(function(global, factory) {
typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) :
typeof define === 'function' && define.amd ? define(['exports'], factory) :
(factory((global.JSEncrypt = {})));
}(this, (function(exports) {
'use strict';
var navigator2 = {
appName: 'Netscape',
userAgent: 'Mozilla/5.0 (iPhone; CPU iPhone OS 9_1 like Mac OS X) AppleWebKit/601.1.46 (KHTML, like Gecko) Version/9.0 Mobile/13B143 Safari/601.1'
};
// 用来替换window2
var window2 = {
ASN1: null,
Base64: null,
Hex: null,
crypto: null,
href: null
};
var BI_RM = "0123456789abcdefghijklmnopqrstuvwxyz";
function int2char(n) {
return BI_RM.charAt(n);
}
//#region BIT_OPERATIONS
// (public) this & a
function op_and(x, y) {
return x & y;
}
// (public) this | a
function op_or(x, y) {
return x | y;
}
// (public) this ^ a
function op_xor(x, y) {
return x ^ y;
}
// (public) this & ~a
function op_andnot(x, y) {
return x & ~y;
}
// return index of lowest 1-bit in x, x < 2^31
function lbit(x) {
if (x == 0) {
return -1;
}
var r = 0;
if ((x & 0xffff) == 0) {
x >>= 16;
r += 16;
}
if ((x & 0xff) == 0) {
x >>= 8;
r += 8;
}
if ((x & 0xf) == 0) {
x >>= 4;
r += 4;
}
if ((x & 3) == 0) {
x >>= 2;
r += 2;
}
if ((x & 1) == 0) {
++r;
}
return r;
}
// return number of 1 bits in x
function cbit(x) {
var r = 0;
while (x != 0) {
x &= x - 1;
++r;
}
return r;
}
//#endregion BIT_OPERATIONS
var b64map = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";
var b64pad = "=";
function hex2b64(h) {
var i;
var c;
var ret = "";
for (i = 0; i + 3 <= h.length; i += 3) {
c = parseInt(h.substring(i, i + 3), 16);
ret += b64map.charAt(c >> 6) + b64map.charAt(c & 63);
}
if (i + 1 == h.length) {
c = parseInt(h.substring(i, i + 1), 16);
ret += b64map.charAt(c << 2);
} else if (i + 2 == h.length) {
c = parseInt(h.substring(i, i + 2), 16);
ret += b64map.charAt(c >> 2) + b64map.charAt((c & 3) << 4);
}
while ((ret.length & 3) > 0) {
ret += b64pad;
}
return ret;
}
// convert a base64 string to hex
function b64tohex(s) {
var ret = "";
var i;
var k = 0; // b64 state, 0-3
var slop = 0;
for (i = 0; i < s.length; ++i) {
if (s.charAt(i) == b64pad) {
break;
}
var v = b64map.indexOf(s.charAt(i));
if (v < 0) {
continue;
}
if (k == 0) {
ret += int2char(v >> 2);
slop = v & 3;
k = 1;
} else if (k == 1) {
ret += int2char((slop << 2) | (v >> 4));
slop = v & 0xf;
k = 2;
} else if (k == 2) {
ret += int2char(slop);
ret += int2char(v >> 2);
slop = v & 3;
k = 3;
} else {
ret += int2char((slop << 2) | (v >> 4));
ret += int2char(v & 0xf);
k = 0;
}
}
if (k == 1) {
ret += int2char(slop << 2);
}
return ret;
}
/*! *****************************************************************************
Copyright (c) Microsoft Corporation. All rights reserved.
Licensed under the Apache License, Version 2.0 (the "License"); you may not use
this file except in compliance with the License. You may obtain a copy of the
License at http://www.apache.org/licenses/LICENSE-2.0
THIS CODE IS PROVIDED ON AN *AS IS* BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, EITHER EXPRESS OR IMPLIED, INCLUDING WITHOUT LIMITATION ANY IMPLIED
WARRANTIES OR CONDITIONS OF TITLE, FITNESS FOR A PARTICULAR PURPOSE,
MERCHANTABLITY OR NON-INFRINGEMENT.
