package com.swpu.uchain.takeawayapplet.service.impl;
import com.swpu.uchain.takeawayapplet.VO.ResultVO;
import com.swpu.uchain.takeawayapplet.config.UrlProperties;
import com.swpu.uchain.takeawayapplet.config.WeChatProperties;
import com.swpu.uchain.takeawayapplet.dto.OrderDTO;
import com.swpu.uchain.takeawayapplet.enums.ResultEnum;
import com.swpu.uchain.takeawayapplet.exception.GlobalException;
import com.swpu.uchain.takeawayapplet.form.PayForm;
import com.swpu.uchain.takeawayapplet.form.RefundForm;
import com.swpu.uchain.takeawayapplet.service.MessageService;
import com.swpu.uchain.takeawayapplet.service.OrderService;
import com.swpu.uchain.takeawayapplet.service.PayService;
import com.swpu.uchain.takeawayapplet.util.GetOpenIdUtil;
import com.swpu.uchain.takeawayapplet.util.PayUtil;
import com.swpu.uchain.takeawayapplet.util.RandomUtil;
import com.swpu.uchain.takeawayapplet.util.ResultUtil;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import javax.servlet.ServletInputStream;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.Map;
//import com.swpu.uchain.takeawayapplet.service.MessageService;
/**
* @ClassName PayServiceImpl
* @Author hobo
* @Date 19-3-21 下午8:11
* @Description
**/
@Service
@Slf4j
public class PayServiceImpl implements PayService {
@Autowired
private WeChatProperties weChatProperties;
@Autowired
private UrlProperties urlProperties;
@Autowired
private OrderService orderService;
@Autowired
private MessageService messageService;
/**
* @return void
* @Author hobo
* @Description : 创建预支付请求
* @Param [orderDTO]
**/
@Override
