package com.controller;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.annotation.IgnoreAuth;
import com.entity.XueshengEntity;
import com.entity.view.XueshengView;
import com.service.XueshengService;
import com.service.TokenService;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.MD5Util;
import com.utils.MPUtil;
import com.utils.CommonUtil;
/**
* 学生
* 后端接口
* @author
* @email
* @date 2021-04-04 11:13:07
*/
@RestController
@RequestMapping("/xuesheng")
public class XueshengController {
@Autowired
private XueshengService xueshengService;
@Autowired
private TokenService tokenService;
/**
* 登录
*/
@IgnoreAuth
@RequestMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
XueshengEntity user = xueshengService.selectOne(new EntityWrapper<XueshengEntity>().eq("xuehao", username));
if(user==null || !user.getMima().equals(password)) {
return R.error("账号或密码不正确");
}
String token = tokenService.generateToken(user.getId(), username,"xuesheng", "学生" );
return R.ok().put("token", token);
}
/**
* 注册
*/
@IgnoreAuth
@RequestMapping("/register")
public R register(@RequestBody XueshengEntity xuesheng){
//ValidatorUtils.validateEntity(xuesheng);
XueshengEntity user = xueshengService.selectOne(new EntityWrapper<XueshengEntity>().eq("xuehao", xuesheng.getXuehao()));
if(user!=null) {
return R.error("注册用户已存在");
}
Long uId = new Date().getTime();
xuesheng.setId(uId);
xueshengService.insert(xuesheng);
return R.ok();
}
/**
* 退出
*/
@RequestMapping("/logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
XueshengEntity user = xueshengService.selectById(id);
return R.ok().put("data", user);
