#ifndef Py_INTERNAL_RUNTIME_INIT_H
#define Py_INTERNAL_RUNTIME_INIT_H
#ifdef __cplusplus
extern "C" {
#endif
#ifndef Py_BUILD_CORE
# error "this header requires Py_BUILD_CORE define"
#endif
#include "pycore_object.h"
/* The static initializers defined here should only be used
in the runtime init code (in pystate.c and pylifecycle.c). */
#define _PyRuntimeState_INIT \
{ \
.gilstate = { \
.check_enabled = 1, \
/* A TSS key must be initialized with Py_tss_NEEDS_INIT \
in accordance with the specification. */ \
.autoTSSkey = Py_tss_NEEDS_INIT, \
}, \
.interpreters = { \
/* This prevents interpreters from getting created \
until _PyInterpreterState_Enable() is called. */ \
.next_id = -1, \
}, \
.global_objects = _Py_global_objects_INIT, \
._main_interpreter = _PyInterpreterState_INIT, \
}
#ifdef HAVE_DLOPEN
# include <dlfcn.h>
# if HAVE_DECL_RTLD_NOW
# define _Py_DLOPEN_FLAGS RTLD_NOW
# else
# define _Py_DLOPEN_FLAGS RTLD_LAZY
# endif
# define DLOPENFLAGS_INIT .dlopenflags = _Py_DLOPEN_FLAGS,
#else
# define _Py_DLOPEN_FLAGS 0
# define DLOPENFLAGS_INIT
#endif
#define _PyInterpreterState_INIT \
{ \
._static = 1, \
.id_refcount = -1, \
DLOPENFLAGS_INIT \
.ceval = { \
.recursion_limit = Py_DEFAULT_RECURSION_LIMIT, \
}, \
.gc = { \
.enabled = 1, \
.generations = { \
/* .head is set in _PyGC_InitState(). */ \
{ .threshold = 700, }, \
{ .threshold = 10, }, \
{ .threshold = 10, }, \
}, \
