## Summary of Changes in version 4.5 ##
### Categories ###
Each test method and test class can be annotated as belonging to a _category_:
public static class SomeUITests {
@Category(UserAvailable.class)
@Test
public void askUserToPressAKey() { }
@Test
public void simulatePressingKey() { }
}
@Category(InternetConnected.class)
public static class InternetTests {
@Test
public void pingServer() { }
}
To run all of the tests in a particular category, you must currently explicitly create a custom request:
new JUnitCore().run(Request.aClass(SomeUITests.class).inCategories(UserAvailable.class));
This feature will very likely be improved before the final release of JUnit 4.5
### Theories ###
- `@Before` and `@After` methods are run before and after each set of attempted parameters
on a Theory, and each set of parameters is run on a new instance of the test class.
- Exposed API's `ParameterSignature.getType()` and `ParameterSignature.getAnnotations()`
- An array of data points can be introduced by a field or method marked with the new annotation `@DataPoints`
- The Theories custom runner has been refactored to make it easier to extend
### JUnit 4 Runner API ###
- There has been a drastic rewrite of the API for custom Runners in 4.5. This
needs to be written up separately before release.
- Tests with failed assumptions are now marked as Ignored, rather than silently passing.
This may change behavior in some client tests, and also will require some new support
on the part of IDE's.
## Summary of Changes in version 4.4 ##
JUnit is designed to efficiently capture developers' intentions about
their code, and quickly check their code matches those intentions.
Over the last year, we've been talking about what things developers
would like to say about their code that have been difficult in the
past, and how we can make them easier.
[Download][]
[Download]: http://sourceforge.net/project/showfiles.php?group_id=15278
### assertThat ###
Two years ago, Joe Walnes built a [new assertion mechanism][walnes] on top of what was
then [JMock 1][]. The method name was `assertThat`, and the syntax looked like this:
[walnes]: http://joe.truemesh.com/blog/000511.html
[JMock 1]: http://www.jmock.org/download.html
assertThat(x, is(3));
assertThat(x, is(not(4)));
assertThat(responseString, either(containsString("color")).or(containsString("colour")));
assertThat(myList, hasItem("3"));
More generally:
assertThat([value], [matcher statement]);
Advantages of this assertion syntax include:
- More readable and typeable: this syntax allows you to think in terms of subject, verb, object
(assert "x is 3") rather than `assertEquals`, which uses verb, object, subject (assert "equals 3 x")
- Combinations: any matcher statement `s` can be negated (`not(s)`), combined (`either(s).or(t)`),
mapped to a collection (`each(s)`), or used in custom combinations (`afterFiveSeconds(s)`)
- Readable failure messages. Compare
assertTrue(responseString.contains("color") || responseString.contains("colour"));
// ==> failure message:
// java.lang.AssertionError:
assertThat(responseString, anyOf(containsString("color"), containsString("colour")));
// ==> failure message:
// java.lang.AssertionError:
// Expected: (a string containing "color" or a string containing "colour")
// got: "Please choose a font"
- Custom Matchers. By implementing the `Matcher` interface yourself, you can get all of the
above benefits for your own custom assertions.
- For a more thorough description of these points, see [Joe Walnes's
original post][walnes].
We have decided to include this API directly in JUnit.
It's an extensible and readable syntax, and it enables
new features, like [assumptions][] and [theories][].
[assumptions]: #assumptions
[theories]: #theories
Some notes:
- The old assert methods are never, ever, going away. Developers may
continue using the old `assertEquals`, `assertTrue`, and so on.
- The second parameter of an `assertThat` statement is a `Matcher`.
We include the Matchers we want as static imports, like this:
import static org.hamcrest.CoreMatchers.is;
or:
import static org.hamcrest.CoreMatchers.*;
- Manually importing `Matcher` methods can be frustrating. [Eclipse 3.3][] includes the ability to
define
"Favorite" classes to import static methods from, which makes it easier
(Search for "Favorites" in the Preferences dialog).
We expect that support for static imports will improve in all Java IDEs in the future.
[Eclipse 3.3]: http://www.eclipse.org/downloads/
- To allow compatibility with a wide variety of possible matchers,
we have decided to include the classes from hamcrest-core,
from the [Hamcrest][] project. This is the first time that
third-party classes have been included in JUnit.
