/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package org.apache.cordova.contacts;
import android.accounts.Account;
import android.accounts.AccountManager;
import android.content.ContentProviderOperation;
import android.content.ContentProviderResult;
import android.content.ContentUris;
import android.content.ContentValues;
import android.content.OperationApplicationException;
import android.database.Cursor;
import android.net.Uri;
import android.os.RemoteException;
import android.provider.ContactsContract;
import android.util.Log;
import org.apache.cordova.CordovaInterface;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.ByteArrayOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
//import android.app.Activity;
//import android.content.Context;
/**
* An implementation of {@link org.apache.cordova.contacts.ContactAccessor} that uses current Contacts API.
* This class should be used on Eclair or beyond, but would not work on any earlier
* release of Android. As a matter of fact, it could not even be loaded.
* <p>
* This implementation has several advantages:
* <ul>
* <li>It sees contacts from multiple accounts.
* <li>It works with aggregated contacts. So for example, if the contact is the result
* of aggregation of two raw contacts from different accounts, it may return the name from
* one and the phone number from the other.
* <li>It is efficient because it uses the more efficient current API.
* <li>Not obvious in this particular example, but it has access to new kinds
* of data available exclusively through the new APIs. Exercise for the reader: add support
* for nickname (see {@link android.provider.ContactsContract.CommonDataKinds.Nickname}) or
* social status updates (see {@link android.provider.ContactsContract.StatusUpdates}).
* </ul>
*/
public class ContactAccessorSdk5 extends ContactAccessor {
/**
* Keep the photo size under the 1 MB blog limit.
*/
private static final long MAX_PHOTO_SIZE = 1048576;
private static final String EMAIL_REGEXP = ".+@.+\\.+.+"; /* <anything>@<anything>.<anything>*/
/**
* A static map that converts the JavaScript property name to Android database column name.
*/
private static final Map<String, String> dbMap = new HashMap<String, String>();
static {
dbMap.put("id", ContactsContract.Data.CONTACT_ID);
dbMap.put("displayName", ContactsContract.Contacts.DISPLAY_NAME);
dbMap.put("name", ContactsContract.CommonDataKinds.StructuredName.DISPLAY_NAME);
dbMap.put("name.formatted", ContactsContract.CommonDataKinds.StructuredName.DISPLAY_NAME);
dbMap.put("name.familyName", ContactsContract.CommonDataKinds.StructuredName.FAMILY_NAME);
dbMap.put("name.givenName", ContactsContract.CommonDataKinds.StructuredName.GIVEN_NAME);
dbMap.put("name.middleName", ContactsContract.CommonDataKinds.StructuredName.MIDDLE_NAME);
dbMap.put("name.honorificPrefix", ContactsContract.CommonDataKinds.StructuredName.PREFIX);
dbMap.put("name.honorificSuffix", ContactsContract.CommonDataKinds.StructuredName.SUFFIX);
dbMap.put("nickname", ContactsContract.CommonDataKinds.Nickname.NAME);
dbMap.put("phoneNumbers", ContactsContract.CommonDataKinds.Phone.NUMBER);
dbMap.put("phoneNumbers.value", ContactsContract.CommonDataKinds.Phone.NUMBER);
dbMap.put("emails", ContactsContract.CommonDataKinds.Email.DATA);
dbMap.put("emails.value", ContactsContract.CommonDataKinds.Email.DATA);
dbMap.put("addresses", ContactsContract.CommonDataKinds.StructuredPostal.FORMATTED_ADDRESS);
dbMap.put("addresses.formatted", ContactsContract.CommonDataKinds.StructuredPostal.FORMATTED_ADDRESS);
dbMap.put("addresses.streetAddress", ContactsContract.CommonDataKinds.StructuredPostal.STREET);
dbMap.put("addresses.locality", ContactsContract.CommonDataKinds.StructuredPostal.CITY);
dbMap.put("addresses.region", ContactsContract.CommonDataKinds.StructuredPostal.REGION);
dbMap.put("addresses.postalCode", ContactsContract.CommonDataKinds.StructuredPostal.POSTCODE);
dbMap.put("addresses.country", ContactsContract.CommonDataKinds.StructuredPostal.COUNTRY);
dbMap.put("ims", ContactsContract.CommonDataKinds.Im.DATA);
dbMap.put("ims.value", ContactsContract.CommonDataKinds.Im.DATA);
dbMap.put("organizations", ContactsContract.CommonDataKinds.Organization.COMPANY);
dbMap.put("organizations.name", ContactsContract.CommonDataKinds.Organization.COMPANY);
dbMap.put("organizations.department", ContactsContract.CommonDataKinds.Organization.DEPARTMENT);
dbMap.put("organizations.title", ContactsContract.CommonDataKinds.Organization.TITLE);
dbMap.put("birthday", ContactsContract.CommonDataKinds.Event.CONTENT_ITEM_TYPE);
dbMap.put("note", ContactsContract.CommonDataKinds.Note.NOTE);
dbMap.put("photos.value", ContactsContract.CommonDataKinds.Photo.CONTENT_ITEM_TYPE);
//dbMap.put("categories.value", null);
dbMap.put("urls", ContactsContract.CommonDataKinds.Website.URL);
dbMap.put("urls.value", ContactsContract.CommonDataKinds.Website.URL);
}
/**
* Create an contact accessor.
