package com.controller;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.*;
import javax.servlet.http.HttpServletRequest;
import com.alibaba.fastjson.JSON;
import com.utils.StringUtil;
import org.apache.commons.lang3.StringUtils;
import org.json.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.ResourceUtils;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.annotation.IgnoreAuth;
import com.baidu.aip.face.AipFace;
import com.baidu.aip.face.MatchRequest;
import com.baidu.aip.util.Base64Util;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.entity.ConfigEntity;
import com.service.CommonService;
import com.service.ConfigService;
import com.utils.BaiduUtil;
import com.utils.FileUtil;
import com.utils.R;
/**
* 通用接口
*/
@RestController
public class CommonController{
private static final Logger logger = LoggerFactory.getLogger(CommonController.class);
@Autowired
private CommonService commonService;
@Autowired
private ConfigService configService;
private static AipFace client = null;
private static String BAIDU_DITU_AK = null;
@RequestMapping("/location")
public R location(String lng,String lat) {
if(BAIDU_DITU_AK==null) {
BAIDU_DITU_AK = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "baidu_ditu_ak")).getValue();
if(BAIDU_DITU_AK==null) {
return R.error("请在配置管理中正确配置baidu_ditu_ak");
}
}
Map<String, String> map = BaiduUtil.getCityByLonLat(BAIDU_DITU_AK, lng, lat);
return R.ok().put("data", map);
}
/**
* 人脸比对
*
* @param face1 人脸1
* @param face2 人脸2
* @return
*/
@RequestMapping("/matchFace")
public R matchFace(String face1, String face2, HttpServletRequest request) {
if(client==null) {
/*String AppID = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "AppID")).getValue();*/
