/**************************************************************************************************
Filename: zcl.c
Revised: $Date: 2007-12-13 15:02:48 -0800 (Thu, 13 Dec 2007) $
Revision: $Revision: 16070 $
Description: This file contains the Zigbee Cluster Library Foundation functions.
Copyright 2006-2007 Texas Instruments Incorporated. All rights reserved.
IMPORTANT: Your use of this Software is limited to those specific rights
granted under the terms of a software license agreement between the user
who downloaded the software, his/her employer (which must be your employer)
and Texas Instruments Incorporated (the "License"). You may not use this
Software unless you agree to abide by the terms of the License. The License
limits your use, and you acknowledge, that the Software may not be modified,
copied or distributed unless embedded on a Texas Instruments microcontroller
or used solely and exclusively in conjunction with a Texas Instruments radio
frequency transceiver, which is integrated into your product. Other than for
the foregoing purpose, you may not use, reproduce, copy, prepare derivative
works of, modify, distribute, perform, display or sell this Software and/or
its documentation for any purpose.
YOU FURTHER ACKNOWLEDGE AND AGREE THAT THE SOFTWARE AND DOCUMENTATION ARE
PROVIDED �AS IS� WITHOUT WARRANTY OF ANY KIND, EITHER EXPRESS OR IMPLIED,
INCLUDING WITHOUT LIMITATION, ANY WARRANTY OF MERCHANTABILITY, TITLE,
NON-INFRINGEMENT AND FITNESS FOR A PARTICULAR PURPOSE. IN NO EVENT SHALL
TEXAS INSTRUMENTS OR ITS LICENSORS BE LIABLE OR OBLIGATED UNDER CONTRACT,
NEGLIGENCE, STRICT LIABILITY, CONTRIBUTION, BREACH OF WARRANTY, OR OTHER
LEGAL EQUITABLE THEORY ANY DIRECT OR INDIRECT DAMAGES OR EXPENSES