*/
public ContactAccessorSdk5(CordovaInterface context) {
mApp = context;
}
/**
* This method takes the fields required and search options in order to produce an
* array of contacts that matches the criteria provided.
* @param fields an array of items to be used as search criteria
* @param options that can be applied to contact searching
* @return an array of contacts
*/
@Override
public JSONArray search(JSONArray fields, JSONObject options) {
// Get the find options
String searchTerm = "";
int limit = Integer.MAX_VALUE;
boolean multiple = true;
if (options != null) {
searchTerm = options.optString("filter");
if (searchTerm.length() == 0) {
searchTerm = "%";
}
else {
searchTerm = "%" + searchTerm + "%";
}
try {
multiple = options.getBoolean("multiple");
if (!multiple) {
limit = 1;
}
} catch (JSONException e) {
// Multiple was not specified so we assume the default is true.
}
}
else {
searchTerm = "%";
}
//Log.d(LOG_TAG, "Search Term = " + searchTerm);
//Log.d(LOG_TAG, "Field Length = " + fields.length());
//Log.d(LOG_TAG, "Fields = " + fields.toString());
// Loop through the fields the user provided to see what data should be returned.
HashMap<String, Boolean> populate = buildPopulatio
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本项目为基于IntelliJ IDEA的变色龙Android项目v2设计源码,采用Gradle构建系统(Maven依赖管理),包含671个文件,涉及204个Java源代码文件、149个PNG图像资源、122个XML配置文件、95个JavaScript文件、18个CSS样式表、13个HTML页面、10个JPEG图像、8个Git忽略文件、8个Gradle配置文件、7个文本文件和少量其他类型文件。项目以Java为主要编程语言,辅以JavaScript、CSS和HTML,支持Android平台开发。
资源推荐
资源详情
资源评论
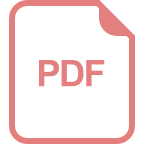
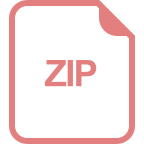
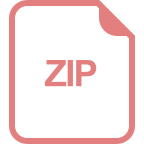
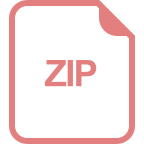
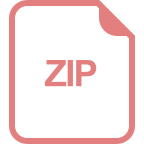
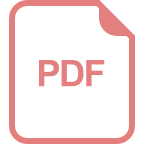
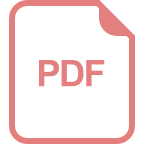
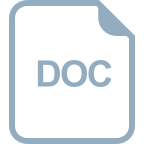
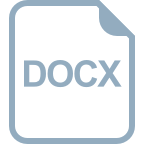
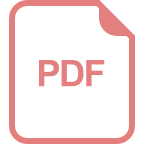
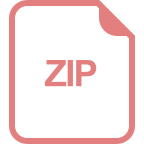
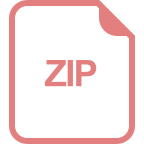
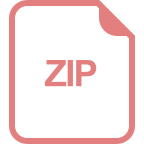
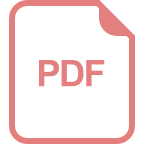
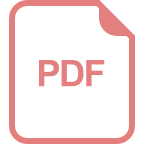
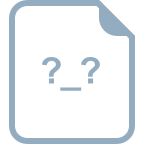
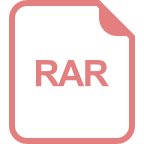
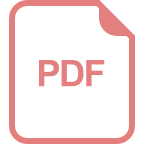
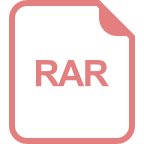
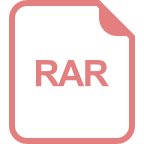
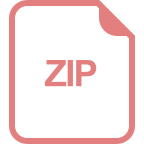
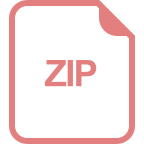
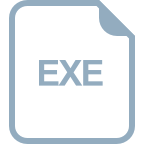
收起资源包目录

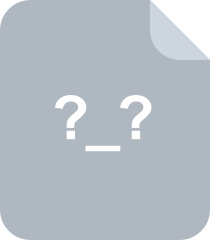
