package com.nx.controller;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.nx.model.*;
import com.nx.service.BookService;
import org.apache.ibatis.annotations.Param;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.http.HttpRequest;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.commons.CommonsMultipartFile;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import java.io.File;
import java.io.IOException;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.UUID;
@Controller
@RequestMapping("/book")
public class BookController {
@Autowired
@Qualifier("bookServiceImpl")
private BookService bookService;
/*@RequestMapping("/toMain")
public String toMain(Model model){
return "main";
}*/
@RequestMapping("/Main")
public String toMain(Model model,@RequestParam(value = "pageNo",defaultValue = "1") Integer pageNo,@RequestParam(value = "pageSize",defaultValue = "12")Integer pageSize){
List<Book> books = bookService.selectBook();
model.addAttribute("books",books);
/*PageInfo<Book> pageInfo = bookService.selectBook(pageNo,pageSize);*/
List<BookCategory> bookCategories = bookService.selectBookCategory();
model.addAttribute("bookCategories",bookCategories);
/* model.addAttribute("books",pageInfo);*/
if(books.size()==0){
model.addAttribute("msg","暂无书籍");
return "main";
}
return "main";
/*session.setAttribute("username",u_name);
session.setAttribute("password",u_password1);*/
}
@RequestMapping("/toAdd")
public String toAdd(Model model){
List<BookCategory> bookCategories = bookService.selectBookCategory();
model.addAttribute("addCategory",bookCategories);
return "addBook";
}
@RequestMapping("/Add")
