age; //milliseconds
final long max_idle_time; //milliseconds
final long excess_max_idle_time; //milliseconds
final long destroy_unreturned_resc_time; //milliseconds
final long expiration_enforcement_delay; //milliseconds
final boolean break_on_acquisition_failure;
final boolean debug_store_checkout_exceptions;
final long pool_start_time = System.currentTimeMillis();
//MT: not-reassigned, thread-safe, and independent
final BasicResourcePoolFactory factory;
final AsynchronousRunner taskRunner;
final RunnableQueue asyncEventQueue;
final ResourcePoolEventSupport rpes;
//MT: protected by this' lock
Timer cullAndIdleRefurbishTimer;
TimerTask cullTask;
TimerTask idleRefurbishTask;
HashSet acquireWaiters = new HashSet();
HashSet otherWaiters = new HashSet();
int pending_acquires;
int pending_removes;
int target_pool_size;
/* keys are all valid, managed resources, value is a PunchCard */
HashMap managed = new HashMap();
/* all valid, managed resources currently available for checkout */
LinkedList unused = new LinkedList();
/* resources which have been invalidated somehow, but which are */
/* still checked out and in use. */
HashSet excluded = new HashSet();
Map formerResources = new WeakHashMap();
Set idleCheckResources = new HashSet();
boolean force_kill_acquires = false;
boolean broken = false;
// long total_acquired = 0;
long failed_checkins = 0;
long failed_checkouts = 0;
long failed_idle_tests = 0;
Throwable lastCheckinFailure = null;
Throwable lastCheckoutFailure = null;
