var __create = Object.create;
var __defProp = Object.defineProperty;
var __getOwnPropDesc = Object.getOwnPropertyDescriptor;
var __getOwnPropNames = Object.getOwnPropertyNames;
var __getProtoOf = Object.getPrototypeOf;
var __hasOwnProp = Object.prototype.hasOwnProperty;
var __commonJS = (cb, mod) => function __require() {
return mod || (0, cb[__getOwnPropNames(cb)[0]])((mod = { exports: {} }).exports, mod), mod.exports;
};
var __export = (target, all) => {
for (var name in all)
__defProp(target, name, { get: all[name], enumerable: true });
};
var __copyProps = (to, from, except, desc) => {
if (from && typeof from === "object" || typeof from === "function") {
for (let key of __getOwnPropNames(from))
if (!__hasOwnProp.call(to, key) && key !== except)
__defProp(to, key, { get: () => from[key], enumerable: !(desc = __getOwnPropDesc(from, key)) || desc.enumerable });
}
return to;
};
var __toESM = (mod, isNodeMode, target) => (target = mod != null ? __create(__getProtoOf(mod)) : {}, __copyProps(
// If the importer is in node compatibility mode or this is not an ESM
// file that has been converted to a CommonJS file using a Babel-
// compatible transform (i.e. "__esModule" has not been set), then set
// "default" to the CommonJS "module.exports" for node compatibility.
isNodeMode || !mod || !mod.__esModule ? __defProp(target, "default", { value: mod, enumerable: true }) : target,
mod
));
// G:/web/考试系统/mock-exam/node_modules/boolbase/index.js
var require_boolbase = __commonJS({
"G:/web/考试系统/mock-exam/node_modules/boolbase/index.js"(exports, module) {
module.exports = {
trueFunc: function trueFunc2() {
return true;
},
falseFunc: function falseFunc() {
return false;
}
};
}
});
// G:/web/考试系统/mock-exam/node_modules/cheerio/lib/esm/options.js
var defaultOpts = {
xml: false,
decodeEntities: true
};
var options_default = defaultOpts;
var xmlModeDefault = {
_useHtmlParser2: true,
xmlMode: true
};
function flatten(options) {
return (options === null || options === void 0 ? void 0 : options.xml) ? typeof options.xml === "boolean" ? xmlModeDefault : { ...xmlModeDefault, ...options.xml } : options !== null && options !== void 0 ? options : void 0;
}
// G:/web/考试系统/mock-exam/node_modules/cheerio/lib/esm/static.js
var static_exports = {};
__export(static_exports, {
contains: () => contains,
html: () => html,
merge: () => merge,
parseHTML: () => parseHTML,
root: () => root,
text: () => text,
xml: () => xml
});
// G:/web/考试系统/mock-exam/node_modules/domutils/lib/esm/index.js
var esm_exports2 = {};
__export(esm_exports2, {
DocumentPosition: () => DocumentPosition,
append: () => append,
appendChild: () => appendChild,
compareDocumentPosition: () => compareDocumentPosition,
existsOne: () => existsOne,
filter: () => filter,
find: () => find,
findAll: () => findAll,
findOne: () => findOne,
findOneChild: () => findOneChild,
getAttributeValue: () => getAttributeValue,
getChildren: () => getChildren,
getElementById: () => getElementById,
getElements: () => getElements,
getElementsByTagName: () => getElementsByTagName,
getElementsByTagType: () => getElementsByTagType,
getFeed: () => getFeed,
getInnerHTML: () => getInnerHTML,
getName: () => getName,
getOuterHTML: () => getOuterHTML,
getParent: () => getParent,
getSiblings: () => getSiblings,
getText: () => getText,
hasAttrib: () => hasAttrib,
hasChildren: () => hasChildren,
innerText: () => innerText,
isCDATA: () => isCDATA,
isComment: () => isComment,
isDocument: () => isDocument,
isTag: () => isTag2,
isText: () => isText,
nextElementSibling: () => nextElementSibling,
prepend: () => prepend,
prependChild: () => prependChild,
prevElementSibling: () => prevElementSibling,