}, \
._initial_thread = _PyThreadState_INIT, \
}
#define _PyThreadState_INIT \
{ \
._static = 1, \
.recursion_limit = Py_DEFAULT_RECURSION_LIMIT, \
.context_ver = 1, \
}
// global objects
#define _PyLong_DIGIT_INIT(val) \
{ \
_PyVarObject_IMMORTAL_INIT(&PyLong_Type, \
((val) == 0 ? 0 : ((val) > 0 ? 1 : -1))), \
.ob_digit = { ((val) >= 0 ? (val) : -(val)) }, \
}
#define _PyBytes_SIMPLE_INIT(CH, LEN) \
{ \
_PyVarObject_IMMORTAL_INIT(&PyBytes_Type, LEN), \
.ob_shash = -1, \
.ob_sval = { CH }, \
}
#define _PyBytes_CHAR_INIT(CH) \
{ \
_PyBytes_SIMPLE_INIT(CH, 1) \
}
#define _PyUnicode_ASCII_BASE_INIT(LITERAL, ASCII) \
{ \
.ob_base = _PyObject_IMMORTAL_INIT(&PyUnicode_Type), \
.length = sizeof(LITERAL) - 1, \
.hash = -1, \
.state = { \
.kind = 1, \
.compact = 1, \
.ascii = ASCII, \
.ready = 1, \
}, \
}
#define _PyASCIIObject_INIT(LITERAL) \
{ \
._ascii = _PyUnicode_ASCII_BASE_INIT(LITERAL, 1), \
._data = LITERAL \
}
#define INIT_STR(NAME, LITERAL) \
._ ## NAME = _PyASCIIObject_INIT(LITERAL)
#define INIT_ID(NAME) \
._ ## NAME = _PyASCIIObject_INIT(#NAME)
#define _PyUnicode_LATIN1_INIT(LITERAL) \
{ \
._latin1 = { \
._base = _PyUnicode_ASCII_BASE_INIT(LITERAL, 0), \
}, \
._data = LITERAL, \
}
/* The following is auto-generated by Tools/scripts/generate_global_objects.py. */
#define _Py_global_objects_INIT { \
.singletons = { \
.small_ints = { \
_PyLong_DIGIT_INIT(-5), \
_PyLong_DIGIT_INIT(-4), \
