1. The Kamailio RPC Control Interface
__________________________________________________________________
1.1. Overview of Operation
1.2. Module API
1.2.1. RPC Functions
1.2.2. Data Types
1.2.3. Getting Parameters
1.2.3.1. scan
1.2.3.2. struct_scan
1.2.3.3. Retrieving Parameters Example
1.2.4. Building Reply
1.2.4.1. fault
1.2.4.2. send
1.2.4.3. add
1.2.4.4. rpl_printf
1.2.4.5. struct_add
1.2.5. Real World Example
1.3. Client Examples
1.4. Implementing New Transports
1.5. Examples using xmlrpc
1.1. Overview of Operation
The RPC (Remote Procedure Call) interface is an interface for
communicating with external applications. Using it an external
application can call a function or procedure that will be executed
inside Kamailio. Function parameters are supported as well as returning
multiple values as results.
By itself RPC consists of two APIs, one for defining RPC functions in a
transport independent way (called the rpc module api) and one for
implementing RPC transports.
The RPC transports are implemented by writing a RPC transport module.
The most used transport modules are ctl , xmlrpc and jsonrpc-s .
ctl implements a proprietary fast and space efficient RPC encoding over
different protocols (unix sockets, UDP, TCP, fifo).
xmlrpc uses the de-facto XML-RPC standard encoding (over HTTP TCP or
TLS).
jsonrpc-s uses the de-facto JSON-RPC standard encoding (over HTTP TCP
or TLS).
For more information about the existing transport modules, please refer
to their documentation.
When writing a RPC procedure or function, one needs only use the RPC
API and it will work automatically with all the transports and
encodings. One needs only to load the desired RPC transport module
(e.g. xmlrpc).
The RPC interface (or API) was created in such a way that would allow
supporting XML-RPC (because XML-RPC is a de-facto standard), while in
the same time being very easy to use.
1.2. Module API
Each module can export RPC functions just like it can export parameters
and functions to be called from the script. Whenever Kamailio receives
an RPC request, it will search through the list of exported RPC
functions and the function with matching name will be executed. A
couple of essential RPC functions are also embedded into the SIP server
core.
This section gives a detailed overview of the whole RPC API.
Section 1.2.1, "RPC Functions" describes the prototype and conventions
used in RPC functions. Section 1.2.2, "Data Types" gives a detailed
overview of available data types that can be used in function
parameters and return value. Section 1.2.3, "Getting Parameters"
describes functions of the RPC API that can be used to retrieve
parameters of the function, and finally Section 1.2.4, "Building Reply"
describes functions of the API that can be used to build the result
value that will be sent in the reply to the caller.
The whole RPC API is described in header file kamailio/rpc.h. This file
defines the set of functions that must be implemented by RPC transport
modules, as described in Section 1.4, "Implementing New Transports",
prototypes of RPC functions and structures used for the communication
between RPC transport modules and ordinary modules exporting RPC
functions.
1.2.1. RPC Functions
RPC functions are standard C functions with the following prototype:
typedef void (*rpc_function_t)(rpc_t* rpc, void* ctx);
RPC functions take two parameters, first parameter is a pointer to
rpc_t structure and the context. The rpc_t structure contains
references to all API functions available to the RPC function as well
as all data necessary to create the response. RPC functions do not
return any value, instead the return value is created using functions
from the context. The motivation for this decision is the fact that RPC
functions should always return a response and even the API functions
called from RPC functions should have the possibility to indicate an
error (and should not rely on RPC functions doing so).
If no reply is sent explicitely, the RPC transport module will
automatically send a "success" reply (e.g. 200 OK for XML-RPC) when the
RPC function finishes. If no values are added to the response, the
reponse will be an empty "success" reply (e.g. a 200 OK with empty body
for XML-RPC). RPC API functions will automatically send an error reply
upon a failure.
Each RPC function has associated an array of documentation strings. The
purpose of the documentation strings is to give a short overview of the
function, accepted parameters, and format of the reply. By convention
the name of the documentation string array is same as the name of the
function with "_doc" suffix.
