/***************************************************************************************************
* Copyright (c) 2017 - 2022 NVIDIA CORPORATION & AFFILIATES. All rights reserved.
* SPDX-License-Identifier: BSD-3-Clause
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* 3. Neither the name of the copyright holder nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
**************************************************************************************************/
/*
\file
\brief Matrix classes with value semantics.
*/
#pragma once
#if !defined(__CUDACC_RTC__)
#include <iosfwd>
#include <cmath>
#endif
#include "cutlass/cutlass.h"
#include "cutlass/array.h"
#include "cutlass/coord.h"
#include "cutlass/fast_math.h"
#include "cutlass/layout/matrix.h"
namespace cutlass {
/////////////////////////////////////////////////////////////////////////////////////////////////
/// Primary template with partial specializations to follow
template <typename Element, int Rows, int Columns> struct Matrix;
/////////////////////////////////////////////////////////////////////////////////////////////////
/// 1-by-2 matrix template class definition
template <typename Element_>
struct Matrix<Element_, 1, 2> {
//
// Type definitions
//
/// Element data type
using Element = Element_;
/// Number of rows in matrix
static int const kRows = 1;
/// Number of columns in matrix
static int const kColumns = 2;
/// Layout of matrix in underlying array
using Layout = layout::RowMajor;
/// Number of elements in matrix
static int const kCount = 2;
//
// Data members
//
/// Elements of the matrix in row-major layout
