package com.controller;
import java.math.BigDecimal;
import java.text.SimpleDateFormat;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.annotation.IgnoreAuth;
import com.entity.ExampaperEntity;
import com.entity.view.ExampaperView;
import com.service.ExampaperService;
import com.service.TokenService;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.MPUtil;
import com.utils.MapUtils;
import com.utils.CommonUtil;
import java.io.IOException;
import com.service.ExamquestionService;
import com.service.ExamquestionbankService;
import com.service.ExamrecordService;
import com.entity.ExamquestionEntity;
import com.entity.ExamquestionbankEntity;
import com.entity.ExamrecordEntity;
/**
* 心理测评表
* 后端接口
* @author
* @email
* @date 2024-05-10 20:38:36
*/
@RestController
@RequestMapping("/exampaper")
public class ExampaperController {
@Autowired
private ExampaperService exampaperService;
@Autowired
private ExamquestionService examquestionService;
@Autowired
private ExamquestionbankService examquestionbankService;
@Autowired
private ExamrecordService examrecordService;
/**
* 后台列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,ExampaperEntity exampaper,
HttpServletRequest request){
EntityWrapper<ExampaperEntity> ew = new EntityWrapper<ExampaperEntity>();
PageUtils page = exampaperService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, exampaper), params), params));
return R.ok().put("data", page);
}
/**
* 前台列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,ExampaperEntity exampaper,
HttpServletRequest request){
EntityWrapper<ExampaperEntity> ew = new EntityWrapper<ExampaperEntity>();
