# axios
[](https://www.npmjs.org/package/axios)
[](https://travis-ci.org/axios/axios)
[](https://coveralls.io/r/mzabriskie/axios)
[](https://packagephobia.now.sh/result?p=axios)
[](http://npm-stat.com/charts.html?package=axios)
[](https://gitter.im/mzabriskie/axios)
[](https://www.codetriage.com/axios/axios)
Promise based HTTP client for the browser and node.js
## Features
- Make [XMLHttpRequests](https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest) from the browser
- Make [http](http://nodejs.org/api/http.html) requests from node.js
- Supports the [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) API
- Intercept request and response
- Transform request and response data
- Cancel requests
- Automatic transforms for JSON data
- Client side support for protecting against [XSRF](http://en.wikipedia.org/wiki/Cross-site_request_forgery)
## Browser Support
 |  |  |  |  |  |
--- | --- | --- | --- | --- | --- |
Latest ✔ | Latest ✔ | Latest ✔ | Latest ✔ | Latest ✔ | 11 ✔ |
[](https://saucelabs.com/u/axios)
## Installing
Using npm:
```bash
$ npm install axios
```
Using bower:
```bash
$ bower install axios
```
Using yarn:
```bash
$ yarn add axios
```
Using cdn:
```html
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
```
## Example
### note: CommonJS usage
In order to gain the TypeScript typings (for intellisense / autocomplete) while using CommonJS imports with `require()` use the following approach:
```js
const axios = require('axios').default;
// axios.<method> will now provide autocomplete and parameter typings
```
Performing a `GET` request
```js
const axios = require('axios');
// Make a request for a user with a given ID
axios.get('/user?ID=12345')
.then(function (response) {
// handle success
console.log(response);
})
.catch(function (error) {
// handle error
console.log(error);
})
.finally(function () {
// always executed
});
// Optionally the request above could also be done as
axios.get('/user', {
params: {
ID: 12345
}
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
})
.finally(function () {
// always executed
});
// Want to use async/await? Add the `async` keyword to your outer function/method.
async function getUser() {
try {
const response = await axios.get('/user?ID=12345');
console.log(response);
} catch (error) {
console.error(error);
}
}
```
> **NOTE:** `async/await` is part of ECMAScript 2017 and is not supported in Internet
> Explorer and older browsers, so use with caution.
Performing a `POST` request
```js
axios.post('/user', {
firstName: 'Fred',
lastName: 'Flintstone'
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
```
Performing multiple concurrent requests
```js
function getUserAccount() {
return axios.get('/user/12345');
}
function getUserPermissions() {
return axios.get('/user/12345/permissions');
}
axios.all([getUserAccount(), getUserPermissions()])
.then(axios.spread(function (acct, perms) {
// Both requests are now complete
}));
```
## axios API
Requests can be made by passing the relevant config to `axios`.
##### axios(config)
```js
// Send a POST request
axios({
method: 'post',
url: '/user/12345',
data: {
firstName: 'Fred',
lastName: 'Flintstone'
}
});
```
```js
// GET request for remote image
axios({
method: 'get',
url: 'http://bit.ly/2mTM3nY',
responseType: 'stream'
})
.then(function (response) {
response.data.pipe(fs.createWriteStream('ada_lovelace.jpg'))
});
```
##### axios(url[, config])
```js
// Send a GET request (default method)
axios('/user/12345');
```
### Request method aliases
For convenience aliases have been provided for all supported request methods.
##### axios.request(config)
##### axios.get(url[, config])
##### axios.delete(url[, config])
##### axios.head(url[, config])
##### axios.options(url[, config])
##### axios.post(url[, data[, config]])
##### axios.put(url[, data[, config]])
##### axios.patch(url[, data[, config]])
###### NOTE
When using the alias methods `url`, `method`, and `data` properties don't need to be specified in config.
### Concurrency
Helper functions for dealing with concurrent requests.