Array<Element, kCount> data;
//
// Methods
//
/// Constructs a zero matrix
CUTLASS_HOST_DEVICE
Matrix() {
data.clear();
}
/// Copy constructor for a 1-by-2 matrix
CUTLASS_HOST_DEVICE
Matrix(Matrix const &rhs) {
data = rhs.data;
}
/// Constucts a 1-by-2 matrix from scalar elements
CUTLASS_HOST_DEVICE
Matrix(
Element _0_0, Element _0_1
) {
data[0] = _0_0; data[1] = _0_1;
}
/// Constructs a matrix from a uniform element
CUTLASS_HOST_DEVICE
static Matrix uniform(Element s) {
Matrix m;
m.data[0] = s;
m.data[1] = s;
return m;
}
/// Constructs a matrix from a uniform element 1
CUTLASS_HOST_DEVICE
static Matrix ones() {
return uniform(Element(1));
}
/// Constructs a matrix from a uniform element 0
CUTLASS_HOST_DEVICE
static Matrix zero() {
return Matrix();
}
/// Returns a transposed matrix
CUTLASS_HOST_DEVICE
Matrix<Element, 2, 1> transpose() const {
Matrix<Element, 2, 1> mt;
mt.data[0] = data[0];
mt.data[1] = data[1];
return mt;
}
/// Accesses an element by coordinate
CUTLASS_HOST_DEVICE
Element at(int i, int j) const {
return data[i * 1 + j];
}
/// Accesses an element by coordinate
CUTLASS_HOST_DEVICE
Element & at(int i, int j) {
return data[i * 1 + j];
}
/// Accesses an element by coordinate
CUTLASS_HOST_DEVICE
Element at(Coord<2> const &coord) const {
return at(coord[0], coord[1]);
}
/// Accesses an element by coordinate
CUTLASS_HOST_DEVICE
Element & at(Coord<2> const &coord) {
return at(coord[0], coord[1]);
}
/// Accesses an element by offset
CUTLASS_HOST_DEVICE
Element &at(int offset) {
return data[offset];
}
/// Accesses an element by offset