removeElement: () => removeElement,
removeSubsets: () => removeSubsets,
replaceElement: () => replaceElement,
testElement: () => testElement,
textContent: () => textContent,
uniqueSort: () => uniqueSort
});
// G:/web/考试系统/mock-exam/node_modules/domelementtype/lib/esm/index.js
var esm_exports = {};
__export(esm_exports, {
CDATA: () => CDATA,
Comment: () => Comment,
Directive: () => Directive,
Doctype: () => Doctype,
ElementType: () => ElementType,
Root: () => Root,
Script: () => Script,
Style: () => Style,
Tag: () => Tag,
Text: () => Text,
isTag: () => isTag
});
var ElementType;
(function(ElementType2) {
ElementType2["Root"] = "root";
ElementType2["Text"] = "text";
ElementType2["Directive"] = "directive";
ElementType2["Comment"] = "comment";
ElementType2["Script"] = "script";
ElementType2["Style"] = "style";
ElementType2["Tag"] = "tag";
ElementType2["CDATA"] = "cdata";
ElementType2["Doctype"] = "doctype";
})(ElementType || (ElementType = {}));
function isTag(elem) {
return elem.type === ElementType.Tag || elem.type === ElementType.Script || elem.type === ElementType.Style;
}
var Root = ElementType.Root;
var Text = ElementType.Text;
var Directive = ElementType.Directive;
var Comment = ElementType.Comment;
var Script = ElementType.Script;
var Style = ElementType.Style;
var Tag = ElementType.Tag;
var CDATA = ElementType.CDATA;
var Doctype = ElementType.Doctype;
// G:/web/考试系统/mock-exam/node_modules/domhandler/lib/esm/node.js
var Node = class {
constructor() {
this.parent = null;
this.prev = null;
this.next = null;
this.startIndex = null;
this.endIndex = null;
}
// Read-write aliases for properties
/**
* Same as {@link parent}.
* [DOM spec](https://dom.spec.whatwg.org)-compatible alias.
*/
get parentNode() {
return this.parent;
}
set parentNode(parent2) {
this.parent = parent2;
}
/**
* Same as {@link prev}.
* [DOM spec](https://dom.spec.whatwg.org)-compatible alias.
*/
get previousSibling() {
return this.prev;
}
set previousSibling(prev2) {
this.prev = prev2;
}
/**
* Same as {@link next}.
* [DOM spec](https://dom.spec.whatwg.org)-compatible alias.
*/
get nextSibling() {
return this.next;
}
set nextSibling(next2) {
this.next = next2;
}
/**
* Clone this node, and optionally its children.
*
* @param recursive Clone child nodes as well.
* @returns A clone of the node.
*/
cloneNode(recursive = false) {
return cloneNode(this, recursive);
}
};
var DataNode = class extends Node {
/**
* @param data The content of the data node
*/
constructor(data2) {
super();
this.data = data2;
}
/**
* Same as {@link data}.
* [DOM spec](https://dom.spec.whatwg.org)-compatible alias.
*/
get nodeValue() {
return this.data;
}
set nodeValue(data2) {
this.data = data2;
}
};
var Text2 = class extends DataNode {
constructor() {
super(...arguments);
this.type = ElementType.Text;
}
get nodeType() {
return 3;
}
};
var Comment2 = class extends DataNode {
constructor() {
super(...arguments);
this.type = ElementType.Comment;
}
get nodeType() {
return 8;
}
};
var ProcessingInstruction = class extends DataNode {
constructor(name, data2) {
super(data2);
this.name = name;
this.type = ElementType.Directive;
}
get nodeType() {
return 1;
}
};
var NodeWithChildren = class extends Node {
/**
* @param children Children of the node. Only certain node types can have children.
*/
constructor(children2) {
super();
this.children = children2;
}
// Aliases
/** First child of the node. */
get firstChild() {
var _a2;
return (_a2 = this.children[0]) !== null && _a2 !== void 0 ? _a2 : null;
}
/** Last child of the node. */
get lastChild() {
return this.children.length > 0 ? this.children[this.children.length - 1] : null;
}
/**
* Same as {@link children}.