Each module containing RPC functions has to export all the RPC
functions to the Kamailio core in order to make them visible to the RPC
transport modules. The export process involves a rpc_export_t structure
(either by itself or in an array):
typedef struct rpc_export {
const char* name; /* Name of the RPC function (null terminated) */
rpc_function_t function; /* Pointer to the function */
const char** doc_str; /* Documentation strings, method signature and desc
ription */
unsigned int flags; /* Various flags, reserved for future use */
} rpc_export_t;
The flags attribute of the rpc_export structure is reserved for future
use and is currently unused.
There are several ways of exporting the RPC functions to the Kamailio
core:
* register a null terminated array of rpc_export_t structures using
the rpc_register_array() function (defined in rpc_lookup.h), from
the module init function (mod_init()). This is the recommended
method for all the new modules.
Example 1. usrloc RPC Exports Declaration
The rpc_export_t array for the modules_s/usrloc module looks like:
rpc_export_t ul_rpc[] = {
{"usrloc.statistics", rpc_stats, rpc_stats_doc, 0},
{"usrloc.delete_aor", rpc_delete_aor, rpc_delete_aor_doc, 0},
{"usrloc.delete_contact", rpc_delete_contact, rpc_delete_contact_doc, 0},
{"usrloc.dump", rpc_dump, rpc_dump_doc, 0},
{"usrloc.flush", rpc_flush, rpc_flush_doc, 0},