INCLUDING BUT NOT LIMITED TO ANY INCIDENTAL, SPECIAL, INDIRECT, PUNITIVE
OR CONSEQUENTIAL DAMAGES, LOST PROFITS OR LOST DATA, COST OF PROCUREMENT
OF SUBSTITUTE GOODS, TECHNOLOGY, SERVICES, OR ANY CLAIMS BY THIRD PARTIES
(INCLUDING BUT NOT LIMITED TO ANY DEFENSE THEREOF), OR OTHER SIMILAR COSTS.
Should you have any questions regarding your right to use this Software,
contact Texas Instruments Incorporated at www.TI.com.
**************************************************************************************************/
/*********************************************************************
* INCLUDES
*/
#include "ZComDef.h"
#include "OSAL.h"
#include "AF.h"
#include "ZDConfig.h"
#include "zcl.h"
#include "zcl_ha.h"
#include "zcl_general.h"
/*********************************************************************
* MACROS
*/
/*** Frame Control ***/
#define zcl_FCType( a ) ( (a) & ZCL_FRAME_CONTROL_TYPE )
#define zcl_FCManuSpecific( a ) ( (a) & ZCL_FRAME_CONTROL_MANU_SPECIFIC )
#define zcl_FCDirection( a ) ( (a) & ZCL_FRAME_CONTROL_DIRECTION )
#define zcl_FCDisableDefaultRsp( a ) ( (a) & ZCL_FRAME_CONTROL_DISABLE_DEFAULT_RSP )
/*** Attribute Access Control ***/
#define zcl_AccessCtrlRead( a ) ( (a) & ACCESS_CONTROL_READ )
#define zcl_AccessCtrlWrite( a ) ( (a) & ACCESS_CONTROL_WRITE )
#define zcl_AccessCtrlCmd( a ) ( (a) & ACCESS_CONTROL_CMD )
#define zclParseCmd( a, b ) zclCmdTable[(a)].pfnParseInProfile( (b) )
#define zclProcessCmd( a, b ) zclCmdTable[(a)].pfnProcessInProfile( (b) )
/*** Table Indexing ***/
#define NEXT_READ_RSP( ptr, len ) (zclReadRspStatus_t *)( (uint8 *)(ptr) + \
sizeof (zclReadRspStatus_t) + (len) )
#define NEXT_WRITE( ptr, len ) (zclWriteRec_t *)( (uint8 *)(ptr) + \
sizeof (zclWriteRec_t) + (len) )