[Hamcrest]: http://code.google.com/p/hamcrest/
- JUnit currently ships with a few matchers, defined in
`org.hamcrest.CoreMatchers` and `org.junit.matchers.JUnitMatchers`.
To use many, many more, consider downloading the [full hamcrest package][].
[full hamcrest package]: http://hamcrest.googlecode.com/files/hamcrest-all-1.1.jar
- JUnit contains special support for comparing string and array
values, giving specific information on how they differ. This is not
yet available using the `assertThat` syntax, but we hope to bring
the two assert methods into closer alignment in future releases.
<a name="assumptions" />
### Assumptions ###
Ideally, the developer writing a test has control of all of the forces that might cause a test to fail.
If this isn't immediately possible, making dependencies explicit can often improve a design.
For example, if a test fails when run in a different locale than the developer intended,
it can be fixed by explicitly passing a locale to the domain code.
However, sometimes this is not desirable or possible.
It's good to be able to run a test against the code as it is currently written,
implicit assumptions and all, or to write a test that exposes a known bug.
For these situations, JUnit now includes the ability to express "assumptions":
import static org.junit.Assume.*
@Test public void filenameIncludesUsername() {
assumeThat(File.separatorChar, is('/'));
assertThat(new User("optimus").configFileName(), is("configfiles/optimus.cfg"));
