<?php
/*
* Copyright (c) 2003-2012, CKSource - Frederico Knabben. All rights reserved.
* For licensing, see LICENSE.html or http://ckeditor.com/license
*/
/**
* \brief CKEditor class that can be used to create editor
* instances in PHP pages on server side.
* @see http://ckeditor.com
*
* Sample usage:
* @code
* $CKEditor = new CKEditor();
* $CKEditor->editor("editor1", "<p>Initial value.</p>");
* @endcode
*/
class CKEditor
{
/**
* The version of %CKEditor.
* \private
*/
var $version = '3.6.3';
/**
* A constant string unique for each release of %CKEditor.
* \private
*/
var $_timestamp = 'C3HA5RM';
/**
* URL to the %CKEditor installation directory (absolute or relative to document root).
* If not set, CKEditor will try to guess it's path.
*
* Example usage:
* @code
* $CKEditor->basePath = '/ckeditor/';
* @endcode
*/
var $basePath;
/**
* An array that holds the global %CKEditor configuration.
* For the list of available options, see http://docs.cksource.com/ckeditor_api/symbols/CKEDITOR.config.html
*
* Example usage:
* @code
* $CKEditor->config['height'] = 400;
* // Use @@ at the beggining of a string to ouput it without surrounding quotes.
* $CKEditor->config['width'] = '@@screen.width * 0.8';
* @endcode
*/
var $config = array();
/**
* A boolean variable indicating whether CKEditor has been initialized.
* Set it to true only if you have already included
* <script> tag loading ckeditor.js in your website.
*/
var $initialized = false;
/**
* Boolean variable indicating whether created code should be printed out or returned by a function.
*
* Example 1: get the code creating %CKEditor instance and print it on a page with the "echo" function.
* @code
* $CKEditor = new CKEditor();
* $CKEditor->returnOutput = true;
* $code = $CKEditor->editor("editor1", "<p>Initial value.</p>");
* echo "<p>Editor 1:</p>";
* echo $code;
* @endcode
*/
var $returnOutput = false;
/**
* An array with textarea attributes.
*
* When %CKEditor is created with the editor() method, a HTML <textarea> element is created,
* it will be displayed to anyone with JavaScript disabled or with incompatible browser.
*/
var $textareaAttributes = array( "rows" => 8, "cols" => 60 );
/**
* A string indicating the creation date of %CKEditor.
* Do not change it unless you want to force browsers to not use previously cached version of %CKEditor.
*/
var $timestamp = "C3HA5RM";
/**
* An array that holds event listeners.
* \private
*/
var $_events = array();
/**
* An array that holds global event listeners.
* \private
*/
var $_globalEvents = array();
/**
* Main Constructor.
*
* @param $basePath (string) URL to the %CKEditor installation directory (optional).
*/
function CKEditor($basePath = null) {
if (!empty($basePath)) {
$this->basePath = $basePath;
}
}
/**
* Creates a %CKEditor instance.
* In incompatible browsers %CKEditor will downgrade to plain HTML <textarea> element.
*
* @param $name (string) Name of the %CKEditor instance (this will be also the "name" attribute of textarea element).
* @param $value (string) Initial value (optional).
* @param $config (array) The specific configurations to apply to this editor instance (optional).
* @param $events (array) Event listeners for this editor instance (optional).
*
* Example usage:
* @code
* $CKEditor = new CKEditor();
* $CKEditor->editor("field1", "<p>Initial value.</p>");
* @endcode
*
* Advanced example:
* @code
* $CKEditor = new CKEditor();
* $config = array();
* $config['toolbar'] = array(
* array( 'Source', '-', 'Bold', 'Italic', 'Underline', 'Strike' ),
* array( 'Image', 'Link', 'Unlink', 'Anchor' )
* );
* $events['instanceReady'] = 'function (ev) {
* alert("Loaded: " + ev.editor.name);
* }';
* $CKEditor->editor("field1", "<p>Initial value.</p>", $config, $events);
* @endcode
*/
function editor($name, $value = "", $config = array(), $events = array())
{
$attr = "";
foreach ($this->textareaAttributes as $key => $val) {
$attr.= " " . $key . '="' . str_replace('"', '"', $val) . '"';
}
$out = "<textarea name=\"" . $name . "\"" . $attr . ">" . htmlspecialchars($value) . "</textarea>\n";
if (!$this->initialized) {
$out .= $this->init();
}
$_config = $this->configSettings($config, $events);
$js = $this->returnGlobalEvents();
if (!empty($_config))
$js .= "CKEDITOR.replace('".$name."', ".$this->jsEncode($_config).");";
else
$js .= "CKEDITOR.replace('".$name."');";
$out .= $this->script($js);
if (!$this->returnOutput) {
print $out;
$out = "";
}
return $out;
}
/**
* Replaces a <textarea> with a %CKEditor instance.