##### axios.all(iterable)
##### axios.spread(callback)
### Creating an instance
You can create a new instance of axios with a custom config.
##### axios.create([config])
```js
const instance = axios.create({
baseURL: 'https://some-domain.com/api/',
timeout: 1000,
headers: {'X-Custom-Header': 'foobar'}
});
```
### Instance methods
The available instance methods are listed below. The specified config will be merged with the instance config.
##### axios#request(config)
##### axios#get(url[, config])
##### axios#delete(url[, config])
##### axios#head(url[, config])
##### axios#options(url[, config])
##### axios#post(url[, data[, config]])
##### axios#put(url[, data[, config]])
##### axios#patch(url[, data[, config]])
##### axios#getUri([config])
## Request Config
These are the available config options for making requests. Only the `url` is required. Requests will default to `GET` if `method` is not specified.
```js
{
// `url` is the server URL that will be used for the request
url: '/user',
// `method` is the request method to be used when making the request
method: 'get', // default
// `baseURL` will be prepended to `url` unless `url` is absolute.
// It can be convenient to set `baseURL` for an instance of axios to pass relative URLs
// to methods of that instance.
baseURL: 'https://some-domain.com/api/',
// `transformRequest` allows changes to the request data before it is sent to the server
// This is only applicable for request methods 'PUT', 'POST', 'PATCH' and 'DELETE'
// The last function in the array must return a string or an instance of Buffer, ArrayBuffer,
// FormData or Stream
// You may modify the headers object.
transformRequest: [function (data, headers) {
// Do whatever you want to transform the data
return data;
}],
// `transformResponse` allows changes to the response data to be made before
// it is passed to then/catch
transformResponse: [function (data) {
// Do whatever you want to transform the data
return data;
}],
// `headers` are custom headers to be sent
headers: {'X-Requested-With': 'XMLHttpRequest'},
// `params` are the URL parameters to be sent with the request
// Must be a plain object or a URLSearchParams object
params: {
ID: 12345
},
// `paramsSerializer` is an optional function in charge of serializing `params`
// (e.g. https://w
没有合适的资源?快使用搜索试试~ 我知道了~
收起资源包目录

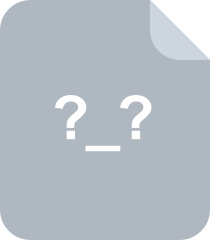
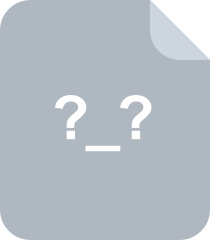
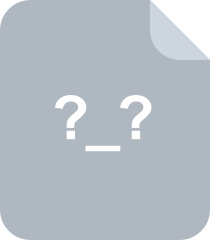
