function varargout = PCM(varargin)
% PCM M-file for PCM.fig
% PCM was designed in order to show how PCM works
%
% To simplify the undesrtanding of this method, the program first takes
% a sine wave. Then you can choose a sampling scheme, and you can see
% the output of the sampler. You can choose one out of three sampling
% methods.
% If you choose natural sampling; then you will have the chance to modify
% the sampling window, and see the effects of this change in the output of
% the sampler.
%
% Once you got the sampled signal you can quantize it by a method that is
% known as two rules and an alorithm.
% The option Squeezing and Stretching shows the best G(x) tha minimizes
% the MSE. You can better understand this using the book
% Telecommunications Demystified written by Carl Nassar. You can find
% information about this on Chapter four of that book.
% You can edit the bit's number and the number of iterations of the
% algorithm. The bigger the number of bits, the smaller the MSE.
% The picture shows the signal after quantization, the first iteration
% in the quantization process and the output of the quantizer
%
% Then, by pressing the Bit Stream button you will see the PCM output
% of the signal that you have selected in the input area.
%
% Everytime you change something, you must push the button that is
% related with the change you have just made. For example if don't
% want to work anymore with the sine wave and you choose the random
% signal, then you have to push the plot button in order to see the
% plot of the random signal, and if you change of sampling method you
% have to push the sampling button, when you changhe the sampling
% window. So if you change the number of codewords or the number of
% the iterations you will have to press the quantize button again.
% Edit the above text to modify the response to help PCM
% Last Modified by GUIDE v2.5 14-Mar-2007 12:32:35
% Begin initialization code - DO NOT EDIT
gui_Singleton = 1;
gui_State = struct('gui_Name', mfilename, ...
'gui_Singleton', gui_Singleton, ...
'gui_OpeningFcn', @PCM_OpeningFcn, ...
'gui_OutputFcn', @PCM_OutputFcn, ...
'gui_LayoutFcn', [] , ...
'gui_Callback', []);
if nargin && ischar(varargin{1})
gui_State.gui_Callback = str2func(varargin{1});
end
if nargout
[varargout{1:nargout}] = gui_mainfcn(gui_State, varargin{:});
else
gui_mainfcn(gui_State, varargin{:});
end
% End initialization code - DO NOT EDIT
% --- Executes just before PCM is made visible.
function PCM_OpeningFcn(hObject, eventdata, handles, varargin)
% This function has no output args, see OutputFcn.
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% varargin command line arguments to PCM (see VARARGIN)
% Choose default command line output for PCM
handles.output = hObject;
% Update handles structure
guidata(hObject, handles);
% UIWAIT makes PCM wait for user response (see UIRESUME)
% uiwait(handles.figure1);
% --- Outputs from this function are returned to the command line.
function varargout = PCM_OutputFcn(hObject, eventdata, handles)
% varargout cell array for returning output args (see VARARGOUT);
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Get default command line output from handles structure
varargout{1} = handles.output;
% --- Executes on button press in pushbutton2.
function pushbutton2_Callback(hObject, eventdata, handles)
% hObject handle to pushbutton2 (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
if (get(handles.radiobutton2,'Value') == get(handles.radiobutton2,'Max')) % Verifies if Sine wave was selected
t=linspace(0,1,60); % Creates the time variable from 0 to 1 with a length of 60 or 60 points
y=sin(2*pi*t); % Creates a sine wave of frequency 1 with the t vector
axes(handles.axesanalog) % Select the proper axes
plot(t,y);
xlabel('Time');
ylabel('Amplitude');
grid on;
elseif (get(handles.radiobutton3,'Value') == get(handles.radiobutton3,'Max')) % Verifies if Random signal was selected
t=linspace(0,60,60); % Creates the time variable from 0 to 60 with a length of 60 or 60 points
y=rand([1 60]); % Creates a random signal of length 60 or with 60 points
axes(handles.axesanalog) % Select the proper axes
plot(t,y);
xlabel('Time');
ylabel('Amplitude');
grid on;
end
handles.amp=y; % Saves the input signal y in the amp variable at the handles structure
handles.time=t; % Saves the input signal t in the time variable at the handles structure
guidata(gcbo,handles); % Save the changes made to the handles structure
% --- Executes on button press in pushbutton3.