*
* @param $id (string) The id or name of textarea element.
* @param $config (array) The specific configurations to apply to this editor instance (optional).
* @param $events (array) Event listeners for this editor instance (optional).
*
* Example 1: adding %CKEditor to <textarea name="article"></textarea> element:
* @code
* $CKEditor = new CKEditor();
* $CKEditor->replace("article");
* @endcode
*/
function replace($id, $config = array(), $events = array())
{
$out = "";
if (!$this->initialized) {
$out .= $this->init();
}
$_config = $this->configSettings($config, $events);
$js = $this->returnGlobalEvents();
if (!empty($_config)) {
$js .= "CKEDITOR.replace('".$id."', ".$this->jsEncode($_config).");";
}
else {
$js .= "CKEDITOR.replace('".$id."');";
}
$out .= $this->script($js);
if (!$this->returnOutput) {
print $out;
$out = "";
}
return $out;
}
/**
* Replace all <textarea> elements available in the document with editor instances.
*
* @param $className (string) If set, replace all textareas with class className in the page.
*
* Example 1: replace all <textarea> elements in the page.
* @code
* $CKEditor = new CKEditor();
* $CKEditor->replaceAll();
* @endcode
*
* Example 2: replace all <textarea class="myClassName"> elements in the page.
* @code
* $CKEditor = new CKEditor();
* $CKEditor->replaceAll( 'myClassName' );
* @endcode
*/
function replaceAll($className = null)
{
$out = "";
if (!$this->initialized) {
$out .= $this->init();
}
$_config = $this->configSettings();
$js = $this->returnGlobalEvents();
if (empty($_config)) {
if (empty($className)) {
$js .= "CKEDITOR.replaceAll();";
}
else {
$js .= "CKEDITOR.replaceAll('".$className."');";
}
}
else {
$classDetection = "";
$js .= "CKEDITOR.replaceAll( function(textarea, config) {\n";
if (!empty($className)) {
$js .= " var classRegex = new RegExp('(?:^| )' + '". $className ."' + '(?:$| )');\n";
$js .= " if (!classRegex.test(textarea.className))\n";
$js .= " return false;\n";
}
$js .= " CKEDITOR.tools.extend(config, ". $this->jsEncode($_config) .", true);";
$js .= "} );";
}
$out .= $this->script($js);
if (!$this->returnOutput) {
print $out;
$out = "";
}
return $out;
}
/**
* Adds event listener.
* Events are fired by %CKEditor in various situations.
*
* @param $event (string) Event name.
* @param $javascriptCode (string) Javascript anonymous function or function name.
*
* Example usage:
* @code
* $CKEditor->addEventHandler('instanceReady', 'function (ev) {
* alert("Loaded: " + ev.editor.name);
* }');
* @endcode
*/
function addEventHandler($event, $javascriptCode)
{
if (!isset($this->_events[$event])) {
$this->_events[$event] = array();
}
// Avoid duplicates.