public ResultVO creat(PayForm payForm, String code, HttpServletRequest request) {
try {
//生成随机字符串
String nonceStr = RandomUtil.getRandomStringByLength(32);
//商品简介
String body = new String(weChatProperties.getTitle().getBytes("ISO-8859-1"), "UTF-8");
//获得本机的ip地址
String spbillCreatIp = PayUtil.getIpAddr(request);
String ordNo = payForm.getId() + "";
//支付金额 单位:分 转为元
String totalFee = payForm.getOrderAmount().multiply(new BigDecimal(100)) + "";
String openId = GetOpenIdUtil.getOpenId(code);
//生成预支付订单
Map<String, String> packageParams = new HashMap<String, String>();
packageParams.put("appid", weChatProperties.getAppid());
packageParams.put("mch_id", weChatProperties.getMchId());
packageParams.put("nonce_str", nonceStr);
packageParams.put("body", body);
packageParams.put("out_trade_no", ordNo);
packageParams.put("totalFee", totalFee);
packageParams.put("spbill_create_ip", spbillCreatIp);
packageParams.put("notify_url", weChatProperties.getNotifyUrl());
packageParams.put("trade_type", weChatProperties.getTradeType());
packageParams.put("open_id", openId);
//除去数组中的空值和签名参数
packageParams = PayUtil.paraFileter(packageParams);
String preStr = PayUtil.createLinkString(packageParams);
//MD5运算生成签名,第一次签名 调用统一下单接口
String mySign = PayUtil.sign(preStr, weChatProperties.getMchKey(), "utf-8").toUpperCase();
log.info("====================第一次签名" + mySign + "====================");
//连同生成的签名一起拼接成xml数据
String xml = "</xml version='1.0' encoding='gdk'>"
+ "<appid>" + weChatProperties.getAppid() + "</appid>"
+ "<body><![CDATA]" + body + "]]></body>"
+ "<mch_id>" + weChatProperties.getMchId() + "</mch_id>"