_PyLong_DIGIT_INIT(-3), \
_PyLong_DIGIT_INIT(-2), \
_PyLong_DIGIT_INIT(-1), \
_PyLong_DIGIT_INIT(0), \
_PyLong_DIGIT_INIT(1), \
_PyLong_DIGIT_INIT(2), \
_PyLong_DIGIT_INIT(3), \
_PyLong_DIGIT_INIT(4), \
_PyLong_DIGIT_INIT(5), \
_PyLong_DIGIT_INIT(6), \
_PyLong_DIGIT_INIT(7), \
_PyLong_DIGIT_INIT(8), \
_PyLong_DIGIT_INIT(9), \
_PyLong_DIGIT_INIT(10), \
_PyLong_DIGIT_INIT(11), \
_PyLong_DIGIT_INIT(12), \
_PyLong_DIGIT_INIT(13), \
_PyLong_DIGIT_INIT(14), \
_PyLong_DIGIT_INIT(15), \
_PyLong_DIGIT_INIT(16), \
_PyLong_DIGIT_INIT(17), \
_PyLong_DIGIT_INIT(18), \
_PyLong_DIGIT_INIT(19), \
_PyLong_DIGIT_INIT(20), \
_PyLong_DIGIT_INIT(21), \
_PyLong_DIGIT_INIT(22), \
_PyLong_DIGIT_INIT(23), \
_PyLong_DIGIT_INIT(24), \
_PyLong_DIGIT_INIT(25), \
_PyLong_DIGIT_INIT(26), \
_PyLong_DIGIT_INIT(27), \
_PyLong_DIGIT_INIT(28), \
_PyLong_DIGIT_INIT(29), \
_PyLong_DIGIT_INIT(30), \
_PyLong_DIGIT_INIT(31), \
_PyLong_DIGIT_INIT(32), \
_PyLong_DIGIT_INIT(33), \
_PyLong_DIGIT_INIT(34), \
_PyLong_DIGIT_INIT(35), \
_PyLong_DIGIT_INIT(36), \
_PyLong_DIGIT_INIT(37), \
_PyLong_DIGIT_INIT(38), \
_PyLong_DIGIT_INIT(39), \
_PyLong_DIGIT_INIT(40), \
_PyLong_DIGIT_INIT(41), \
_PyLong_DIGIT_INIT(42), \
_PyLong_DIGIT_INIT(43), \
_PyLong_DIGIT_INIT(44), \
_PyLong_DIGIT_INIT(45), \
_PyLong_DIGIT_INIT(46), \
_PyLong_DIGIT_INIT(47), \
_PyLong_DIGIT_INIT(48), \
_PyLong_DIGIT_INIT(49), \
_PyLong_DIGIT_INIT(50), \