}
@Test public void correctBehaviorWhenFilenameIsNull() {
assumeTrue(bugFixed("13356")); // bugFixed is not included in JUnit
assertThat(parse(null), is(new NullDocument()));
}
With this release, a failed assumption will lead to the test being marked as passing,
regardless of what the code below the assumption may assert.
In the future, this may change, and a failed assumption may lead to the test being ignored:
however, third-party runners do not currently allow this option.
We have included `assumeTrue` for convenience, but thanks to the
inclusion of Hamcrest, we do not need to create `assumeEquals`,
`assumeSame`, and other analogues to the `assert*` methods. All of
those functionalities are subsumed in `assumeThat`, with the appropriate
matcher.
A failing assumption in a `@Before` or `@BeforeClass` method will have the same effect
as a failing assumption in each `@Test` method of the class.
<a name="theories" />
### Theories ###
More flexible and expressive assertions, combined with the ability to
state assumptions clearly, lead to a new kind of statement of intent,
which we call a "Theory". A test captures the intended behavior in
one particular scenario. A theory captures some aspect of the
intended behavior in possibly
infinite numbers of potential scenarios. For example:
@RunWith(Theories.class)
public class UserTest {
@DataPoint public static String GOOD_USERNAME = "optimus
没有合适的资源?快使用搜索试试~ 我知道了~
单元测试工具 junit4.6
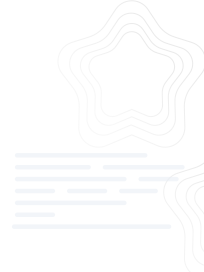
共697个文件
class:431个
java:152个
html:84个


温馨提示
java下的一个很好用的单元测试工具。学习一下吧,有问题就问我吧,qq:87356667
资源推荐
资源详情
资源评论
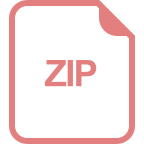
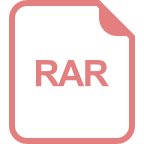
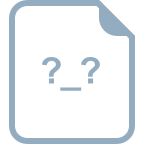
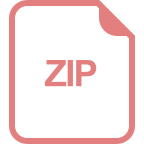
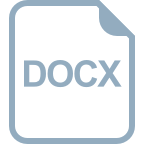
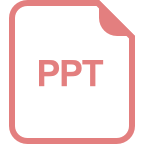
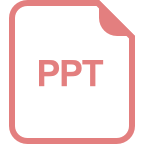
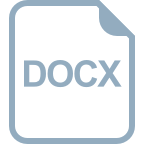
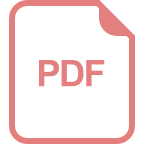
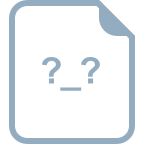
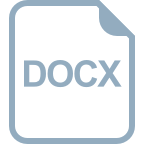
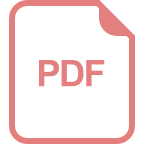
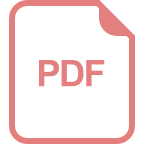
收起资源包目录

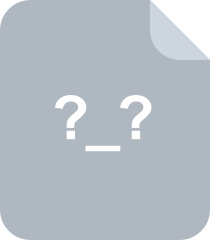
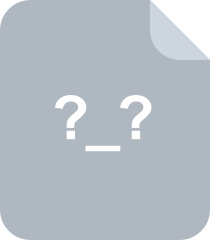
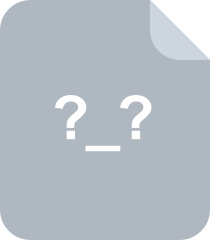
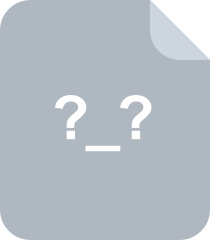
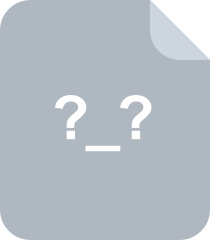
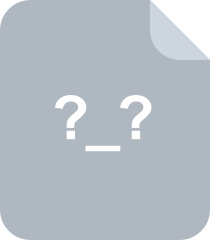
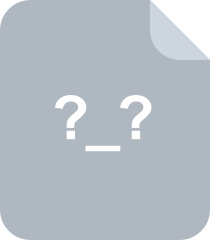
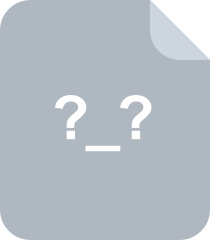
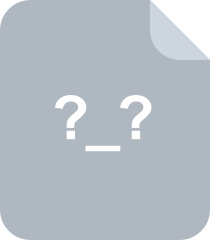
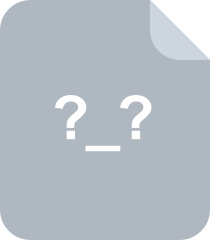
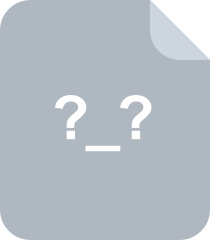
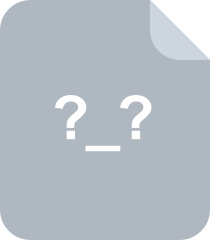
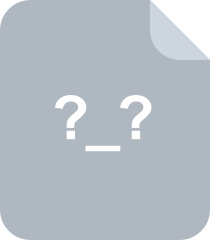
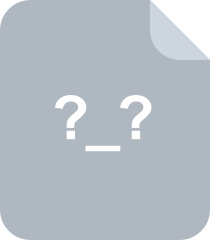
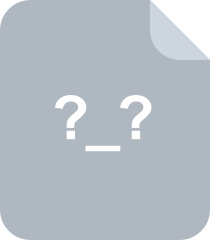
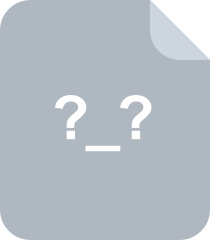
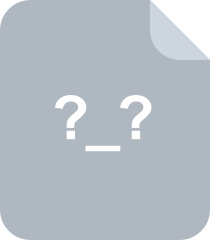
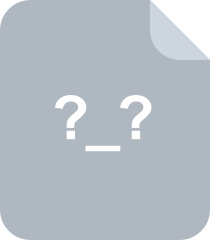
