//===========================================================================
// Testcases for FilterPushDown
// It tests pushing Filter below Transform, Join, Group By, Sort, Merge
// It handles predicate decomposition (Dividing Filter predicates into conjunctive sub-predicates)
//===========================================================================
$data_books = [
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Deathly Hallows',
year: 2007,
format: {cover: 'hard', pages: 1302},
price: [3, 34.0, 102222, 3.3]},
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Chamber of Secrets',
year: 1999,
format: {cover: 'hard', pages: 1302}},
{publisher: 'XYZ',
author: 'J. K. Rowling',
title: 'Deathly Hallows',
year: 1998,
format: {cover: 'soft', pages: 1200},
price: [120.5, 110.0]},
{publisher: 'Ray ST',
author: 'New Rowling',
title: 'Chamber of Secrets',
year: 1998,
reviews: [{rating: 10, user: 'joe', review: 'The best ...'}]},
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Sorcerers Stone',
year: 1996,
format: {cover: 'hard', pages: 742},
price: [11.0, 17.5]},
{publisher: 'Scholastic',
author: 'R. L. Stine',
title: 'Monster Blood IV',
year: 1995,
format: {cover: 'soft', pages: 302}},
{publisher: 'Grosset',
author: 'Carolyn Keene',
title: 'The Secret of Kane',
year: 1988},
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Deathly Hallows',
year: 1987},
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Chamber of Secrets',
year: 1987,
reviews: [
{rating: 10, user: 'joe', review: 'The best ...'},
{rating: 6, user: 'mary', review: 'Average ...'}]},
{publisher: 'XYZ',
author: 'J. K. Rowling',
title: 'Deathly Hallows',
year: 1986},
{publisher: 'Foster',
author: 'Old Rowling',
title: 'Chamber of Secrets',
year: 1979,
reviews: [{rating: 10, user: 'joe', review: 'The best ...'}]},
