<?php
/**
* @package Options_Framework
* @author Devin Price <devin@wptheming.com>
* @license GPL-2.0+
* @link http://wptheming.com
* @copyright 2010-2014 WP Theming
*/
class Options_Framework_Interface {
/**
* Generates the tabs that are used in the options menu
*/
static function optionsframework_tabs() {
$counter = 0;
$options = & Options_Framework::_optionsframework_options();
$menu = '';
foreach ( $options as $value ) {
// Heading for Navigation
if ( $value['type'] == "heading" ) {
$counter++;
$class = '';
$class = ! empty( $value['id'] ) ? $value['id'] : $value['name'];
$class = preg_replace( '/[^a-zA-Z0-9._\-]/', '', strtolower($class) ) . '-tab';
$menu .= '<a id="options-group-'. $counter . '-tab" class="nav-tab ' . $class .'" title="' . esc_attr( $value['name'] ) . '" href="' . esc_attr( '#options-group-'. $counter ) . '">' . esc_html( $value['name'] ) . '</a>';
}
}
return $menu;
}
/**
* Generates the options fields that are used in the form.
*/
static function optionsframework_fields() {
global $allowedtags;
$options_framework = new Options_Framework;
$option_name = $options_framework->get_option_name();
$settings = get_option( $option_name );
$options = & Options_Framework::_optionsframework_options();
$counter = 0;
$menu = '';
foreach ( $options as $value ) {
$val = '';
$select_value = '';
$output = '';
// Wrap all options
if ( ( $value['type'] != "heading" ) && ( $value['type'] != "info" ) ) {
// Keep all ids lowercase with no spaces
$value['id'] = preg_replace('/[^a-zA-Z0-9._\-]/', '', strtolower($value['id']) );
$id = 'section-' . $value['id'];
$class = 'section';
if ( isset( $value['type'] ) ) {
$class .= ' section-' . $value['type'];
}
if ( isset( $value['class'] ) ) {
