import json
import os
import time
import uuid
from flask import request, Blueprint
from config import GetConfig
from server_utils.MongoDBOP import MongoDBOP
from user_manage.user_views import uo
class DataTree:
def __init__(self):
self.__mongo = MongoDBOP(**config.get_mongo_connection())
self.__collection = "user_data_tree"
self.__file_set = "file_store"
self.__icon_set = "icon"
self.__short_cut = "video_shortcut"
self.node_tem = {
"id": "",
"pid": "",
"uid": "",
"name": "",
"icon": "",
"children": [],
"data": []
}
self.data_tem = {
"fid": "",
"f_type": "",
"file_name": "",
"file_type": "",
"file_size": 0.0,
"create_time": 0.0,
"description": ""
}
def new_node(self, uid, name, pid=None, icon=None, children=None, data=None):
node = self.node_tem.copy()
node["id"] = str(uuid.uuid4())
node["name"] = name if name and isinstance(name, str) else ""
node["pid"] = pid if pid and isinstance(pid, str) else ""
node["uid"] = uid if uid and isinstance(uid, str) else ""
node["icon"] = icon if icon and isinstance(icon, str) else ""
node["children"] = children if children and isinstance(data, list) else []
node["data"] = data if data and isinstance(data, list) else []
return node
def new_file(self, file_name, f_path, f_type, description=None):
n_file = self.data_tem.copy()
n_file["fid"] = ""
n_file["f_type"] = f_type
n_file["file_name"] = file_name
n_file["file_type"] = os.path.splitext(f_path)[1]
n_file["file_size"] = round(os.path.getsize(f_path) / float(1024 * 1024), 2)
n_file["create_time"] = time.time()
n_file["description"] = description if description and isinstance(description, str) else ""
return n_file
@staticmethod
def __remove_oid(target):
if dict(target).__contains__("_id"):
del target["_id"]
return target
def create_tree(self, uid):
user_tree = self.new_node(uid, "root", icon="mif-tree")
if self.__mongo.exist(self.__collection, {"uid": uid, "name": "root"}):
self.__mongo.delete(self.__collection, {"uid": uid, "name": "root"})
res = self.__mongo.insert(self.__collection, user_tree.copy())
if res is not None:
return config.build_response("create_tree", user_tree, 200)
else:
return config.build_response("create_tree", "", 300)
def del_tree(self, uid):
res = self.__mongo.search_by_kv_pair(self.__collection, {"uid": uid})
op_list = []
data_list = []
for each in res:
op_list.append({"id": each["id"]})
data_list.extend(each["data"])
res = self.__mongo.bulk_operation(self.__collection, op_list, "delete")
self.__mongo.delete_files(self.__file_set, data_list)
op_count = len(op_list)
del op_list
if res.deleted_count == op_count:
return config.build_response("del_tree", {"nodes": res.deleted_count, "file": len(data_list)}, 200)
else:
return config.build_response("del_tree", "", 300)
def get_tree(self, uid):
res = self.__mongo.search_by_kv_pair(self.__collection, {"uid": uid, "name": "root"})
res_child = self.__mongo.search_by_kv_pair(self.__collection, {"uid": uid, "name": {"$ne": "root"}})
if len(res) > 0:
root = self.__remove_oid(res[0])
self.get_node(root, res_child)
return config.build_response("get_tree", root, 200)
else:
return config.build_response("get_tree", "", 102)
def get_node(self, root, res_child):
children = root["children"]
root["childes"] = []
for each in children:
for x in res_child:
if x["id"] == each:
x["childes"] = []
if len(x["children"]) > 0:
self.get_node(x, res_child)
root["childes"].append(self.__remove_oid(x))
def add_node(self, uid, pid, name, icon=None):
node = self.new_node(uid, name, pid, icon)
res_in = self.__mongo.insert(self.__collection, node.copy())
res_up = self.__mongo.update(self.__collection, {"id": pid}, "$push", {"children": node["id"]})
if res_up and res_in:
return config.build_response("add_node", node, 200)
else:
return config.build_response("add_node", "", 300)
def rename_node(self, sid, name, icon="mif-folder"):
res = self.__mongo.search_by_kv_pair(self.__collection, {"id": sid})
if len(res) > 0:
if res[0]["name"] != "root":
