<?php
if(!defined('IN_DISCUZ')) {
exit('Access Denied');
}
class plugin_dc_sell {
var $cvar=null;
var $open=false;
var $free=false;
var $extcredits=null;
var $lang=null;
var $reprule = null;
var $sellrule = null;
public function __construct(){
global $_G;
$this->cvar = $_G['cache']['plugin']['dc_sell'];
if(!$this->cvar['open']||(!in_array($_G['fid'], (array)unserialize($this->cvar['fids']))&&$_G['fid']))
return;
$this->open=true;
if(in_array($_G['groupid'], (array)unserialize($this->cvar['free'])))
$this->free = true;
$ext=array();
foreach($_G['setting']['extcredits'] as $k=>$v){
$ext[]=$k;
}
$extstr=implode('|',$ext);
$this->reprule = '/\[sell=([\d+]+?),('.$extstr .')\]([\s\S]+?)((\[\/sell\])|\.\.\.|$)/is';
$this->sellrule = '/\[sell=([\d+]+?),('.$extstr .')\]([\s\S]+?)\[\/sell\]/is';
$this->lang = @include DISCUZ_ROOT.'/source/plugin/dc_sell/config/lang.php';
}
public function discuzcode($p) {
global $_G;
if($p['caller']=='messagecutstr'){
$_G['discuzcodemessage'] = preg_replace($this->reprule, $this->lang('nopayhide'), $_G['discuzcodemessage']);
}else{
if($_GET['from']=='preview'||$_GET['action']=='printable')
$_G['discuzcodemessage'] = preg_replace($this->sellrule, $this->lang('nopayhide'), $_G['discuzcodemessage']);
}
}
function lang($str){
if(!empty($this->lang[$str]))
return $this->lang[$str];
else
return lang('plugin/dc_sell', $str);
}
}
class plugin_dc_sell_forum extends plugin_dc_sell{
function viewthread_postbottom_output() {
global $_G, $postlist,$post;
if(!$this->open)
return;
if(!empty($postlist)){
foreach($postlist as $id => $p) {
$postlist[$id] = $this->sellcodeprase($p);
}
}
if(!empty($post)){
$post=$this->sellcodeprase($post);
}
}
function sellcodeprase($post){
global $_G;
$message = $post['message'];
$pid = $post['pid'];
if(!in_array($post['groupid'], (array)unserialize($this->cvar['allow'])))
return $post;
preg_match_all($this->sellrule,$message,$matches);
if(!count($matches[3]))
return $post;
$plarr=C::t('#dc_sell#dc_sell_log')->fetch_by_pcuid(array('pid'=>$pid,'uid'=>$_G['uid']));
$sell=array();
foreach($plarr as $r){
$sell[$r['cid']]['display']=1;
}
for($i=0;$i<count($matches[3]);$i++){
if($sell[$i]['display']==1||$this->free||$post['authorid']==$_G['uid']||$_G['dc_plugin']['vip']['hook']['dc_sell']['view'])
$message = preg_replace($this->sellrule, $this->sell_reply($pid,$i), $message,1);
else
$message = preg_replace($this->sellrule, $this->sell_reply_hidden($pid,$post['authorid'],$matches[1][$i],$matches[2][$i],$i), $message,1);
}
$post['message']=$message;
return $post;
}
function sell_reply_hidden($pid,$authorid,$price,$credit,$i){
global $_G;
if($price>$this->cvar['maxprice'])
$price=$this->cvar['maxprice'];
$ck=substr(md5($_G['uid'].'|'.$pid.'|'.$authorid.'|'.$i.'|'.$price.'|'.$credit.'|'. TIMESTAMP .'|'.$_G['config']['security']['authkey']), 0, 8);
$sid=base64_encode($pid.'|'.$authorid.'|'.$i.'|'.$price.'|'.$credit.'|'.TIMESTAMP.'|'.$ck);
$str = '<div class="locked">';
$str .=str_replace(array('{username}','{price}'),array($_G['uid']?$_G['username']:lang('forum/template', 'guest'),$price.$_G['setting']['extcredits'][$credit]['unit'].$_G['setting']['extcredits'][$credit]['title']),$this->lang('hidetext'));
