cli
===
[](https://travis-ci.org/urfave/cli)
[](https://ci.appveyor.com/project/urfave/cli)
[](https://godoc.org/github.com/urfave/cli)
[](https://codebeat.co/projects/github-com-urfave-cli)
[](https://goreportcard.com/report/urfave/cli)
[](https://codecov.io/gh/urfave/cli)
cli is a simple, fast, and fun package for building command line apps in Go. The
goal is to enable developers to write fast and distributable command line
applications in an expressive way.
<!-- toc -->
- [Overview](#overview)
- [Installation](#installation)
* [Supported platforms](#supported-platforms)
* [Using the `v2` branch](#using-the-v2-branch)
* [Using `v1` releases](#using-v1-releases)
- [Getting Started](#getting-started)
- [Examples](#examples)
* [Arguments](#arguments)
* [Flags](#flags)
+ [Placeholder Values](#placeholder-values)
+ [Alternate Names](#alternate-names)
+ [Ordering](#ordering)
+ [Values from the Environment](#values-from-the-environment)
+ [Values from files](#values-from-files)
+ [Values from alternate input sources (YAML, TOML, and others)](#values-from-alternate-input-sources-yaml-toml-and-others)
+ [Precedence](#precedence)
* [Subcommands](#subcommands)
* [Subcommands categories](#subcommands-categories)
* [Exit code](#exit-code)
* [Combining short options](#combining-short-options)
* [Bash Completion](#bash-completion)
+ [Enabling](#enabling)
+ [Distribution](#distribution)
+ [Customization](#customization)
* [Generated Help Text](#generated-help-text)
+ [Customization](#customization-1)
* [Version Flag](#version-flag)
+ [Customization](#customization-2)
+ [Full API Example](#full-api-example)
- [Contribution Guidelines](#contribution-guidelines)
<!-- tocstop -->
## Overview
Command line apps are usually so tiny that there is absolutely no reason why
your code should *not* be self-documenting. Things like generating help text and
parsing command flags/options should not hinder productivity when writing a
command line app.
**This is where cli comes into play.** cli makes command line programming fun,
organized, and expressive!
## Installation
Make sure you have a working Go environment. Go version 1.10+ is supported. [See
the install instructions for Go](http://golang.org/doc/install.html).
To install cli, simply run:
```
$ go get github.com/urfave/cli
```
Make sure your `PATH` includes the `$GOPATH/bin` directory so your commands can
be easily used:
```
export PATH=$PATH:$GOPATH/bin
```
### Supported platforms
cli is tested against multiple versions of Go on Linux, and against the latest
released version of Go on OS X and Windows. For full details, see
[`./.travis.yml`](./.travis.yml) and [`./appveyor.yml`](./appveyor.yml).
### Using the `v2` branch
**Warning**: The `v2` branch is currently unreleased and considered unstable.
There is currently a long-lived branch named `v2` that is intended to land as
the new `master` branch once development there has settled down. The current
`master` branch (mirrored as `v1`) is being manually merged into `v2` on
an irregular human-based schedule, but generally if one wants to "upgrade" to
`v2` *now* and accept the volatility (read: "awesomeness") that comes along with
that, please use whatever version pinning of your preference, such as via
`gopkg.in`:
```
$ go get gopkg.in/urfave/cli.v2
```
``` go
...
import (
"gopkg.in/urfave/cli.v2" // imports as package "cli"
)
...
```
### Using `v1` releases
```
$ go get github.com/urfave/cli
```
```go
...
import (
"github.com/urfave/cli"
)
...
```
## Getting Started
One of the philosophies behind cli is that an API should be playful and full of
discovery. So a cli app can be as little as one line of code in `main()`.
<!-- {
"args": ["--help"],
"output": "A new cli application"
} -->
``` go
package main
import (
"log"
"os"
"github.com/urfave/cli"
)
func main() {
err := cli.NewApp().Run(os.Args)
if err != nil {
log.Fatal(err)
}
}
```
This app will run and show help text, but is not very useful. Let's give an
action to execute and some help documentation:
<!-- {
"output": "boom! I say!"