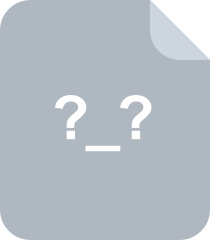
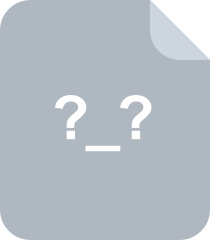
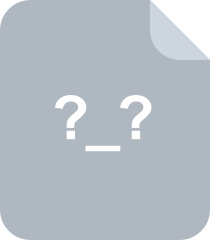
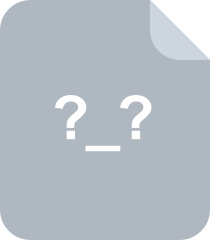
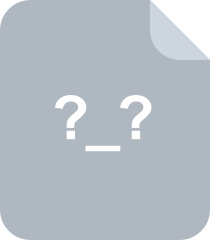
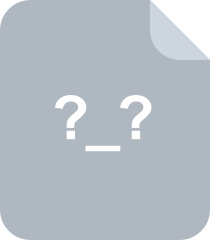
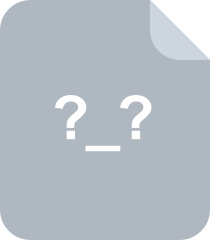
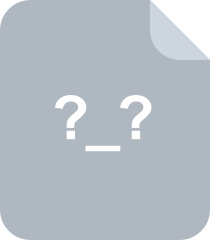
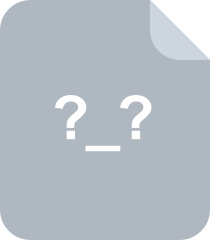
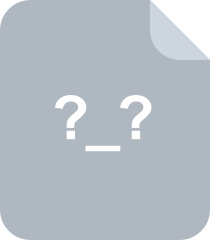
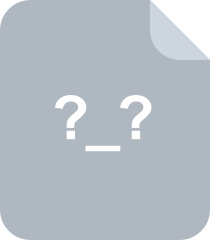
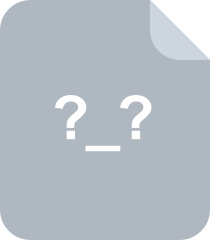
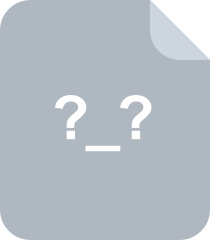
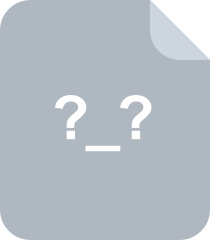
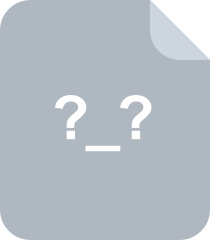
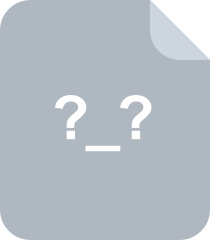
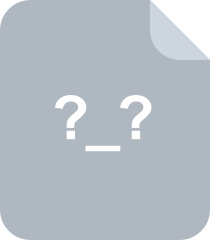
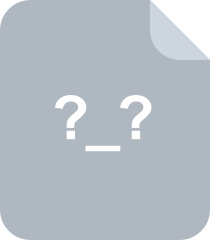
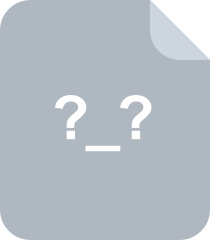
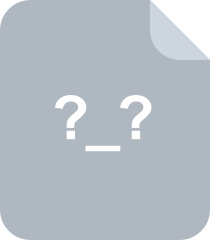
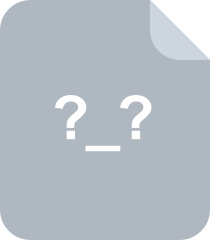
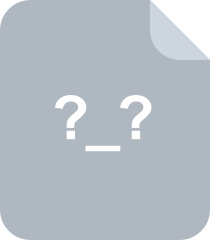
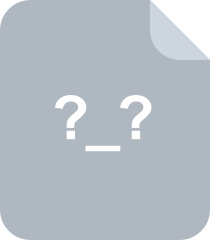
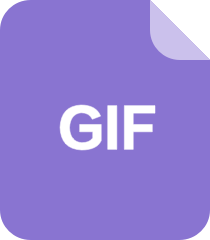
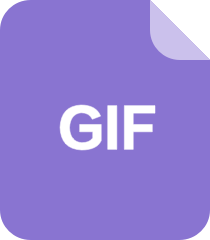
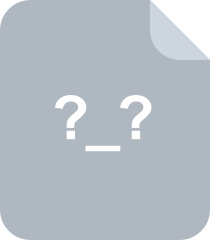
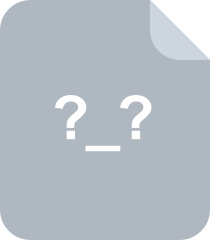
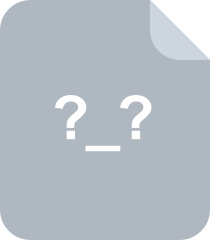
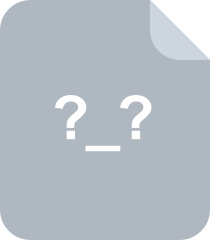
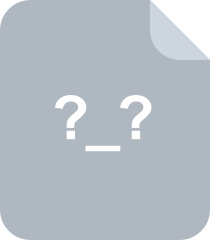
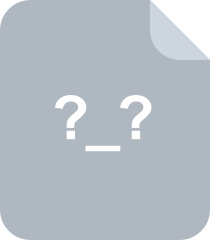