{"usrloc.add_contact", rpc_add_contact, rpc_add_contact_doc, 0},
{"usrloc.show_contacts", rpc_show_contacts, rpc_show_contacts_doc, 0},
{0, 0, 0, 0}
};
To register it from the module init function one would use
something similar to:
if (rpc_register_array(ul_rpc) != 0) {
ERR("failed to register RPC commands\n");
return -1;
}
* register RPCs one by one using the rpc_register_function() (defined
in rpc_lookup.h), from the module init function.
* register a null terminated array of rpc_export_t structures using
the Kamailio module interface SER_MOD_INTERFACE For this purpose,
the module_exports structure of the Kamailio module API contains a
new attribute called rpc_methods:
struct module_exports {
char* name; /* null terminated module name */
cmd_export_t* cmds; /* null terminated array of the exported command
s */
rpc_export_t* rpc_methods; /* null terminated array of exported rpc methods
*/
param_export_t* params; /* null terminated array of the exported module
parameters */
init_function init_f; /* Initialization function */
response_function response_f; /* function used for responses */
destroy_function destroy_f; /* function called upon shutdown */
onbreak_function onbr
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该开源项目是针对大型VoIP和实时通信平台的SIP服务器kamailio的设计源码,由6692个文件构成,主要使用C语言编写,辅以Shell、Python、C++、Java、PHP、HTML、JavaScript、Lua、CSS、Ruby和C#等多种编程语言。文件类型多样,包括1430个C源文件、1407个头文件、1042个XML配置文件、201个SQL脚本文件、185个配置文件、157个文本文件、131个lintian-overrides文件、105个Shell脚本文件、72个JSON文件、59个SIP协议相关文件等。kamailio适用于构建高性能的VoIP和实时通信系统。