res_up = self.__mongo.update(self.__collection, {"id": sid}, "$set", {"name": name, "icon": icon})
if res_up:
return config.build_response("rename_node", "", 200)
else:
return config.build_response("rename_node", "", 300)
else:
return config.build_response("rename_node", 'can"t rename root', 300)
else:
return config.build_response("rename_node", 'node doesn"t find', 300)
def del_node(self, uid, sid):
try:
res_self = self.__mongo.search_by_kv_pair(self.__collection, {"uid": uid, "id": sid})
if res_self[0].get("pid"):
if self.__mongo.exist(self.__collection, {"id": res_self[0]["pid"]}):
self.__mongo.update(self.__collection, {"id": res_self[0]["pid"]}, "$pull", {"children": sid})
index = [0, 0]
self.reload_del(uid, sid, index)
return config.build_response("del_node", {"delete_count": index}, 200)
except:
return config.build_response("del_node", "", 300)
def reload_del(self, uid, sid, index):
res_self = self.__mongo.search_by_kv_pair(self.__collection, {"uid": uid, "id": sid})
op_list = []
data_list = []
children = []
for each in res_self:
op_list.append({"id": each["id"]})
data_list.extend(list(map(lambda item: item["fid"], each["data"])))
children = each["children"]
index[0] = index[0] + 1
index[1] = index[1] + len(data_list)
self.__mongo.bulk_operation(self.__collection, op_list, "delete")
self.__mongo.delete_files(self.__file_set, data_list)
for each in children:
self.reload_del(uid, each, index)
def add_file(self, uni_id, f_type, description, sid):
tmp_path = config.get_tmp_by_type(f_type) + "\\" + uni_id + "\\"
file_list = [(tmp_path + i) for i in os.listdir(tmp_path)]
descriptions = json.loads(description)
rsp = []
for i, f_path in enumerate(file_list):
if os.path.isfile(f_path):
n_file = self.new_file(os.path.split(f_path)[1], f_path, f_type, str(descriptions[f_path]))
fid = self.__mongo.insert_singlefile_by_path(self.__file_set, f_path, n_file)
if fid and self.__mongo.exist(self.__collection, {"id": sid}):
os.remove(f_path)
n_file["fid"] = fid
res = self.__mongo.update(self.__collection, {"id": sid}, "$push", {"data": n_file})
if res.modified_count == 1:
rsp.append(n_file)
else:
rsp.append({"fid": fid, "status": False})
else:
rsp.append({"fid": "", "status": False})
else:
rsp.append({"fid": "", "status": False})
os.rmdir(tmp_path)
return config.build_response("add_file", rsp, 200)
def del_file(self,
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
管理系统是一种通过计算机技术实现的用于组织、监控和控制各种活动的软件系统。这些系统通常被设计用来提高效率、减少错误、加强安全性,同时提供数据和信息支持。以下是一些常见类型的管理系统: 学校管理系统: 用于学校或教育机构的学生信息、教职员工信息、课程管理、成绩记录、考勤管理等。学校管理系统帮助提高学校的组织效率和信息管理水平。 人力资源管理系统(HRM): 用于处理组织内的人事信息,包括员工招聘、培训记录、薪资管理、绩效评估等。HRM系统有助于企业更有效地管理人力资源,提高员工的工作效率和满意度。 库存管理系统: 用于追踪和管理商品或原材料的库存。这种系统可以帮助企业避免库存过剩或不足的问题,提高供应链的效率。 客户关系管理系统(CRM): 用于管理与客户之间的关系,包括客户信息、沟通记录、销售机会跟踪等。CRM系统有助于企业更好地理解客户需求,提高客户满意度和保留率。 医院管理系统: 用于管理医院或医疗机构的患者信息、医生排班、药品库存等。这种系统可以提高医疗服务的质量和效率。 财务管理系统: 用于记录和管理组织的财务信息,包括会计凭证、财务报表、预算管理等。财务管理系统
资源推荐
资源详情
资源评论
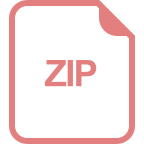
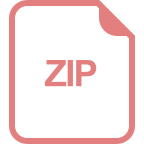
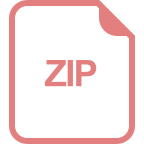
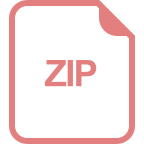
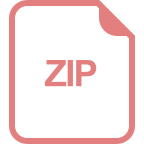
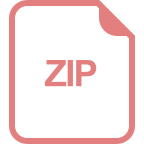
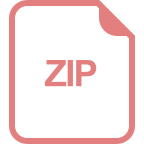
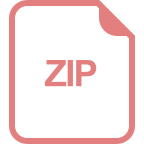
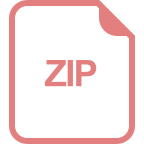
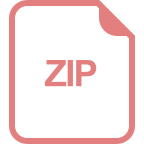
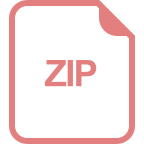
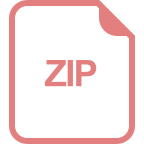
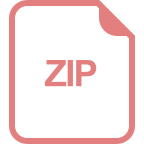
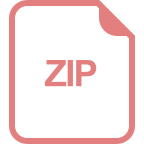
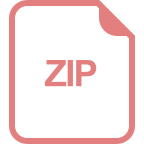
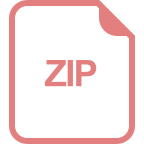
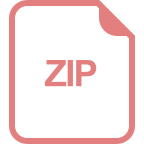
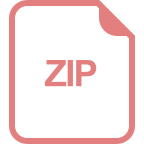
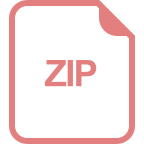
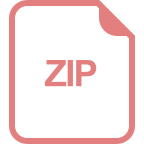
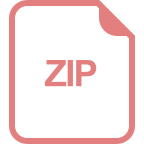
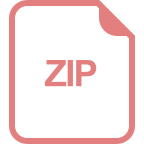
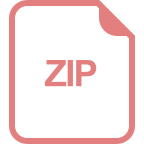
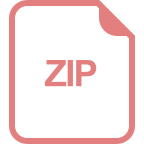
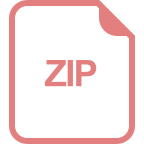
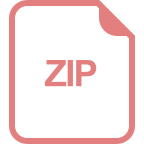
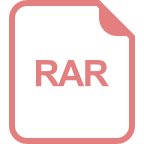
收起资源包目录