CUTLASS_HOST_DEVICE
Element at(int offset) const {
return data[offset];
}
/// Accesses an element by coordinate
CUTLASS_HOST_DEVICE
Element operator[](Coord<2> const &coord) const {
return at(coord[0], coord[1]);
}
/// Accesses an element by coordinate
CUTLASS_HOST_DEVICE
Element & operator[](Coord<2> const &coord) {
return at(coord[0], coord[1]);
}
/// Accesses an element by offset
CUTLASS_HOST_DEVICE
Element & operator[](int offset) {
return data[offset];
}
/// Accesses an element by offset
CUTLASS_HOST_DEVICE
Element operator[](int offset) const {
return data[offset];
}
/// Gets a submatrix with optional offset
CUTLASS_HOST_DEVICE
Matrix<Element, 1, 2> slice_1x2(int i = 0, int j = 0) const {
Matrix<Element, 1, 2> m;
m.data[0] = data[i * 2 + j + 0];
m.data[1] = data[i * 2 + j + 1];
return m;
}
/// Overwrites a submatrix with optional offset
CUTLASS_HOST_DEVICE
Matrix & set_slice_1x2(Matrix<Element, 1, 2> const &m, int i = 0, int j = 0) {
data[i * 2 + j + 0] = m.data[0];
data[i * 2 + j + 1] = m.data[1];
return *this;
}
CUTLASS_HOST_DEVICE
Matrix<Element, 1, 2> row(int i) const {
return slice_1x2(i, 0);
}
CUTLASS_HOST_DEVICE
Matrix &set_row(Matrix<Element, 1, 2> const &v, int i = 0) {
return set_slice_1x2(v, i, 0);
}
/// Forms a 1-by-2 matrix by horizontally concatenating an Element with an Element
CUTLASS_HOST_DEVICE
static Matrix hcat(Element lhs, Element rhs) {
return Matrix(
lhs, rhs);
}
/// Concatenates this matrix with a an Element to form a 1-by-3 matrix
CUTLASS_HOST_DEVICE
Matrix<Element, 1, 3> hcat(Element rhs) const {
return Matrix<Element, 1, 3>::hcat(*this, rhs);