资源推荐
资源详情
资源评论
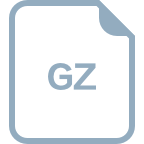
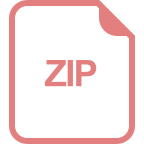
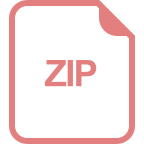
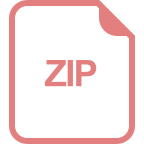
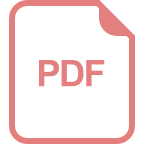
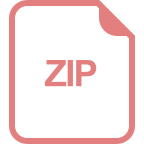
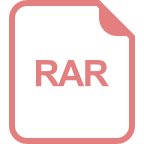
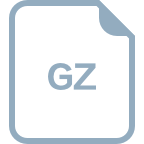
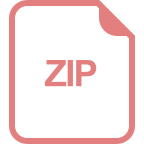
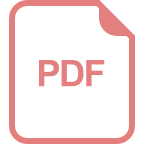
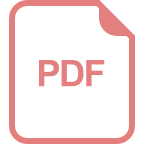
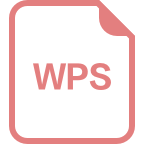
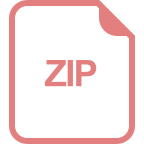
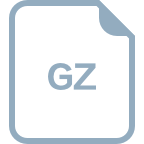
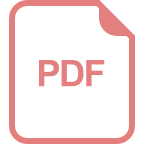
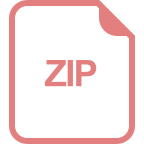
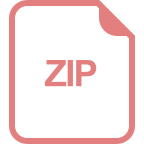
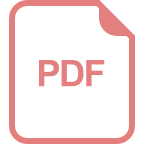
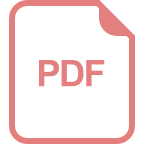
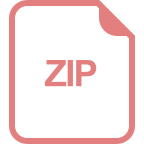
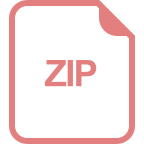
收起资源包目录

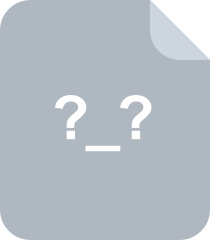
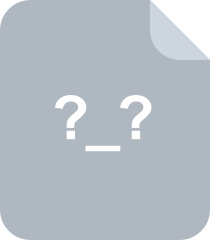
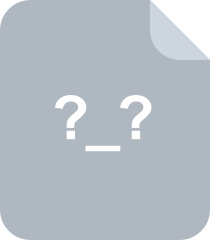
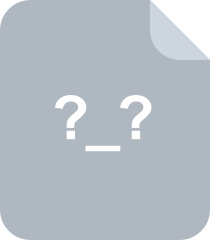
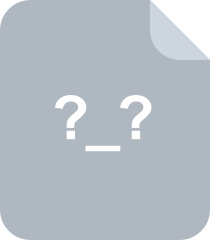
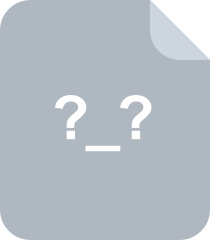
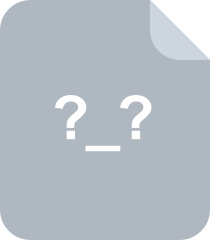
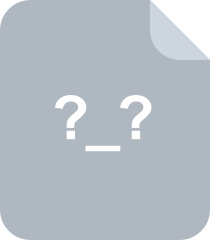
