import datetime
import json
from operator import length_hint
import time
from django.conf import settings
from django.shortcuts import render, redirect
from django.contrib import messages
from news.models import Comments, News, User, Category
from django.http import JsonResponse
from django.core.paginator import Paginator # Django内置分页功能模块
from django.core import serializers
import os
import jieba
import numpy as np
import tensorflow as tf
import re
from gensim.models import KeyedVectors
# #加载预训练词向量
# cn_model = KeyedVectors.load_word2vec_format('/Users/lynn/Desktop/mysite/sgns.weibo.word.bz2', binary=False)
# embedding_dim_c = cn_model['山东大学'].shape[0]
# print("评论模型加载完毕")
# n_model = KeyedVectors.load_word2vec_format('/Users/lynn/Desktop/mysite/sgns.sogou.word.bz2', binary=False)
# embedding_dim_n = n_model['山东大学'].shape[0]
# print("新闻模型加载完毕")
# 文本处理(去除不必要的标签)
def remove_tags(text):
clean_text = re.compile(r'<[^>]+>')
return clean_text.sub('', text)
# 用于清理文本
def clean_text(x):
x = str(x)
x.replace('/>', ' /> ')
for punct in "/-'":
x = x.replace(punct, '')
for punct in ' 一…了':
x = x.replace(punct, '')
for punct in '&':
x = x.replace(punct, f' {punct} ')
for punct in '?!.,"#$%\'()*+-/:;<=>@[\\]^_`{|}~丨「」|①④()、|:|' + '“”’':
x = x.replace(punct, '')
return x
def data_process(train_reviews, max_len, cn_model, embedding_dim):
print("评论:" + train_reviews[0])
# 进行分词和tokenize
train_tokenize = []
for text in train_reviews:
text = remove_tags(text)
text = clean_text(text)
cut = jieba.cut(text)
cut_list = [i for i in cut]
for i, word in enumerate(cut_list):
try:
cut_list[i] = cn_model.key_to_index[word]
except KeyError:
cut_list[i] = 0
train_tokenize.append(cut_list)
# 反索引化功能
def reverse_tokens(tokens):
text = ''
for i in tokens:
if i != 0:
text = text + cn_model.index_to_key[i]
else:
text = text + ''
return text
reverse = reverse_tokens(train_tokenize[0])
print("反索引化的文本为:" + reverse)
print("原始文本:" + train_reviews[0])
# 选取频率最高的50000词
num_words = 50000
embedding_matrix = np.zeros((num_words, embedding_dim))
for i in range(num_words):
embedding_matrix[i, :] = cn_model[cn_model.index_to_key[i]]
embedding_matrix = embedding_matrix.astype('float32')
print(np.sum(cn_model[cn_model.index_to_key[333]] == embedding_matrix[333]))
print(embedding_matrix.shape)
# 填充和修剪
train_pad = tf.keras.preprocessing.sequence.pad_sequences(train_tokenize, maxlen=max_len, padding='post', truncating='post')
# 超出50000个词向量的词用0代替
train_pad[train_pad >= num_words] = 0
return train_pad
context={
'user_num':User.objects.count(),
'comment_num':Comments.objects.count(),
'news_num':News.objects.count()
}
def index(request):
# 用户登录
if request.method == "POST":
username = request.POST.get('username', None)
password = request.POST.get('password', None)
if username and password: # 确保用户名和密码都不为空
username = username.strip()
try:
user = User.objects.get(user_name=username)
if user.password == password:
messages.add_message(request, messages.INFO, "登录成功!")
request.session['is_login'] = True
request.session['user_id'] = user.user_id
request.session['user_name'] = user.user_name
request.session['is_admin'] = user.is_admin
request.session['login_time'] = str(datetime.datetime.now())[0:19]
return redirect('/index')
else:
messages.add_message(request, messages.INFO, "密码不正确")
except:
return redirect('/index')
# 新闻显示
physical=[]
economy=[]
entertain=[]
education=[]
technique=[]
policy=[]
news=News.objects.all()
for new in news:
if new.category_id==3:
physical.append(new)
elif new.category_id == 5:
economy.append(new)
elif new.category_id==4:
entertain.append(new)
elif new.category_id==7:
education.append(new)
elif new.category_id==8:
technique.append(new)
elif new.category_id==1:
policy.append(new)
news_show={'physical':physical[:5],'economy':economy[:18],'entertain':entertain[:18],
'education':education[:40], 'technique':technique[:18], 'policy':policy[:18]}
return render(request, 'index.html', news_show)
# 退出登录
def logout(request):
request.session.flush()
messages.add_message(request, messages.INFO, "已退出登录!")
