import random
import tkinter as tk
from tkinter import *
from tkinter import messagebox, ttk
import pymysql as pymysql
'''登录操作'''
def login(cursor, username, password, entered_code, expected_code):
if username == '' or password == '' or entered_code == '':
messagebox.showerror('Error', '请输入完整信息!')
return
if entered_code.upper() != expected_code.upper():
messagebox.showerror('Error', '验证码错误!')
return
ret = cursor.execute("SELECT * FROM ROOTPSW_INFO WHERE 用户名=%s AND 密码 = %s", [username, password])
if ret:
messagebox.showinfo('Success', '登录成功')
student_management_system()
# 进行其他操作
else:
messagebox.showerror('Error', '用户名或密码错误,请注册!')
'''注册操作'''
def register():
# 连接数据库
db = pymysql.connect(host='localhost', user='root', password='111111', database='information_login')
cursor = db.cursor()
# 注册功能
def confirm():
# 获取注册页面输入框信息
username = register_entry1.get()
password = register_entry2.get()
confirm_password = register_entry3.get()
# 检查用户名是否存在
cursor.execute("SELECT 用户名 FROM ROOTPSW_INFO WHERE 用户名=%s", [username])
existing_username = cursor.fetchone()
if len(password) != 6:
messagebox.showerror('失败', '密码必须是6位')
elif password != confirm_password:
messagebox.showerror('失败', '两次输入密码不同')
elif existing_username:
messagebox.showerror('失败', '用户名已存在')
else:
# 执行数据库语句以存入用户名密码
sql_insert = "INSERT INTO ROOTPSW_INFO(用户名,密码) VALUE(%s,%s)"
cursor.execute(sql_insert, (username, password))
db.commit()
messagebox.showinfo('成功', '注册成功')
second_page.destroy()
# 关闭窗口
def cancel():
second_page.destroy()
second_page = tk.Toplevel()
second_page.title('注册账号')
second_page.geometry('400x600+550+100')
second_page.maxsize(400, 600)
second_page.minsize(400, 600)
canvas = tk.Canvas(second_page, width=400, height=600)
image_file_register = tk.PhotoImage(file="image/4.gif")
canvas.create_image(190, 630, anchor='s', image=image_file_register)
canvas.pack()
register_title = tk.Label(second_page, text='注册页面', bg='cadetblue',activeforeground='purple')
register_title.place(x=58, y=30, width=300, height=40)
# 用户名
register_name = tk.Label(second_page, text=' 用户名 :', bg='khaki')
register_name.place(x=80, y=300, width=50, height=30)
register_entry1 = tk.Entry(second_page)
register_entry1.place(x=135, y=305)
# 密码
register_psw = tk.Label(second_page, text='密 码 :', bg='khaki')
register_psw.place(x=81, y=340, width=50, height=30)
register_entry2 = tk.Entry(second_page, show='*')
register_entry2.place(x=135, y=345)
register_psw_info = tk.Label(second_page, text='请输入6位密码')
register_psw_info.place(x=285, y=345)
# 确认密码
register_psw1 = tk.Label(second_page, text='确认密码:', bg='khaki')
register_psw1.place(x=81, y=380, width=50, height=30)
register_entry3 = tk.Entry(second_page, show='*')
register_entry3.place(x=135, y=385)
# 确认按钮
register_button1 = tk.Button(second_page, text='确认', command=confirm)
register_button1.place(x=125, y=440, width=50, height=30)
# 取消按钮
register_button1 = tk.Button(second_page, text='取消', command=cancel)
register_button1.place(x=230, y=440, width=50, height=30)
second_page.mainloop()
# 关闭数据库连接
cursor.close()
db.close()
"""管理操作"""
def student_management_system():
"""连接数据库"""
db = pymysql.Connect(host='localhost', user='root', password='111111', database='information_login')
cursor = db.cursor()
'''供选择的班级名'''
class_values = ["一班", "二班", "三班", "四班"]
def addinfo():
def add_confirm():
xm = text_xm.get()
xh = text_xh.get()
classname = text_class.get()
ret_add = cursor.execute("SELECT xh FROM STUDENT_INFO WHERE xh=%s", xh)
if ret_add:
messagebox.showerror('windows', '此学号存在,若需修改请前往修改页面')