i
没有合适的资源?快使用搜索试试~ 我知道了~
asp源码-CuzCms成创企业网站内容管理系统 asp版 v2.1.zip
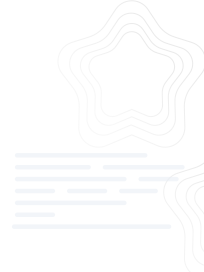
共1632个文件
js:675个
gif:340个
css:144个

1 下载量 64 浏览量
2024-02-12
10:50:34
上传
评论
收藏 7.13MB ZIP 举报
温馨提示
anaconda安装 asp源码—CuzCms成创企业网站内容管理系统 asp版 v2.1.zip asp源码—CuzCms成创企业网站内容管理系统 asp版 v2.1.zip asp源码—CuzCms成创企业网站内容管理系统 asp版 v2.1.zip asp源码—CuzCms成创企业网站内容管理系统 asp版 v2.1.zip asp源码—CuzCms成创企业网站内容管理系统 asp版 v2.1.zip asp源码—CuzCms成创企业网站内容管理系统 asp版 v2.1.zip asp源码—CuzCms成创企业网站内容管理系统 asp版 v2.1.zip
资源推荐
资源详情
资源评论
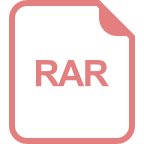
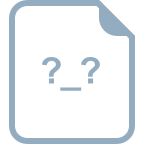
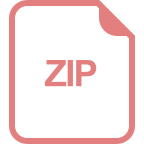
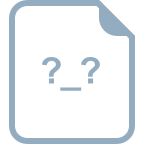
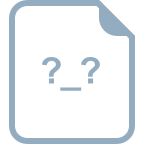
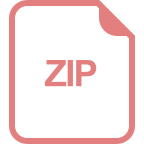
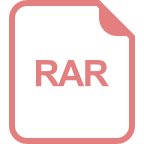
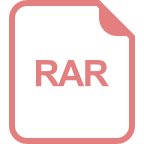
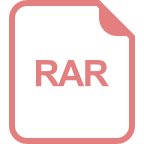
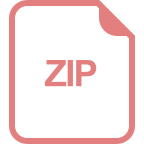
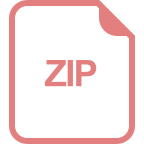
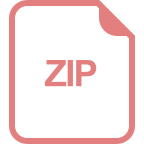
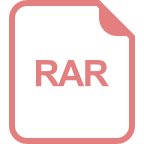
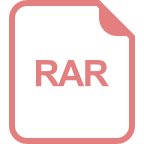
收起资源包目录

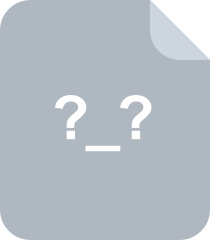