} -->
``` go
package main
import (
"fmt"
"log"
"os"
"github.com/urfave/cli"
)
func main() {
app := cli.NewApp()
app.Name = "boom"
app.Usage = "make an explosive entrance"
app.Action = func(c *cli.Context) error {
fmt.Println("boom! I say!")
return nil
}
err := app.Run(os.Args)
if err != nil {
log.Fatal(err)
}
}
```
Running this already gives you a ton of functionality, plus support for things
like subcommands and flags, which are covered below.
## Examples
Being a programmer can be a lonely job. Thankfully by the power of automation
that is not the case! Let's create a greeter app to fend off our demons of
loneliness!
Start by creating a directory named `greet`, and within it, add a file,
`greet.go` with the following code in it:
<!-- {
"output": "Hello friend!"
} -->
``` go
package main
import (
"fmt"
"log"
"os"
"github.com/urfave/cli"
)
func main() {
app := cli.NewApp()
app.Name = "greet"
app.Usage = "fight the loneliness!"
app.Action = func(c *cli.Context) error {
fmt.Println("Hello friend!")
return nil
}
err := app.Run(os.Args)
if err != nil {
log.Fatal(err)
}
}
```
Install our command to the `$GOPATH/bin` directory:
```
$ go install
```
Finally run our new command:
```
$ greet
Hello friend!
```
cli also generates neat help text:
```
$ greet help
NAME:
greet - fight the loneliness!
USAGE:
greet [global options] command [command options] [arguments...]
VERSION:
0.0.0
COMMANDS:
help, h Shows a list of commands or help for one command
GLOBAL OPTIONS
