package com.dongliu.service.impl;
import com.dongliu.dao.BookDao;
import com.dongliu.dao.LentRecordDao;
import com.dongliu.dao.UserDao;
import com.dongliu.domain.Book;
import com.dongliu.domain.LentRecord;
import com.dongliu.domain.User;
import com.dongliu.service.LentRecordService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.Date;
import java.util.List;
@Service
public class LentRecordServiceImpl implements LentRecordService {
@Autowired
private LentRecordDao lentRecordDao;
@Autowired
private BookDao bookDao;
@Autowired
private UserDao userDao;
public boolean save(LentRecord lentRecord) {
return lentRecordDao.save(lentRecord) > 0;
}
public boolean update(LentRecord lentRecord) {
return lentRecordDao.update(lentRecord) > 0;
}
public boolean delete(Integer lentId) {
return lentRecordDao.delete(lentId) > 0;
}
public List<LentRecord> getByUsername(String username) {
return lentRecordDao.getByUsername(username);
}
public LentRecord getByLentId(Integer lentId){
return lentRecordDao.getByLentId(lentId);
}
public List<LentRecord> getAll() {
return lentRecordDao.getAll();
}
public int getCount(){
return lentRecordDao.getCount();
}
public boolean createRecord(Book book, User user){
try {
LentRecord lentRecord = new LentRecord();
int lentNumber = book.getLentNumber();
lentNumber++;
book.setLentNumber(lentNumber);
bookDao.update(book);
int lentNumber2 = user.getLentNumber();
lentNumber2++;
user.setLentNumber(lentNumber2);
userDao.updateById(user);