return redirect('/index')
# 类别界面
def newsModule(request, category_id):
newsList=[]
news = News.objects.filter(category_id=category_id).all()
n=0
for new in news:
data=dict()
data['id']=new.news_id
data['title']=new.n_title
data['time']=str(new.n_time)[0:19]
data['content']=new.n_content[0:150]+'...'
newsList.append(data)
n+=1
if n==6:
break
return render(request, 'newsModule.html', {'newsList':newsList, "category_id":category_id})
def newsShow(request, news_id):
news = News.objects.filter(news_id=news_id).all()
for new in news:
category=Category.objects.filter(category_id=new.category_id).all()
for c in category:
newsContent={
'id':new.news_id,
'title':new.n_title,
'author':new.author,
'time':str(new.n_time)[0:19],
'content':new.n_content,
'category':new.category_id,
'category_name':c.category_name,
'img':new.img
}
comments=News.objects.get(news_id=news_id).comments_set.all()
name_list=[]
for comment in comments:
name_list.append(User.objects.get(user_id=comment.user_id).user_name)
comment_list=zip(name_list, comments)
#提交用户评论
if request.method == "POST":
user_id=request.session.get('user_id')
if user_id is not None:
content = request.POST.get('content', None)
# 确定类别id
id_list=[]
for c in Comments.objects.all():
id_list.append(c.comment_id)