$class .= ' ' . $value['class'];
}
$output .= '<div id="' . esc_attr( $id ) .'" class="' . esc_attr( $class ) . '">'."\n";
if ( isset( $value['name'] ) ) {
$output .= '<h4 class="heading">' . esc_html( $value['name'] ) . '</h4>' . "\n";
}
if ( $value['type'] != 'editor' ) {
$output .= '<div class="option">' . "\n" . '<div class="controls">' . "\n";
}
else {
$output .= '<div class="option">' . "\n" . '<div>' . "\n";
}
}
// Set default value to $val
if ( isset( $value['std'] ) ) {
$val = $value['std'];
}
// If the option is already saved, override $val
if ( ( $value['type'] != 'heading' ) && ( $value['type'] != 'info') ) {
if ( isset( $settings[($value['id'])]) ) {
$val = $settings[($value['id'])];
// Striping slashes of non-array options
if ( !is_array($val) ) {
$val = stripslashes( $val );
}
}
}
// If there is a description save it for labels
$explain_value = '';
if ( isset( $value['desc'] ) ) {
$explain_value = $value['desc'];
}
// Set the placeholder if one exists
$placeholder = '';
if ( isset( $value['placeholder'] ) ) {
$placeholder = ' placeholder="' . esc_attr( $value['placeholder'] ) . '"';
}
if ( has_filter( 'optionsframework_' . $value['type'] ) ) {
$output .= apply_filters( 'optionsframework_' . $value['type'], $option_name, $value, $val );
}
switch ( $value['type'] ) {
// Basic text input
case 'text':
$output .= '<input id="' . esc_attr( $value['id'] ) . '" class="of-input" name="' . esc_attr( $option_name . '[' . $value['id'] . ']' ) . '" type="text" value="' . esc_attr( $val ) . '"' . $placeholder . ' />';
break;
// Password input
case 'password':
$output .= '<input id="' . esc_attr( $value['id'] ) . '" class="of-input" name="' . esc_attr( $option_name . '[' . $value['id'] . ']' ) . '" type="password" value="' . esc_attr( $val ) . '" />';