public String Add(@RequestParam("bookPhoto") CommonsMultipartFile file, Book book, Model model, HttpServletRequest request) throws IOException, ParseException {
List<BookCategory> bookCategories = bookService.selectBookCategory();
model.addAttribute("bookCategories",bookCategories);
//上传路径保存设置
//String path = request.getServletContext().getRealPath("/upload");
File realPath = new File(request.getServletContext().getRealPath("/images/book"));
if (!realPath.exists()){
realPath.mkdir();
}
//上传文件地址
System.out.println("上传文件保存地址:"+realPath);
System.out.println(request.getServletContext().getRealPath("/images/book"));
String upload=UUID.randomUUID()+file.getOriginalFilename();
//通过CommonsMultipartFile的方法直接写文件(注意这个时候)
file.transferTo(new File(realPath +"/"+upload));
book.setB_photo(upload);
System.out.println(book.getB_photo());
SimpleDateFormat simpleDateFormat=new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date date=simpleDateFormat.parse(simpleDateFormat.format(new Date()));
book.setB_createTime(date);
int insertBook = bookService.insertBook(book);
System.out.println(insertBook);
if(insertBook>0) {
List<Book> books = bookService.selectBook();
model.addAttribute("books", books);
return "redirect:/book/Main";
}
return "redirect:/book/toAdd";
}
@RequestMapping("/query")
public String Query(String b_name,Model model){
List<BookCategory> bookCategories = bookService.selectBookCategory();
model.addAttribute("bookCategories",bookCategories);
List<Book> books=bookService.selectBookAmbiguous(b_name);
if(books.size()==0){
model.addAttribute("msg","暂无此书籍");
return "main";
}
model.addAttribute("books",books);
return "main";
}
@RequestMapping("/Category")