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Sorcerers Stone',
year: 1978},
{publisher: 'Scholastic',
author: 'R. L. Stine',
title: 'Monster Blood IV',
year: 1977,
price: [3.6, 20.5, 41.0]
},
{publisher: 'Grosset',
author: 'Carolyn Keene',
title: 'The Secret of Kane',
year: 1920,
format: {cover: 'hard', pages: 66}}
];
$data_books2 = [
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Deathly Hallows',
year: 2007,
format: {cover: 'hard', pages: 1302},
price: [30.5, 33.5, 34.0]},
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Chamber of Secrets',
year: 1999,
format: {cover: 'hard', pages: 1302},
price: [20.1, 25.0]
},
{publisher: 'XYZ',
author: 'J. K. Rowling',
title: 'Deathly Hallows',
year: 1998,
format: {cover: 'soft', pages: 1200},
price: [120.5, 110.0]},
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Sorcerers Stone',
year: 1996,
format: {cover: 'hard', pages: 742},
price: [11.0, 17.5]},
{publisher: 'Scholastic',
author: 'R. L. Stine',
title: 'Monster Blood IV',
year: 1995,
format: {cover: 'soft', pages: 302}},
{publisher: 'Grosset',
author: 'Carolyn Keene',
title: 'The Secret of Kane',
year: 1988},
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Deathly Hallows',
year: 1987},
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Chamber of Secrets',
year: 1987,
reviews: [
{rating: 10, user: 'joe', review: 'The best ...'},
{rating: 6, user: 'mary', review: 'Average ...'}]},
{publisher: 'XYZ',
author: 'J. K. Rowling',
title: 'Deathly Hallows',
year: 1986},
{publisher: 'Foster',
author: 'Old Rowling',
title: 'Chamber of Secrets',
year: 1979,
reviews: [{rating: 10, user: 'joe', review: 'The best ...'}]},
{publisher: 'Scholastic',
author: 'J. K. Rowling',
title: 'Sorcerers Stone',
year: 1978},
{publisher: 'Scholastic',
author: 'R. L. Stine',
title: 'Monster Blood IV',
year: 1977,
price: [3.6, 20.5, 41.0]