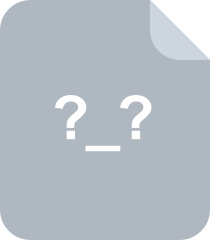
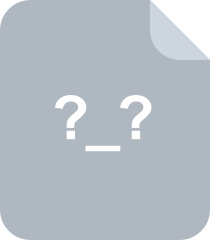
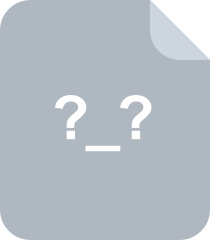
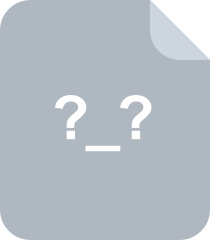
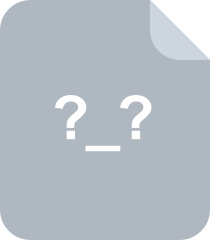
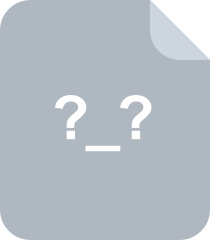
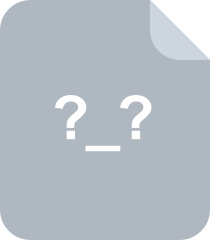
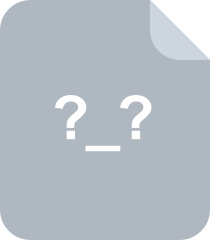
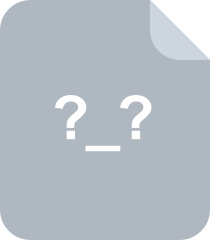
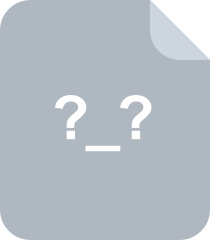
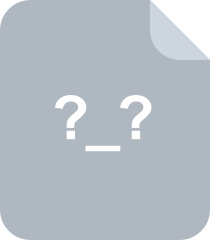
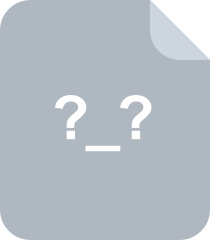
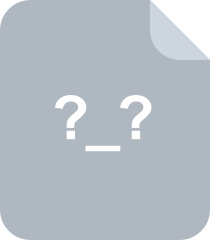
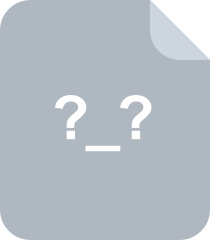
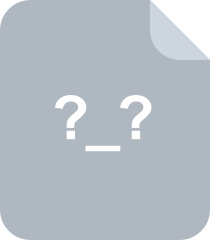
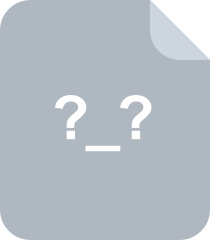
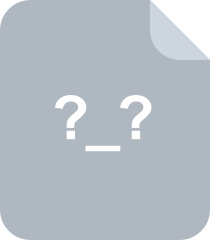
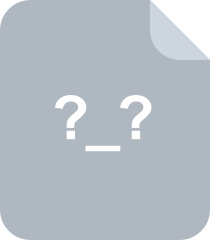
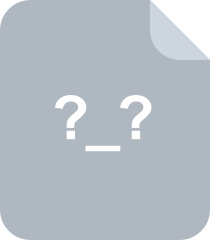
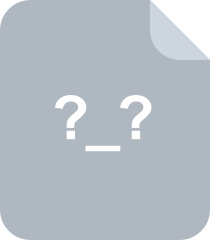
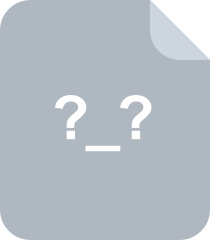
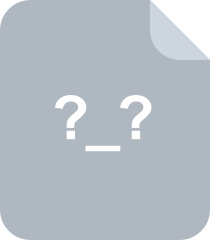
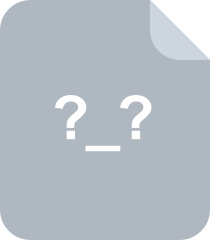
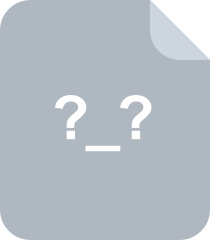
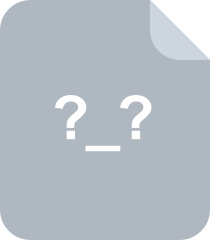
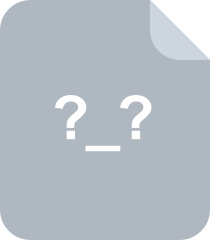
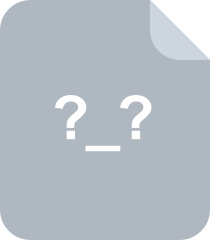
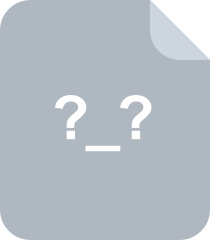
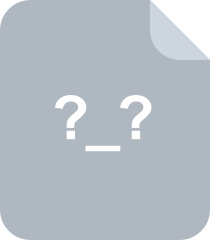
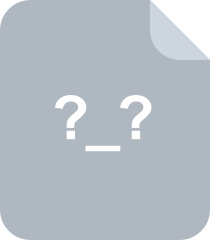