#define NEXT_REPORT( ptr, len ) (zclReport_t *)( (uint8 *)(ptr) + \
sizeof (zclReport_t) + (len) )
#define NEXT_CFG_REPORT( ptr, len ) (zclCfgReportRec_t *)( (uint8 *)(ptr) + \
sizeof (zclCfgReportRec_t) + (len) )
#define NEXT_REPORT_RSP( ptr, len ) (zclReportCfgRspRec_t *) ( (uint8 *)(ptr) + \
sizeof (zclReportCfgRspRec_t) + (len) )
// Commands that have corresponding responses
#define CMD_HAS_RSP( cmd ) ( (cmd) == ZCL_CMD_READ || \
(cmd) == ZCL_CMD_WRITE || \
(cmd) == ZCL_CMD_WRITE_UNDIVIDED || \
(cmd) == ZCL_CMD_CONFIG_REPORT || \
(cmd) == ZCL_CMD_READ_REPORT_CFG || \
(cmd) == ZCL_CMD_DISCOVER || \
(cmd) == ZCL_CMD_DEFAULT_RSP ) // exception
// There is no attribute in the Mandatory Reportable Attribute list for now
#define zcl_MandatoryReportableAttribute( a ) ( a == NULL )
/*********************************************************************
* CONSTANTS
*/
// Used by Configure Reporting Command
#define SEND_ATTR_REPORTS 0x00
#define EXPECT_ATTR_REPORTS 0x01
#define ZCL_MIN_REPORTING_INTERVAL 5
/*********************************************************************
* TYPEDEFS
*/
typedef struct zclLibPlugin
{
struct zclLibPlugin *next;
uint16 startLogCluster; // starting logical cluster ID
uint16 endLogCluster; // ending logical cluster ID
zclInHdlr_t pfnIncomingHdlr; // function to handle incoming message
} zclLibPlugin_t;
// Record to store a profile's cluster conversion table
typedef struct zclProfileClusterConvertRec
{
struct zclProfileClusterConvertRec *next;
uint16 profileID;
uint16 numClusters;
GENERIC zclConvertClusterRec_t *list;
} zclProfileClusterConvertRec_t;
// Attribute record list item
typedef struct zclAttrRecsList
{
struct zclAttrRecsList *next;
uint8 endpoint; // Used to link it into the endpoint descriptor