* [DOM spec](https://dom.spec.whatwg.org)-compatible alias.
*/
get childNodes()
没有合适的资源?快使用搜索试试~ 我知道了~
基于JavaScript的Vue、CSS、HTML、TypeScript的模拟考试系统设计源码
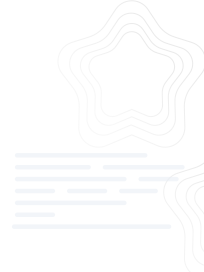
共549个文件
js:144个
vue:117个
json:98个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 61 浏览量
2024-09-29
20:14:00
上传
评论
收藏 5.96MB ZIP 举报
温馨提示
该项目是一款集JavaScript、Vue、CSS、HTML和TypeScript于一体的模拟考试系统源码,总计516个文件,涵盖144个JavaScript文件、110个Vue组件、103个Markdown文档、94个JSON配置文件、29个SCSS样式文件、14个PNG图片文件、6个JPG图片文件、5个CSS样式文件、3个字体文件以及3个WXS组件文件。此系统适用于模拟考试场景,能够提供全面的考试体验和功能支持。
资源推荐
资源详情
资源评论
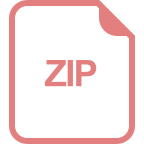
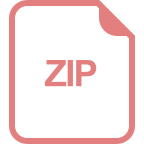
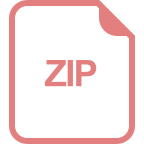
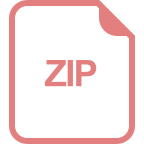
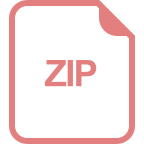
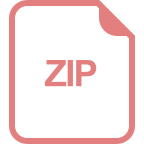
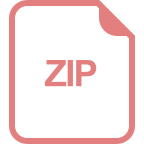
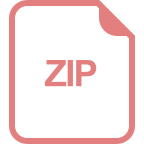
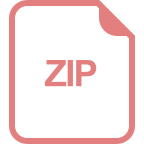
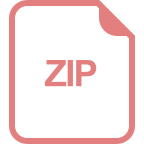
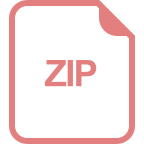
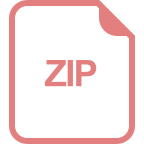
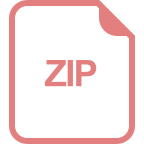
