/*
* GeoServer-Manager - Simple Manager Library for GeoServer
*
* Copyright (C) 2007 - 2016 GeoSolutions S.A.S.
* http://www.geo-solutions.it
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.mapfinal.server.geoserver.rest;
import com.mapfinal.server.geoserver.rest.encoder.GSBackupEncoder;
import com.mapfinal.server.geoserver.rest.encoder.GSLayerEncoder;
import com.mapfinal.server.geoserver.rest.encoder.GSLayerGroupEncoder;
import com.mapfinal.server.geoserver.rest.encoder.GSNamespaceEncoder;
import com.mapfinal.server.geoserver.rest.encoder.GSPostGISDatastoreEncoder;
import com.mapfinal.server.geoserver.rest.encoder.GSResourceEncoder;
import com.mapfinal.server.geoserver.rest.encoder.GSResourceEncoder.ProjectionPolicy;
import com.mapfinal.server.geoserver.rest.encoder.GSWorkspaceEncoder;
import com.mapfinal.server.geoserver.rest.encoder.coverage.GSCoverageEncoder;
import com.mapfinal.server.geoserver.rest.encoder.feature.GSFeatureTypeEncoder;
import com.mapfinal.server.geoserver.rest.manager.GeoServerRESTStructuredGridCoverageReaderManager;
import com.mapfinal.server.geoserver.rest.manager.GeoServerRESTStructuredGridCoverageReaderManager.ConfigureCoveragesOption;
import com.mapfinal.server.geoserver.rest.manager.GeoServerRESTStyleManager;
import com.mapfinal.server.geoserver.rest.manager.GeoServerRESTImporterManager;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URL;
import java.net.URLEncoder;
import java.util.zip.ZipFile;
import org.apache.commons.httpclient.NameValuePair;
import org.apache.commons.io.FilenameUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.alibaba.fastjson.JSONObject;
import com.lambkit.common.util.HttpUtils;
import com.mapfinal.server.geoserver.rest.decoder.RESTCoverage;
import com.mapfinal.server.geoserver.rest.decoder.RESTCoverageStore;
import com.mapfinal.server.geoserver.rest.decoder.RESTStructuredCoverageGranulesList;
import com.mapfinal.server.geoserver.rest.decoder.RESTStyleList;
import com.mapfinal.server.geoserver.rest.decoder.utils.NameLinkElem;
/**
* Connect to a GeoServer instance to publish or modify its contents via REST API.
* <P>
* There are no modifiable instance fields, so all the calls are thread-safe.
*
* @author ETj (etj at geo-solutions.it)
* @author Carlo Cancellieri - carlo.cancellieri@geo-solutions.it
* @author Lennart Karsten - lennart.k@thinking-aloud.eu
*/
public class GeoServerRESTPublisher {
public static final String DEFAULT_CRS = "EPSG:4326";
/** The logger for this class */
private static final Logger LOGGER = LoggerFactory.getLogger(GeoServerRESTPublisher.class);
/**
* GeoServer instance base URL. E.g.: <TT>http://localhost:8080/geoserver</TT>.
*/
private final String restURL;
/**
* GeoServer instance privileged username, with read & write permission on REST API
*/
private final String gsuser;
/**
* GeoServer instance password for privileged username with r&w permission on REST API
*/
private final String gspass;
private final GeoServerRESTStyleManager styleManager;
private final GeoServerRESTImporterManager importerManager;
/**
* Creates a <TT>GeoServerRESTPublisher</TT> to connect against a GeoServer instance with the given URL and user credentials.
*
* @param restURL the base GeoServer URL (e.g.: <TT>http://localhost:8080/geoserver</TT>)
* @param username auth credential
* @param password auth credential
*/
public GeoServerRESTPublisher(String restURL, String username, String password) {
this.restURL = HttpUtils.decurtSlash(restURL);
this.gsuser = username;
this.gspass = password;
URL url = null;
try {
url = new URL(restURL);
} catch (MalformedURLException ex) {
LOGGER.error("Bad URL: Calls to GeoServer are going to fail" , ex);
}
styleManager = new GeoServerRESTStyleManager(url, username, password);
importerManager = new GeoServerRESTImporterManager(url, username, password);
}
// ==========================================================================
// === BACKUP and RESTORE
// ==========================================================================
/**
* Issues a GeoServer BACKUP.