See the Apache Version 2.0 License for specific language governing permissions
and limitations under the License.
***************************************************************************** */
/* global Reflect, Promise */
var extendStatics = function(d, b) {
extendStatics = Object.setPrototypeOf ||
({
__proto__: []
}
instanceof Array && function(d, b) {
d.__proto__ = b;
}) ||
function(d, b) {
for (var p in b)
if (b.hasOwnProperty(p)) d[p] = b[p];
};
return extendStatics(d, b);
};
function __extends(d, b) {
extendStatics(d, b);
function __() {
this.constructor = d;
}
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
}
// Hex JavaScript decoder
// Copyright (c) 2008-2013 Lapo Luchini <lapo@lapo.it>
// Permission to use, copy, modify, and/or distribute this software for any
// purpose with or without fee is hereby granted, provided that the above
// copyright notice and this permission notice appear in all copies.
//
// THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
// WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
// MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
// ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
// WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
// ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
// OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
/*jshint browser: true, strict: true, immed: true, latedef: true, undef: true, regexdash: false */
var decoder;
var Hex = {
decode: function(a) {
var i;
if (decoder === undefined) {
var hex = "0123456789ABCDEF";
var ignore = " \f\n\r\t\u00A0\u2028\u2029";
decoder = {};
for (i = 0; i < 16; ++i) {
decoder[hex.charAt(i)] = i;
}
hex = hex.toLowerCase();
for (i = 10; i < 16; ++i) {
decoder[hex.charAt(i)] = i;
}
for (i = 0; i < ignore.length; ++i) {
decoder[ignore.charAt(i)] = -1;
}
}
var out = [];
var bits = 0;
var char_count = 0;
for (i = 0; i < a.length; ++i) {
var c = a.charAt(i);
if (c == "=") {
break;
}
c = decoder[c];
if (c == -1) {
continue;
}
if (c === undefined) {
throw new Error("Illegal character at offset " + i);
}
bits |= c;
if (++char_count >= 2) {
out[out.length] = bits;
bits = 0;
char_count = 0;
} else {
bits <<= 4;
}
}
if (char_count) {
throw new Error("Hex encoding incomplete: 4 bits missing");
}
return out;
}
};
// Base64 JavaScript decoder
// Copyright (c) 2008-2013 Lapo Luchini <lapo@lapo.it>
// Permission to use, copy, modify, and/or distribute this software for any
// purpose with or without fee is hereby granted, provided that the above
// copyright notice and this permission notice appear in all copies.
//
// THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
// WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
// MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
// ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
// WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
// ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
// OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
/*jshint browser: true, strict: true, immed: true, latedef: true, undef: true, regexdash: false */
var decoder$1;
var Base64 = {
decode: function(a) {
var i;
if (decoder$1 === undefined) {
var b64 = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";
var ignore = "= \f\n\r\t\u00A0\u2028\u2029";
decoder$1 = Object.create(null);
for (i = 0; i < 64; ++i) {
decoder$1[b64.charAt(i)] = i;
}
for (i = 0; i < ignore.length; ++i) {
decoder$1[ignore.charAt(i)] = -1;
}
}
var out = [];
var bits = 0;
var char_count = 0;
for (i = 0; i < a.length; ++i) {
var c = a.charAt(i);
if (c == "=") {
break;
}
c = decoder$1[c];
if (c == -1) {
continue;
}
if (c === undefined) {
throw new Error("Illegal character at offset " + i);
}
bits |= c;
if (++char_count >= 4) {
out[out.length] = (bits >> 16);
out[out.length] = (bits >> 8) & 0xFF;
out[out.l
没有合适的资源?快使用搜索试试~ 我知道了~
基于Vue框架开发的新白马项目访问登记App设计源码
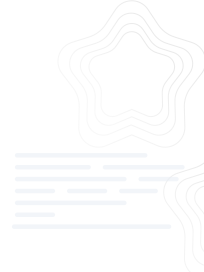
共332个文件
vue:89个
json:85个
js:57个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 132 浏览量
2024-09-26
04:04:59
上传
评论
收藏 1.58MB ZIP 举报
温馨提示
该项目为基于Vue框架构建的新白马项目访问登记App的设计源码,共包含377个文件,涵盖92个Markdown文件、89个Vue组件文件、85个JSON配置文件、57个JavaScript脚本文件、24个PNG图片文件、15个SCSS样式文件、5个CSS样式文件、3个字体文件(.ttf)、2个WXS组件文件以及1个Git忽略文件。该应用采用Vue、JavaScript、CSS和HTML等多种语言开发,旨在实现高效便捷的访问登记管理功能。
资源推荐
资源详情
资源评论
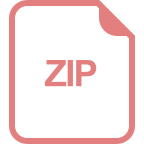
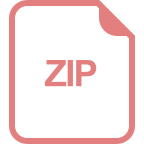