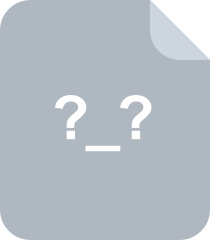
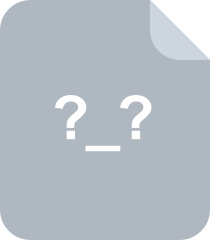
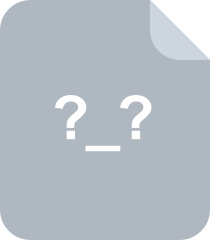
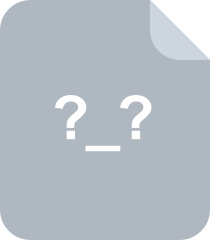
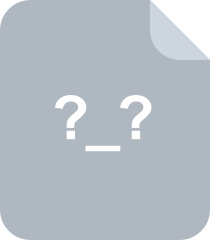
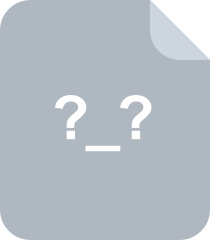
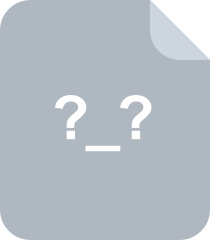
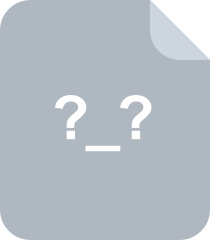
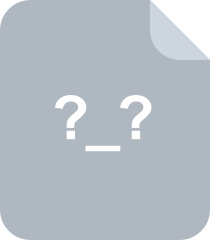
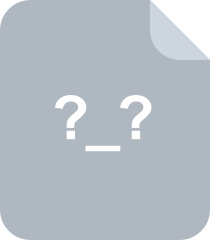
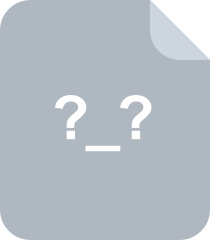
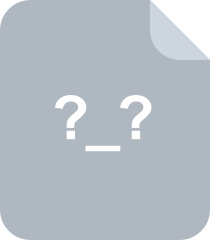
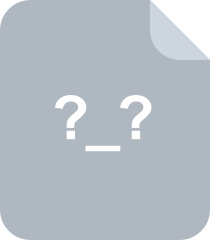
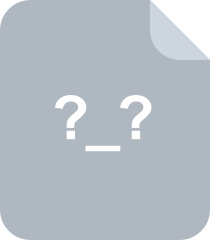
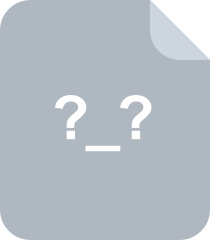
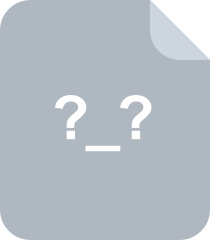
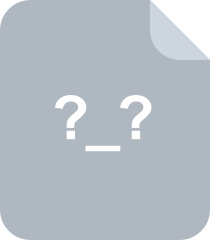
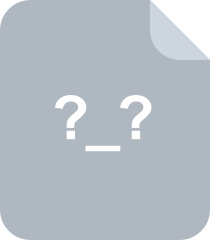
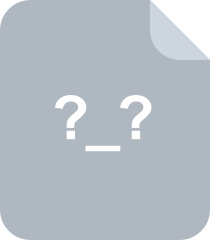
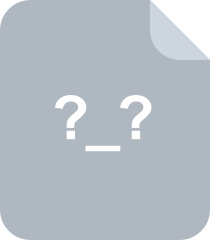
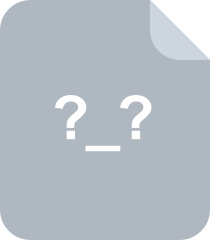
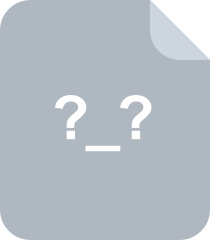
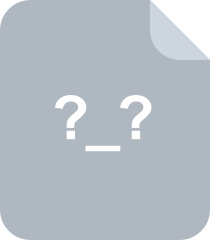
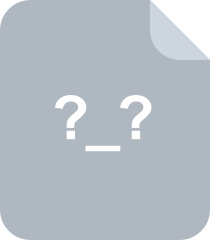
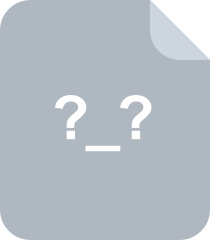
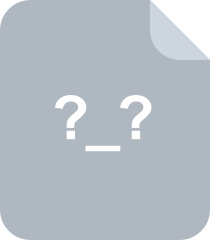
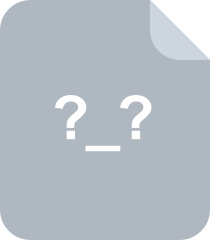
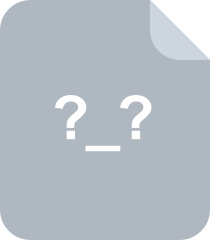
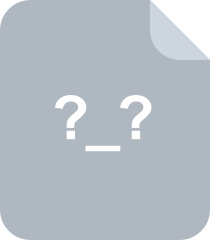
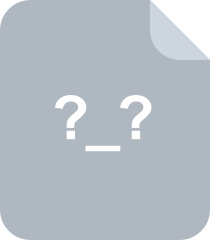
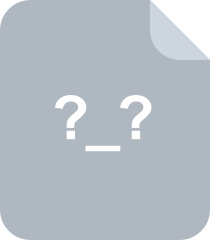
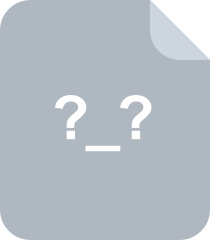
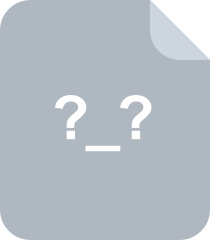
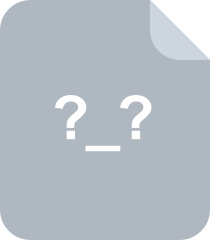
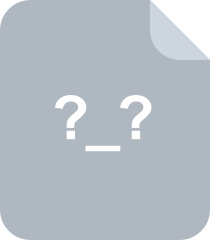
