package com.dominate_orientation.subwayfootprint;
import static java.lang.Thread.sleep;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.content.Intent;
import android.os.Bundle;
import android.Manifest;
import android.annotation.SuppressLint;
import android.app.AlertDialog;
import android.app.Dialog;
import android.content.Context;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.drawable.AnimationDrawable;
import android.graphics.drawable.Drawable;
import android.location.Location;
import android.location.LocationManager;
import android.os.Bundle;
import android.os.Looper;
import android.os.StrictMode;
import android.provider.Settings;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import com.google.android.gms.location.FusedLocationProviderClient;
import com.google.android.gms.location.LocationCallback;
import com.google.android.gms.location.LocationRequest;
import com.google.android.gms.location.LocationResult;
import com.google.android.gms.location.LocationServices;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import android.widget.Toast;
import org.json.JSONArray;
import org.json.JSONObject;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.Map;
import okhttp3.MediaType;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
import com.google.android.gms.location.FusedLocationProviderClient;
import com.google.android.gms.location.LocationCallback;
import com.google.android.gms.location.LocationRequest;
import com.google.android.gms.location.LocationResult;
import com.google.android.gms.location.LocationServices;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
public class route extends AppCompatActivity {
String msg="";
Info[] infos = null;
Intent it = null;
TextView ls =null;
TextView ps = null;
TextView ns = null;
TextView digText1 = null;
TextView digText2 = null;
TextView buryText1 = null;
TextView buryText2 = null;
ImageView pre_line = null;
String present_line="";
ImageView next_line = null;
ImageView last_line = null;
ImageView last_pre = null;
ImageView pre_next = null;
String line_1 = "@drawable/line_1";