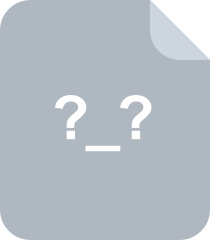
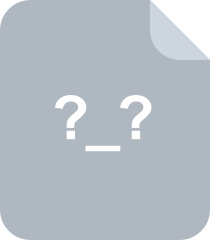
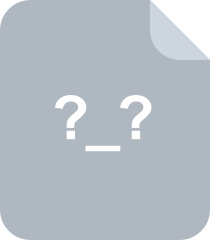
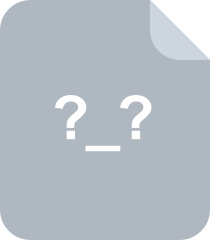
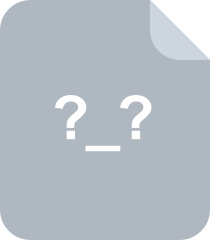
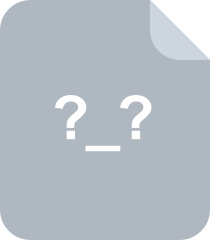
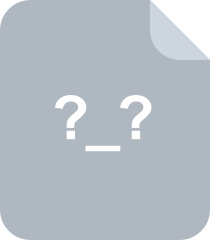
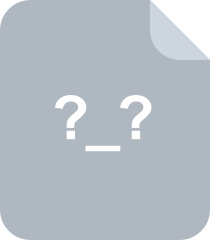
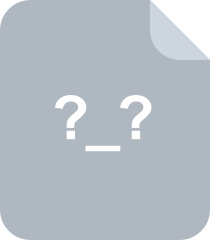
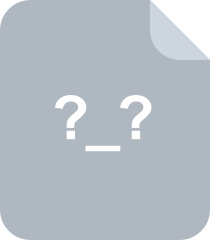
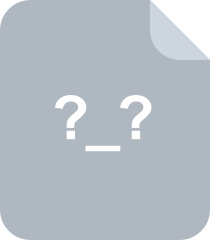
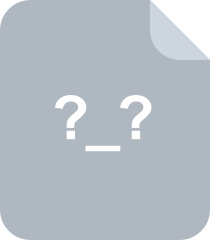
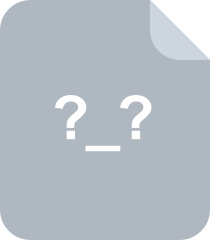
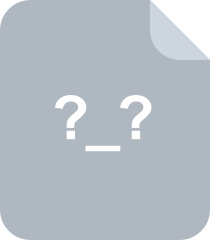
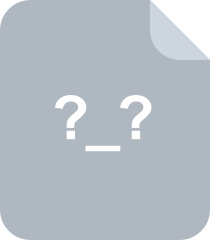
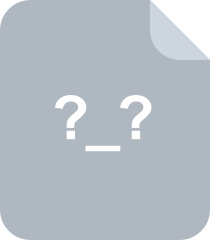
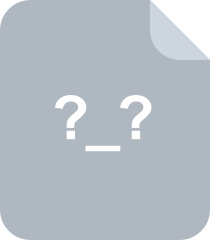
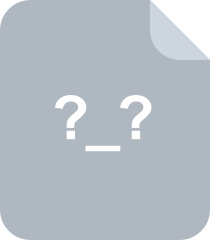
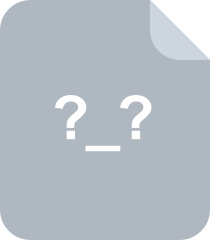
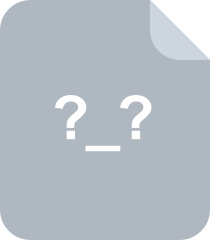
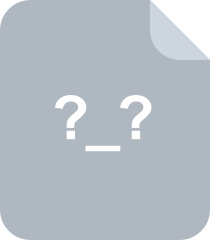
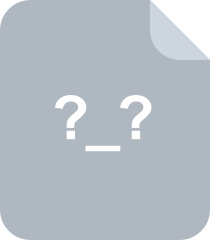
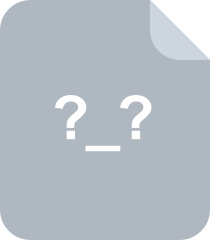
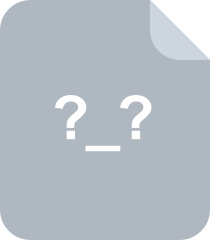
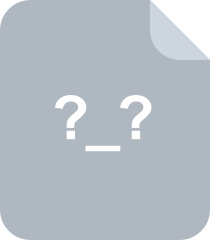
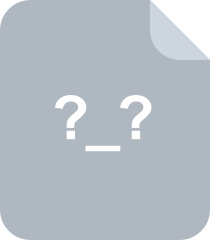
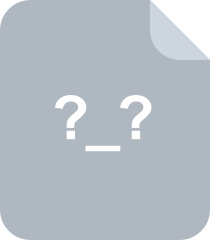
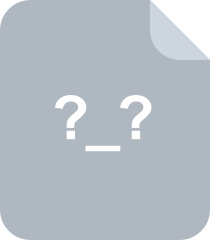
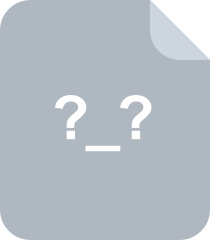
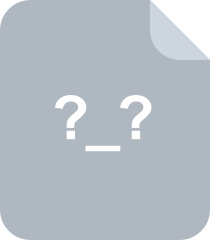
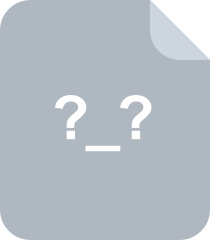
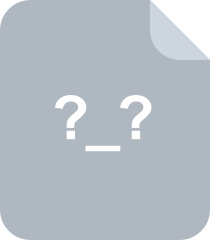
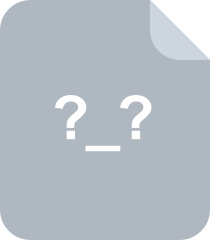