_PyLong_DIGIT_INIT(51), \
_PyLong_DIGIT_INIT(52), \
_PyLong_DIGIT_INIT(53), \
_PyLong_DIGIT_INIT(54), \
_PyLong_DIGIT_INIT(55), \
_PyLong_DIGIT_INIT(56), \
_PyLong_DIGIT_INIT(57), \
_PyLong_DIGIT_INIT(58), \
_PyLong_DIGIT_INIT(59), \
_PyLong_DIGIT_INIT(60), \
_PyLong_DIGIT_INIT(61), \
_PyLong_DIGIT_INIT(62), \
_PyLong_DIGIT_INIT(63), \
_PyLong_DIGIT_INIT(64), \
_PyLong_DIGIT_INIT(65), \
_PyLong_DIGIT_INIT(66), \
_PyLong_DIGIT_INIT(67), \
_PyLong_DIGIT_INIT(68), \
_PyLong_DIGIT_INIT(69), \
_PyLong_DIGIT_INIT(70), \
_PyLong_DIGIT_INIT(71), \
_PyLong_DIGIT_INIT(72), \
_PyLong_DIGIT_INIT(73), \
_PyLong_DIGIT_INIT(74), \
_PyLong_DIGIT_INIT(75), \
_PyLong_DIGIT_INIT(76), \
_PyLong_DIGIT_INIT(77), \
_PyLong_DIGIT_INIT(78), \
_PyLong_DIGIT_INIT(79), \
_PyLong_DIGIT_INIT(80), \
_PyLong_DIGIT_INIT(81), \
_PyLong_DIGIT_INIT(82), \
_PyLong_DIGIT_INIT(83), \
_PyLong_DIGIT_INIT(84), \
_PyLong_DIGIT_INIT(85), \
_PyLong_DIGIT_INIT(86), \
_PyLong_DIGIT_INIT(87), \
_PyLong_DIGIT_INIT(88), \
_PyLong_DIGIT_INIT(89), \
_PyLong_DIGIT_INIT(90), \
_PyLong_DIGIT_INIT(91), \
_PyLong_DIGIT_INIT(92), \
_PyLong_DIGIT_INIT(93), \
_PyLong_DIGIT_INIT(94), \
_PyLong_DIGIT_INIT(95), \
_PyLong_DIGIT_INIT(96), \
_PyLong_DIGIT_INIT(97), \
_PyLong_DIGIT_INIT(98), \
_PyLong_DIGIT_INIT(99), \
_PyLong_DIGIT_INIT(100), \
_PyLong_DIGIT_INIT(101), \
_PyLong_DIGIT_INIT(102), \
_PyLong_DIGIT_INIT(103), \
_PyLong_DIGIT_INIT(104), \
