'''
此代码需要用到mysql数据库 在其他电脑可能无法运行
'''
import pymysql
from tkinter import ttk
import tkinter as tk
import tkinter.font as tkFont
from tkinter import * # 图形界面库
import tkinter.messagebox as messagebox # 弹窗
class StartPage:
def __init__(self, parent_window):
parent_window.destroy() # 销毁子界面
self.window = tk.Tk() # 初始框的声明
self.window.title('学生信息管理系统')
self.window.geometry('300x470')
label = Label(self.window, text="学生信息管理系统", font=("Verdana", 20))
label.pack(pady=100) # pady=100 界面的长度
Button(self.window, text="管理员登陆", font=tkFont.Font(size=16), command=lambda: AdminPage(self.window), width=30,
height=2,
fg='white', bg='gray', activebackground='black', activeforeground='white').pack()
Button(self.window, text="学生登陆", font=tkFont.Font(size=16), command=lambda: StudentPage(self.window), width=30,
height=2, fg='white', bg='gray', activebackground='black', activeforeground='white').pack()
Button(self.window, text="账号系统", font=tkFont.Font(size=16), command=lambda: AboutPage(self.window),
width=30,
height=2,
fg='white', bg='gray', activebackground='black', activeforeground='white').pack()
Button(self.window, text='退出系统', height=2, font=tkFont.Font(size=16), width=30, command=self.window.destroy,
fg='white', bg='gray', activebackground='black', activeforeground='white').pack()
self.window.mainloop() # 主消息循环
# 管理员登陆页面
class AdminPage:
def __init__(self, parent_window):
parent_window.destroy() # 销毁主界面
self.window = tk.Tk() # 初始框的声明
self.window.title('管理员登陆页面')
self.window.geometry('300x450')
label = tk.Label(self.window, text='管理员登陆', bg='green', font=('Verdana', 20), width=30, height=2)
label.pack()
Label(self.window, text='管理员账号:', font=tkFont.Font(size=14)).pack(pady=25)
self.admin_username = tk.Entry(self.window, width=30, font=tkFont.Font(size=14), bg='Ivory')
self.admin_username.pack()
Label(self.window, text='管理员密码:', font=tkFont.Font(size=14)).pack(pady=25)
self.admin_pass = tk.Entry(self.window, width=30, font=tkFont.Font(size=14), bg='Ivory', show='*')
self.admin_pass.pack()
Button(self.window, text="登陆", width=8, font=tkFont.Font(size=12), command=self.login).pack(pady=40)
Button(self.window, text="返回首页", width=8, font=tkFont.Font(size=12), command=self.back).pack()
self.window.protocol("WM_DELETE_WINDOW", self.back) # 捕捉右上角关闭点击
self.window.mainloop() # 进入消息循环
def login(self):
print(str(self.admin_username.get()))
print(str(self.admin_pass.get()))
admin_pass = None
# 数据库操作 查询管理员表
db = pymysql.connect(host='localhost', port=3306, db='student', user='root', password='chen218510') # 打开数据库连接
cursor = db.cursor() # 使用cursor()方法获取操作游标
sql = "SELECT * FROM admin_login_k WHERE admin_id = '%s'" % (self.admin_username.get()) # SQL 查询语句
try:
# 执行SQL语句
cursor.execute(sql)
# 获取所有记录列表
results = cursor.fetchall()
for row in results:
admin_id = row[0]
admin_pass = row[1]
# 打印结果
print("admin_id=%s,admin_pass=%s" % (admin_id, admin_pass))
except:
print("Error: unable to fecth data")
messagebox.showinfo('警告!', '用户名或密码不正确!')
db.close() # 关闭数据库连接
print("正在登陆管理员管理界面")
print("self", self.admin_pass)
print("local", admin_pass)
if self.admin_pass.get() == admin_pass:
AdminManage(self.window) # 进入管理员操作界面
else:
messagebox.showinfo('警告!', '用户名或密码不正确!')