--version Shows version information
```
### Arguments
You can lookup arguments by calling the `Args` function on `cli.Context`, e.g.:
<!-- {
"output": "Hello \""
} -->
``` go
package main
import (
"fmt"
"log"
"os"
"github.com/urfave/cli"
)
func main() {
app := cli.NewApp()
app.Action = func(c *cli.Context) error {
fmt.Printf("Hello %q", c.Args().Get(0))
return nil
}
err := app.Run(os.Args)
if err != nil {
log.Fatal(err)
}
}
```
### Flags
Setting and querying flags is simple.
<!-- {
"output": "Hello Nefertiti"
} -->
``` go
package main
import (
"fmt"
"log"
"os"
"github.com/urfave/cli"
)
func main() {
app := cli.NewApp()
app.Flags = []cli.Flag {
cli.StringFlag{
Name: "lang",
Value: "english",
Usage: "language for the greeting",
},
}
app.Action = func(c *cli.Context) error {
name := "Nefertiti"
if c.NArg() > 0 {
name = c.Args().Get(0)
}
if c.String("lang") == "spanish" {
fmt.Println("Hola", name)
} else {
fmt.Println("Hello", name)
}
return nil
}
err := app.Run(os.Args)
if err != nil {
log.Fatal(err)
}
}
```
You can also set a destination variable for a flag, to which the content will be
scanned.
<!-- {
"output": "Hello someone"
} -->
``` go
package main
import (
"log"
"os"
"fmt"
"github.com/urfave/cli"
)
func main() {
var language string
app := cli.NewApp()
app.Flags = []cli.Flag {
cli.StringFlag{
Name: "lang",
Value: "english",
Usage: "language for the greeting",
Destination: &language,
},
}
app.Action = func(c *cli.Context) error {