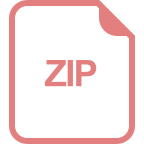
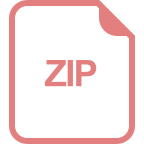
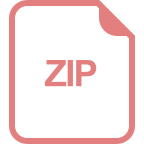
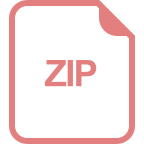
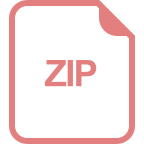
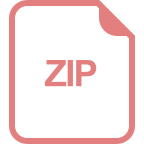
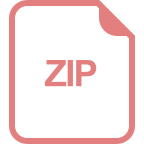
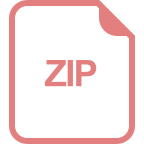
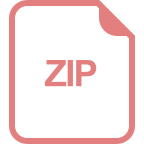
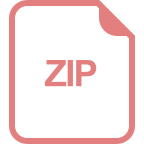
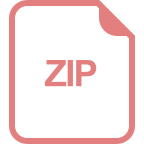
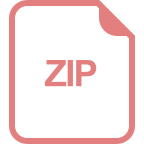
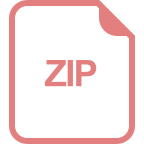
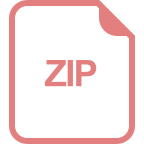
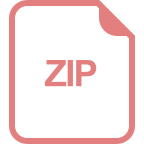
收起资源包目录

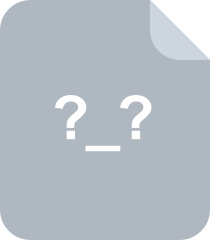
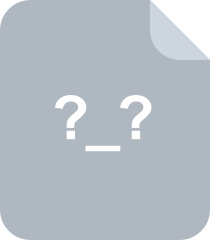
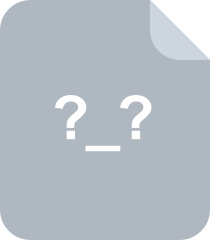
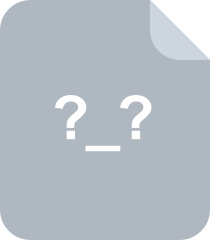
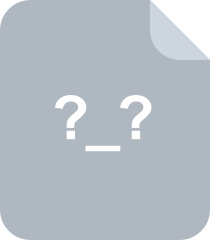
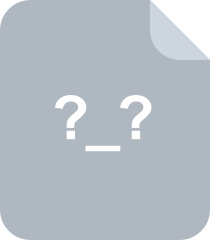
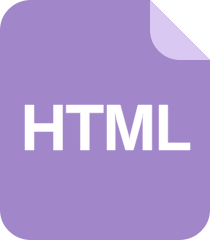
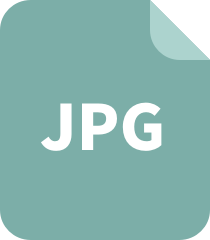
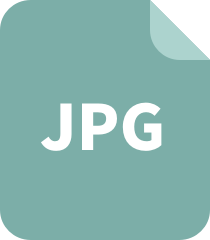
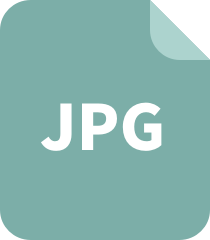
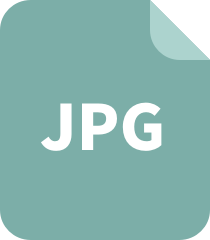
