package com.ikingtech.framework.sdk.utils;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.databind.json.JsonMapper;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.google.common.base.Joiner;
import com.google.common.base.Splitter;
import jakarta.servlet.http.HttpServletRequest;
import lombok.*;
import lombok.extern.slf4j.Slf4j;
import nl.basjes.parse.useragent.UserAgent;
import nl.basjes.parse.useragent.UserAgentAnalyzer;
import okhttp3.*;
import okhttp3.internal.sse.RealEventSource;
import okhttp3.sse.EventSource;
import okhttp3.sse.EventSourceListener;
import org.apache.velocity.VelocityContext;
import org.apache.velocity.tools.generic.DateTool;
import org.apache.velocity.tools.generic.MathTool;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.lionsoul.ip2region.xdb.Searcher;
import org.springframework.cglib.beans.BeanCopier;
import org.springframework.cglib.beans.BeanMap;
import org.springframework.cglib.core.Converter;
import org.springframework.scheduling.support.CronExpression;
import org.springframework.util.AntPathMatcher;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.util.StringUtils;
import javax.crypto.Cipher;
import javax.crypto.Mac;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.io.*;
import java.lang.reflect.InvocationTargetException;
import java.math.BigDecimal;
import java.net.URLEncoder;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.security.*;
import java.security.spec.InvalidKeySpecException;
import java.security.spec.PKCS8EncodedKeySpec;
import java.time.*;
import java.time.format.DateTimeFormatter;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ThreadLocalRandom;
import java.util.concurrent.TimeUnit;
import java.util.function.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
/**
* The type Tools.
*
* @author tie yan
*/
@NoArgsConstructor(access = AccessLevel.PRIVATE)
public class Tools {
/**
* 字符串处理
*/
@NoArgsConstructor(access = AccessLevel.PRIVATE)
public static class Str {
/**
* The constant EMPTY.
*/
public static final String EMPTY = "";
/**
* The constant COMMA.
*/
public static final String COMMA = ",";
/**
* The constant COLON.
*/
public static final String COLON = ":";
/**
* The constant SLASH.
*/
public static final String SLASH = "/";
/**
* The constant DEFAULT_PLACEHOLDER.
*/
public static final String DEFAULT_PLACEHOLDER = "{}";
/**
* The constant CONTENT_PLACEHOLDER_PREFIX.
*/
public static final String CONTENT_PLACEHOLDER_PREFIX = "${";
/**
* The constant CONTENT_PLACEHOLDER_SUFFIX.
*/
public static final String CONTENT_PLACEHOLDER_SUFFIX = "}";
/**
* Equals boolean.
*
* @param str1 the str 1
* @param str2 the str 2
* @return the boolean
*/
public static boolean equals(String str1, String str2) {
if (null == str1) {
return str2 == null;
} else if (null == str2) {
return false;
} else {
return str1.equalsIgnoreCase(str2);
}
}
/**
* Is blank boolean.
*
* @param str the str
* @return the boolean
*/
public static boolean isBlank(String str) {
if (str == null || str.isEmpty()) {
return true;
} else {
for (int i = 0; i < str.length(); i++) {
if (!Character.isWhitespace(str.charAt(i))) {
return false;
}
}
}
return true;
}
/**
* Is not blank boolean.
*
* @param str the str
* @return the boolean
*/
public static boolean isNotBlank(String str) {
if (str == null || str.isEmpty()) {
return false;
} else {
for (int i = 0; i < str.length(); i++) {
if (!Character.isWhitespace(str.charAt(i))) {
return true;
}
}
}
return false;
}
/**
* Is string.
*
* @param origin the origin
* @return the string
*/
public static String is(String origin) {
return isBlank(origin) ? EMPTY : origin;
}
/**
* Is string.
*
* @param origin the origin
* @param defaultStr the default str
* @return the string
*/
public static String is(String origin, String defaultStr) {
return isBlank(origin) ? defaultStr : origin;
}
/**
* Replace string.