public String QueryByCategory(int c_id,Model model){
List<BookCategory> bookCategories = bookService.selectBookCategory();
model.addAttribute("bookCategories",bookCategories);
List<Book> books=bookService.selectBookByCategory(c_id);
System.out.println(books);
if(books.size()==0){
model.addAttribute("msg","暂无此类书籍");
return "main";
}
model.addAttribute("books",books);
return "main";
}
@RequestMapping("/myQuery")
public String toQuery(String b_name,Model model,HttpSession session){
List<BookCategory> bookCategories = bookService.selectBookCategory();
model.addAttribute("bookCategory",bookCategories);
List<Book> books=bookService.selectMyBookAmbiguous(b_name,(Integer) session.getAttribute("userId"));
if(books.size()==0){
model.addAttribute("msg","暂无此书籍");
return "myShop";
}
model.addAttribute("myBooks",books);
return "myShop";
}
@RequestMapping("/toMyShop")
public String toMyShop(){
return "myShop";
}
@RequestMapping("/myCategory")
public String QueryByMyCategory(Integer b_cid,Integer b_uid,Model model){
List<BookCategory> bookCategories = bookService.selectBookCategory();
model.addAttribute("bookCategory",bookCategories);
List<Book> books=bookService.selectBookByUserIdCategory(b_uid,b_cid);
System.out.println(books);
if(books.size()==0){
model.addAttribute("msg","暂无此类书籍");
return "myShop";
}
model.addAttribute("myBooks",books);
return "myShop";
}
@RequestMapping("/MyShop")
public String MyShop(HttpSession session,Model model){
Integer userId = (Integer) session.getAttribute("userId");
List<BookCategory> bookCategories = bookService.selectBookCategory();
model.addAttribute("bookCategory",bookCategories);