_PyLong_DIGIT_INIT(105), \
_PyLong_DIGIT_INIT(106), \
_PyLong_DIGIT_INIT(107), \
_PyLong_DIGIT_INIT(108), \
_PyLong_DIGIT_INIT(109), \
_PyLong_DIGIT_INIT(110), \
_PyLong_DIGIT_INIT(111), \
_PyLong_DIGIT_INIT(112), \
_PyLong_DIGIT_INIT(113), \
_PyLong_DIGIT_INIT(114)
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
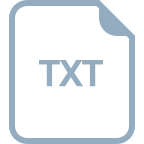
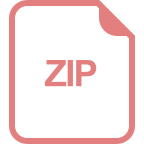
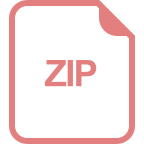
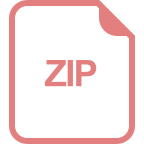
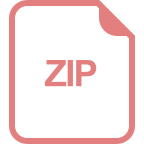
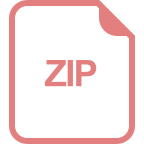
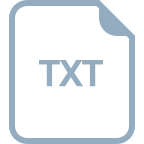
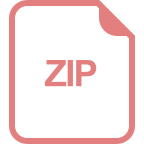
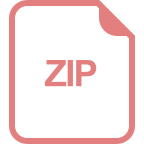
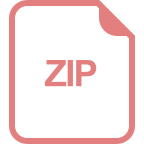
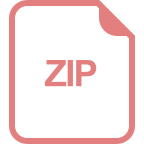
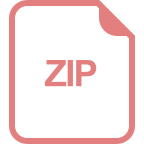
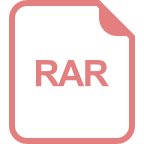
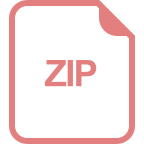
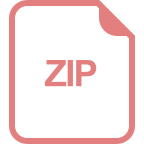
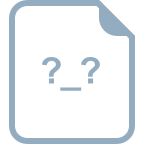
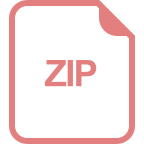
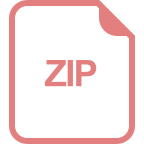
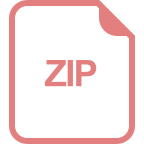
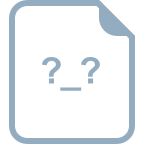
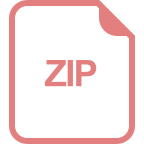
收起资源包目录

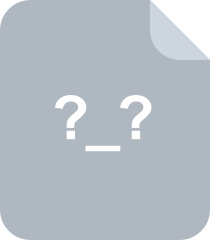
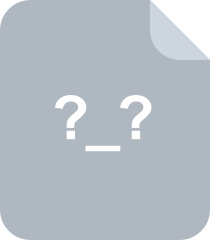
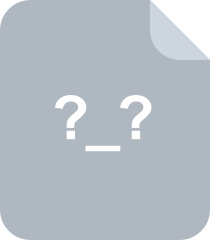
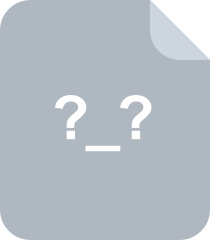
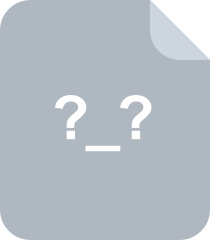