*
* @param str the str
* @param placeHolderPrefix the place holder prefix
* @param placeHolderSuffix the place holder suffix
* @param fillStrMap the fill str map
* @return the string
*/
public static String replace(String str, String placeHolderPrefix, String placeHolderSuffix, Map<String, Object> fillStrMap) {
if (isBlank(str)) {
return str;
}
if (!str.contains(placeHolderPrefix) || !str.contains(placeHolderSuffix)) {
return str;
}
do {
String param = str.substring(str.indexOf(placeHolderPrefix) + placeHolderPrefix.length(), str.indexOf(placeHolderSuffix));
str = str.replace(placeHolderPrefix + param + placeHolderSuffix,
fillStrMap.containsKey(param) && null != fillStrMap.get(param) ?
(CharSequence) fillStrMap.get(param) : Str.EMPTY);
} while (str.contains(placeHolderPrefix));
return str;
}
/**
* Format string.
*
* @param template the template
* @param params the params
* @return the string
*/
public static String format(String template, List<String> params) {
if (null == template || template.isEmpty()) {
return "";
}
if (!template.contains(DEFAULT_PLACEHOLDER)) {
return template;
}
StringBuilder builder = new StringBuilder(template.length() + 16 * params.size());
int templateStart = 0;
int i = 0;
while (i < params.size()) {