comment_id=max_id(id_list)
Comments.objects.create(comment_id=comment_id+1,
comment_content=content,
comment_time=str(datetime.datetime.now())[0:19],
comment_senti=3,
news_id=news_id,
user_id=user_id)
else:
messages.add_message(request, messages.INFO, "请登录后发表评论")
return redirect('/newsShow/'+str(news_id))
return render(request, 'newsShow.html', {'newsContent':newsContent, 'comment_list':comment_list})
def search(request):
categories=Category.objects.all()
return render(request, 'search.html',{'categories':categories})
# 新闻筛选
def searchData(request):
category = request.GET.get("data[category]")
year= request.GET.get("data[year]")
senti= request.GET.get("data[senti]")
news_list=News.objects.filter(n_senti=2).all()
news_list=News.objects.all()
if category=='' and year=='' and senti=='':
news_list=Ne
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目 python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目python期末大作业课程设计基于情感分析的新闻管理系统源码。纯手打项目
资源推荐
资源详情
资源评论
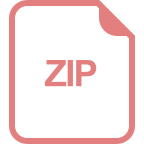
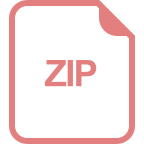
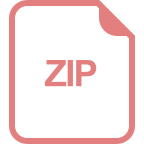
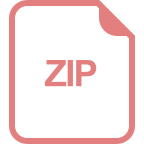
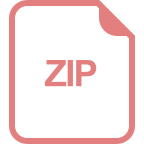
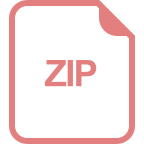
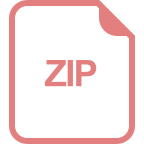