break;
// Textarea
case 'textarea':
$rows = '8';
if ( isset( $value['settings']['rows'] ) ) {
$custom_rows = $value['settings']['rows'];
if ( is_numeric( $custom_rows ) ) {
$rows = $custom_rows;
}
}
$val = stripslashes( $val );
$output .= '<textarea id="' . esc_attr( $value['id'] ) . '" class="of-input" name="' . esc_attr( $option_name . '[' . $value['id'] . ']' ) . '" rows="' . $rows . '"' . $placeholder . '>' . esc_textarea( $val ) . '</textarea>';
break;
// Select Box
case 'select':
$output .= '<select class="of-input" name="' . esc_attr( $option_name . '[' . $value['id'] . ']' ) . '" id="' . esc_attr( $value['id'] ) . '">';
foreach ($value['options'] as $key => $option ) {
$output .= '<option'. selected( $val, $key, false ) .' value="' . esc_attr( $key ) . '">' . esc_html( $option ) . '</option>';
}
$output .= '</select>';
break;
// Radio Box
case "radio":
$name = $option_name .'['. $value['id'] .']';
foreach ($value['options'] as $key => $option) {
$id = $option_name . '-' . $value['id'] .'-'. $key;
$output .= '<input class="of-input of-radio" type="radio" name="' . esc_attr( $name ) . '" id="' . esc_attr( $id ) . '" value="'. esc_attr( $key ) . '" '. checked( $val, $key, false) .' /><label for="' . esc_attr( $id ) . '">' . esc_html( $option ) . '</label>';
}
break;
// Image Selectors
case "images":
$name = $option_name .'['. $value['id'] .']';
foreach ( $value['options'] as $key => $option ) {
$selected = '';
if ( $val != '' && ($val == $key) ) {
$selected = ' of-radio-img-selected';
}
$output .= '<input type="radio" id="' . esc_attr( $value['id'] .'_'. $key) . '" class="of-radio-img-radio" value="' . esc_attr( $key ) . '" name="' . esc_attr( $name ) . '" '. checked( $val, $key, false ) .' />';
