### Android界面开发基础知识点 #### 一、Android UI概述 Android UI(User Interface)是指用户与Android应用程序进行交互的视觉和操作界面。一个优秀的UI不仅能够提升用户体验,还能够提高应用的下载量和活跃度。本章节将从Android UI的基础概念出发,深入探讨UI设计原理及其在Android平台上的实现方式。 #### 二、UI组件简介 1. **视图(View)**:是UI的基本组成单元,用于显示单一类型的界面元素。 2. **视图组(ViewGroup)**:是一个容器,可以包含多个View或ViewGroup,用来组织和管理UI布局。 3. **布局(Layout)**:定义了UI元素之间的相对位置和大小,常见的布局有LinearLayout(线性布局)、RelativeLayout(相对布局)等。 4. **控件(Widget)**:通常指的是具有复杂功能的视图,如Button(按钮)、EditText(输入框)等。 #### 三、布局管理器详解 1. **LinearLayout**:线性布局是最简单的布局类型之一,它将内部的子视图沿水平方向或垂直方向排列。通过设置`android:orientation`属性来控制子视图的排列方向。 - 示例代码: ```xml <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="TextView"/> </LinearLayout> ``` 2. **RelativeLayout**:相对布局允许根据其他视图的位置来定位当前视图。例如,可以将一个视图置于另一个视图的上方或右侧。 - 示例代码: ```xml <RelativeLayout android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 1"/> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_toRightOf="@id/button1" android:text="Button 2"/> </RelativeLayout> ``` 3. **ConstraintLayout**:这是一种更先进的布局方式,它支持复杂的布局约束,可以替代RelativeLayout,并且提供了更多的灵活性和优化性能的能力。 - 示例代码: ```xml <androidx.constraintlayout.widget.ConstraintLayout android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 1" app:layout_constraintBottom_toTopOf="@+id/button2" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent"/> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 2" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent"/> </androidx.constraintlayout.widget.ConstraintLayout> ``` #### 四、自定义UI控件 除了系统提供的标准控件外,开发者还可以根据需求自定义UI控件,以满足特定的功能需求。自定义控件通常涉及到以下几个步骤: 1. **继承自View或其子类**:创建一个新的类并继承自View或某个已有的控件类。 2. **重写绘图方法**:重写`onDraw()`方法来绘制控件的图形。 3. **处理触摸事件**:重写`onTouchEvent()`方法来响应用户的触摸操作。 4. **添加属性**:可以通过XML属性文件为自定义控件添加可配置的属性。 #### 五、样式和主题 样式和主题是Android UI设计中非常重要的概念,它们可以帮助开发者快速统一应用的整体风格。 1. **样式**:定义了UI元素的外观特性,如颜色、字体大小等。可以在XML文件中定义样式,并在需要的地方引用。 - 示例代码: ```xml <!-- styles.xml --> <resources> <style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> <!-- Customize your theme here. --> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> </style> </resources> ``` 2. **主题**:定义了一组相关样式的集合,用于设置整个应用的默认样式。可以通过在AndroidManifest.xml中指定主题来全局应用。 - 示例代码: ```xml <!-- AndroidManifest.xml --> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <!-- 应用主题 --> ... </application> ``` #### 六、动画效果 动画效果是增强用户交互体验的有效手段之一,通过合理地运用动画可以让应用更加生动有趣。 