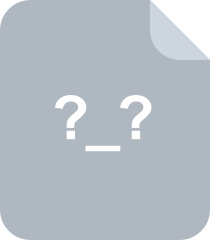
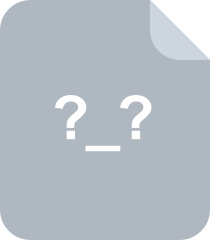
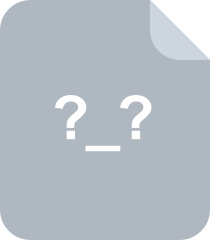
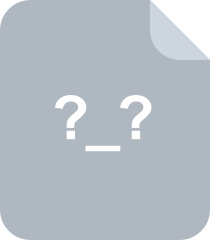
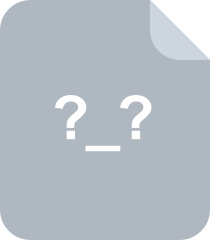
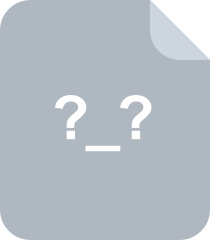
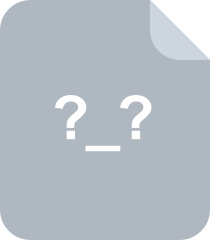
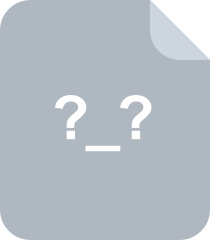
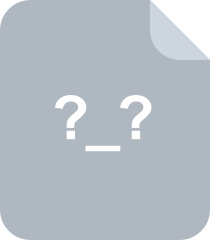
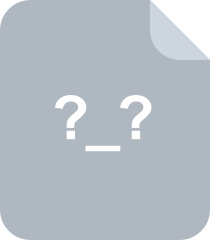
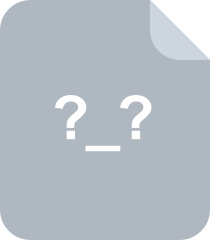
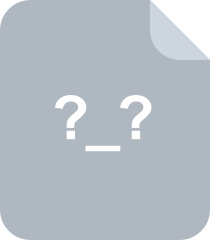
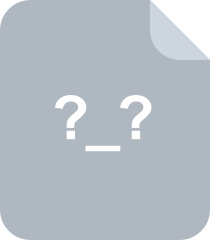
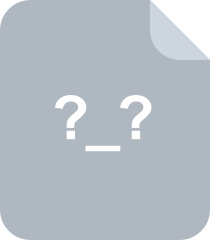
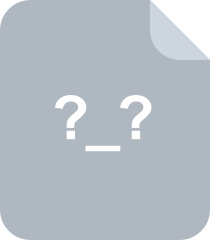
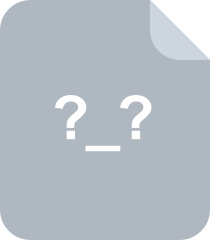
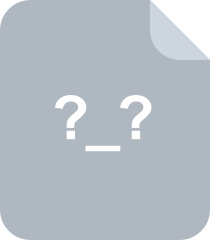
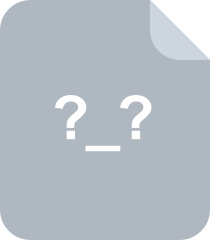
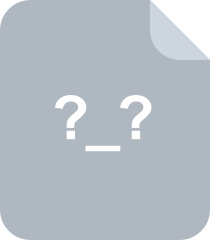
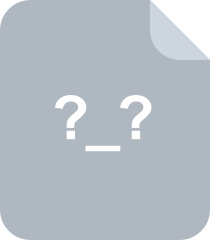
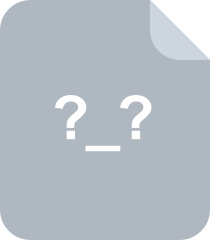
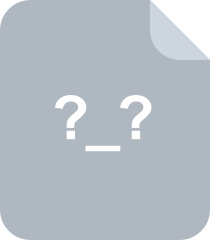
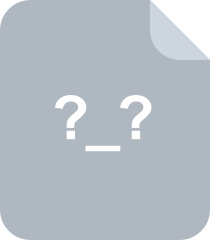
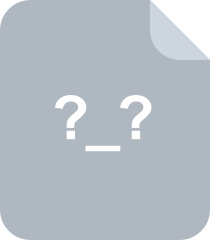
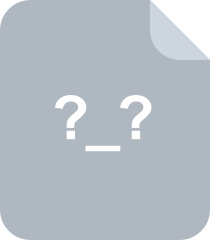
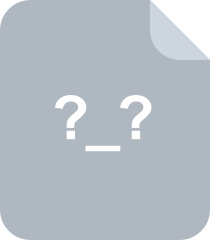
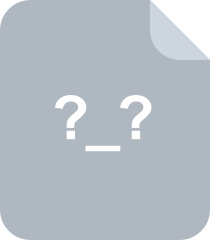
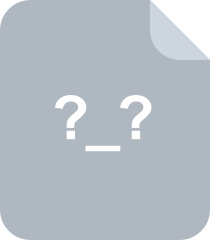
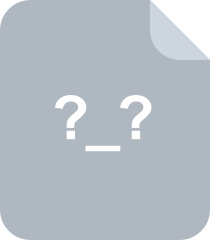
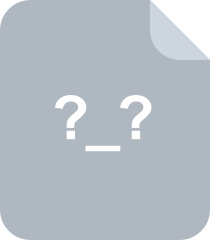