add_window.destroy()
else:
if xm != '' and xh != '' and classname != '' and len(xh) == 10:
cursor.execute("INSERT INTO STUDENT_INFO(xm,xh,class)VALUE(%s,%s,%s)", (xm, xh, classname))
db.commit()
add_window.destroy()
messagebox.showinfo('windows', '添加成功')
else:
messagebox.showwarning('windows', '输入不能为空且学号长度10位')
def add_cancel():
add_window.destroy()
add_window = Toplevel()
add_window.title('添加学生信息')
add_window.geometry('300x200+560+300')
add_window.resizable(0, 0)
add_label1 = Label(add_window, text='姓名:')
add_label1.place(x=50, y=20)
add_label2 = Label(add_window, text='学号:')
add_label2.place(x=50, y=60)
add_label3 = Label(add_window, text='班级:')
add_label3.place(x=52, y=100)
add_label4 = Label(add_window, text='10位学号', bg='darkgray')
add_label4.place(x=240, y=60)
text_xm = Entry(add_window)
text_xm.place(x=90, y=20)
text_xh = Entry(add_window)
text_xh.place(x=90, y=60)
text_class = ttk.Combobox(add_window, state='readonly', values=class_values)
text_class.place(x=90, y=100, width=150)
add_button1 = Button(add_window, text='确认', command=add_confirm)
add_button1.place(x=88, y=136, width=50)
add_button2 = Button(add_window, text='取消', command=add_cancel)
add_button2.place(x=173, y=135, width=50)
add_window.mainloop()
def updateinfo():
def jiansuo():
text_update_entry = update_entry_xh.get()
ret = cursor.execute("SELECT * FROM student_info WHERE xh=%s", text_update_entry)
if ret:
get_mysql_infomation = cursor.fetchone()
jiansuo_text1.set(get_mysql_infomation[0])
jiansuo_text2.set(get_mysql_infomation[1])
jiansuo_text3.set(get_mysql_infomation[2])
def update_confirm():
text_update_entry = update_entry_xh.get()
text_update_xm = update_entry2.get()
text_update_class = update_entry3.get()
ret2 = cursor.execute("SELECT * FROM student_info WHERE xh=%s", text_update_entry)
if ret2:
cursor.execute("UPDATE student_info SET xm=%s,class=%s WHERE xh=%s",
[text_update_xm, text_update_class, text_update_entry])
db.commit()
messagebox.showinfo('windows', '添加成功!')
update_window.destroy()
elif text_update_xm == '' and text_update_entry == '' and text_update_class == '':
messagebox.showerror('windows', '不能为空!')
else:
messagebox.showwarning('windows', '此学号未被添加!')
def update_cancel():
update_window.destroy()
update_window = Toplevel()
update_window.title('修改学生信息')
update_window.geometry('300x240+560+300')
update_window.resizable(0, 0)
jiansuo_text1 = StringVar()
jiansuo_text2 = StringVar()
jiansuo_text3 = StringVar()
update_label1 = Label(update_window, text='学生学号:')
update_la
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本资源使用python中tkinter库ui设计(含登陆页面、注册页面、信息管理页面)。登陆账号密码核对、验证码、注册登录信息、添加学生信息、删除学生信息、修改(更新)学生信息、管理员按钮实现展示所有信息等功能。 通过连接个人MySQL数据库进行存储登陆账号密码,并实现从所存储的表中读取学生姓名学号班级等信息,进行增删改查。
资源推荐
资源详情
资源评论
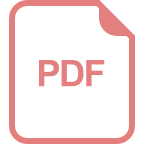
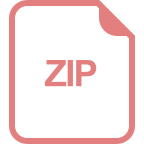
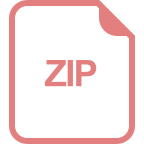
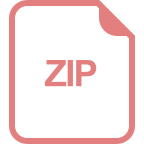
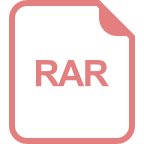
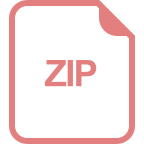
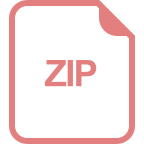
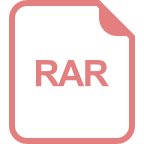
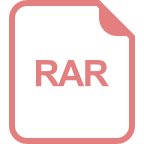
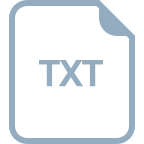
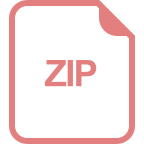
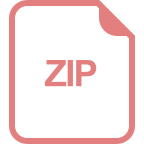
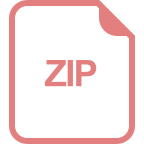
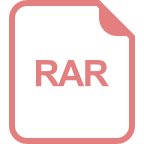
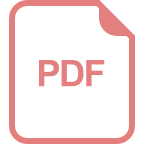
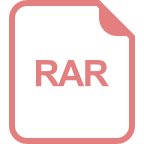
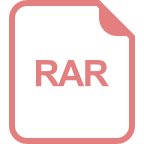
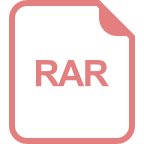
收起资源包目录