$output .= '<div class="of-radio-img-label">' . esc_html( $key ) . '</div>';
$output .= '<img src="' . esc_url( $option ) . '" alt="' . $option .'" class="of-radio-img-img' . $selected .'" onclick="document.getElementById(\''. esc_attr($value['id'] .'_'. $key) .'\').checked=true;" />';
}
break;
// Checkbox
case "checkbox":
$output .= '<input id="' . esc_attr( $value['id'] ) . '" class="checkbox of-input" type="checkbox" name="' . esc_attr( $option_name . '[' . $value['id'] . ']' ) . '" '. checked( $val, 1, false) .' />';
$output .= '<label class="explain" for="' . esc_attr( $value['id'] ) . '">' . wp_kses( $explain_value, $allowedtags) . '</label>';
break;
// Multicheck
case "multicheck":
foreach ($value['options'] as $key => $option) {
$checked = '';
$label = $option;
$option = preg_replace('/[^a-zA-Z0-9._\-]/', '', strtolower($key));
$id = $option_name . '-' . $value['id'] . '-'. $option;
$name = $option_name . '[' . $value['id'] . '][' . $option .']';
if ( isset($val[$option]) ) {
$checked = checked($val[$option], 1, false);
}
$output .= '<input id="' . esc_attr( $id ) . '" class="checkbox of-input" type="checkbox" name="' . esc_attr( $name ) . '" ' . $checked . ' /><label for="' . esc_attr( $id ) . '">' . esc_html( $label ) . '</label>';
}
break;
// Color picker
case "color":
$default_color = '';
if ( isset($value['std']) ) {
if ( $val != $value['std'] )
$default_color = ' data-default-color="' .$value['std'] . '" ';
}
$output .= '<input name="' . esc_attr( $option_name . '[' . $value['id'] . ']' ) . '" id="' . esc_attr( $value['id'] ) . '" class="of-color" type="text" value="' . esc_attr( $val ) . '"' . $default_color .' />';