Throwable lastIdleTestFailure = null;
Throwable lastResourceTestFailure = null;
Throwable lastAcquisitionFailiure = null;
//DEBUG only!
Object exampleResource;
public long getStartTime()
{ return pool_start_time; }
public long getUpTime()
{ return System.currentTimeMillis() - pool_start_time; }
public synchronized long getNumFailedCheckins()
{ return failed_checkins; }
public synchronized long getNumFailedCheckouts()
{ return failed_checkouts; }
public synchronized long getNumFailedIdleTests()
{ return failed_idle_tests; }
public synchronized Throwable getLastCheckinFailure()
{ return lastCheckinFailure; }
//must be called from a pre-existing sync'ed block
private void setLastCheckinFailure(Throwable t)
{
assert ( Thread.holdsLock(this));
this.lastCheckinFailure = t;
this.lastResourceTestFailure = t;
}
public synchronized Throwable getLastCheckoutFailure()
{ return lastCheckoutFailure; }
//must be called from a pre-existing sync'ed block
private void setLastCheckoutFailure(Throwable t)
{
assert ( Thread.holdsLock(this));
this.lastCheckoutFailure = t;
this.lastResourceTestFailure = t;
}
public synchronized Throwable getLastIdleCheckFailure()
{ return lastIdleTestFailure; }
//must be called from a pre-existing sync'ed block
private void setLastIdleCheckFailure(Throwable t)
{
assert ( Thread.holdsLock(this));
this.lastIdleTestFailure = t;
this.lastResourceTestFailure = t;
}
public synchronized Throwable getLastResourceTestFailure()
{ return lastResourceTestFailure; }