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
XueshengEntity user = xueshengService.selectOne(new EntityWrapper<XueshengEntity>().eq("xuehao", username));
if(user==null) {
return R.error("账号不存在");
}
user.setMima("123456");
xueshengService.updateById(user);
return R.ok("密码已重置为:123456");
}
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,XueshengEntity xuesheng,
HttpServletRequest request){
EntityWrapper<XueshengEntity> ew = new EntityWrapper<XueshengEntity>();
PageUtils page = xueshengService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, xuesheng), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,XueshengEntity xuesheng, HttpServletRequest request){
EntityWrapper<XueshengEntity> ew = new EntityWrapper<XueshengEntity>();
PageUtils page = xueshengService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, xuesheng), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( XueshengEntity xuesheng){
EntityWrapper<XueshengEntity> ew = new EntityWrapper<XueshengEntity>();
ew.allEq(MPUtil.allEQMapPre( xuesheng, "xuesheng"));
return R.ok().put("data", xueshengService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(XueshengEntity xuesheng){
EntityWrapper< XueshengEntity> ew = new EntityWrapper< XueshengEntity>();
ew.allEq(MPUtil.allEQMapPre( xuesheng, "xuesheng"));
XueshengView xueshengView = xueshengService.selectView(ew);
return R.ok("查询学生成功").put("data", xueshengView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
XueshengEntity xuesheng = xueshengService.selectById(id);
return R.ok().put("data", xuesheng);
}
/**
* 前端详情
*/