},
{publisher: 'Grosset',
author: 'Carolyn Keene',
title: 'The Secret of Kane',
year: 1920,
format: {cover: 'hard', pages: 66}}
];
//=========================Testing Filter-Transform===================================================
//Returns 8 records --Should be pusheddown
$data_books -> transform {x: $.author, $.year} -> filter $.x == 'J. K. Rowling';
//Returns count of 14 records --Should be pusheddown TWICE
$data_books -> transform each $d {x: $d.author, $d.format.cover, y:$d.price} -> transform each $g [$g.x, $g.y] -> filter true ->count();
//Returns count of 3 records --Should be pusheddown (All the three predicates)
$data_books -> transform {n: $.author, x: $, $.year , $.format.cover, $, c: $.format.cover, f: $.price-> count(), k: "33333"} -> filter each $dd $dd.cover == 'hard' and $dd.k == '33333' and $dd."".author == 'J. K. Rowling' -> count();
//Returns count of 2 records --(Predicates 1, 2, and 4 should be pusheddown) (Predicate 3 stays as is)
$data_books -> transform {n: $.author, x: $, $.year , $.format.cover, $, c: $.format.cover, f: $.price-> count(), k: "33333"} -> filter $.cover == 'hard' and $.k == '33333' and $.f > 1 and $."".author == 'J. K. Rowling' -> count() ;
//Returns 3 records --Should be pusheddown
$data_books -> transform each $d {n: $d.author, m: $d.year, $d.year , c: $d.format} -> filter each $x $x.m >= 2004 or $x.c.cover == 'soft';
//Returns 6 records --Should be pusheddown
$data_books -> transform ($.year) -> filter $ > 1990;
//Returns 4 records --Should be pusheddown
$data_books -> transform ($.format) -> filter $.cover == 'hard';
//Returns 4 records --Should be pusheddown
$data_books -> transform {$.author, cnt: $.year + $.format.pages * 2} -> filter each $x $x.cnt > 3000;
//Returns 2 records --Should be pusheddown TWICE