public synchronized Throwable getLastAcquisitionFailure()
{ return lastAcquisitionFailiure; }
// ought not be called while holding this' lock
private synchronized void setLastAcquisitionFailure( Throwable t )
{ this.lastAcquisitionFailiure = t; }
public synchronized int getNumCheckoutWaiters()
{ return acquireWaiters.size(); }
public synchronized int getNumPendingAcquireTasks()
{ return pending_acquires; }
public synchronized int getNumPendingRemoveTasks()
{ return pending_removes; }
public synchronized int getNumThreadsWaitingForResources()
{ return acquireWaiters.size(); }
public synchronized String[] getThreadNamesWaitingForResources()
{
int len = acquireWaiters.size();
String[] out = new String[len];
int i = 0;
for (Iterator ii = acquireWaiters.iterator(); ii.hasNext(); )
out[i++] = ((Thread) ii.next()).getName();
Arrays.sort( out );
return out;
}
public synchronized int getNumThreadsWaitingForAdministrativeTasks()
{ return otherWaiters.size(); }
public synchronized String[] getThreadNamesWaitingForAdministrativeTasks()
{
int len = otherWaiters.size();
String[] out = new String[len];
int i = 0;
for (Iterator ii = otherWaiters.iterator(); ii.hasNext(); )
out[i++] = ((Thread) ii.next()).getName();
Arrays.sort( out );
return out;
}
private void addToFormerResources( Object resc )
{ formerResources.put( resc, null ); }
private boolean isFormerResource( Object resc )
{ return formerResources.keySet().contains( resc ); }
/**
* @param factory may be null