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
XueshengEntity xuesheng = xueshengService.selectById(id);
return R.ok().put("data", xuesheng);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody XueshengEntity xuesheng, HttpServletRequest request){
xuesheng.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(xuesheng);
XueshengEntity user = xueshengService.selectOne(new EntityWrapper<XueshengEntity>().eq("xuehao", xuesheng.getXuehao()));
if(user!=null) {
return R.error("用户已存在");
}
xuesheng.setId(new Date().getTime());
xueshengService.insert(xuesheng);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody XueshengEntity xuesheng, HttpServletRequest request){
xuesheng.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(xuesheng);
XueshengEntity user = xueshengService.selectOne(new EntityWrapper<XueshengEntity>().eq("xuehao", xuesheng.getXuehao()));
if(user!=null) {
return R.error("用户已存在");
}
xuesheng.setId(new Date().getTime());
xueshengService.insert(xuesheng);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody XueshengEntity xuesheng, HttpServletRequest request){
//ValidatorUtils.validateEntity(xuesheng);
xueshengService.updateById(xuesheng);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
xueshengService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 提醒接口
*/
@RequestMapping("/remind/{columnName}/{type}")
public R remindCount(@PathVariable("columnName") String columnName, HttpServletRequest request,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate
没有合适的资源?快使用搜索试试~ 我知道了~
springboot师生健康信息管理系统.zip
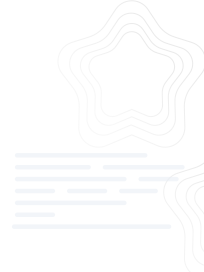