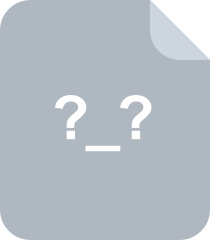
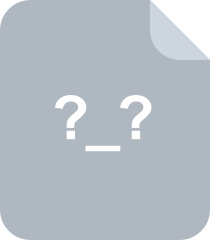
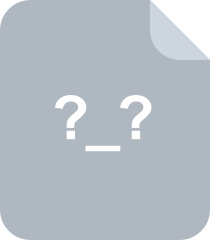
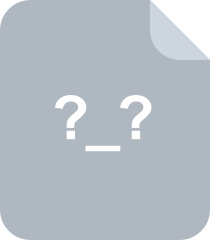
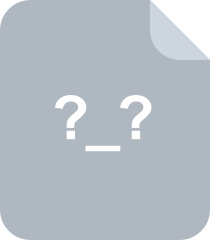
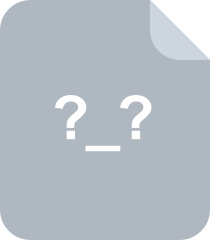
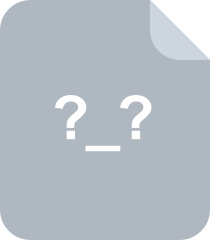
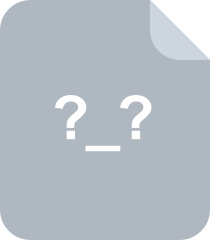
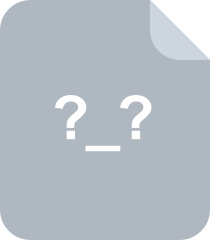
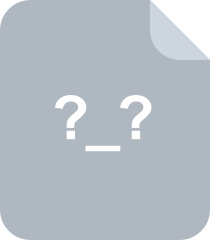
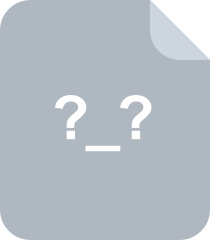
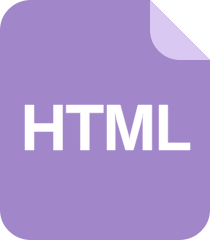
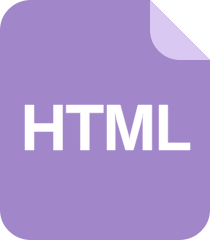
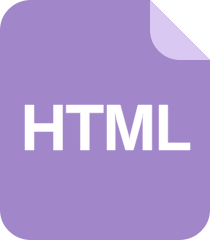
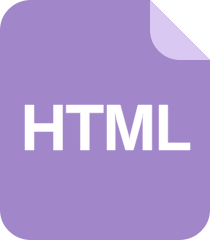
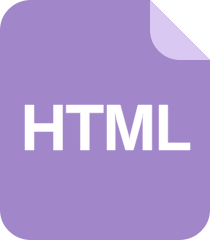
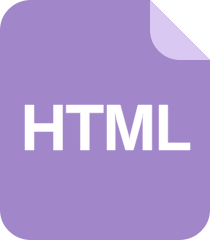
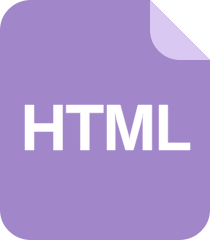
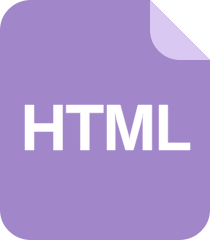
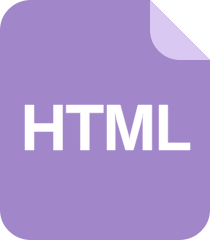
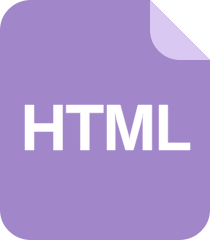
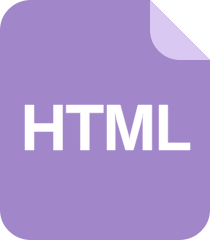
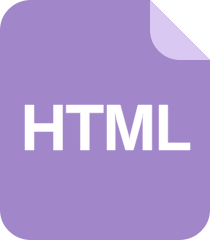
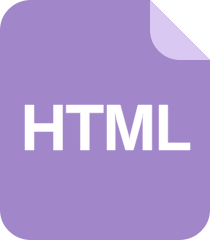
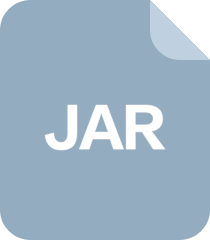
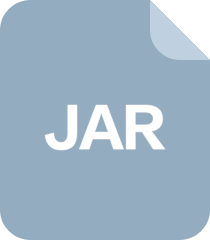
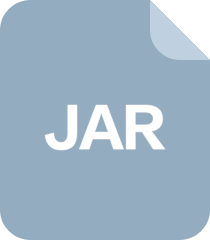
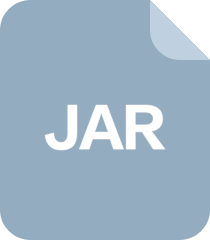
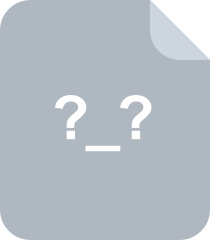
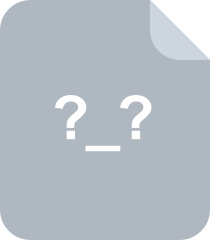
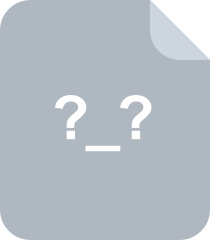