PageUtils page = exampaperService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, exampaper), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( ExampaperEntity exampaper){
EntityWrapper<ExampaperEntity> ew = new EntityWrapper<ExampaperEntity>();
ew.allEq(MPUtil.allEQMapPre( exampaper, "exampaper"));
return R.ok().put("data", exampaperService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(ExampaperEntity exampaper){
EntityWrapper< ExampaperEntity> ew = new EntityWrapper< ExampaperEntity>();
ew.allEq(MPUtil.allEQMapPre( exampaper, "exampaper"));
ExampaperView exampaperView = exampaperService.selectView(ew);
return R.ok("查询心理测评表成功").put("data", exampaperView);
}
/**
* 后台详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
ExampaperEntity exampaper = exampaperService.selectById(id);
return R.ok().put("data", exampaper);
}
/**
* 前台详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
ExampaperEntity exampaper = exampaperService.selectById(id);
return R.ok().put("data", exampaper);
}
/**
* 后台保存
*/
@RequestMapping("/save")
public R save(@RequestBody ExampaperEntity exampaper, HttpServletRequest request){
//ValidatorUtils.validateEntity(exampaper);
exampaperService.insert(exampaper);
return R.ok();
}
/**
* 前台保存
*/
@RequestMapping("/add")
public R add(@RequestBody ExampaperEntity exampaper, HttpServletRequest request){
//ValidatorUtils.validateEntity(exampaper);
exampaperService.insert(exampaper);
return R.ok();
}
/**
* 获取用户密保
*/
@RequestMapping("/security")
@IgnoreAuth
public R security(@RequestParam String username){
ExampaperEntity exampaper = exampaperService.selectOne(new EntityWrapper<ExampaperEntity>().eq("", username));
return R.ok().put("data", exampaper);
}
/**
* 修改
*/
@RequestMapping("/update")