共421个文件
svg:161个
java:114个
vue:44个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 95 浏览量
2024-11-02
09:03:08
上传
评论
收藏 8.75MB ZIP 举报
温馨提示
随着校园疫情的常态化,为了更有效地监测和管理师生的健康状况,我们开发了这款师生健康信息管理系统。该系统旨在通过信息化手段,实时掌握师生的健康数据,确保校园的安全与稳定。 系统包含多个核心功能模块。首先,“首页”作为用户访问的入口,提供了系统的概览和导航功能。用户可以通过点击“个人中心”查看和修改个人信息,包括修改密码等。此外,“学生管理”和“教师管理”模块分别用于管理学生和教师的健康信息,包括健康打卡、体温记录等。 “信息采集管理”模块用于收集和处理师生的基本信息和健康数据,确保数据的准确性和完整性。而“问卷分类管理”、“疫情问卷管理”和“问卷调查管理”则用于制定和发布各类问卷,以收集师生的健康反馈和意见,为校园疫情防控提供数据支持。 最后,“返校信息管理”模块用于管理和追踪师生的返校情况,包括返校申请、审批流程等,确保返校工作的顺利进行。此外,系统还提供了“数据采集管理”功能,用于对各类健康数据进行整合和分析,为校园疫情防控提供科学依据。 整个系统设计简洁明了,操作便捷,旨在为用户提供高效、便捷的健康信息管理服务。
资源推荐
资源详情
资源评论
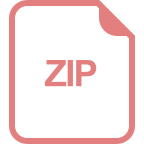
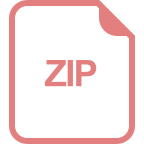
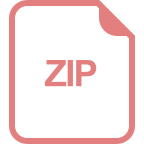
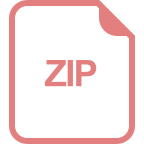
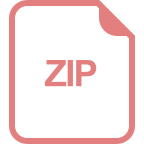
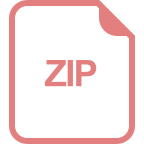
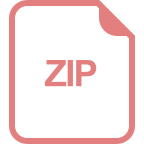
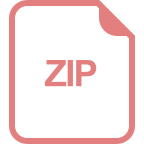
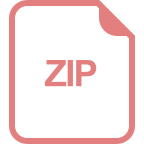
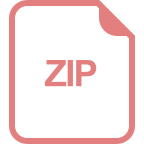
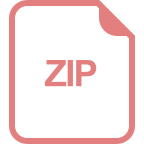
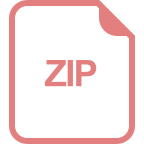
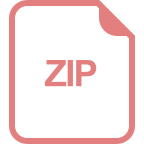
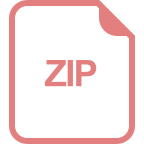
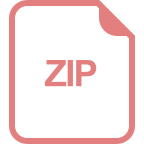
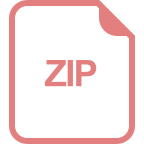
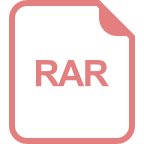
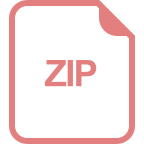
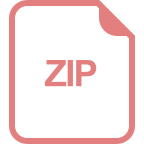
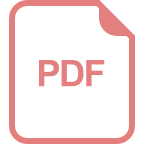
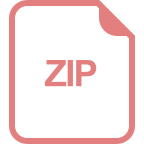
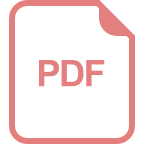
收起资源包目录

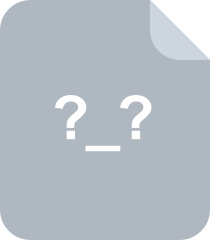