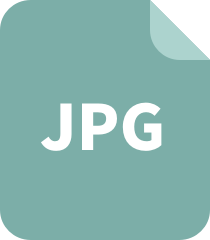
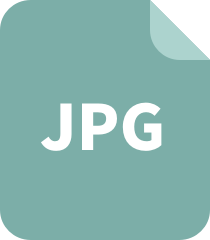
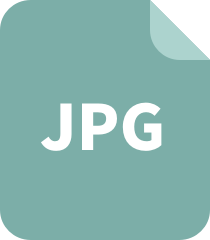
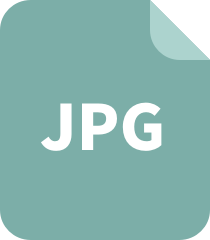
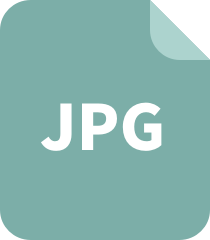
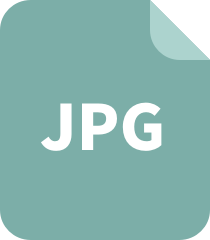
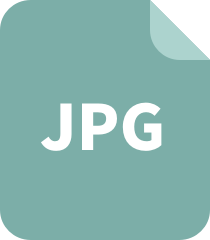
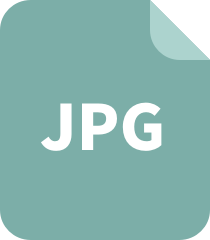
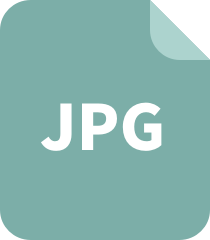
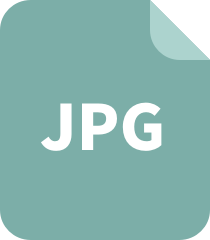
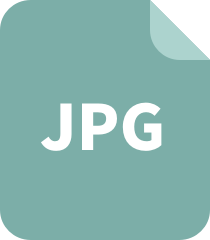
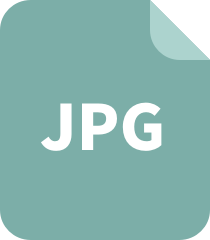
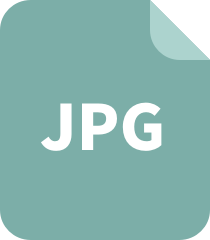
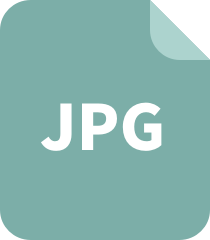
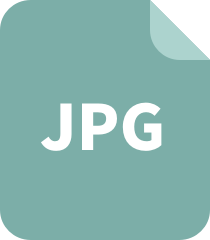
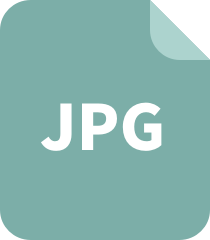
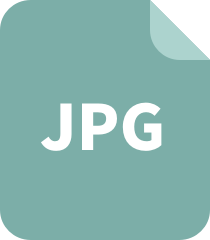
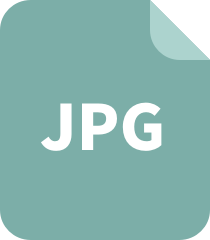
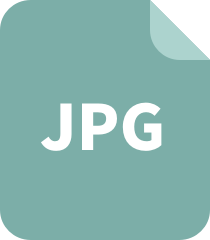
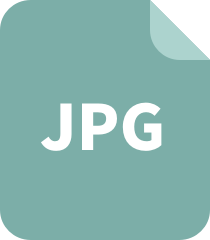
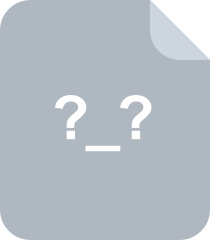
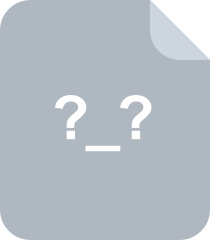
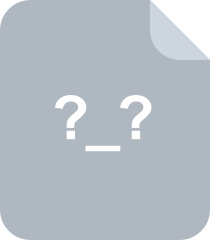
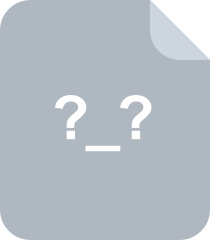
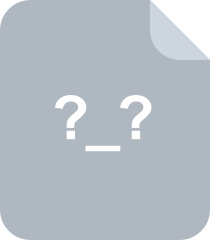
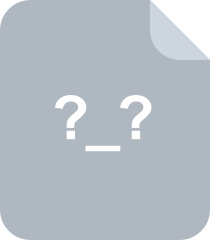
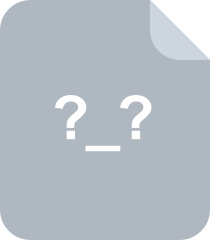