@Transactional
@IgnoreAuth
public R update(@RequestBody ExampaperEntity exampaper, HttpServletRequest request){
//ValidatorUtils.validateEntity(exampaper);
exampaperService.updateById(exampaper);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
exampaperService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 前台智能排序
*/
@IgnoreAuth
@RequestMapping("/autoSort")
public R autoSort(@RequestParam Map<String, Object> params,ExampaperEntity exampaper, HttpServletRequest request,String pre){
EntityWrapper<ExampaperEntity> ew = new EntityWrapper<ExampaperEntity>();
Map<String, Object> newMap = new HashMap<String, Object>();
Map<String, Object> param = new HashMap<String, Object>();
Iterator<Map.Entry<String, Object>> it = param.entrySet().iterator();
while (it.hasNext()) {
Map.Entry<String, Object> entry = it.next();
String key = entry.getKey();
String newKey = entry.getKey();
if (pre.endsWith(".")) {
newMap.put(pre + newKey, entry.getValue());
} else if (StringUtils.isEmpty(pre)) {
newMap.put(newKey, entry.getValue());
} else {
newMap.put(pre + "." + newKey, entry.getValue());
}
}
params.put("sort", "clicktime");
params.put("order", "desc");
PageUtils page = exampaperService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, exampaper), params), params));
return R.ok().put("data", page);
}
/**
* 组卷
*/
@RequestMapping("/compose")
public R compose(HttpServletRequest request,@RequestParam Long paperid, @RequestParam String papername, @RequestParam Integer radioNum,
@RequestParam Integer multipleChoiceNum, @RequestParam Integer determineNum, @RequestParam Integer fillNum, @RequestParam Integer subjectivityNum){
//如果已存在考试记录,不能进行重新组卷