String APIKey = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "APIKey")).getValue();
String SecretKey = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "SecretKey")).getValue();
String token = BaiduUtil.getAuth(APIKey, SecretKey);
if(token==null) {
return R.error("请在配置管理中正确配置APIKey和SecretKey");
}
client = new AipFace(null, APIKey, SecretKey);
client.setConnectionTimeoutInMillis(2000);
client.setSocketTimeoutInMillis(60000);
}
JSONObject res = null;
try {
File file1 = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+face1);
File file2 = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+face2);
String img1 = Base64Util.encode(FileUtil.FileToByte(file1));
String img2 = Base64Util.encode(FileUtil.FileToByte(file2));
MatchRequest req1 = new MatchRequest(img1, "BASE64");
MatchRequest req2 = new MatchRequest(img2, "BASE64");
ArrayList<MatchRequest> requests = new ArrayList<MatchRequest>();
requests.add(req1);
requests.add(req2);
res = client.match(requests);
System.out.println(res.get("result"));
} catch (FileNotFoundException e) {
e.printStackTrace();
return R.error("文件不存在");
} catch (IOException e) {
e.printStackTrace();
}
return R.ok().put("data", com.alibaba.fastjson.JSONObject.parse(res.get("result").toString()));
}
/**
* 获取table表中的column列表(联动接口)
* @return
*/
@RequestMapping("/option/{tableName}/{columnName}")
@IgnoreAuth
public R getOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,String level,String parent) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
if(StringUtils.isNotBlank(level)) {
params.put("level", level);
}
if(StringUtils.isNotBlank(parent)) {
params.put("parent", parent);
}