int placeholderStart = template.indexOf(DEFAULT_PLACEHOLDER, templateStart);
if (placeholderStart == -1) {
break;
}
builder.append(template, templateStart, placeholderStart);
builder.append(params.get(i++));
templateStart = placeholderStart + 2;
}
builder.append(template, templateStart, template.length());
return builder.toString();
}
/**
* Format string.
*
* @param template the template
* @param params the params
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本项目是一套基于Java核心并融入Lua扩展功能的现代化企业级技术中台源码,总计包含1470个文件,其中Java源文件1154个,XML配置文件183个,其他文件类型涵盖YML、PNG、SQL、属性文件等多种格式。该中台设计简洁、高效、稳定且开放,欢迎贡献Star和Pull Request。
资源推荐
资源详情
资源评论
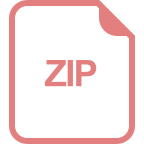
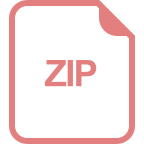
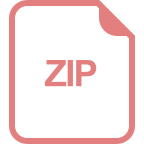
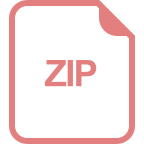
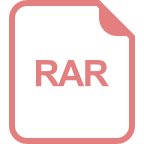
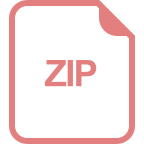
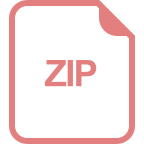
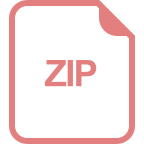
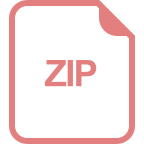
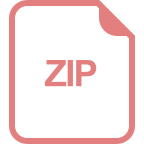
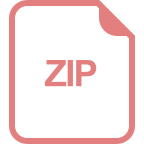
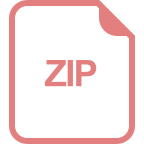
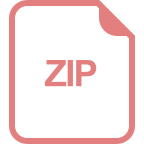
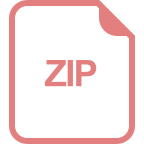
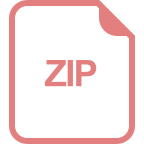