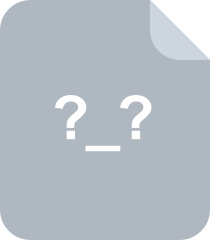
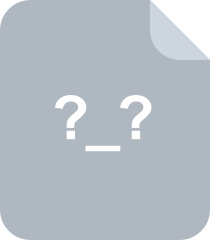
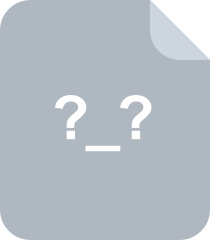
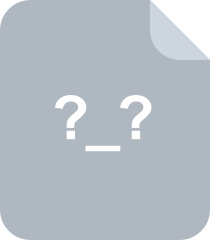
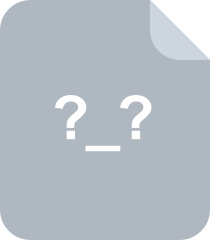
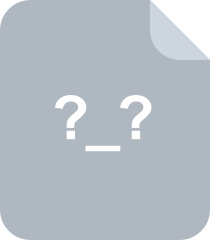
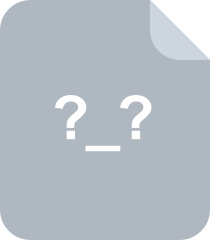
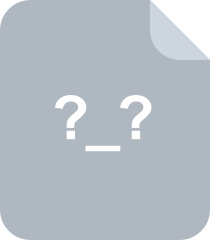
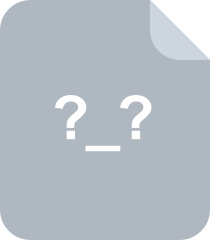
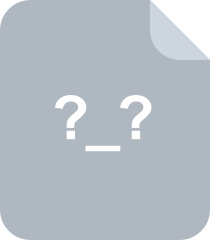
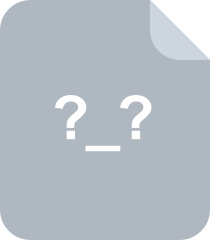
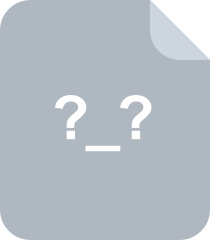
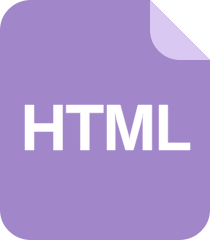
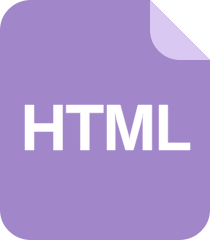
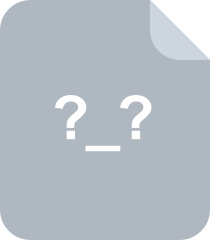
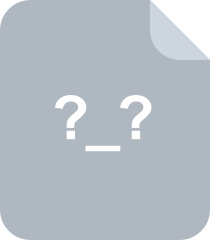
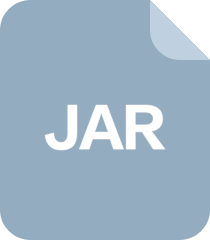
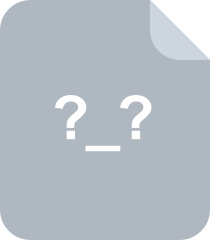
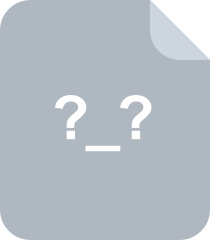
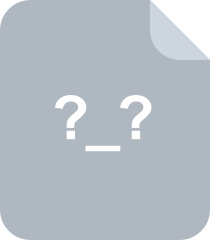
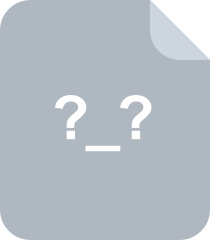
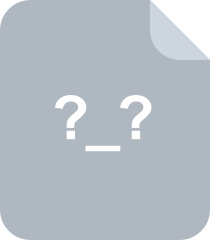
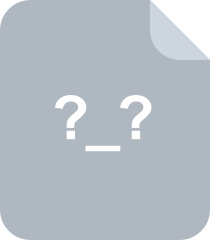
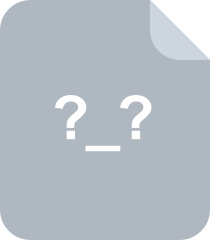
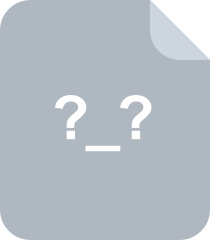
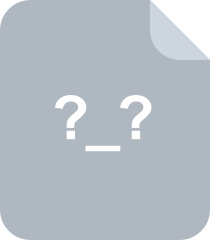
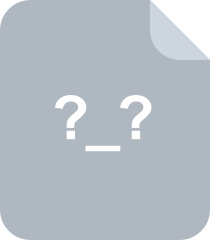
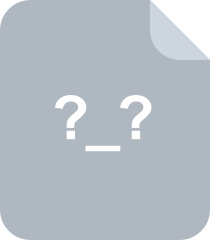
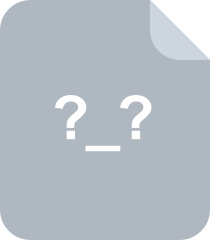
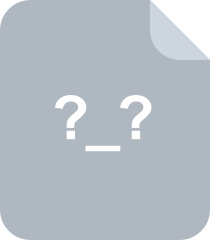
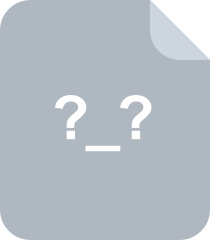
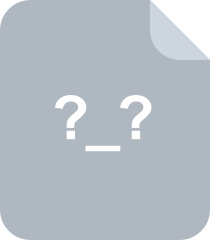
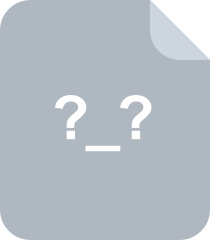
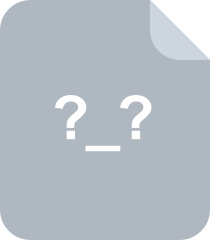
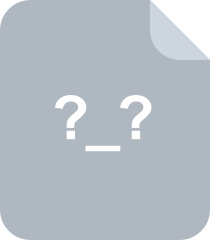
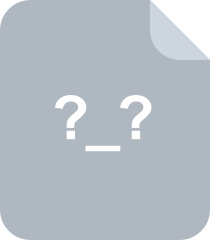
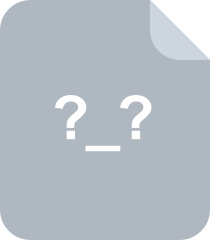
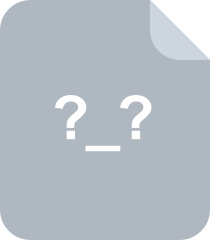
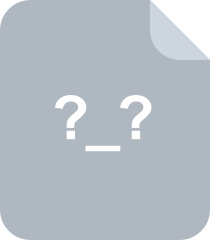