String line_2 = "@drawable/line_2";
String line_4 = "@drawable/line_4";
String line_5 = "@drawable/line_5";
String line_6 = "@drawable/line_6";
String line_7 = "@drawable/line_7";
String line_8 = "@drawable/line_8";
String line_9 = "@drawable/line_9";
String line_10 = "@drawable/line_10";
String line_11 = "@drawable/line_11";
String line_13 = "@drawable/line_13";
String line_14 = "@drawable/line_14";
String line_15 = "@drawable/line_15";
String line_16 = "@drawable/line_16";
String line_17 = "@drawable/line_17";
String line_19 = "@drawable/line_19";
String line_fs = "@drawable/line_fs";
String line_cp = "@drawable/line_cp";
String line_yz = "@drawable/line_yz";
String line_yf = "@drawable/line_yf";
String line_s1 = "@drawable/line_s1";
String line_xj = "@drawable/line_xj";
String line_yzt1 = "@drawable/line_yzt1";
String line_capair = "@drawable/line_capair";
String line_dxair = "@drawable/line_air";
String present_city = null;
LinkedHashMap<String,String> city_to_pid = null;
//地理位置
FusedLocationProviderClient mFusedLocationClient;
TextView latitudeTextView, longitTextView;
int PERMISSION_ID = 44;
Boolean no_next_passed_station = false;
Boolean last_station_used = false;
Boolean no_station_left=false;
Integer infos_index = 0;
Integer passed_station_index = 0;
Button go_to_next=null;
Button dig = null;
Button ensconce = null;
Button closeBury=null;
View view_bury;
View view_dig;
AlertDialog buryAlert = null;
AlertDialog digAlert = null;
AlertDialog.Builder builder = null;
Context mContext;
Intent intent;
ImageView img_show;
AnimationDrawable anim;
String token;
ArrayList<Treasure> treasureList;
static String TAG ="route";
boolean resumeFlag=false;
Treasure digTreasure=null;
User_treasure passTreasure;
boolean canBury=true;
@Override
protected void onCreate(Bundle savedInstanceState) {
//允许post
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_route);
it = getIntent();
msg = it.getStringExtra("msg");
ls = (TextView) findViewById(R.id.last_station);
ps = (TextView) findViewById(R.id.pre_station);
ns = (TextView) findViewById(R.id.next_station);