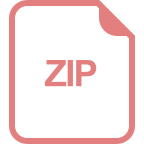
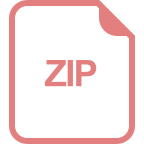
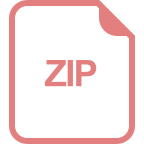
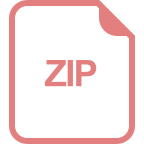
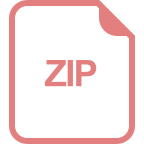
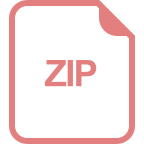
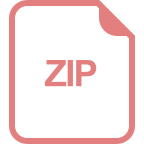
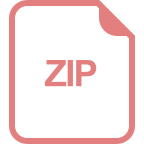
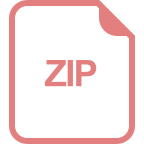
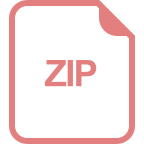
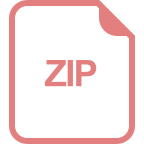
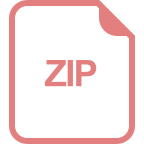
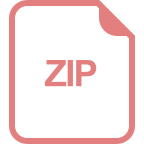
收起资源包目录

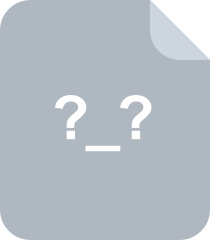
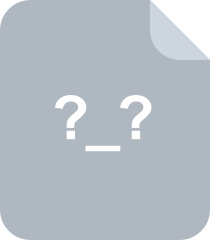
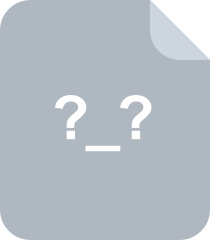
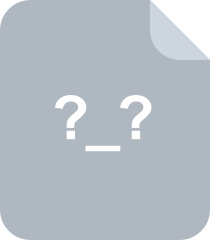
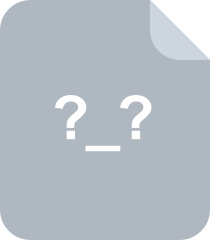
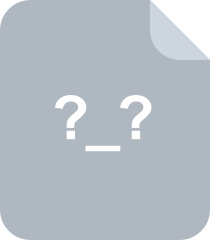
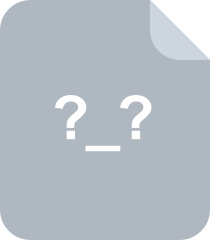
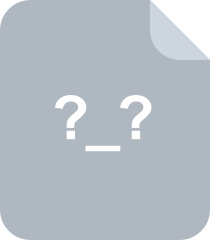
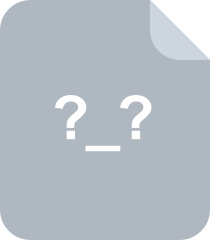
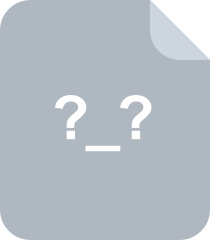
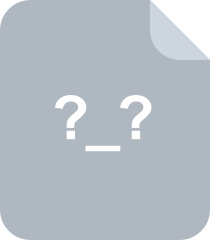
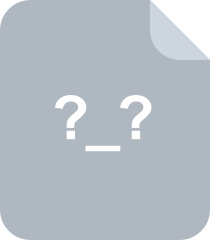
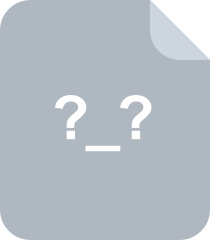
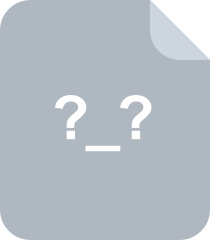
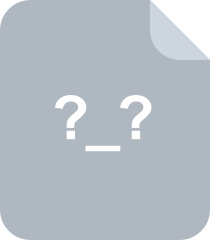
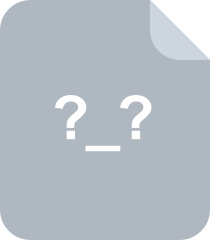
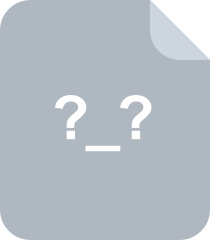