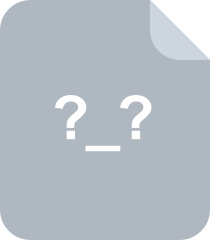
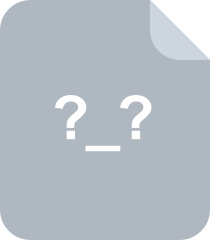
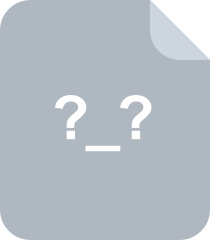
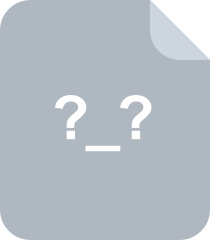
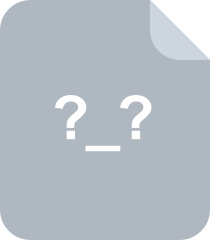
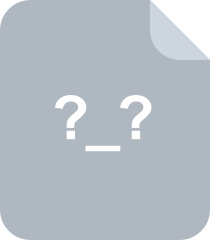
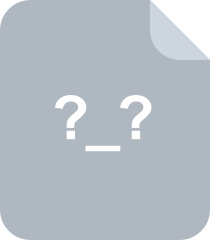
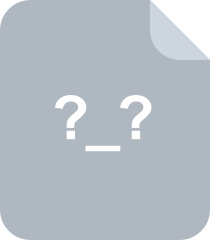
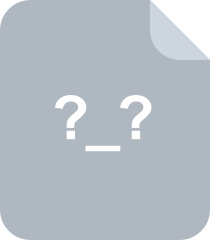
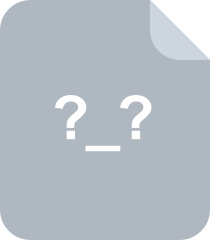
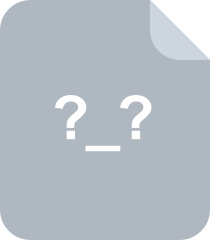
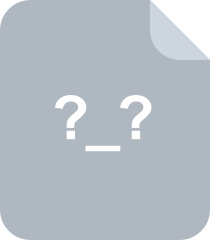
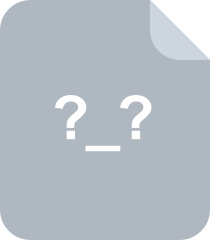
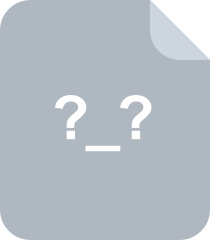
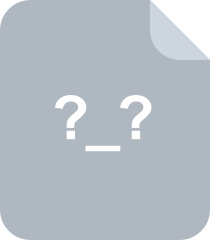
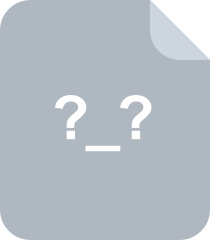
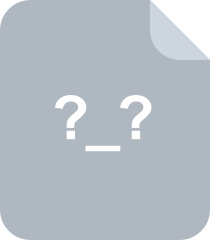
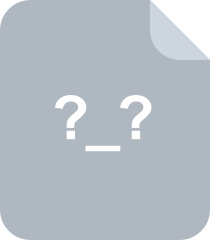
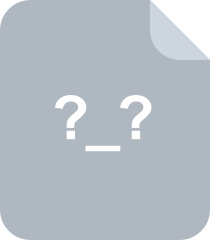
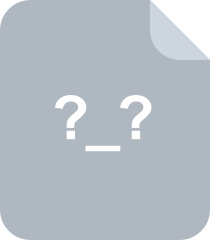
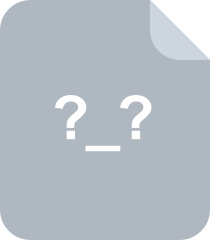
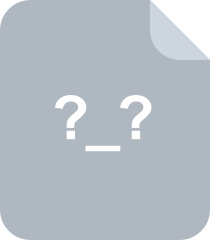
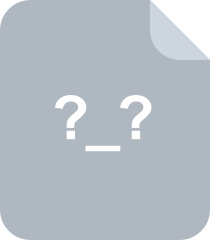
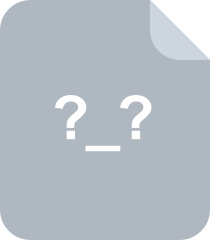
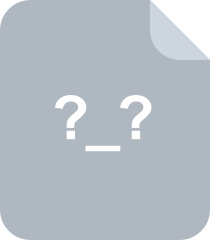
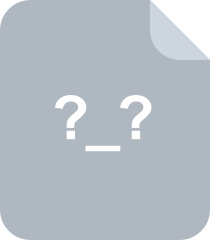
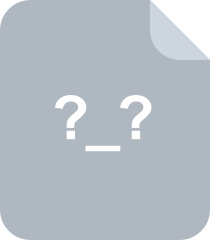
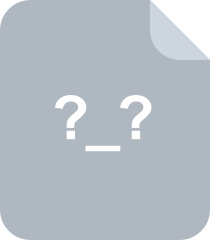
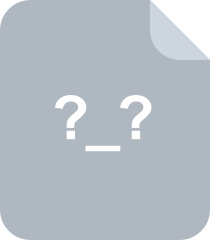
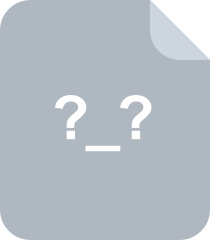