lentRecord.setBookId(book.getId());
lentRecord.setBookName(book.getName());
lentRecord.setUsername(user.getUsername());
lentRecord.setName(user.getName());
lentRecord.setLentDate(new Date());
return lentRecordDao.save(lentRecord) > 0;
}catch (Exception e){
System.out.println("创建借书记录出错!");
}
return false;
}
public List<LentRecord> getAllByUsername(String username) {
return lentRecordDao.getAllByUsername(username);
}
public List<LentRecord> getNotReturnByUsername(String username) {
return lentRecordDao.getNotReturnByUsername(username);
}
public LentRecord returnBook(LentRecord lentRecord) {
Date date = new Date();
// 还书要扣钱
int days = (int)Math.ceil(((date.getTime() - lentRecord.getLentDate().getTime()) / (1.0*1000*3600*24)));
lentRecord.setReturnDate(date);
User user = userDao.getByUsername(lentRecord.getUsername());
float bill = (float) (0.1*days);
float money = (float) (user.getMoney()-bill);
user.setMoney(money);
// 已借数量--
user.setLentNumber(user.getLentNumber()-1);
userDao.update(user);
lentRecord.setBill(bill);
lentRecordDao.update(lentRecord);
// 书已借数量--
Book book = bookDao.getById(lentRecord.getBookId());
book.setLentNumber(book.getLentNumber()-1);
bookDao.update(book);
boolean flag = lentRecordDao.update(lentRecord) > 0;
return flag ? lentRecord : null;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于springboot+vue的图书管理系统源码+数据库.zip高分通过项目,已获老师指导。本项目是一套基于springboot+vue的图书管理系统系统,主要针对计算机相关专业的正在做毕设的学生和需要项目实战练习的Java学习者。也可作为课程设计、期末大作业 包含:项目源码、数据库脚本、软件工具、项目说明等,该项目可以直接作为毕设使用。 