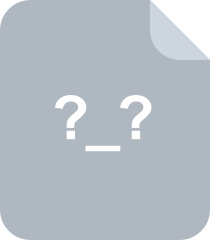
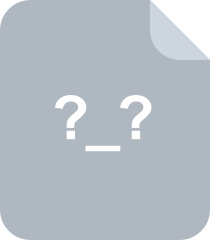
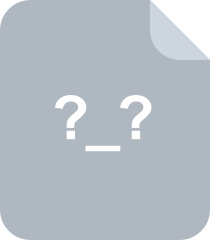
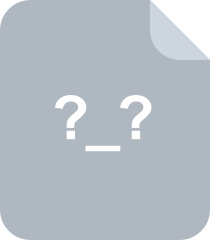
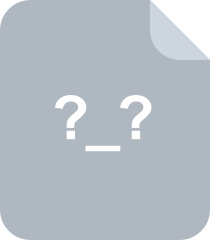
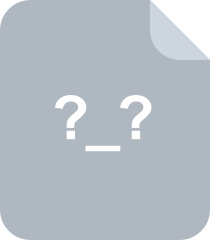
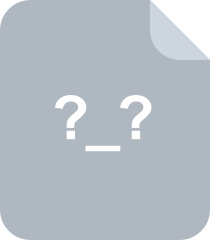
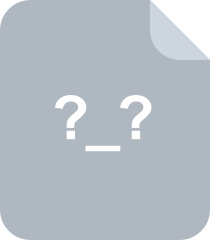
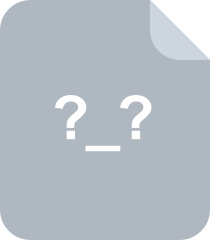
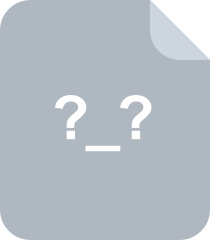
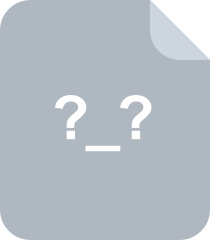
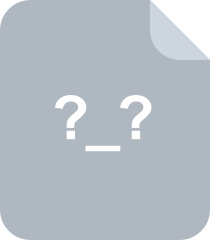
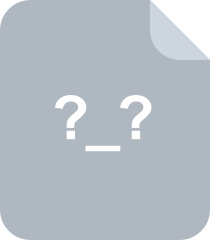
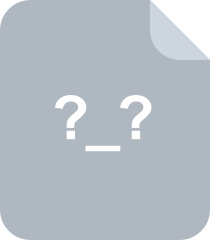
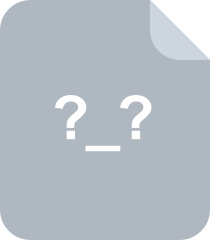
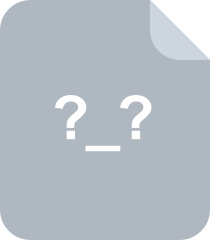
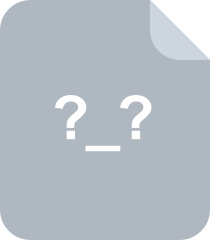
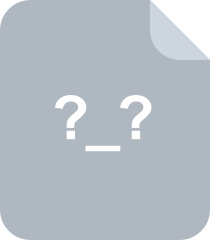
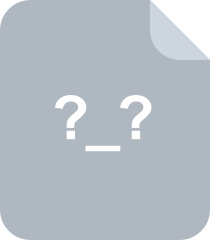
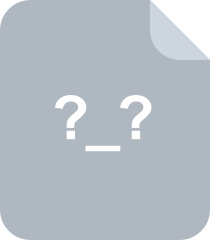
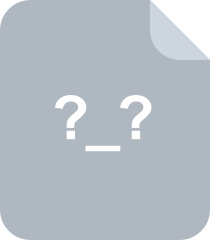
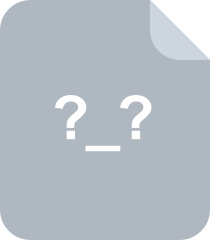
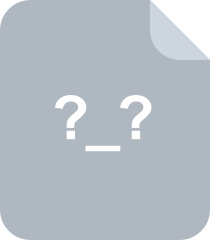
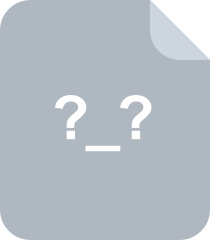
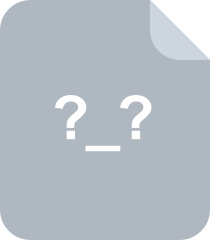
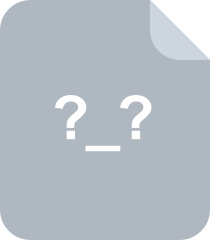
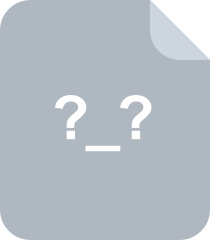
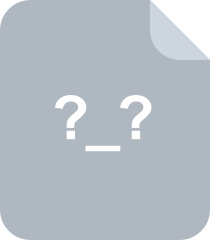
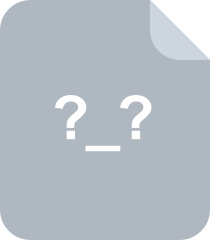
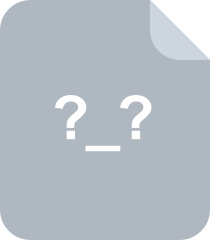
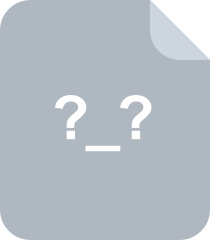