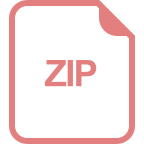
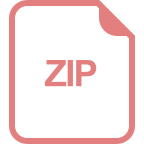
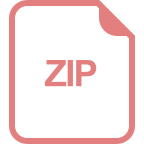
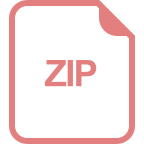
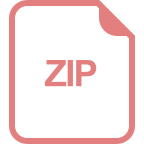
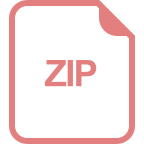
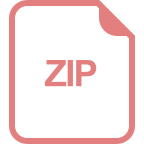
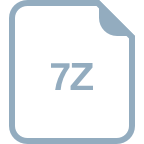
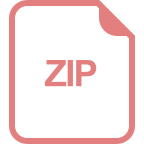
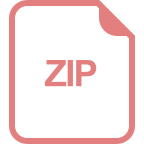
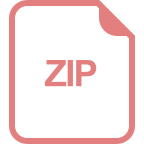
收起资源包目录

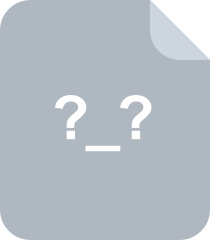
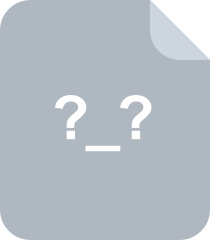
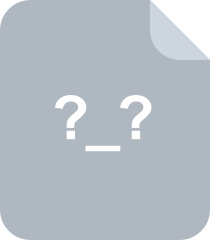
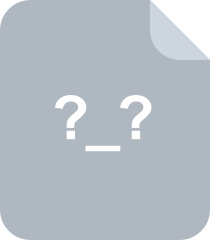
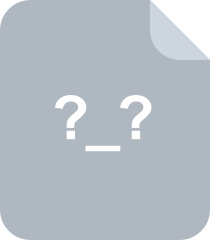
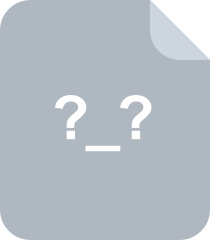
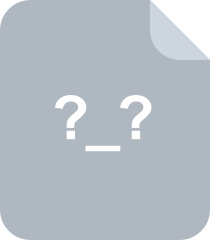
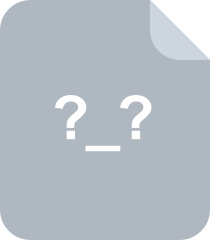
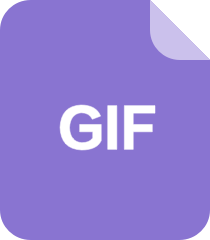
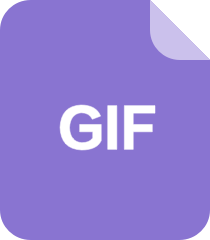
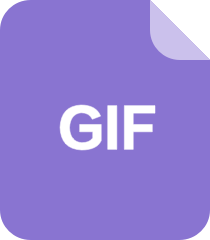
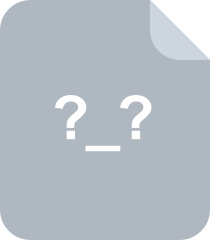
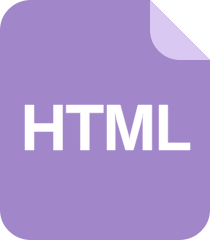
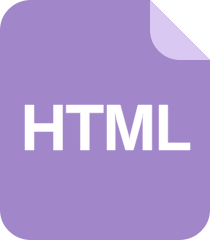
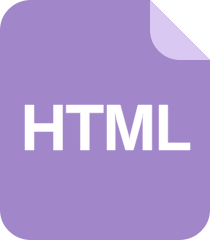
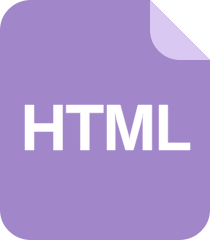
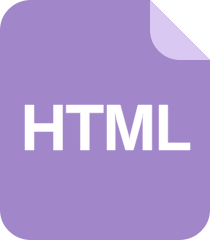
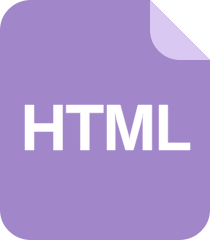
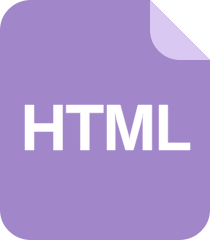
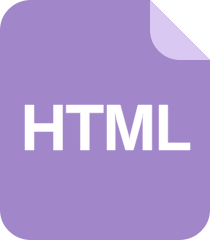