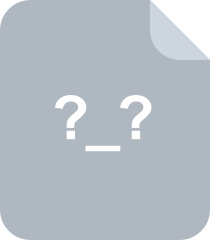
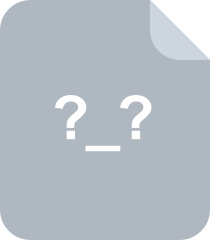
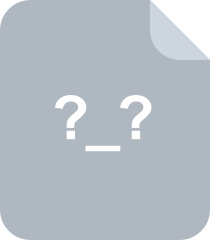
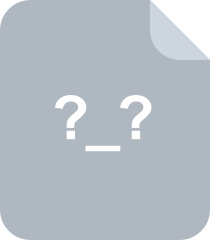
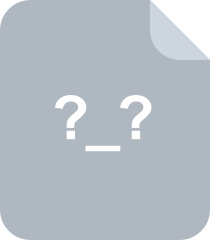
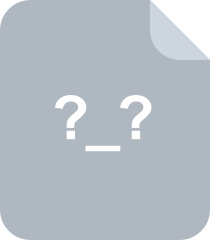
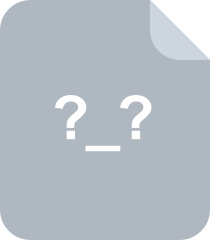
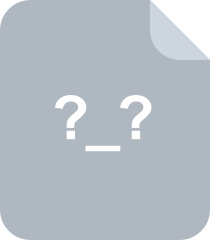
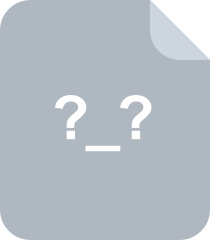
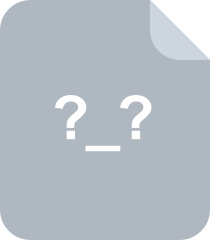
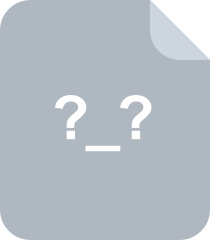
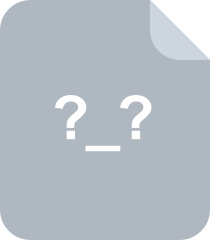
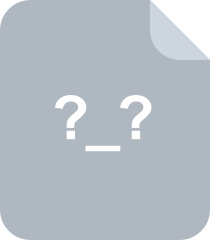
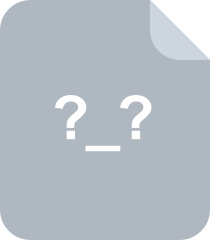
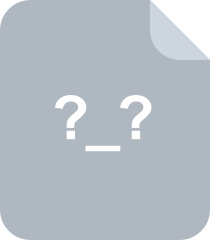
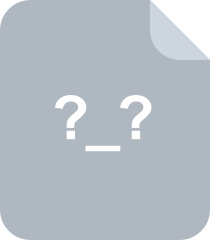
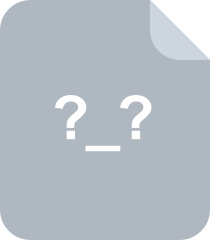
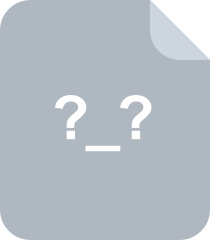
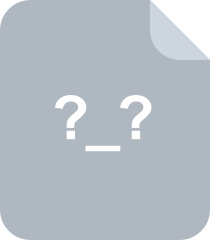
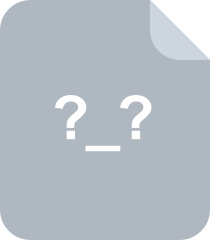
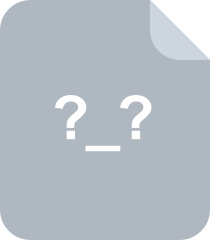
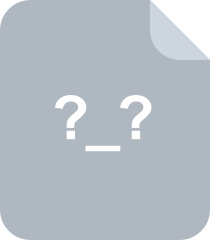
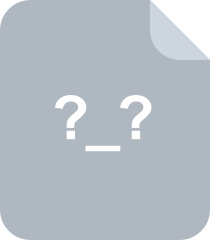
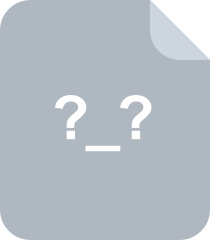
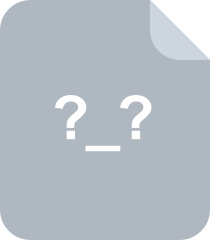
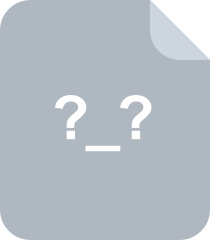