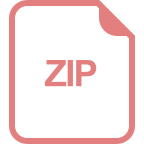
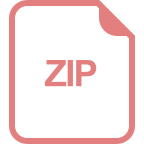
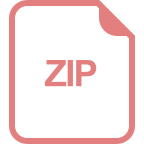
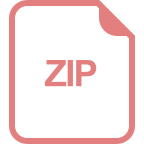
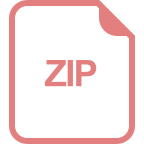
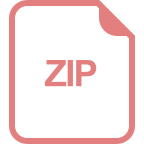
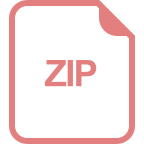
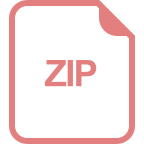
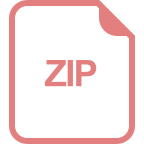
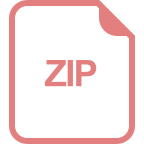
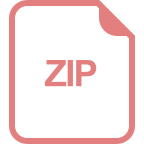
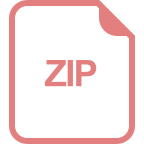
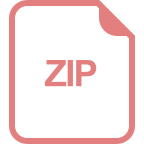
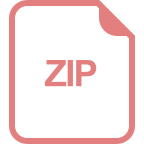
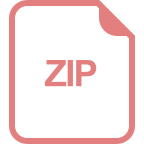
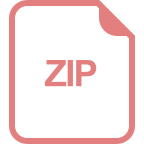
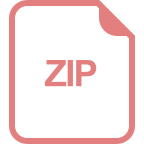
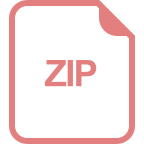
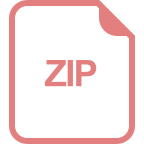
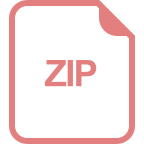
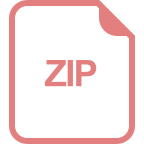
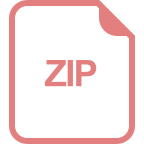
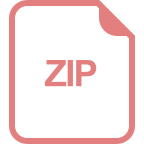
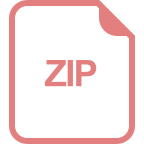
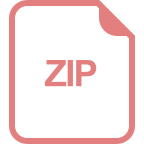
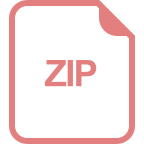
收起资源包目录

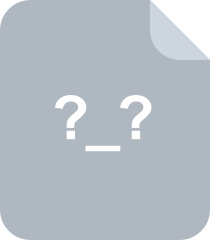
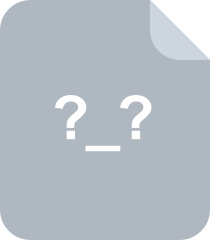
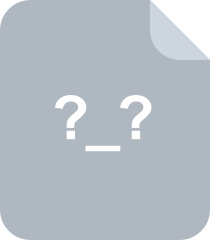
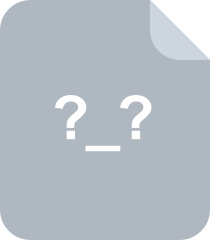
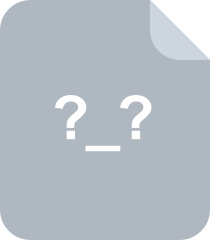
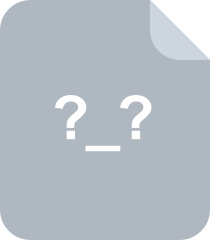
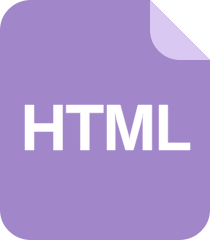
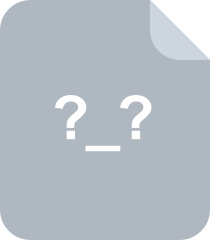
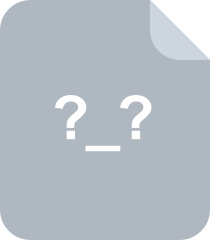
