%% Firefly Algorithm image color quantization using clustering
clear;
clc;
warning('off');
img=imread('r.jpg');
img=im2double(img);
% Separating color channels
R=img(:,:,1);
G=img(:,:,2);
B=img(:,:,3);
% Reshaping each channel into a vector and combine all three channels
X=[R(:) G(:) B(:)];
%% Starting FA Clustering
k = 6; % Number of Colors (cluster centers)
%---------------------------------------------------
CostFunction=@(m) ClusterCost(m, X); % Cost Function
VarSize=[k size(X,2)]; % Decision Variables Matrix Size
nVar=prod(VarSize); % Number of Decision Variables
VarMin= repmat(min(X),k,1); % Lower Bound of Variables
VarMax= repmat(max(X),k,1); % Upper Bound of Variables
% Firefly Algorithm Parameters
MaxIt = 100; % Maximum Number of Iterations
nPop = 7; % Number of Fireflies (Swarm Size)
gamma = 1; % Light Absorption Coefficient
beta0 = 2; % Attraction Coefficient Base Value
alpha = 0.2; % Mutation Coefficient
alpha_damp = 0.98; % Mutation Coefficient Damping Ratio
delta = 0.05*(VarMax-VarMin); % Uniform Mutation Range
m = 2;
if isscalar(VarMin) && isscalar(VarMax)
dmax = (VarMax-VarMin)*sqrt(nVar);
else
dmax = norm(VarMax-VarMin);
end
% Start
% Empty Firefly Structure
firefly.Position = [];
firefly.Cost = [];
firefly.Out = [];
% Initialize Population Array
pop = repmat(firefly, nPop, 1);
% Initialize Best Solution Ever Found
BestSol.Cost = inf;
% Create Initial Fireflies
for i = 1:nPop
pop(i).Position = unifrnd(VarMin, VarMax, VarSize);
[pop(i).Cost, pop(i).Out] = CostFunction(pop(i).Position);
if pop(i).Cost <= BestSol.Cost
BestSol = pop(i);
end
end
% Array to Hold Best Cost Values
BestCost = zeros(MaxIt, 1);
%% Firefly Algorithm Main Loop
for it = 1:MaxIt
newpop = repmat(firefly, nPop, 1);
for i = 1:nPop
newpop(i).Cost = inf;
for j = 1:nPop
if pop(j).Cost < pop(i).Cost
rij = norm(pop(i).Position-pop(j).Position)/dmax;
beta = beta0.*exp(-gamma.*rij^m);
e = delta.*unifrnd(-1, +1, VarSize);
%e = delta*randn(VarSize);
newsol.Position = pop(i).Position ...
+ beta.*rand(VarSize).*(pop(j).Position-pop(i).Position) ...
+ alpha.*e;
newsol.Position = max(newsol.Position, VarMin);
newsol.Position = min(newsol.Position, VarMax);
[newsol.Cost newsol.Out] = CostFunction(newsol.Position);
if newsol.Cost <= newpop(i).Cost
newpop(i) = newsol;
if newpop(i).Cost <= BestSol.Cost
BestSol = newpop(i);
end
end
end
end
end
% Merge
pop = [pop
newpop];
% Sort
[~, SortOrder] = sort([pop.Cost]);
pop = pop(SortOrder);
% Truncate
pop = pop(1:nPop);
% Store Best Cost Ever Found
BestCost(it) = BestSol.Cost;
