package com.github.tangyi.exam.service;
import com.github.pagehelper.PageInfo;
import com.github.tangyi.common.basic.vo.UserVo;
import com.github.tangyi.common.core.constant.CommonConstant;
import com.github.tangyi.common.core.constant.MqConstant;
import com.github.tangyi.common.core.exceptions.CommonException;
import com.github.tangyi.common.core.model.ResponseBean;
import com.github.tangyi.common.core.service.CrudService;
import com.github.tangyi.common.core.utils.DateUtils;
import com.github.tangyi.common.core.utils.JsonMapper;
import com.github.tangyi.common.core.utils.PageUtil;
import com.github.tangyi.common.core.utils.ResponseUtil;
import com.github.tangyi.common.security.utils.SysUtil;
import com.github.tangyi.exam.api.constants.AnswerConstant;
import com.github.tangyi.exam.api.dto.AnswerDto;
import com.github.tangyi.exam.api.dto.RankInfoDto;
import com.github.tangyi.exam.api.dto.StartExamDto;
import com.github.tangyi.exam.api.dto.SubjectDto;
import com.github.tangyi.exam.api.enums.SubmitStatusEnum;
import com.github.tangyi.exam.api.module.Answer;
import com.github.tangyi.exam.api.module.Examination;
import com.github.tangyi.exam.api.module.ExaminationRecord;
import com.github.tangyi.exam.api.module.ExaminationSubject;
import com.github.tangyi.exam.enums.SubjectTypeEnum;
import com.github.tangyi.exam.handler.AnswerHandleResult;
import com.github.tangyi.exam.handler.impl.ChoicesAnswerHandler;
import com.github.tangyi.exam.handler.impl.JudgementAnswerHandler;
import com.github.tangyi.exam.handler.impl.MultipleChoicesAnswerHandler;
import com.github.tangyi.exam.handler.impl.ShortAnswerHandler;
import com.github.tangyi.exam.mapper.AnswerMapper;
import com.github.tangyi.exam.utils.AnswerHandlerUtil;
import com.github.tangyi.exam.utils.ExamRecordUtil;
