package com.cl.controller;
import java.math.BigDecimal;
import java.text.SimpleDateFormat;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.cl.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.cl.annotation.IgnoreAuth;
import com.cl.entity.OrdersEntity;
import com.cl.entity.view.OrdersView;
import com.cl.service.OrdersService;
import com.cl.service.TokenService;
import com.cl.utils.PageUtils;
import com.cl.utils.R;
import com.cl.utils.MPUtil;
import com.cl.utils.CommonUtil;
import java.io.IOException;
/**
* 商品订单
* 后端接口
* @author
* @email
* @date 2024-03-16 20:05:23
*/
@RestController
@RequestMapping("/orders")
public class OrdersController {
@Autowired
private OrdersService ordersService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,OrdersEntity orders,
HttpServletRequest request){
if(!request.getSession().getAttribute("role").toString().equals("管理员")) {
orders.setUserid((Long)request.getSession().getAttribute("userId"));
}
EntityWrapper<OrdersEntity> ew = new EntityWrapper<OrdersEntity>();
PageUtils page = ordersService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, orders), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,OrdersEntity orders,
HttpServletRequest request){
EntityWrapper<OrdersEntity> ew = new EntityWrapper<OrdersEntity>();
PageUtils page = ordersService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, orders), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( OrdersEntity orders){
EntityWrapper<OrdersEntity> ew = new EntityWrapper<OrdersEntity>();
ew.allEq(MPUtil.allEQMapPre( orders, "orders"));
return R.ok().put("data", ordersService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(OrdersEntity orders){