}
/// Concatenates this matrix with a a 1-by-2 matrix to form a 1-by-4 matrix
CUTLASS_HOST_DEVICE
Matrix<Element, 1, 4> hcat(Matrix<Element, 1, 2> const & rhs) const {
return Matrix<Element, 1, 4>::hcat(*this, rhs);
}
/// Concatenates this matrix with a a 1-by-2 matrix to form a 2-by-2 matrix
CUTLASS_HOST_DEVICE
Matrix<Element, 2, 2> vcat(Matrix<Element, 1, 2> const & rhs) const {
return Matrix<Element, 2, 2>::vcat(*this, rhs);
}
/// Concatenates this matrix with a a 2-by-2 matrix to form a 3-by-2 matrix
CUTLASS_HOST_DEVICE
Matrix<Element, 3, 2> vcat(Matrix<Element, 2, 2> const & rhs) const {
return Matrix<Element, 3, 2>::vcat(*this, rhs);
}
/// Concatenates this matrix with a a 3-by-2 matrix to form a 4-by-2 matrix
CUTLASS_HOST_DEVICE
Matrix<Element, 4, 2> vcat(Matrix<Element, 3, 2> const & rhs) const {
return Matrix<Element, 4, 2>::vcat(*this, rhs);
}
/// Elementwise add operator (1-by-2)
CUTLASS_HOST_DEVICE
Matrix add(Matrix const &rhs) const {
Matrix result;
result.data[0] = data[0] + rhs.data[0];
resu
没有合适的资源?快使用搜索试试~ 我知道了~
Linuxtiny-cuda-nn直接安装
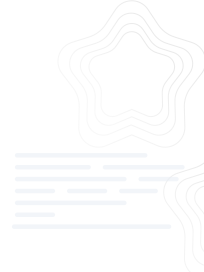
共2000个文件
html:1278个
h:545个
js:76个

需积分: 5 16 下载量 11 浏览量
2023-11-19
20:29:54
上传
评论 1
收藏 160.27MB ZIP 举报
温馨提示
根据配套的文章命令,就可以轻松的在自己电脑上(服务器linux上)安装成功tiny-cuda-nn啦!!git不下来?没关系,我下载好啦!安装总是报错?没关系,我下载的这个是全套完整的!!跟着安装命令步骤来,准没错!芜湖~~
资源推荐
资源详情
资源评论
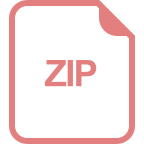
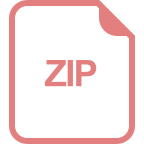
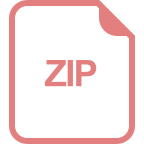
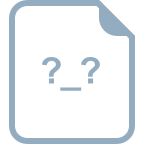
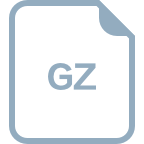
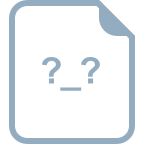