import com.github.tangyi.user.api.feign.UserServiceClient;
import lombok.AllArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.amqp.core.AmqpTemplate;
import org.springframework.beans.BeanUtils;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.ZSetOperations;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.time.LocalDateTime;
import java.util.*;
import java.util.stream.Collectors;
/**
* 答题service
*
* @author tangyi
* @date 2018/11/8 21:17
*/
@Slf4j
@AllArgsConstructor
@Service
public class AnswerService extends CrudService<AnswerMapper, Answer> {
private final UserServiceClient userServiceClient;
private final AmqpTemplate amqpTemplate;
private final SubjectService subjectService;
private final ExamRecordService examRecordService;
private final ExaminationService examinationService;
private final ExaminationSubjectService examinationSubjectService;
private final ChoicesAnswerHandler choicesHandler;
private final MultipleChoicesAnswerHandler multipleChoicesHandler;
private final JudgementAnswerHandler judgementHandler;
private final ShortAnswerHandler shortAnswerHandler;
private final RedisTemplate<String, String> redisTemplate;
/**
* 查找答题
*
* @param answer answer
* @return Answer
* @author tangyi
* @date 2019/1/3 14:27
*/
@Override
@Cacheable(value = "answer#" + CommonConstant.CACHE_EXPIRE, key = "#answer.id")
public Answer get(Answer answer) {
return super.get(answer);
}
/**
* 根据用户ID、考试ID、考试记录ID、题目ID查找答题
*
* @param answer answer
* @return Answer
* @author tangyi
* @date 2019/01/21 19:41
*/
public Answer getAnswer(Answer answer) {
return this.dao.getAnswer(answer);
}
/**
* 更新答题
*
* @param answer answer
* @return int
* @author tangyi
* @date 2019/1/3 14:27
*/
@Override
@Transactional
@CacheEvict(value = "answer", key = "#answer.id")
public int update(Answer answer) {