+ "<nonce_str>" + nonceStr + "</nonce_str>"
+ "<openid>" + openId + "</openid>"
+ "<out_trade_no>" + ordNo + "<out_trade_no>"
+ "<spbill_create_ip>" + spbillCreatIp + "</spbill_create_ip>"
+ "<total_fee>" + totalFee + "</total_fee>"
+ "<trade_type>" + weChatProperties.getTradeType() + "</trade_type>"
+ "<sign>" + mySign + "</sign>"
+ "</xml>";
log.info("====================调试请求XML数据====================");
System.out.println(xml);
//调用统一下单接口
String result = PayUtil.httpRequest(urlProperties.getPayUrl(), "POST", xml);
log.info("====================调试返回XML数据====================");
System.out.println(result);
// 将解析结果放入HashMap中
Map map = PayUtil.doXMLParse(result);
String return_code = (String) map.get("return_code");
//返回移动端需要的参数
Map<String, Object> response = new HashMap<String, Object>();
//return_code 为成功 return_msg 为失败
if ("SUCCESS".equals(return_code)) {
//业务结果
String prepay_id = (String) map.get("prepay_id");
response.put("nonceStr", nonceStr);
response.put("package", "prepay_id" + prepay_id);
Long timeStamp = System.currentTimeMillis() / 1000;
//时间 类型转换为微信官方要求的String
response.put("time_stamp", timeStamp + "");
String stringSignTemp = "appId=" + weChatProperties.getAppid() + "&nonceStr=" + nonceStr
+ "&package=prepay_id" + prepay_id + "&signType=MD5" + "&timeStamp=" + timeStamp;
//再次签名 用于小程序端调用wx.requestPayment方法
String paySign = PayUtil.sign(stringSignTemp, weChatProperties.getMchKey(), "utf-8").toUpperCase();
log.info("===================第二次签名:" + paySign + "====================");
response.put("paySign", paySign);
}