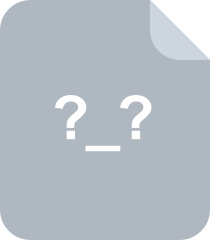

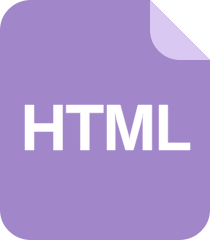
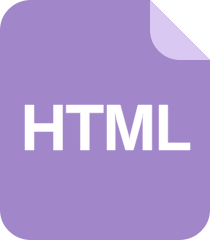
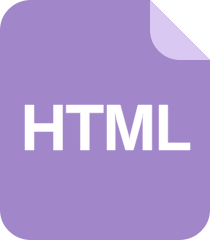

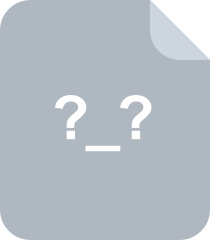
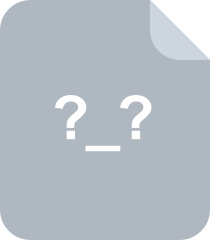
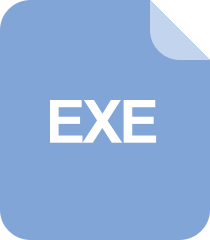