*/
public BasicResourcePool(Manager mgr,
int start,
int min,
没有合适的资源?快使用搜索试试~ 我知道了~
基于java的开发源码-数据库连接池 C3P0.src.zip
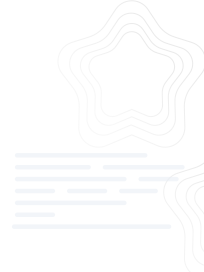
共178个文件
java:117个
html:25个
xml:8个

0 下载量 161 浏览量
2024-02-29
21:07:06
上传
评论
收藏 895KB ZIP 举报
温馨提示
基于java的开发源码-数据库连接池 C3P0.src.zip 基于java的开发源码-数据库连接池 C3P0.src.zip 基于java的开发源码-数据库连接池 C3P0.src.zip 基于java的开发源码-数据库连接池 C3P0.src.zip 基于java的开发源码-数据库连接池 C3P0.src.zip 基于java的开发源码-数据库连接池 C3P0.src.zip 基于java的开发源码-数据库连接池 C3P0.src.zip 基于java的开发源码-数据库连接池 C3P0.src.zip 基于java的开发源码-数据库连接池 C3P0.src.zip 基于java的开发源码-数据库连接池 C3P0.src.zip 基于java的开发源码-数据库连接池 C3P0.src.zip
资源推荐
资源详情
资源评论
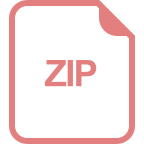
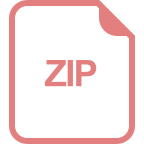
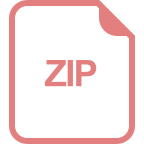
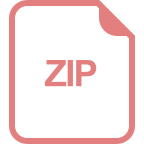
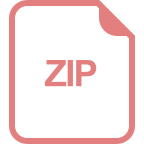
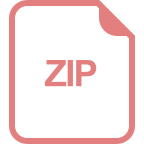
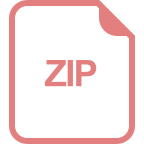
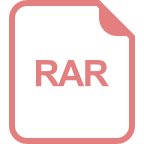
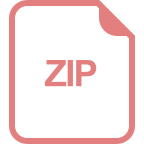
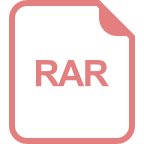
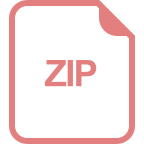
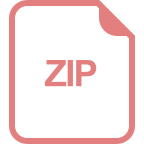
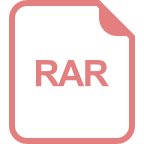
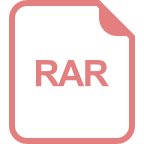
收起资源包目录

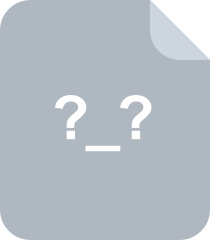
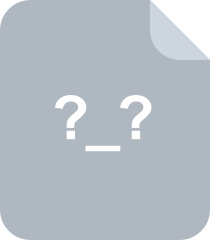
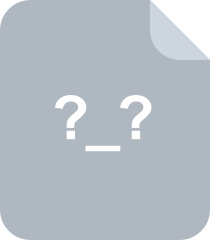
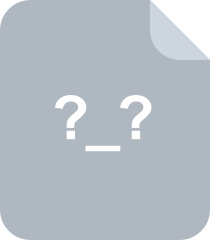
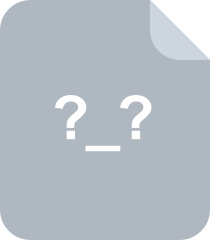
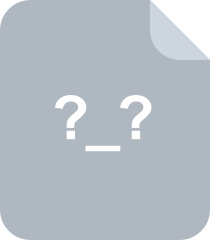
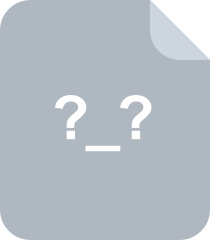