answer.setAnswer(AnswerHandlerUtil.replaceComma(answer.getAnswer()));
return super.update(answer);
}
/**
* 更新答题总分
*
* @param answer answer
* @return int
* @author tangyi
* @date 2019/1/3 14:27
*/
@Transactional
@CacheEvict(value = "answer", key = "#answer.id")
public int updateScore(Answer answer) {
answer.setAnswer(AnswerHandlerUtil.replaceComma(answer.getAnswer()));
// 加分减分逻辑
Answer oldAnswer = this.get(answer);
if (!oldAnswer.getAnswerType().equals(answer.getAnswerType())) {
ExaminationRecord record = new ExaminationRecord();
record.setId(oldAnswer.getExamRecordId());
record = examRecordService.get(record);
if (record == null) {
throw new CommonException("ExamRecord is null");
}
Double oldScore = record.getScore();
if (AnswerConstant.RIGHT.equals(answer.getAnswerType())) {
// 加分
record.setCorrectNumber(record.getInCorrectNumber() + 1);
record.setInCorrectNumber(record.getInCorrectNumber() - 1);
record.setScore(record.getScore() + answer.getScore());
} else if (AnswerConstant.WRONG.equals(answer.getAnswerType())) {
// 减分
record.setCorrectNumber(record.getInCorrectNumber() - 1);
record.setInCorrectNumber(record.getInCorrectNumber() + 1);
record.setScore(record.getScore() - answer.getScore());
}
if (examRecordService.update(record) > 0) {
log.info("Update answer success, examRecordId: {}, oldScore: {}, newScore: {}", oldAnswer.getExamRecordId(), oldScore, record.getScore());
}
}
return super.update(answer);
}
/**
* 删除答题
*
* @param answer answer
* @return int
* @author tangyi
* @date 2019/1/3 14:27
*/
@Override
@Transactional
@CacheEvict(value = "answer", key = "#answer.id")
public int delete(Answer answer) {
return super.delete(answer);
}
/**
* 批量删除答题
*
* @param ids ids
* @return int
* @author tangyi
* @date 2019/1/3 14:27
*/
@Override
@Transactional