* <P>
* Won't include data, cached tiles, or logs. Use {@link #backup(String, boolean, boolean, boolean)} to control these parameters.
*
* @param backupDir the target Backup Dir String.
*
* @return <TT>id</TT> of the backup.
* @throws IllegalArgumentException if the backupDir is null or empty
*/
public String backup(final String backupDir) throws IllegalArgumentException {
/*
* This is the equivalent call with cUrl:
*
* {@code curl -u admin:geoserver -XPOST \ -H 'Content-type: text/xml' \ --data
* "<task><path>${BACKUP_DATADIR}</path></task>" \ ${restURL}/rest/bkprst/backup}
*/
return backup(backupDir, false, false, false);
}
/**
* Issues a GeoServer BACKUP.
*
* @param backupDir the target Backup Dir String.
* @param includedata whether or not include the data dir Boolean.
* @param includegwc whether or not include the geowebcache dir Boolean.
* @param includelog whether or not include the log dir Boolean.
*
* @return <TT>id</TT> of the backup.
* @throws IllegalArgumentException if the backupDir is null or empty.
*/
public String backup(final String backupDir, final boolean includedata,
final boolean includegwc, final boolean includelog) throws IllegalArgumentException {
/*
* This is the equivalent call with cUrl:
*
* {@code curl -u admin:geoserver -XPOST \ -H 'Content-type: text/xml' \ --data
* "<task><path>${BACKUP_DATADIR}</path><includedata>${includedata}</includedata><includegwc>${includegwc}</includegwc><includelog>${includelog}</includelog></task>"
* \ ${restURL}/rest/bkprst/backup}
*/
if ((backupDir == null) || backupDir.isEmpty()) {
throw new IllegalArgumentException("The backup_dir must not be null or empty");
}
StringBuilder bkpUrl = new StringBuilder(restURL);
bkpUrl.append("/rest/bkprst/backup");
final GSBackupEncoder bkpenc = new GSBackupEncoder(backupDir);
bkpenc.setIncludeData(includedata);
bkpenc.setIncludeGwc(includegwc);
bkpenc.setIncludeLog(includelog);
final String result = HttpUtils.post(bkpUrl.toString(), bkpenc.toString(), "text/xml",
gsuser, gspass);
return result;
}
/
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该平台源码是基于PostGIS、GeoTools和GeoServer构建的GIS内容管理系统——mapfinal-portal,类似于GeoServer Portal。项目包含2328个文件,涵盖了611个Java源文件、554个JavaScript文件、313个CSS文件、245个PNG图片、227个GIF图片、212个HTML文件、53个JPG图片、17个Markdown文件、13个SVG文件、11个地图文件等。它是一个专为快速应用开发和极速体验设计的WebGIS服务平台。
资源推荐
资源详情
资源评论
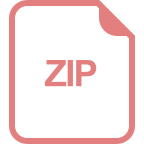
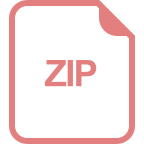
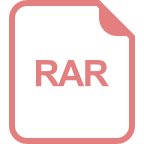
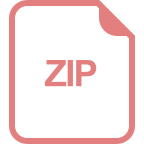
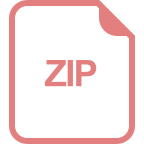