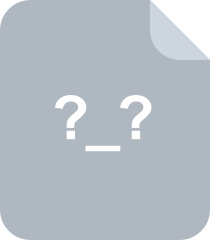
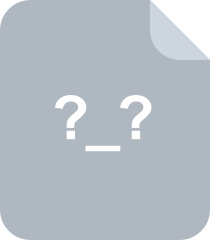
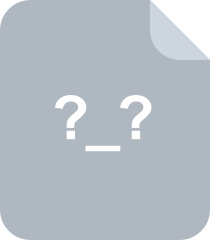
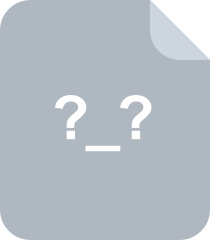
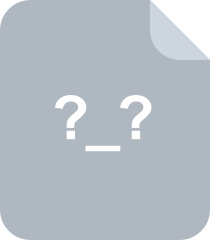
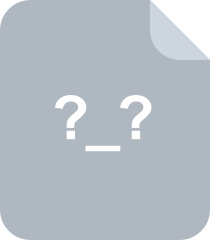
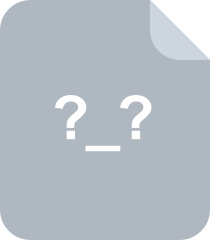
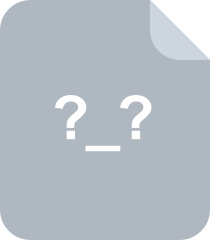
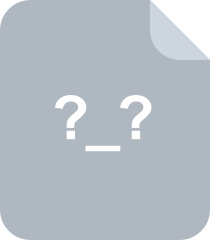
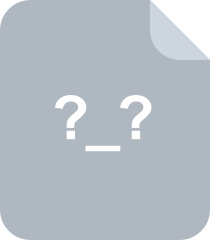
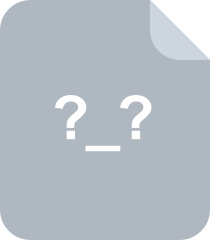
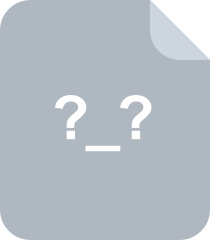
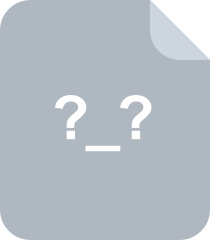
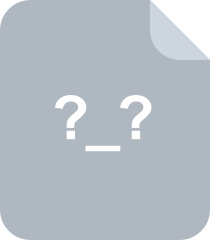
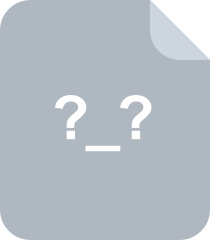
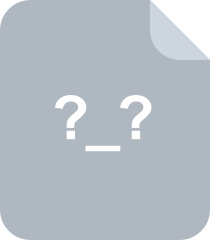
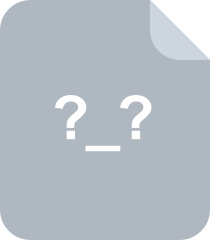
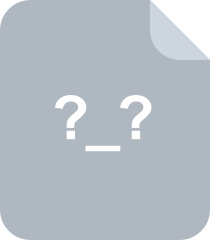
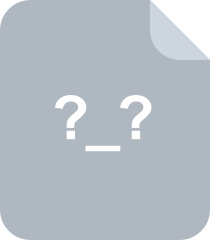
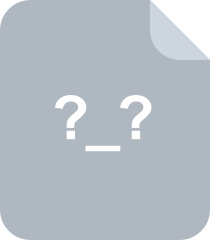
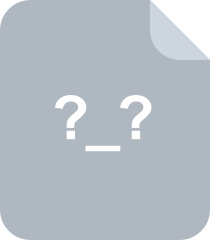
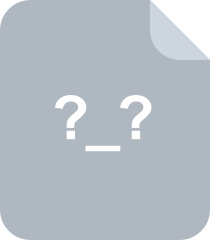
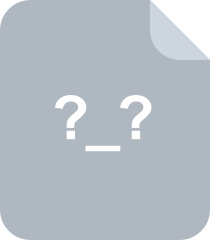
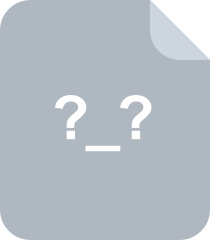
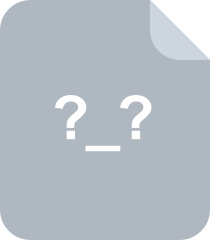
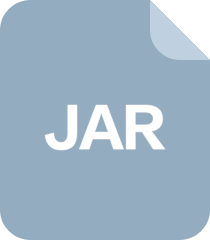
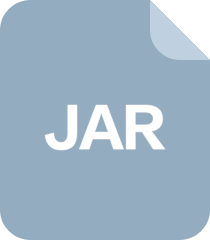
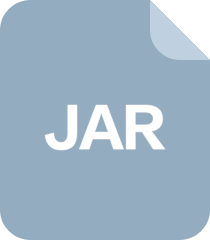
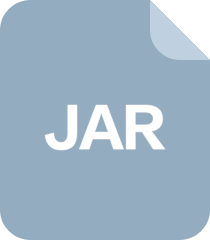
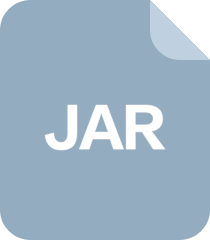