BestRes(it)=BestSol.Cost;
disp(['Iteration ' num2str(it) ': Best Cost = ' num2str(BestCost(it))]);
% Damp Mutation Coefficient
alpha = alpha*alpha_damp;
FACenters=Res(X, BestSol);
end
FAlbl=BestSol.Out.ind;
% Plot FA Train
figure;
plot(BestRes,'--k','linewidth',2);
title('FA Train');
xlabel('FA Iteration Number');
ylabel('FA Best Cost Value');
%% Converting cluster centers and its indexes into image
Z=FACenters(FAlbl',:);
R2=reshape(Z(:,1),size(R));
G2=reshape(Z(:,2),size(G));
B2=reshape(Z(:,3),size(B));
% Attaching color channels
quantized=zeros(size(img));
quantized(:,:,1)=R2;
quantized(:,:,2)=G2;
quantized(:,:,3)=B2;
% Plot Results
figure;
subplot(1,2,1);
imshow(img);title('Original');
subplot(1,2,2);
imshow(quantized);title('Quantized Image');


Matlab领域
- 粉丝: 3w+
- 资源: 3764
最新资源
- 风力发电MPPT并网模型的策略模块封装及步长选择策略:变步长与固定步长两种策略对比,风力发电mppt并网模型,策略模块已mask封装,可以选定步长和变步长2种策略 ,核心关键词:风力发电; MPP
- 基于51单片机的Proteus仿真:人数检测显示与报警系统实战应用,基于51单片机的人数检测显示及报警检测Proteus仿真 ,基于51单片机;人数检测显示;报警检测;Proteus仿真,"基于51单
- 基于STM32F系列开发的高效稳定企业级变频器方案-原理图与硬件设计说明大全,基于STM32开发变频器-企业级成熟量产方案 包含:变频控制板-原理图、PCB 变频逆变器功率板-原理图 PCB 控制板
- 基于事件触发模型的倒立摆控制仿真实验-Simulink模型与详细参考文献,【有参考文献】事件触发模型 可实现倒立摆控制仿真实验 simulink模型可直接运行 含详细参考文献 ,关键词:事件触发模
- 智能小车路径规划算法:融合RRT与Dubins及A*方法的创新路径规划策略,智能小车路径规划 算法:RRT与Dubins相结合的方法,混合A*与Dubins相结合的方法 实现智能小车最短路径规划
- 基于FOC、SMO与PLL融合技术的Simlink仿真模型研究,FOC+SMO+PLL的Simlink仿真模型 ,关键词:FOC(Field Oriented Control);SMO(Smooth
- 三相异步电机矢量控制仿真模型的构建与性能分析,三相异步电机矢量控制仿真模型 ,核心关键词:三相异步电机;矢量控制;仿真模型;分号分隔的关键词为:三相异步电机;矢量控制技术;仿真模型构建 ,三相异步电
- 模拟IC设计学习与实战:探索知名大厂DCDC电路,高转换效率标准单元库器件与Cadence环境下的原理图实战应用,模拟IC模拟IC设计,集成电路,知名大厂的逆向DCDC电路(buck-boost功能)
- Boost电路电压闭环仿真研究:包含PI控制与零极点补偿器的模型及其性能分析,boost电路电压闭环仿真 有pi控制和零极点补偿器两种 仿真误差0.00705,仿真波形如图二所示 所搭建的模型输入电压
- TCR+FC型SVC无功补偿双封装Simulink仿真模型详解与实例资料集锦,TCR+FC型svc无功补偿simulink仿真模型,一共两个仿真,如下图所示,两个其实大致内容差不多,只是封装不同,有详
- COMSOL模拟动水条件下裂隙注浆扩散过程:粘度时变影响研究,COMSOL模拟动水条件联系裂隙注浆扩散,考虑粘度时变 ,核心关键词:COMSOL模拟; 动水条件; 裂隙注浆扩散; 粘度时变; 注浆扩散
- 分布式电源接入对配电网的潮流影响分析:Matlab程序模拟的探索与探索 ,分布式电源接入对配电网的影响(matlab程序) 分布式电源的接入使得配电系统从放射状无源网络变为分布有中小型电源的有源网络
- XC7V2000T与TMS320C6678设计文件集:原理图、PCB等齐全,验证通过,直接生产使用,XC7V2000T+TMS320C6678设计文件,包含原理图,PCB等文件,已验证,可直接生产
- 基于STM32G431主控的最新磁链观测器优化方案:无感foc浮点运算,弦波pmsm无刷电机控制源代码集,卓越效果无库文件原理图解,磁链观测器,无感foc ,弦波 pmsm 无刷电机控制方案 最新优
- 基于Matlab的无线充电仿真:LCC谐振器与不同拓扑的磁耦合谐振无线电能传输系统解析与建模,无线充电仿真 simulink 磁耦合谐振 无线电能传输 MCR WPT lcc ss llc拓扑补偿
- 直流无刷电机:高效磨头加工利器,直径38mm,转速高达25000rpm,功率达200W,直流无刷电机,直径38mm,径向长23.8mm,转速25000rpm,功率200W,可用于磨头加工 ,核心关键
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


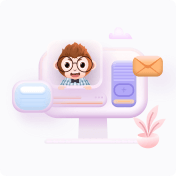