List<Book> books=bookService.selectBookByUserId(userId);
System.out.println(books);
if(books.size()==0){
model.addAttribute("msg","暂无上架书籍");
return "myShop";
}
model.addAttribute("myBooks",books);
return "myShop";
}
@RequestMapping("/bookMessage")
public String toBookMessage(HttpSession session,Model model){
Integer bookMessageId = (Integer) session.getAttribute("bookMessageId");
String bookPossess = (String) session.getAttribute("bookPossess");
Book bookMessage= bookService.selectBookById(bookMessageId);
String bookCategoryName = bookService.selectBookCategoryById(bookMessage.getB_cid());
model.addAttribute("bookMessages",bookMessage);
model.addAttribute("bookUser",bookPossess);
model.addAttribute("bookCategoryName",bookCategoryName);
model.addAttribute("username",session.getAttribute("username"));
List<Comment> comments = bookService.selectBookCommentById(bookMessageId);
model.addAttribute("comments",comments);
return "bookMessage";
}
@RequestMapping("/MyBookMessage")
public String toMyBookMessage(Model model,HttpSession session){
Integer myBookMessageId = (Integer) session.getAttribute("myBookMessageId");
String bookMyPossess = (String) session.getAttribute("bookMyPossess");
B
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。 java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。java毕业设计基于ssm的二手书籍交易管理系统源码+数据库。已获高分通过的项目。
资源推荐
资源详情
资源评论
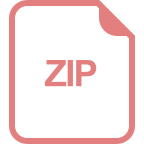
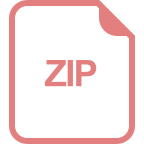
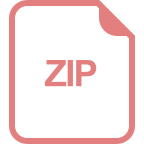
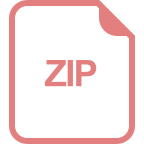
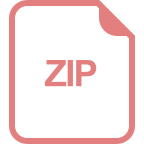
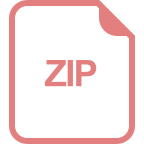
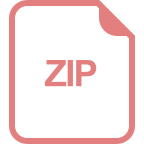