$data_books -> transform each $d {x: $d.author, $d.format.cover, y:$d.price} -> transform each $g [$g.x, $g.y] -> filter $[0] == 'R. L. Stine';
//Returns 1 record --First predicate should be pushed TWICE. The second predicate should be pusheddown ONCE
$data_books -> transform each $d {x: $d.author, $d.format.cover, y:sum($d.price)} -> transform each $g [$g.x, $g.y] -> filter $[0] == 'R. L. Stine' and $[1]> 20;
//Returns 4 records --Should be pusheddown ONCE
$data_books -> transform [$, $.author, $.format, "3333" ,{name: $.publisher, y: $.year}, max($.price)] -> transform {c:$[2].cover, $[4].name, max: $[5] } -> filter $.max > 2;
//Returns 2 records --Should be pusheddown TWICE
$data_books -> transform [$, $.author, $.format, "3333" ,{name: $.publisher, y: $.year}, max($.price)] -> transform {c:$[2].cover, $[4].name, max: $[5] } -> filter $.c == 'soft';
//Returns 2 records --Should be pusheddown TWICE
$data_books -> transform each $d {x: $d.author, $d.format.cover, y:$d.price} -> transform each $g [$g.x, $g.y] -> filter count($[1]) > 2;
//Returns 1 record --(Predicate $.a == 1 should be pushed down.
// Predicate count($.less)>4 remains as is).
[{a:1, b:[1,2,3,4,5,6,6,77,87,43,122]}, {a:2, b:[12,3,34434,766,33,11,10,2]}]
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于java的开发源码-JSON查询语言 Jaql.zip 基于java的开发源码-JSON查询语言 Jaql.zip 基于java的开发源码-JSON查询语言 Jaql.zip 基于java的开发源码-JSON查询语言 Jaql.zip 基于java的开发源码-JSON查询语言 Jaql.zip 基于java的开发源码-JSON查询语言 Jaql.zip 基于java的开发源码-JSON查询语言 Jaql.zip 基于java的开发源码-JSON查询语言 Jaql.zip 基于java的开发源码-JSON查询语言 Jaql.zip 基于java的开发源码-JSON查询语言 Jaql.zip 基于java的开发源码-JSON查询语言 Jaql.zip
资源推荐
资源详情
资源评论
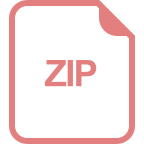
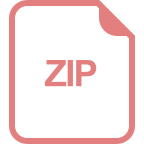
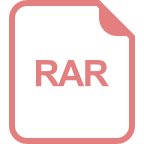
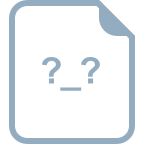
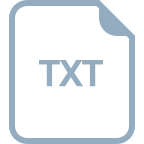
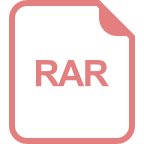