@CacheEvict(value = "answer", allEntries = true)
public int deleteAll(Long[] ids) {
return super.deleteAll(ids);
}
/**
* 保存
*
* @param answer answer
* @return int
* @author tangyi
* @date 2019/04/30 18:03
*/
@Transactional
public int save(Answer answer) {
answer.setCommonValue(SysUtil.getUser(), SysUtil.getSysCode(), SysUtil.getTenantCode());
answer.setAnswer(AnswerHandlerUtil.replaceComma(answer.getAnswer()));
return super.save(answer);
}
/**
* 保存答题,返回下一题信息
*
* @param answerDto answerDto
* @param type 0:下一题,1:上一题
* @param nextSubjectId nextSubjectId
* @param nextSubjectType 下一题的类型,选择题、判断题
* @return SubjectDto
* @author tangyi
* @date 2019/05/01 11:42
*/
@Transactional
public SubjectDto saveAndNext(AnswerDto answerDto, Integer type, Long nextSubjectId, Integer nextSubjectType) {
String userCode = SysUtil.getUser();
String sysCode = SysUtil.getSysCode();
String tenantCode = SysUtil.getTenantCode();
if (this.save(answerDto, userCode, sysCode, tenantCode) > 0) {
// 查询下一题
return this.subjectAnswer(answerDto.getSubjectId(), answerDto.getExamRecordId(),
type, nextSubjectId, nextSubjectType);
}
return null;
}
/**
*
没有合适的资源?快使用搜索试试~ 我知道了~
基于Java和跨平台技术的micro-service架构设计源码
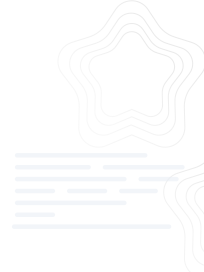
共989个文件
java:351个
js:141个
vue:100个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 126 浏览量
2024-10-05
07:00:23
上传
评论
收藏 28.88MB ZIP 举报
温馨提示
该项目是一个基于Java和跨平台技术的micro-service架构设计源码,总计包含992个文件,涵盖多种编程语言和文件类型。其中,Java源文件351个,JavaScript文件141个,Vue组件100个,SVG图形82个,XML配置文件58个,图像文件(包括PNG、GIF、JPEG、JPG)共226个,以及YAML文件19个。该架构支持多语言开发,包括JavaScript、Vue、CSS、HTML等,旨在实现高效的跨平台服务部署。
资源推荐
资源详情
资源评论
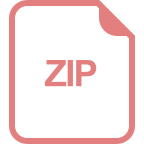
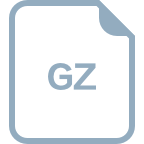
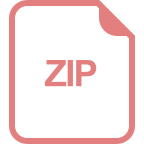
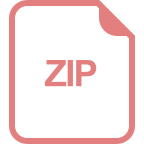
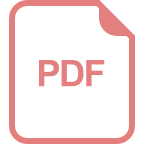