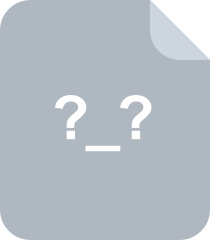
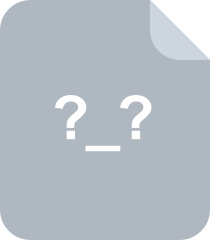
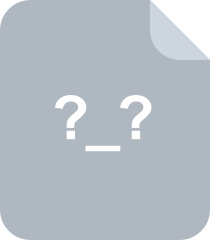
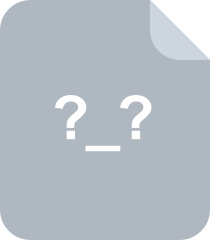
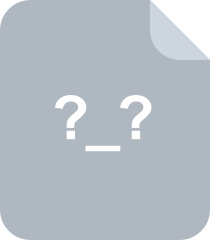
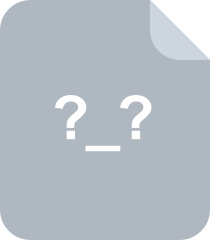
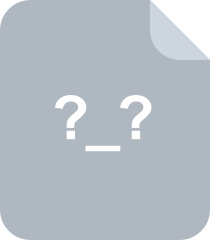
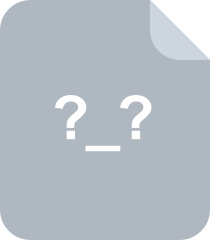
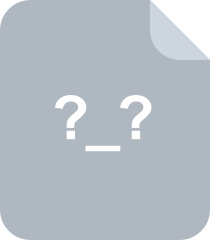
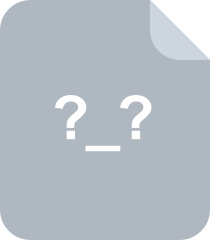
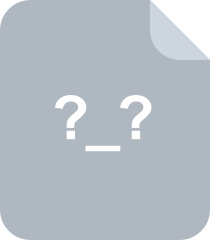
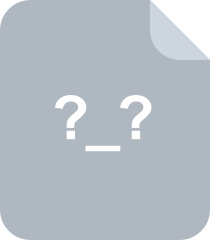
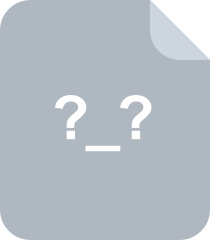
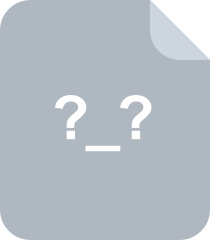
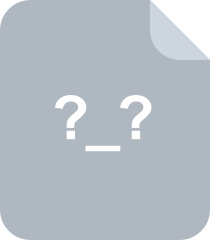
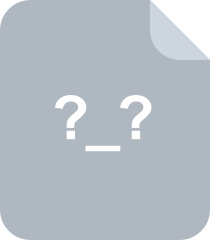
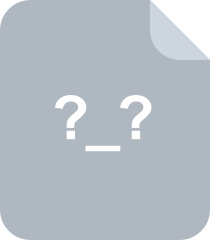
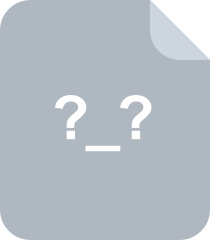
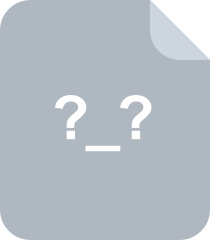
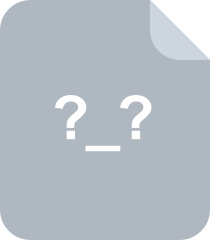
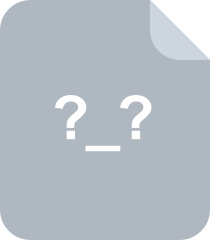
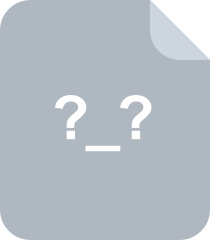
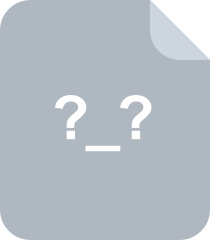
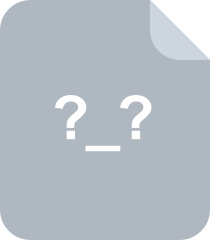
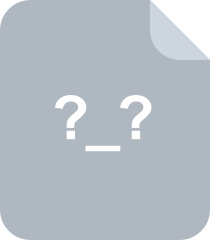
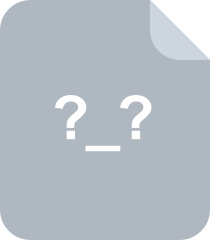
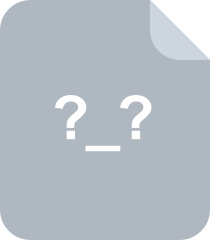