uint8 numAttributes; // Number of the following records
CONST zclAttrRec_t *attrs; // attribute records
} zclAttrRecsList;
typedef void *(*zclParseInProfileCmd_t)( zclParseCmd_t *pCmd );
typedef uint8 (*zclProcessInProfileCmd_t)( zclIncoming_t *pInMsg );
typedef struct
{
zclParseInProfileCmd_t pfnParseInProfile;
zclProcessInProfileCmd_t pfnProcessInProfile;
} zclCmdItems_t;
/*********************************************************************
* GLOBAL VARIABLES
*/
uint8 zcl_TaskID;
// The Application should register its attribute data validation function
zclValidateAttrData_t zcl_ValidateAttrDataCB = NULL;
/*********************************************************************
* EXTERNAL VARIABLES
*/
/*********************************************************************
* EXTERNAL FUNCTIONS
*/
/*********************************************************************
* LOCAL VARIABLES
*/
static zclLibPlugin_t *plugins;
static zclProfileClusterConvertRec_t *profileClusterList;
static zclAttrRecsList *attrList;
static byte zcl_TransID = 0; // This is the unique message ID (counter)
/*********************************************************************
* LOCAL FUNCTIONS
*/
static void zclProcessMessageMSG( afIncomingMSGPacket_t *pkt );
static uint8 *zclBuildHdr( zclFrameHdr_t *hdr, uint8 *pData );
static uint8 zclCalcHdrSize( zclFrameHdr_t *hdr );
static uint16 zclConvertClusterID( uint16 clusterID, uint16 profileID,
uint8 convertToLogical );
static zclLibPlugin_t *zclFindPlugin( uint16 realclusterID, uint16 profileID );
static uint8 zcl_DeviceOperational( uint8 srcEP, uint16 realClusterID, uint8 frameType, ui
没有合适的资源?快使用搜索试试~ 我知道了~
zigbee组网小实验3—添加传感数据采集功能
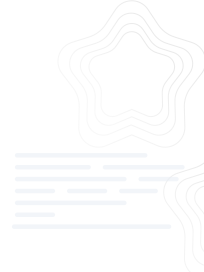