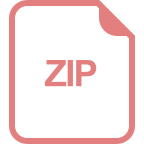
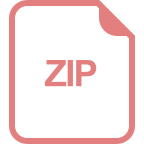
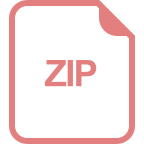
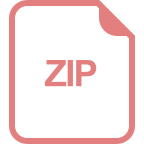
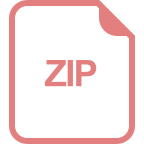
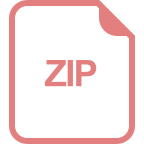
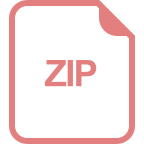
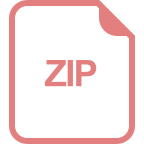
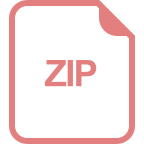
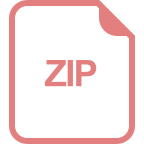
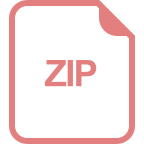
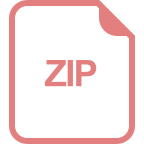
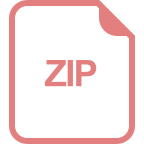
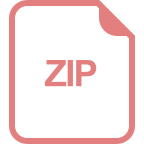
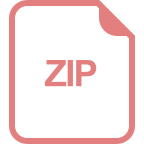
收起资源包目录

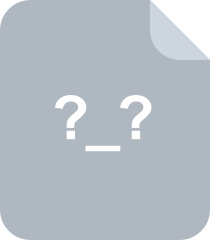
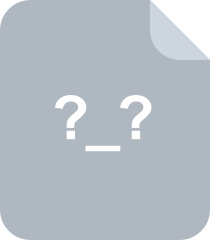
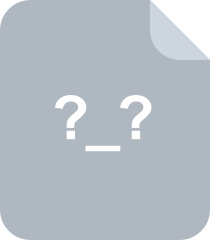
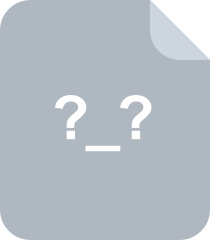
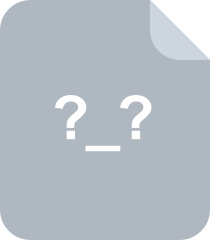
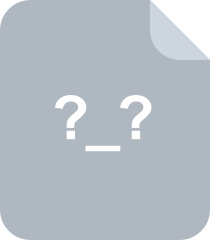
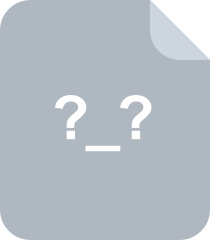
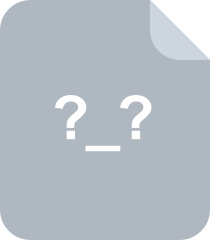
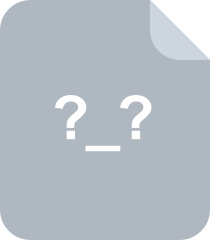
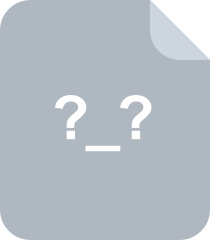
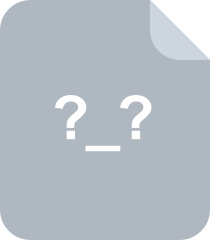
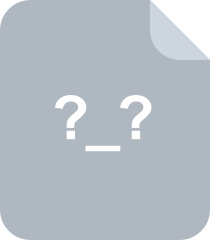
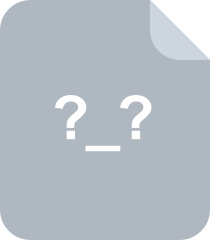
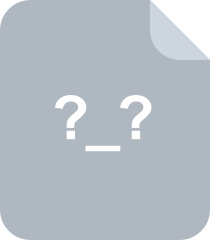
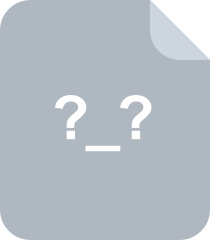
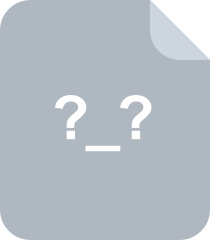
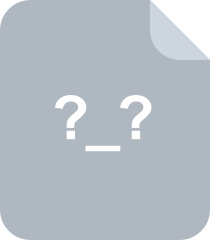
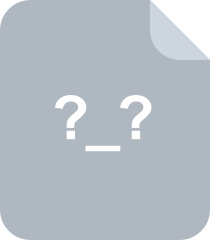
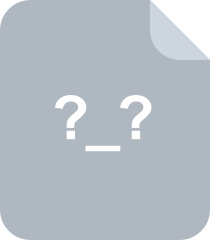
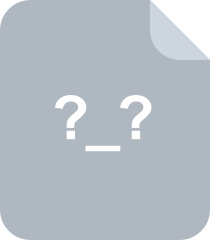
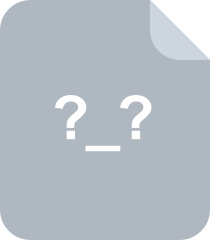
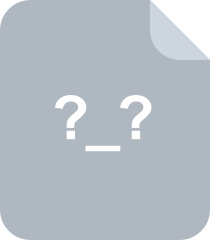
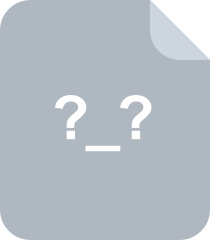
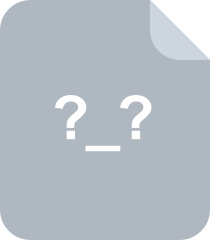
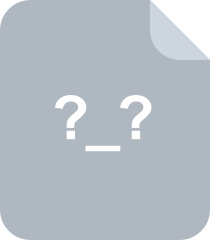