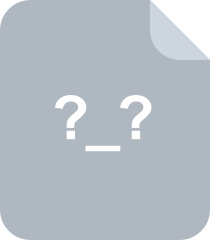
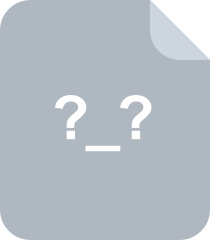
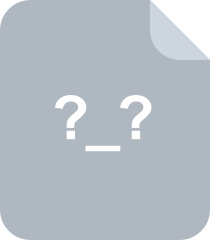
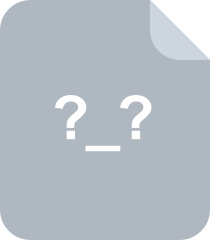
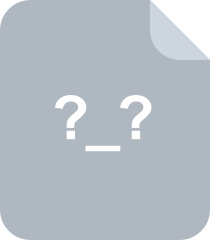
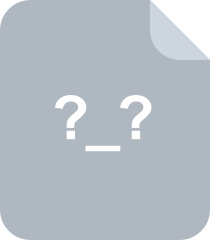
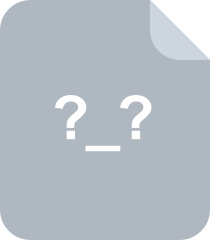
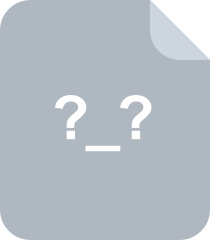
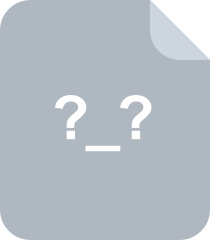
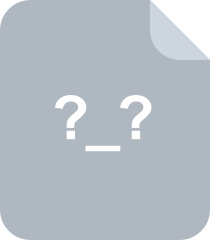
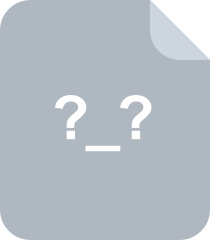
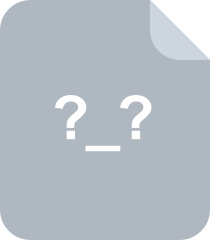
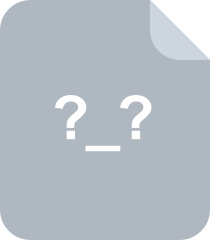
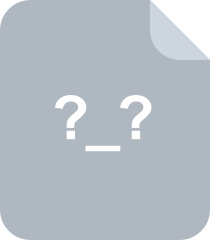
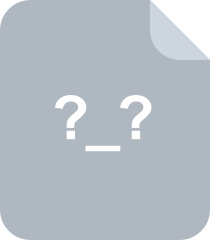
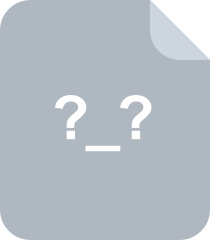
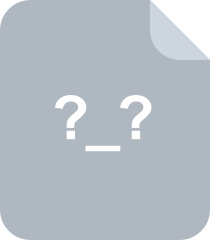
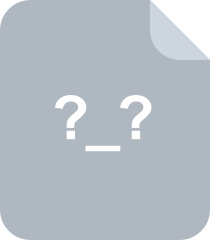
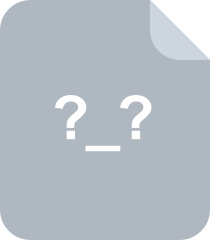
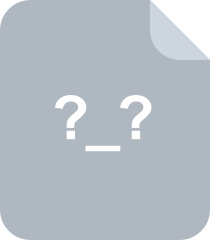
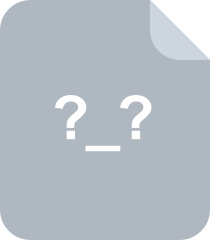
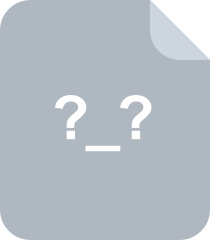
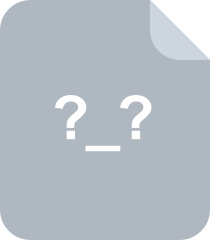
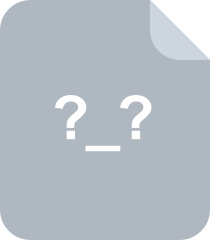
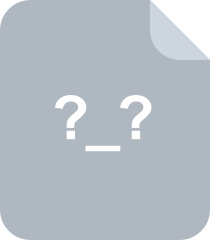
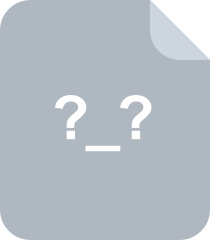
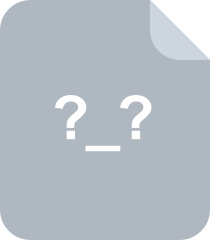