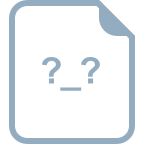
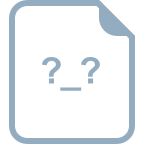
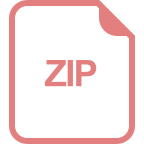
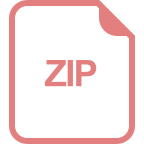
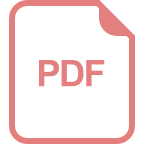
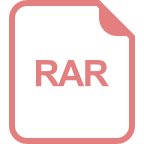
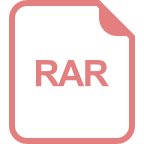
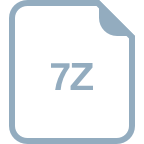
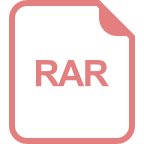
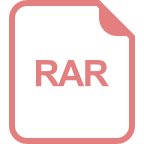
收起资源包目录

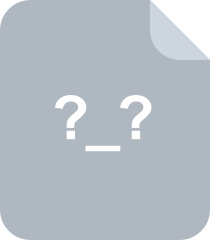
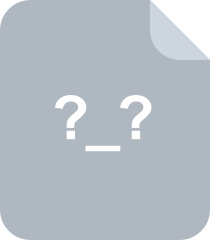
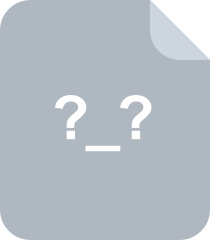
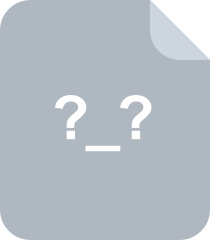
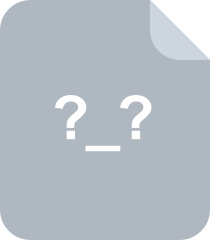
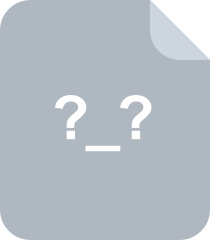
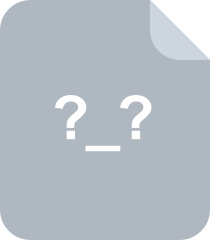
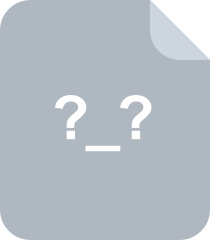
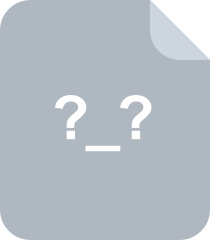
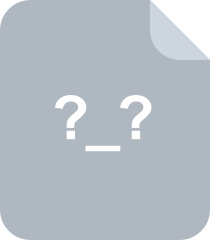
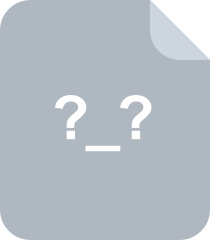
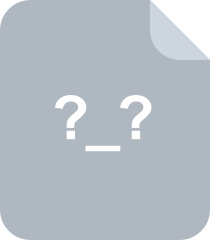
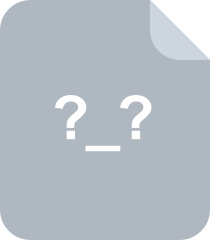
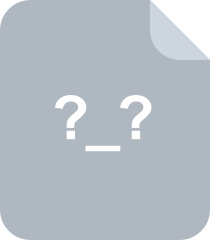
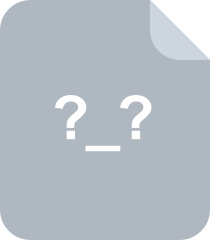
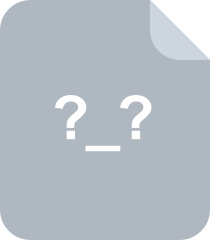
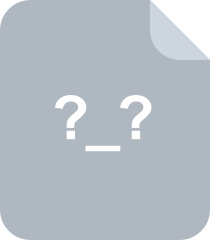
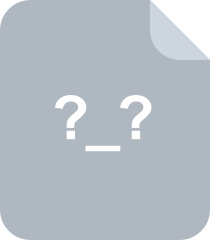
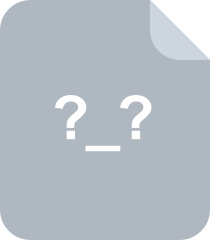
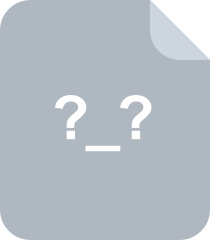
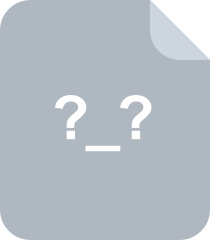
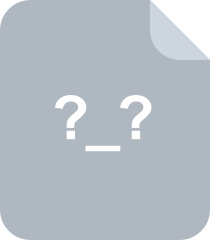
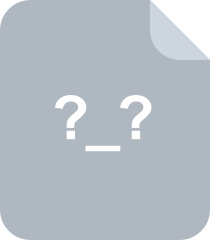
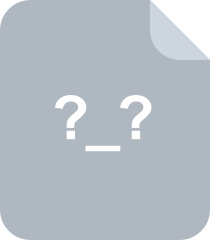
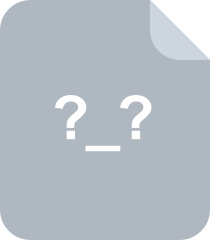
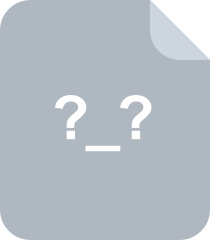
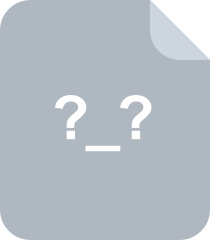
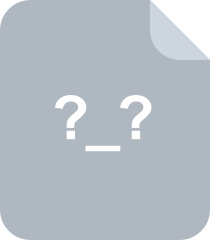