EntityWrapper< OrdersEntity> ew = new EntityWrapper< OrdersEntity>();
ew.allEq(MPUtil.allEQMapPre( orders, "orders"));
OrdersView ordersView = ordersService.selectView(ew);
return R.ok("查询商品订单成功").put("data", ordersView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
OrdersEntity orders = ordersService.selectById(id);
orders = ordersService.selectView(new EntityWrapper<OrdersEntity>().eq("id", id));
return R.ok().put("data", orders);
}
/**
* 前端详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
OrdersEntity orders = ordersService.selectById(id);
orders = ordersService.selectView(new EntityWrapper<OrdersEntity>().eq("id", id));
return R.ok().put("data", orders);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody OrdersEntity orders, HttpServletRequest request){
orders.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(orders);
orders.setUserid((Long)request.getSession().getAttribute("userId"));
ordersService.insert(orders);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody OrdersEntity orders, HttpServletRequest request){
orders.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(orders);
ordersService.insert(orders);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
@Transactional
public R update(@RequestBody OrdersEntity orders, HttpServletRequest request){
//ValidatorUtils.validateEntity(orders);
ordersService.updateById(orders);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
ordersService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* (按值统计)
*/
@RequestMapping("/value/{xColumnName}/{yColumnName}")
public R value(@PathVariable("yColumnName") String yColumnName, @PathVariable("xColumnName") String xColumnName,HttpServletRequest request) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("xColumn", xColumnName);
params.put("yColumn", yColumnName);
EntityWrapper<OrdersEntity> ew = new EntityWrapper<OrdersEntity>();
ew.in("status", new String[]{"已支付","已发货","已完成"}).ne("type",2);
List<Map<String, Object>> result = ordersService.selectValue(params, ew);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
for(Map<String, Object> m : result) {
for(String k : m.keySet()) {
if(m.get(k) instanceof Date) {
m.put(k, sdf.format((Date)m.get(k)));
}
}
}
return R.ok().put("data", result);
}
/**
* (按值统计(多))
*/
@RequestMapping("/valueMul/{xColumnName}")
public R valueMul(@PathVariable("xColumnName") String xColumnName,@RequestParam String yColumnNameMul, HttpServletRequest request) {
String[] yColumnNames = yColumnNameMul.split(",");
Map<String, Object> params = new HashMap<String, Object>();
params.put("xColumn", xColumnName);
List<List<Map<String, Object>>> result2 = new ArrayList<List<Map<String,Object>>>();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
EntityWrapper<OrdersEntity> ew = new EntityWrapper<OrdersEntity>();
for(int i=0;i<yColumnNames.length;i++) {
params.put("yColumn", yColumnNames[i]);
List<Map<String, Object>> result = ordersService.selectValue(params, ew);
for(Map<String, Object> m : result) {
for(String k : m.keySet()) {
if(m.get(k) instanceof Date) {
m.put(k, sdf.format((Date)m.get(k)));
}
}
}
result2.add(result);
}
return R.ok().put("data", result2);
}
/**
* (按值统计)时间统计类型
*/
@RequestMapping("/value/{xColumnName}/{yColumnName}/{timeStatType}")