def back(self):
StartPage(self.window) # 显示主窗口 销毁本窗口
# 学生登陆页面
class StudentPage:
def __init__(self, parent_window):
parent_window.destroy() # 销毁主界面
self.window = tk.Tk() # 初始框的声明
self.window.title('学生登陆')
self.window.geometry('300x450')
label = tk.Label(self.window, text='学生登陆', bg='pink', font=('Verdana', 20), width=30, height=2)
label.pack()
Label(self.window, text='学生账号:', font=tkFont.Font(size=14)).pack(pady=25)
self.student_id = tk.Entry(self.window, width=30, font=tkFont.Font(size=14), bg='Ivory')
self.student_id.pack()
Label(self.window, text='学生密码:', font=tkFont.Font(size=14)).pack(pady=25)
self.student_pass = tk.Entry(self.window, width=30, font=tkFont.Font(size=14), bg='Ivory', show='*')
self.student_pass.pack()
Button(self.window, text="登陆", width=8, font=tkFont.Font(size=12), command=self.login).pack(pady=40)
Button(self.window, text="返回首页", width=8, font=tkFont.Font(size=12), command=self.back).pack()
self.window.protocol("WM_DELETE_WINDOW", self.back) # 捕捉右上角关闭点击
self.window.mainloop() # 进入消息循环
def login(self):
print(str(self.student_id.get()))
print(str(self.student_pass.get()))
stu_pass = None
# 数据库操作 查询管理员表
db = pymysql.connect(host='localhost', port=3306, db='student', user='root', password='chen218510') # 打开数据库连接
cursor = db.cursor() # 使用cursor()方法获取操作游标
sql = "SELECT * FROM stu_login_k WHERE stu_id = '%s'" % (self.student_id.get()) # SQL 查询语句
try:
# 执行SQL语句
cursor.execute(sql)
# 获取所有记录列表
results = cursor.fetchall()
for row in results:
stu_id = row[0]
stu_pass = row[1]
# 打印结果
print("stu_id=%s,stu_pass=%s" % (stu_id, stu_pass))
except:
print("Error: unable to fecth data")
messagebox.showinfo('警告!', '用户名或密码不正确!')
db.close() # 关闭数据库连接
print("正在登陆学生信息查看界面")
print("self", self.student_pass.get())
print("local", stu_pass)
if self.student_pass.get() == stu_pass:
StudentView(self.window, self.student_id.get()) # 进入学生信息查看界面
else:
messagebox.showinfo('警告!', '用户名或密码不正确!')
def back(self):
StartPage(self.window) # 显示主窗口 销毁本窗口
# 管理员操作界面
class AdminManage:
def __init__(self, parent_window):
parent_window.destroy() # 销毁主界面
self.window = Tk() # 初始框的声明
self.window.title('管理员操作界面')
self.frame_left_top = tk.Frame(width=300, height=200)
self.frame_right_top = tk.Frame(width=200, height=200)
self.frame_center = tk.Frame(width=500, height=400)
self.frame_bottom = tk.Frame(width=650, height=50)
# 定义下方中心列表区域
self.columns = ("学号", "姓名", "性别", "年龄")
self.tree = ttk.Treeview(self.frame_center, show="headings", height=18, columns=self.columns)

与临溪
- 粉丝: 207
- 资源: 15
最新资源
- (源码)基于SimPy和贝叶斯优化的流程仿真系统.zip
- (源码)基于Java Web的个人信息管理系统.zip
- (源码)基于C++和OTL4的PostgreSQL数据库连接系统.zip
- (源码)基于ESP32和AWS IoT Core的室内温湿度监测系统.zip
- (源码)基于Arduino的I2C协议交通灯模拟系统.zip
- coco.names 文件
- (源码)基于Spring Boot和Vue的房屋租赁管理系统.zip
- (源码)基于Android的饭店点菜系统.zip
- (源码)基于Android平台的权限管理系统.zip
- (源码)基于CC++和wxWidgets框架的LEGO模型火车控制系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


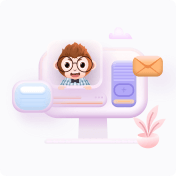
- 1
- 2
前往页