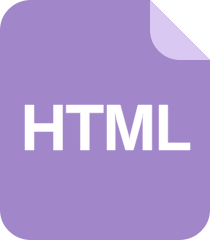
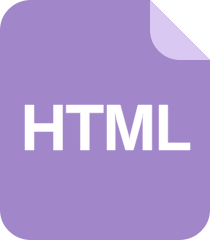
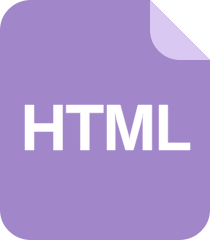
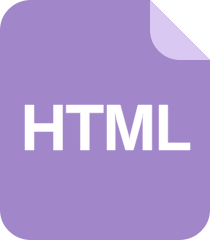
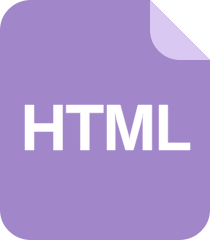
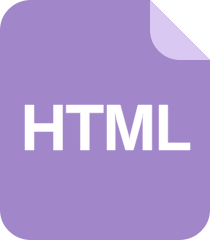
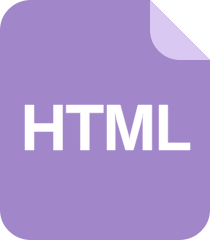
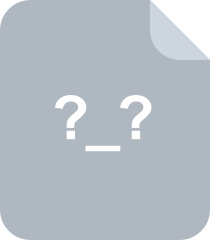
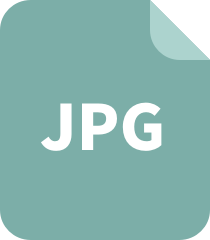
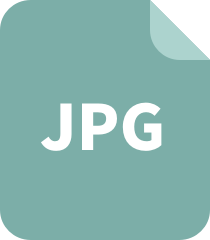
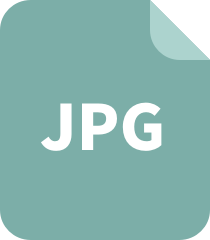
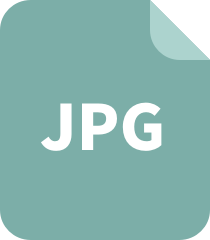
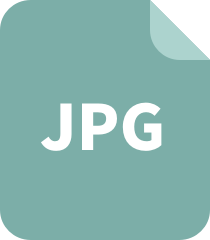
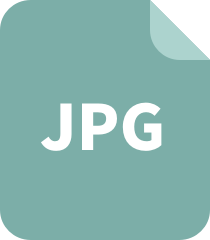
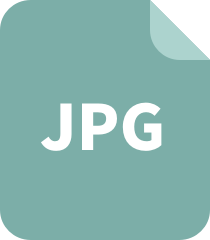
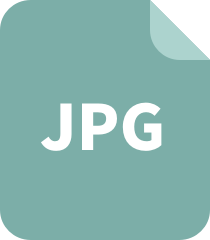
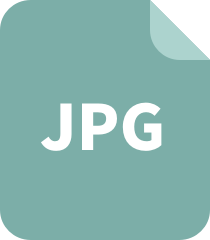
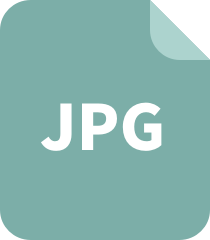
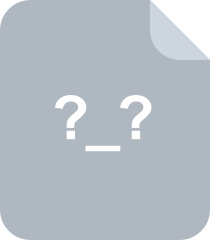
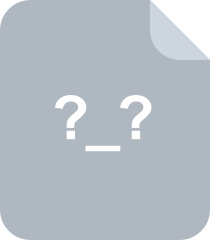
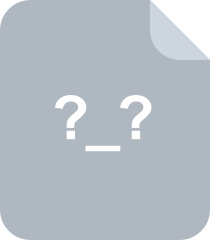
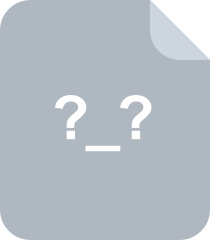
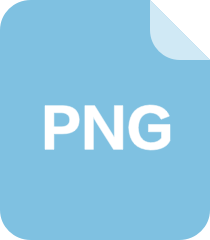
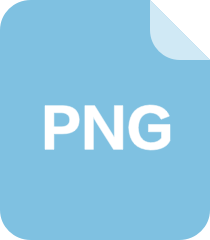
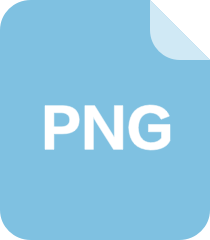
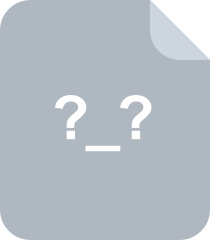
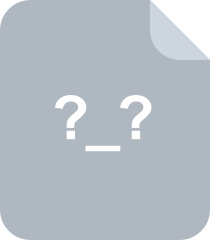
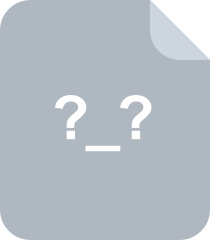
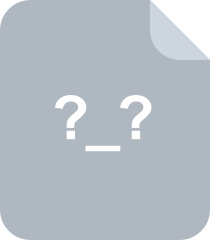
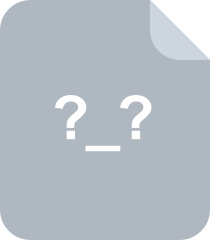
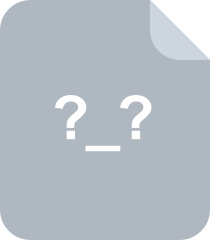