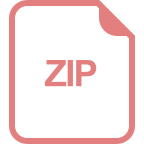
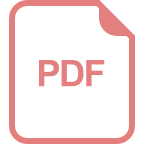
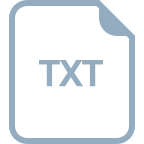
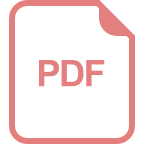
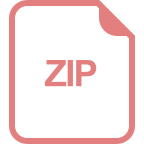
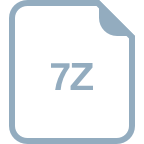
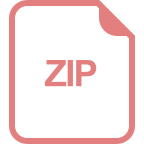
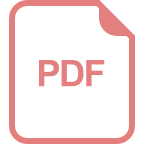
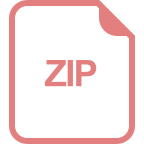
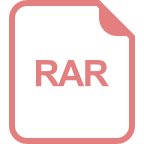
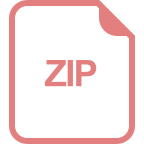
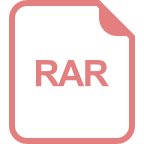
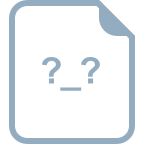
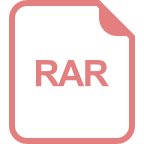
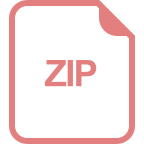
收起资源包目录

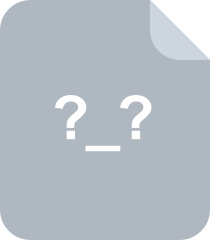
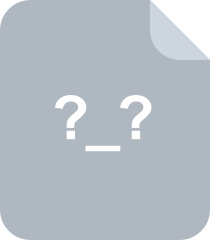
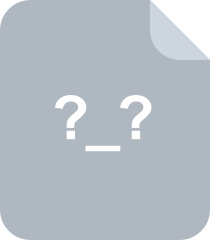
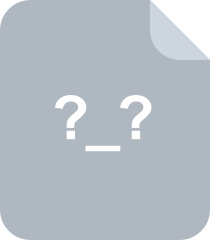
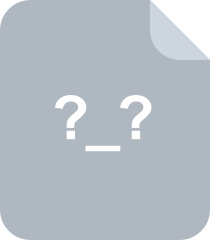
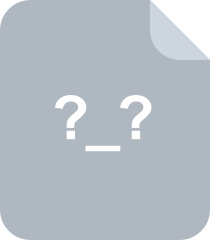
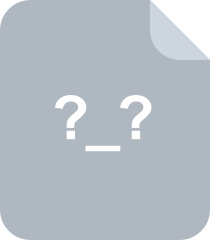
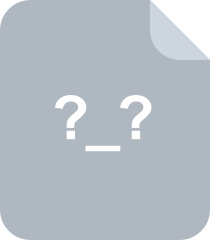
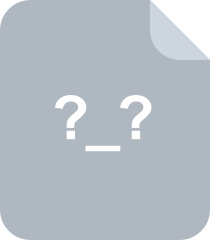
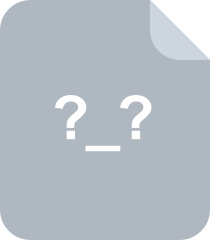
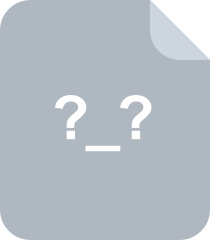
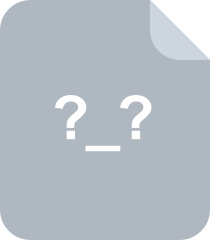
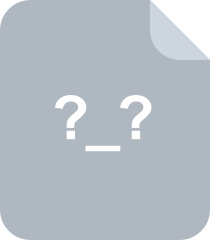
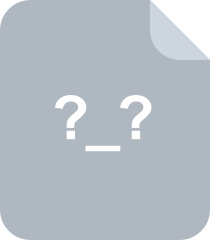
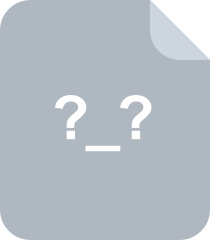
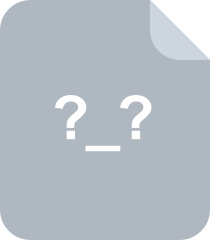