List<String> data = commonService.getOption(params);
return R.ok().put("data", data);
}
/**
* 根据table中的column获取单条记录
* @return
*/
@RequestMapping("/follow/{tableName}/{columnName}")
@IgnoreAuth
public R getFollowByOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName, @RequestParam String columnValue) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
params.put("columnValue", columnValue);
Map<String, Object> result = commonService.getFollowByOption(params);
return R.ok().put("data", result);
}
/**
* 修改table表的sfsh状态
* @param map
* @return
*/
@RequestMapping("/sh/{tableName}")
public R sh(@PathVariable("tableName") String tableName, @RequestBody Map<String, Object> map) {
map.put("table", tableName);
commonService.sh(map);
return R.ok();
}
/**
* 获取需要提醒的记录数
* @param tableName
* @param columnName
* @param type 1:数字 2:日期
* @param map
* @return
*/
@RequestMapping("/remind/{tableName}/{columnName}/{type}")
@IgnoreAuth
public R remindCount(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("table", tableName);
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindStart);
remindStartDate = c.getTime();
map.put("remindstart", sdf.format(remindStartDate));
}
if(map.get("remindend")!=null) {
Integer remindEnd = Integer.parseInt(map.get("remindend").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindEnd);
remindEndDate = c.getTime();
map.put("remindend", sdf.format(remindEndDate));
}
}
int count = commonService.remindCount(map);
return R.ok().put("count", count);
}
/**
* 圖表统计
*/
@IgnoreAuth
@RequestMapping("/group/{tableName}")
public R group1(@PathVariable("tableName") String tableName, @RequestParam Map<String,Object> params) {
params.put("table1", tableName);