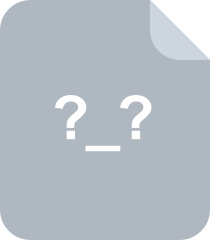
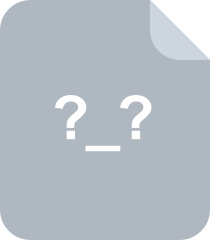
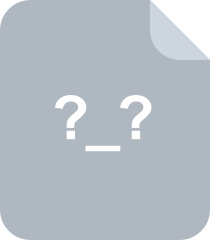
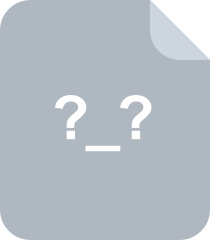
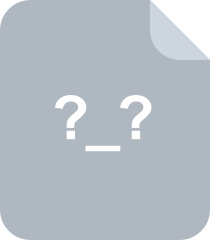
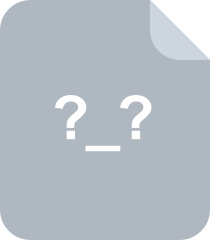
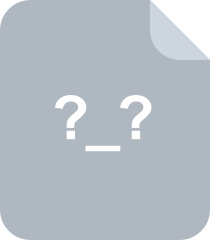
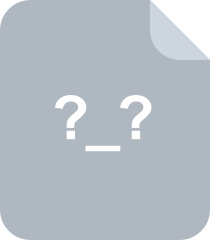
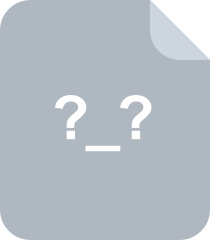
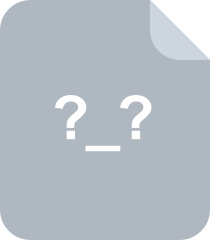
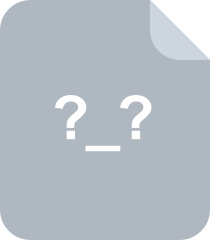
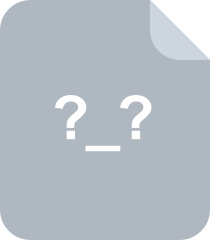
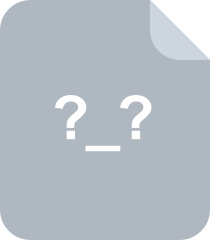
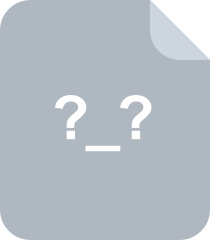
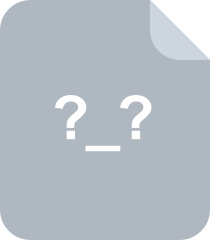
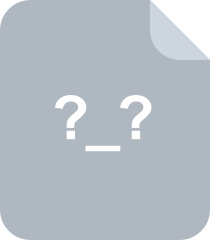
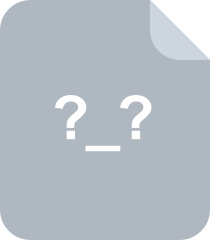
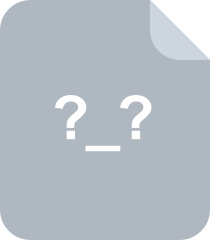
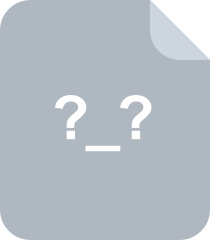
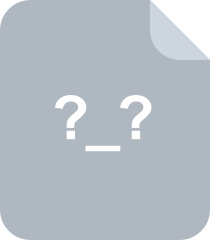
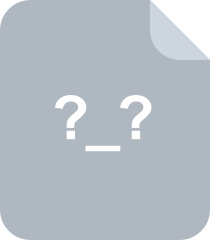
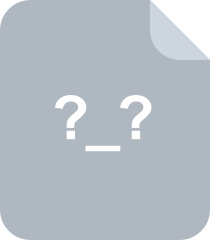
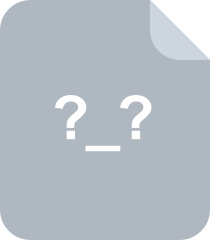
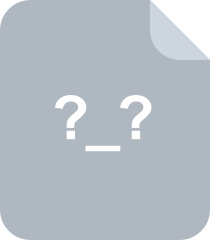
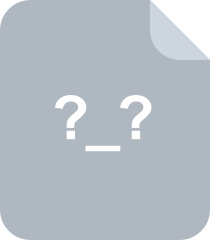
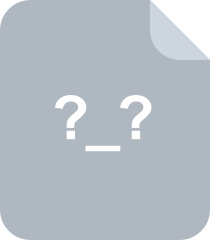
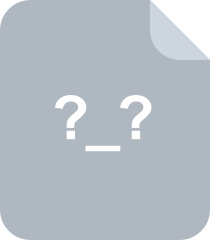
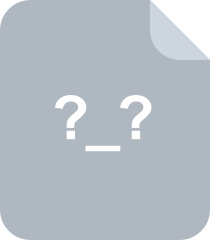
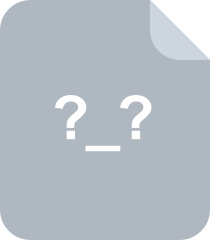
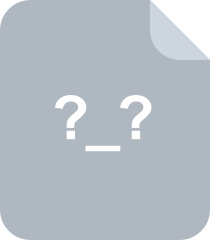
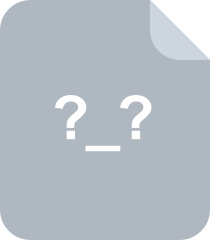
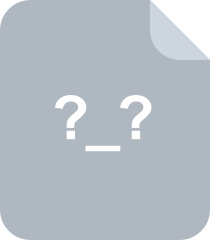
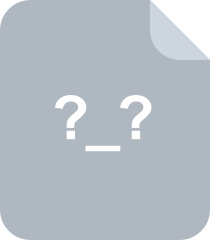
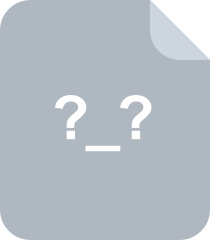