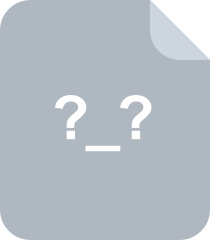
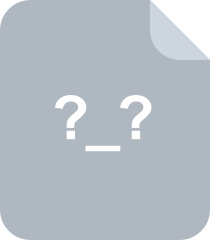
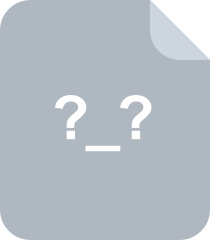
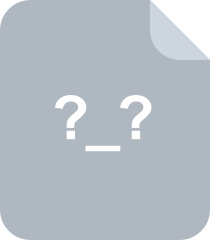
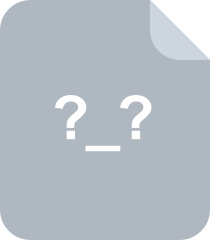
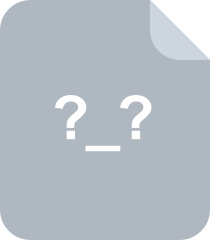
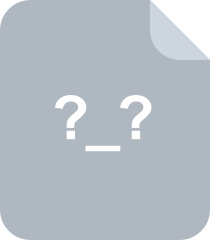
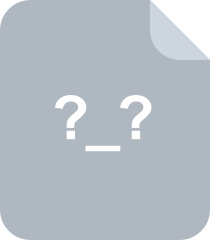
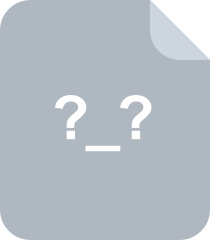
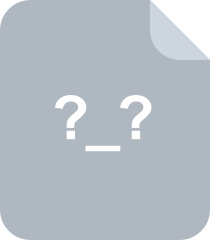
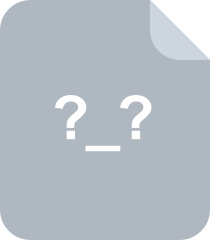
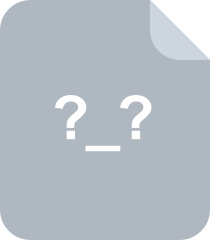
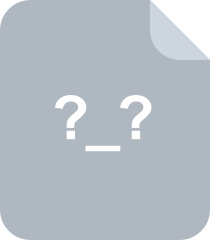
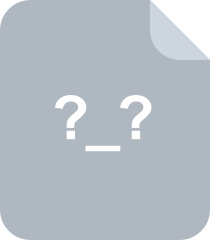
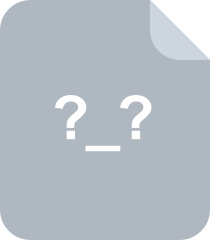
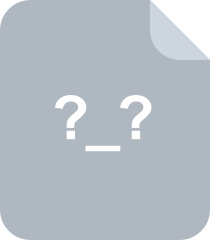
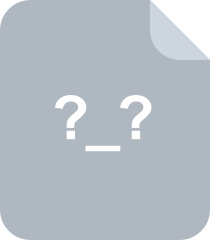
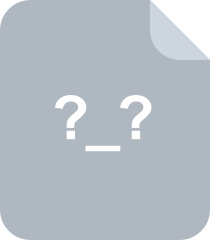
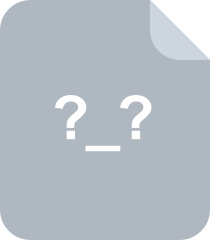
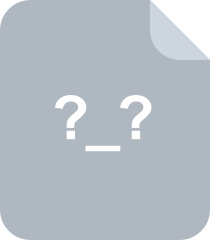
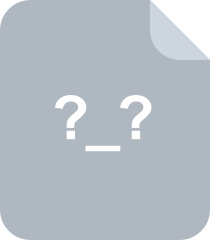
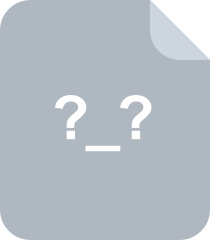
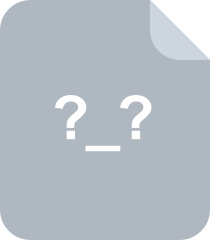
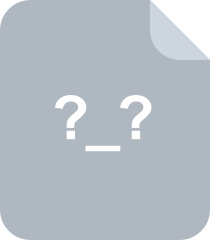
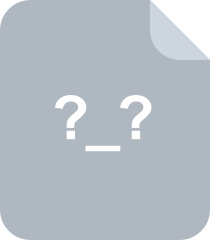
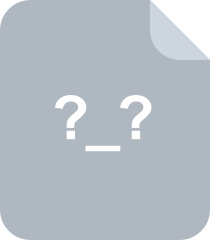
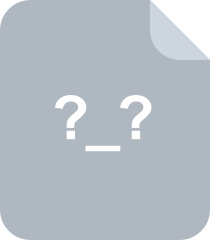
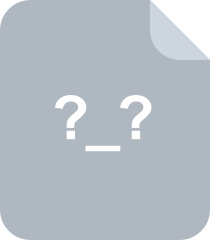
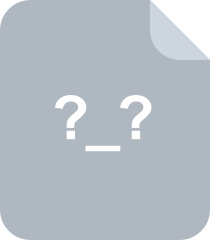
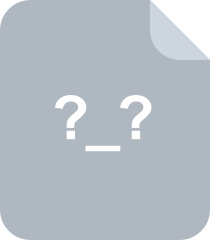
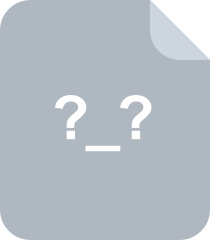
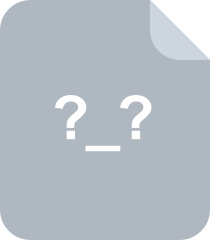
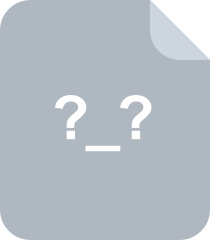
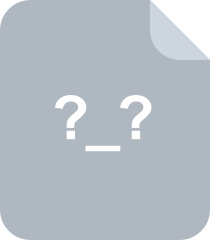
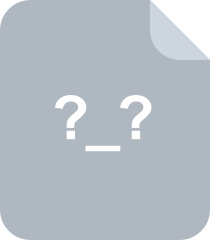
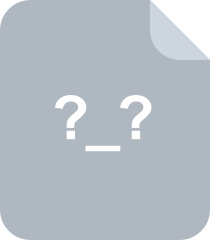