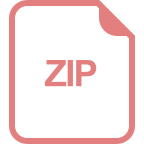
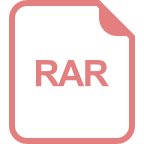
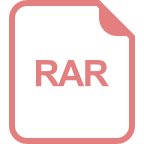
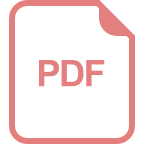
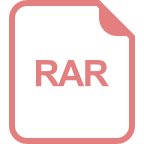
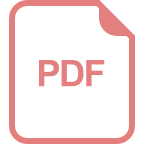
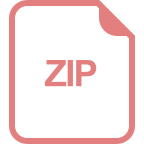
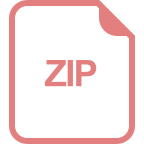
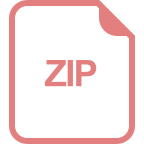
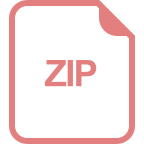
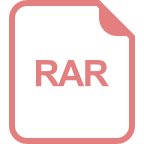
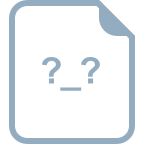
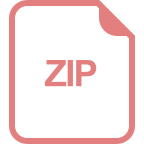
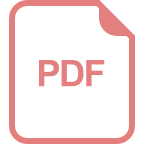
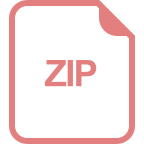
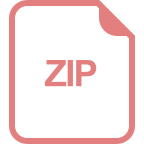
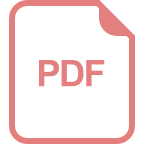
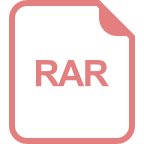
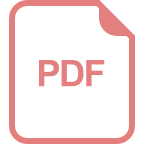
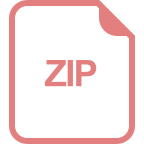
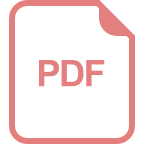
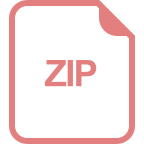
收起资源包目录

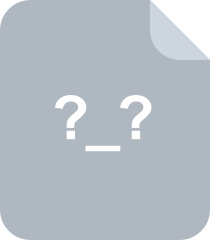
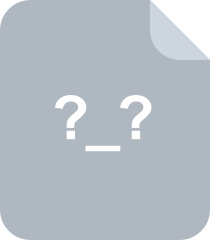
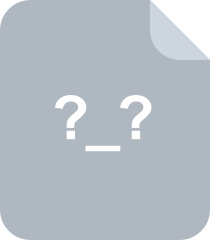
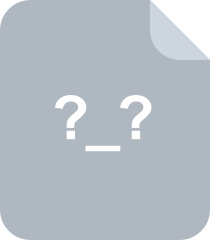
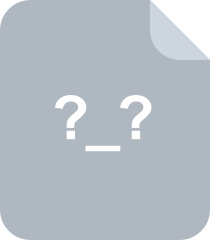
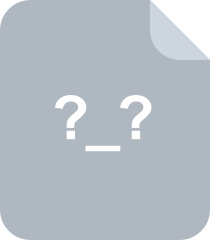
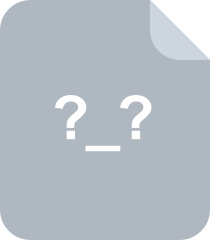
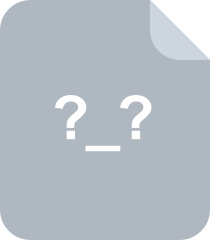
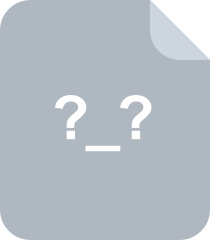
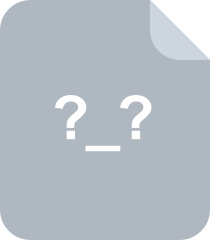
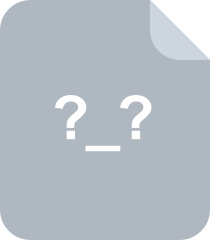
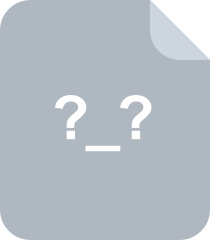
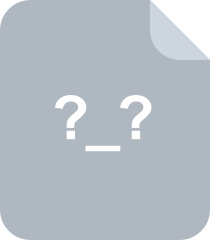
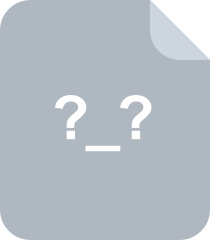
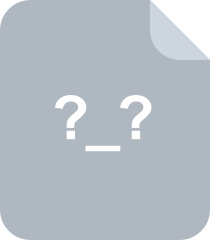
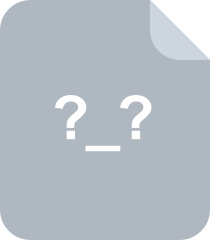
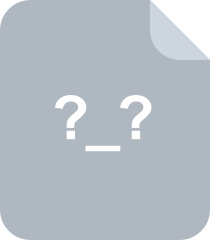
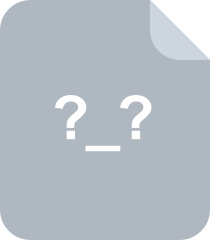
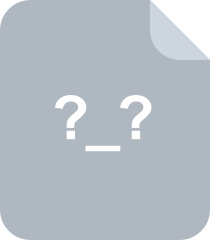
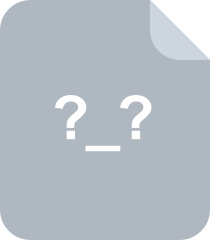
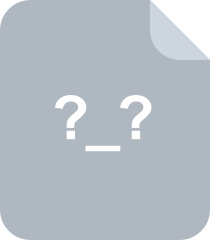