List<Map<String, Object>> result = commonService.chartBoth(params);
return R.ok().put("data", result);
}
/**
* 单列求和
*/
@RequestMapping("/cal/{tableName}/{columnName}")
@IgnoreAuth
public R cal(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
Map<String, Object> result = commonService.selectCal(params);
return R.ok().put("data", result);
}
/**
* 分组统计
*/
@RequestMapping("/group/{tableName}/{columnName}")
@IgnoreAuth
public R group(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableN
没有合适的资源?快使用搜索试试~ 我知道了~
科研工作量管理系统的设计与实现.zip
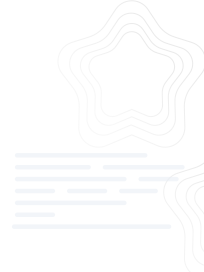
共802个文件
svg:322个
vue:108个
class:101个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 76 浏览量
2024-10-31
16:25:57
上传
评论
收藏 32.19MB ZIP 举报
温馨提示
科研工作量管理系统的出现,旨在解决科研机构或高校在科研管理工作中所面临的种种挑战,如工作量统计不准确、管理效率低下等问题。该系统通过集成多个功能模块,为科研人员和管理人员提供了一个全面、高效的管理平台。 系统界面设计简洁明了,以棕色为主色调,营造出专业和正式的氛围。上方导航栏包括“首页”和“个人中心”等选项,方便用户快速访问所需功能。在页面的左侧,则列出了系统的核心功能模块,包括“基础数据管理”、“教师管理”、“秘书管理”、“科研获奖管理”、“科研论文管理”以及“科研项目管理”等。 “基础数据管理”模块负责维护系统所需的基础数据,如科研人员信息、科研项目类别等,为其他模块提供数据支持。而“教师管理”和“秘书管理”模块则分别针对科研人员和行政管理人员,提供了个性化的管理功能,如工作量统计、任务分配等。 此外,“科研获奖管理”、“科研论文管理”和“科研项目管理”模块则是系统的重点。它们分别负责记录和管理科研成果的获奖情况、发表的科研论文以及进行中的科研项目,支持数据的录入、查询、统计和分析等功能,帮助科研机构或高校更好地掌握科研成果的产出情况,提高管理效率。
资源推荐
资源详情
资源评论
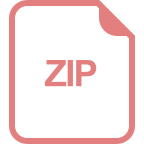
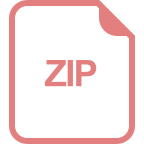
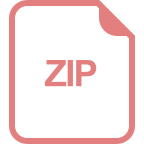
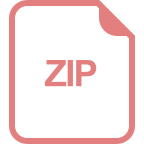
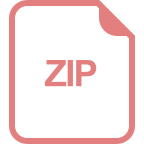
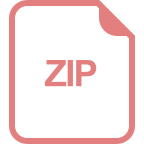
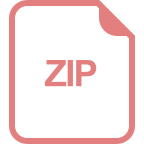
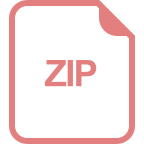
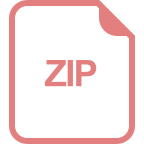
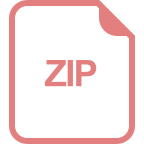
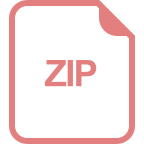