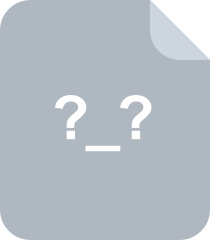
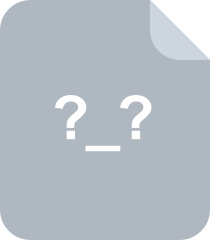
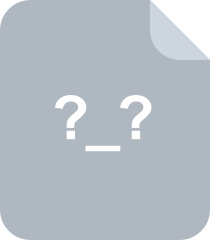
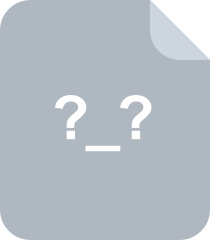
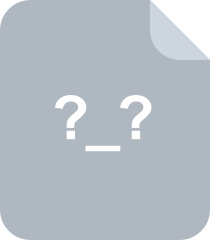
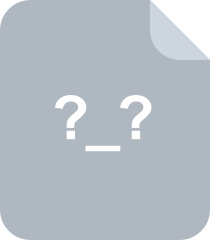
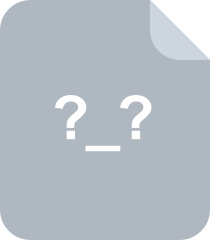
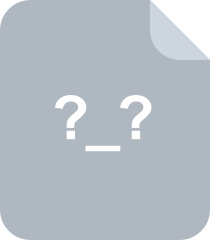
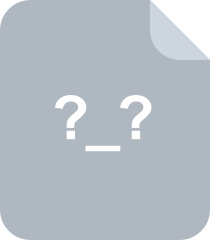
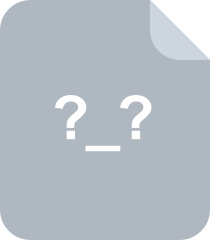
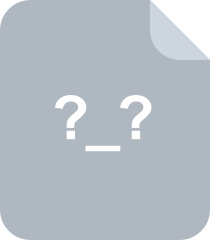
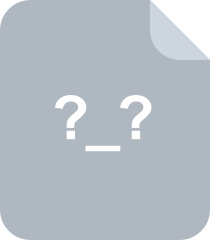
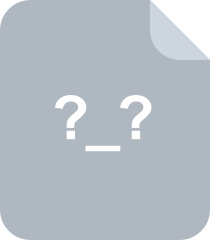
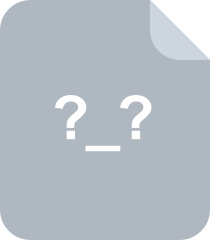
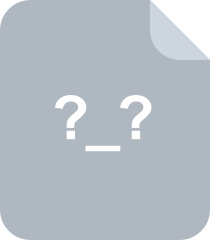
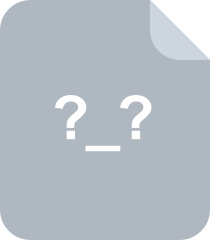
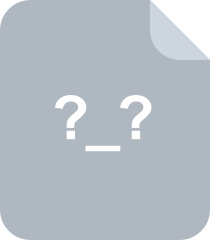
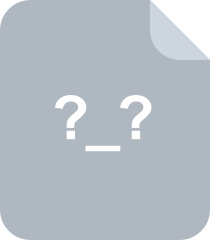
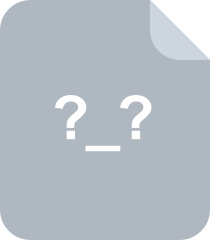
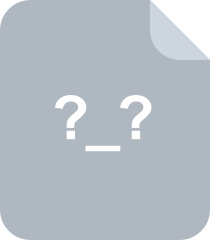
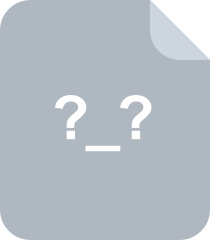
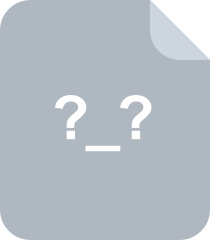
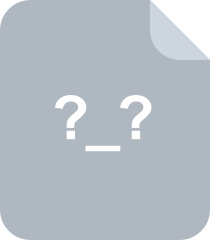
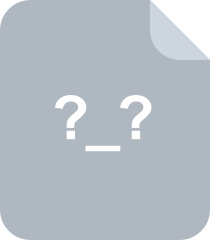
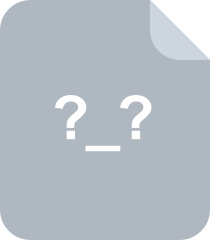
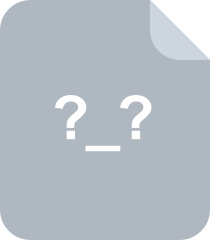
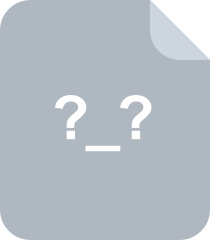
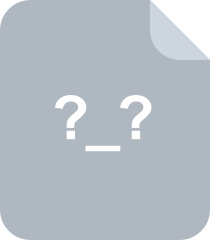
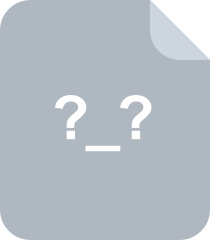
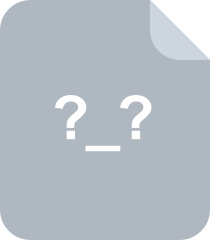
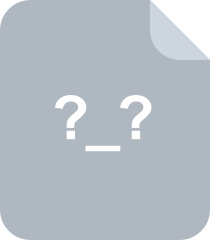
