#link to other classes by importing
from person import *
from account import *
#import needed libaries
import pickle
import tkinter as tk
from tkinter import messagebox
class BankSystem(object):
def __init__(self):
self.customerList = []
self.adminList = []
self.loadBankData() #used to received bank data when testing
self.save() #saves loadBankData into a file
self.load() #loads bank data from a file
def save(self):
#put customer and admin list into another list
data1 = (self.adminList, self.customerList)
#makes a file and writes bank data into it
output = open('bankData.pkl', 'wb')
pickle.dump(data1, output)
output.close()
def load(self):
#loads bank data from another file, then closes that file
pkl_file = open('bankData.pkl', 'rb')
data = pickle.load(pkl_file)
pkl_file.close()
#set the admin and customer list
self.adminList = data[0]
self.customerList = data[1]
def loadBankData(self):
#create customers and add them to the cusomterList
#create specific accounts for customers
#used to reset data when testing
customer_1 = Customer("amin", "1234", ["14", " Street2", "tabriz" , "hesabjari"])
account_no = 90455673
account_1 = hesabjari(account_no, "hesabjari", "1234", 5000)
customer_1.openAccount(account_1)
self.customerList.append(customer_1)
customer_2 = Customer("Davood", "password", ["60", "Street1", "tehran" , "hesabboland"])
account_no += 1
account_2 = hesabjari(account_no, "hesabjari", "2601", 3200)
account_no += 1
account_3 = hesabboland(account_no, "hesabboland", "2601", 600)
customer_2.openAccount(account_2)
customer_2.openAccount(account_3)
self.customerList.append(customer_2)
customer_3 = Customer("Niloo", "moonlight", ["5", "Street3", "tabriz", "hesabkotah"])
account_no += 1
account_4 = hesabkotah(account_no, "hesabkotah", "1010", 18000)
customer_3.openAccount(account_4)
self.customerList.append(customer_3)
customer_4 = Customer("Ali", "150A",["44", "nishan tashi", "istanbul", "hesabjari"])
account_no+= 1
account_5 = hesabjari(account_no, "hesabjari", "6666", 50)
customer_4.openAccount(account_5)
self.customerList.append(customer_4)
#create admins and add them to admins_list
admin_1 = Admin("ahora", "1441", True, ["12", "maqsodiye", "tehran" , ""])
self.adminList.append(admin_1)
def customerLogin(self, name, password):
#check the data inputed
foundCustomer = self.searchCustomersByName(name)
if foundCustomer == None:
return("The customer has not been found! \n")
else:
#make sure the correct password is entered
if (foundCustomer.checkPassword(password) == True):
self.runCustomerOptions(foundCustomer)
else:
return("you have input a wrong password \n")
def searchCustomersByName(self, customerName):
#finds a customer from the customers_list and compares wuth inputted data
foundCustomer = None
for a in self.customerList:
name = a.getName()
if name == customerName:
foundCustomer = a
break
if foundCustomer == None:
print("The customer %s does not exist! Try again...\n" %customerName)
#returns the found_customer to be used customer_login
return foundCustomer
def mainMenu(self):
#print the options you have
print()
print()
print ("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
print ("Welcome to the EN Simple Bank System")
print ("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
print ("1) Admin login")
print ("2) Customer login")
print ("3) Quit")
print (" ")
while True:
try:
option = int(input("\nChoose your option: "))
break
except ValueError:
print("Option not valid. Try again. \n")
return option
def runMainOption(self):
#options when code first runs
loop = 1
while loop == 1:
choice = self.mainMenu()
if choice == 1:
name = input("\nPlease input admin name: ")
password = input("\nPlease input admin password: ")
#calls admin_login to check the data inputted
msg = self.adminLogin(name, password)
print(msg)
elif choice == 2:
name = input("\nPlease input customer name: ")
password = input("\nPlease input customer password: ")
#calls customer_login to check data inputted
msg = self.customerLogin(name, password)
print(msg)
elif choice == 3:
#uses a GUI for a message box to confirm if the user wants to quit
root = tk.Tk()
if messagebox.askyesno("Quit", "Are you sure you want to quit?"):
root.destroy() #gets rid of blank GUI box the tkinter makes
self.save() #saves all changes
loop = 0 #quits
else:
print("Option not valid \n")
print ("Thank you for stopping by the bank! \n")
def customerMenu(self, customerName):
#print the options you have when logged in as a customer
print (" ")
print ("Welcome %s : Your transaction options are:" %customerName)
print ("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
print ("1) Account operations")
print ("2) Profile settings")
print ("3) Sign out")
print (" ")
while True:
try:
option = int(input("\nChoose your option: "))
break
except ValueError:
print("Option not valid. Try again.")