共923个文件
r51:236个
s51:218个
lst:212个


温馨提示
zigbee组网小实验3—添加传感数据采集功能 具体参见http://wjf88223.blog.163.com/blog/static/351680012010102983647815/ 这个是整个工程的压缩包,以前看到有80多M以为传不了,实验室网速实在不怎么好,压缩后才发现就11M,果断传吧~~~ 这只是个人做的实验,提供给需要的朋友参考参考~请勿转传!!谢谢~ 2011.3.11 小峰
资源推荐
资源详情
资源评论
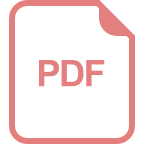
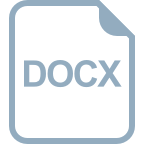
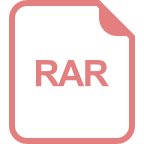
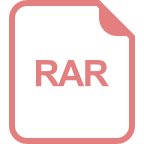
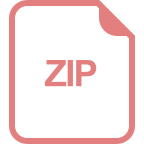
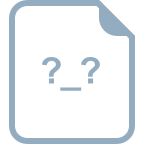
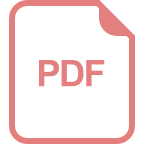
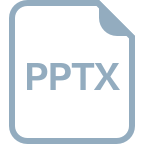
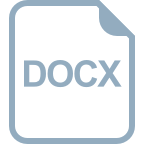
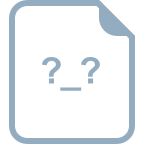
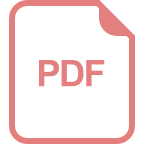
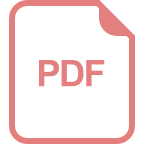
收起资源包目录

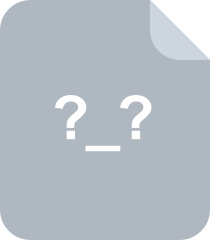
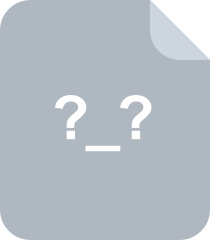
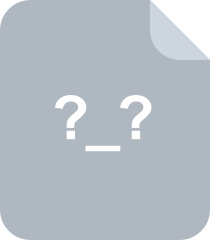
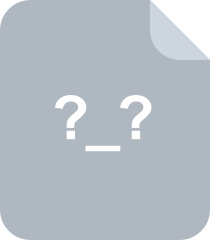
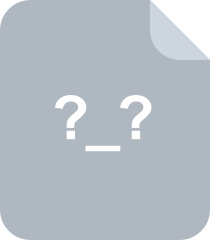
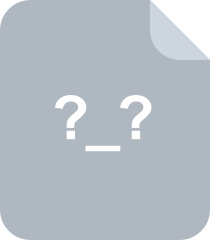
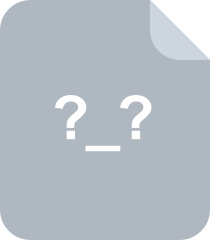
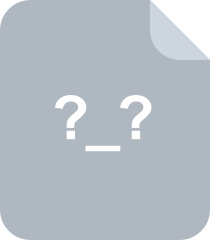
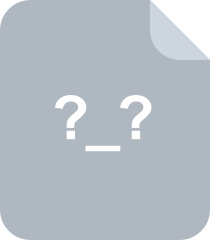
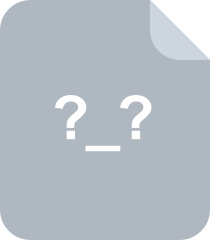
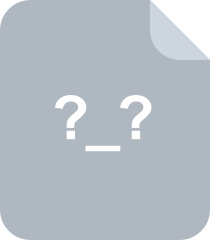
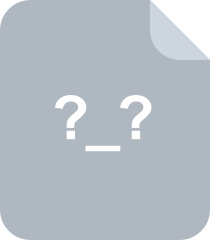
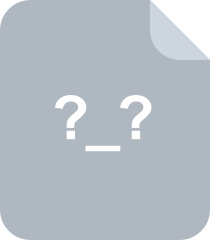
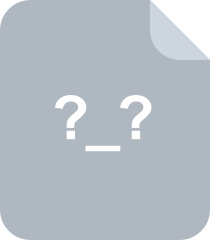
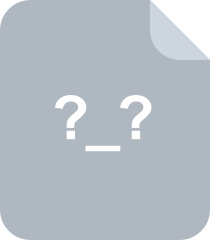
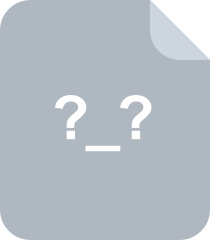
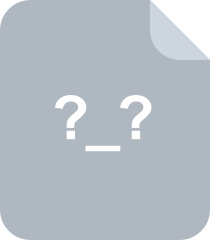
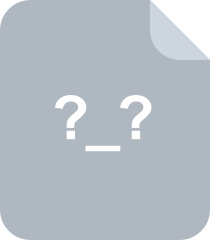
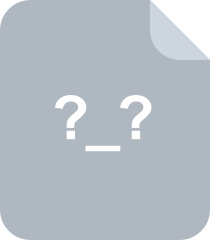
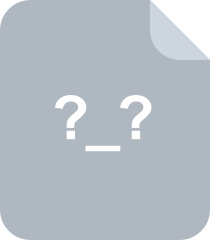
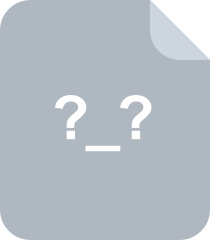
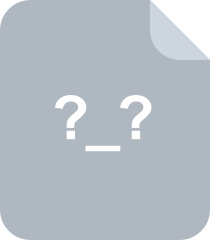
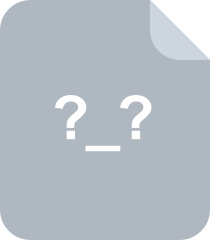
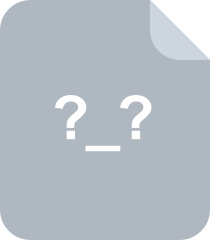