break;
// Uploader
case "upload":
$output .= Options_Framework_Media_Uploader::optionsframework_uploader( $value['id'], $val, null );
break;
// Typography
case 'typography':
unset( $font_size, $font_style, $font_face, $font_color );
$typography_defaults = array(
'size' => '',
'face' => '',
'style' => '',
'color' => ''
);
$typography_stored = wp_parse_args( $val, $typography_defaults );
$typo
没有合适的资源?快使用搜索试试~ 我知道了~
Dobby主题 大气响应式WordPress博客主题模板修复版.zip
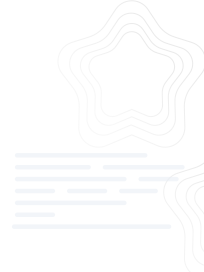
共129个文件
png:53个
php:35个
js:15个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 97 浏览量
2021-11-09
09:17:50
上传
评论
收藏 2MB ZIP 举报
温馨提示
这个是修复css js版本 Dobby主题 大气响应式WordPress博客主题模板 开源并且拥有自适应效果的主题,他能够在任何浏览器下进行友好体验的访问。Dobby 秉持了专心写作专心阅读的特点,简单大方的主页构造,使得博客能在臃肿杂乱的环境中脱颖而出。Dobby 主题内置了强大的主题后台控制平台,可以轻松设置关键字及站点描述,自定义的顶部样式,强大的底部社交化组件,以及漂亮的博客订阅功能组件,让你的网站更加与众不同,更多的页面搭配风格等你来发掘! Dobby 主题特色: 响应式设计,在电脑、平板和手机端完美展现 自定义头部图片 内置图片轮播组件 页脚社交化小工具 内置多种广告栏小工具 强大的后台订制功能 自定义的主题配色 支持 WordPress 3.5 以上版本,并完美支持最新的 WordPress 5.3
资源详情
资源评论
资源推荐
收起资源包目录

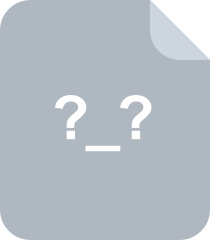
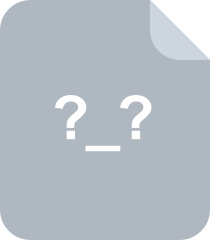
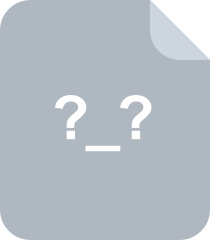
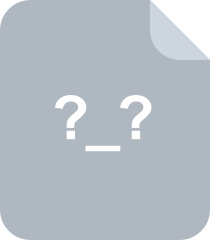
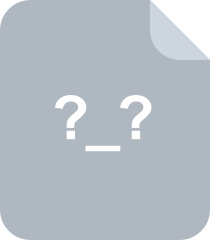
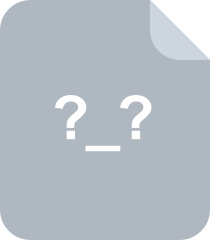
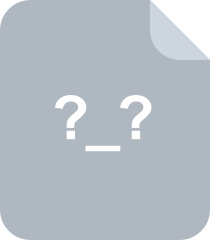
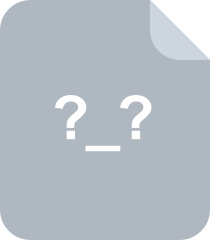
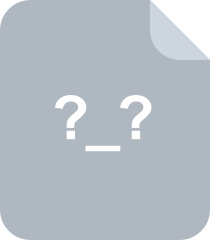
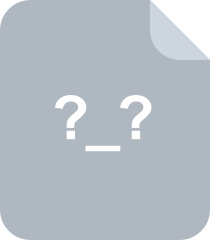
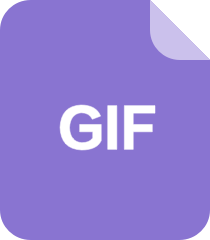
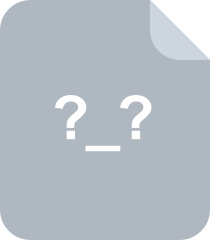
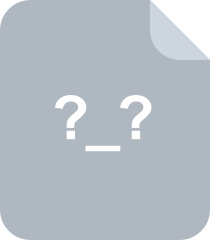
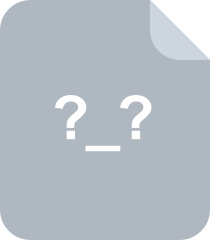
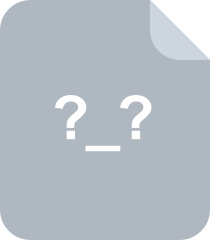
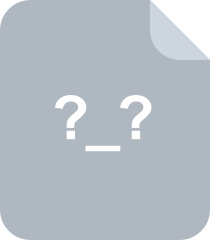