go_to_next=(Button)findViewById(R.id.go_to_next_station);
dig =(Button)findViewById(R.id.dig);
ensconce =(Button)findViewById(R.id.ensconce);
ensconce.setEnabled(false);
latitudeTextView = (TextView)findViewById(R.id.latitudeTextView);
longitTextView = (TextView)findViewById(R.id.longitTextView);
mFusedLocationClient = LocationServices.getFusedLocationProviderClient(this);
city_to_pid = new LinkedHashMap<>();
addMap();
pre_line = (ImageView)findViewById(R.id.img_pre_station);
next_line = (ImageView)findViewById(R.id.img_next_station);
last_line= (ImageView)findViewById(R.id.img_last_station);
last_pre = (ImageView)findViewById(R.id.last_pre_exchange);
pre_next = (ImageView)findViewById(R.id.pre_next_exchange);
last_pre.setAlpha(0);
pre_next.setAlpha(0);
//地理位置区
latitudeTextView = (TextView)findViewById(R.id.latitudeTextView);
longitTextView = (TextView)findViewById(R.id.longitTextView);
mFusedLocationClient = LocationServices.getFusedLocationProviderClient(this);
//线路显示区
pre_line = (ImageView)findViewById(R.id.img_pre_station);
next_line = (ImageView)findViewById(R.id.img_next_station);
last_line= (ImageView)findViewById(R.id.img_last_station);
last_pre = (ImageView)findViewById(R.id.last_pre_exchange);
pre_next = (ImageView)findViewById(R.id.pre_next_exchange);
last_pre.setAlpha(0);
pre_next.setAlpha(0);
digTreasure=new Treasure();
//token
Token app = (Token)getApplicationContext();
token=app.getToken();
// token="eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1aWQiOiIyMDE5MjExOTk2QGJ1cHQuZWR1LmNuIiwiZXhwIjoxNjUyMjQ3NzU3fQ.GzUepl2fYoK2fIPunLD4BFaVhek36YPZboMJNiEhQGI";
passTreasure=new User_treasure();
mContext=route.this;
builder=new AlertDialog.Builder(mContext);
final LayoutInflater inflater = route.this.getLayoutInflater();
view_bury = inflater.inflate(R.layout.bottom_sheet_bury, null,false);
builder.setView(view_bury);
builder.setCancelable(false);
buryAlert = builder.create();
view_dig = inflater.inflate(R.layout.bottom_sheet_dig, null,false);
builder.setView(view_dig);
digAlert=builder.create();