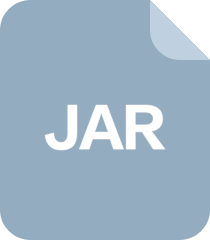
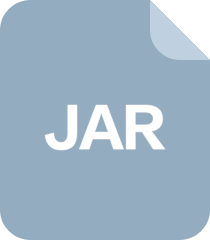
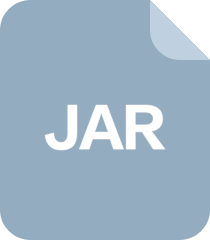
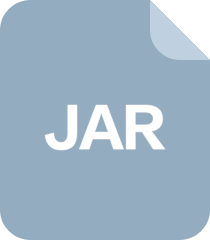
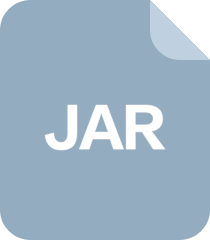
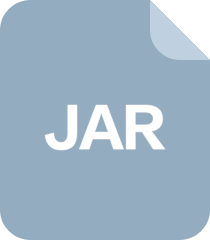
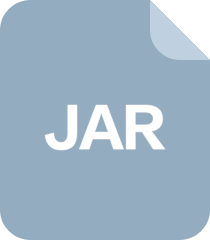
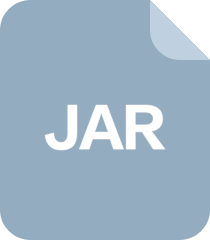
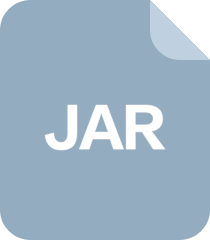
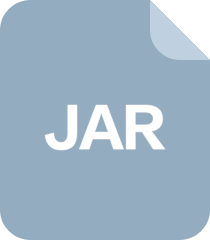
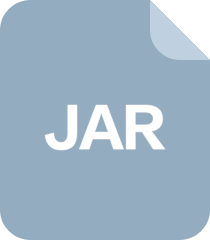
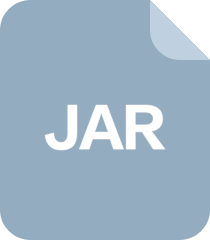
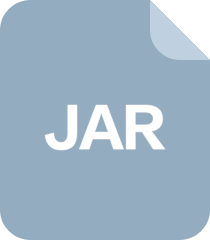
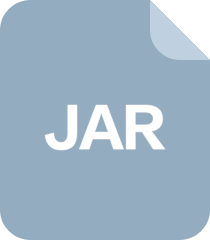
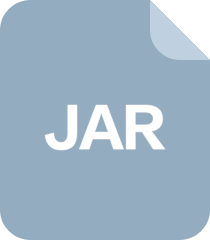
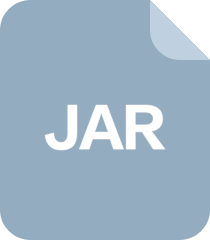
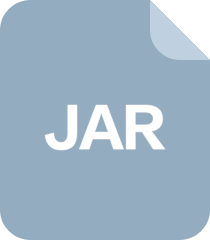
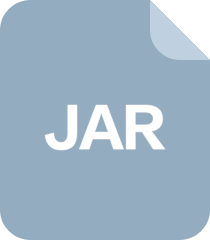
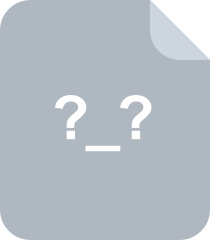
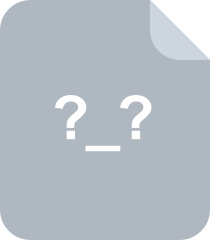
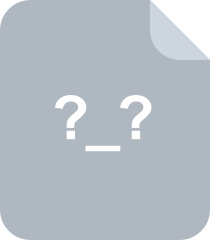
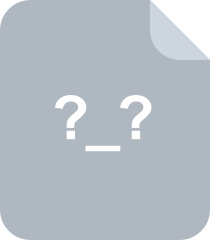
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
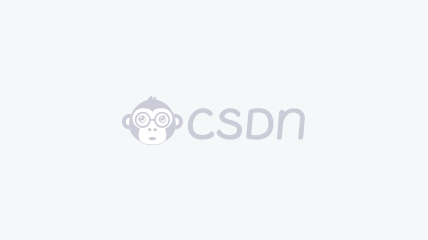

wjs2024
- 粉丝: 2230
- 资源: 5451
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

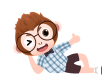
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


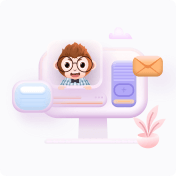
安全验证
文档复制为VIP权益,开通VIP直接复制
