function dst = BEMD(src,a,b,c,d,e)
% BEMD This program calculates the Bidimensional EMD of a 2-d signal using
% the process of sifting. It is dependent on the function SIFT.
% Assigning the initial values of 'h' (data for sifting) and 'k' (imf index)
h_func = src;
k=1;
% The process of Sifting
dst=zeros(size(h_func));
% figure,imshow(orig);
while(k<5)
[imf_temp, residue_temp] = sift(h_func);
imf_matrix(:,:,k) = imf_temp; %#ok<AGROW>
k = k+1;
h_func = residue_temp;%余量图像
end
% Assigning the final residue to the last IMF index
imf_matrix(:,:,k) = residue_temp;%4个IMF图像和一个余量图像
% orig =orig-residue_temp;
% orig =imf_matrix(:,:,1)+imf_matrix(:,:,2)+imf_matrix(:,:,3);
dst =a*imf_matrix(:,:,1)+b*imf_matrix(:,:,2)+c*imf_matrix(:,:,3)+d*imf_matrix(:,:,4)+e*imf_matrix(:,:,5);
% orig =orig+h_f;
%imf_matrix(:,:,k+1)=orig;
% End of BEMD Computation
% subplot(3,2,1),imshow(cast(imf_matrix(:,:,1) ,'uint8'));
% subplot(3,2,2),imshow(cast(imf_matrix(:,:,2) ,'uint8'));
% subplot(3,2,3),imshow(cast(imf_matrix(:,:,3) ,'uint8'));
% subplot(3,2,4),imshow(cast(imf_matrix(:,:,4) ,'uint8'));
% subplot(3,2,5),imshow(cast(imf_matrix(:,:,5) ,'uint8'));
% figure(2),imshow(cast(dst ,'uint8'));
% figure,imshow(cast(imf_matrix(:,:,1) ,'int8'));
% figure,imshow(cast(imf_matrix(:,:,2) ,'int8'));
% figure,imshow(cast(imf_matrix(:,:,3) ,'int8'));
% figure,imshow(cast(imf_matrix(:,:,4) ,'int8'));
% figure,imshow(cast(imf_matrix(:,:,5) ,'int8'));
% %subplot(3,2,4),imshow(imf_matrix(:,:,4));
% % subplot(3,2,5),imshow(imf_matrix(:,:,5));
% orig=cast(orig ,'int8');
%figure,imshow(im );
% figure,imshow(orig);
end
%%
function [ h_imf residue ] = sift( input_image )
% This function sifts for a single IMF of the given 2D signal input
% Pre-processing
[len bre] = size(input_image);
x = 1:len;
y = 1:bre;
input_image_temp = input_image;
while(1)
% Finding the extrema in the 2D signal
[zmax imax zmin imin] = extrema2(input_image_temp);
[xmax ymax] = ind2sub(size(input_image_temp),imax);
[xmin ymin] = ind2sub(size(input_image_temp),imin);
% Interpolating the extrema to get the extrema suraces
[zmaxgrid , ~, ~] = gridfit(ymax,xmax,zmax,y,x);
[zmingrid, ~, ~] = gridfit(ymin,xmin,zmin,y,x);
% Averaging the extrema to get the Zavg surface
zavggrid = (zmaxgrid + zmingrid)/2;
% Computation of the h_imf (IMF for the 'h' input)
h_imf = input_image_temp - zavggrid;
% Computing IMF cost
eps = 0.00000001;
num = sum(sum((h_imf-input_image_temp).^2));
den = sum(sum((input_image_temp).^2)) + eps;
cost = num/den;
% Checking the IMF accuracy
if cost<0.2
break;
else
input_image_temp = h_imf;
end
end
% Computation of the Residue after IMF computation
residue = input_image - h_imf;
end
%%
function [xmax,imax,xmin,imin] = extrema(x)
%EXTREMA Gets the global extrema points from a time series.
% [XMAX,IMAX,XMIN,IMIN] = EXTREMA(X) returns the global minima and maxima
% points of the vector X ignoring NaN's, where
% XMAX - maxima points in descending order
% IMAX - indexes of the XMAX
% XMIN - minima points in descending order
% IMIN - indexes of the XMIN
%
% DEFINITION (from http://en.wikipedia.org/wiki/Maxima_and_minima):
% In mathematics, maxima and minima, also known as extrema, are points in
% the domain of a function at which the function takes a largest value
% (maximum) or smallest value (minimum), either within a given
% neighbourhood (local extrema) or on the function domain in its entirety
% (global extrema).
%
% Example:
% x = 2*pi*linspace(-1,1);
% y = cos(x) - 0.5 + 0.5*rand(size(x)); y(40:45) = 1.85; y(50:53)=NaN;
% [ymax,imax,ymin,imin] = extrema(y);
% plot(x,y,x(imax),ymax,'g.',x(imin),ymin,'r.')
%
% See also EXTREMA2, MAX, MIN
% Written by
% Lic. on Physics Carlos Adri醤 Vargas Aguilera
% Physical Oceanography MS candidate
% UNIVERSIDAD DE GUADALAJARA
% Mexico, 2004
%
% nubeobscura@hotmail.com
% From : http://www.mathworks.com/matlabcentral/fileexchange
% File ID : 12275
% Submited at: 2006-09-14
% 2006-11-11 : English translation from spanish.
% 2006-11-17 : Accept NaN's.
% 2007-04-09 : Change name to MAXIMA, and definition added.
xmax = [];
imax = [];
xmin = [];
imin = [];
% Vector input?