public R valueDay(@PathVariable("yColumnName") String yColumnName, @PathVariable("xColumnName") String xColumnName, @PathVariable("timeStatType") String timeStatType
没有合适的资源?快使用搜索试试~ 我知道了~
基于Spring Boot+Vue技术的湖南特产销售网站(编号:17755125).zip
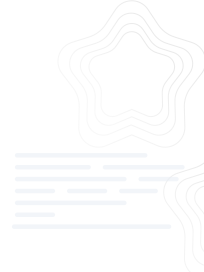
共367个文件
java:100个
vue:74个
png:47个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 149 浏览量
2024-12-23
15:03:33
上传
评论
收藏 11.27MB ZIP 举报
温馨提示
基于Spring Boot+Vue技术的湖南特产销售网站(编号:17755125).zip
资源推荐
资源详情
资源评论
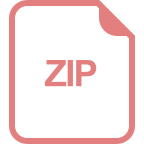
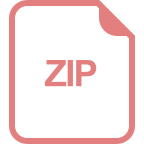
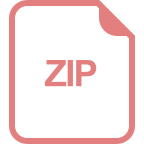
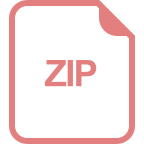
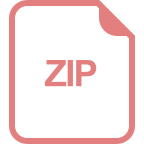
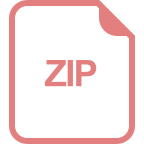
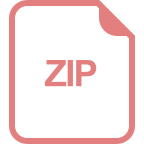
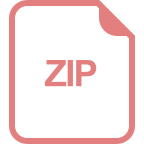
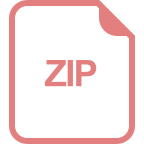
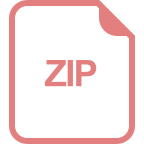
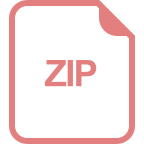
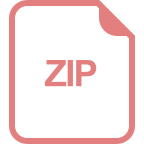
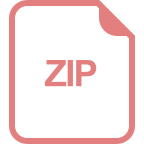
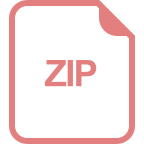
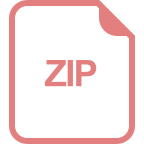
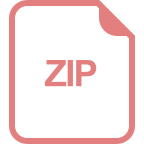
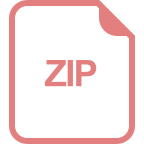
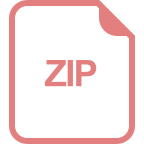
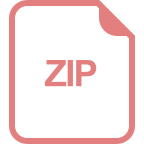
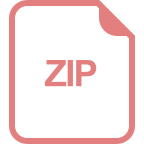
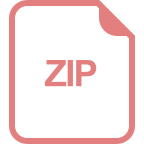
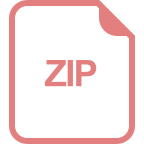
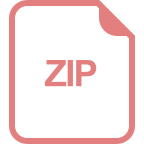
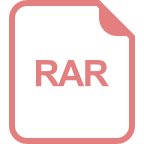
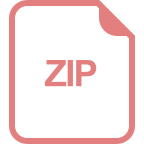
收起资源包目录

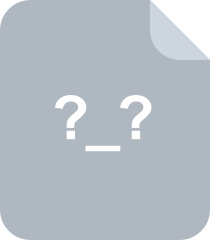
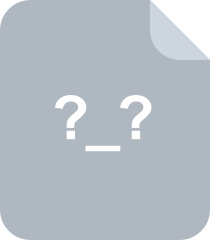
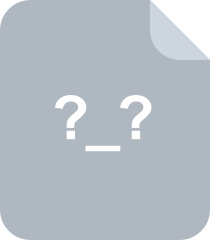