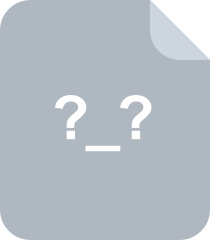
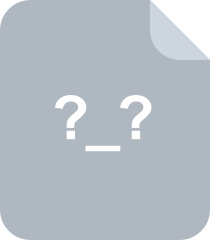
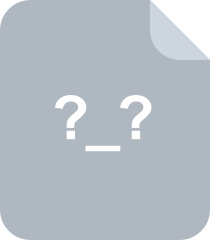
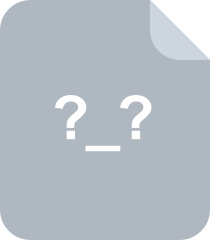
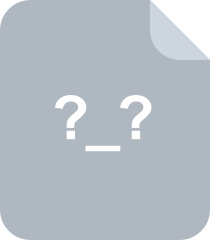
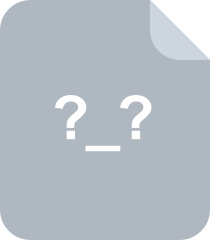
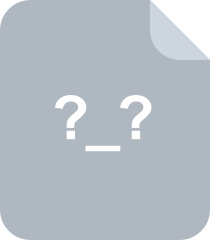
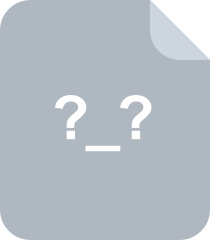
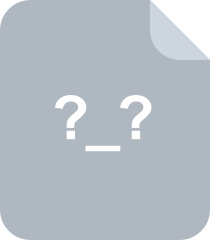
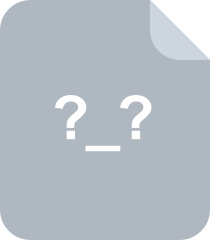
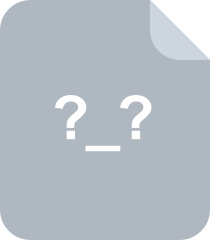
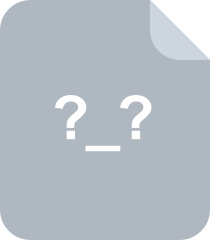
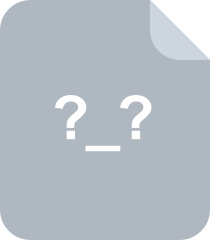
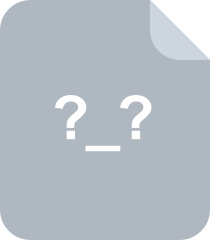
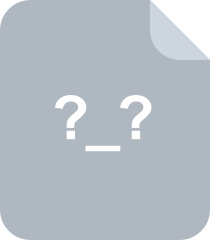
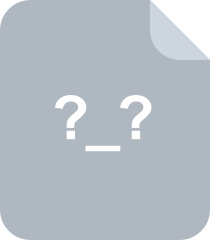
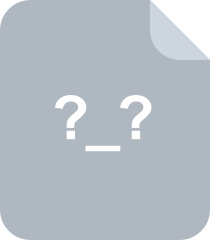
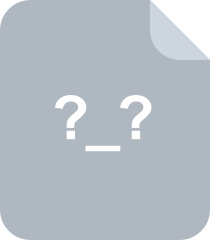
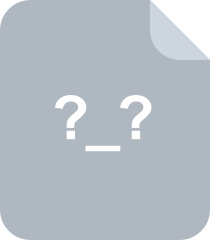
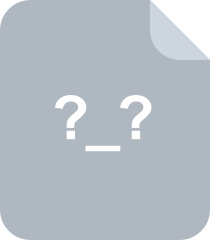
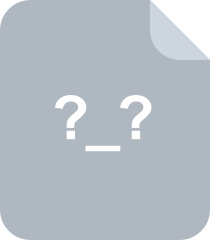
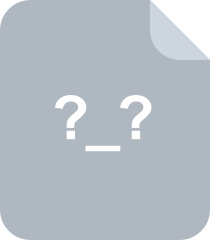
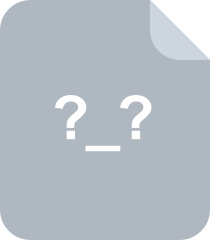
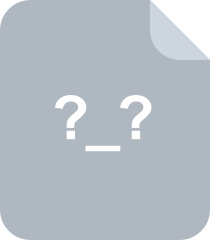
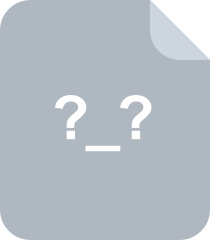
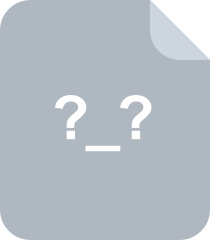
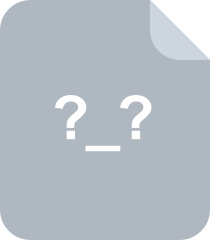
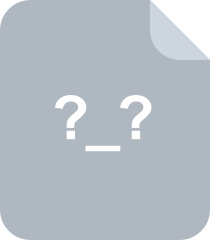
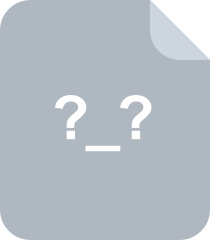
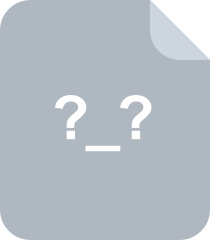
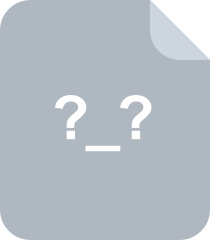
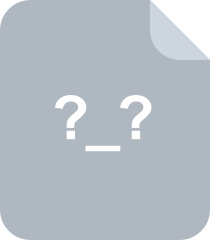
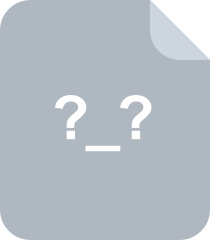
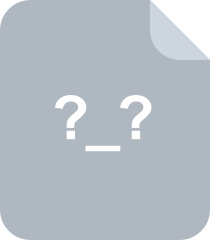
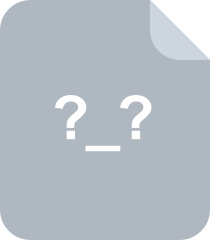
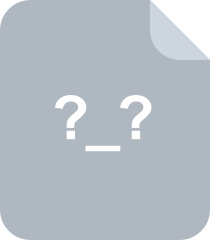
