#include <graphics.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#define WIDTH 64
#define HEIGHT 20
int map[HEIGHT][WIDTH];
int piece[4][3] = {{1,1,0},{0,1,1},{1,0,1},{1,1,1}};
int dx = 0, dy = 0;
int newPiecePos(int x, int y);
int score = 0;
bool gameOver = false;
bool drawNextPiece = false;
void draw() { ... } // From previous segment
void update() { ... } // From previous segment
void newPiece() { ... } // Placeholder function for future development.
void handleInput() {
if (_kbhit()) { // Check if a key is pressed.
char key = _getch(); // Get the pressed key.
switch (key) { // Check the pressed key.
case 'a': // Left arrow key.
if (dx > 0) dx--; break; // Move the piece left.
case 'd': // Right arrow key.
if (dx < 3) dx++; break; // Move the piece right.
case 'w': // Up arrow key. Rotate the piece clockwise.
piece[2][0] = piece[2][0] == 0 ? 1 : 0; // Flip the third row of the piece. (Rotation).
piece[2][1] = piece[2][1] == 1 ? 0 : 1; // Flip the third column of the piece. (Rotation).
drawNextPiece = true; break; // Trigger a redraw with the new rotation. Note: Placeholder for future development. DrawNextPiece should be handled in draw() function.
case 's': // Down arrow key. Move the piece down if it's not in the ground or out of bounds. Otherwise, it's a suicide move. No need to handle it here since it'll cause an error in update() function.
break; // Not implemented yet.
case 'r': // Reset the game.
gameOver = false; break; // Set gameOver to false to start a new game.
default: break; // Other keys are not handled here.
}
}
}

LY-SGVision
- 粉丝: 0
- 资源: 3
最新资源
- 绩效考核管理制度.doc
- 企业绩效考核制度及方案(实例).doc
- 长虹集团绩效管理手册.doc
- 绩效考核制度.doc
- 美的干部绩效考核办法.doc
- 生产型企业绩效考核方案.doc
- 中国联通:绩效管理操作手册.doc
- 公司绩效考核全套流程表格.docx
- 中通关键岗位薪酬设计方案.doc
- “探讨功率因素调节中MPPT和SVPWM技术在三相光伏并网逆变器仿真模型中的应用:精确谐波畸变控制与性能优化”,500kW三相光伏并网逆变器的仿真模型: 1.光伏PV, DC DC采用MPPT最大功率
- buildnumber-maven-plugin-javadoc-1.2-7.el7.x64-86.rpm.tar.gz
- DSP2837系列串口升级方案:基于VS2013的双核与单核升级解决方案及源代码分享,DSP28377D串口升级方案 串口双核升级,上位机采用vs2013开发 稍微修改可支持2837x系列的单、双核
- bwidget-1.9.0-6.el7.x64-86.rpm.tar.gz
- 蓄电池与超级电容混合储能系统的功率分配及SOC管理策略-基于Matlab Simulink仿真模型探究,蓄电池与超级电容混合储能并网matlab simulink仿真模型 (1)混合储能采用低通滤
- byacc-1.9.20130304-3.el7.x64-86.rpm.tar.gz
- 1737485585760.png
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


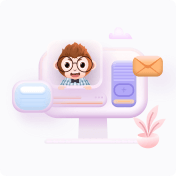