# [<img src="https://khan.github.io/KaTeX/katex-logo.svg" width="130" alt="KaTeX">](https://khan.github.io/KaTeX/)
[](https://travis-ci.org/Khan/KaTeX)
[](https://codecov.io/gh/Khan/KaTeX)
[](https://gitter.im/Khan/KaTeX?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
KaTeX is a fast, easy-to-use JavaScript library for TeX math rendering on the web.
* **Fast:** KaTeX renders its math synchronously and doesn't need to reflow the page. See how it compares to a competitor in [this speed test](http://www.intmath.com/cg5/katex-mathjax-comparison.php).
* **Print quality:** KaTeX’s layout is based on Donald Knuth’s TeX, the gold standard for math typesetting.
* **Self contained:** KaTeX has no dependencies and can easily be bundled with your website resources.
* **Server side rendering:** KaTeX produces the same output regardless of browser or environment, so you can pre-render expressions using Node.js and send them as plain HTML.
KaTeX supports all major browsers, including Chrome, Safari, Firefox, Opera, Edge, and IE 9 - IE 11. More information can be found on the [list of supported commands](https://khan.github.io/KaTeX/function-support.html) and on the [wiki](https://github.com/khan/katex/wiki).
## Usage
You can [download KaTeX](https://github.com/khan/katex/releases) and host it on your server or include the `katex.min.js` and `katex.min.css` files on your page directly from a CDN:
```html
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/KaTeX/0.9.0/katex.min.css" integrity="sha384-TEMocfGvRuD1rIAacqrknm5BQZ7W7uWitoih+jMNFXQIbNl16bO8OZmylH/Vi/Ei" crossorigin="anonymous">
<script src="https://cdnjs.cloudflare.com/ajax/libs/KaTeX/0.9.0/katex.min.js" integrity="sha384-jmxIlussZWB7qCuB+PgKG1uLjjxbVVIayPJwi6cG6Zb4YKq0JIw+OMnkkEC7kYCq" crossorigin="anonymous"></script>
```
#### In-browser rendering
Call `katex.render` with a TeX expression and a DOM element to render into:
```js
katex.render("c = \\pm\\sqrt{a^2 + b^2}", element);
```
If KaTeX can't parse the expression, it throws a `katex.ParseError` error.
#### Server side rendering or rendering to a string
To generate HTML on the server or to generate an HTML string of the rendered math, you can use `katex.renderToString`:
```js
var html = katex.renderToString("c = \\pm\\sqrt{a^2 + b^2}");
// '<span class="katex">...</span>'
```
Make sure to include the CSS and font files, but there is no need to include the JavaScript. Like `render`, `renderToString` throws if it can't parse the expression.
#### Security
Any HTML generated by KaTeX *should* be safe from `<script>` or other code
injection attacks.
(See `maxSize` below for preventing large width/height visual affronts.)
Of course, it is always a good idea to sanitize the HTML, though you will need
a rather generous whitelist (including some of SVG and MathML) to support
all of KaTeX.
#### Handling errors
If KaTeX encounters an error (invalid or unsupported LaTeX), then it will
throw an exception of type `katex.ParseError`. The message in this error
includes some of the LaTeX source code, so needs to be escaped if you want
to render it to HTML. In particular, you should convert `&`, `<`, `>`
characters to `&`, `<`, `>` (e.g., using `_.escape`)
before including either LaTeX source code or exception messages in your
HTML/DOM. (Failure to escape in this way makes a `<script>` injection
attack possible if your LaTeX source is untrusted.)
#### Rendering options
You can provide an object of options as the last argument to `katex.render` and `katex.renderToString`. Available options are:
- `displayMode`: `boolean`. If `true` the math will be rendered in display mode, which will put the math in display style (so `\int` and `\sum` are large, for example), and will center the math on the page on its own line. If `false` the math will be rendered in inline mode. (default: `false`)
- `throwOnError`: `boolean`. If `true`, KaTeX will throw a `ParseError` when it encounters an unsupported command. If `false`, KaTeX will render the unsupported command as text in the color given by `errorColor`. (default: `true`)
- `errorColor`: `string`. A color string given in the format `"#XXX"` or `"#XXXXXX"`. This option determines the color which unsupported commands are rendered in. (default: `#cc0000`)
- `macros`: `object`. A collection of custom macros. Each macro is a property with a name like `\name` (written `"\\name"` in JavaScript) which maps to a string that describes the expansion of the macro. Single-character keys can also be included in which case the character will be redefined as the given macro (similar to TeX active characters).
- `colorIsTextColor`: `boolean`. If `true`, `\color` will work like LaTeX's `\textcolor`, and take two arguments (e.g., `\color{blue}{hello}`), which restores the old behavior of KaTeX (pre-0.8.0). If `false` (the default), `\color` will work like LaTeX's `\color`, and take one argument (e.g., `\color{blue}hello`). In both cases, `\textcolor` works as in LaTeX (e.g., `\textcolor{blue}{hello}`).