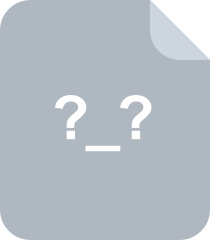
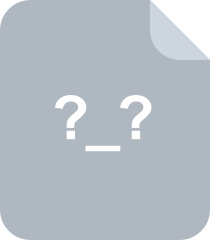

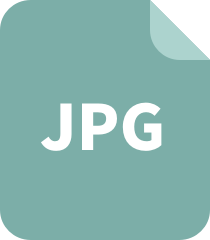
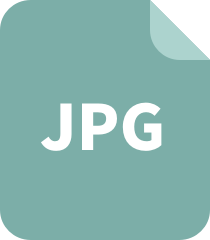
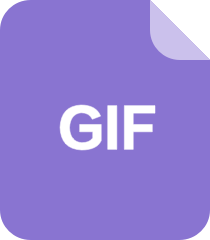
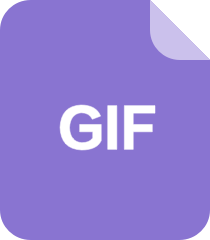
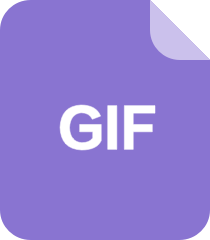

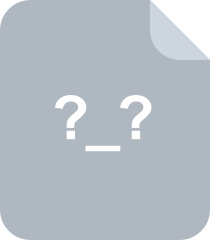
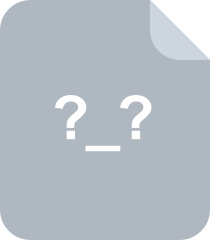
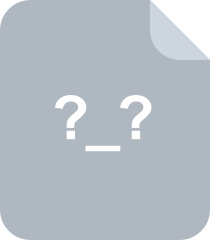

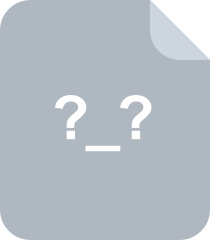
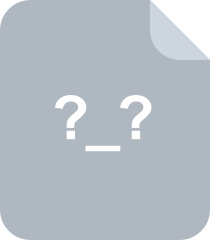
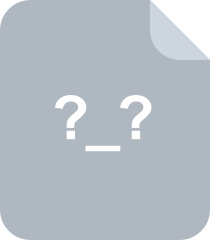
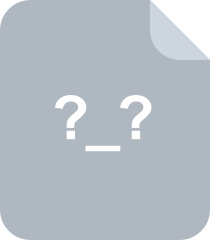
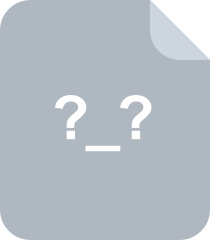

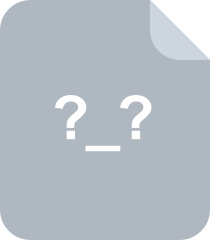
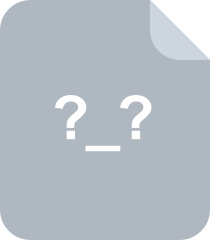
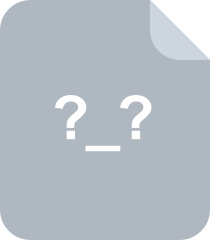
共 18 条
- 1

csaclw
- 粉丝: 10
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

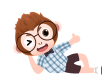
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页