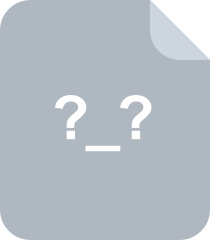
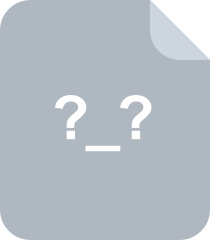
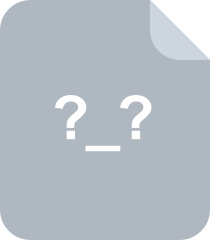
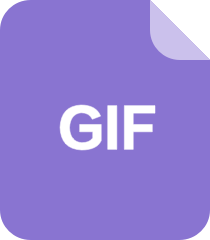
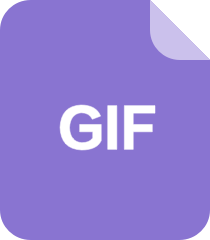
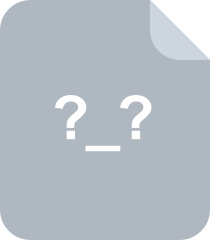
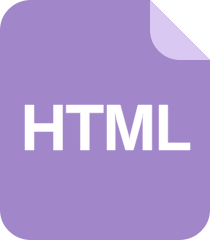
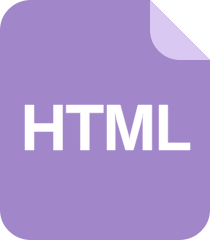
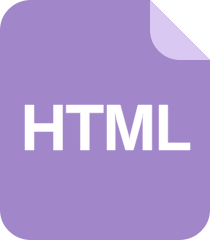
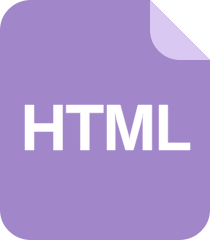
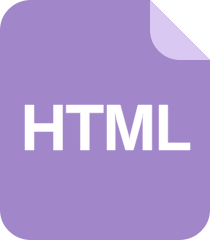
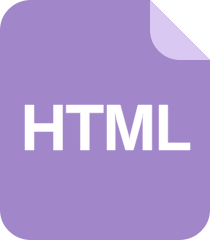
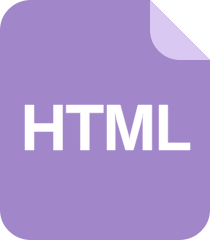
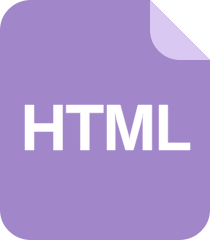
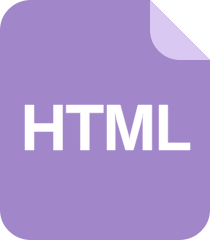
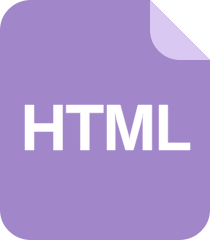
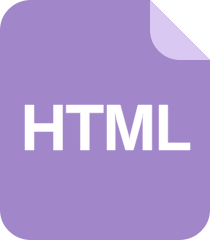
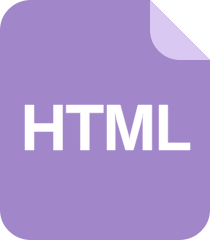
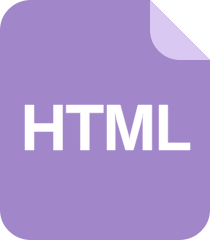
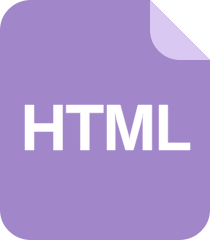
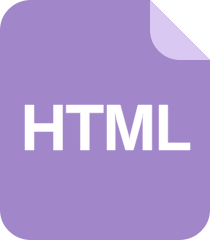
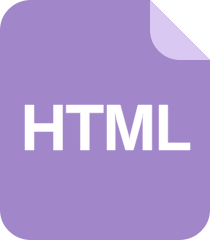
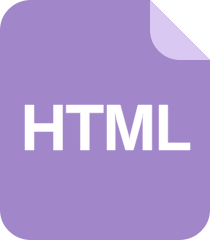
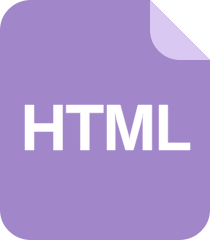
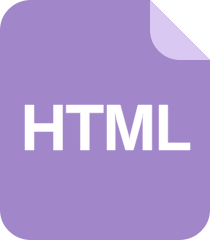
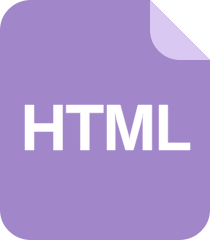
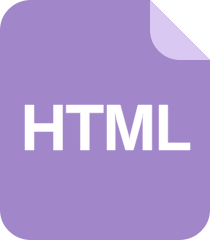
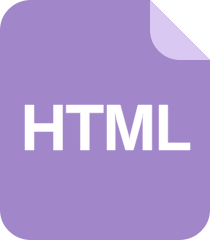
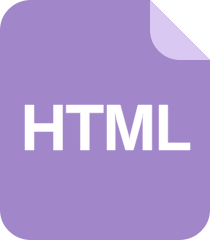
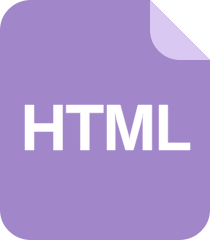
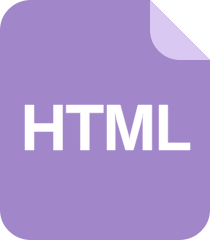
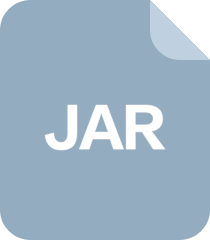
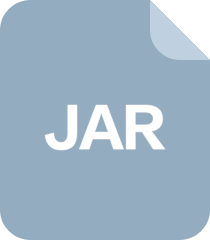
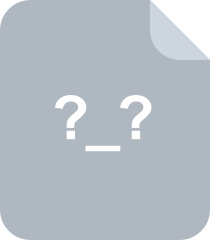
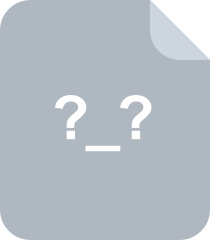
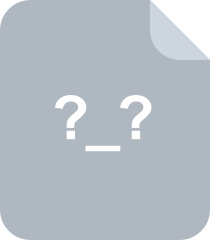