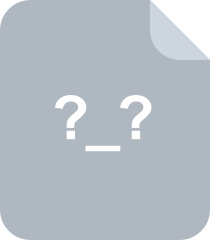
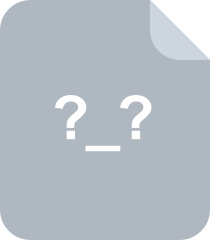
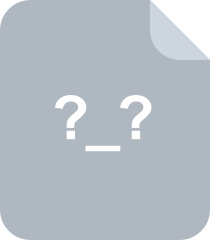
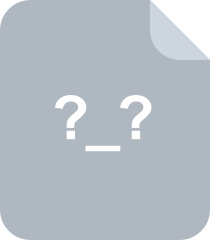
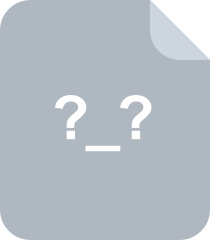
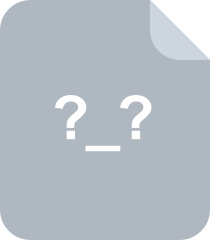
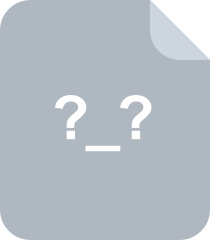
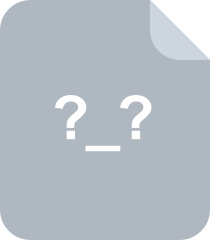
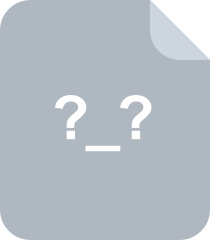
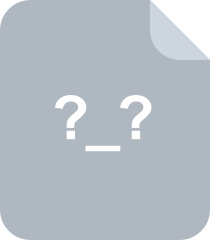
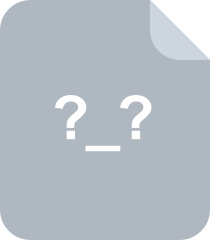
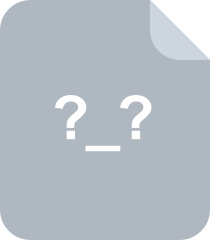
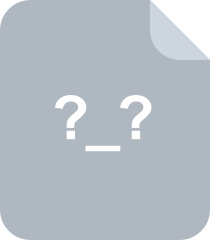
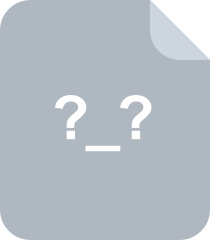
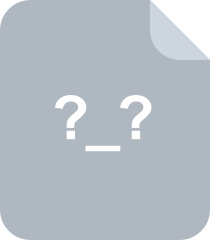
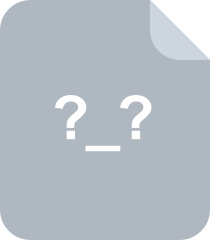
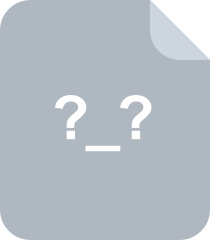
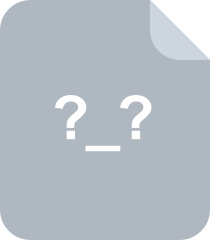
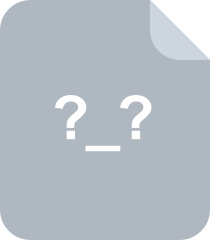
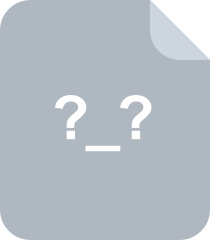
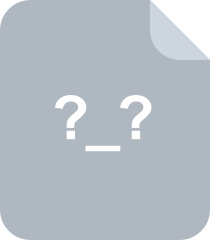
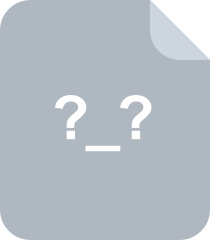
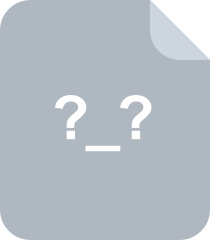
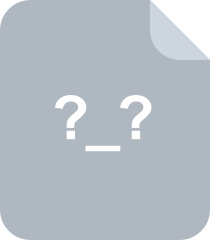
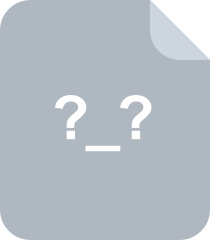
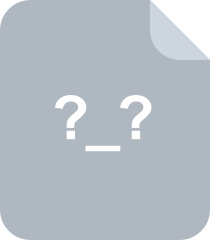
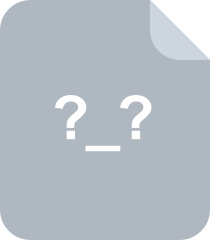