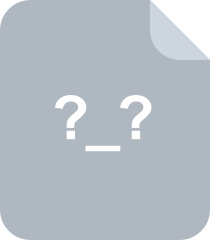
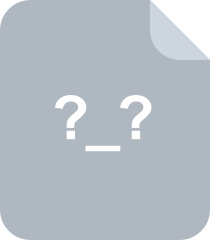
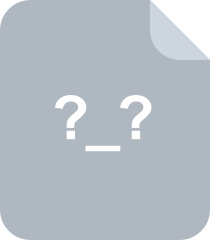
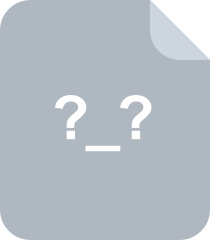
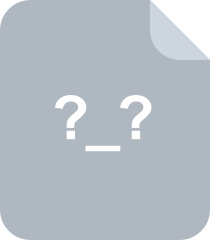
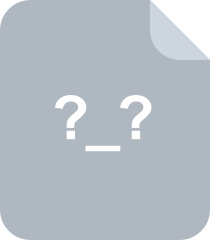
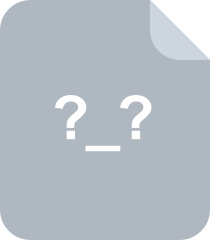
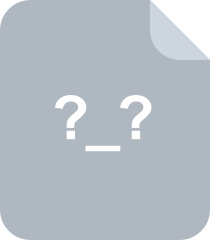
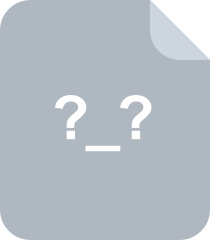
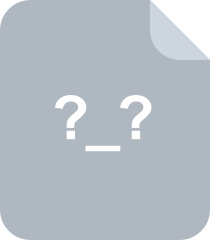
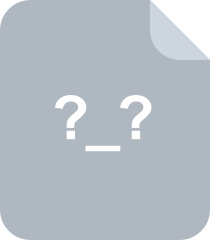
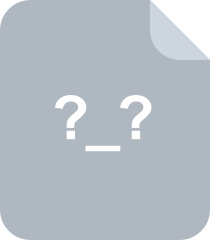
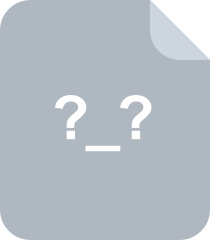
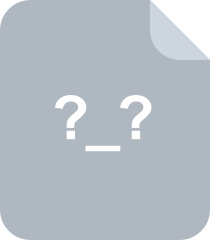
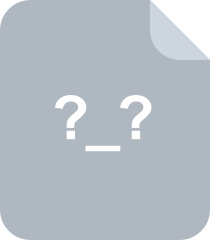
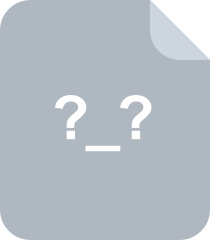
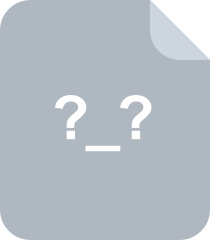
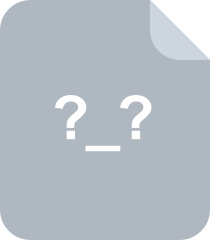
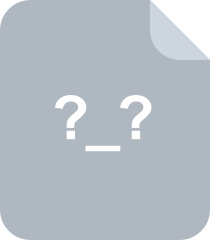
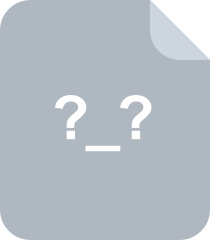
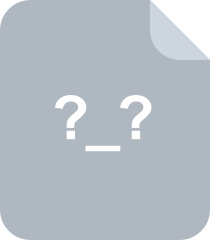
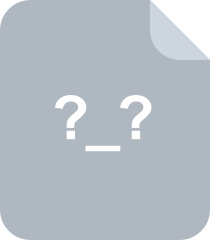
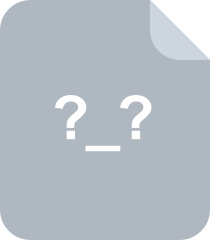
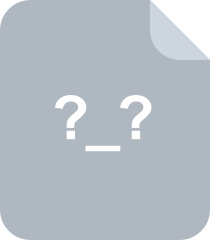
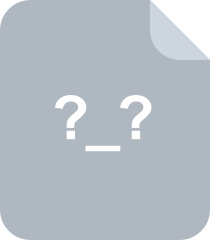
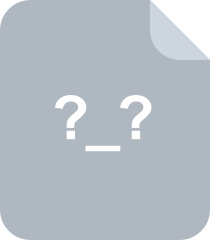
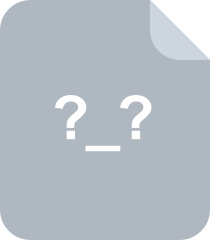
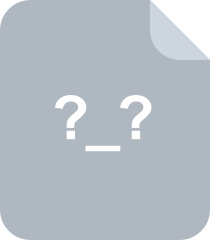
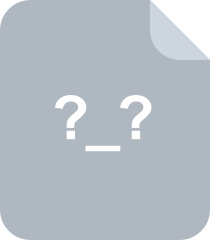
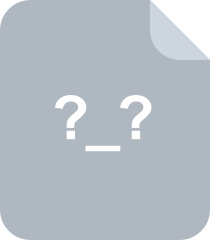
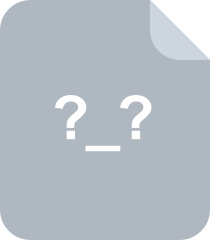