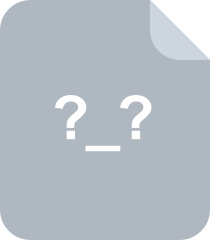
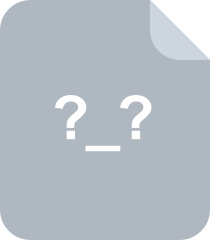
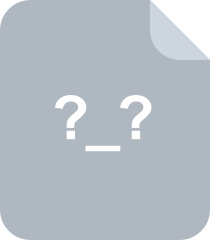
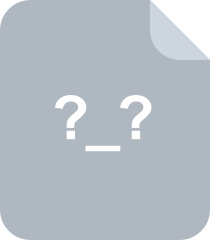
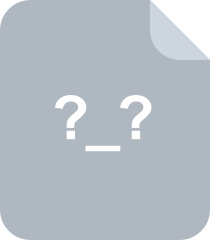
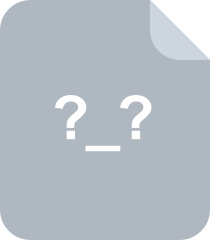
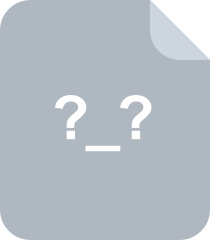
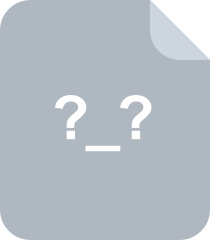
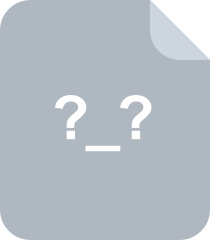
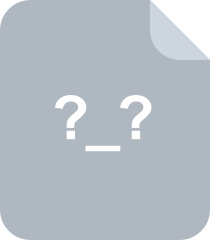
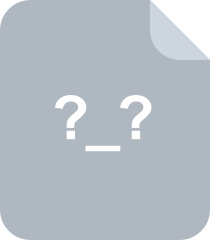
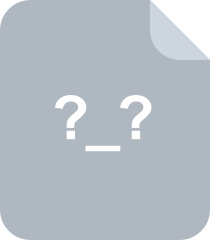
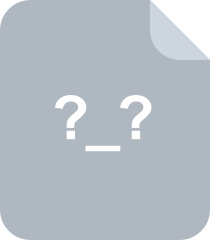
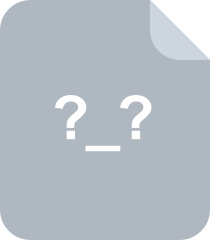
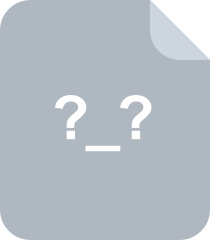
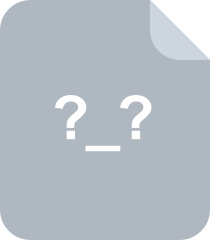
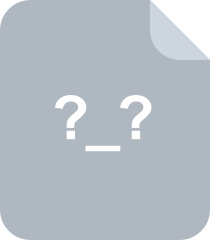
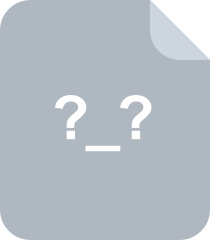
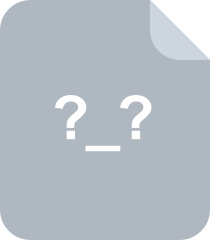
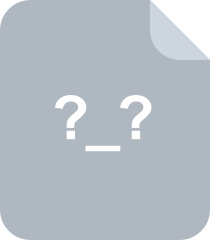
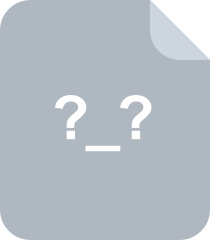
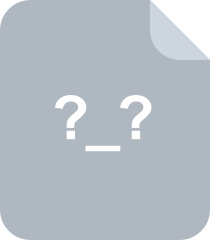
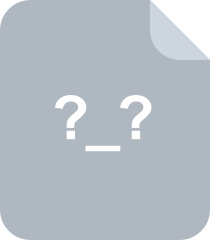
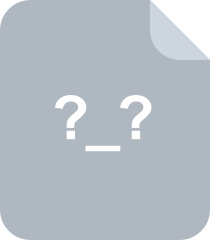
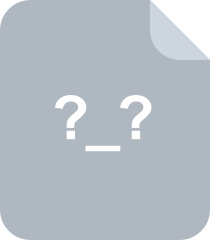
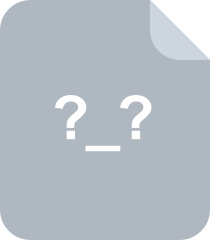
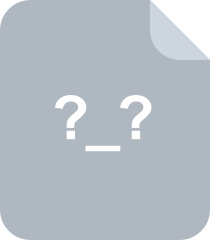
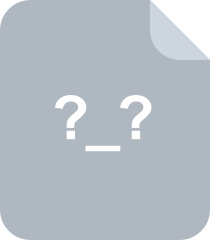
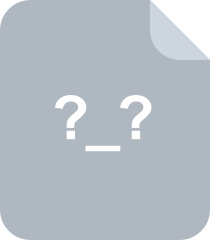
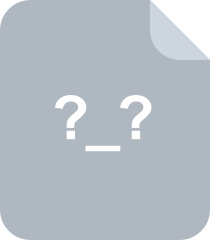
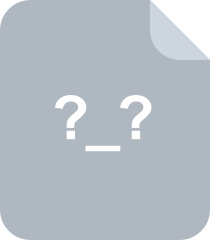
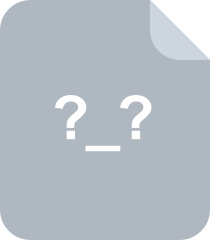
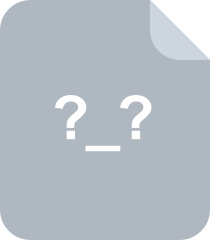
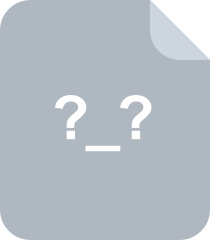
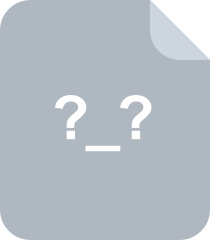
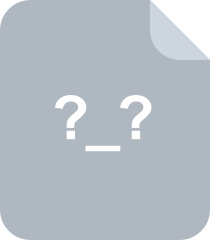
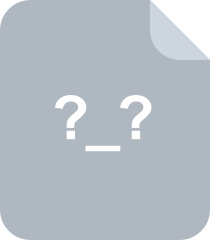
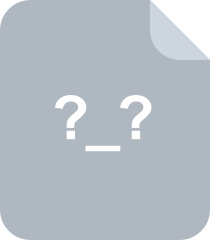
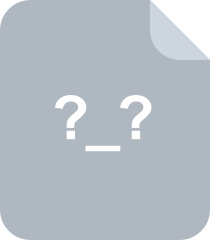