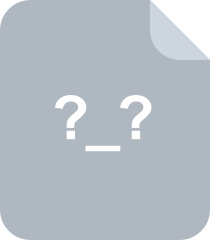
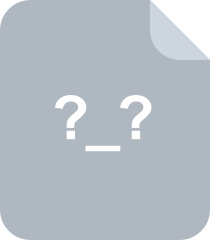
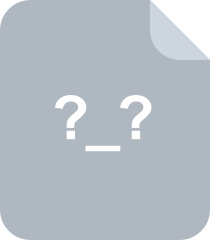
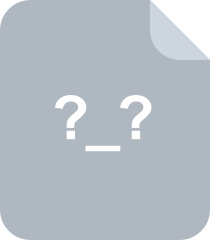
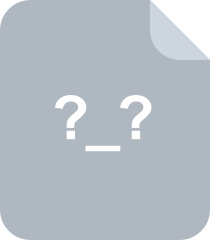
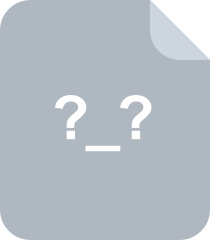
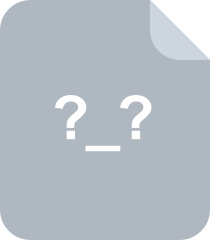
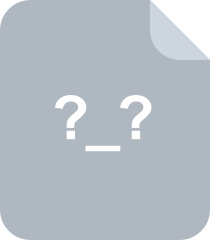
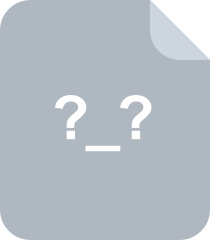
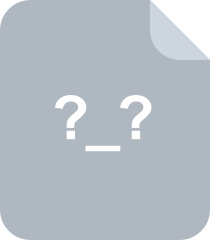
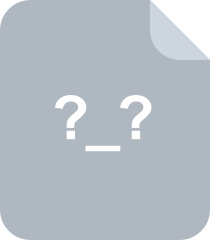
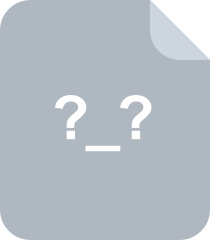
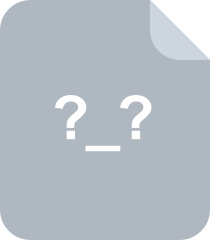
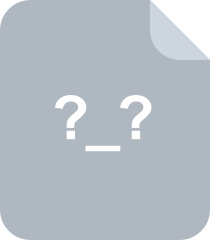
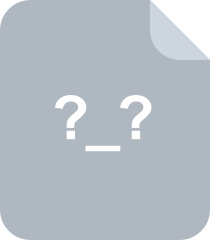
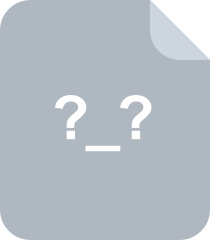
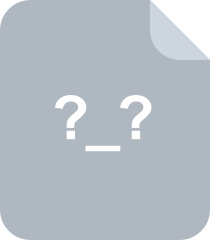
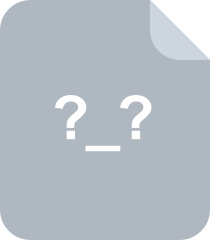
共 1632 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
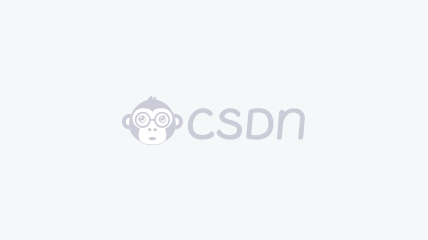

快乐无限出发
- 粉丝: 1206
- 资源: 7390
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

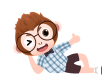
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


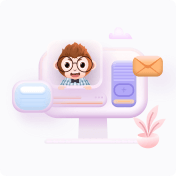
安全验证
文档复制为VIP权益,开通VIP直接复制