name := "someone"
if c.NArg() > 0 {
name = c.Args()[0]
}
if language == "spanish" {
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Get runC 在旧金山举行的DockerCon大会上, 开放容器项目 和一个运行容器的CLI工具runC同时和大家见面。runC是 libcontainer 的一层包装(wrapper )。 它是Docker捐献给开放容器项目用来作为参考实现的。这听起来很有意思,尤其是主要的供应商是OCI的一部分,就像rkt之于CoreOS。 让我们签出 runC 源码用它把Docker容器跑起来吧。 一、runc简介 RunC 是一个轻量级的工具,它是用来运行容器的,只用来做这一件事,并且这一件事要做好。我们可以认为它就是个命令行小工具,可以不用通过 docker 引擎,直接运行容器。事实上,runC 是标准化的产物,它根据 OCI 标准来创建和运行容器。而 OCI(Open Container Initiative)组织,旨在围绕容器格式和运行时制定一个开放的工业化标准。 二、安装runc RunC 是用 golang 创建的项目,因此编译它之前需要在本地安装 golang 的开发环境。Golang 的安装请参考《CentOS7使用yum安装Golang(超详细)》一文,这里不再赘述。
资源推荐
资源详情
资源评论
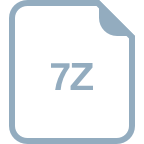
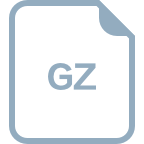
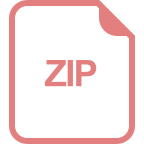
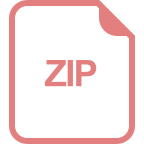
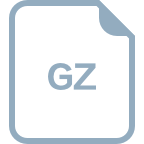
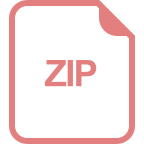
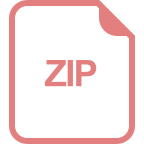
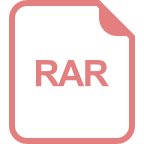
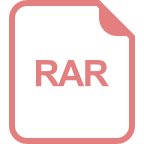
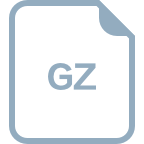
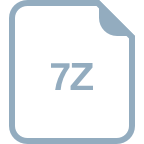
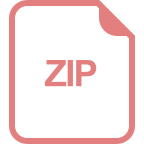
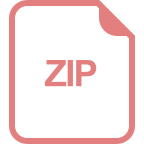
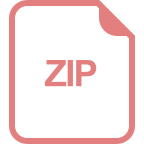
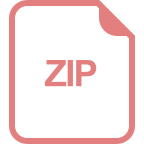
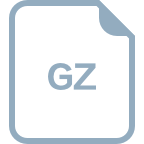
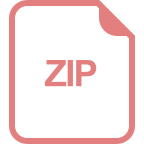
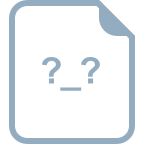
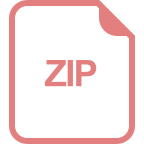
收起资源包目录

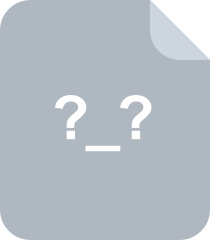