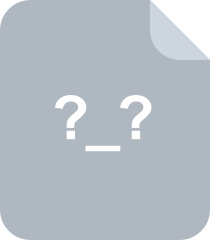
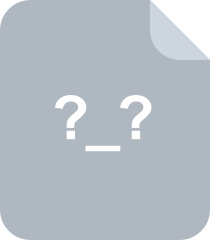
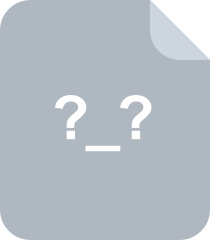
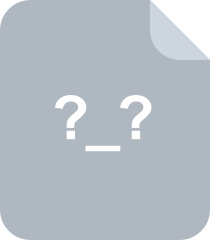
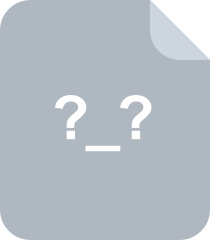
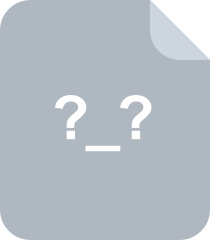
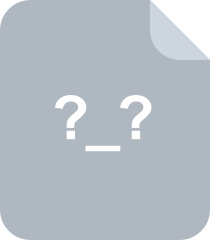
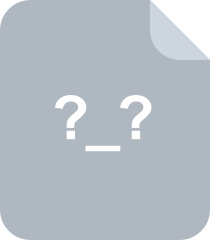
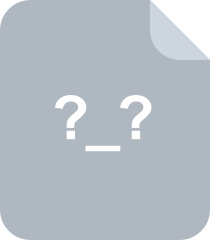
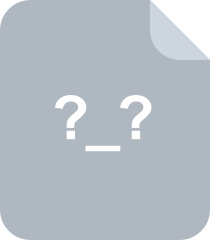
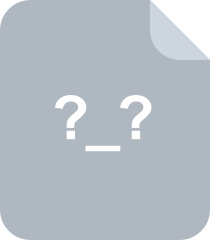
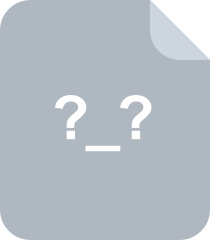
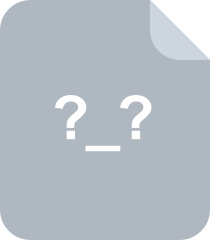
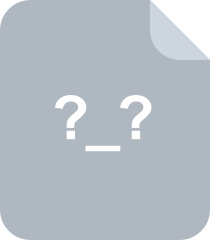
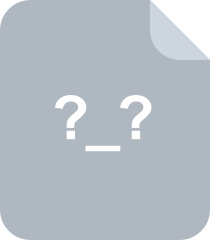
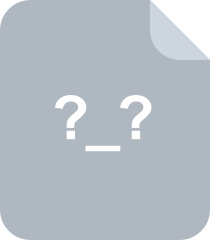
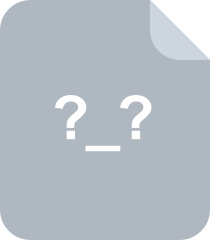
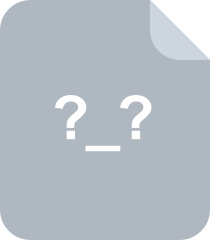
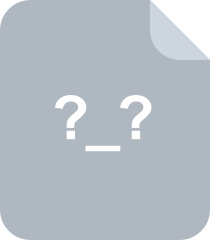
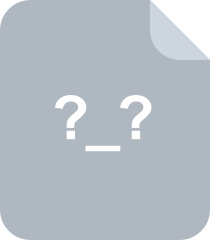
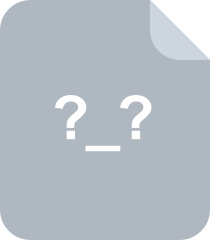
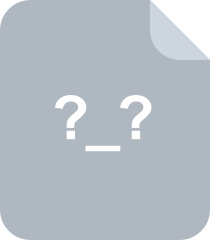
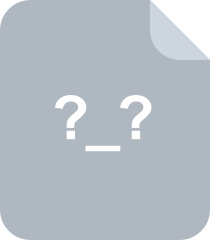
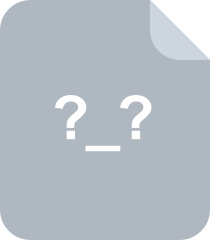
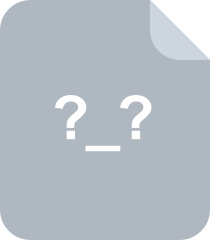
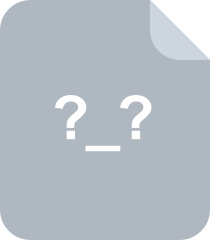
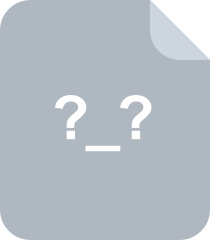