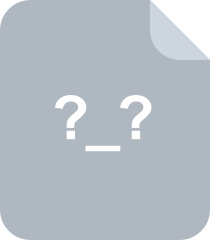
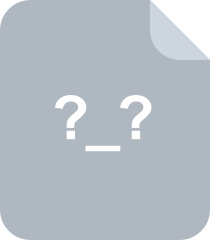
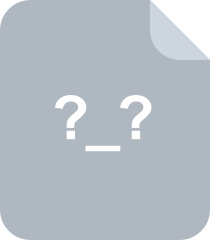
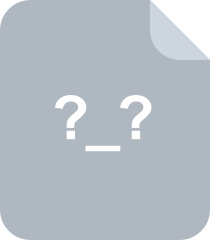
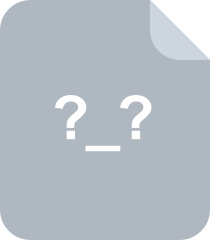
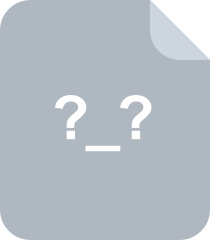
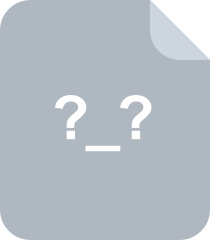
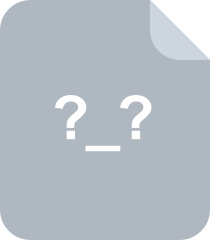
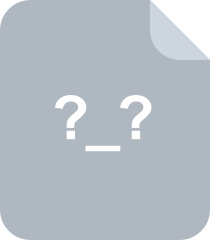
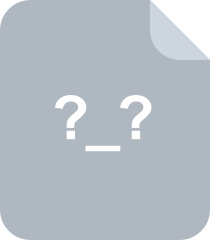
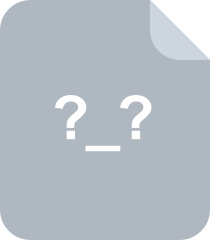
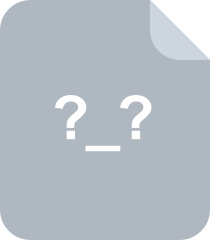
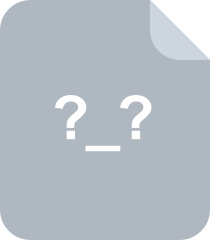
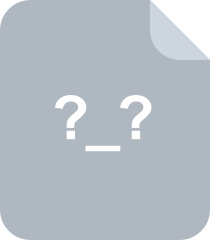
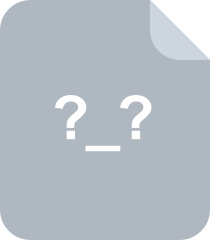
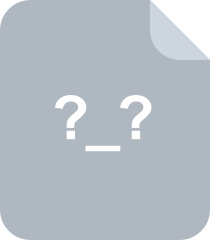
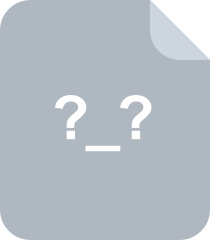
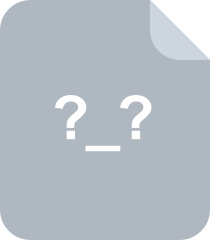
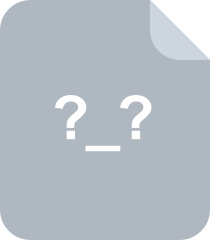
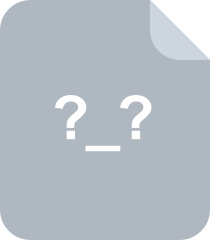
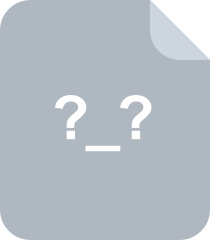
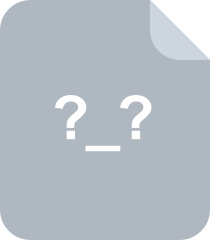
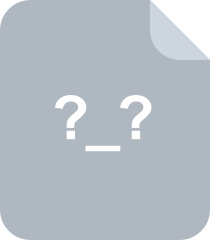
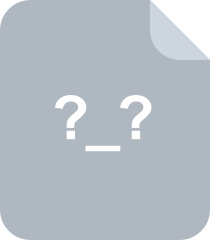
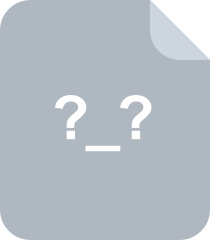
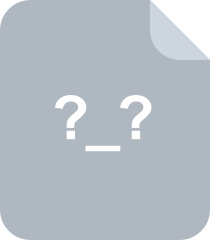
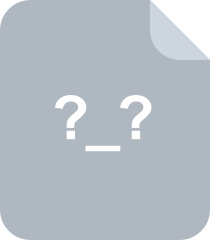
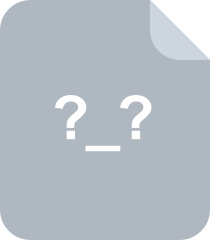
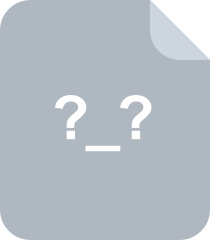
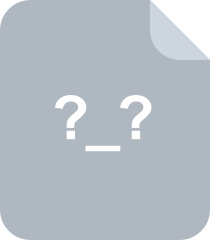
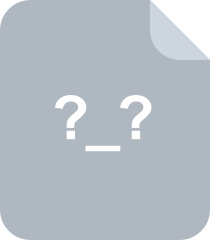