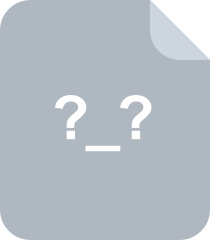
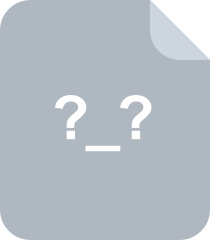
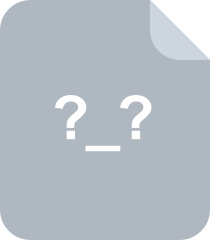
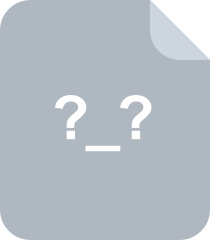
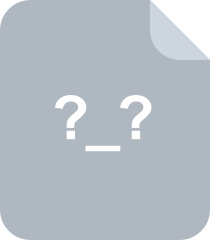
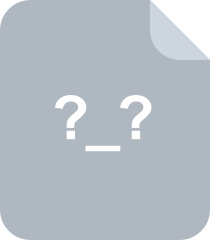
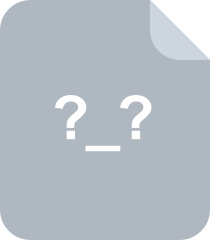
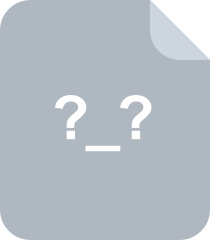
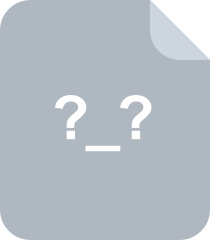
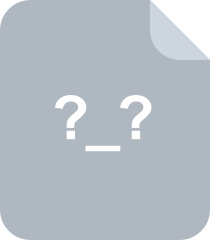
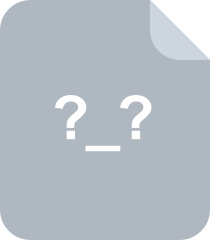
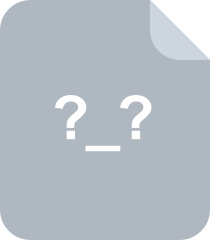
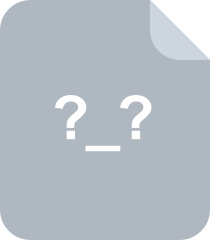
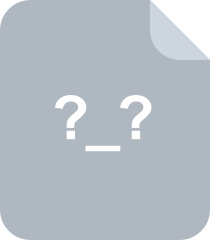
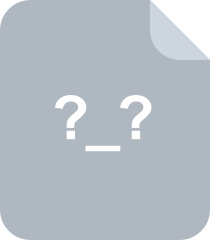
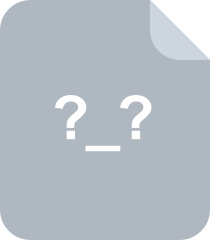
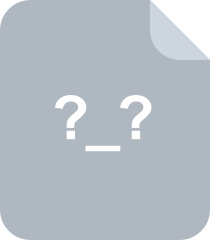
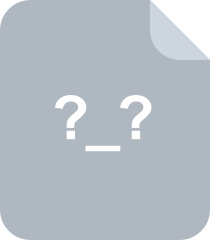
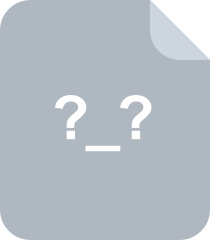
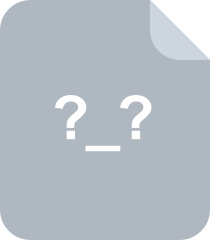
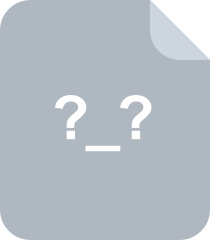
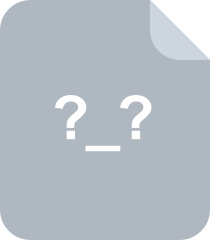
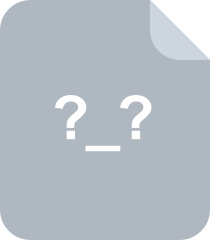
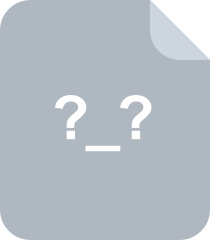
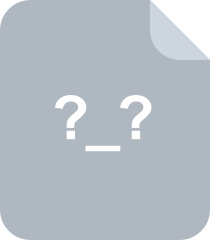
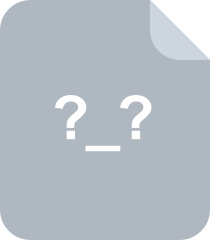
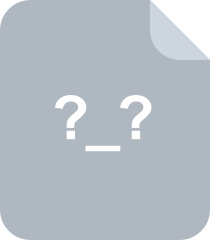
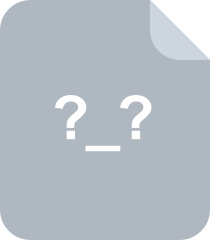
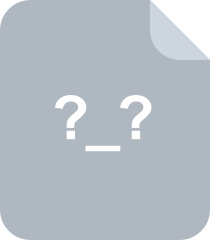
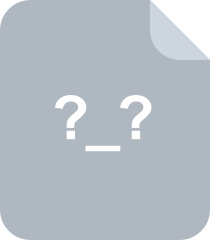
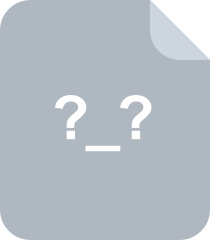
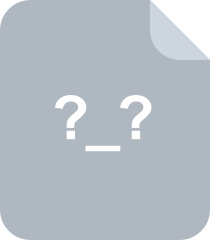
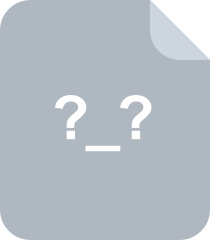
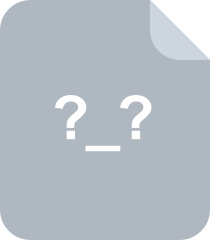
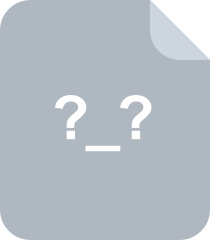
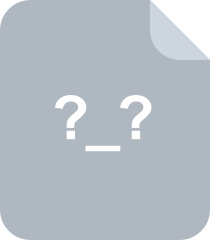