Nt = numel(x);
if Nt ~= length(x)
error('Entry must be a vector.')
end
% NaN's:
inan = find(isnan(x));
indx = 1:Nt;
if ~isempty(inan)
indx(inan) = [];
x(inan) = [];
Nt = length(x);
end
% Difference between subsequent elements:
dx = diff(x);
% Is an horizontal line?
if ~any(dx)
return
end
% Flat peaks? Put the middle element:
a = find(dx~=0); % Indexes where x changes
lm = find(diff(a)~=1) + 1; % Indexes where a do not changes
d = a(lm) - a(lm-1); % Number of elements in the flat peak
a(lm) = a(lm) - floor(d/2); % Save middle elements
a(end+1) = Nt;
% Peaks?
xa = x(a); % Serie without flat peaks
b = (diff(xa) > 0); % 1 => positive slopes (minima begin)
% 0 => negative slopes (maxima begin)
xb = diff(b); % -1 => maxima indexes (but one)
% +1 => minima indexes (but one)
imax = find(xb == -1) + 1; % maxima indexes
imin = find(xb == +1) + 1; % minima indexes
imax = a(imax);
imin = a(imin);
nmaxi = length(imax);
nmini = length(imin);
% Maximum or minumim on a flat peak at the ends?
if (nmaxi==0) && (nmini==0)
if x(1) > x(Nt)
xmax = x(1);
imax = indx(1);
xmin = x(Nt);
imin = indx(Nt);
elseif x(1) < x(Nt)
xmax = x(Nt);
imax = indx(Nt);
xmin = x(1);
imin = indx(1);
end
return
end
% Maximum or minumim at the ends?
if (nmaxi==0)
imax(1:2) = [1 Nt];
elseif (nmini==0)
imin(1:2) = [1 Nt];
else
if imax(1) < imin(1)
imin(2:nmini+1) = imin;
imin(1) = 1;
else
imax(2:nmaxi+1) = imax;
imax(1) = 1;
end
if imax(end) > imin(end)
imin(end+1) = Nt;
else
imax(end+1) = Nt;
end
end
xmax = x(imax);
xmin = x(imin);
% NaN's:
if ~isempty(inan)
imax = indx(imax);
imin = indx(imin);
end
% Same size as x:
imax = reshape(imax,size(xmax));
imin = reshape(imin,size(xmin));
% Descending order:
[~,inmax] = sort(-xmax); clear temp
xmax = xmax(inmax);
imax = imax(inmax);
[xmin,inmin] = sort(xmin);
imin = imin(inmin);
end
%%
function [xymax,smax,xymin,smin] = extrema2(xy,varargin)
%EXTREMA2 Gets the extrema points from a surface.
% [XMAX,IMAX,XMIN,IMIN] = EXTREMA2(X) returns the maxima and minima
% elements of the matriz X ignoring NaN's, where
% XMAX - maxima points in descending order (the bigger first and so on)
% IMAX - linear indexes of the XMAX
% XMIN - minima points in descending order
% IMIN - linear indexes of the XMIN.
% The program uses EXTREMA.
%
% The extrema points are searched only through the column, the row and
% the diagonals crossing each matrix element, so it is not a perfect
% mathematical program and for this reason it has an optional argument.
% The user should be aware of these limitations.
%
% [XMAX,IMAX,XMIN,IMIN] = EXTREMA2(X,1) does the same but without
% searching through the diagonals (less strict and perhaps the user gets
% more output points).
%
% DEFINITION (from http://en.wikipedia.org/wiki/Maxima_and_minima):
% In mathematics, maxima and minima, also known as extrema, are points in
% the domain of a function at which the function takes a largest value
% (maximum) or smallest value (minimum), either within a given
% neighbourhood (local extrema) or on the function domain in its entirety
% (global extrema).
%
% Note: To change the linear index to (i,j) use IND2S


Matlab领域
- 粉丝: 3w+
- 资源: 3636
最新资源
- Matlab_水下无线光通信相关的类、函数和脚本.zip
- Matlab_数字图像处理的基本原理:用Matlab举例的实用方法.zip
- Matlab_硕士课题设计多车控制系统.zip
- Matlab_随机微分方程数值解的Matlab工具箱.zip
- Matlab_所制作的数字图像信号处理小程序可以实现对图像的读入与保存截取感兴趣的区域并对该区域进行各种几何变换图像信.zip
- Matlab_斯坦福大学机器学习,作者:Andrew Ng.zip
- Matlab_特征学习的Matlab代码.zip
- Matlab_提供包括Matlab和Python在内的代码,用于可视化数值实验结果.zip
- Matlab_梯度下降算法的Matlab库101版.zip
- Matlab_提取图像的灰度共生矩阵GLCM根据GLCM求解图像的概率特征利用特征训练SVM分类器对目标分类.zip
- Matlab_通过训练数据集学习特征约简投影和分类器模型,并将其应用于测试数据集的分类。本文比较了几种特征约简方法,主.zip
- Matlab_通过层析成像重建、教育研究和非营利用途来支持体积增材制造的软件.zip
- Matlab_通用Matlab工具箱.zip
- Matlab_图上多机器人路径规划的A算法求解.zip
- Matlab_头脑风暴软件MEG EEG fNIRS ECoG sEEG和电生理学.zip
- 基于Matlab开发的克里金插值,克里格插值GUI程序,内置四个模块,有数据浏览,数据预处理,经验半方差函数拟合以及克里金插值四个模块,稳定运行; 支持四种数据变处理,同时展示直方图和QQ图验证数据是
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


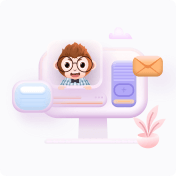