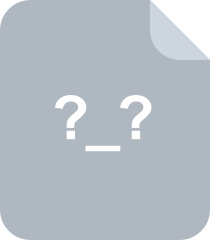
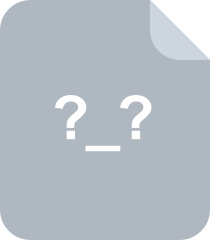
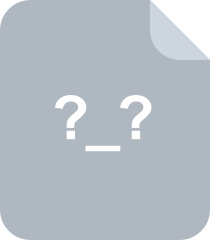
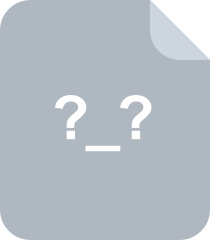
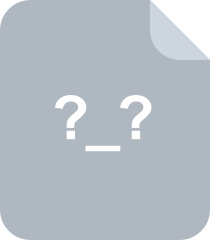
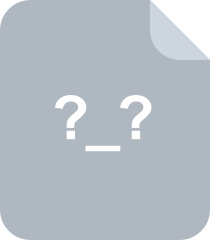
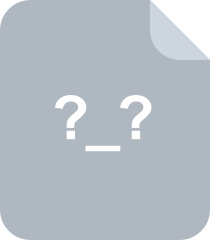
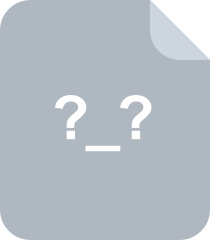
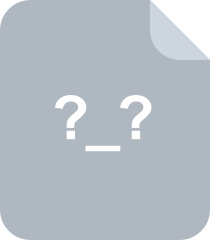
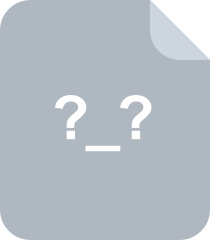
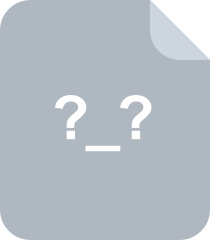
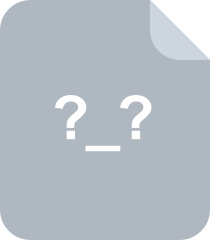
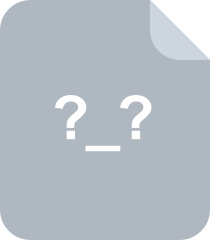
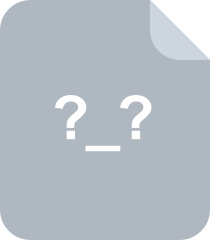
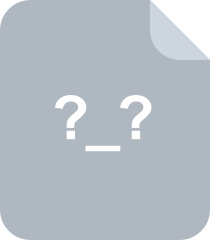
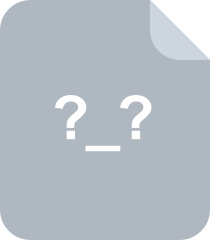
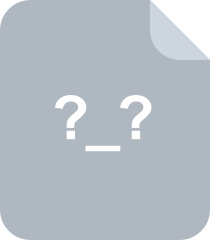
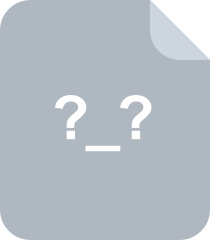
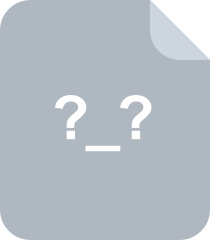
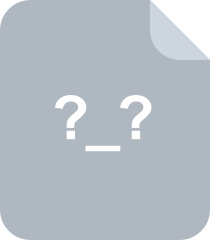
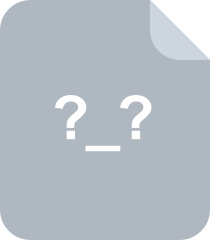
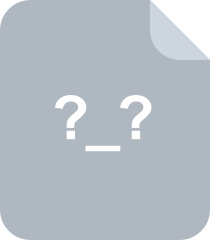
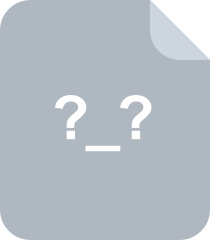
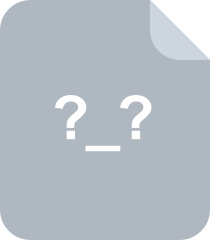
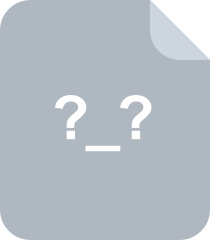
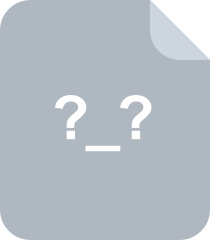
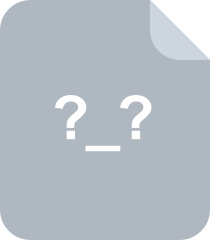
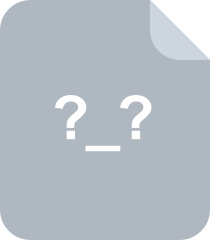
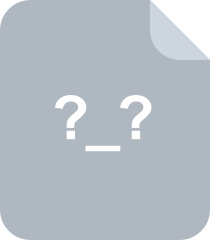
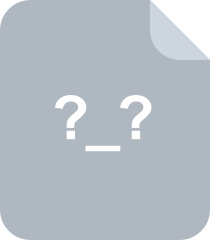
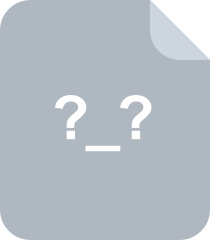
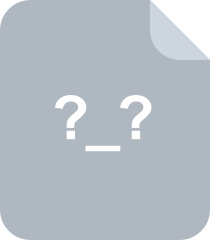
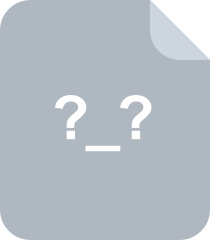
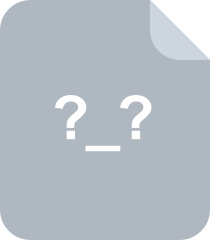