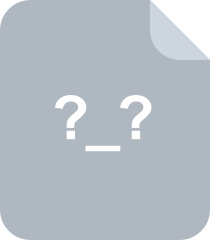
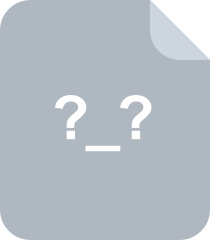
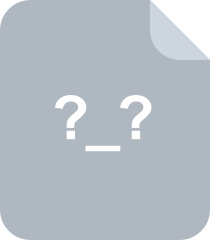
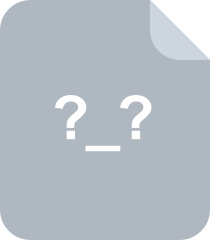
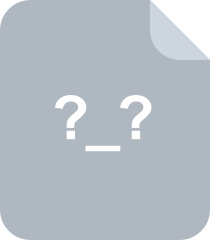
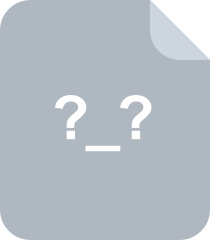
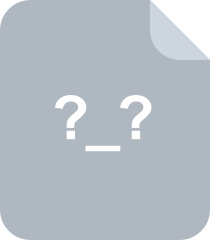
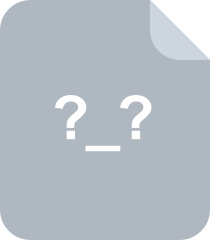
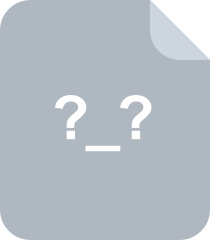
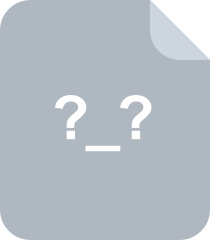
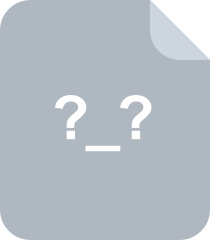
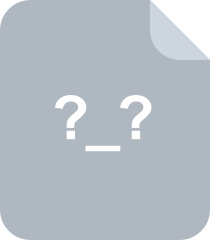
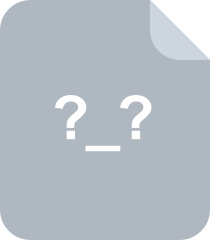
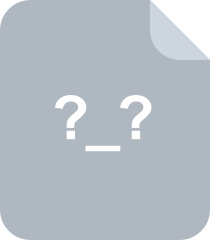
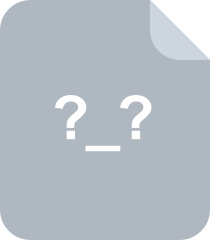
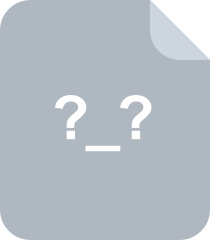
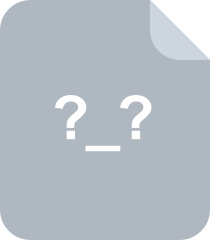
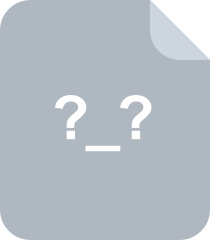
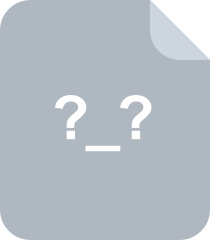
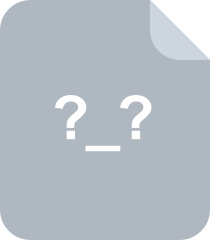
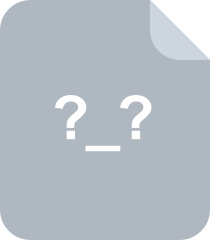
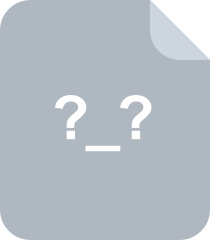
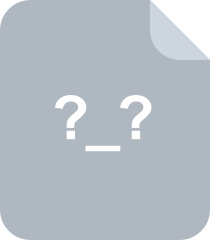
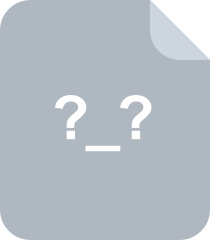
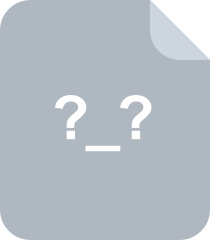
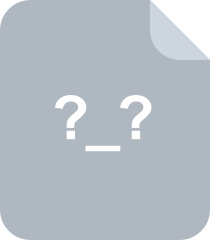
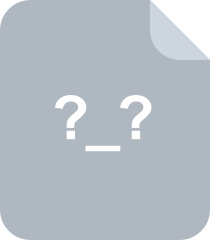
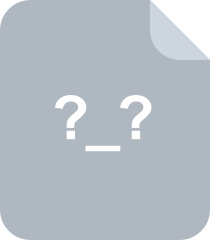
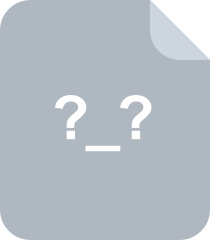
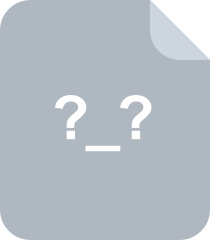
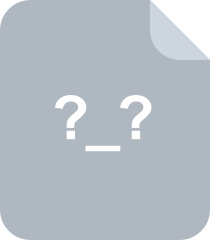
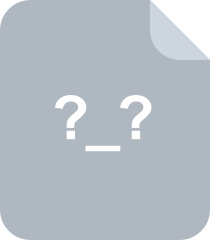
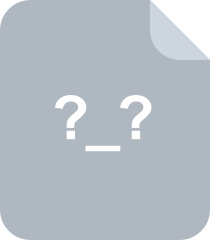
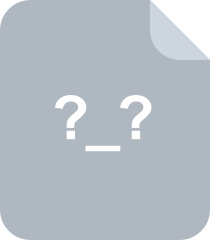
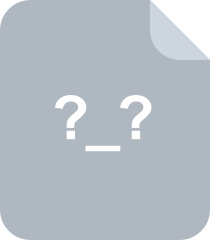
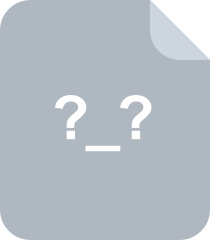