function pushbutton3_Callback(hObject, eventdata, handles)
% hObject handle to pushbutton3 (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
close; % Close the application
% --- Executes on button press in pushbutton4.
function pushbutton4_Callback(hObject, eventdata, handles)
% hObject handle to pushbutton4 (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
if (get(handles.radiobutton4,'Value') == get(handles.radiobutton4,'Max'))
t=handles.time; % recover the saved variable t from the handles structure
y=handles.amp; % recover the saved variable y from the handles structure
p=ones(1, length(t)); % creates a vector containing only ones
outideal=p.*y; % Multiplies the two vectors to get the output of an ideal sampler
axes(handles.axessampled) % Select the proper axes
stem(t,outideal,'ro');
xlabel('Time');
ylabel('Amplitude');
grid on;
handles.signal=outideal;
guidata(gcbo,handles);
elseif (get(handles.radiobutton5,'Value') == get(handles.radiobutton5,'Max'))
t=handles.time; % recover the saved variable t from the handles structure
y=handles.amp; % recover the saved variable y from the handles structure
p=ones(1, length(t)); % creates a vector containing only ones
outhold=p.*y; % Multiplies the two vectors to get the output of an ideal sampler
axes(handles.axessampled) % Select the proper axes
stairs(t,outhold,'r'); %Plot the signal in a stairs shape making it looks like a zero order hold sampler
xlabel('Time');
ylabel('Amplitude');
grid on;
handles.signal=outhold;
guidata(gcbo,handles);
elseif (get(handles.radiobutton6,'Value') == get(handles.radiobutton6,'Max'))
t=handles.time; % recover the saved variable t from the handles structure
y=handles.amp; % recover the saved variable y from the handles structure
test1=eval(get(handles.edit1,'String')); % Evals the value that is contained in the Edit 1
if isnan(test1) % Test if it is a number or not. If not it displays an error message
errordlg('You must enter a numeric value','Bad Input','modal')
end
lenp=length(t)/length(test1); %Calculates the length of the vector so it can make it a periodic signal with the
%right size so it can work properly
p=ones(1, lenp); % Creates a vector of only ones of lenght lenp
per=test1'*p; % Creates a matrix, containing lenp times the vector test1