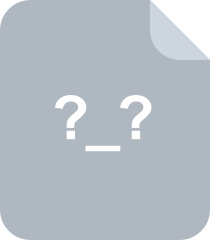
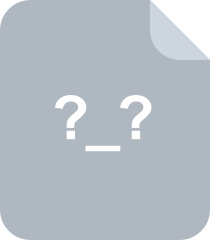
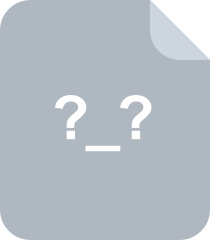
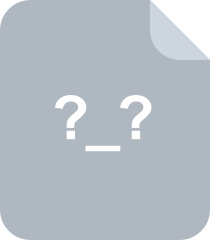
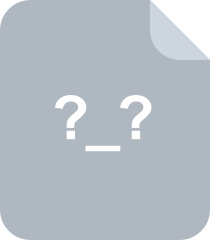
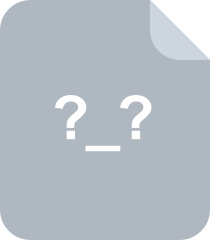
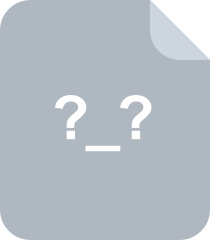
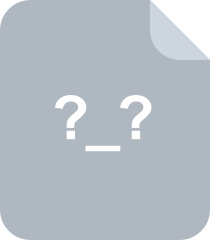
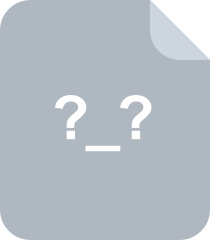
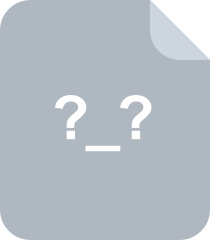
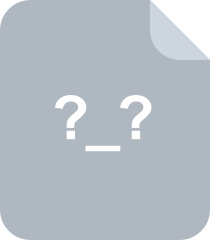
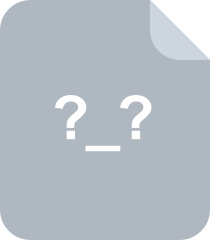
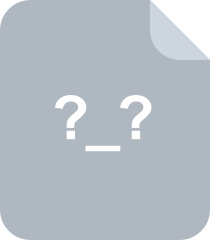
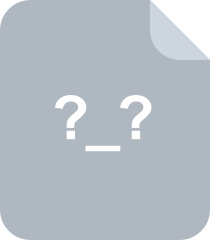
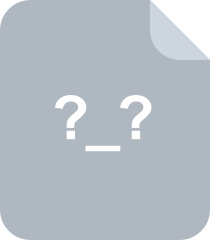
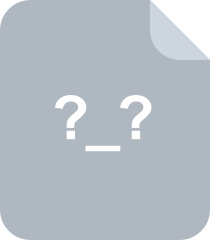
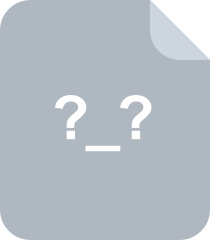
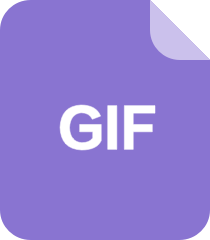
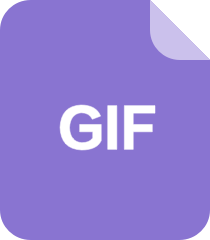
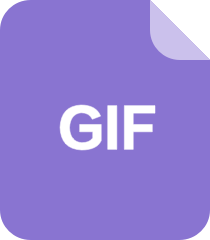
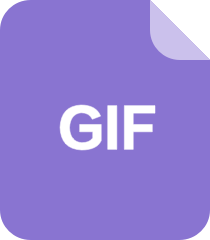
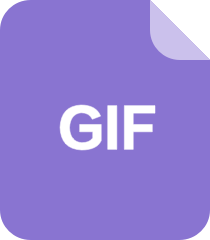
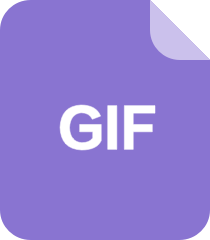
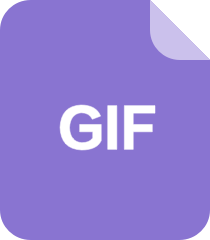
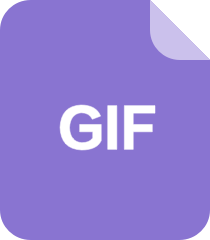
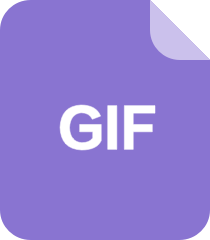
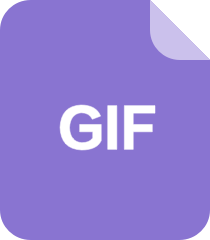
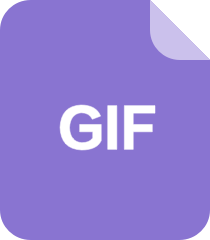
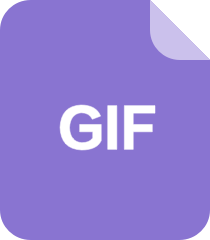
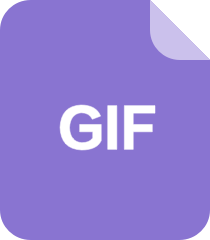
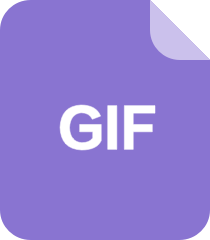
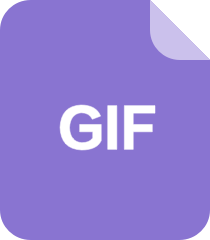
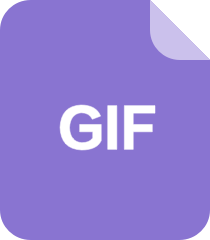
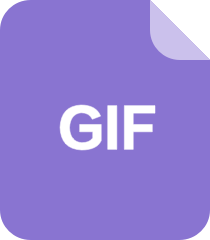
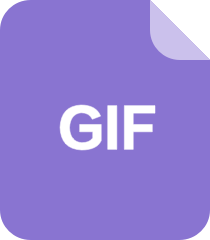
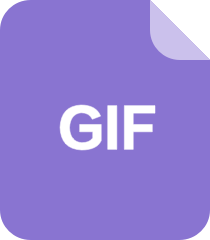
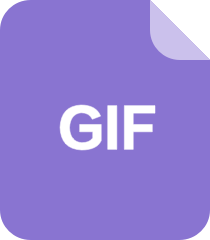
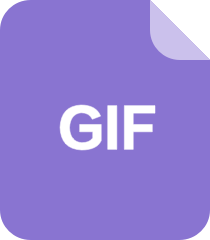
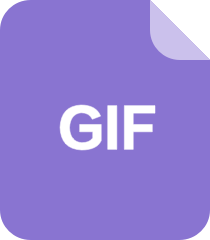
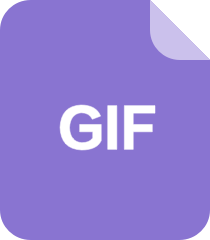
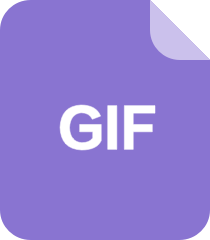
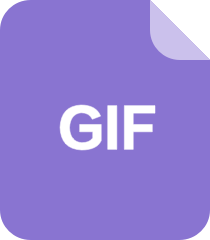
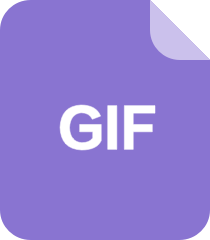
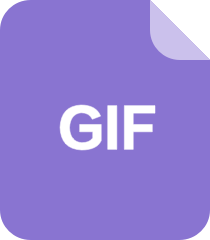