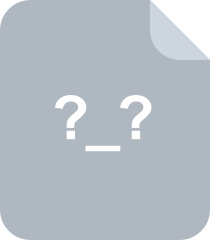
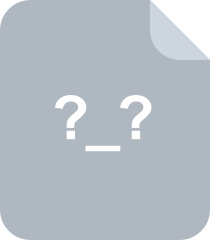
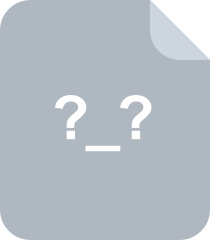
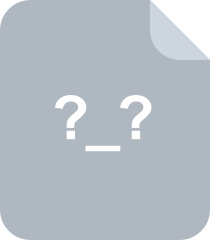
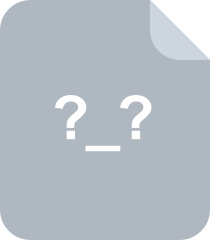
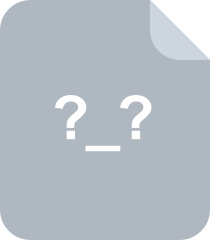
共 671 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
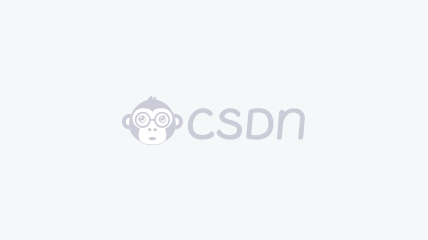

wjs2024
- 粉丝: 2370
- 资源: 5538
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

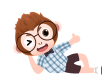
最新资源
- 基于Node.js和WebSocket的音频数据流分析音乐节奏展示设计源码
- 基于Surface框架的CURD和后台页面快速搭建设计源码
- 基于Snowflake算法的分布式唯一ID生成器UidGenerator在SpringBoot中的整合与应用设计源码
- 四轴直交机械手工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 基于Java语言的RabbitMQ精品课程设计源码
- 四合一测试设备(含bom)sw17可编辑工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 基于SSM框架和JavaScript的教材管理系统设计源码
- 基于JqueryMobile框架的kLink通讯录应用设计源码
- 基于2024暑假鸿蒙应用师资班培训的TeachObject20240715_01设计源码
- 卧式气动膏体灌装机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 基于Vue的JavaScript光雨电子书后台源码
- 基于山东大学经验的转专业学生攻略设计源码
- 基于51单片机的蓝牙循迹小车设计源码
- Teaching Small Language Models to Reason 小模型如何在大模型中生效
- 基于Html和Ruby语言的test项目设计源码
- 线材激光焊接裁断机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


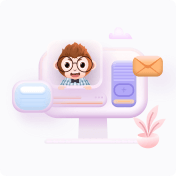
安全验证
文档复制为VIP权益,开通VIP直接复制