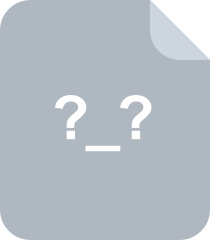
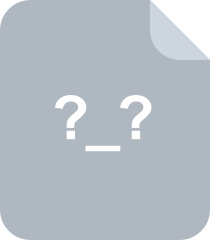
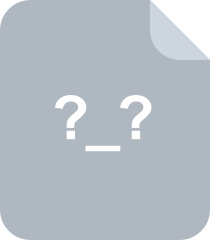
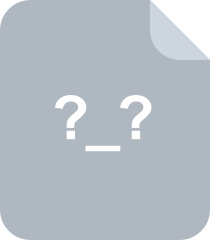
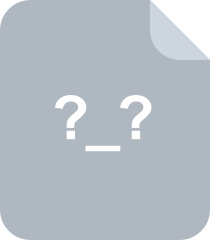
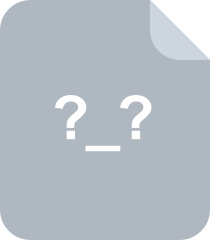
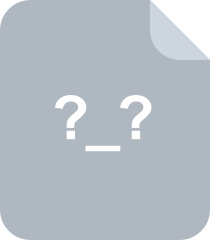
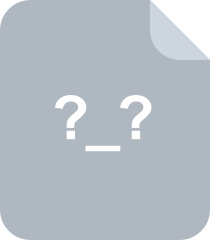
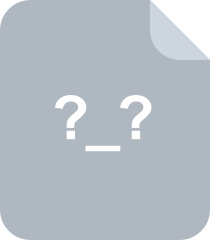
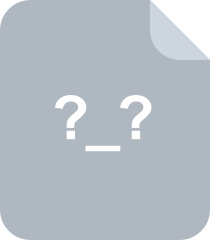
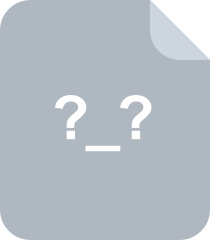
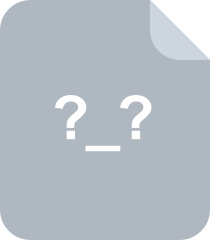
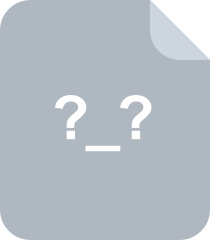
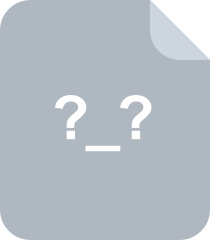
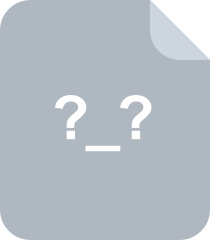
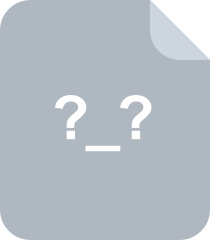
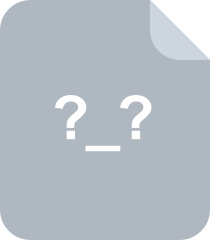
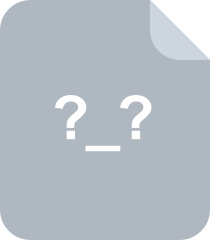
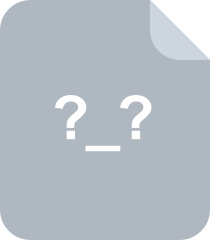
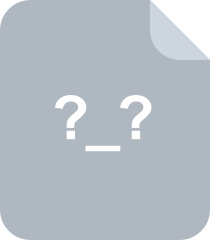
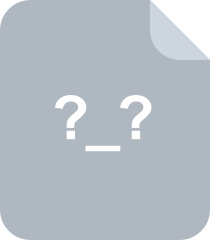
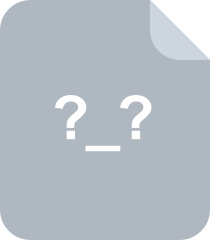
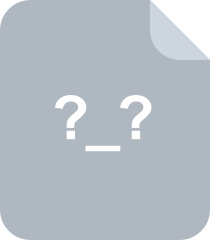
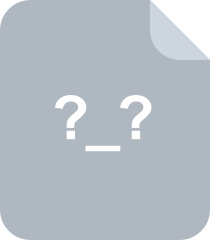
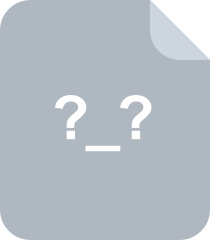
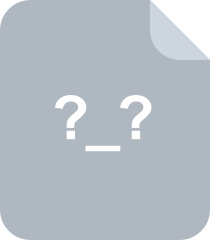
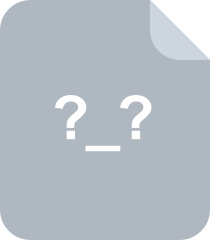
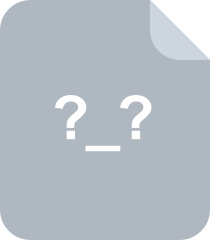
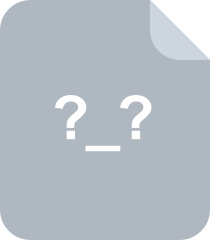
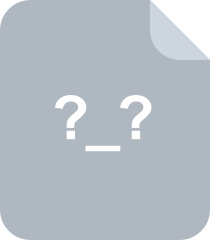
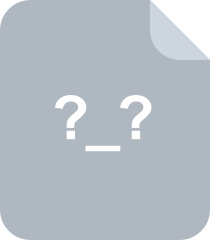
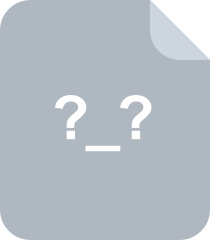
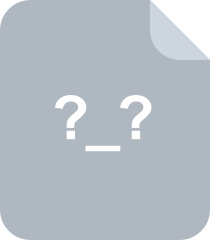
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
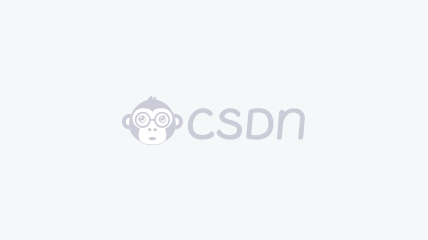


小秋今天也要加油吖
- 粉丝: 1
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

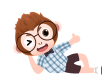
安全验证
文档复制为VIP权益,开通VIP直接复制