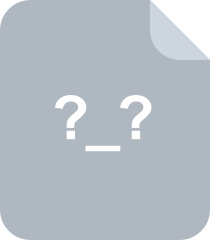
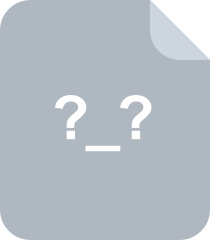
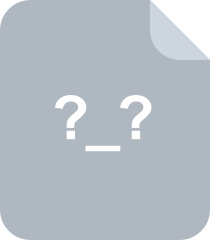
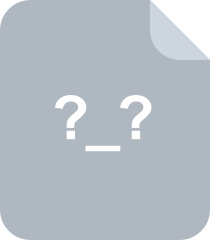
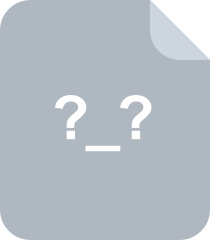
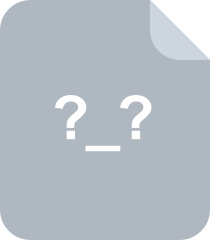
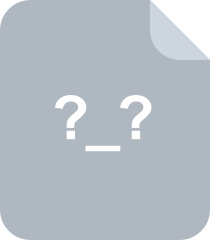
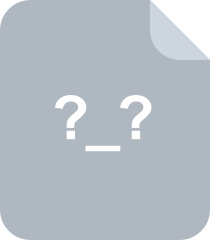
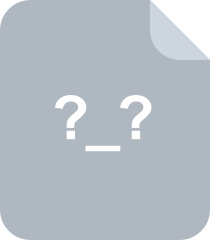
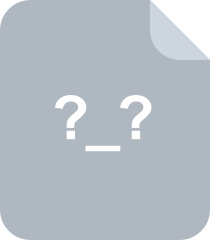
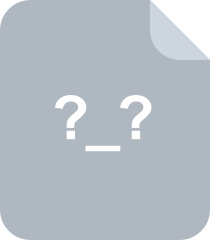
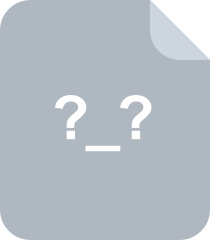
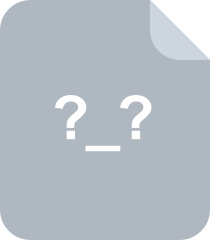
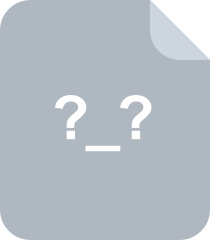
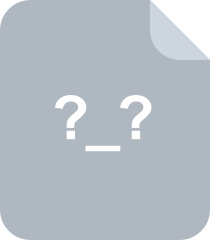
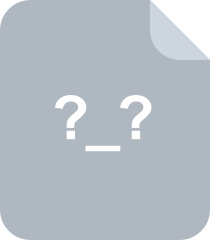
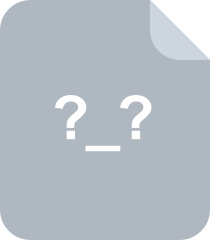
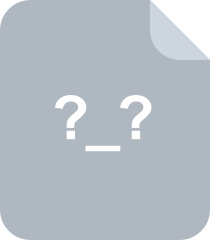
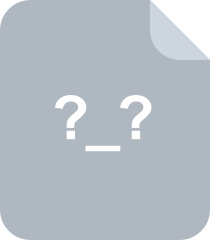
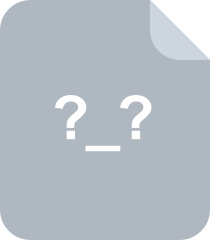
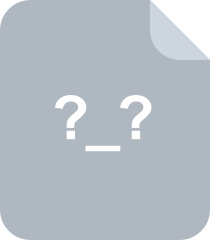
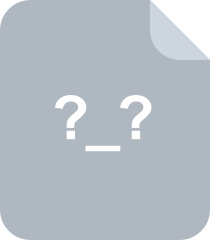
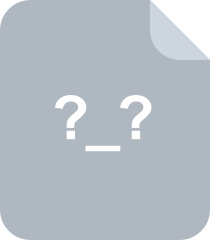
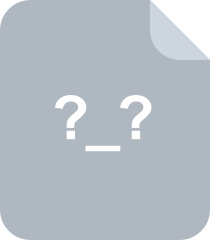
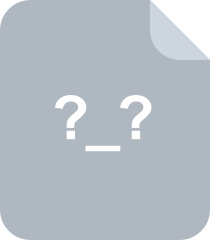
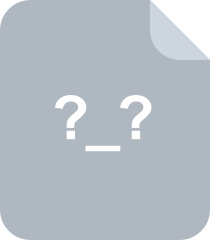
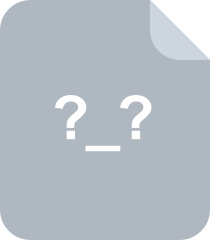
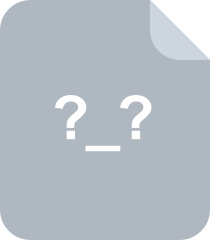
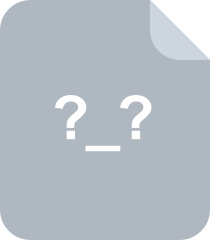
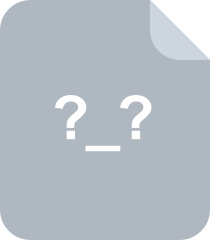