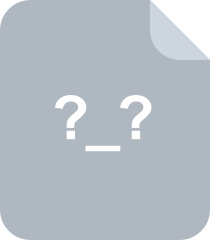

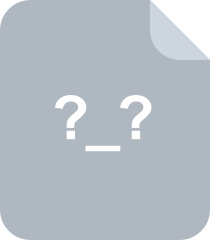
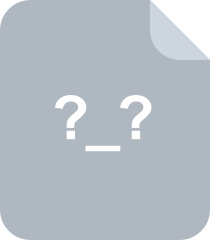
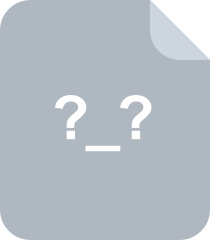
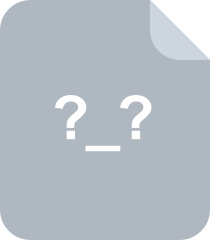
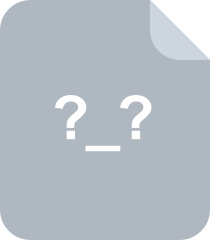
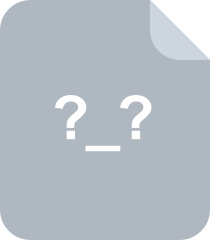
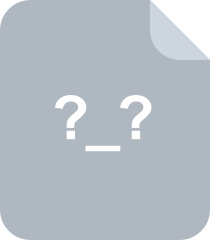
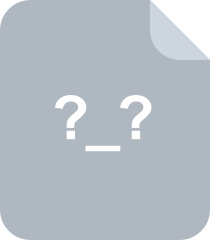

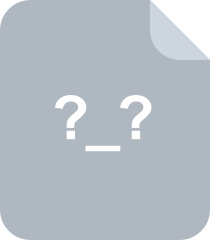
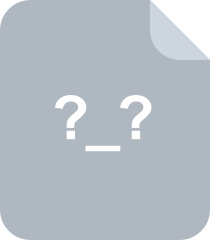
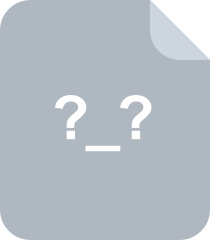
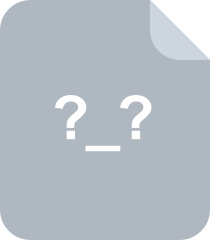

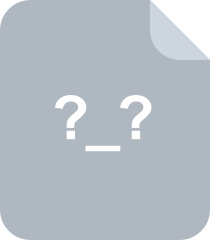
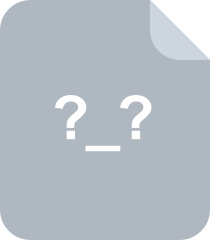
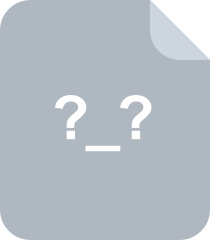
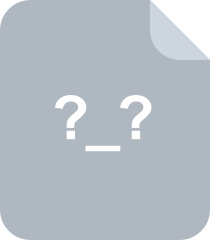
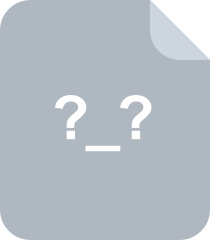
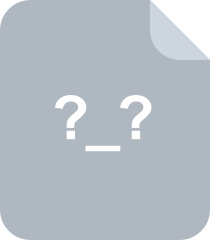
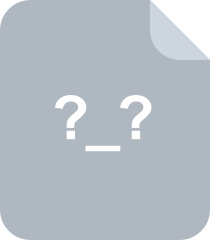
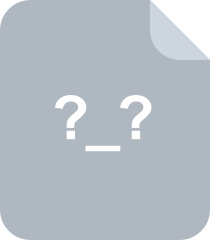
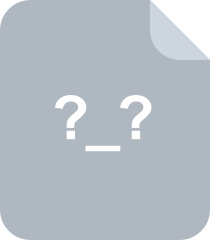