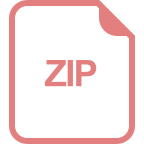
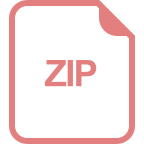
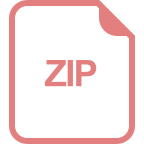
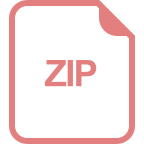
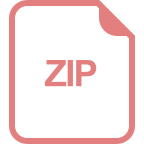
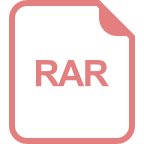
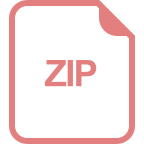
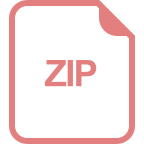
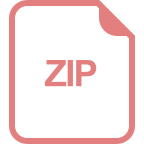
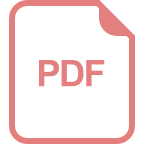
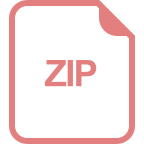
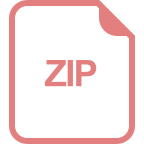
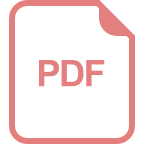
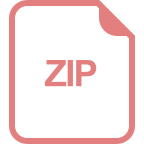
收起资源包目录

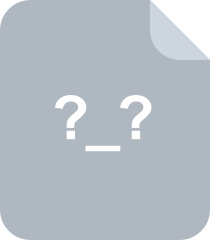
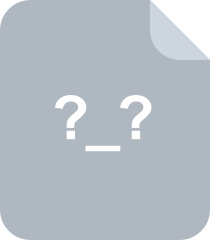
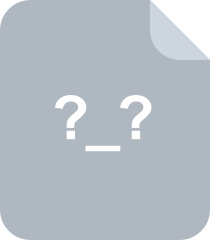
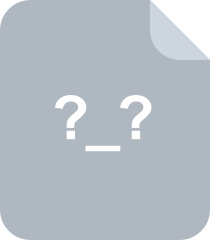
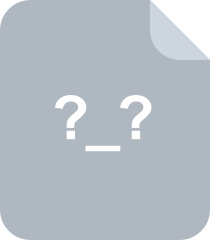
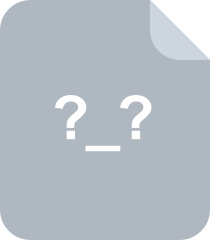
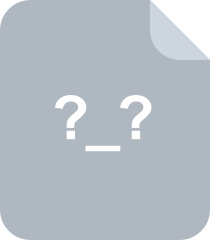
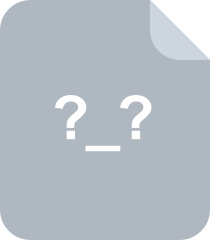
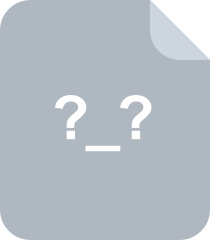
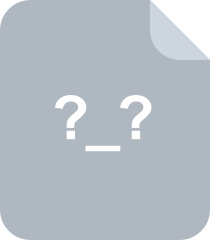
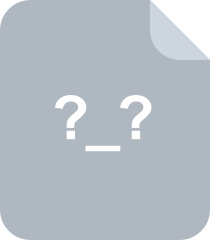
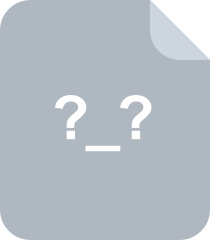
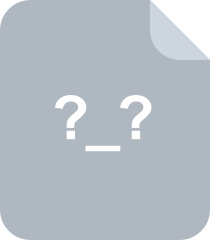
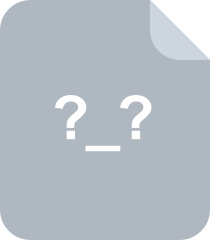
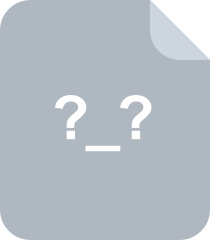
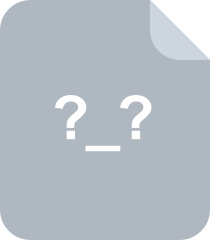
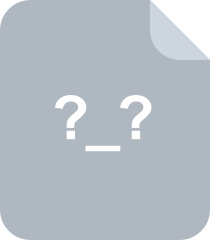
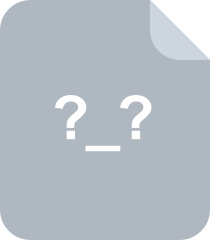
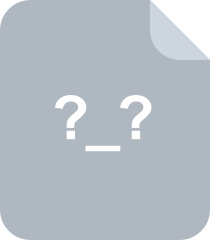
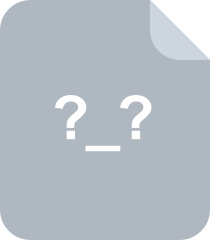
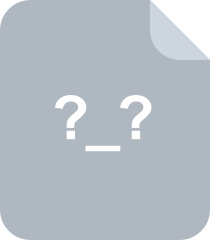
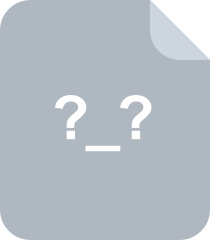
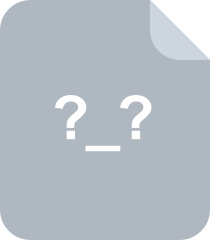
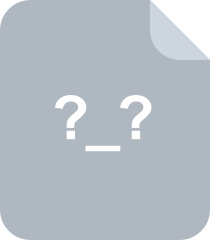
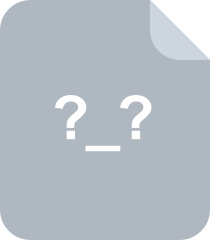
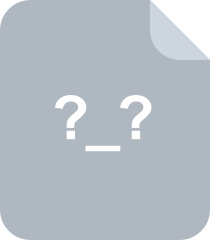
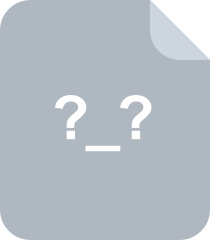