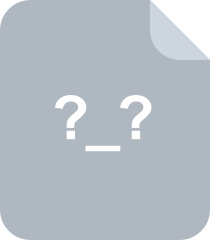
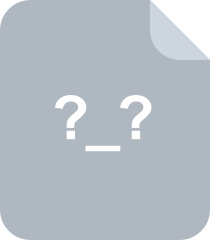
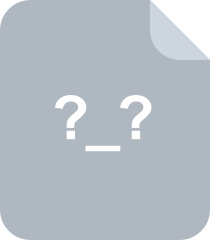
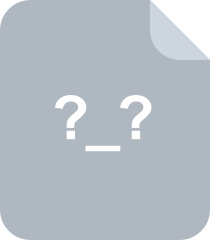
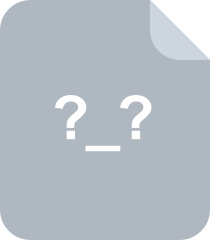
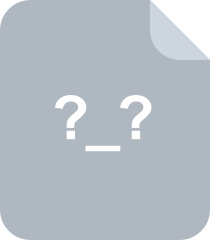
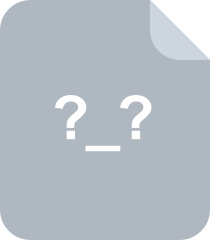
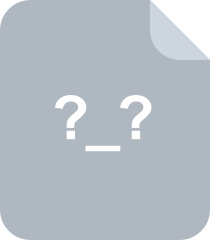
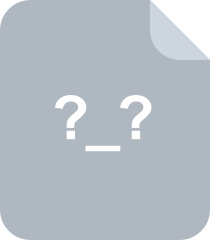
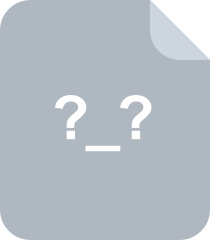
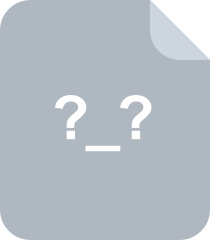
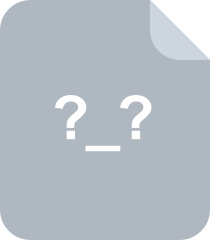
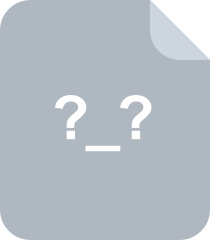
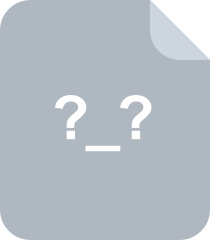
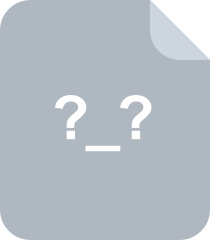
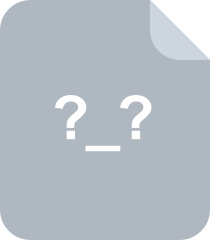
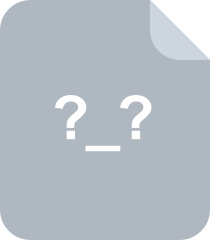
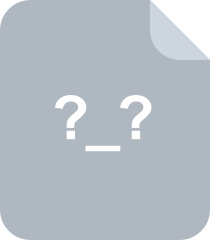
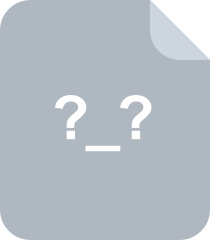
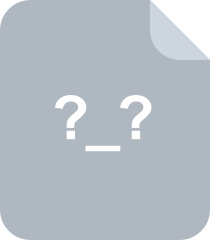
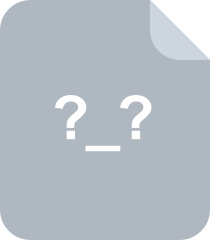
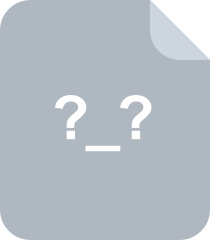
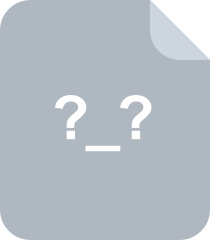
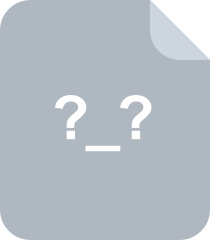
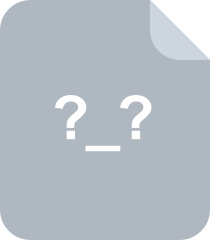
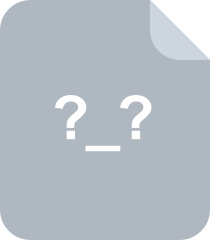
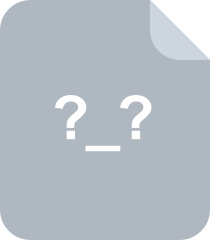
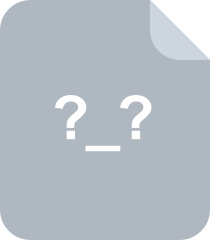
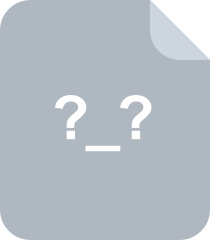
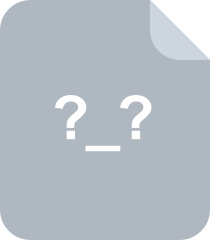
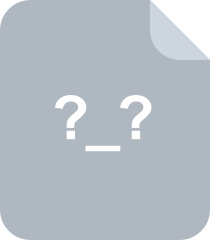
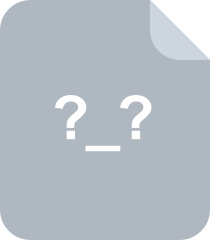
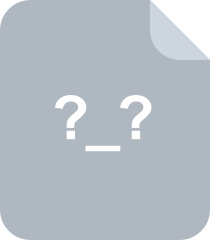
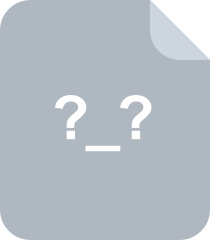
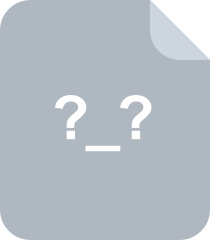