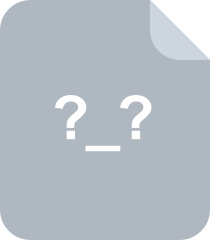
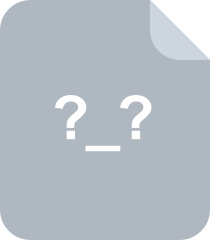
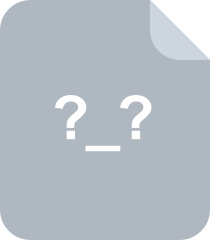
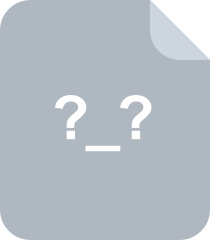
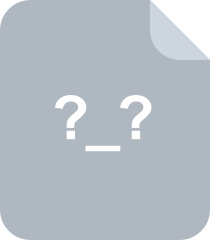
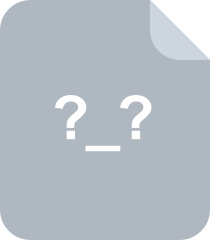
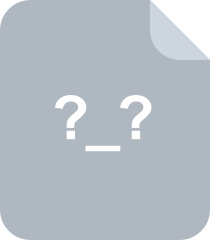
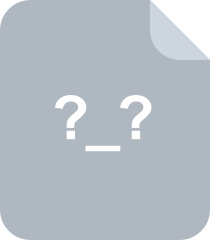
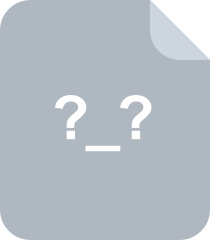
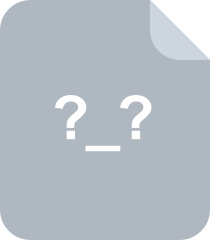
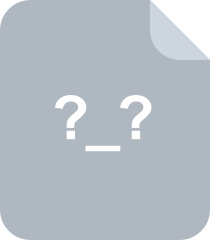
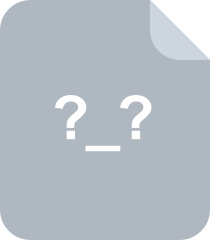
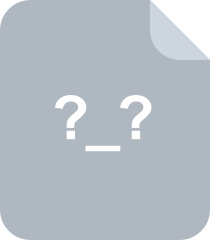
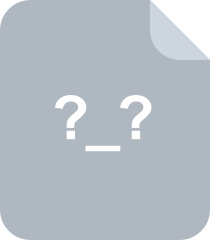
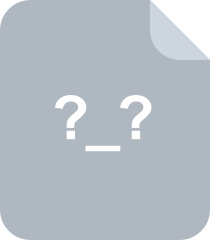
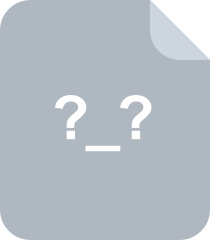
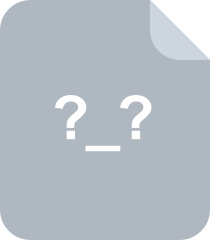
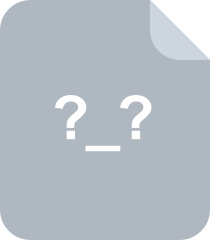
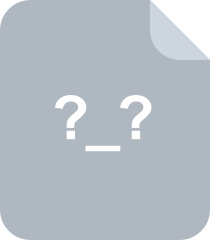
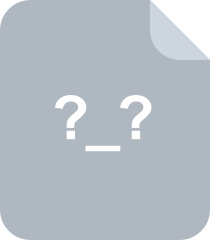
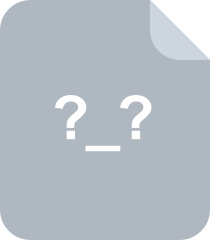
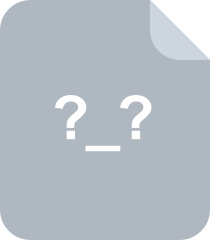
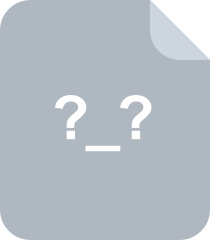
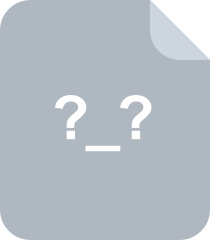
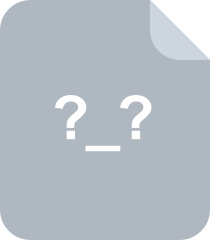
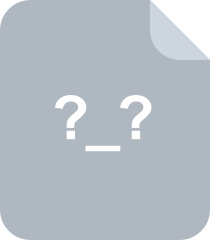
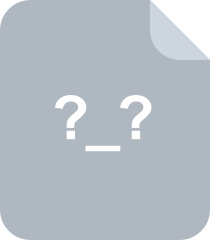
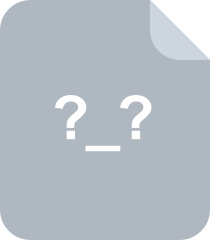
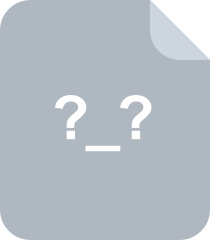
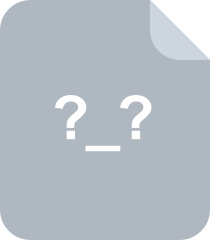
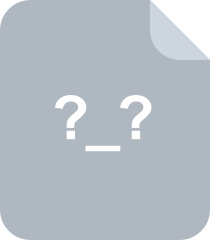
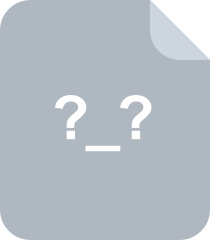
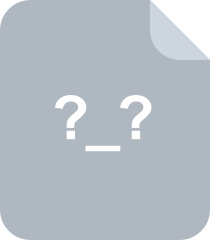
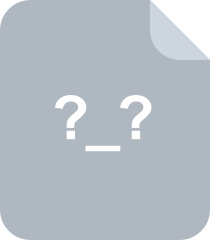
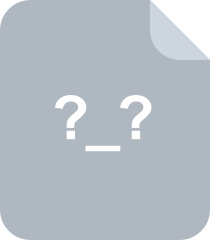
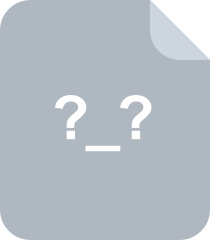
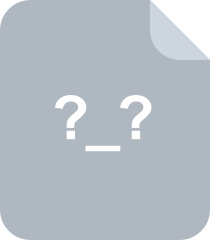
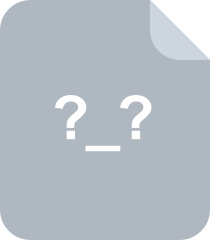
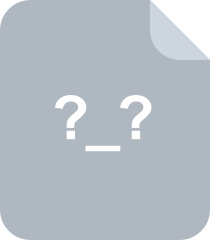
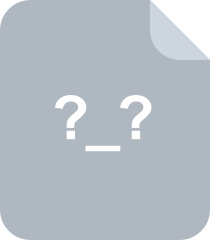