if(examrecordService.selectCount(new EntityWrapper<ExamrecordEntity>().eq("paperid", paperid))>0) {
return R.error("已存在考试记录,无法重新组卷");
}
//组卷之前删除该试卷之前的所有题目
examquestionService.deleteByMap(new MapUtils().put("paperid", paperid));
List<ExamquestionbankEntity> questionList = new ArrayList<ExamquestionbankEntity>();
//单选题
if(radioNum>0) {
Wrapper<ExamquestionbankEntity> countEw0 = new EntityWrapper<Examqu
没有合适的资源?快使用搜索试试~ 我知道了~
【java毕业设计】大学生心理测评与分析系统(springboot+vue+mysql+说明文档+LW).zip
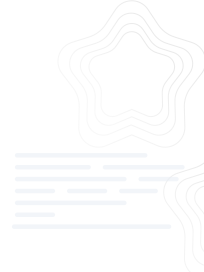
共792个文件
java:211个
svg:159个
vue:149个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 12 浏览量
2024-11-09
12:55:46
上传
评论
收藏 21.06MB ZIP 举报
温馨提示
项目经过测试均可完美运行! 环境说明: 开发语言:java jdk:jdk1.8 数据库:mysql 5.7+ 数据库工具:Navicat11+ 管理工具:maven 开发工具:idea/eclipse
资源推荐
资源详情
资源评论
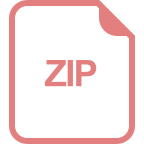
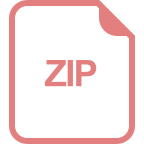
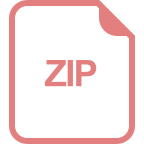
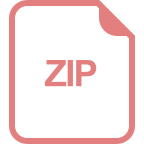
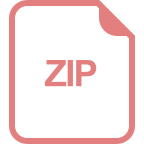
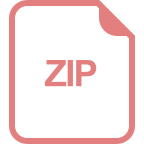
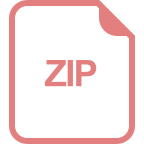
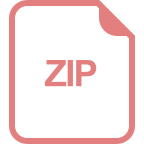
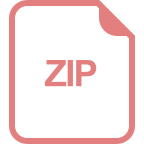
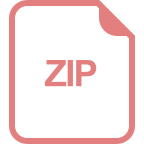
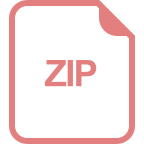
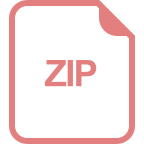
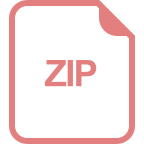
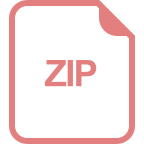
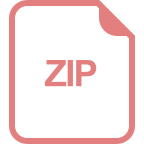
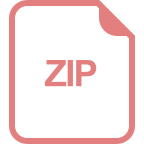
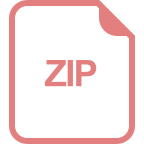
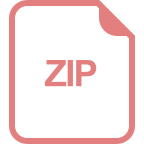
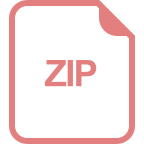
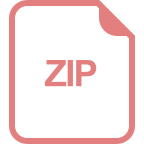
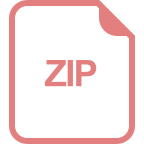
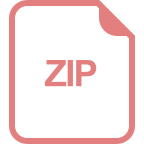