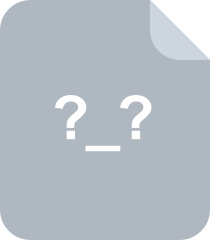
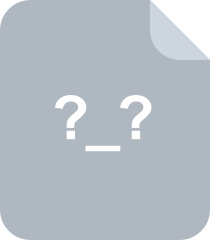
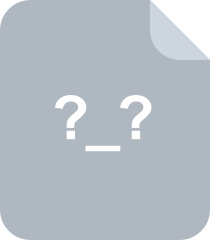
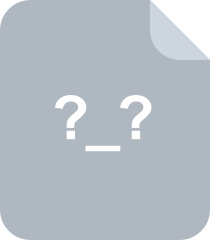
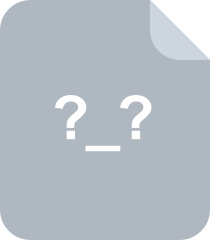
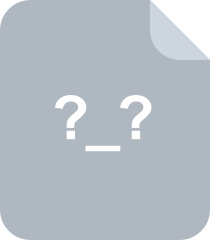
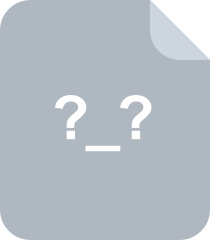
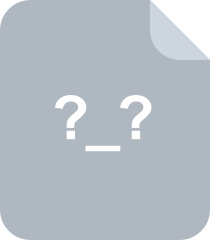
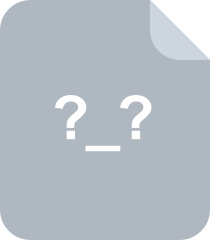
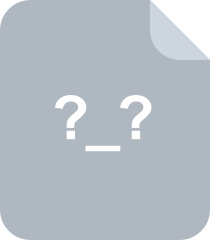
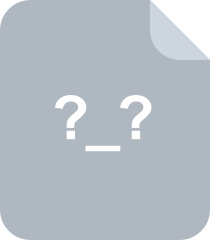
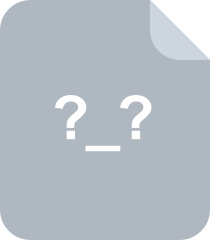
共 697 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
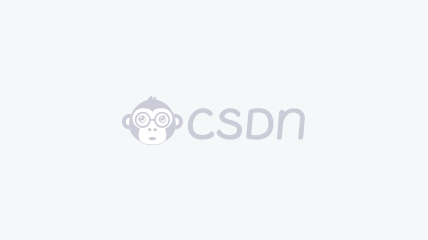
- sunhe9692012-11-21使用比较方便,很好,多谢楼主分享
- 亚里斯2012-10-07使用比较方便,很好,多谢楼主分享

wgs7909
- 粉丝: 1
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

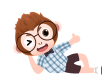
最新资源
- DirectX,C++ 上的 ThreeKings2.zip
- Android实现桌面小部件:今天吃什么
- DirectX-Headers 的一个分支,与 Zig 构建系统一起打包,并兼容交叉编译.zip
- 基于 Java+Mysql 实现的图书馆管理系统课程设计(源码+答辩 ppt+项目流程图)
- lca_EasyConnectPhone.apk-1-1732680277502.apk
- DirectX.jl 是 Windows 版本上 DirectX , Direct3D 的 Julia 接口 .zip
- DirectX11 2D 和 DirectX11 2D 解决方案.zip
- 基于C# 实现的扫雷游戏【课程设计 】
- DirectX11 上的简单 3D 引擎.zip
- DirectX11 与 discord 挂钩.zip
- DirectX11 和 DirectX11 的区别.zip
- 基于 C++ 实现的GNSS信号BPSK、BOC调制性能仿真分析课程设计
- DirectX11 坦克大战.zip
- DirectX11 的示例程序.zip
- DirectX11-12 教程 中文翻译.zip
- __UNI__1502013__20241126182436.apk.1.1
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


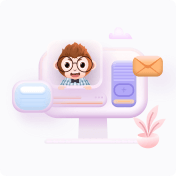
安全验证
文档复制为VIP权益,开通VIP直接复制