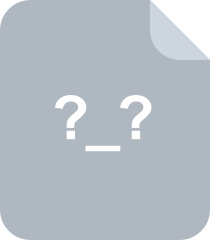
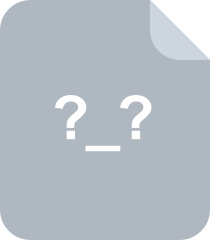
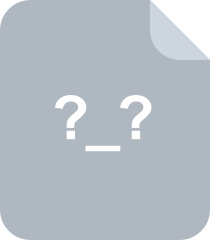
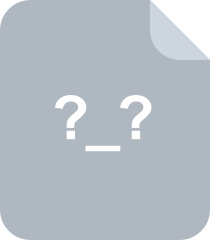
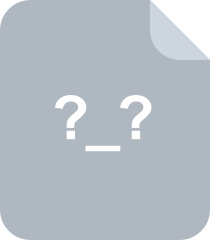
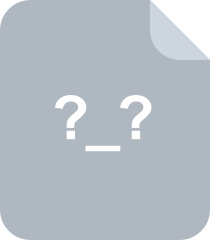
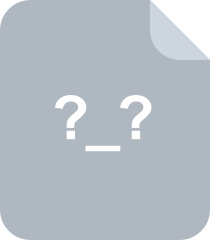
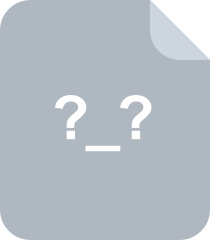
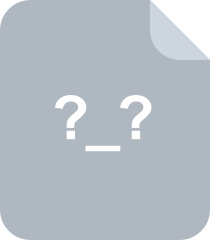
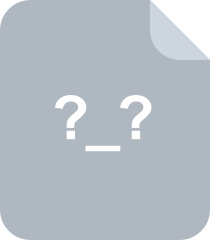
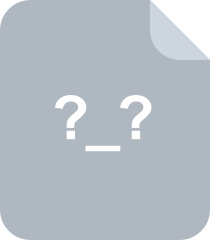
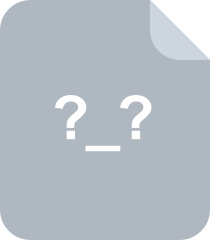
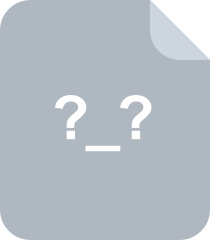
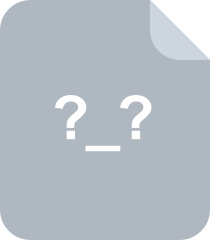
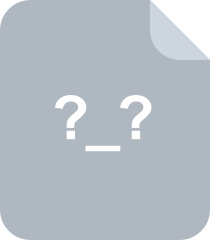
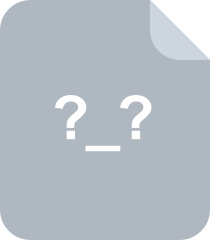
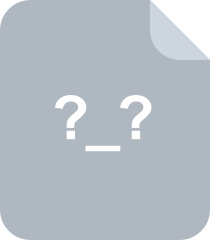
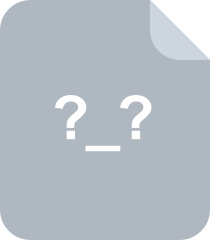
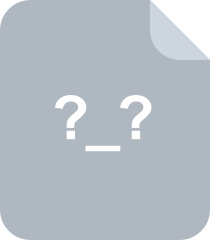
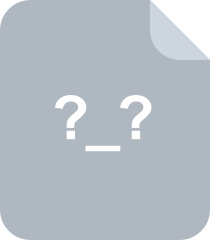
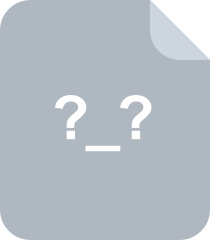
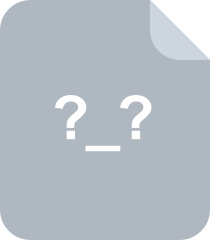
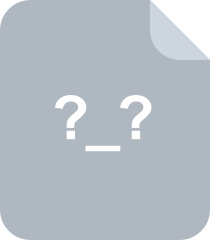
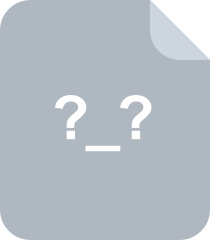
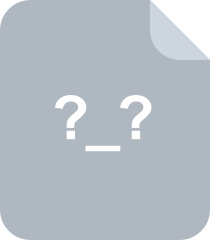
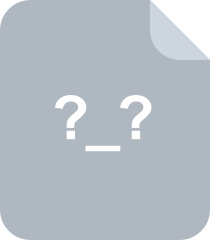
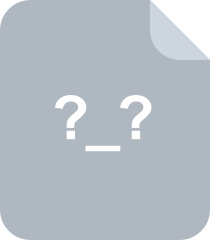
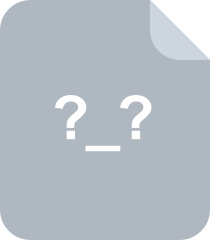
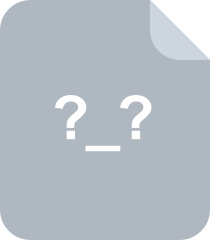
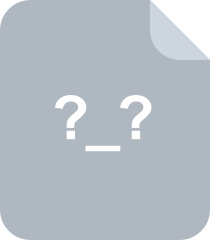
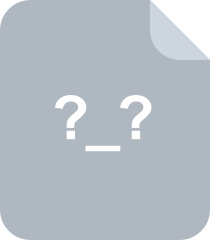
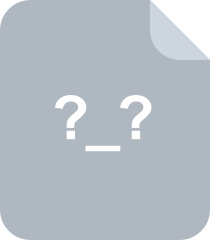
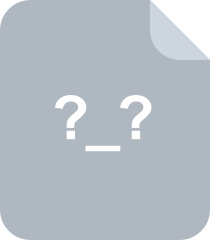
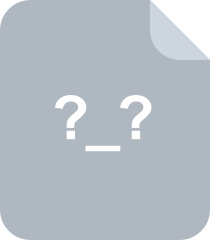