per=per(:); % Concatenates the columns of the matrix so it become
没有合适的资源?快使用搜索试试~ 我知道了~
【数字信号调制】 GUI PCM编码调制【含Matlab源码 453期】.zip
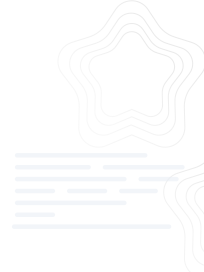
共3个文件
png:1个
m:1个
fig:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 89 浏览量
2024-06-22
11:35:31
上传
评论
收藏 262KB ZIP 举报
温馨提示
Matlab领域上传的代码均可运行,亲测可用,直接替换数据即可,适合小白; 1、代码压缩包内容 主函数:main.m; 调用函数:其他m文件;无需运行 运行结果效果图; 2、代码运行版本 Matlab 2019b;若运行有误,根据提示修改;若不会,私信博主; 3、运行操作步骤 步骤一:将所有文件放到Matlab的当前文件夹中; 步骤二:双击打开main.m文件; 步骤三:点击运行,等程序运行完得到结果; 4、仿真咨询 如需其他服务,可私信博主或扫描博客文章底部QQ名片; 4.1 博客或资源的完整代码提供 4.2 期刊或参考文献复现 4.3 Matlab程序定制 4.4 科研合作 功率谱估计: 故障诊断分析: 雷达通信:雷达LFM、MIMO、成像、定位、干扰、检测、信号分析、脉冲压缩 滤波估计:SOC估计 目标定位:WSN定位、滤波跟踪、目标定位 生物电信号:肌电信号EMG、脑电信号EEG、心电信号ECG 通信系统:DOA估计、编码译码、变分模态分解、管道泄漏、滤波器、数字信号处理+传输+分析+去噪、数字信号调制、误码率、信号估计、DTMF、信号检测识别融合、LEACH协议、信号检测、水声通信
资源推荐
资源详情
资源评论
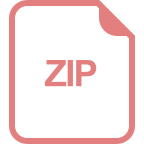
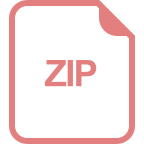
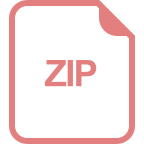
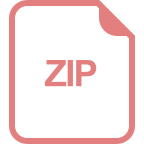
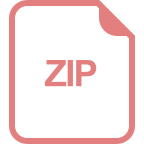
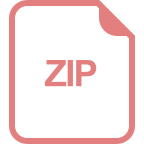
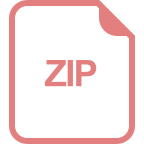
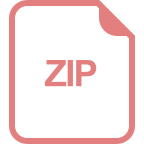
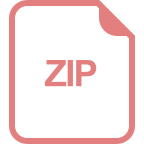
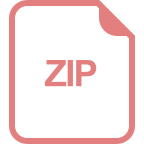
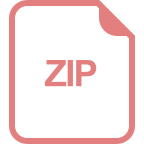
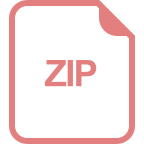
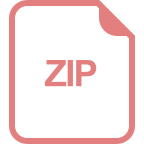
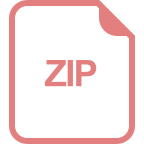
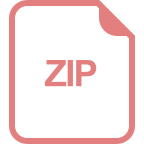
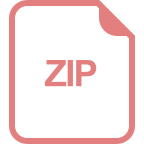
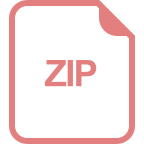
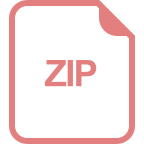
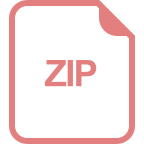
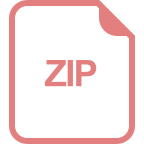
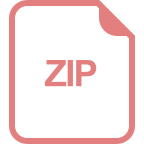
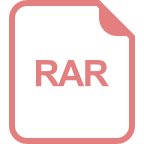
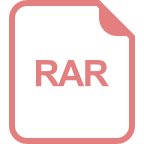
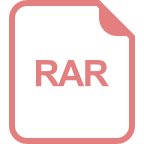
收起资源包目录


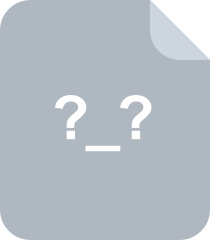
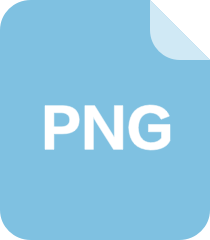
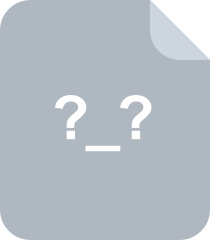
共 3 条
- 1
资源评论
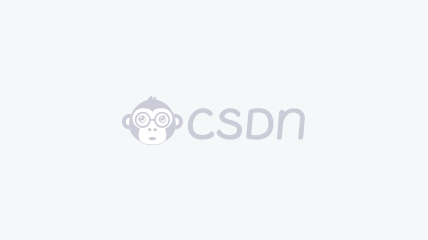


Matlab领域
- 粉丝: 2w+
- 资源: 2639
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

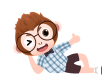
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


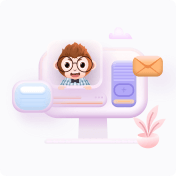
安全验证
文档复制为VIP权益,开通VIP直接复制