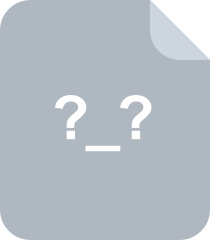
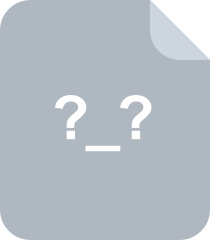
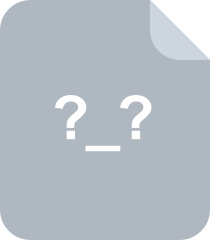
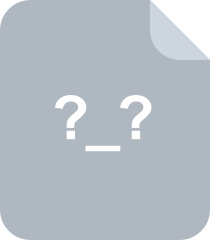
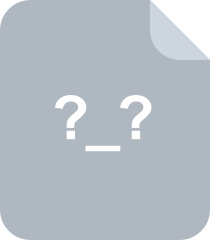
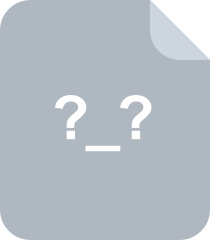
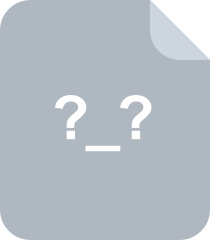
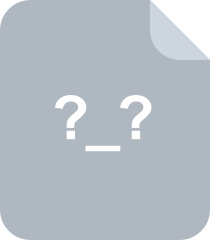
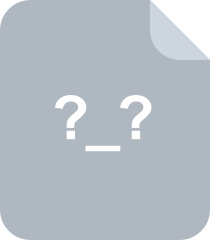
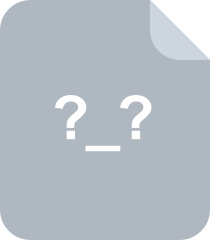
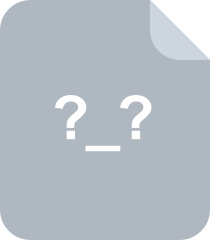
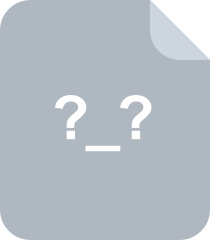
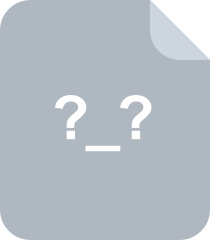
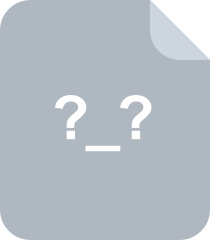
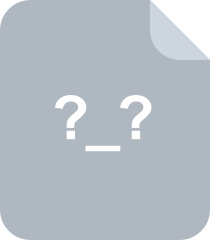
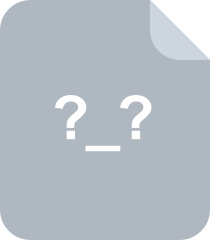
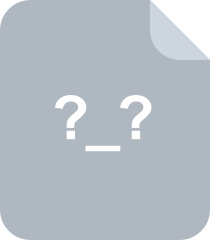
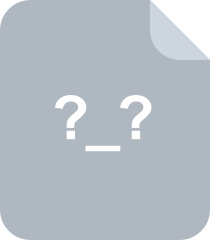
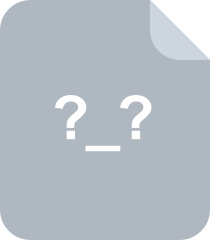
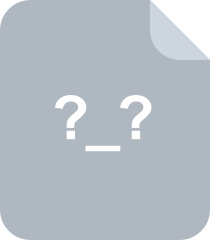
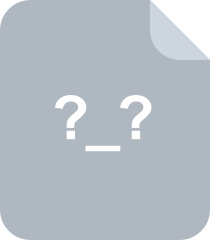
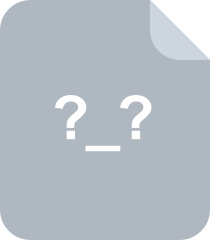
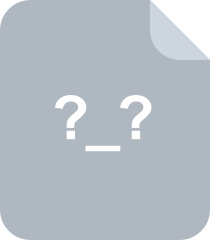
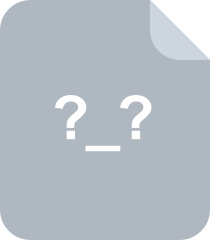
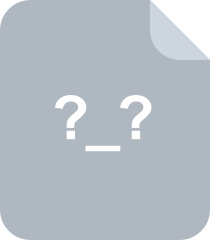
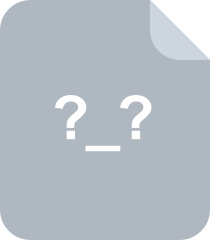
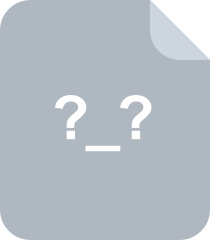
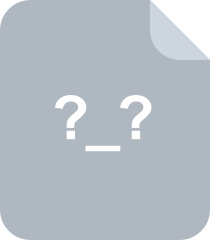
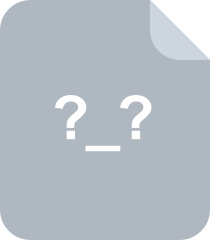
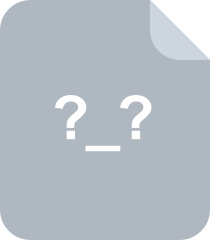
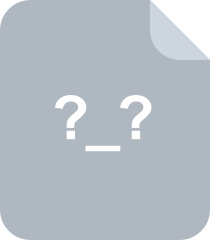
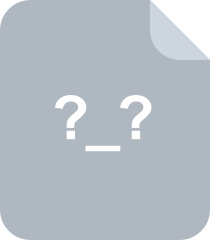
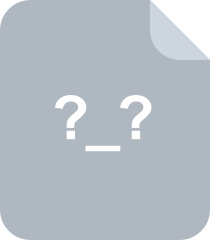
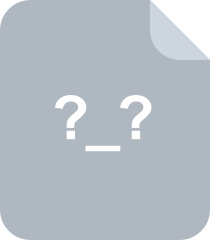
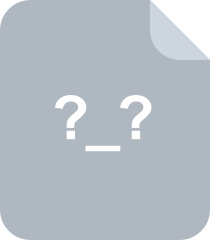
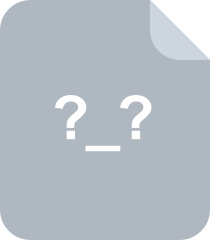
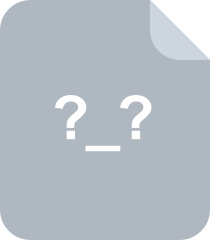
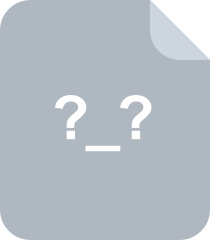
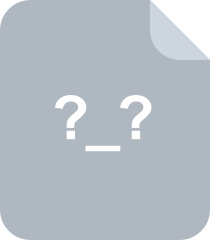
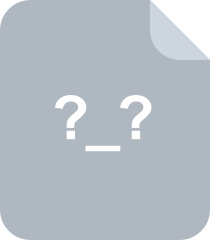
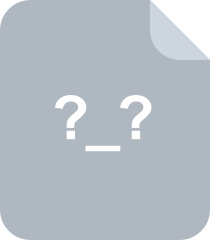