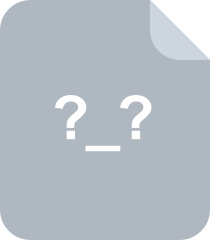
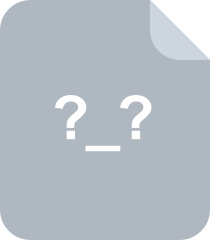
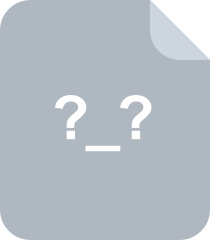
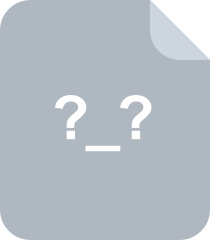
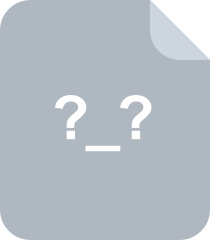
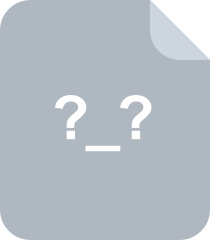
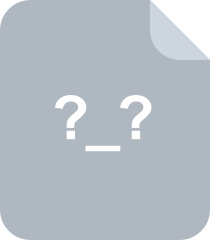
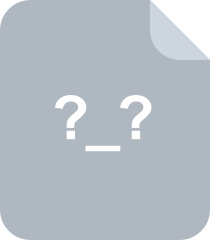
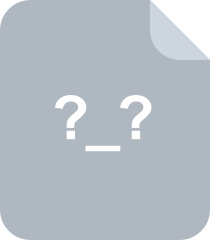
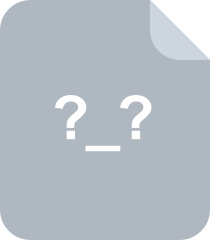
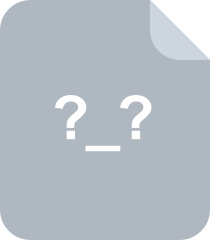
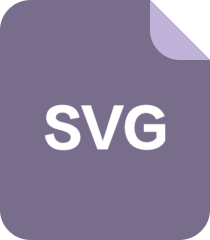
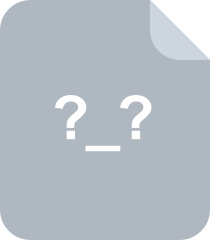
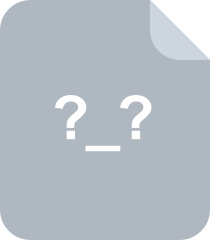
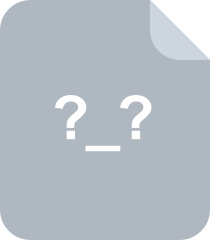
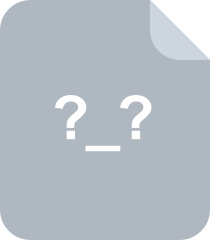
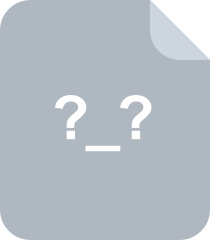
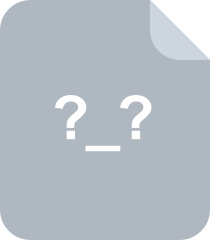
共 101 条
- 1
- 2
资源评论
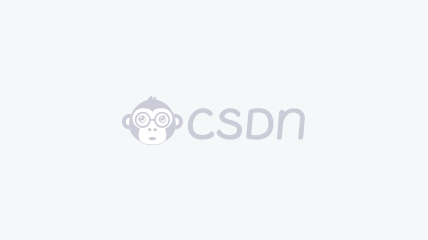
- y1y1111112022-09-21请问这大致步骤时啥呀
- m0_684320712023-12-15感谢大佬分享的资源,对我启发很大,给了我新的灵感。
- m0_590906782022-12-09资源很受用,资源主总结的很全面,内容与描述一致,解决了我当下的问题。
- lyqh20192023-04-15超赞的资源,感谢资源主分享,大家一起进步!
- lemon_coffee2023-05-31资源很实用,对我启发很大,有很好的参考价值,内容详细。

程序员张小妍
- 粉丝: 1w+
- 资源: 3134
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

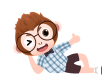
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


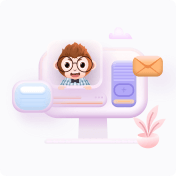
安全验证
文档复制为VIP权益,开通VIP直接复制