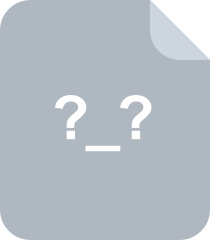
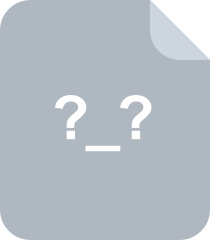
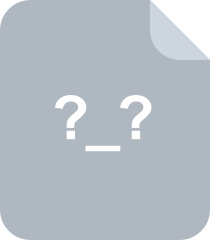
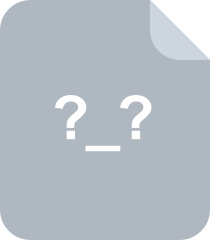
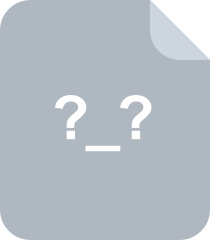
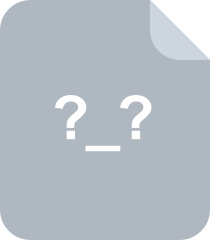
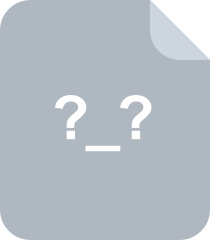
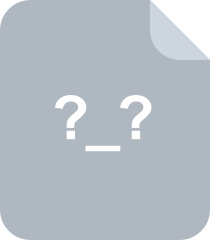
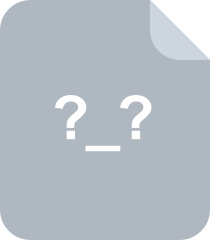
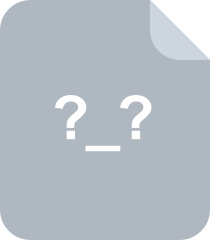
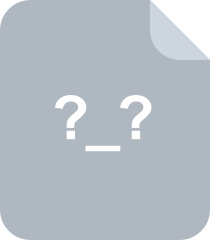
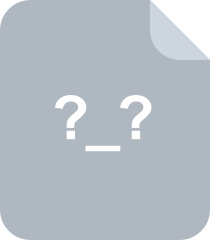
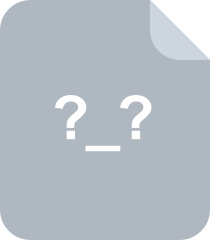
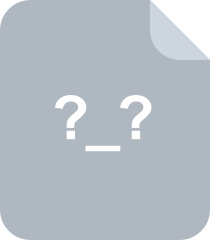
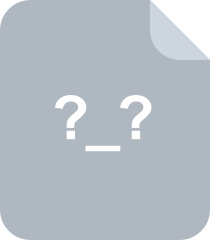
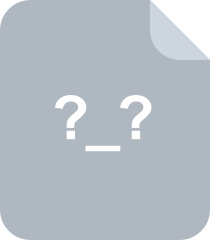
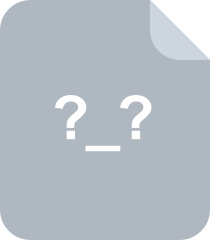
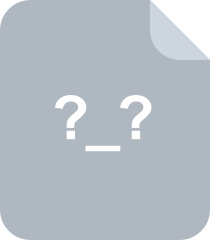
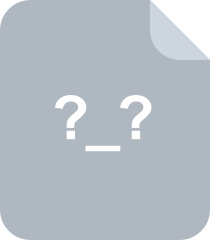
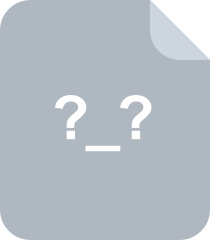
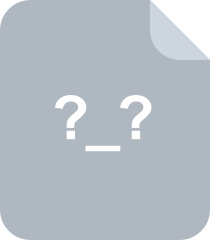
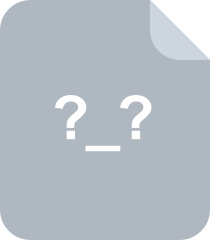
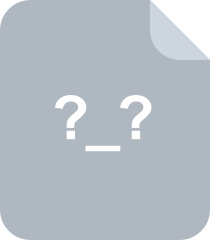
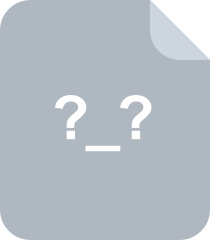
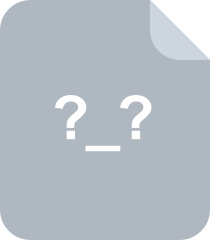
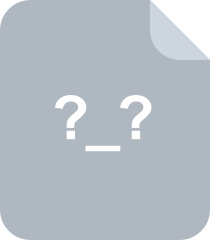
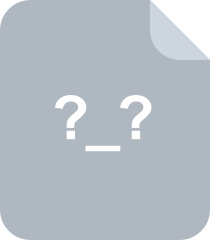
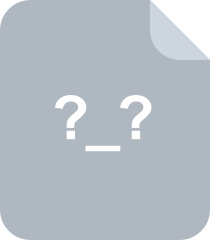
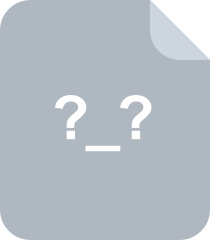
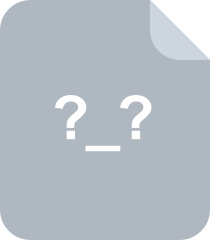
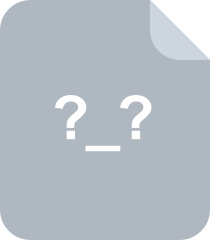
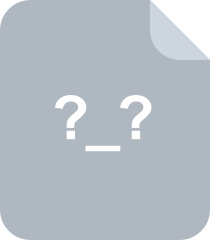
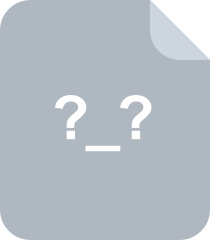
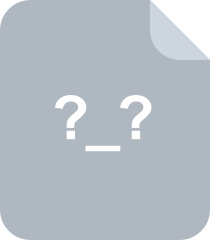
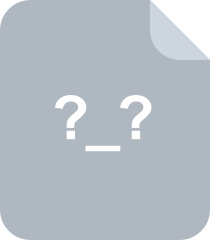
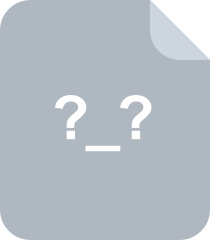
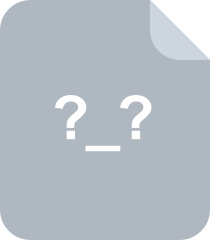
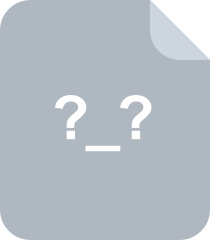
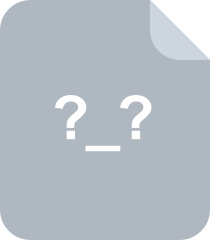
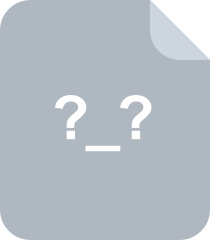
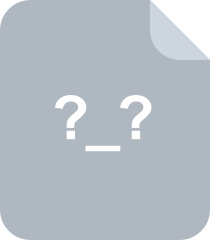
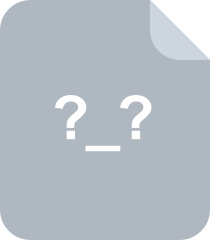