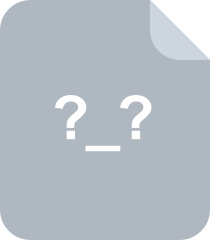
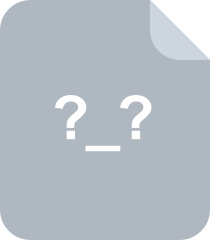
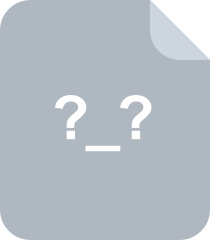
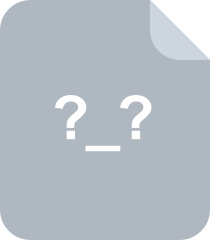
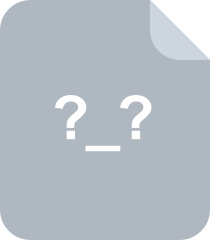
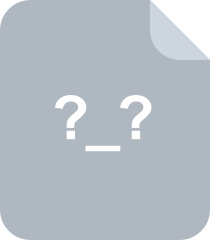
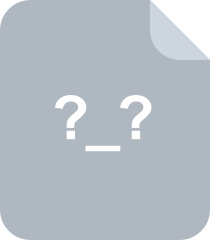
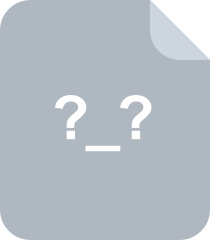
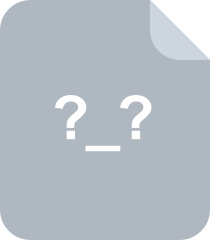
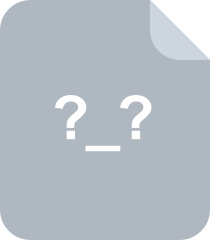
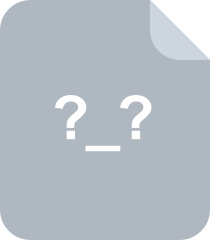
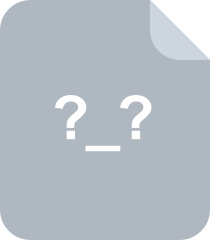
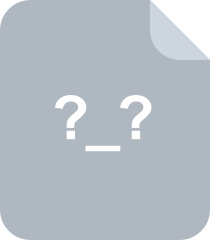
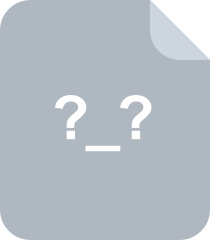
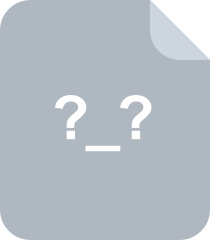
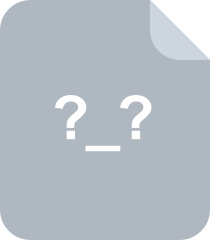
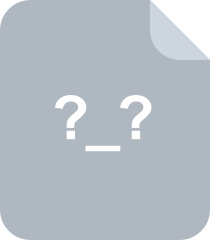
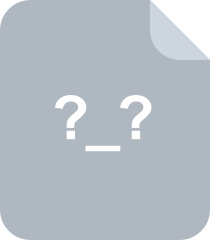
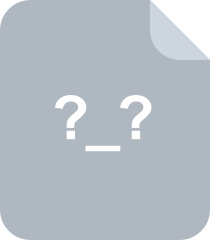
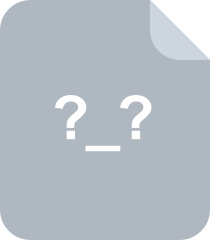
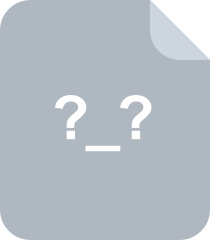
共 178 条
- 1
- 2
资源评论
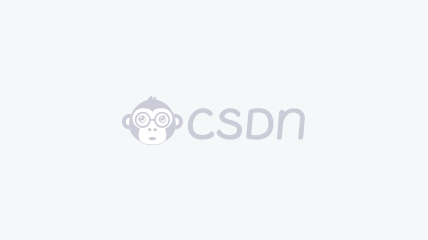

快乐无限出发
- 粉丝: 1200
- 资源: 7394
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

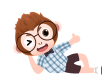
最新资源
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
- (源码)基于Java和JSP的校园论坛系统.zip
- (源码)基于ROS Kinetic框架的AGV激光雷达导航与SLAM系统.zip
- (源码)基于PythonDjango框架的资产管理系统.zip
- (源码)基于计算机系统原理与Arduino技术的学习平台.zip
- (源码)基于SSM框架的大学消息通知系统服务端.zip
- (源码)基于Java Servlet的学生信息管理系统.zip
- (源码)基于Qt和AVR的FestosMechatronics系统终端.zip
- (源码)基于Java的DVD管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


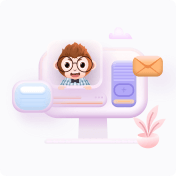
安全验证
文档复制为VIP权益,开通VIP直接复制