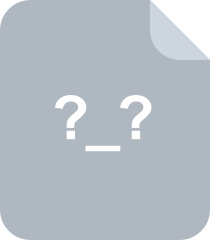
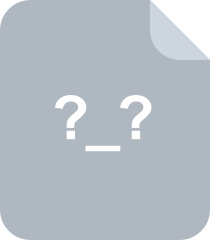
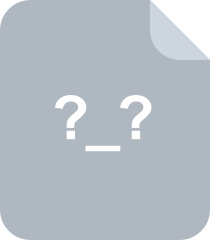
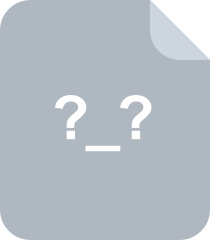
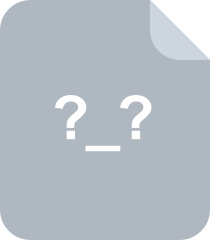
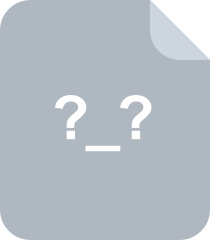
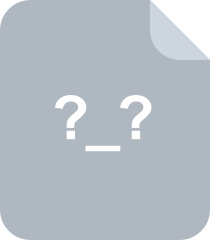
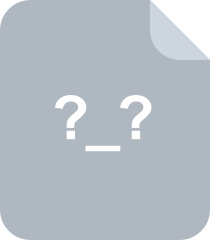
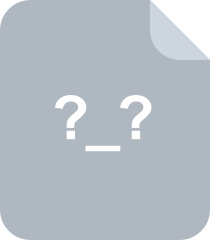
共 549 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
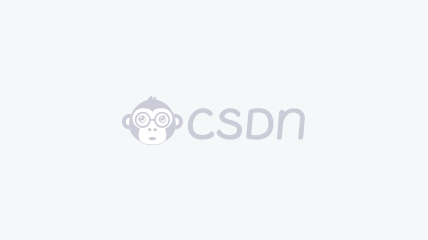

wjs2024
- 粉丝: 2421
- 资源: 5546
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

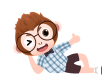
最新资源
- 【岗位说明】中药采购员岗位职责.doc
- 【岗位说明】中药士岗位职责.doc
- 【岗位说明】中药药剂师岗位职责.doc
- 【岗位说明】中药调剂员岗位职责.doc
- 【岗位说明】中药房工作人员岗位职责01.doc
- 【岗位说明】中药岗位职责01.doc
- 【岗位说明】最新医疗器械经营企业岗位职责.doc
- 【岗位说明】中医师岗位职责.doc
- 【岗位说明】主管药师工作职责.doc
- 【岗位说明】中医药工作制度.doc
- 【岗位说明】药剂人员岗位职责01.docx
- 【岗位说明】药师岗位说明书.docx
- 【岗位说明】医务科主任岗位说明书.docx
- 【岗位说明】中药师岗位说明书.docx
- 【岗位说明】中医科医师岗位职责.docx
- 【岗位说明】最新版中医针灸科岗位职责.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


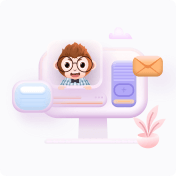
安全验证
文档复制为VIP权益,开通VIP直接复制