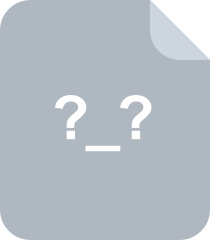
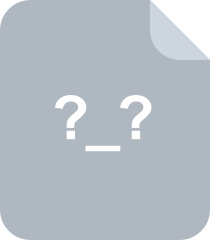
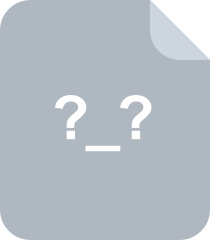
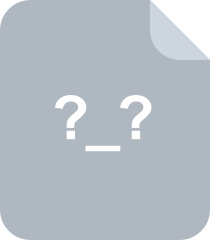
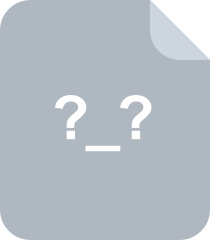
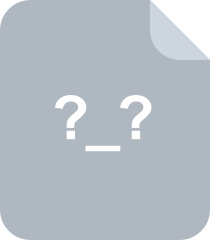
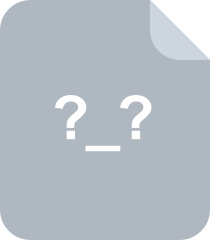
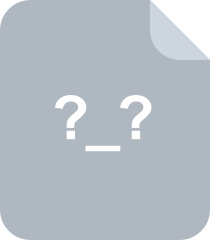
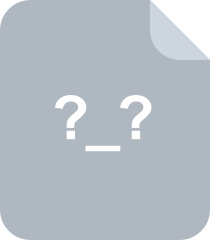
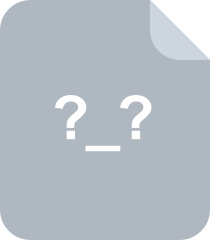
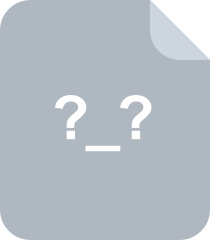
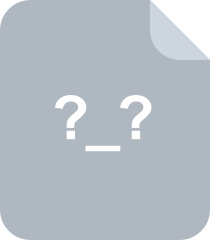
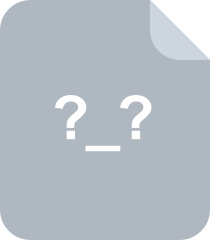
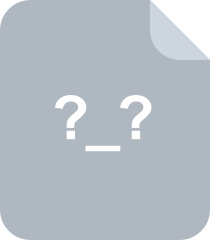
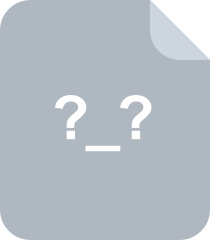
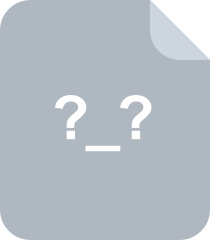
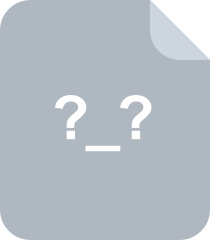
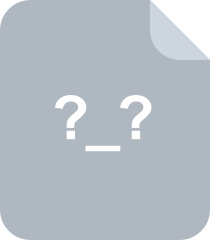
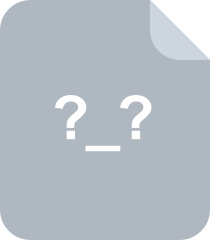
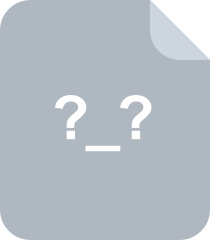
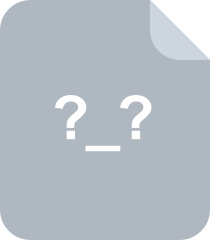
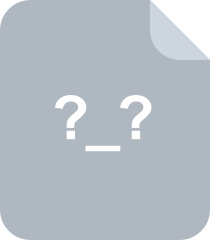
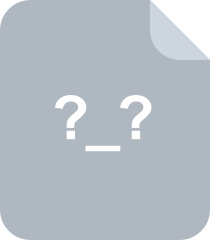
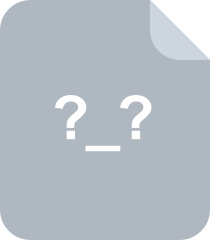
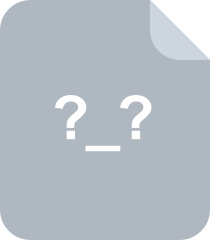
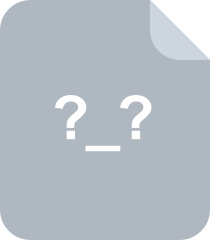
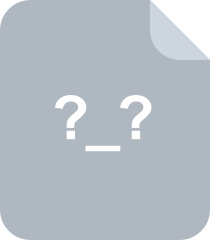
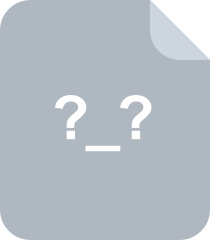
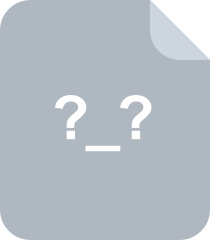
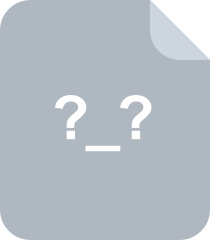
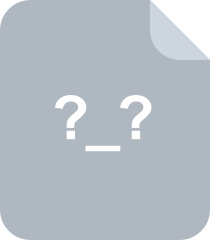
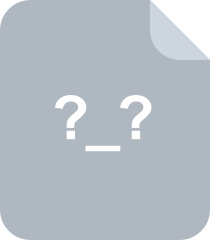
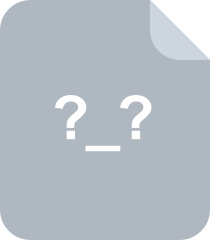
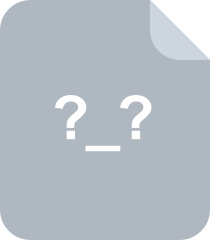
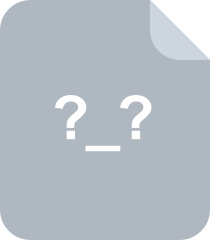
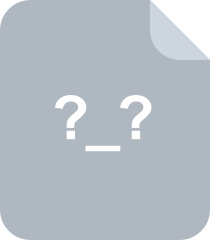
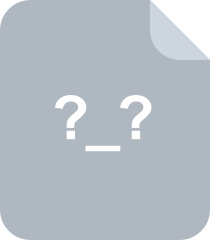
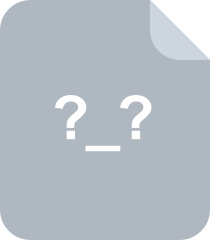
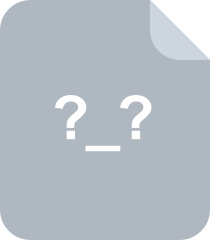
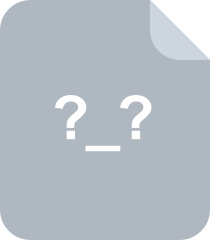
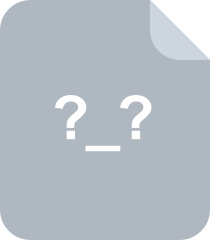
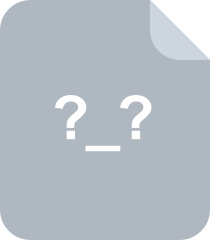