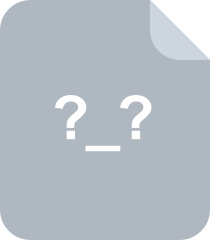
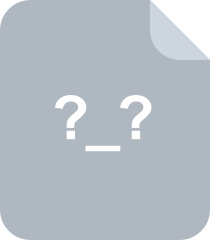
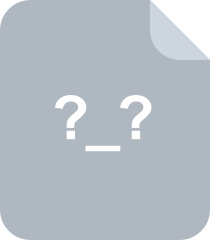
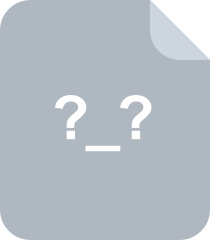
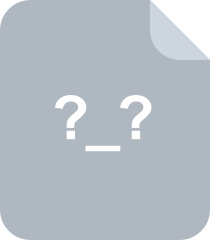
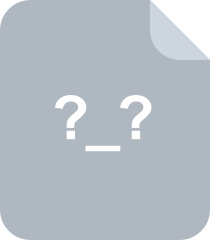
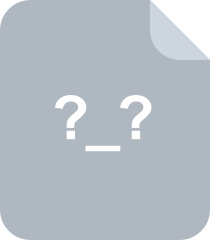
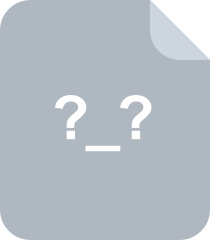
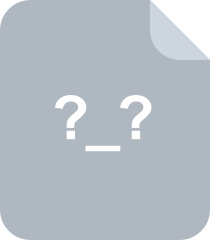
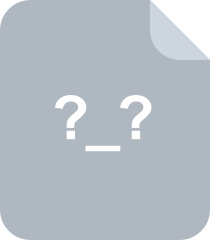
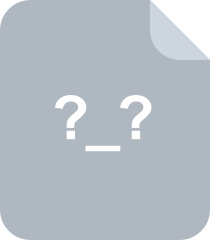
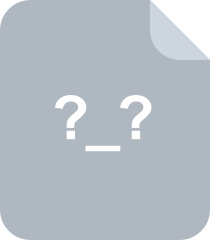
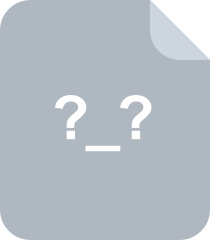
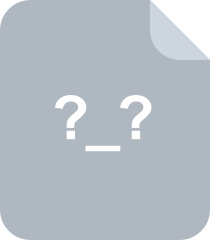
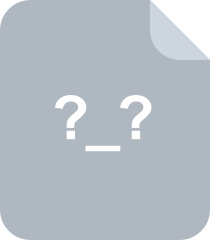
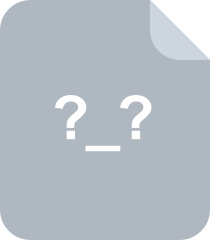
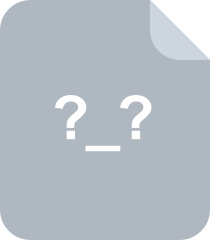
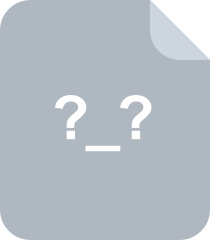
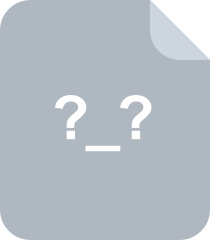
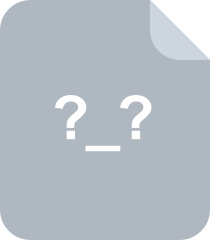
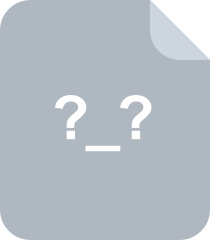
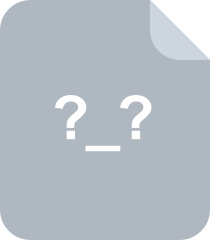
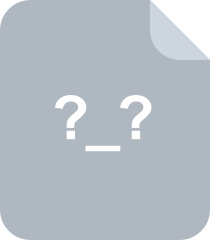
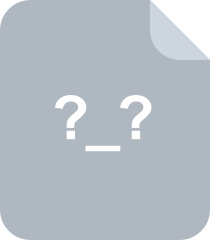
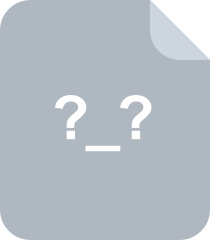
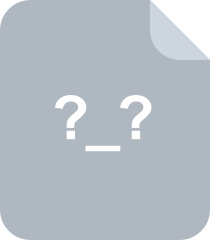
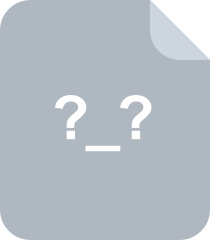
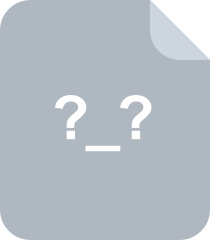
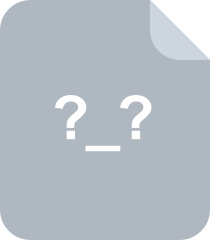
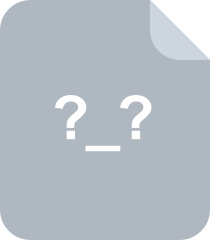
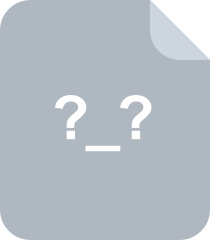
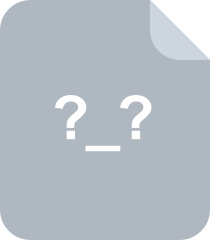
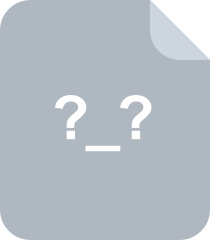
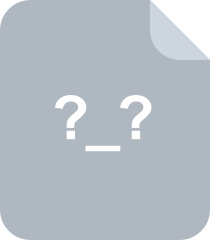
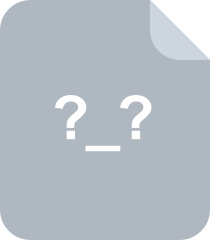
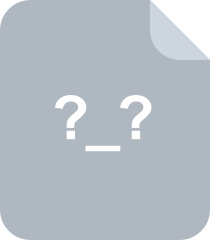