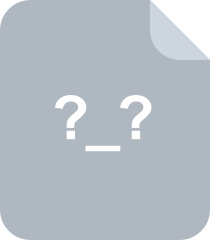
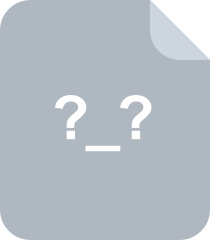
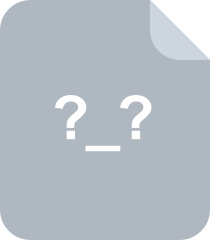
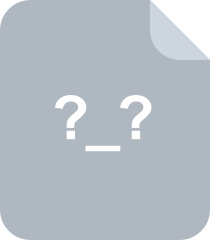

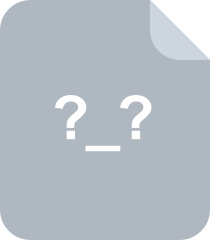


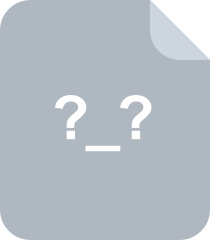
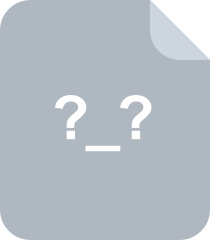

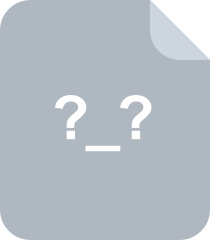
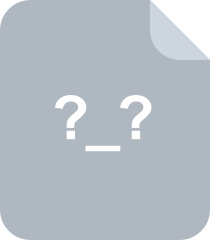
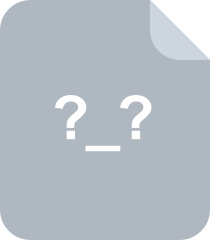

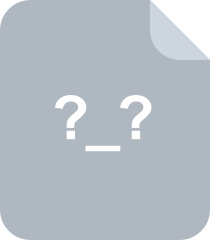
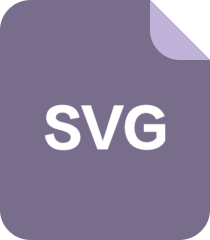
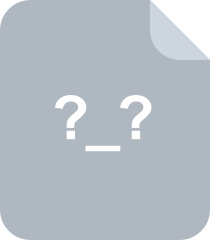
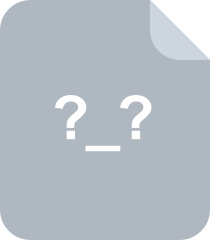
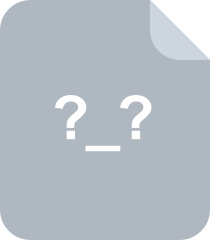
共 44 条
- 1
资源评论
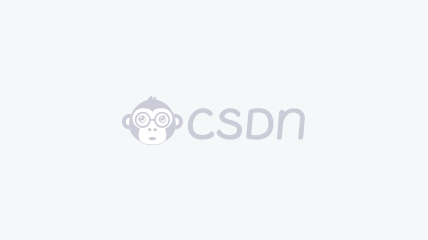

JJJ69
- 粉丝: 6350
- 资源: 5918
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

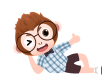
最新资源
- Chrome代理 switchyOmega
- GVC-全球价值链参与地位指数,基于ICIO表,(Wang等 2017a)计算方法
- 易语言ADS指纹浏览器管理工具
- 易语言奇易模块5.3.6
- cad定制家具平面图工具-(FG)门板覆盖柜体
- asp.net 原生js代码及HTML实现多文件分片上传功能(自定义上传文件大小、文件上传类型)
- whl@pip install pyaudio ERROR: Failed building wheel for pyaudio
- Constantsfd密钥和权限集合.kt
- 基于Java的财务报销管理系统后端开发源码
- 基于Python核心技术的cola项目设计源码介绍
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


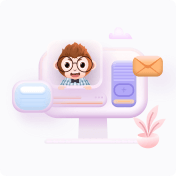
安全验证
文档复制为VIP权益,开通VIP直接复制