view_bury.findViewById(R.id.btn_cancle).setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View v){
buryAlert.dismiss();
}
});
view_bury.findViewById(R.id.btn_close).setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(Vi
没有合适的资源?快使用搜索试试~ 我知道了~
使用Android Studio 开发的地铁足迹系统源码.zip
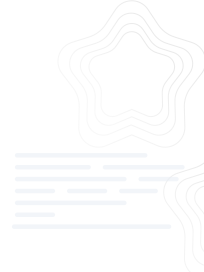
共308个文件
xml:129个
png:66个
java:64个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 151 浏览量
2022-10-29
18:44:22
上传
评论
收藏 18.48MB ZIP 举报
温馨提示
使用Android Studio 开发的地铁足迹系统源码.zip
资源推荐
资源详情
资源评论
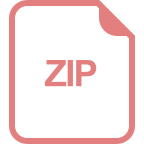
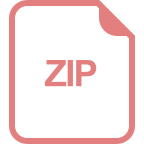
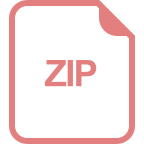
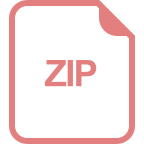
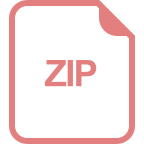
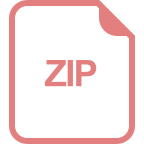
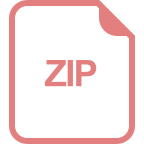
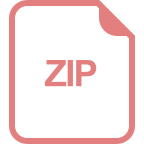
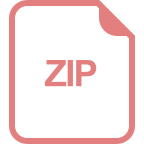
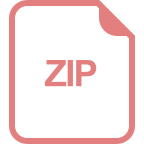
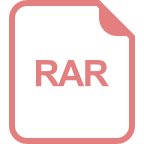
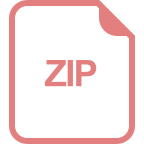
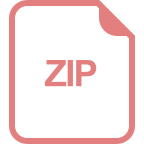
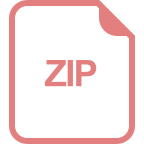
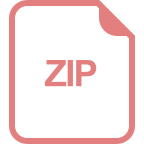
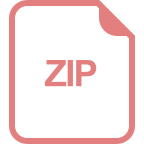
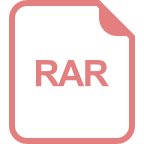
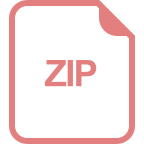
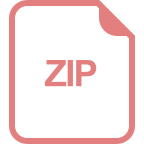
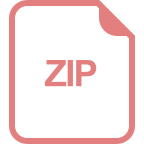
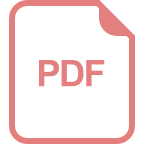
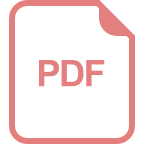
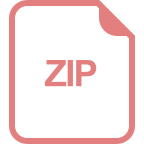
收起资源包目录

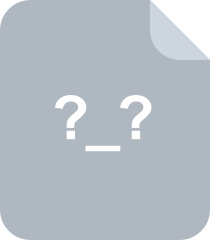
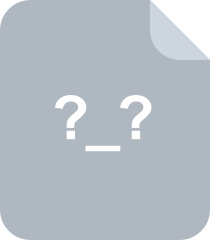
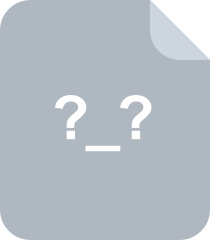
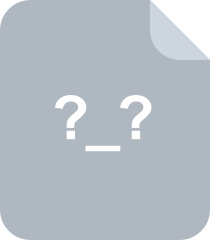
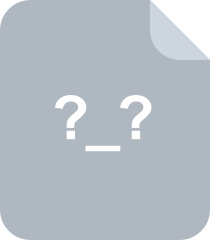
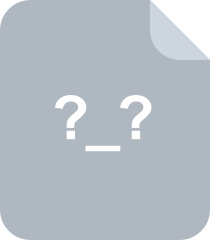
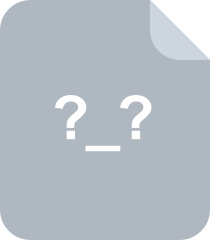
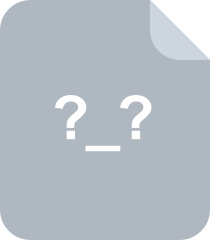
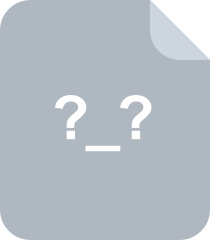
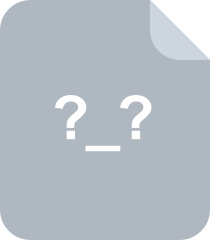
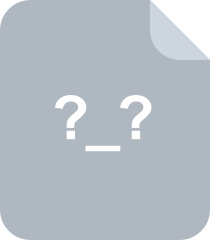
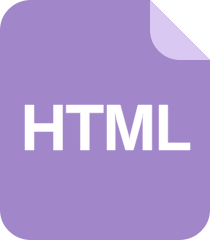
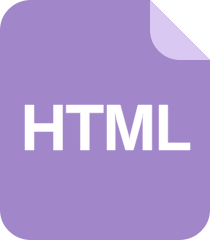
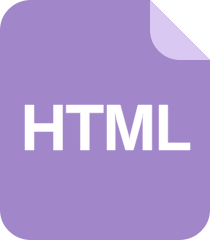
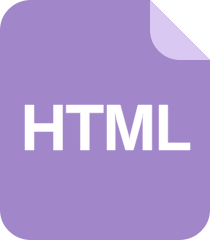