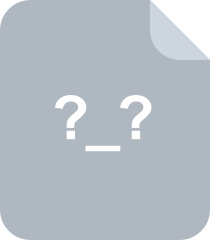
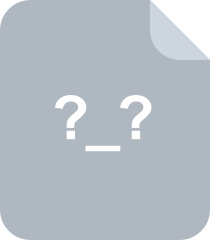
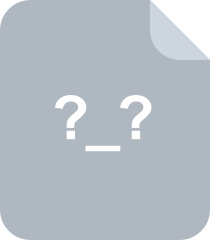
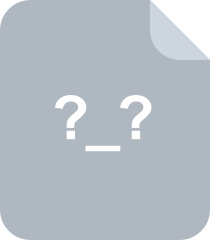
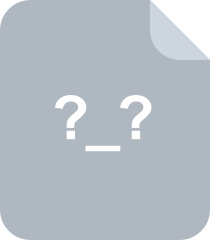
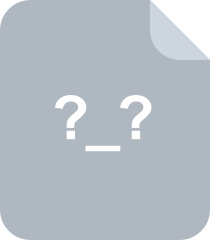
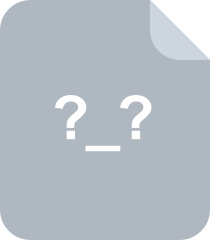
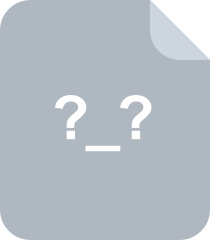
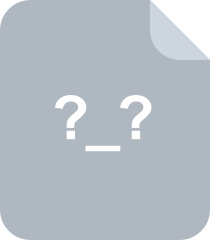
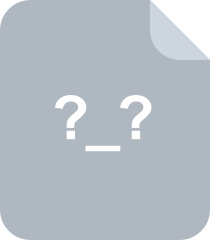
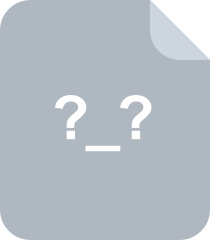
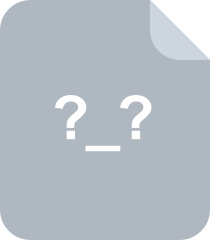
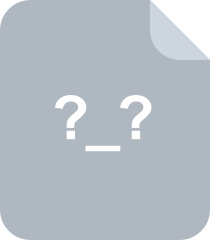
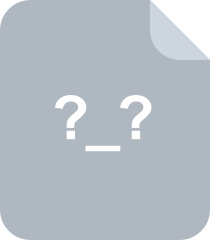
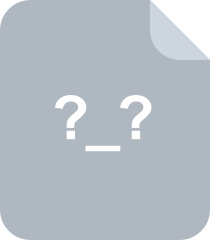
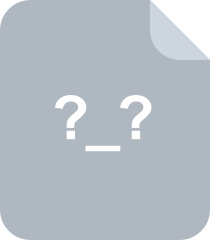
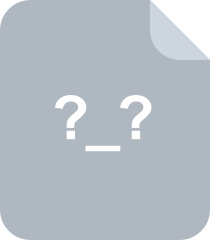
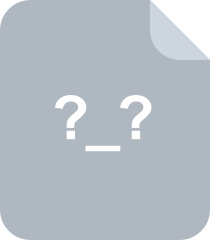
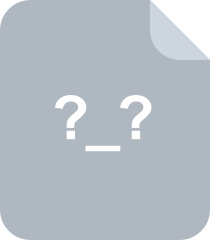
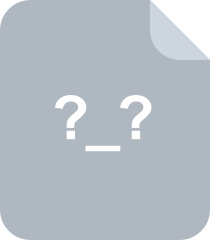
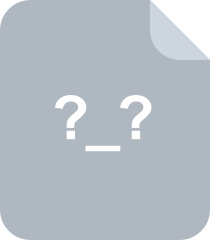
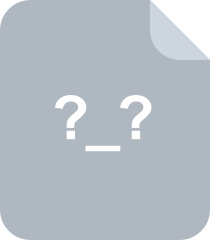
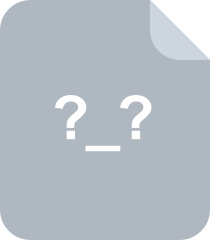
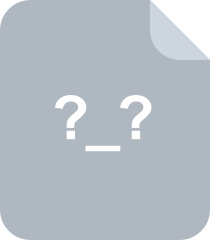
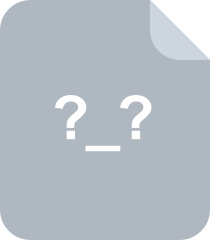
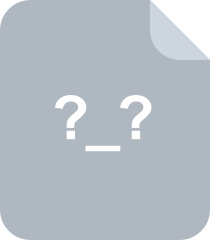
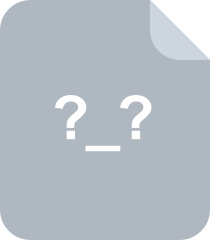
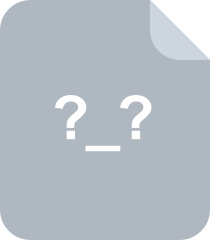
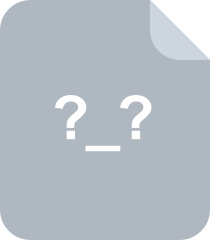
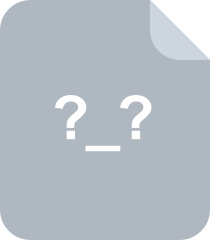
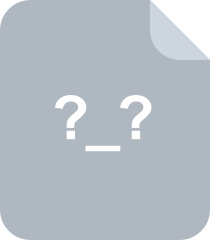
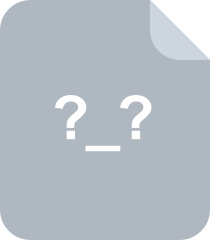
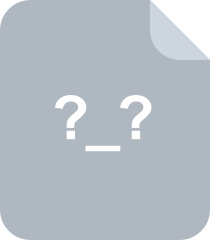
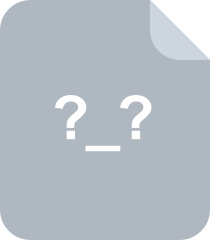
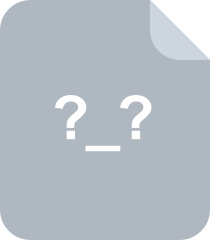
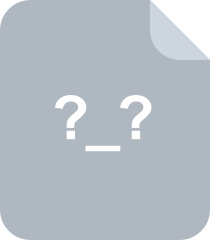
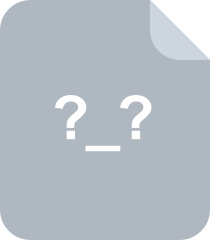
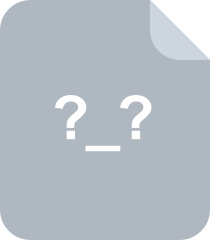
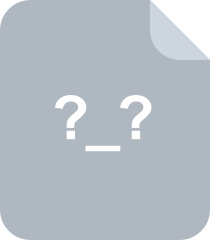