response.put("appid", weChatProperties.getAppid());
return ResultUtil.success(response);
} catch (Exception e) {
e.printStackTrace();
return ResultUtil.error(ResultEnum.PAY_REQUEST_FAIL);
}
}
/**
* @return void
* @Author hobo
* @Description : 异步通知判断签名
* @Param [notifyData]
**/
@Override
public ResultVO notify(HttpServletRequest request, HttpServletResponse response) throws Exception {
BufferedReader br = new BufferedReader(new InputStreamReader((ServletInputStream) request.getInputStream()));
String line = null;
StringBuffer sb = new StringBuffer();
while ((line = br.readLine()) != null) {
sb.append(line);
}
br.close();
String notifyXml = sb.toString();
String resXml = "";
log.info("接收到的报文 notifyXml={}", notifyXml);
Map map = PayUtil.doXMLParse(notifyXml);
//从报文中获取值
String returnCode = (String) map.get("return_code");
String outTradeNo = (String) map.get("out_trade_no");
String totalFee = (String) map.get("total_fee");
Long orderId = Long.parseLong(outTradeNo);
OrderDTO orderDTO = orderService.findOrder(orderId);
if (!(orderDTO.getOrderAmount() + "").equals(totalFee)) {
throw new GlobalException(ResultEnum.AMOUNT_ERROR);
}
if ("SUCCESS".equals(returnCode)) {
//验证签名是�
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
如果您下载了本程序,但是该程序存在问题无法运行,那么您可以选择退款或者寻求我们的帮助(如果找我们帮助的话,是需要追加额外费用的)。另外,您不会使用资源的话(这种情况不支持退款),也可以找我们帮助(需要追加额外费用) 随着移动互联网技术的发展和用户需求的变化,【小程序名称】应运而生,以其轻量化、便捷化的设计理念为用户提供了一种全新的服务模式。作为一款无需下载安装即可使用的应用,【小程序名称】依托于微信庞大的生态系统,让用户在微信内就能轻松实现各种功能操作。 【小程序名称】的核心功能主要集中在【具体服务领域】,例如在线购物、本地生活服务、教育学习或健康管理等。它简化了传统APP繁琐的注册登录流程,支持微信一键授权登录,极大地提升了用户体验。用户通过搜索或扫描二维码,瞬间即可开启使用,享受快速加载、流畅运行的服务。 