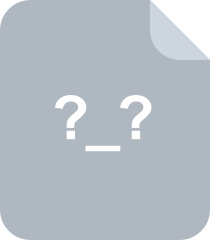
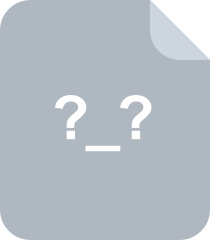
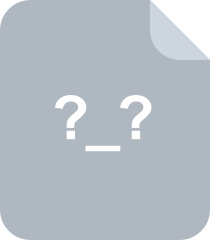
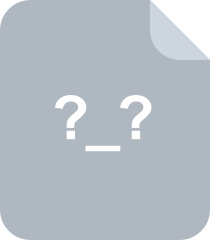
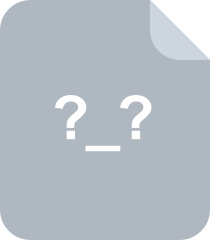
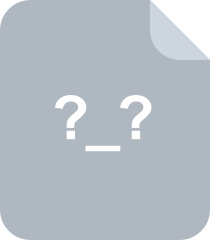
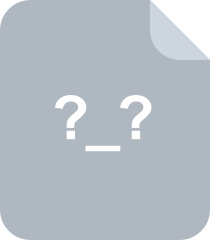
共 306 条
- 1
- 2
- 3
- 4
资源推荐
资源预览
资源评论
2021-07-01 上传
2021-10-05 上传
2022-03-22 上传
154 浏览量
178 浏览量
2021-10-05 上传
106 浏览量
2021-08-20 上传
183 浏览量
176 浏览量
125 浏览量
2021-02-19 上传
145 浏览量
186 浏览量
2024-06-10 上传
2024-03-05 上传
185 浏览量
2022-06-18 上传
2019-09-10 上传

146 浏览量
160 浏览量
172 浏览量
2019-09-03 上传
2021-10-30 上传
123 浏览量
2021-05-04 上传

194 浏览量

资源评论
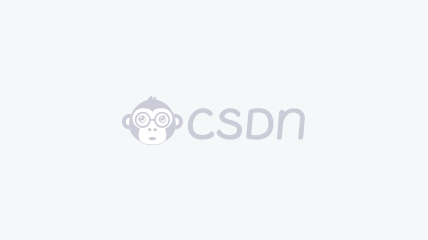

开测开测
- 粉丝: 1043
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

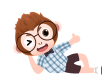
最新资源
- (免费下载)LogiSim 华中科技大学优化版 - 逻辑电路/电路设计/单片机
- Android大作业-仿抖音APP源代码+文档答辩ppt+演示视频(高分期末大作业)
- 植物大战僵尸Mixed
- 毕设-c语言UDP传输系统源码8.zip
- 毕设-c语言24点游戏源码6.zip
- 毕设-C语言超市管理系统1.zip
- 毕设-c语言Turbo C下写的俄罗斯方块7.zip
- 毕设-c语言别踩白块儿(双人版)源码10.zip
- 毕设-c语言吃逗游戏源码2.zip
- 毕设-c语言奔跑的火柴人游戏源码9.rar
- 毕设-c语言打字游戏代码4.zip
- 毕设-c语言打字母游戏源码3.zip
- 毕设-c语言大丰收游戏源码5.zip
- 17325458887980.zip
- 12.5MN冲孔压机机架结构及焊接工艺性浅析.pdf
- 12Cr1MoVG与TP347H(Ф38mm×5mm)钢管的钨极氩弧焊焊接工艺 - .pdf
安全验证
文档复制为VIP权益,开通VIP直接复制