项目都经过严格调试,确保可以运行! 技术实现 前端:vue 后台框架:SpringBoot 数据库:MySQL 开发环境:JDK、IDEA 该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值。该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值。该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值。该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值。该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值。
资源推荐
资源详情
资源评论
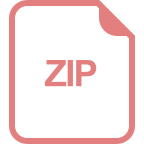
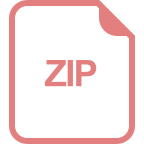
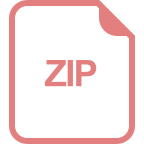
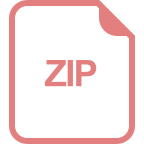
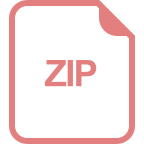
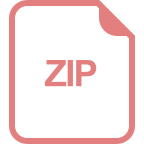
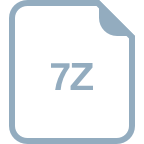
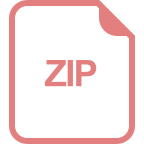
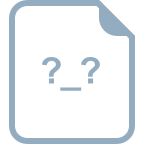
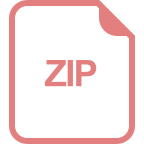
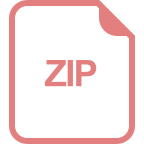
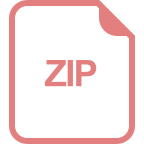
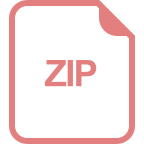
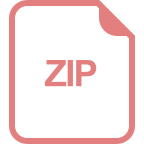
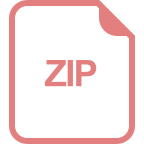
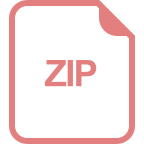
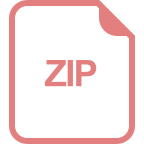
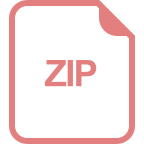
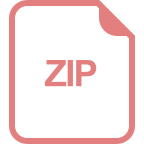
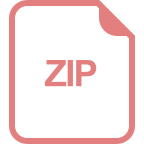
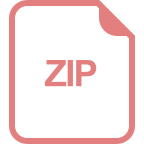
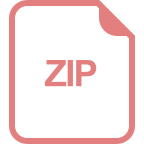
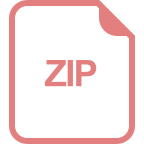
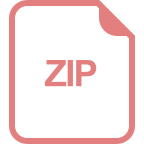
收起资源包目录

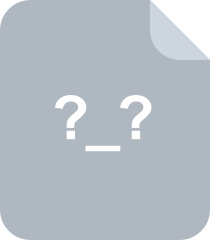
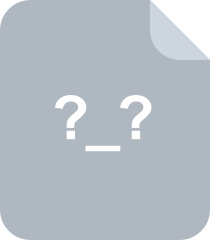