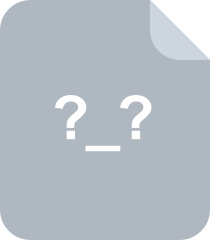
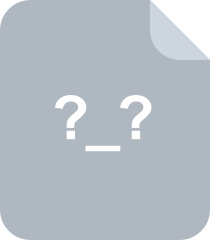
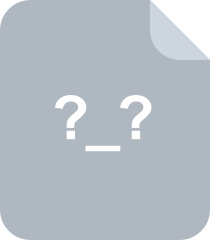
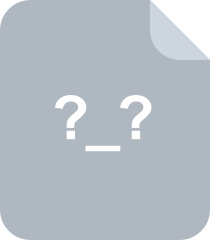
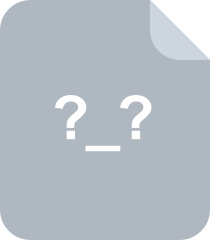
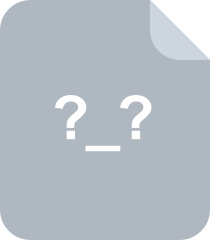
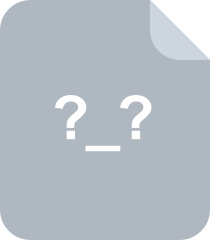
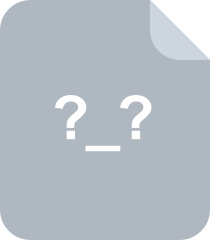
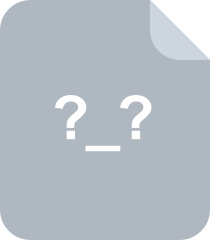
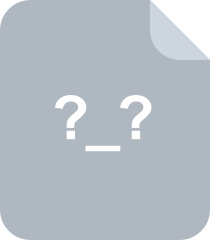
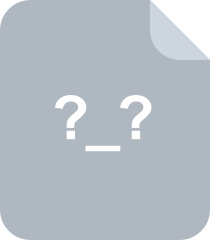
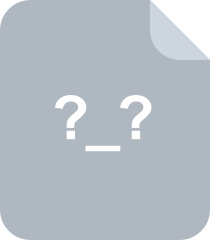
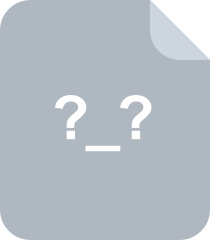
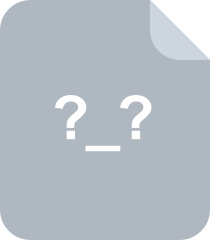
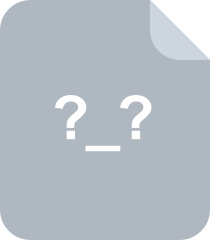
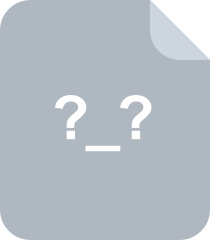
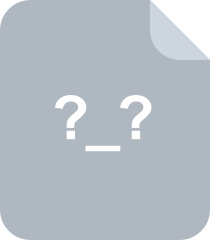
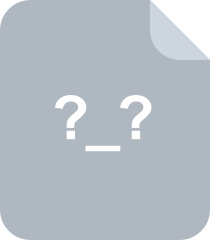
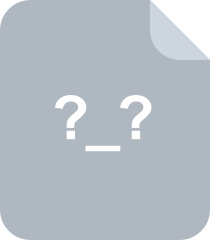
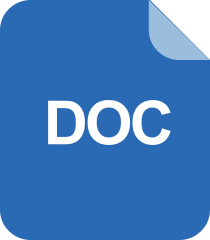
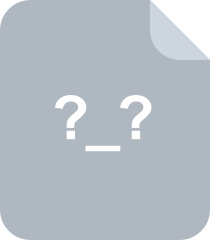
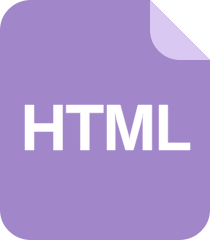
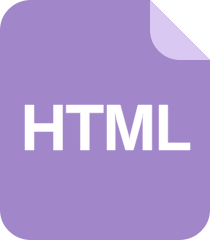
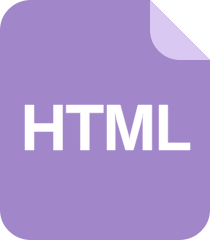
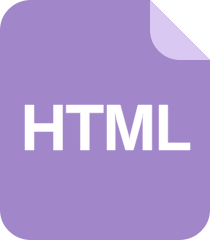
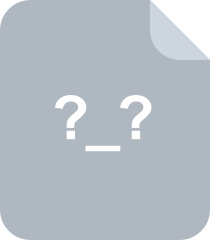
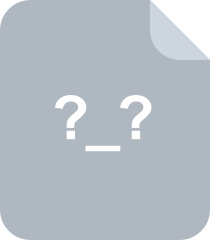
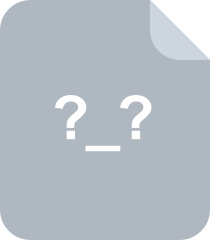
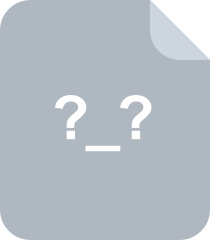
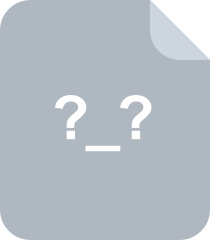
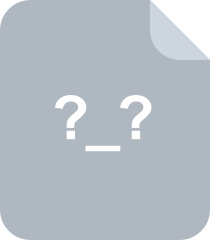
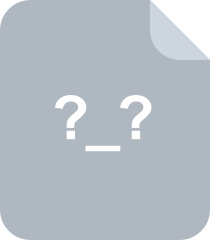
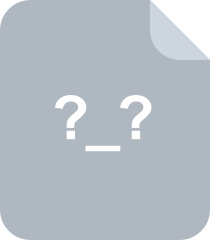
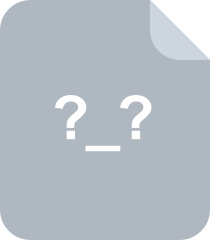
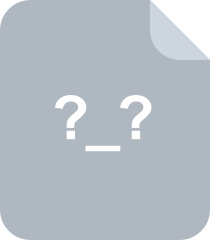
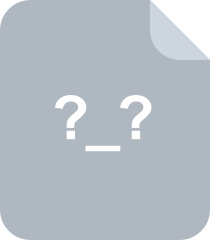
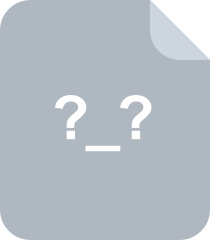
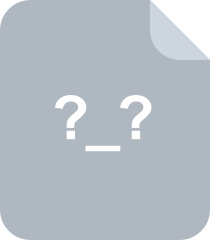
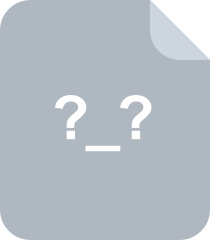
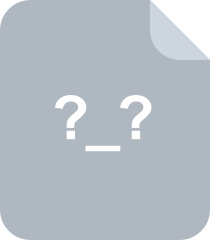