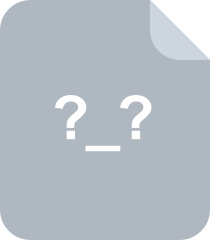
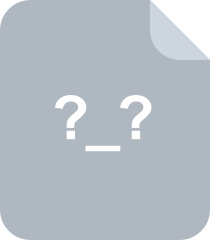
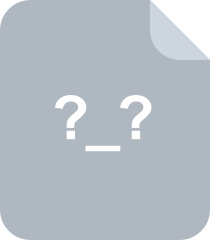
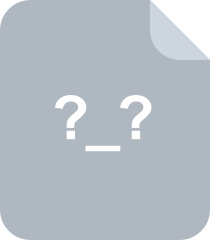
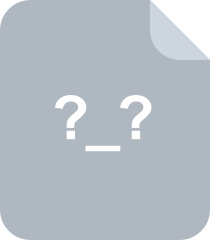
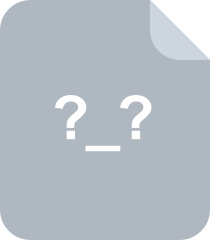
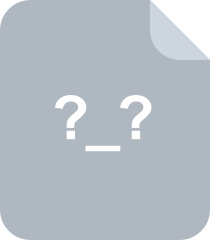
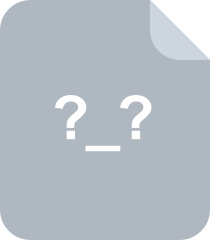
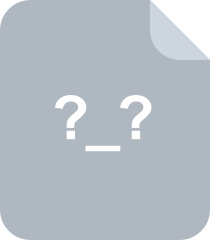
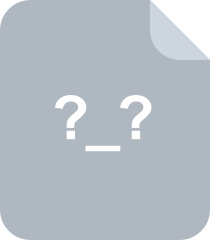
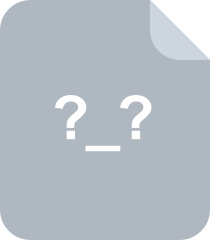
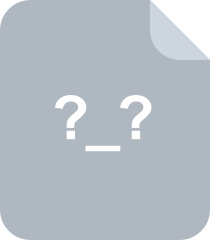
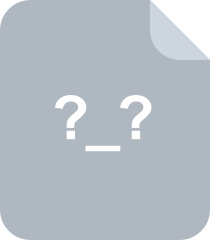
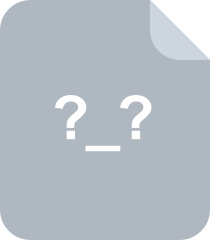
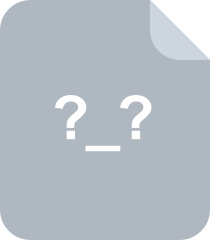
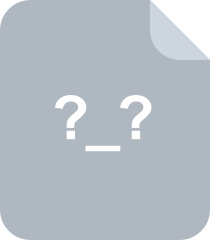
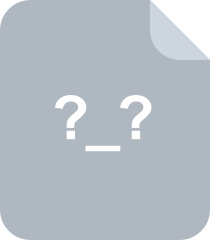
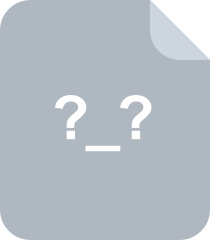
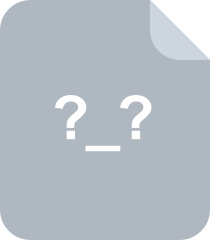
共 332 条
- 1
- 2
- 3
- 4
资源评论
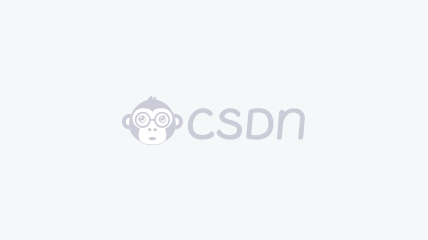

wjs2024
- 粉丝: 2143
- 资源: 5434
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

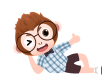
最新资源
- python实现Excel表格合并
- Java实现读取Excel批量发送邮件.zip
- 【java毕业设计】商城后台管理系统源码(springboot+vue+mysql+说明文档).zip
- 【java毕业设计】开发停车位管理系统(调用百度地图API)源码(springboot+vue+mysql+说明文档).zip
- 星耀软件库(升级版).apk.1
- 基于Django后端和Vue前端的多语言购物车项目设计源码
- 基于Python与Vue的浮光在线教育平台源码设计
- 31129647070291Eclipson MXS R.zip
- 基于Html与Java的会员小程序后台管理系统设计源码
- 基于Python的RabbitMQ消息队列安装使用及脚本开发设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


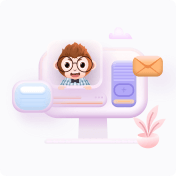
安全验证
文档复制为VIP权益,开通VIP直接复制