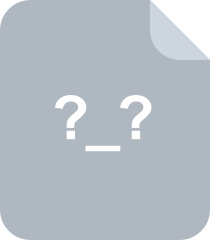
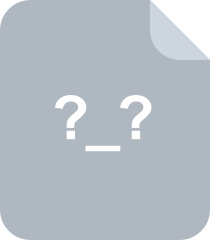
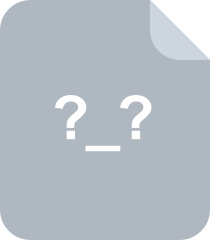
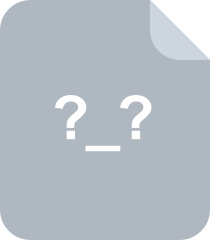
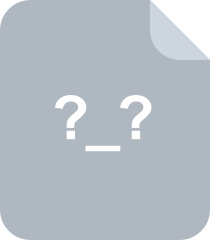
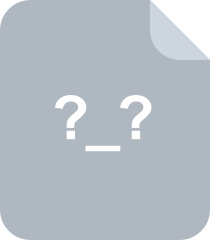
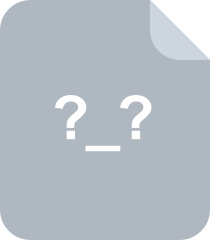
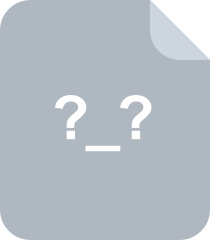
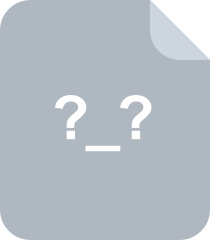
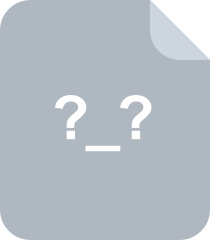
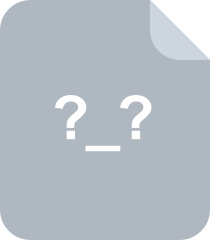
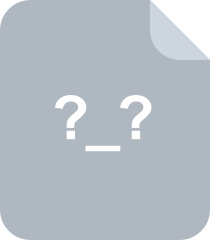
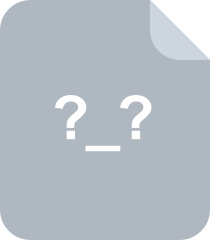
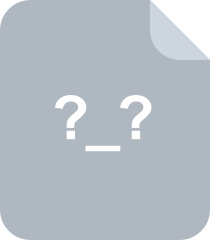
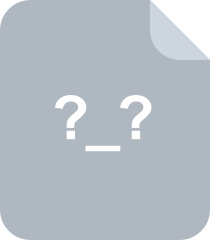
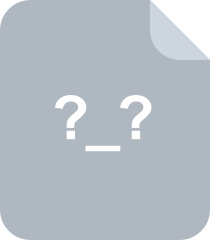
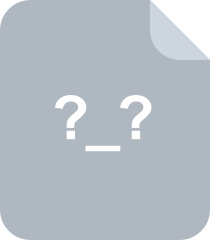
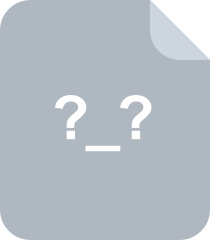
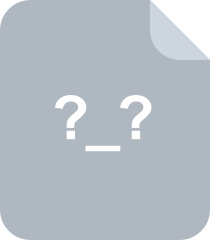
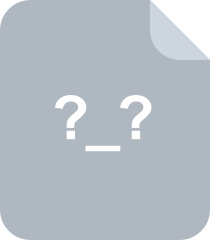
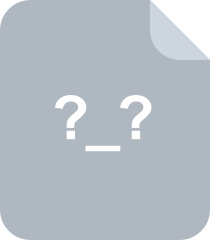
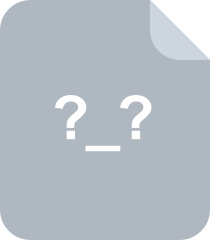
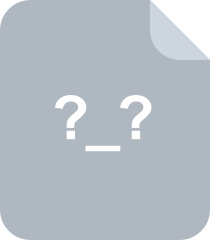
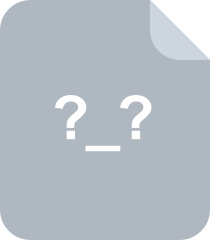
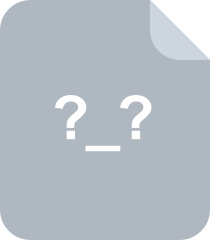
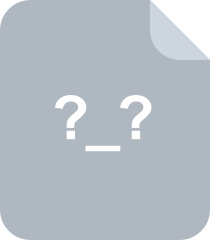
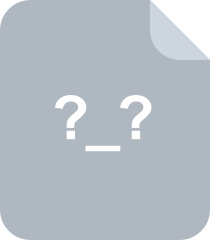
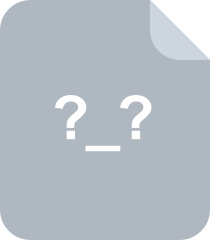
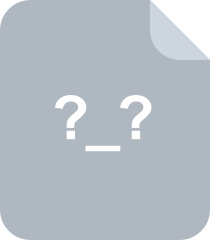
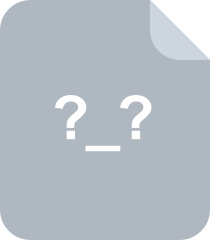
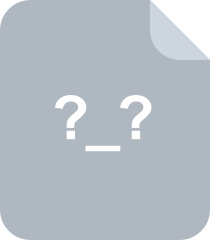
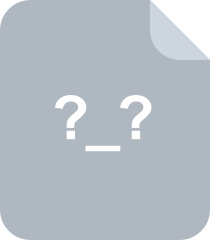
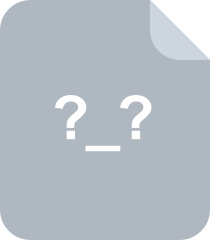
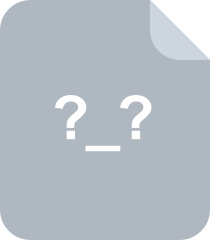
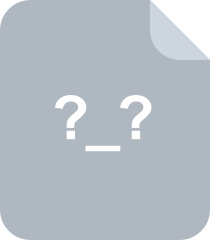
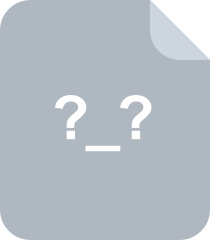