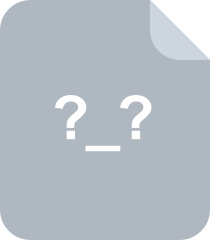
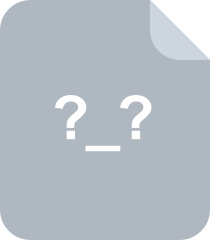
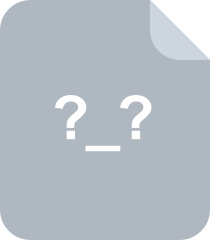
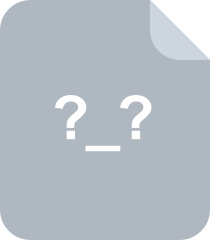
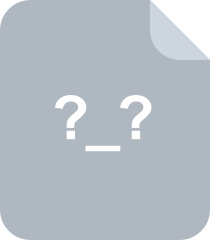
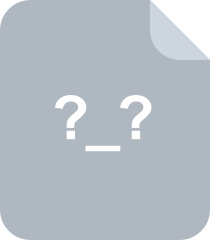
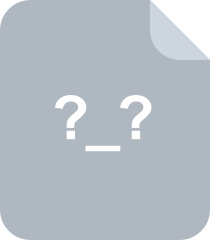
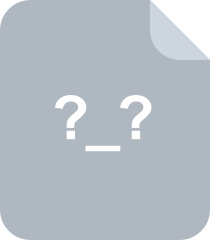
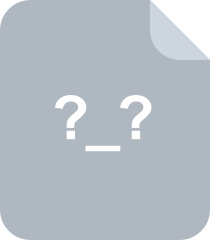
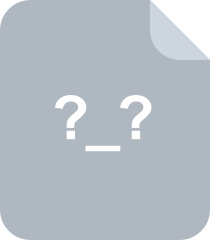
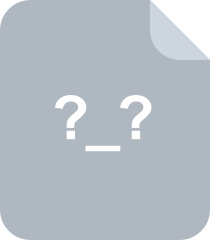
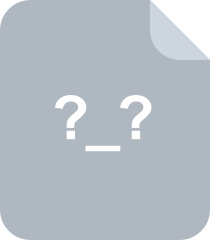
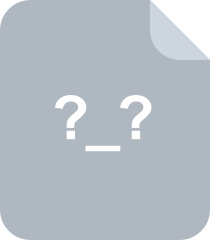
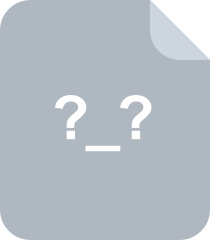
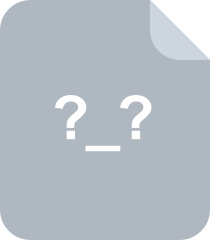
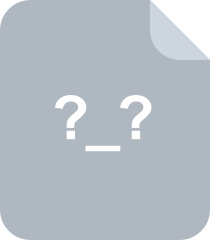
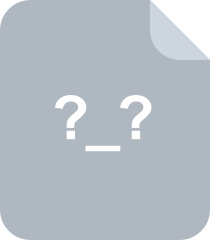
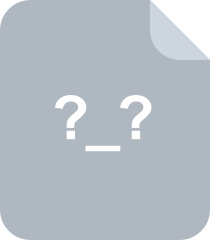
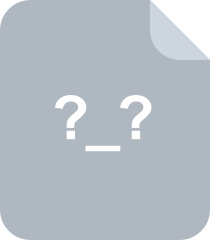
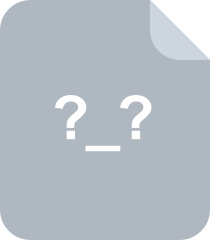
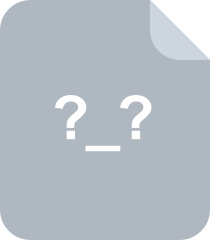
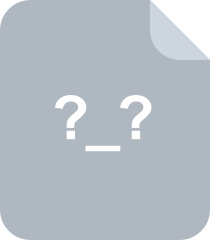
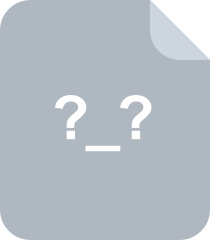
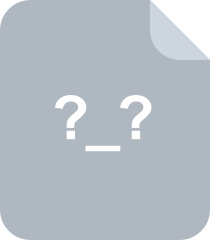
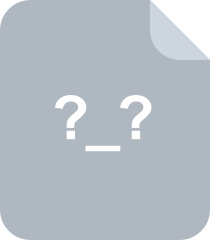
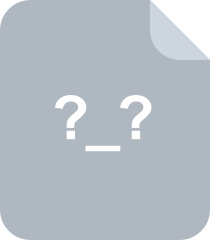
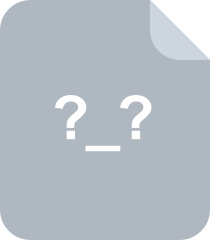
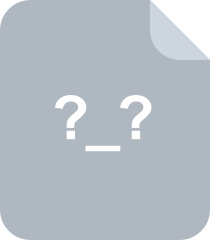
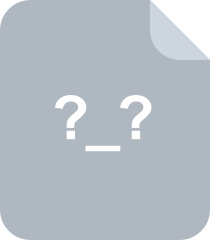
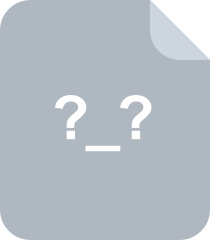
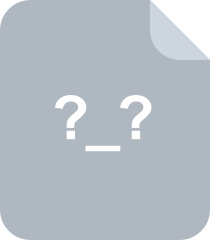
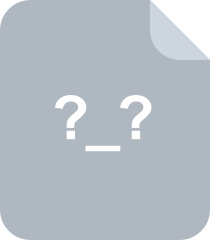
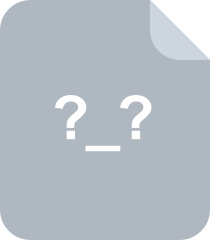
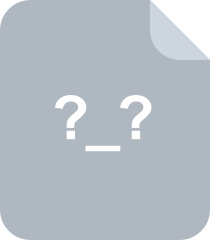
共 923 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10

小峰_想睡觉
- 粉丝: 14
- 资源: 18
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

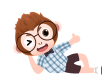
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


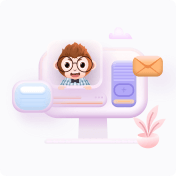
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页