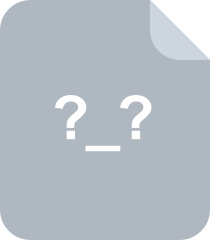
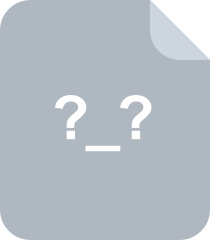
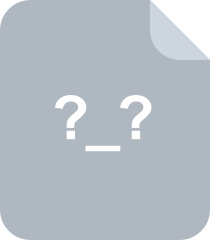
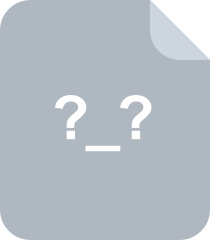
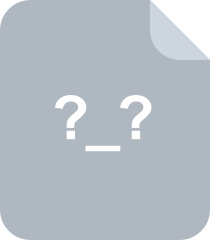
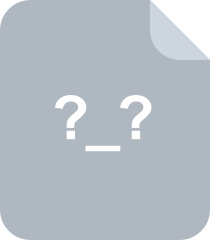
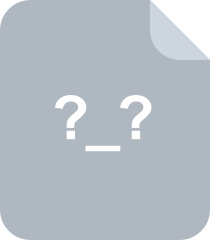
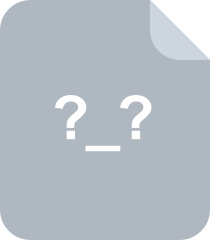
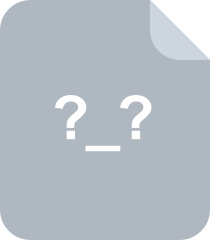
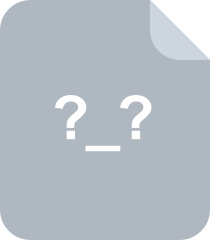
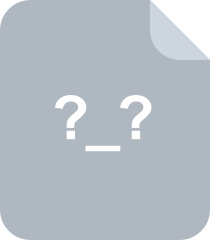
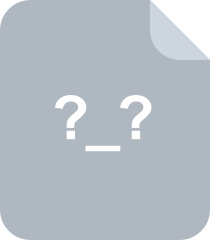
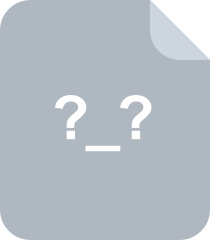
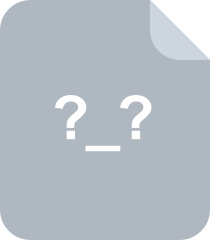
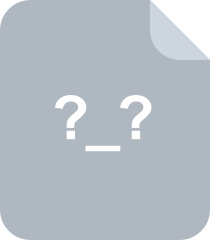
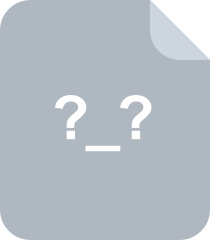
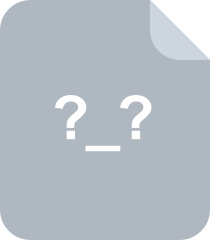
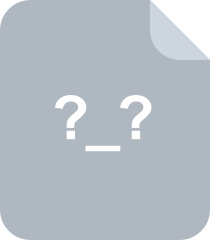
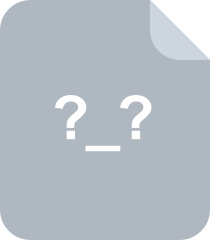
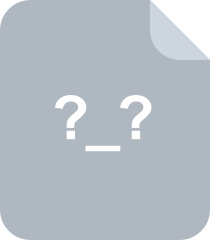
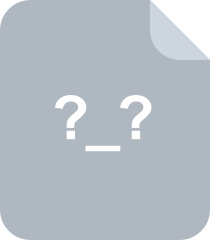
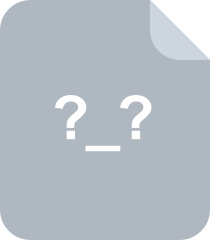
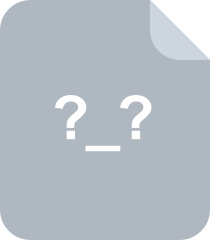
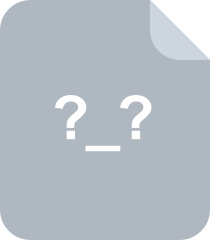
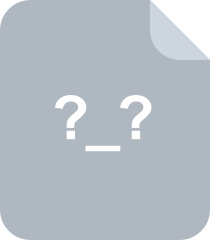
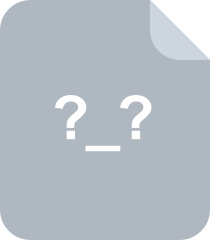
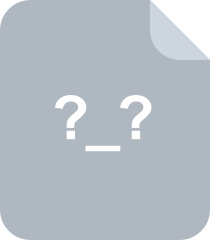
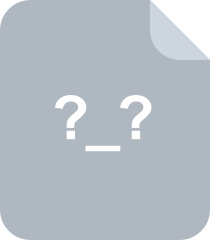
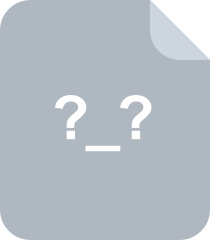
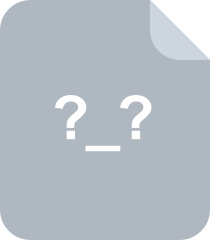
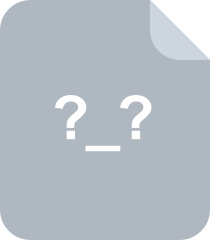
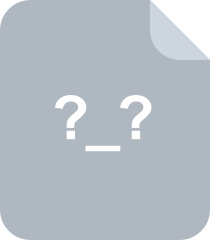
共 802 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
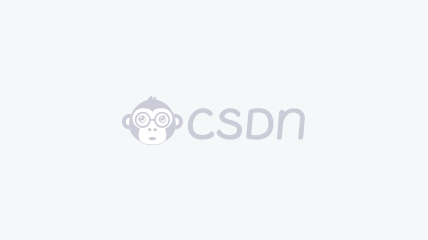

计算机学长阿伟
- 粉丝: 2717
- 资源: 481
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

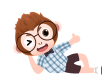
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


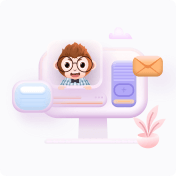
安全验证
文档复制为VIP权益,开通VIP直接复制