- `maxSize`: `number`. If non-zero, all user-specified sizes, e.g. in `\rule{500em}{500em}`, will be capped to `maxSize` ems. Otherwise, users can make elements and spaces arbitrarily large (the default behavior).
For example:
```js
katex.render("c = \\pm\\sqrt{a^2 + b^2}\\in\\RR", element, {
displayMode: true,
macros: {
"\\RR": "\\mathbb{R}"
}
});
```
#### Automatic rendering of math on a page
Math on the page can be automatically rendered using the auto-render extension. See [the Auto-render README](contrib/auto-render/README.md) for more information.
#### Font size and lengths
By default, KaTeX math is rendered in a 1.21× larger font than the surrounding
context, which makes super- and subscripts easier to read. You can control
this using CSS, for example:
```css
.katex { font-size: 1.1em; }
```
KaTeX supports all TeX units, including absolute units like `cm` and `in`.
Absolute units are currently scaled relative to the default TeX font size of
10pt, so that `\kern1cm` produces the same results as `\kern2.845275em`.
As a result, relative and absolute units are both uniformly scaled relative
to LaTeX with a 10pt font; for example, the rectangle `\rule{1cm}{1em}` has
the same aspect ratio in KaTeX as in LaTeX. However, because most browsers
default to a larger font size, this typically means that a 1cm kern in KaTeX
will appear larger than 1cm in browser units.
### Common Issues
- Many Markdown preprocessors, such as the one that Jekyll and GitHub Pages use,
have a "smart quotes" feature. This changes `'` to `’` which is an issue for
math containing primes, e.g. `f'`. This can be worked around by defining a
single character macro which changes them back, e.g. `{"’", "'"}`.
- KaTeX follows LaTeX's rendering of `aligned` and `matrix` environments unlike
MathJax. When displaying fractions one above another in these vertical
layouts there may not be enough space between rows for people who are used to
MathJax's rendering. The distance between rows can be adjusted by using
`\\[0.1em]` instead of the standard line separator distance.
- KaTeX does not support the `align` environment because LaTeX doesn't support
`align` in math mode. The `aligned` environment offers the same functionality
but in math mode, so use that instead or define a macro that maps `align` to
`aligned`.
## Libraries
### Angular2+
- [ng-katex](https://github.com/garciparedes/ng-katex) Angular module to write beautiful math expressions with TeX syntax boosted by KaTeX library
### Ruby
- [katex-ruby](https://github.com/glebm/katex-ruby) Provides server-side rendering and integration with popular Ruby web frameworks (Rails

程序员张小妍
- 粉丝: 1w+
- 资源: 3582
最新资源
- 储能变流器PQ并网和VF离网 含学习资料 一、图1、图2、图3为储能变流器PQ控制能量可实现能量双向流动 1储能电压外环,电流内环 2逆变器采用PQ控制,可实现能量双向流动 3仿真实现的内
- 机械设计双工位PCM焊接机sw18非常好的设计图纸100%好用.zip
- 基于java+springboot+vue+mysql的仓库管理系统 源码+数据库+论文(高分毕业设计)
- 最新版Unity Racing Game Starter Kit2
- Comsol仿真-相场法多晶铁电体介电击穿模拟 复现参考文献:Revisiting the Dielectric Breakdown in a Polycrystalline Ferroelectr
- 基于国内某高校校园一卡通系统一个月的运行数据,使用数据分析和建模的方法,挖掘数据中所蕴含的信息,分析学生在校园内的学习生活行为+python项目源码+文档说明
- Comsol仿真-相场法多晶铁电体介电击穿模拟 复现参考文献:Revisiting the Dielectric Breakdown in a Polycrystalline Ferroelectr
- 基于永磁同步电机旋转高频信号注入法零低速无位置控制仿真 1相比高频方波信号注入法,旋转高频信号注入法噪声更小损耗更低 2该模型注入1000Hz旋转高频电压信号到电机中用于产生激励电流,在低速1
- 基于永磁同步电机旋转高频信号注入法零低速无位置控制仿真 1相比高频方波信号注入法,旋转高频信号注入法噪声更小损耗更低 2该模型注入1000Hz旋转高频电压信号到电机中用于产生激励电流,在低速1
- 基于java+springboot+vue+mysql的校园二手物品交易平台 源码+数据库+论文(高分毕业设计)
- 中国生态功能保护区shp数据
- html+css+js网页设计 美食 好厨艺西餐美食企业网站模板6个页面
- STM32F407单片机上使用HAL库实现can总线的接收和发送
- STM32F767 UCOS开发手册-V1.0.zip
- 光伏蓄电池单相并网模型 带参考文件,模型说明文件 模型内容: 1.光伏+MPPT+boost升压电路+桥式逆变 2.电池模型+电池控制器+直流母线控制 3.稳定交流负载+功率控制器+pwm调制 仿真
- 一个加载UCOSII嵌入式操作系统的STM32项目模板.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


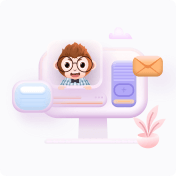