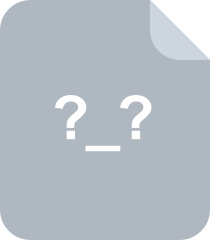
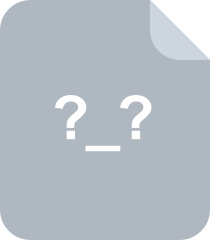
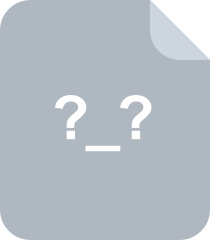
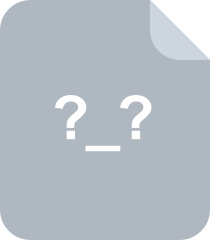
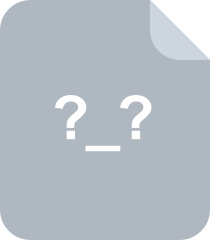
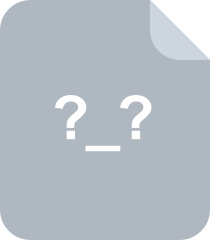
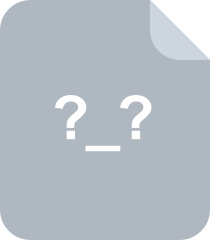
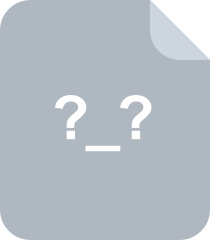
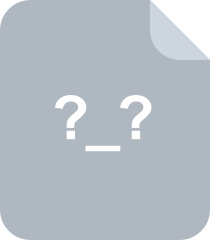
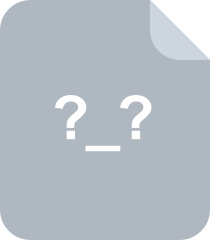
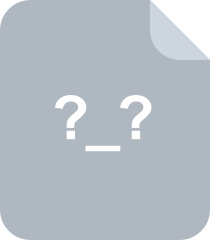
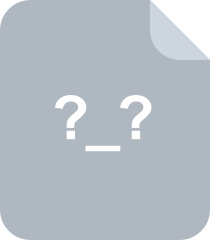
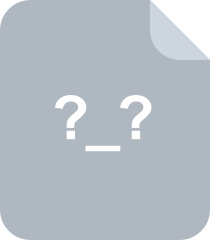
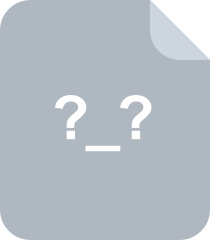
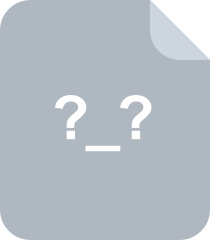
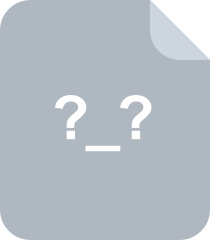
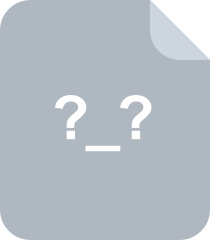
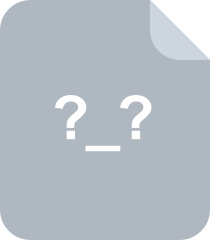
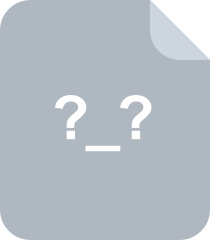
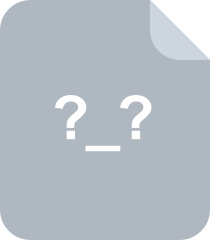
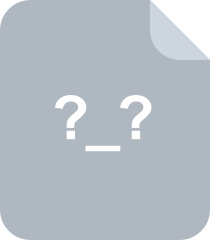
共 421 条
- 1
- 2
- 3
- 4
- 5
资源评论
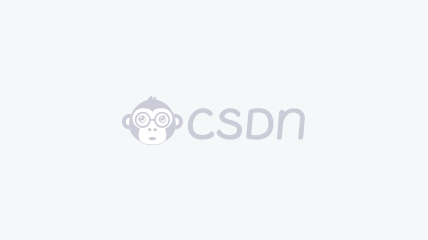

计算机学长阿伟
- 粉丝: 2882
- 资源: 505
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

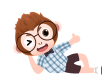
最新资源
- 计算机毕业设计-基于Python+Django的社区爱心养老管理系统(源码+运行教程+讲解+演示视频).zip
- funasr-wss-client.cpp
- 《探索哈夫曼树:数据结构中的瑰宝》pdf
- 定制圣诞树:在Python中为枝叶创建多样形状和尺寸
- 计算机毕业设计-基于Python+Django的个性化餐饮管理系统(源码+运行教程+讲解+演示视频).zip
- 使用 Win32 和 COM API 以 C++ 编写桌面程序
- 数据库分区技术详解:原理、实践与性能优化
- 肥胖风险分析数据集文件
- 计算机毕业设计-基于Python+Django的花卉商城系统(源码+运行教程+讲解+演示视频).rar
- 基于python3 + django3 + mysql8 + redis + uwsgi + nginx 实现的多主题博客系统
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


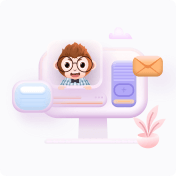
安全验证
文档复制为VIP权益,开通VIP直接复制