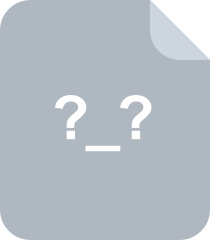
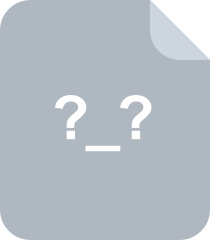
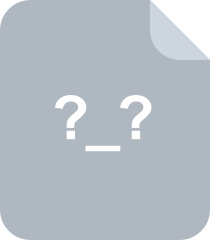
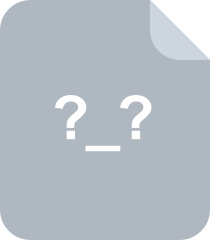
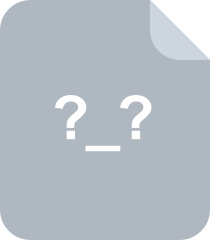
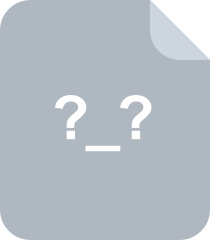
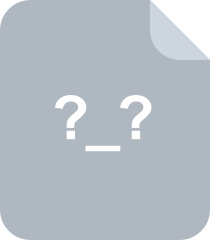
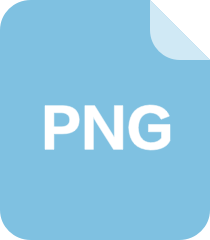
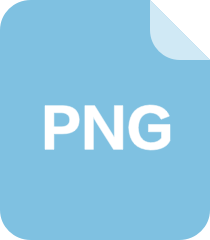
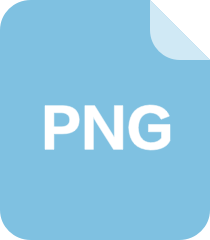
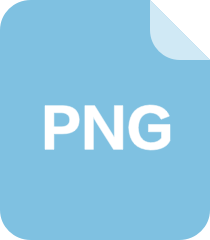
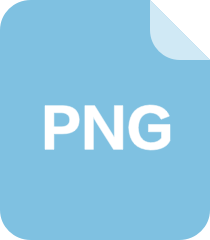
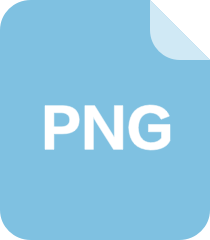
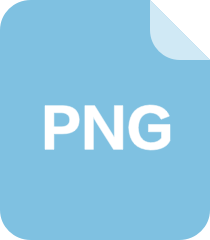
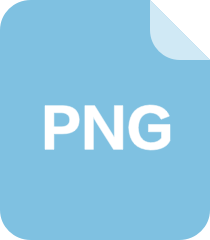
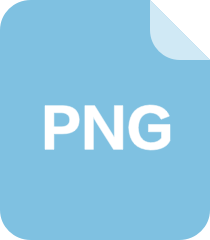
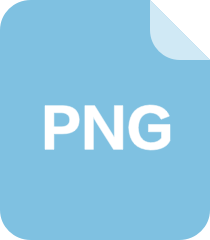
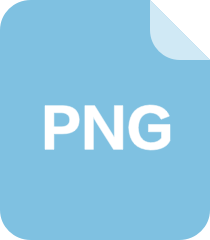
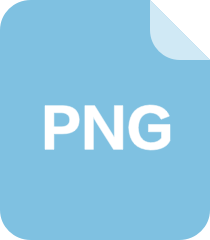
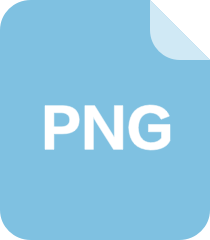
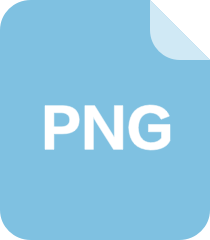
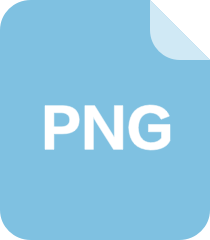
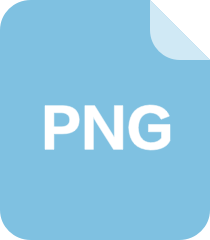
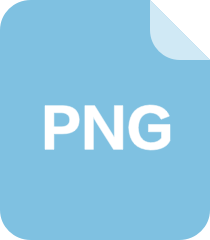
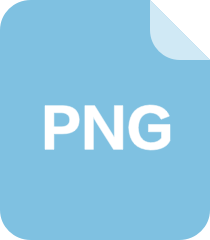
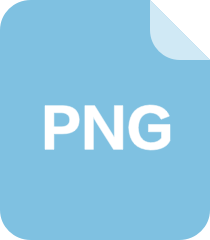
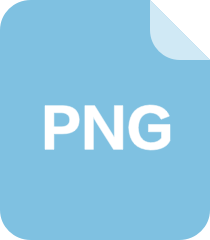
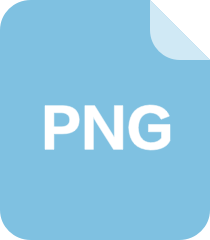
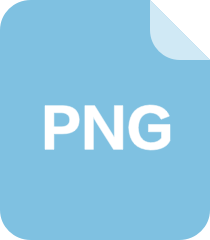
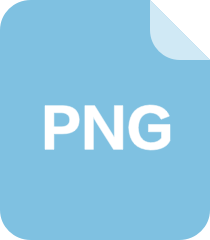
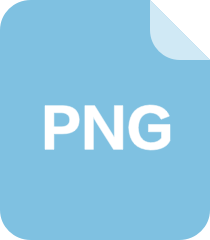
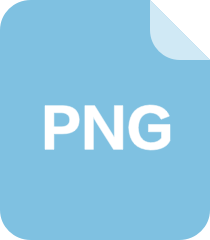
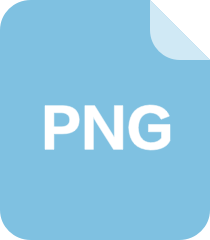
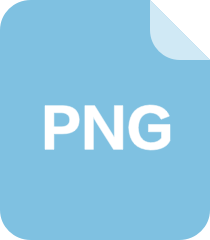
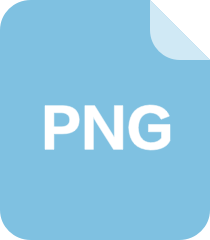
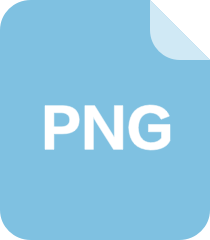
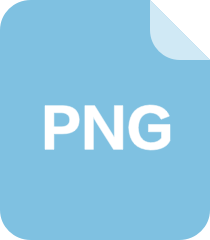
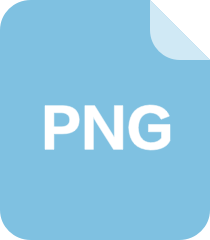
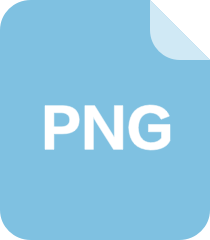
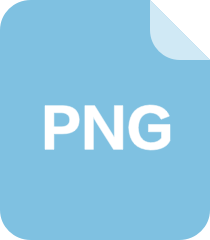
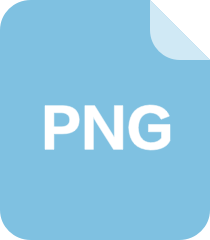
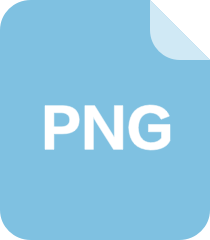