该小程序界面设计简洁明了,布局合理,易于上手。同时,其特色功能如实时更新的信息推送、个性化推荐以及社交分享功能,让用户能够及时获取所需信息,并方便地将优质内容分享至朋友圈或好友,实现信息的高效传播与互动。 【小程序名称】注重数据安全与隐私保护,严格遵守国家法律法规和微信平台的规定,确保用户数据的安全无虞。此外,其背后的开发团队持续迭代更新,根据用户反馈不断优化产品性能,提升服务质量,致力于打造一个贴近用户需求、充满活力的小程序生态。 总结来说,【小程序名称】凭借其小巧便携、快捷高效的特性,不仅节省了用户的手机存储空间,更为用户提供了无缝衔接的便利服务,是现代生活中不可或缺的一部分,真正实现了“触手可及”的智能生活新体验。只需轻点屏幕,无限精彩尽在掌握之中。
资源推荐
资源详情
资源评论
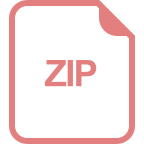
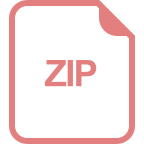
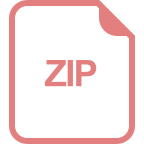
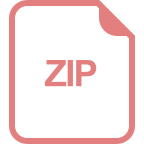
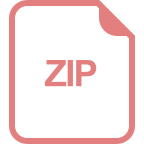
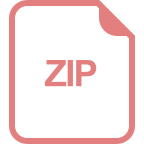
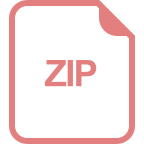
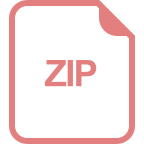
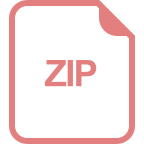
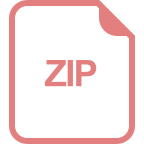
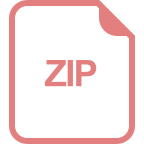
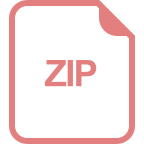
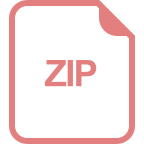
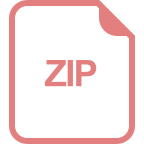
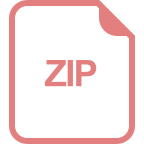
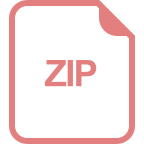
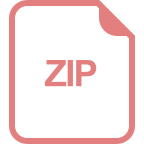
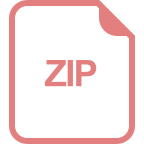
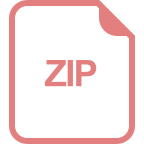
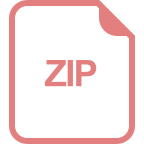
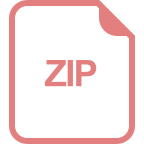
收起资源包目录

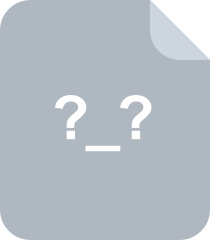
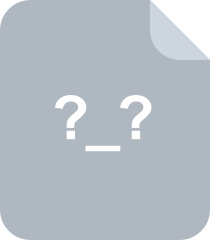
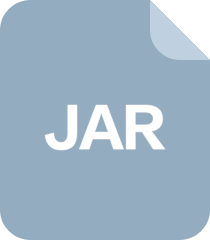
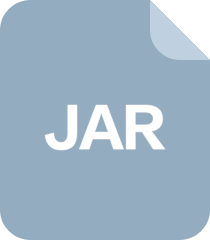
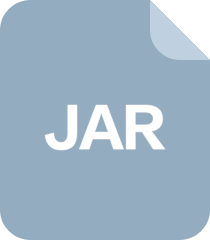
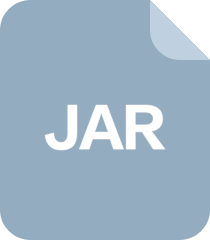
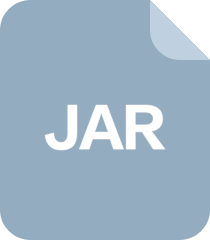
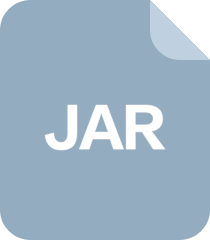
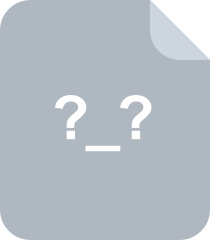