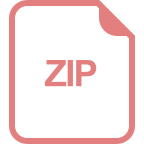
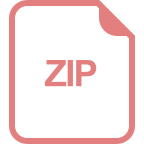
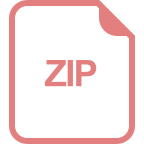
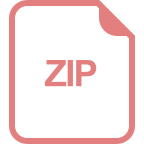
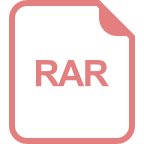
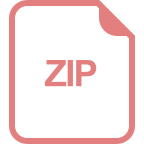
收起资源包目录

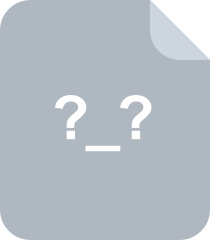
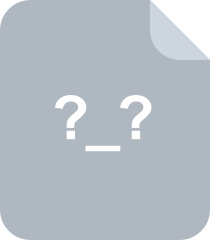
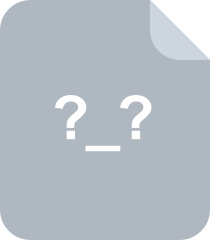
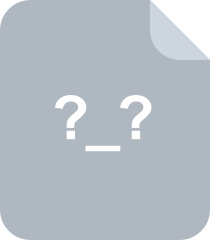
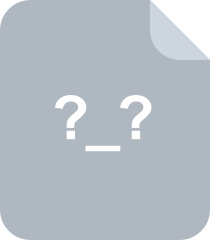
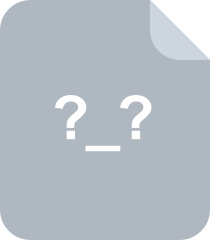
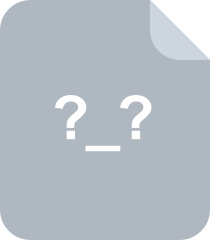
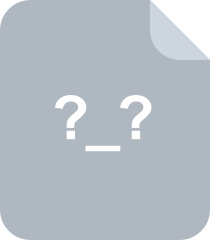
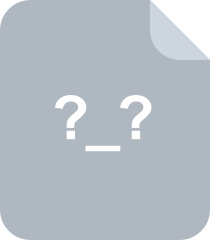
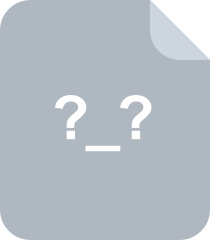
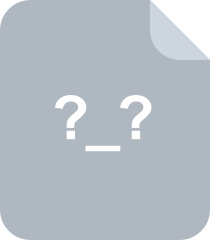
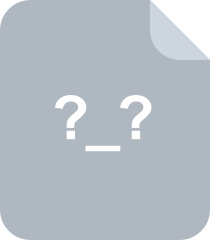
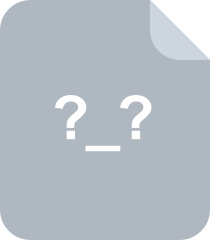
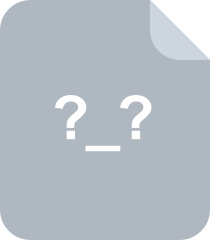
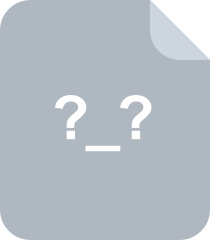
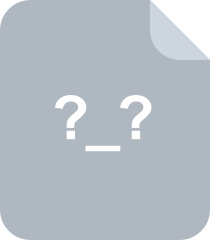
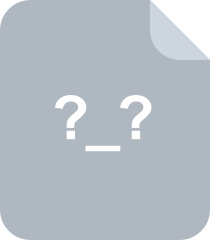
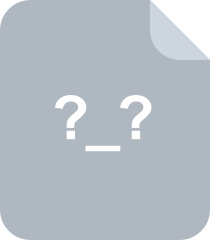
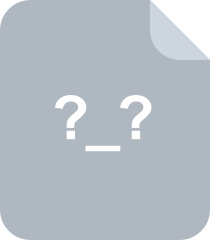
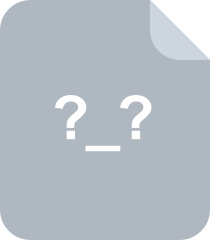
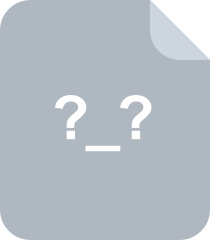
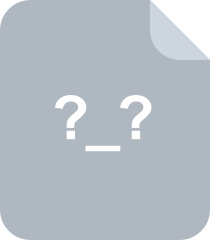
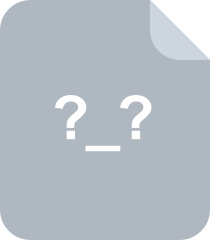
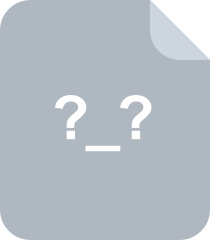
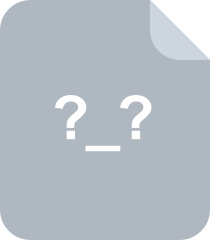
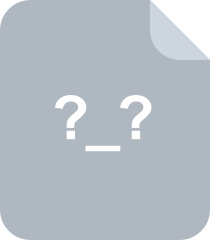
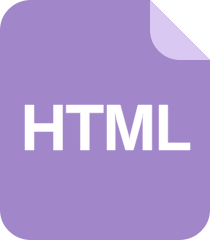
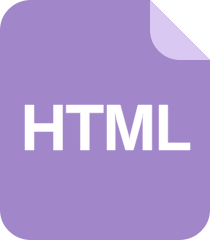
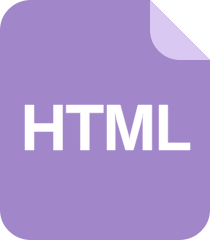
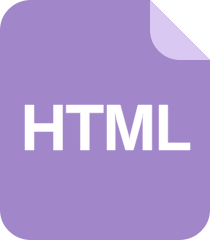
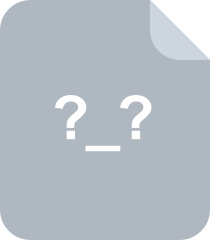
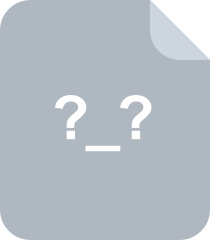
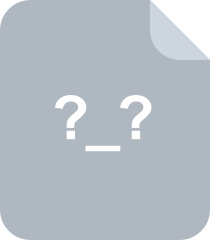
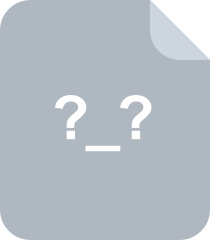
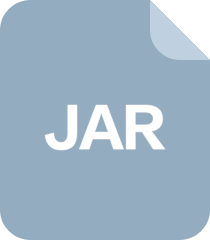