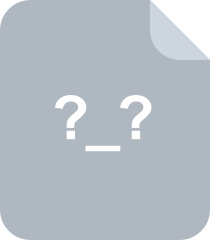
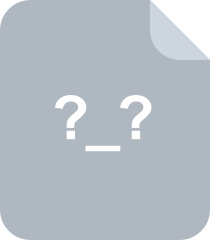
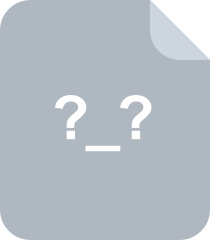
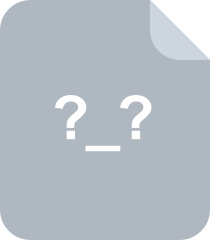
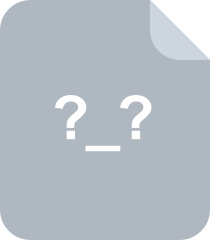
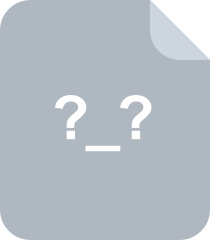
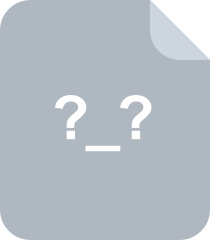
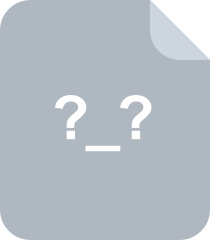
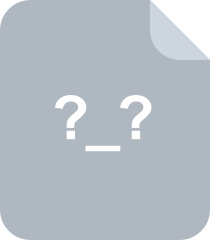
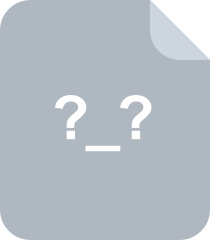
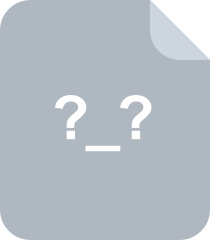
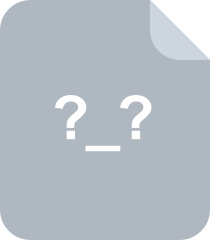
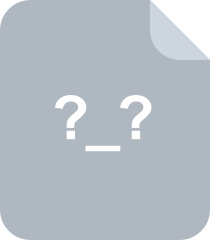
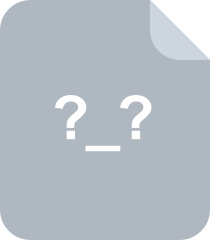
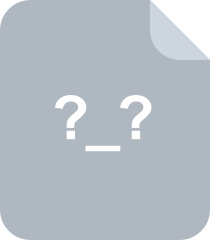
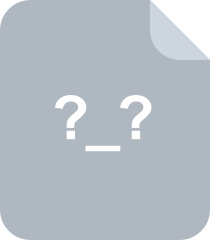
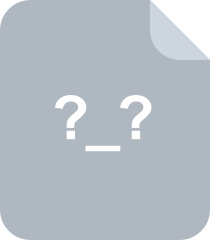
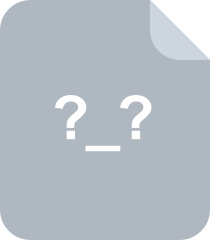
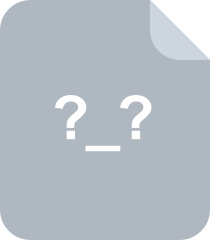
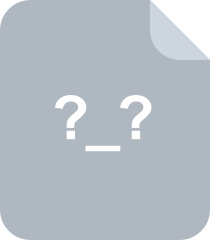
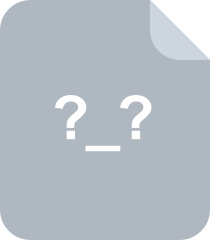
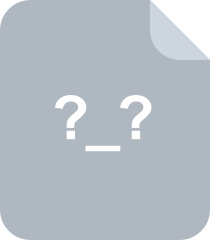
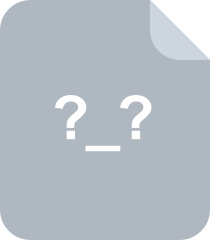
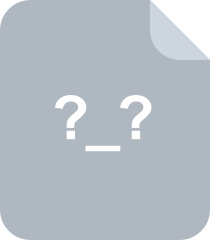
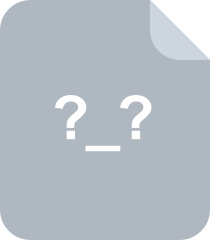
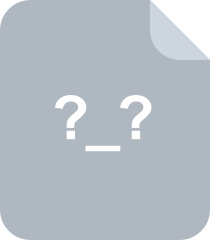
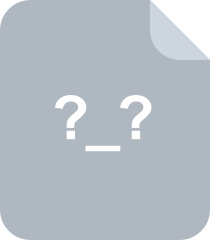
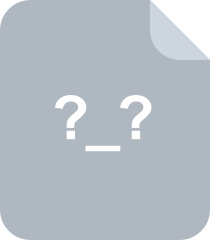
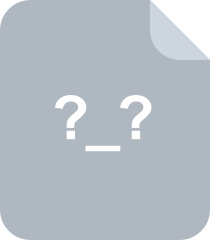
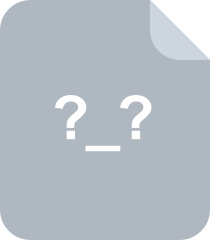
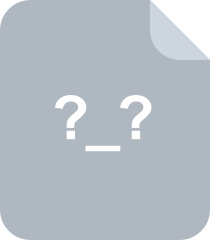
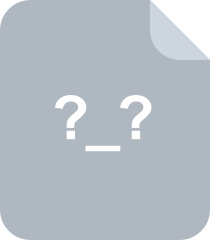
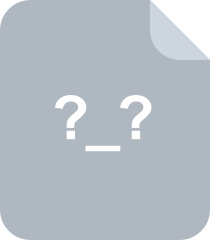
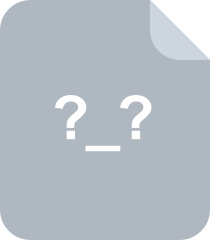
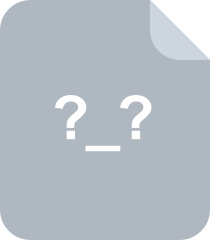
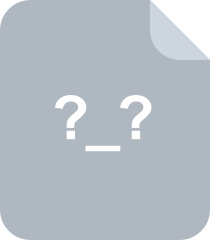
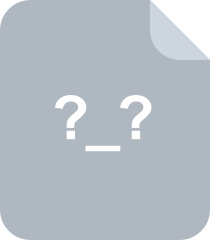
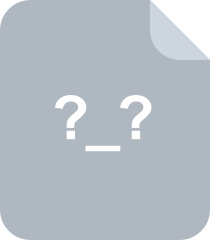
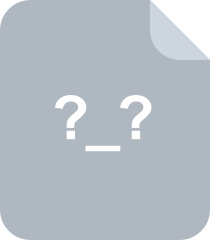
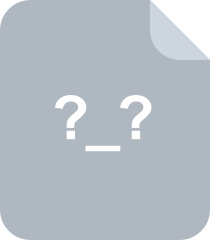