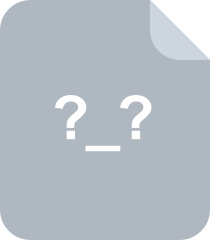
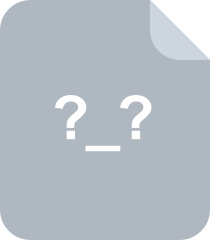
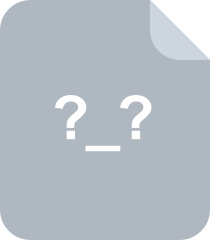
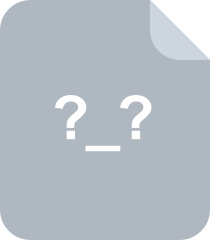
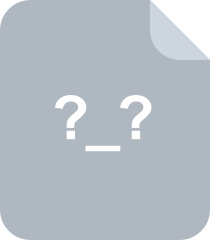
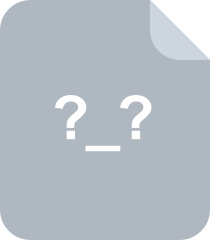
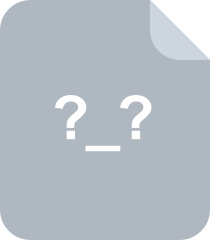
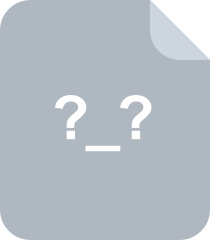
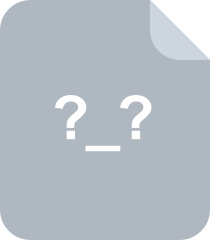
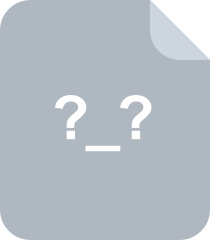
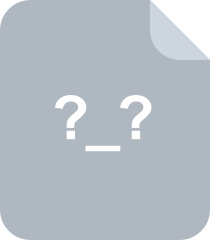
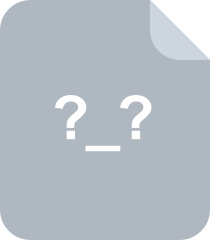
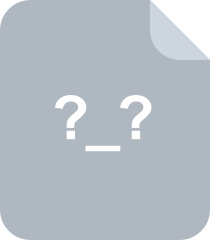
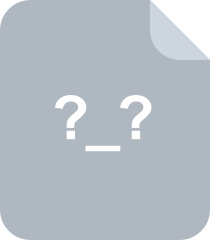
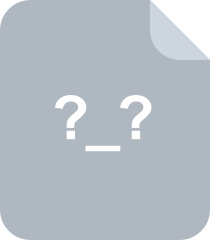
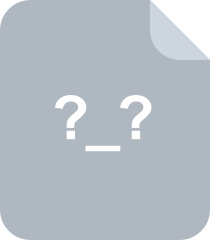
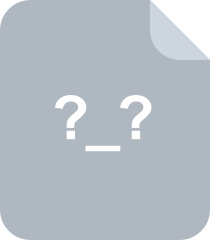
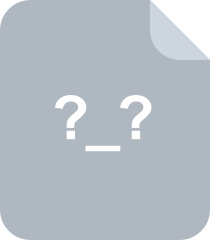
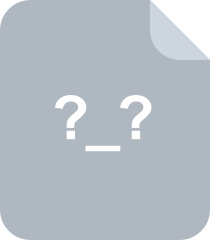
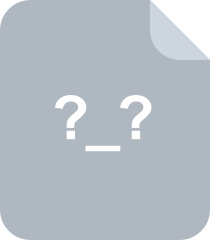
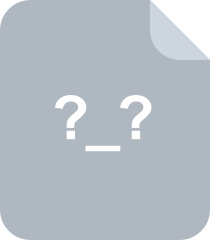
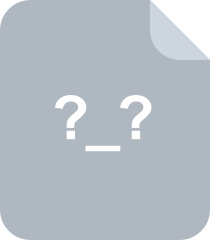
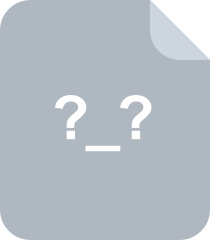
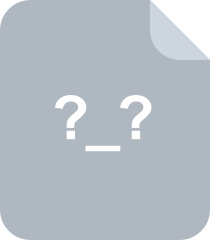
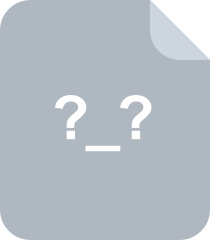
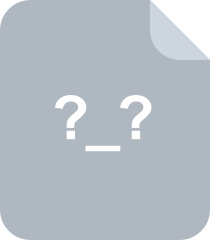
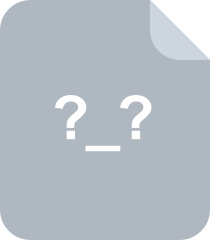
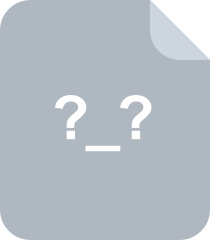
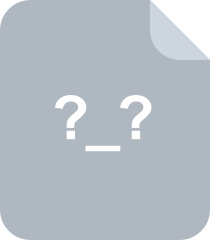
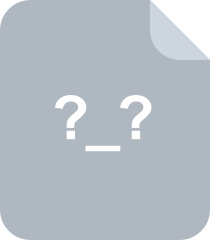
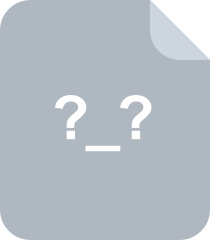
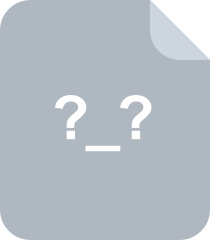
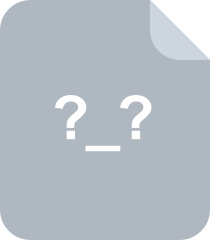
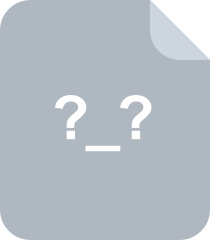
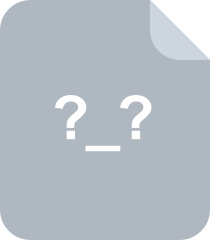
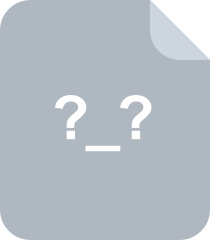
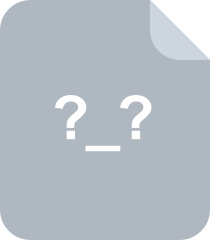
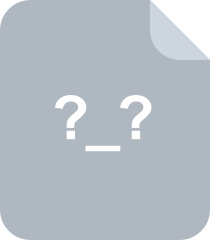