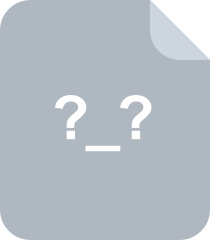
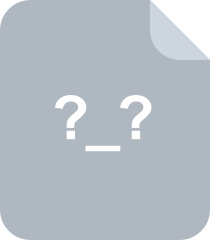
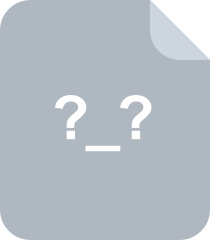
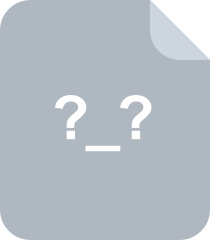
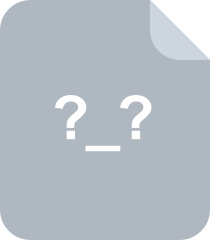
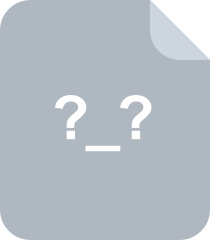
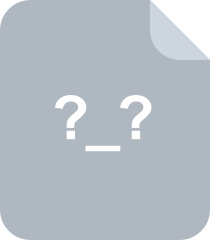
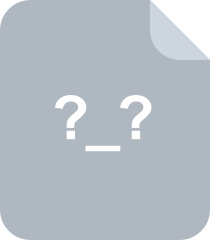
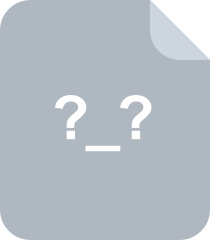
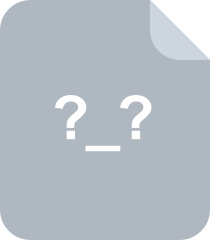
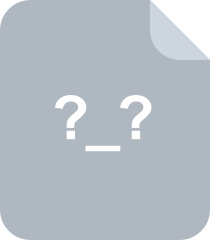
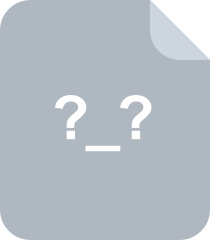
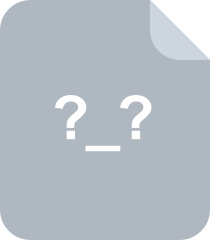
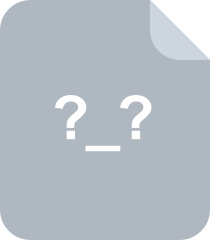
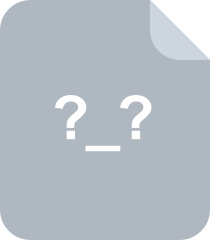
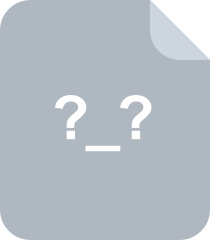
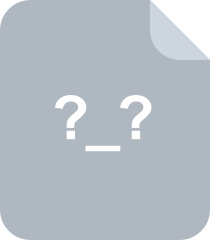
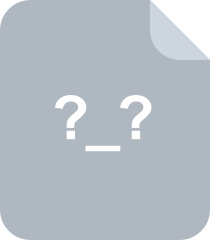
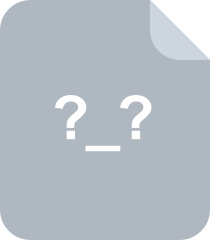
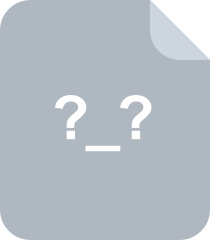
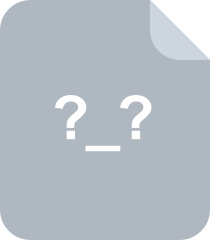
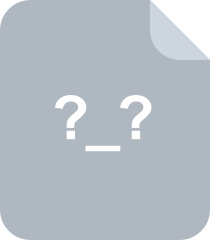
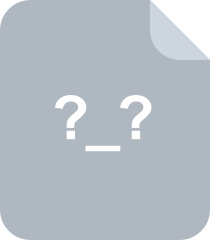
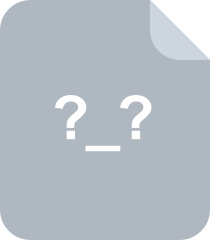
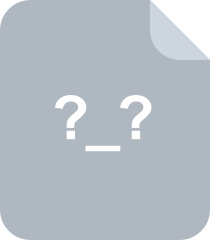
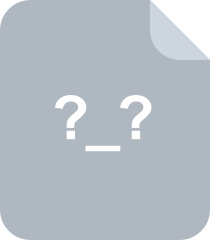
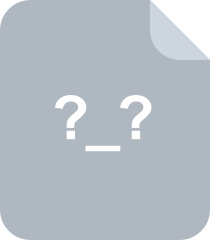
共 191 条
- 1
- 2
资源评论
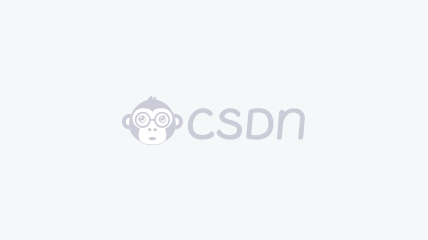

LR939
- 粉丝: 213
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

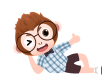
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


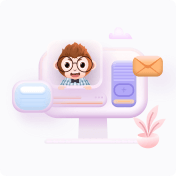
安全验证
文档复制为VIP权益,开通VIP直接复制