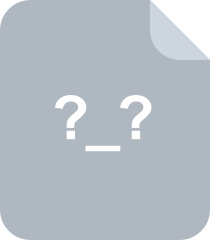
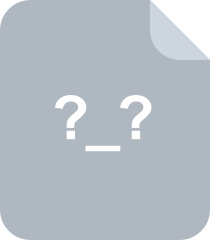
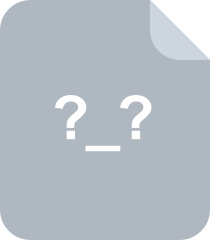
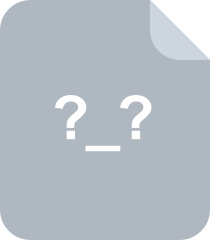
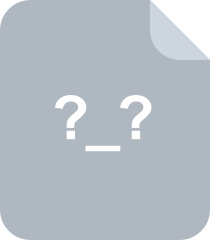
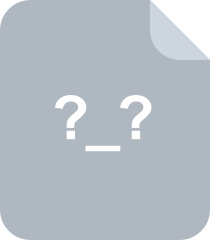
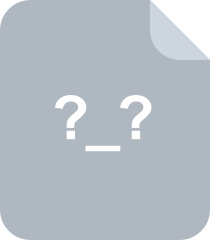
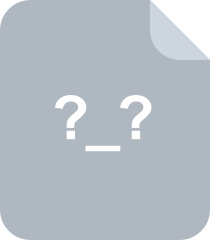
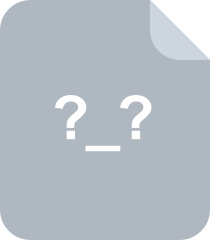
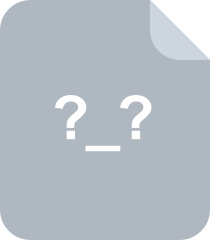
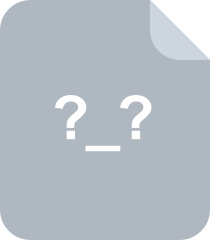
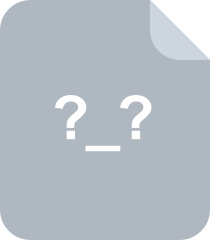
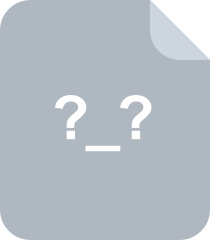
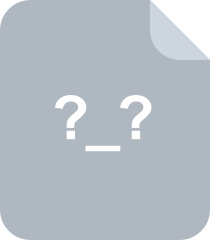
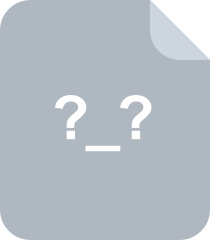
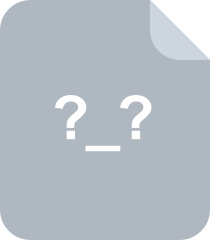
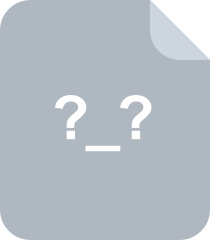
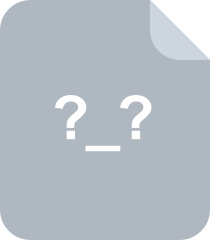
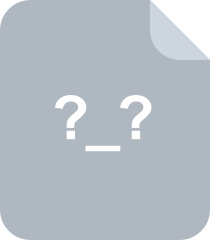
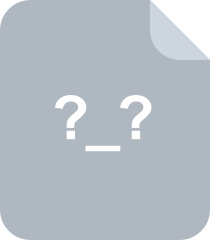
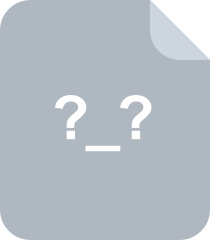
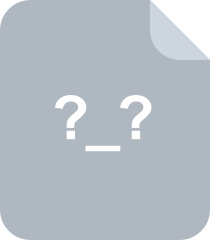
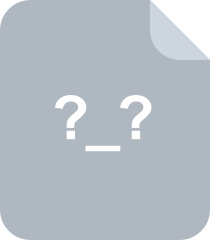
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
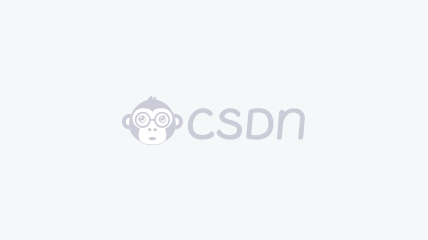

wjs2024
- 粉丝: 2368
- 资源: 5526
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

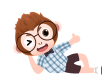
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


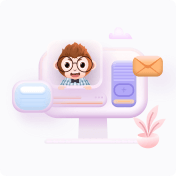
安全验证
文档复制为VIP权益,开通VIP直接复制