return option
def runCustomerOptions(self, customer):
loop = 1
while loop == 1:
choice = self.customerMenu(customer.getName())
if choice == 1:
accName = input("\nPlease input name of account: ")
account = customer.searchAccountByName(accName)
if account == None:
print ("Account could not be found \n")
else:
account.runAccountOptions(customer, self)
elif choice == 2:
customer.runProfileOptions()
elif choice == 3:
loop = 0
print ("Exit account operations \n")
def adminLogin(self, name, password):
#checks admin login same as for customers
foundAdmin = self.searchAdminByName(name)
if foundAdmin== None:
return("The admin has not been found! \n")
else:
if (foundAdmin.checkPassword(password) == True):
self.runAdminOptions(foundAdmin)
else:
return("you have input a wrong password \n")
def searchAdminByName(self, adminName):
#searchs for admins using admin list
foundAdmin = None
for a in self.adminList:
name = a.getName()
if name == adminName:
foundAdmin = a
break
if foundAdmin == None:
print("The admin %s does not exist! Try again...\n" %adminName)
return foundAdmin
def interest(self):
#allows admins to manually apply interest to all accounts
for customer in

小禄Diary
- 粉丝: 3
- 资源: 6
最新资源
- 基于多智能体事件触发的一致性控制:状态轨迹、控制输入与事件触发图详解(附参考图形),基于多智能体事件触发的一致性控制:状态轨迹、控制输入与事件触发机制详解及图形展示,多智能体事件触发、一致性控制 状态
- 基于BP神经网络结合高阶累积量的信号识别技术:实现BPSK、QPSK、8PSK、32QAM信号的准确识别与Matlab实现详解,BP神经网络结合高阶累积量信号识别技术:实现BPSK、QPSK、8PSK
- 大学生校园社团小程序源码云开发前后端完整代码.zip
- 高性能直流无刷电机参数详解:外径41mm,转速高达6000rpm,功率达200W,槽满率67.5%,效率80.7%,最大输出功率达320W,高性能直流无刷电机参数详解:转速高达6000rpm,功率达2
- 小麦种子图像分类数据集【已标注,约2,000张数据】
- 校园帮-WeChat-基于微信小程序的“校园帮”系统设计与实现(毕业论文)
- 多功能工具箱微信小程序源码.zip
- 狼人杀微信娱乐游戏小程序源码.zip
- 基于BP神经网络的回归预测:多个输出数据的分析与MATLAB代码实现,基于BP神经网络的多元输出数据回归预测技术研究与实践-以MATLAB代码为例,基于BP神经网络的多个输出数据的回归预测 matl
- 云开发谁是卧底线下小游戏发牌助手微信小程序源码.zip
- 最新版去水印小程序源码.zip
- 探索各类光波场的仿真与可视化:MATLAB的应用与实践,深入探索:各类光波场在MATLAB环境下的仿真研究与应用,各类光波场的MATLAB仿真 ,光波场仿真; MATLAB仿真; 仿真分析; 模拟;
- https://upload.csdn.net/creation/uploadResources?spm=1011.2124.3001.5646
- 应用商店-安卓-基于Android的应用商店设计与实现(毕业论文)
- 基于高斯过程回归算法的精准时间序列区间预测技术,高斯过程回归在时序区间预测中的应用与研究,基于高斯过程回归(GPR)的时间序列区间预测 ,基于高斯过程回归(GPR); 时间序列; 区间预测,基于高斯过
- 多相交错图腾柱PFC的C代码实现:优化算法与电流纹波控制技术,深度探究多相交错图腾柱PFC的C代码实现:优化算法以减小电流纹波,多相交错图腾柱PFC,C代码实现,电流纹波小 ,多相交错图腾柱PFC
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


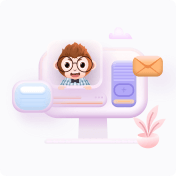