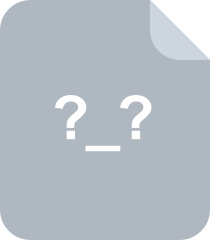
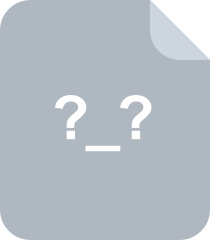
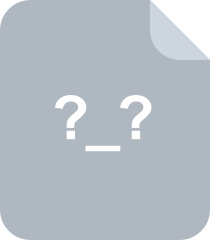
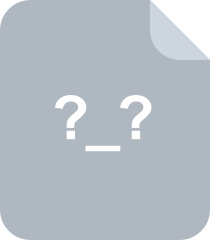
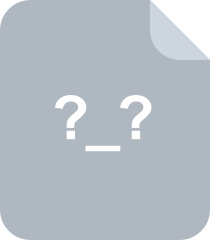
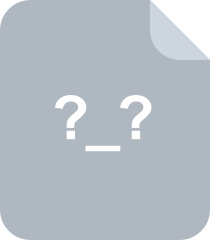
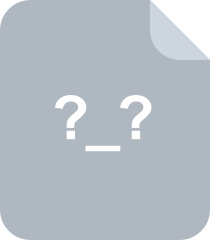
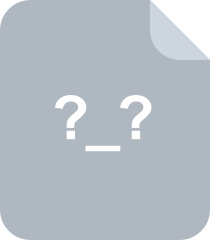
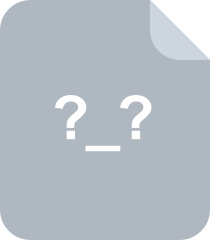
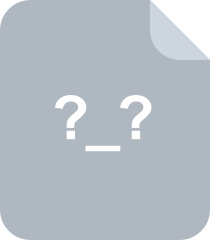
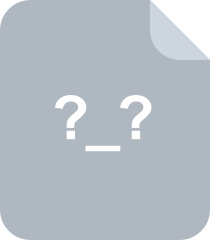
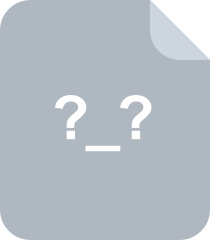
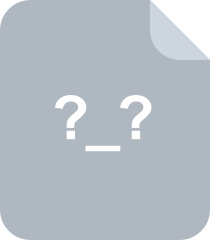
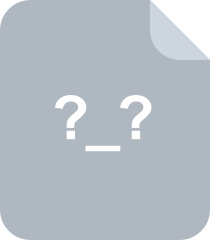
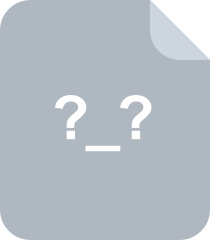
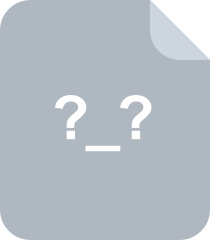
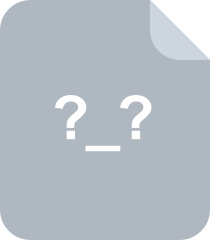
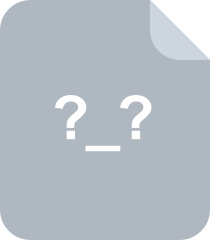
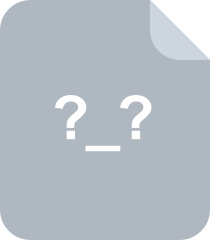
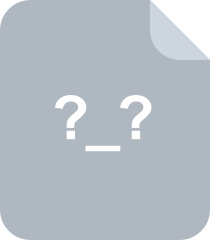
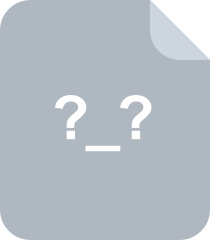
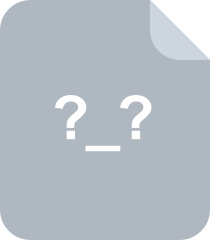
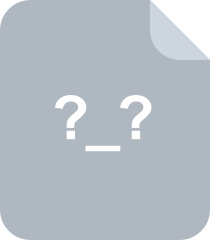
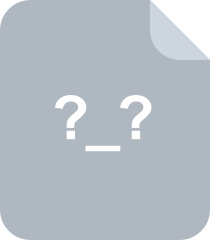
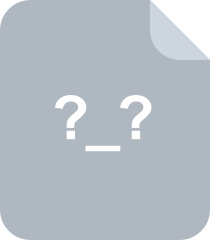
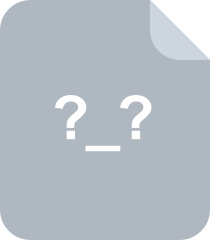
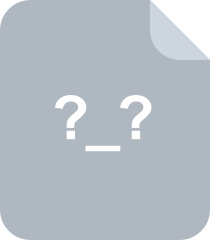
共 1204 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
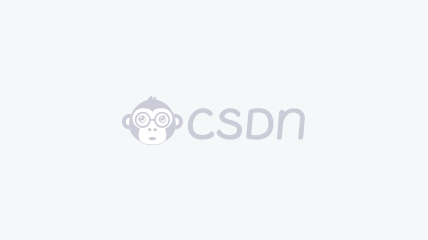

坦笑&&life
- 粉丝: 6w+
- 资源: 1424
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

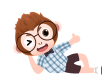
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


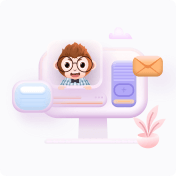
安全验证
文档复制为VIP权益,开通VIP直接复制