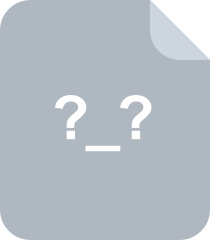
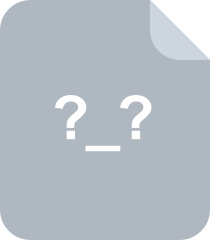
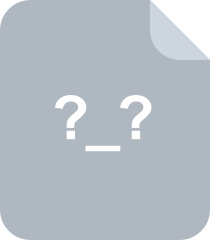
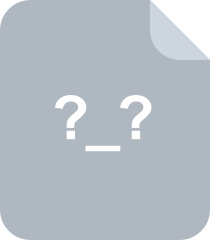
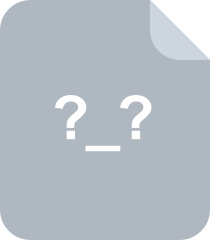
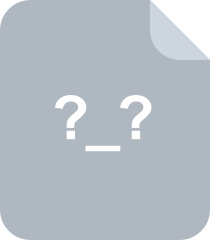
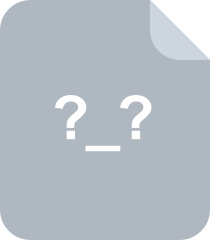
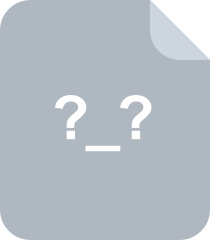
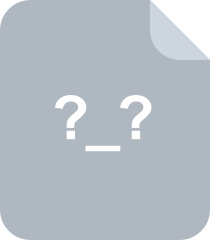
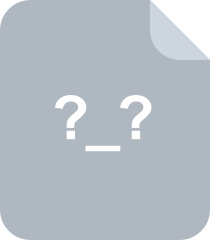
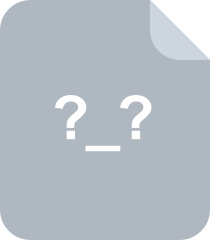
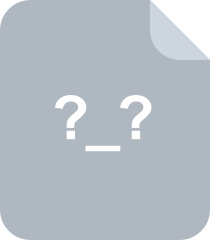
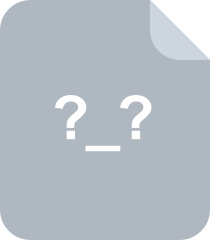
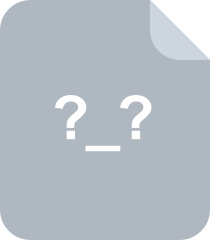
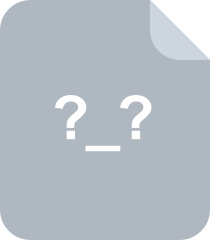
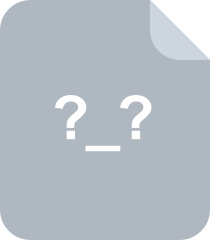
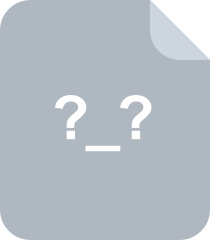
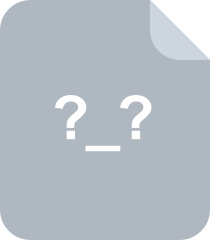
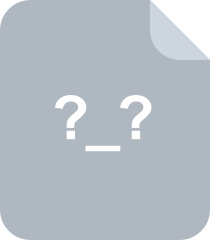
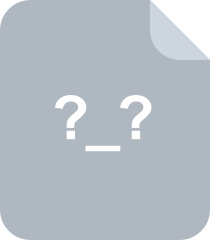
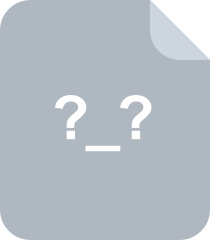
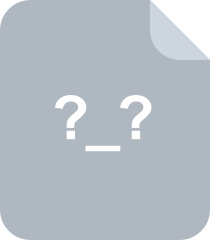
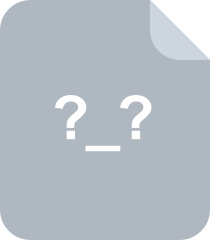
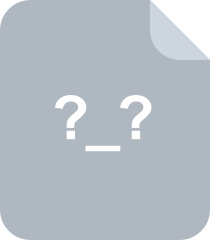
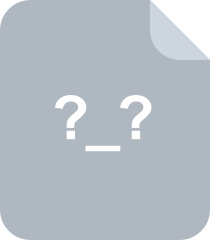
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
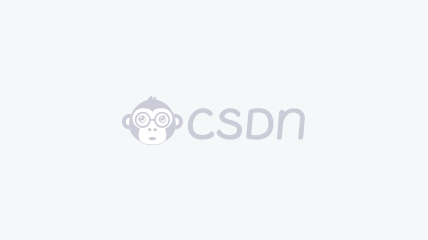

wjs2024
- 粉丝: 2236
- 资源: 5454
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

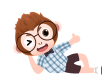
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


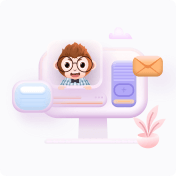
安全验证
文档复制为VIP权益,开通VIP直接复制