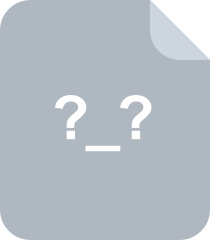
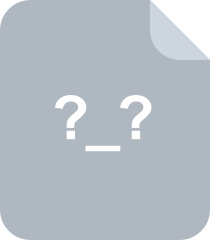
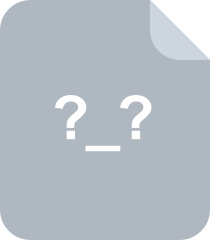
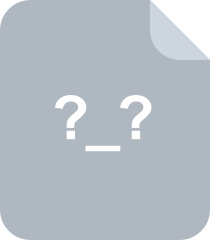
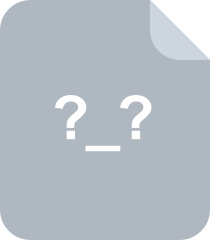
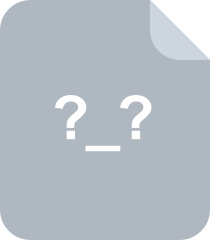
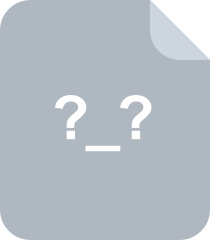
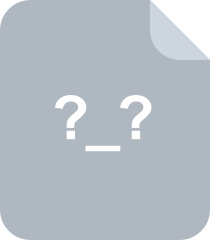
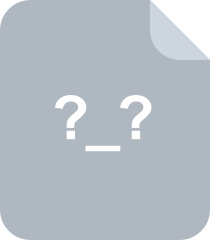
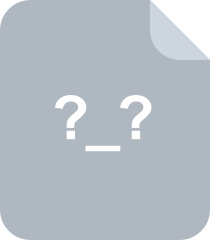
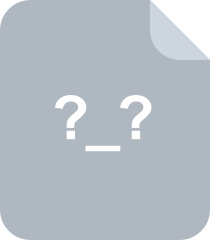
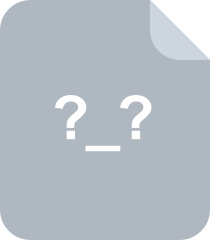
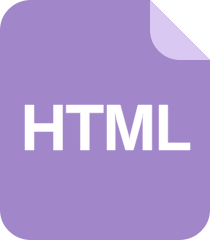
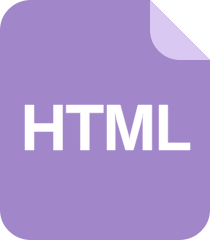
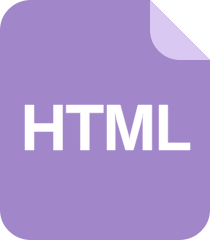
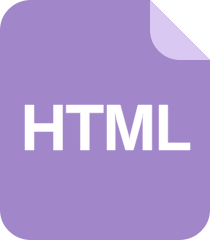
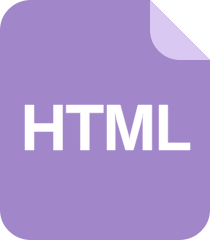
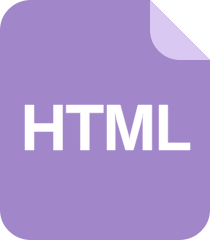
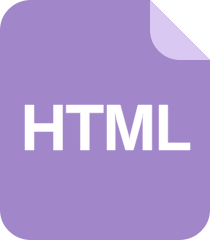
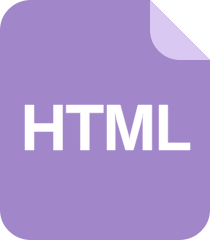
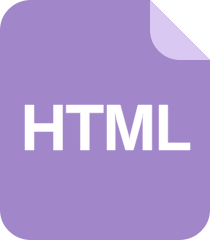
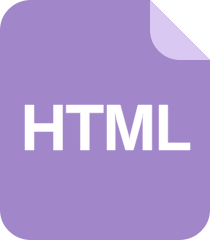
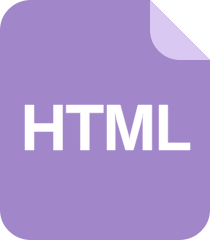
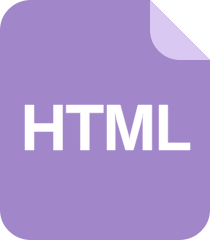
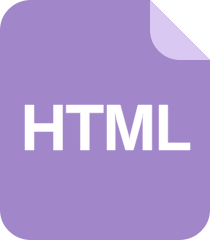
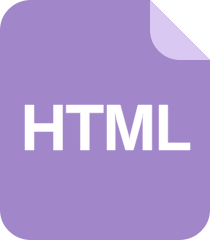
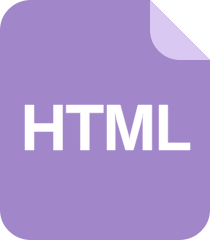
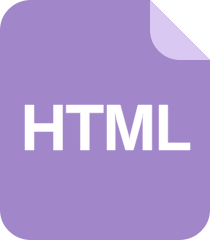
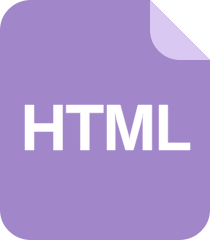
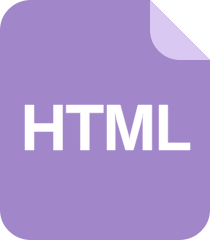
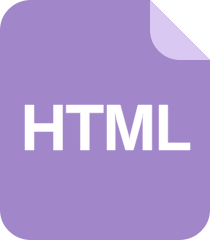
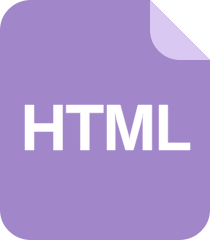
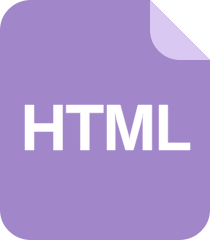
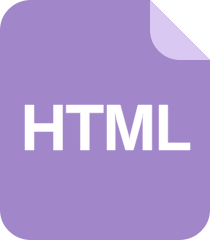
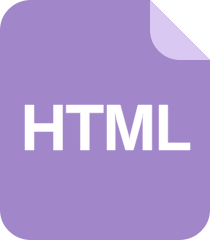
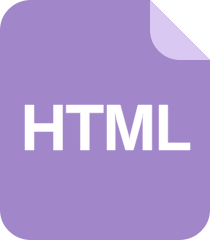