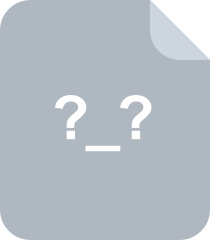
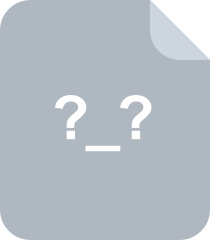
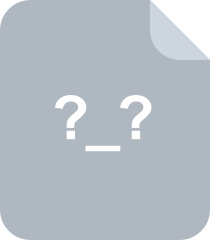
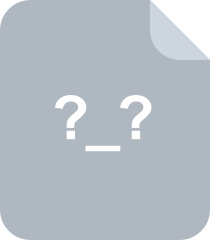
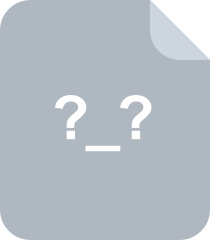
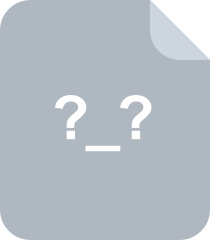
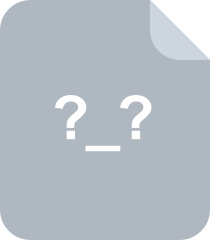
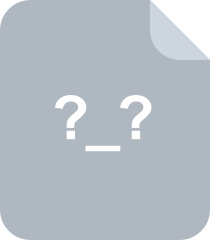
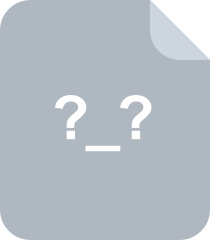
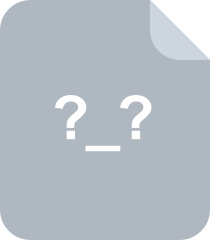
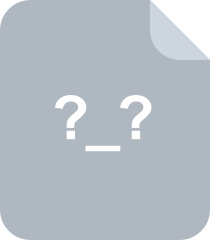
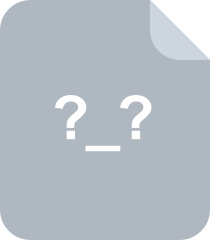
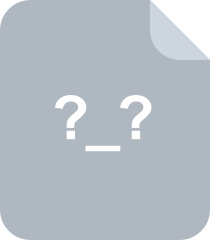
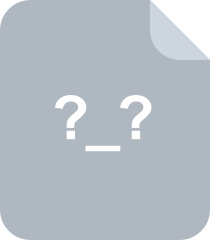
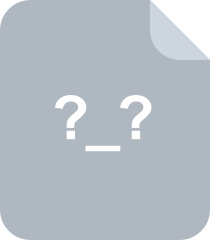
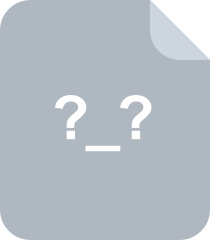
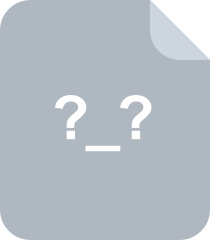
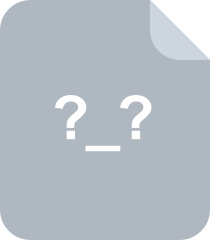
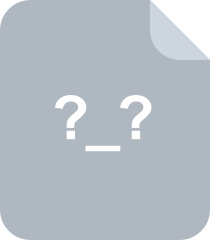
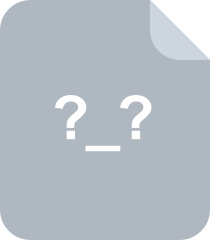
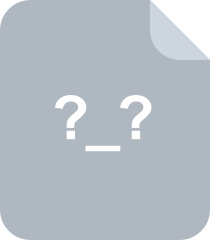
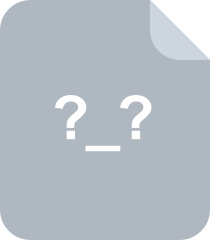
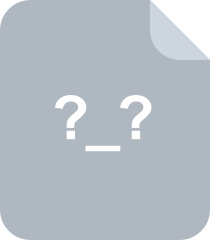
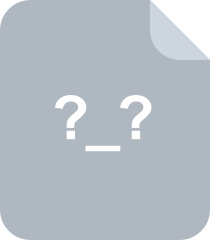
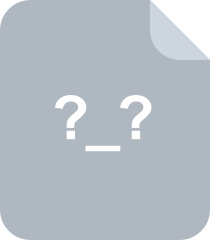
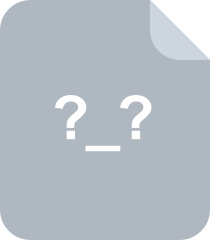
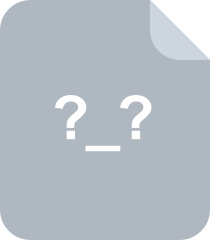
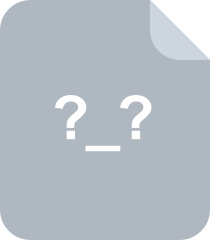
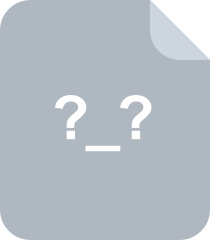
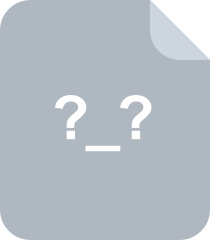
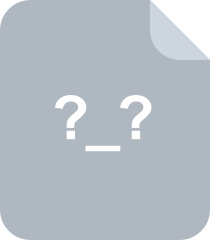
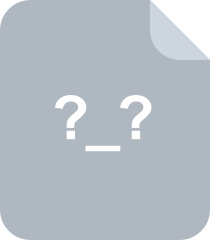
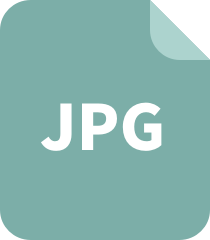
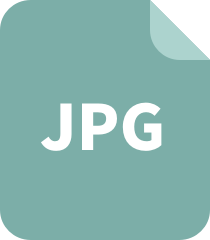
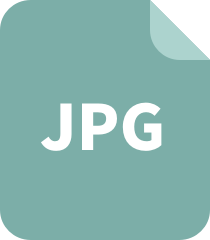
共 220 条
- 1
- 2
- 3

程序员张小妍
- 粉丝: 1w+
- 资源: 3474
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

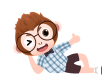
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页