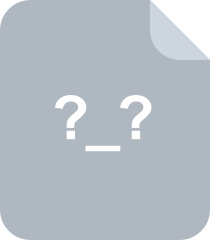
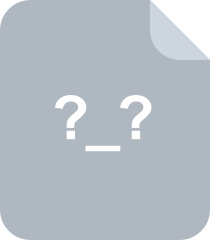
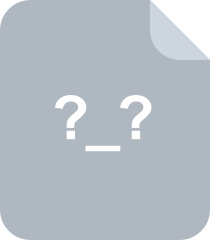
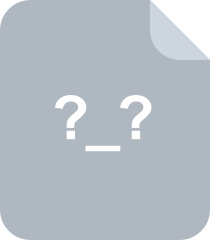
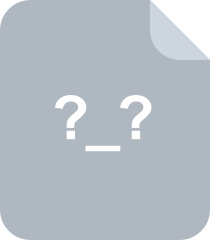
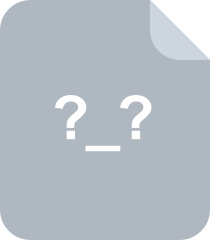
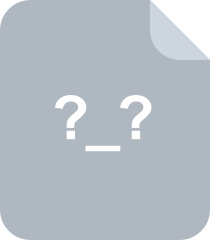
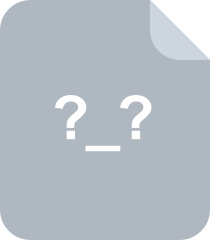
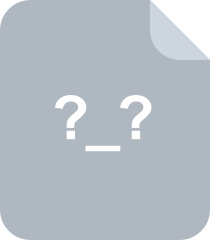
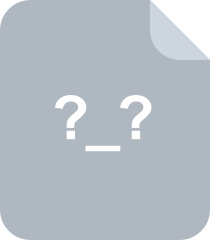
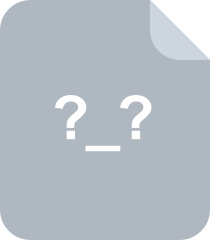
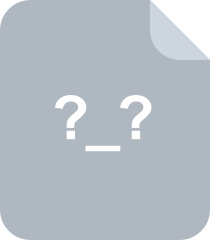
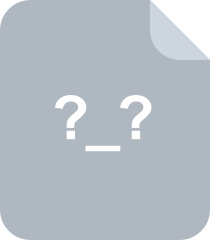
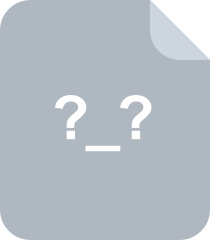
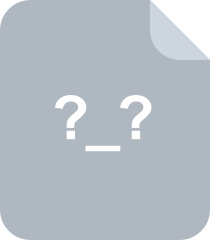
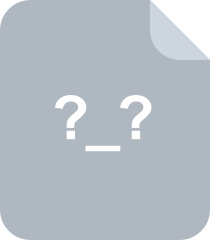
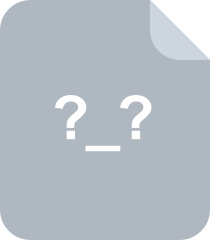
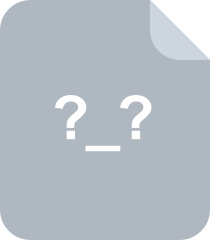
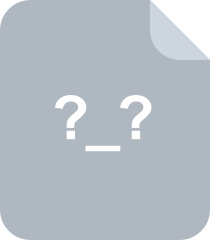
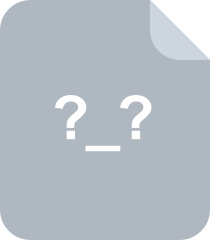
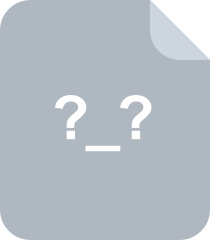
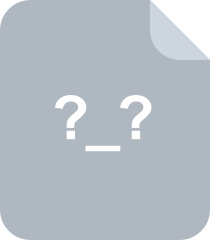
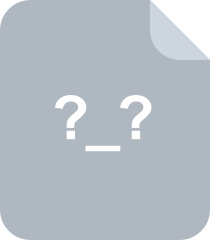
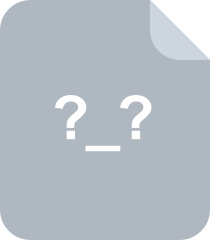
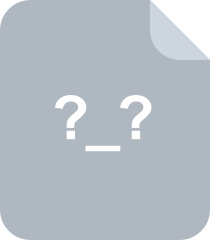
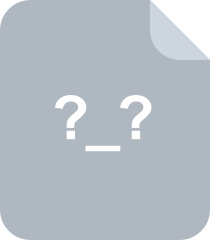
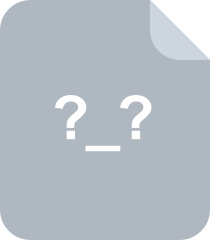
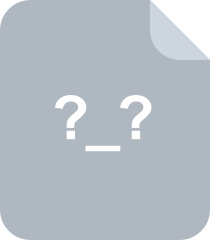
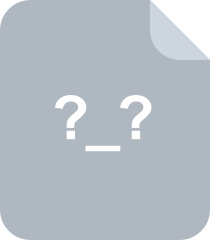
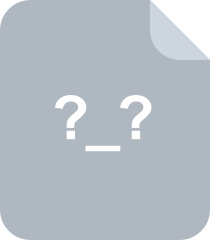
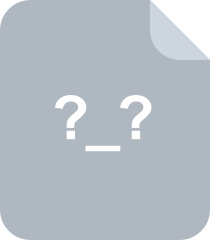
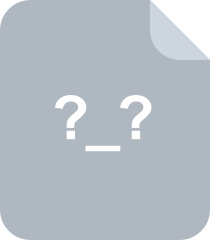
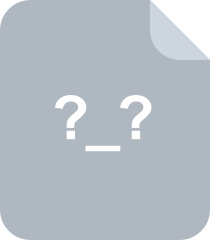
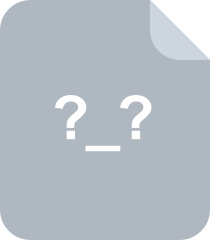
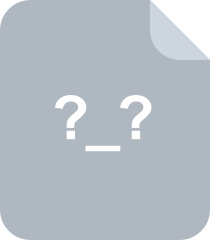
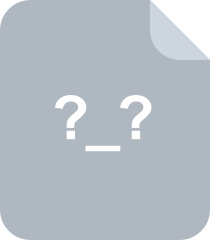
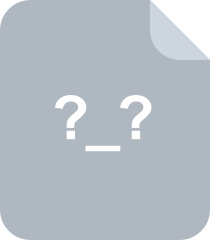
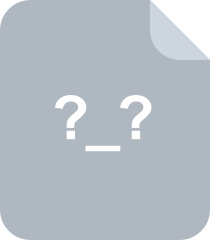
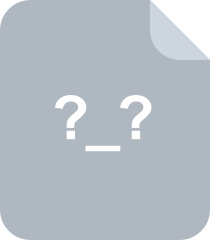
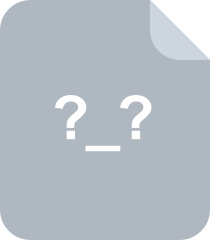
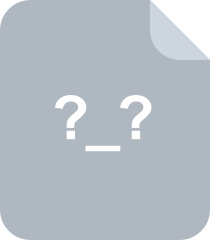