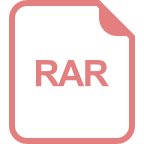
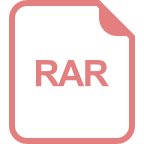
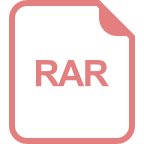
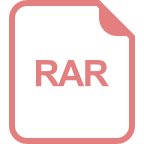
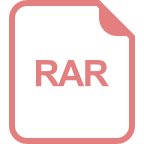
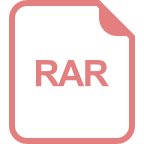
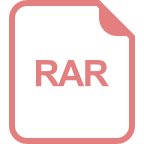
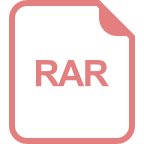
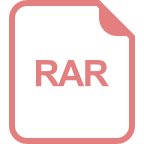
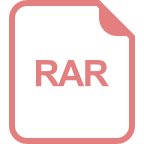
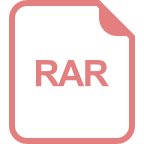
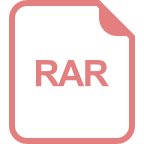
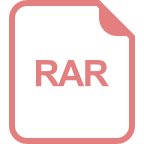
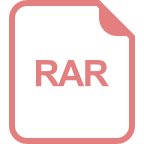
收起资源包目录

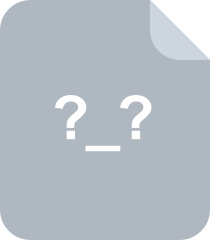
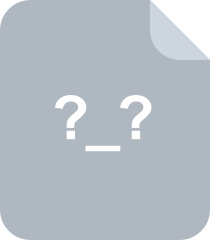
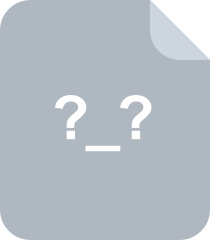
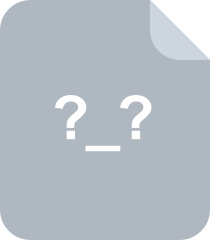
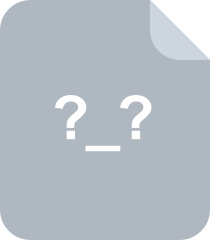
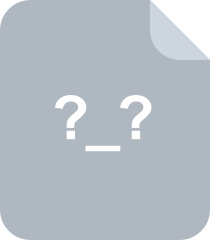
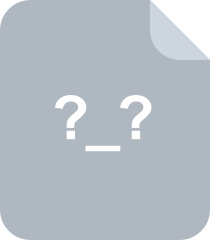
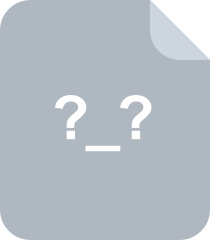
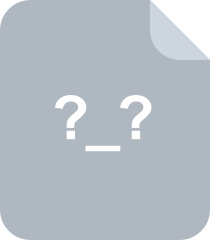
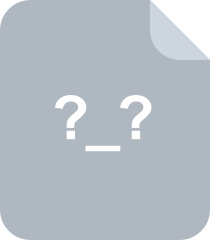
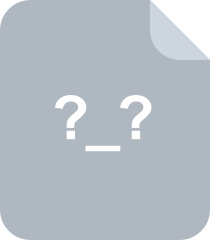
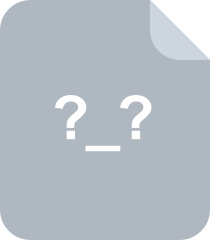
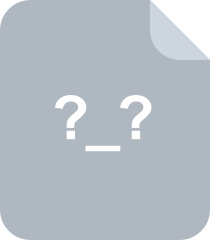
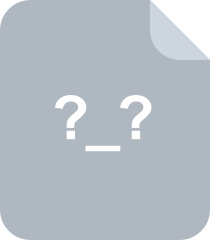
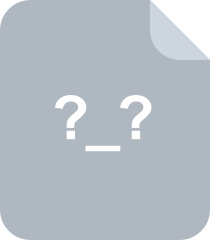
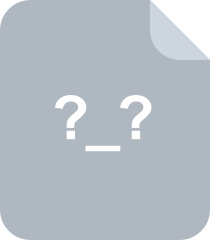
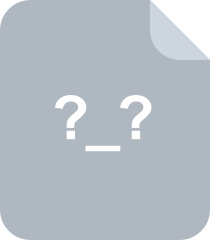
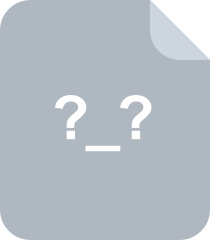
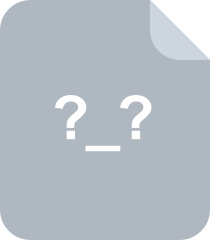
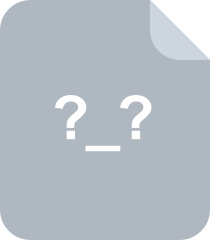
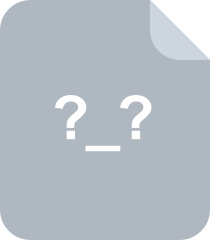
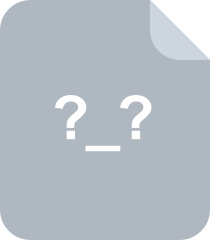
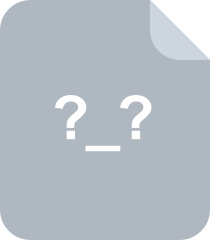
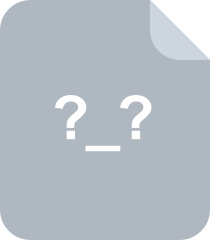
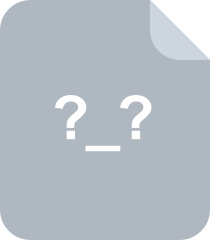
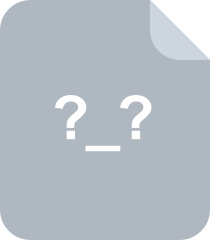
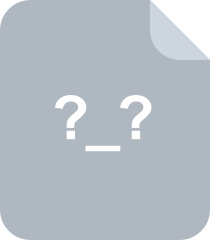
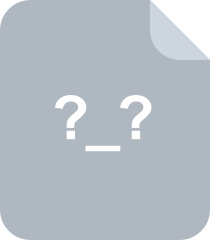
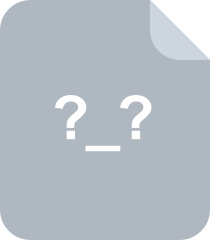
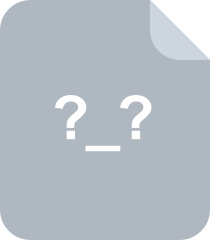