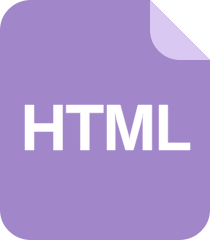
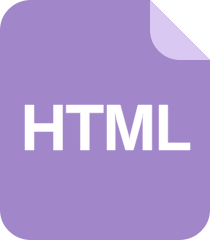
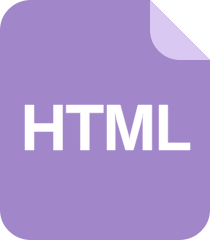
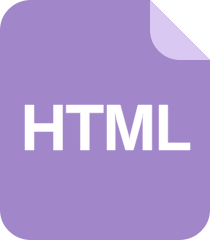
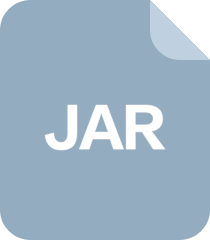
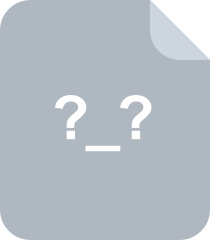
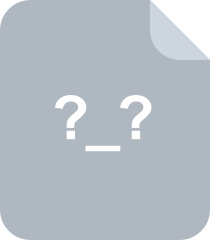
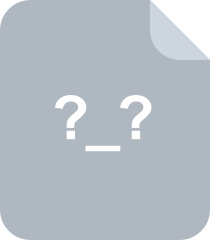
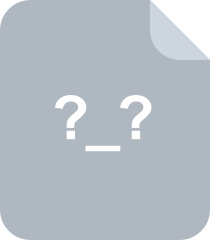
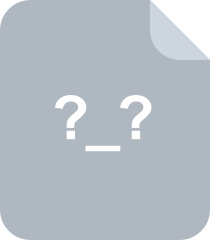
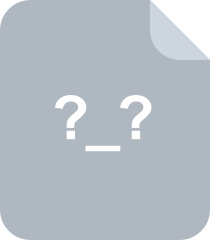
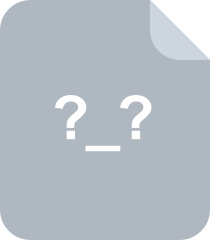
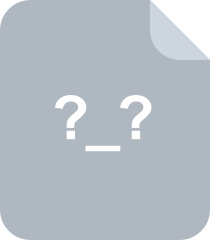
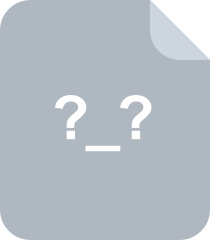
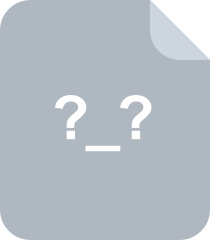
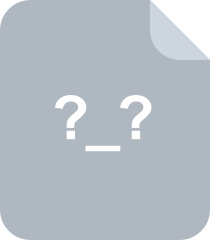
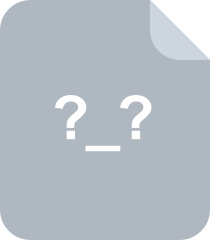
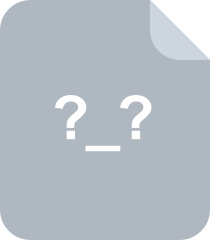
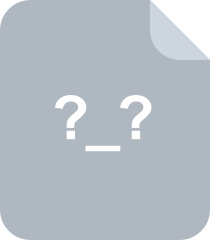
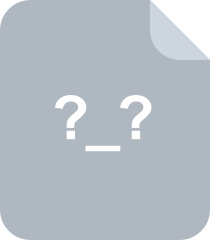
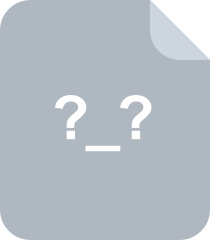
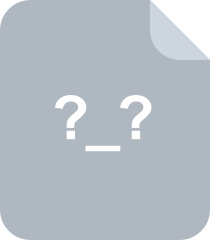
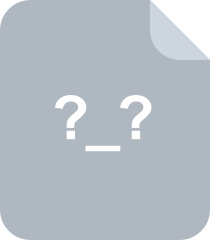
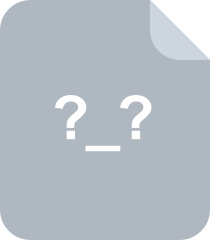
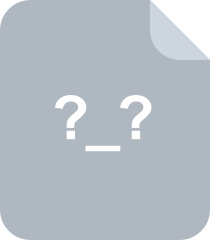
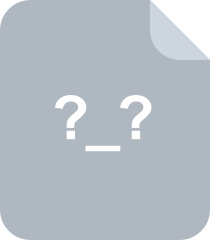
共 286 条
- 1
- 2
- 3
资源评论
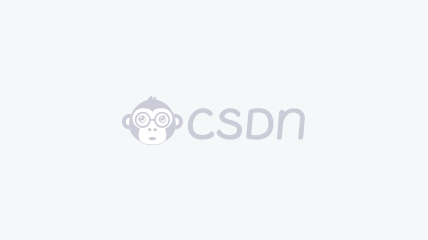
- m0_750287012023-12-09资源值得借鉴的内容很多,那就浅学一下吧,值得下载!
- Serei6232023-01-20资源内容总结的很到位,内容详实,很受用,学到了~

程序员张小妍
- 粉丝: 1w+
- 资源: 3474
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

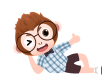
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


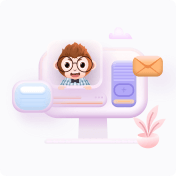
安全验证
文档复制为VIP权益,开通VIP直接复制