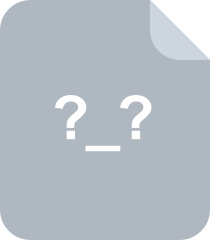
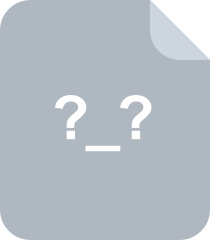
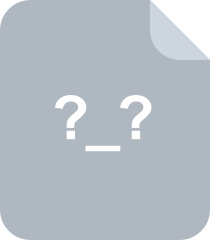
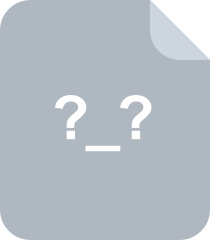
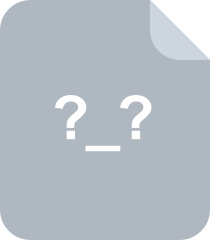
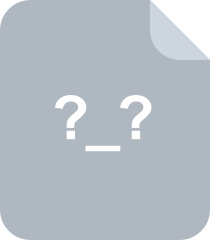
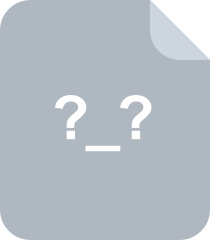
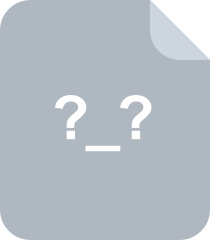
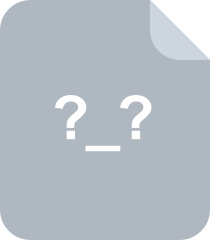
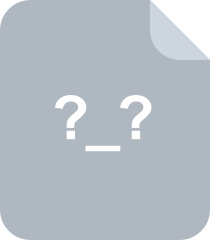
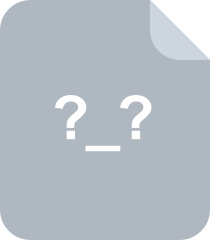
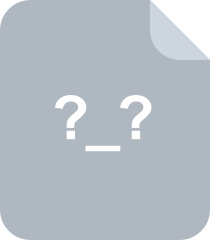
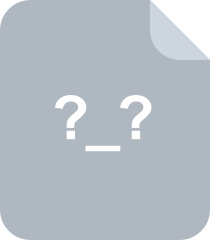
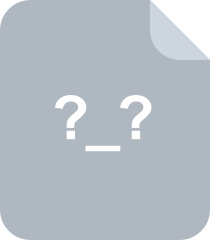
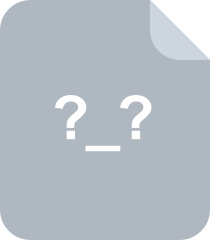
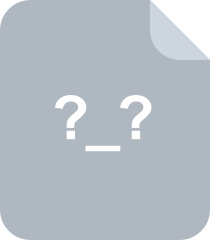
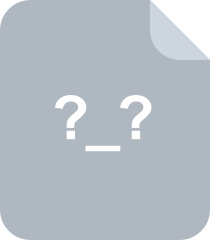
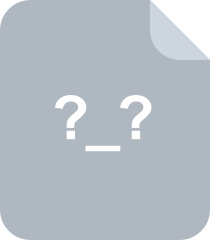
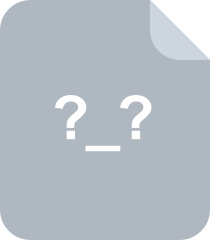
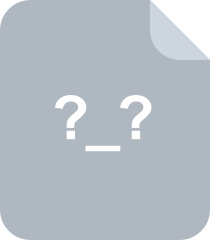
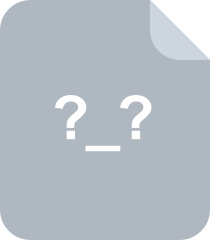
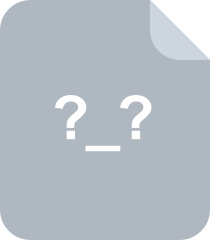
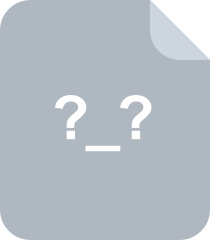
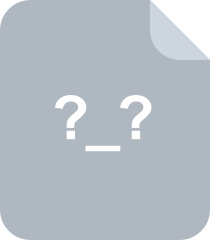
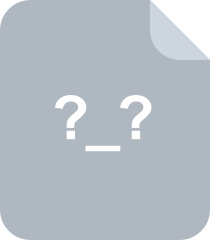
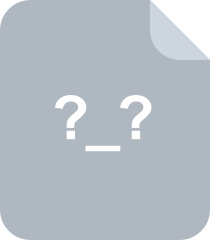
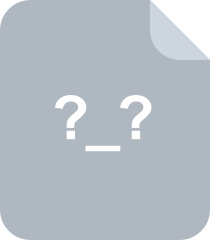
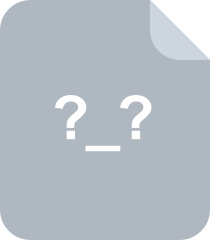
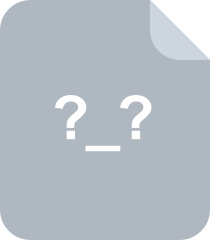
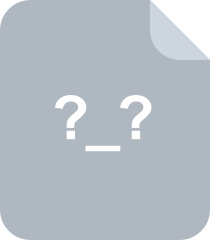
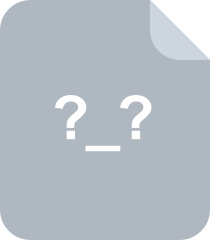
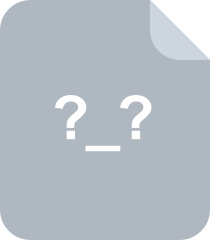
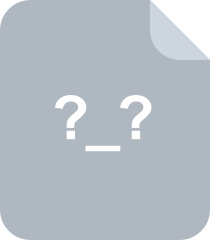
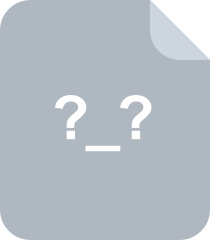
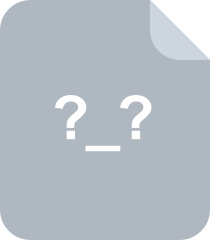
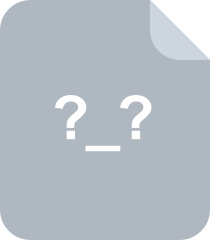
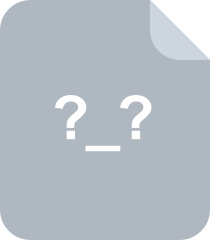
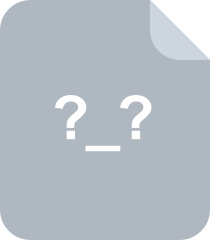
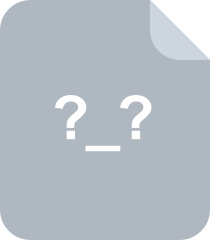