1. **补间动画**:补间动画是通过改变对象的状态来创建动画效果,包括淡入淡出、缩放、旋转和平移等。 - 示例代码: ```xml <set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/accelerate_interpolator"> <alpha android:duration="1000" android:fromAlpha="0.0" android:toAlpha="1.0"/> <scale android:duration="1000" android:fromXScale="1.0" android:toXScale="1.5" android:fromYScale="1.0" android:toYScale="1.5" android:pivotX="50%" android:pivotY="50%"/> </set> ``` 2. **属性动画**:属性动画是一种更灵活的动画形式,它可以改变任何对象的属性值。 - 示例代码: ```java ObjectAnimator animator = ObjectAnimator.ofFloat(button, "rotation", 0f, 360f); animator.setDuration(1000); animator.start(); ``` #### 七、响应式布局 随着不同尺寸和分辨率的设备越来越多,为了确保应用在各种设备上都能提供良好的用户体验,响应式布局成为了必要的技术手段。 1. **资源目录划分**:Android支持根据不同屏幕尺寸和密度来划分资源目录,例如,`layout-sw600dp`用于平板电脑等大屏幕设备。 - 示例代码: ```xml <!-- layout/main_activity.xml (手机) --> <LinearLayout ...> <TextView .../> </LinearLayout> <!-- layout-sw600dp/main_activity.xml (平板) --> <LinearLayout ...> <TextView .../> <Button .../> </LinearLayout> ``` 2. **动态布局调整**:通过程序代码来动态调整布局,以适应不同的屏幕尺寸。 - 示例代码: ```java if (getResources().getBoolean(R.bool.isTablet)) { // 平板设备特有的布局逻辑 } else { // 手机设备特有的布局逻辑 } ``` 通过以上介绍,我们对Android UI开发有了一个较为全面的认识。从基本的概念到具体的实现方法,这些知识点对于初学者来说是非常宝贵的资源。希望读者能够在实践中不断探索和完善自己的技能,成为一名优秀的Android界面开发者。
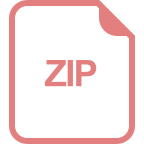
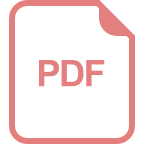
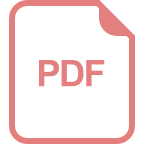
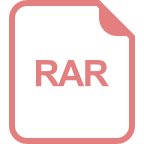
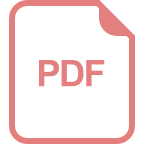
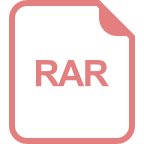
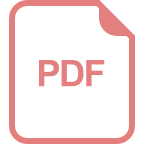
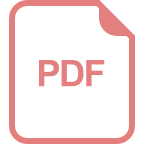
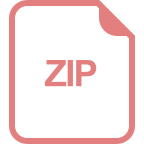
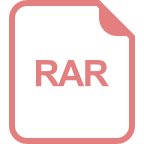
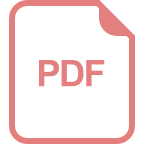
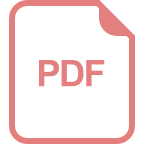
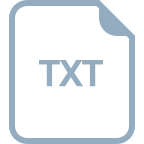
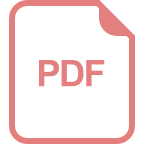
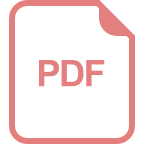
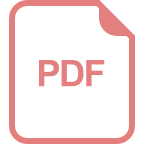
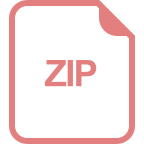
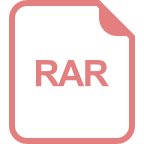
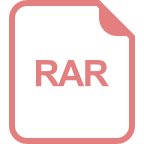
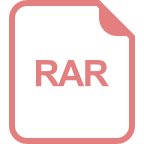
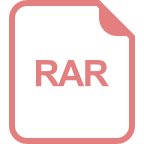
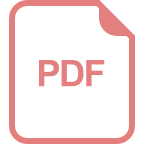
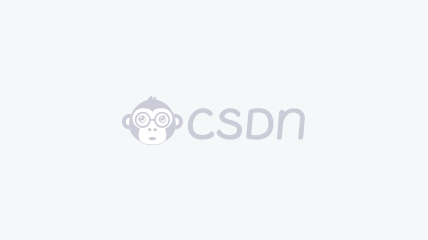

- 粉丝: 11
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

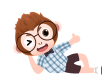
最新资源

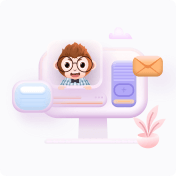