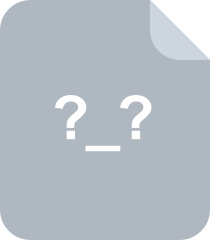
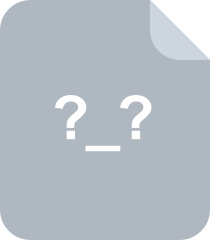
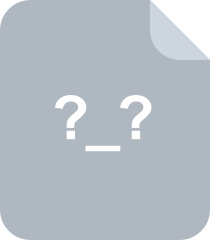
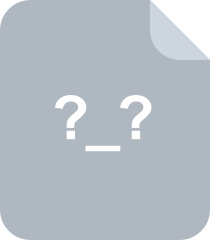
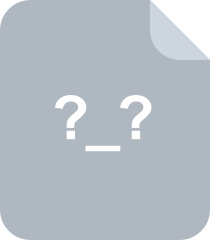
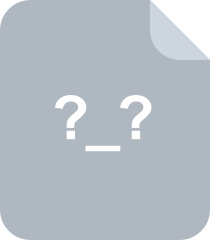
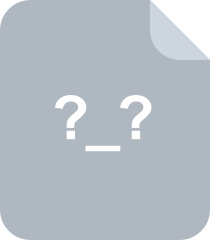
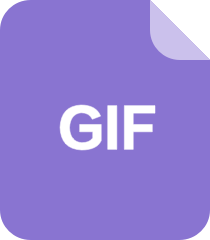
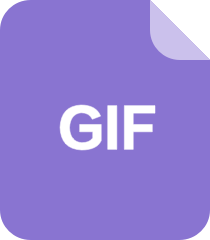
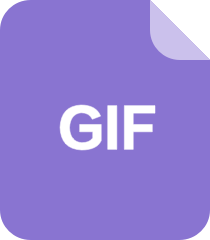
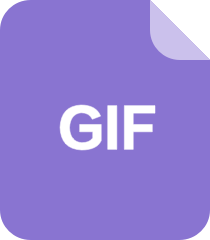
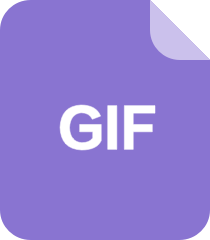
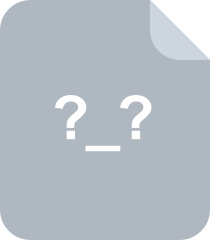
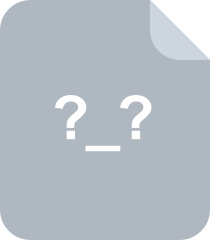
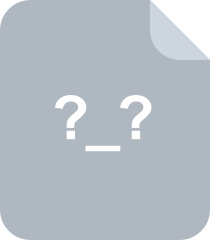
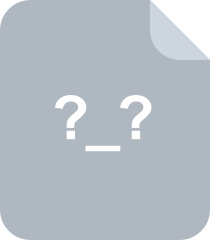
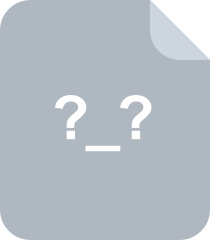
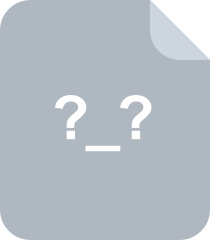
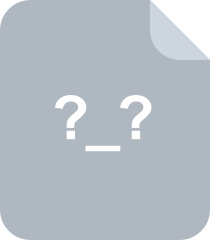
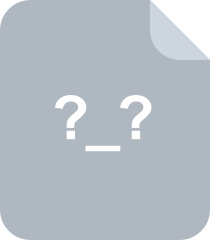
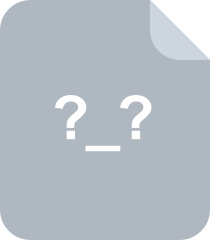
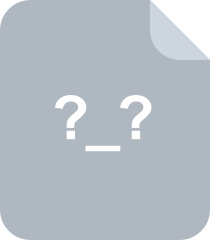
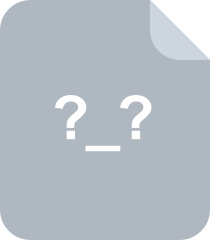
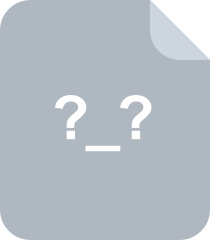
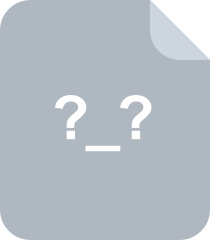
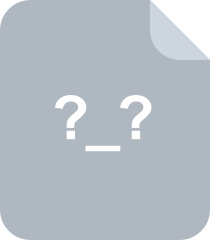
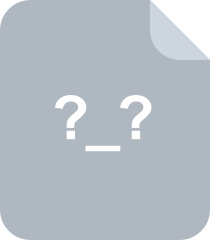
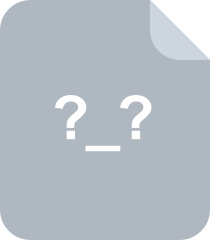
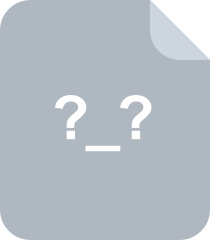
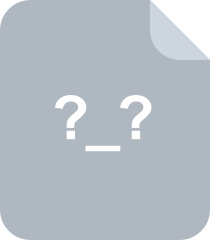
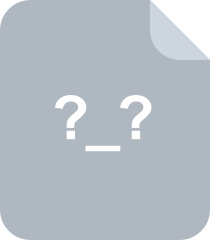
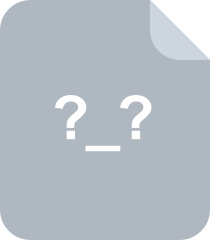
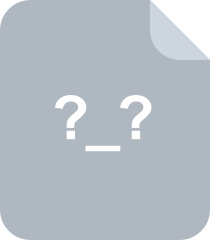
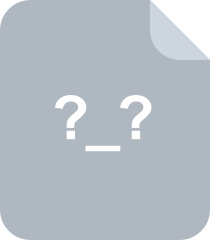
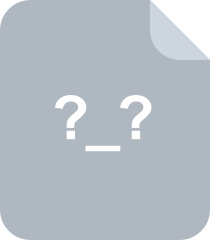
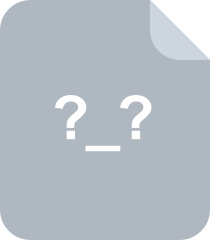
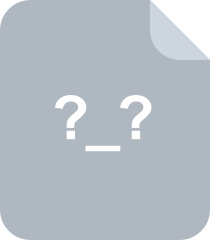
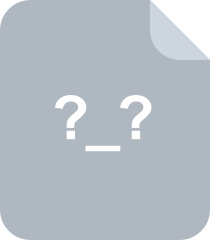
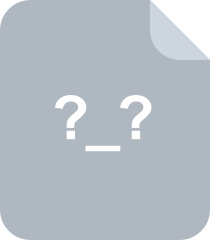
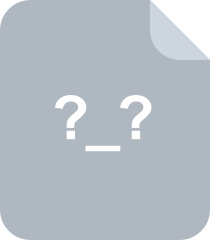
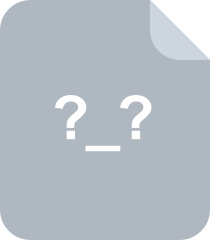
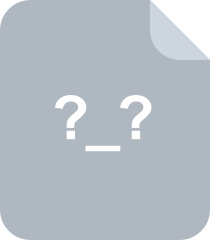
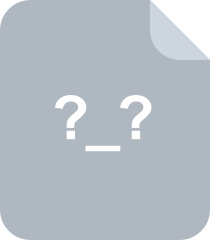
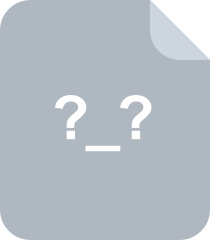
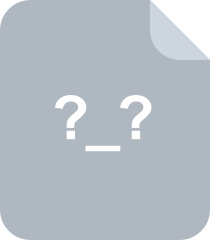