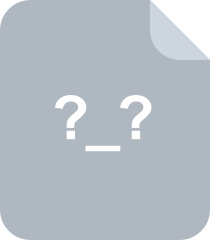
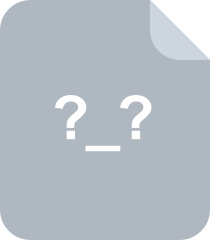
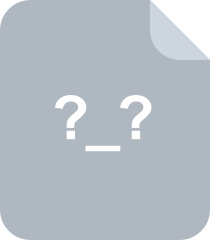
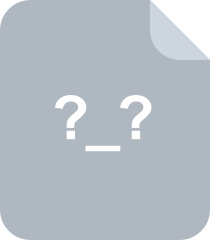
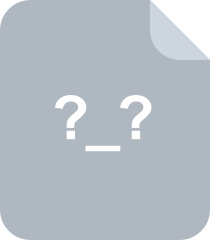
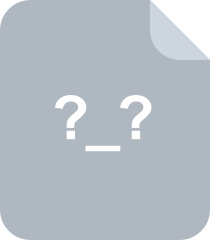
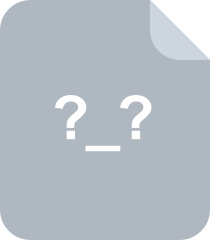
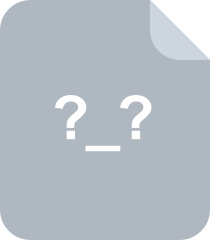
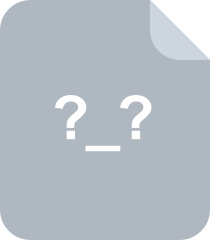
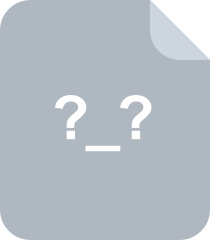
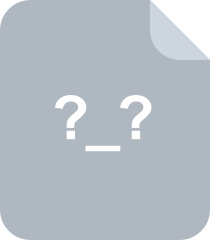
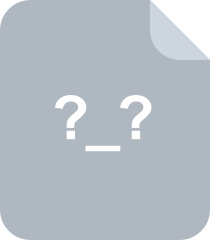
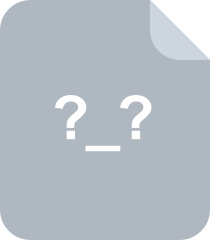
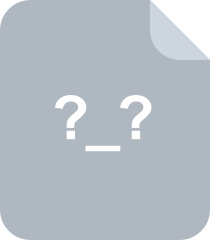
共 118 条
- 1
- 2
资源评论
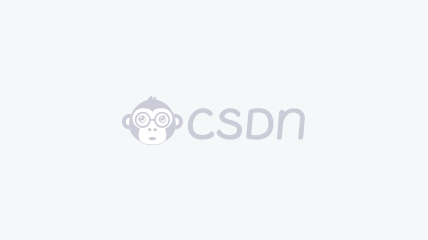

JJJ69
- 粉丝: 6366
- 资源: 5917
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

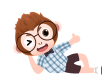
最新资源
- 基于微信平台的ssm高校毕业论文管理系统小程序(源码 + 数据库+LW+PPT)
- (25152814)VMware相关服务一键启动/关闭.bat
- 机器学习(预测模型):英特尔公司历史股票数据的数据集
- (29953412)个人博客微信QQ小程序源码包.7z
- Java毕设项目:基于spring+mybatis+maven+mysql实现的化妆品配方及工艺管理系统【含源码+数据库+开题报告+任务书+毕业论文】
- (5175244)在Microsoft Visual C++ 6.0环境下通过对Active X控件的编程来实现串口的通信的一般方
- pyinstaller -onefile -add-data "C:\\liteon\\HRZhaoMu\\SmartEsop\\whisper\\assets\\mel-filters.npz
- CFA知识点梳理系列:CFA Level II, Reading 7 Economics of Regulation
- (5857632)串口调试助手 串口调试
- (59423620)指纹识别基于matlab GUI指纹识别【含Matlab源码 1353期】.zip
- 2024最强Java面试八股文-最新面试题
- (6755822)基于TCP的VC++聊天室
- (8424006)动态网页设计
- (13391206)基于51单片机的计算器
- (172705856)软件工程导论(第六版)课后习题答案1
- (174525210)机器学习期末复习题选择题库
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


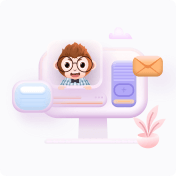
安全验证
文档复制为VIP权益,开通VIP直接复制