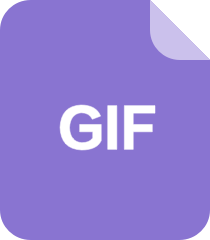
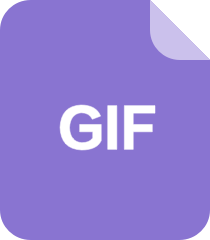
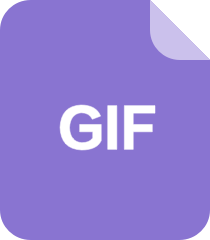
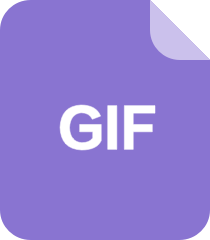
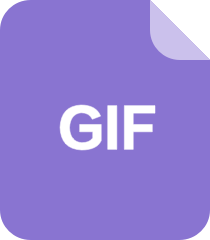
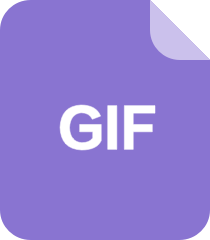
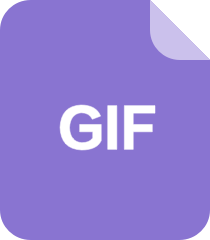
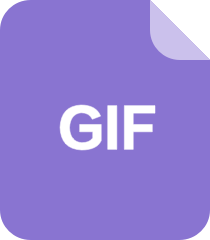
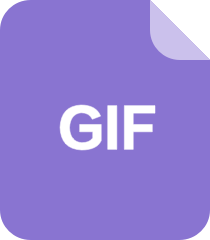
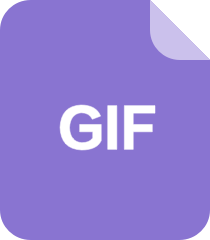
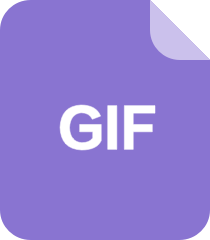
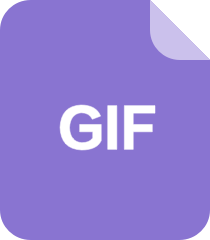
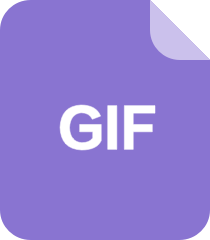
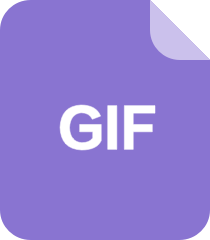
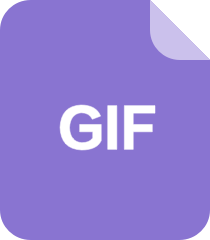
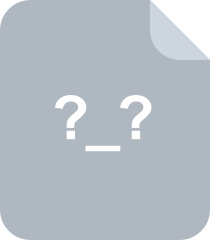
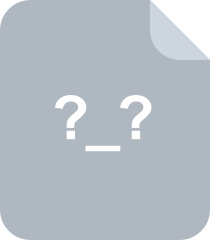
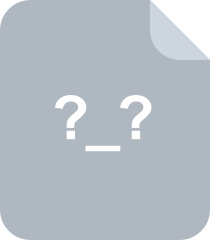
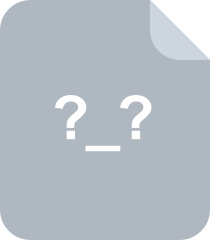
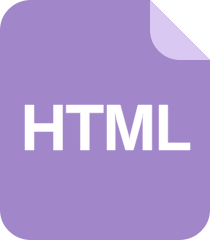
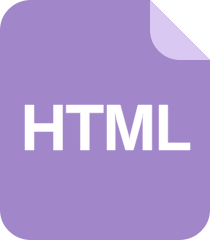
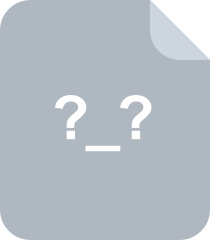
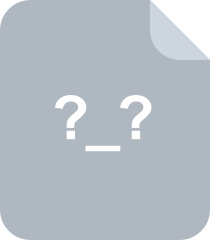
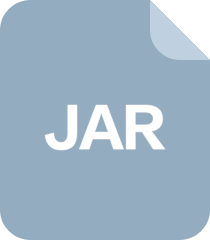
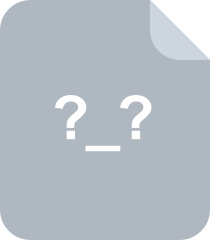
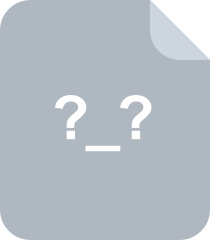
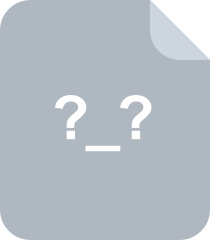
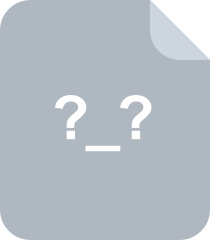
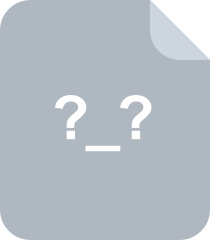
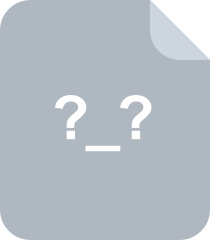
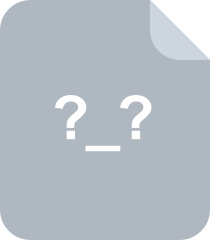
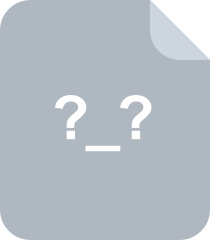
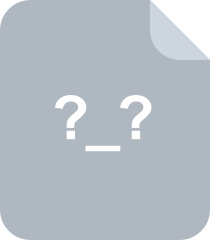
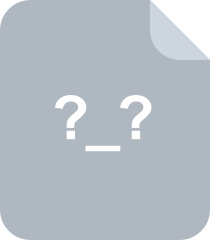
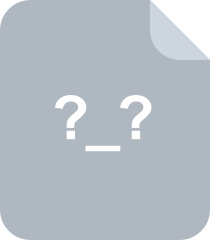
共 989 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
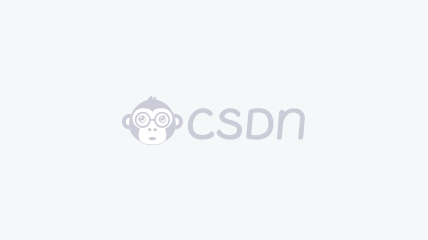

wjs2024
- 粉丝: 1889
- 资源: 5386
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

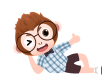
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


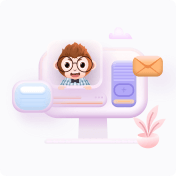
安全验证
文档复制为VIP权益,开通VIP直接复制