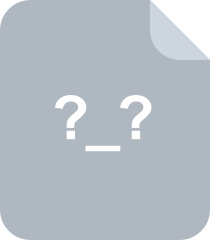
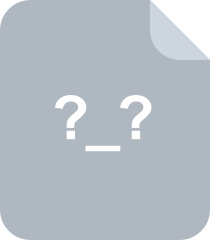
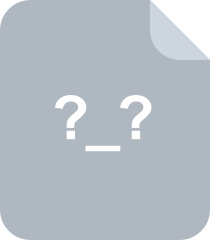
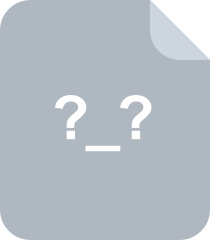
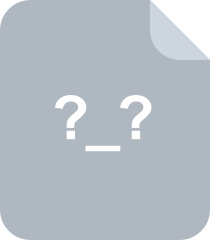
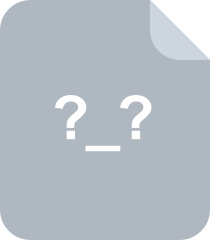
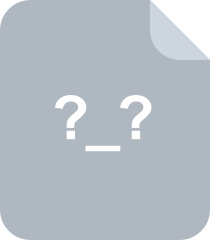
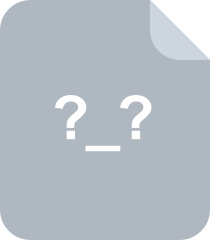
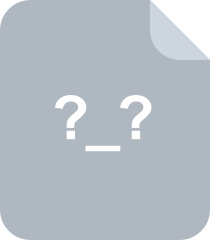
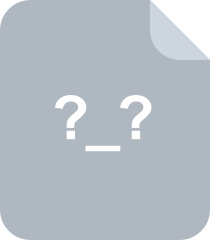
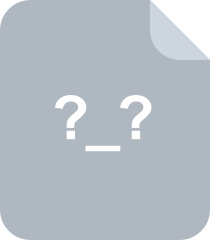
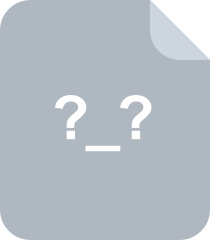
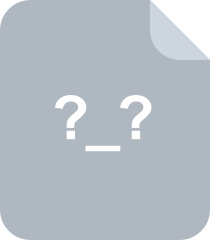
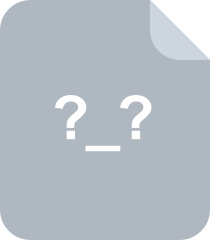
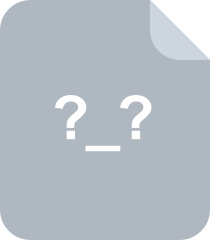
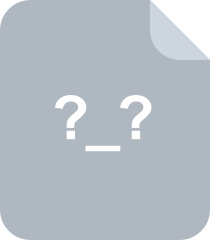
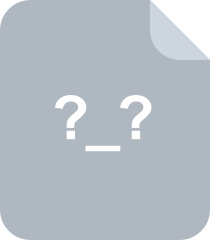
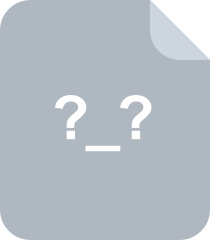
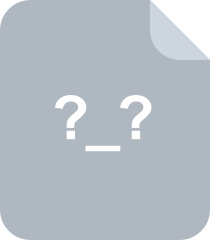
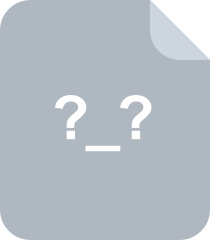
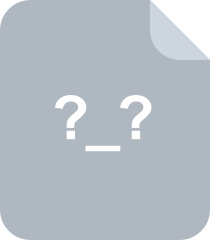
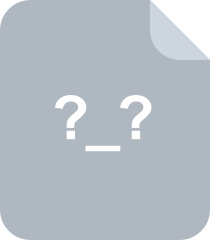
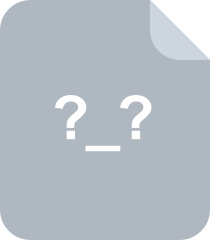
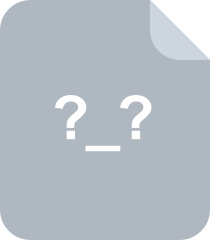
共 792 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
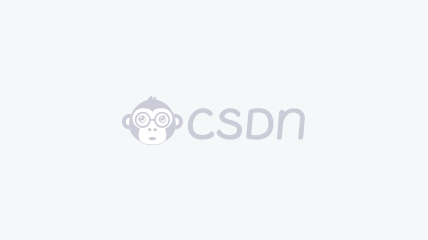

计算机学长阿伟
- 粉丝: 3196
- 资源: 849
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

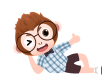
最新资源
- (源码)基于Redis和Elasticsearch的日志与指标处理系统.zip
- 学习记录111111111111111111111111
- (源码)基于Python和Selenium的jksb系统健康申报助手.zip
- (源码)基于HiEasyX库的学习工具系统.zip
- (源码)基于JSP+Servlet+JDBC的学生宿舍管理系统.zip
- (源码)基于Arduino和Raspberry Pi的自动化花园系统.zip
- (源码)基于JSP和Servlet的数据库管理系统.zip
- (源码)基于Python的文本相似度计算系统.zip
- (源码)基于Spring Boot和Redis的高并发秒杀系统.zip
- (源码)基于Java的Web汽车销售管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


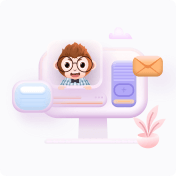
安全验证
文档复制为VIP权益,开通VIP直接复制