共 129 条
- 1
- 2
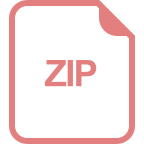
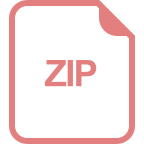
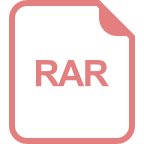
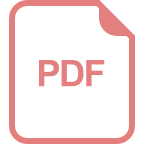
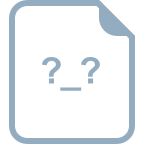
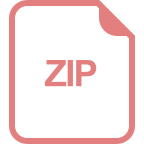
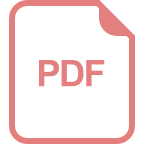
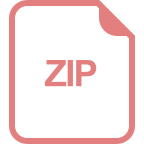
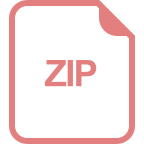
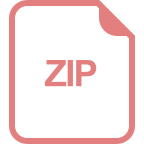
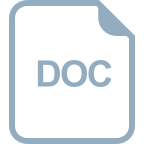
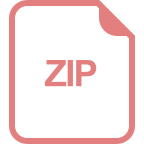
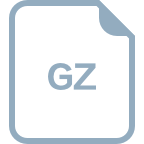
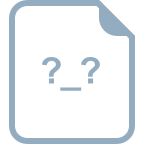
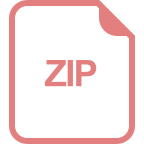
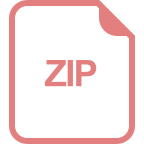
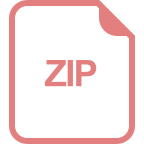
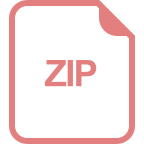
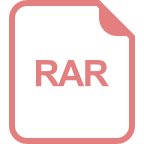
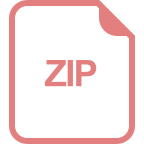
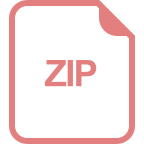
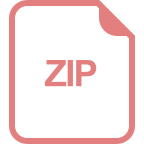
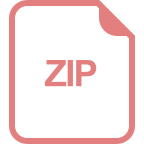

执刀人的工具库
- 粉丝: 1448
- 资源: 1563
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

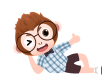
最新资源
- 环境监测系统源代码全套技术资料.zip
- 前端分析-2023071100789
- 前端分析-2023071100789
- 基于springboot的调查问卷管理系统源代码全套技术资料.zip
- MATLAB代码:计及碳排放交易及多种需求响应的微网 电厂日前优化调度 关键词:碳排放交易 需求响应 空调负荷 电动汽车 微网 电厂优化调度 参考文档:计及电动汽车和需求响应的多类电力市场下
- 全国高校计算机能力挑战赛往届真题整理
- 小程序毕业设计项目-音乐播放器
- MATLAB代码:考虑多微网电能互补与需求响应的微网双层优化模型 关键词:多微网 电能互补 需求响应 双层优化 动态定价 能量管理 参考文档:《自编文档》 仿真平台:MATLAB+CPLEX 主要
- 智慧校园后勤管理系统源代码全套技术资料.zip
- MATLAB代码:含多种需求响应及电动汽车的微网 电厂日前优化调度 关键词:需求响应 空调负荷 电动汽车 微网优化调度 电厂调度 仿真平台:MATLAB+CPLEX 主要内容:代码主要做的是一
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


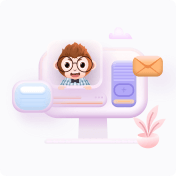
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0