$str .= '<a href="javascript:;" class="y viewpay" title="'.$this->lang('paytext').'" onclick="showWindow(\'dc_sell\', \'plugin.php?id=dc_sell:pay&sid='.$sid.'&tid='.$_G['tid'].'\')">'.$this->lang('payfor').'</a></div>';
return $str;
}
function sell_reply($pid,$i){
global $_G;
return '<div class="showhide"><h4>'.$this->lang('selltext').($this->cvar['islog']?' <a href="javascript:;" title="'.$this->lang('selllog').'" onclick="showWindow(\'dc_sellview\', \'plugin.php?id=dc_sell:sellview&pid='.$pid.'&cid='.$i.'\')">'.$this->lang('selllog').'</a>':'').' </h4>\\3</div>';
}
function ad_headerbanner($params){
global $postlist;
if(defined('IN_ARCHIVER')){
foreach($postlist as $key => $post){
$post['message'] = preg_replace($this->reprule, $this->lang('nopayhide'), $post['message']);
$postlist[$key]=$post;
}
}
return $params['content'];
}
function post_editorctrl_left() {
global $_G;
if(!$this->open)
return;
if(!in_array($_G['groupid'], (array)unserialize($this->cvar['allow'])))
return;
return "<link rel=\"stylesheet\" href=\"source/plugin/dc_sell/img/btn_pay.css\" type=\"text/css\" /><script src=\"plugin.php?id=dc_sell:add\" type=\"text/javascript\"></script><a id=\"btn_sell\" title=\"".$this->lang('sellinsert')."\" onClick=\"addsell('btn_sell')\" href='javascript:void(0);' >".$this->lang('sell')."</a>";
}
}
class plugin_dc_sell_search extends plugin_dc_sell{
function forum_top_output(){
global $threadlist;
foreach($threadlist as $key => $post){
$post['message'] = preg_replace($this->reprule, $this->lang('nopayhide'), $post['message']);
$threadlist[$key]=$post;
}
}
}
class plugin_dc_sell_portal extends plugin_dc_sell{
function portalcp_bottom_output(){
global $thread,$article_content,$_G;
if(!$_GET['from_idtype']=='tid'||!$_GET['from_id'])
return;
if(!$this->cvar['open']||!in_array($thread['fid'], (array)unserialize($this->cvar['fids'])))
return;
$u=C::t('common_member')->fetch($thread['authorid']);
if(!in_array($u['groupid'], (array)unserialize($this->cvar['allow'])))
return;
$article_content['content'] = preg_replace($this->sellrule, str_replace('{tid}',$_GET['from_id'],$this->lang('carticle')), $article_content['content']);
}
}
class mobileplugin_dc_sell extends plugin_dc_sell{
public function discuzcode() {
global $_G;
$_G['discuzcodemessage'] = preg_replace($this->sellrule, $this->lang('nopayhide'), $_G['discuzcodemessage']);
}
}
?>

执刀人的工具库
- 粉丝: 1449
- 资源: 1563
最新资源
- 音乐网站(JSP+SERVLET).rar
- 抢购软件:快速复制信息
- oracle错误代码和信息速查手册chm版最新版本
- MATLAB【逆变器二次调频模型】 微电网分布式电源逆变器DROOP控制二次调频模型,加入二次控制实现二次调频控制,及二次调压控制,程序可实现上图功能,工况有所改变 需要matlab2021A版
- 基于python的网页自动化工具项目全套技术资料100%好用.zip
- Oracle数据库命令速查手册doc版最新版本
- 程序名称:转向设计计算程序 开发平台:基于matlab平台 计算内容:阿克曼转角,转弯半径,转向阻力矩,回正力矩,转向主参数,转向传动比,力矩波动,转向梯形,EPS匹配,HPS匹配,齿轮齿条传动比,循
- 基于二阶自抗扰ADRC的轨迹跟踪控制,对车辆的不确定性和外界干扰具有一定抗干扰性,基于carsim和simulink仿真 跟踪轨迹为双移线,效果良好,有对应复现资料,是学习自抗扰技术快速入门很好的资料
- TianleSoftwareOracle学习手册中文pdf格式最新版本
- MATLAB代码:基于分布式ADMM算法的考虑碳排放交易的电力系统优化调度研究 关键词:分布式调度 ADMM算法 交替方向乘子法 碳排放 最优潮流 仿真平台:MATLAB+CPLEX GUROBI
- Oracle安装配置使用WORD文档doc格式最新版本
- 西门子840D HMI ADVANCED FOR PC 也可用于810D,840DSL中文版 1、软件可安装到台式机或笔记本上,可以连接到机床的NCU进行NC与PLC的数据备份与恢复,备份和恢复的数
- OraclePLSQL简单安装指南WORD文档doc格式最新版本
- 网页数据采集软件项目全套技术资料100%好用.zip
- Oracle高级SQL培训与讲解WORD文档doc格式最新版本
- 超智能体写的人工智能深度学习pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


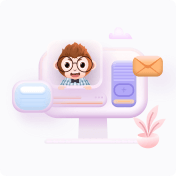