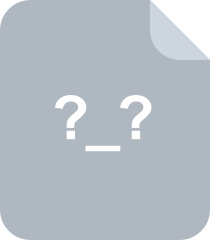
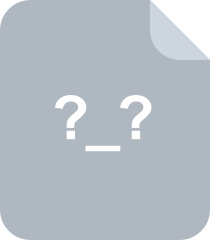
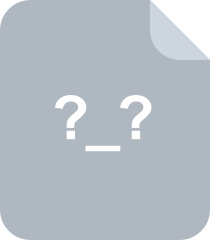
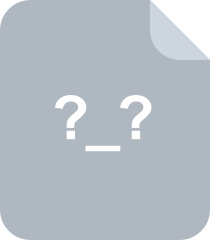
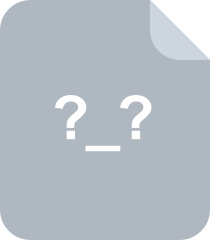
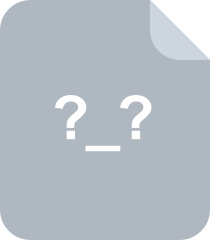
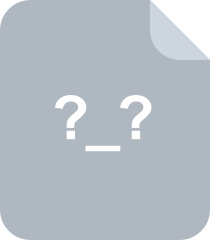
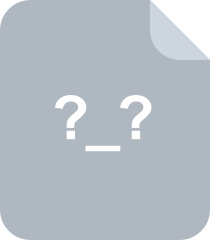
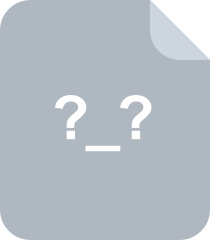
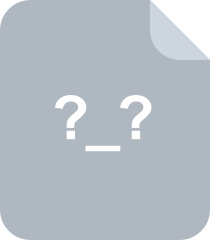
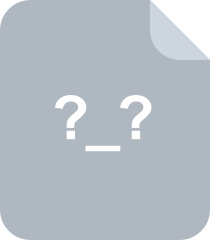
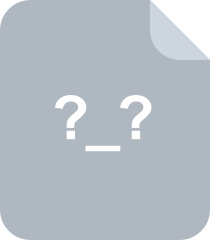
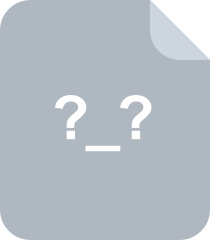
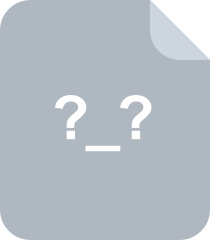
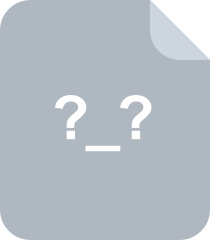
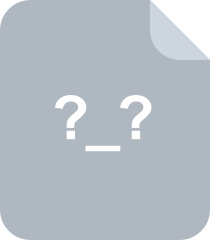
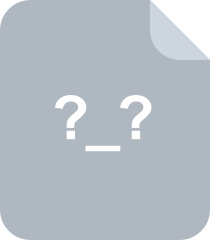
共 367 条
- 1
- 2
- 3
- 4
资源评论
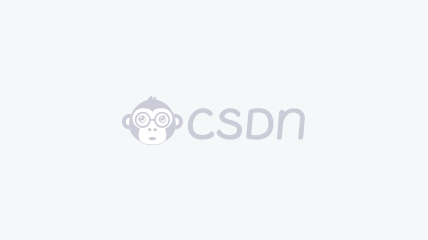
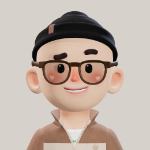
清水白石008
- 粉丝: 1w+
- 资源: 1462
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

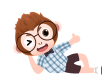
最新资源
- 102 -网店客服员工手册.docx
- 139 -员工手册(管理篇).docx
- 193 -XX电子商务公司员工手册.doc.docx
- 11 -联想员工手册.doc
- 08 -江苏宏图三胞员工手册.doc
- 新员工关怀问卷 2页.docx
- 新员工关怀事项 2页.doc
- 新员工关怀计划第一次面谈表 1页.doc
- 入职关怀操作流程 5页.doc
- 新员工满月关怀记录表.xlsx
- 新员工闯关任务表(员工关怀).xls
- 新员工入职关怀引导表.xls
- 新员工关爱管理办法 2页.doc
- 多目标粒子群优化算法,MOPSO,采用mopso求解多目标优化问题,解得pareto最优解 Matlab环境下的.m程序,采用模块化编程,便于修改,注释率高,易于理解学习 欢迎各位大佬前来咨询
- 主控芯片dsp tms320f28335,基于Matlab Simulink开发的嵌入式模型,模型可自动生成ccs工程代码,生成的代码可直接运行在主控芯片中 该模型利用id=0的矢量控制,实现了永磁
- 电机马达DSP28335 永磁同步电机代码 CCS编辑,有PI控制算法、速度电流双闭环控制 有方波有感无感算法,无感为3段反电势过零点 有pmsm有感无感算法,有感有hall的foc,有磁编
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


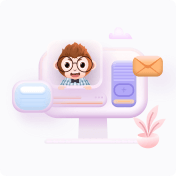
安全验证
文档复制为VIP权益,开通VIP直接复制