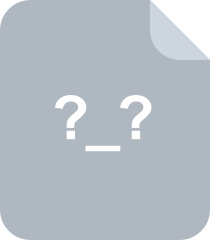
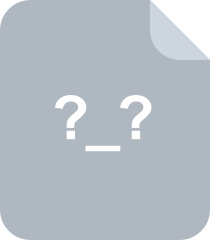
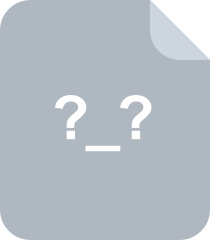
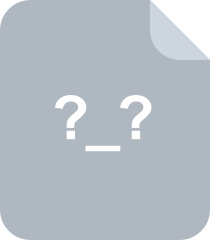
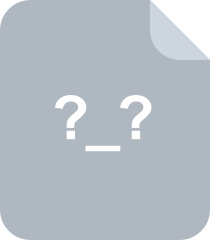
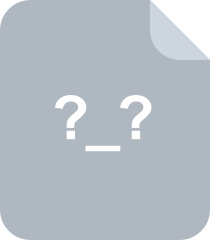
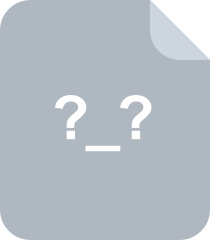
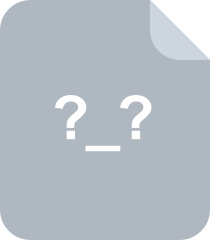
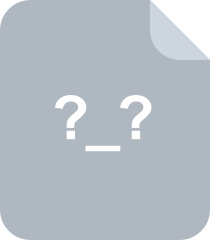
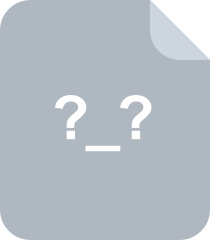
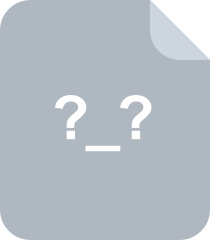
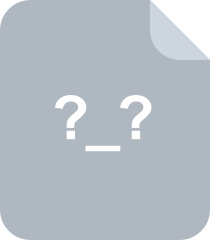
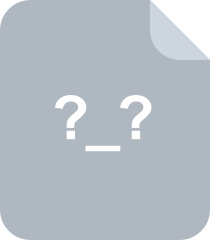
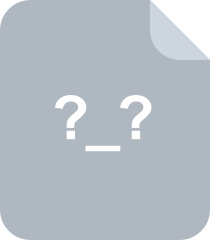
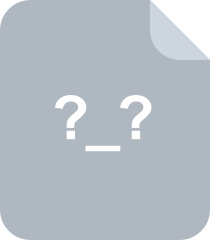
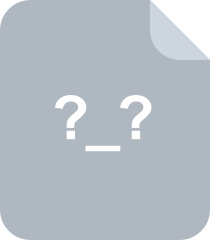
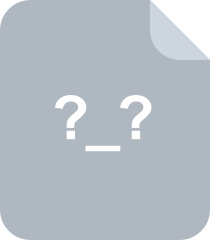
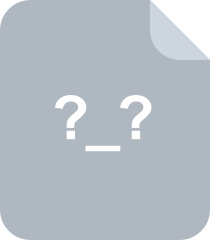
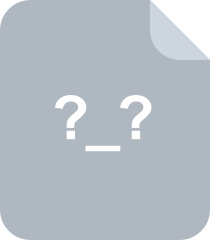
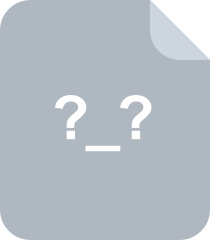
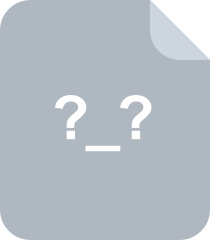
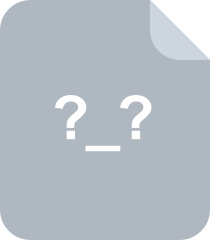
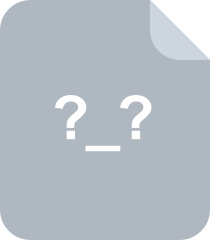
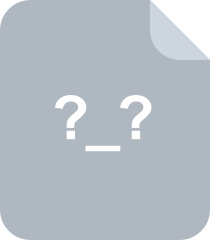
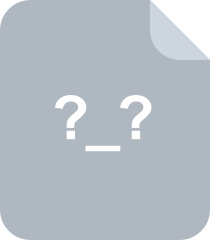
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
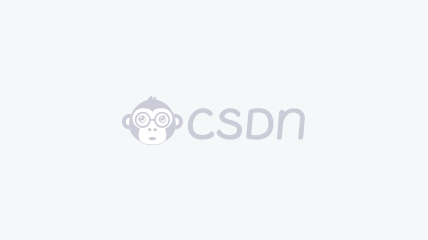

快乐无限出发
- 粉丝: 1206
- 资源: 7390
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

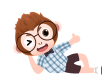
最新资源
- java全大撒大撒大苏打
- pca20241222
- LabVIEW实现LoRa通信【LabVIEW物联网实战】
- CS-TY4-4WCN-转-公版-XP1-8B4WF-wifi8188
- 计算机网络期末复习资料(课后题答案+往年考试题+复习提纲+知识点总结)
- 从零学习自动驾驶Lattice规划算法(下) 轨迹采样 轨迹评估 碰撞检测 包含matlab代码实现和cpp代码实现,方便对照学习 cpp代码用vs2019编译 依赖qt5.15做可视化 更新:
- 风光储、风光储并网直流微电网simulink仿真模型 系统由光伏发电系统、风力发电系统、混合储能系统(可单独储能系统)、逆变器VSR+大电网构成 光伏系统采用扰动观察法实现mppt控
- (180014016)pycairo-1.18.2-cp35-cp35m-win32.whl.rar
- (180014046)pycairo-1.21.0-cp311-cp311-win32.whl.rar
- DS-7808-HS-HF / DS-7808-HW-E1
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


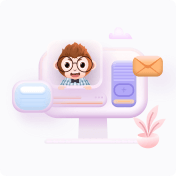
安全验证
文档复制为VIP权益,开通VIP直接复制