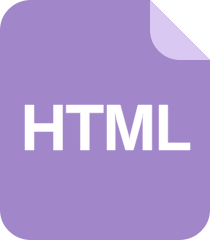
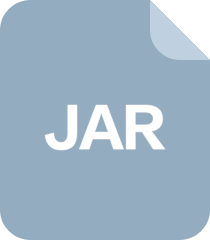
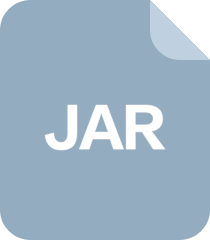
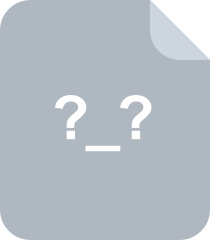
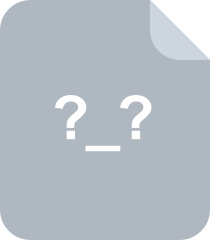
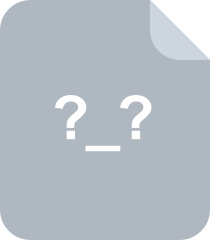
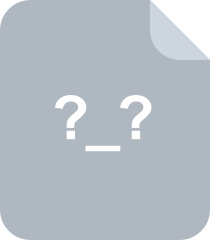
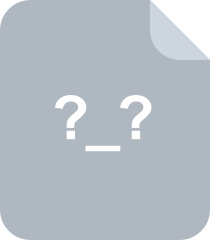
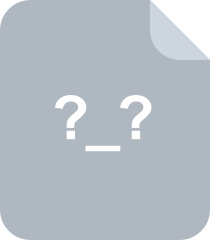
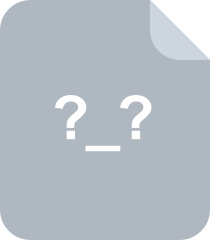
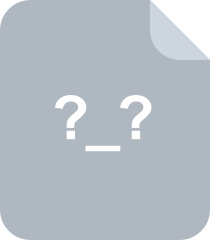
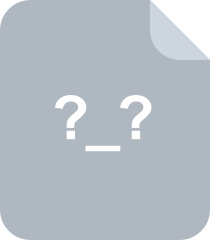
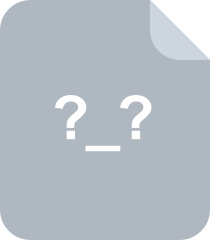
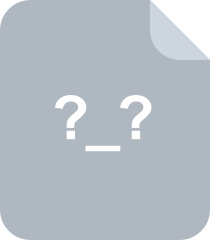
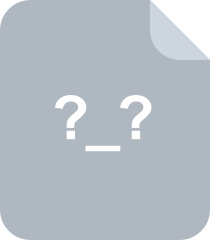
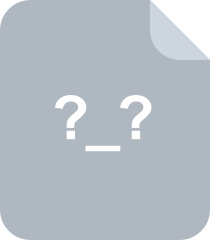
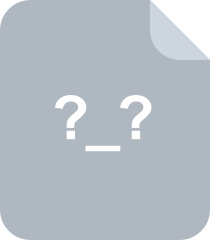
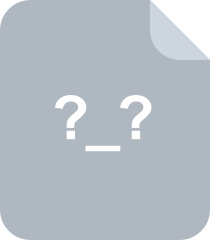
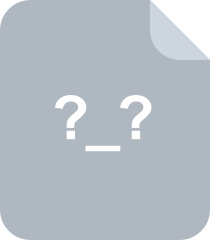
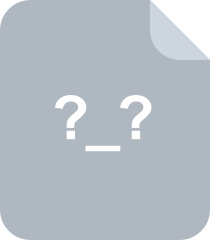
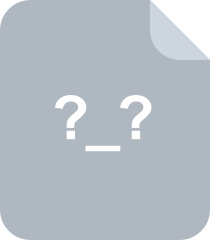
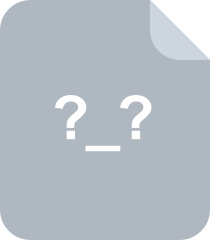
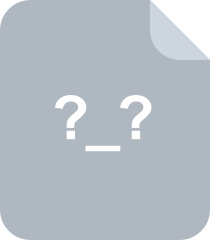
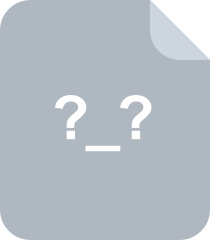
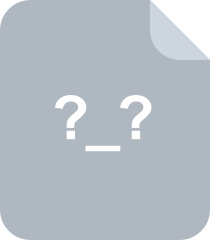
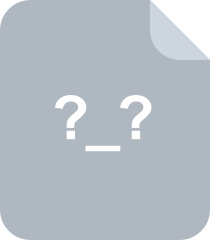
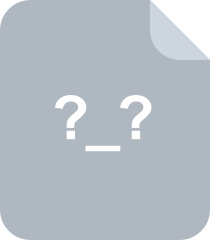
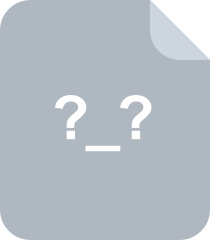
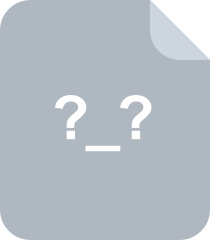
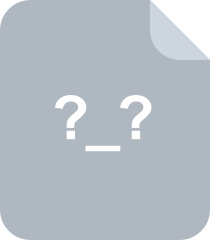
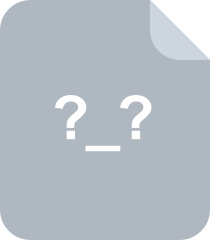
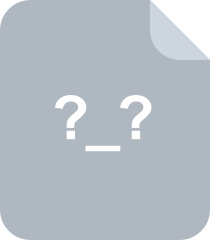
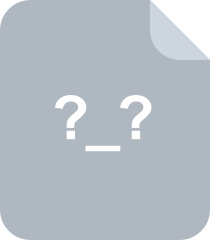
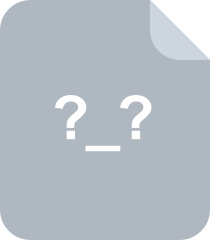
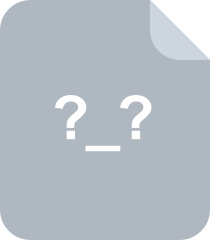
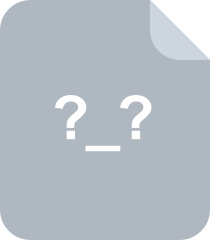
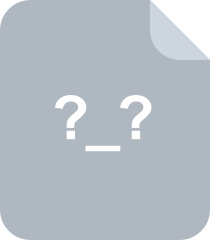
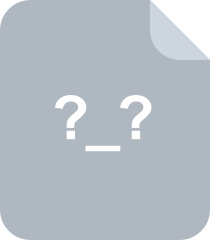
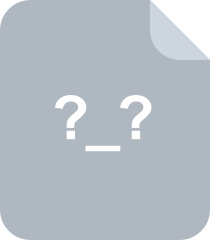
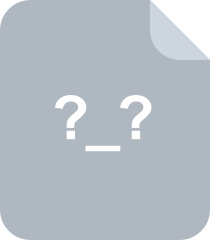
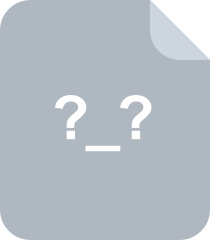
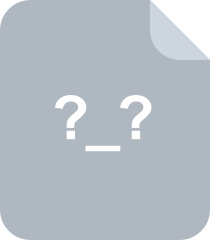
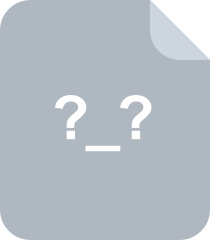
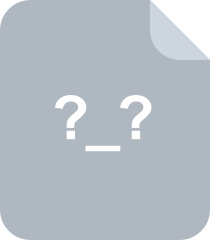
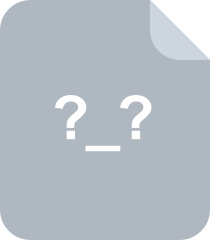
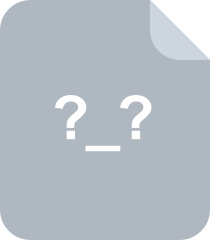
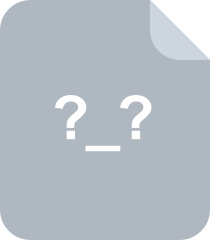
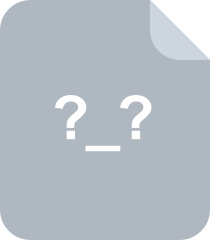
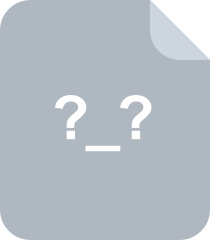
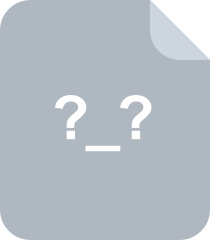
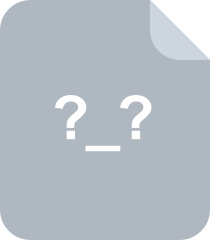
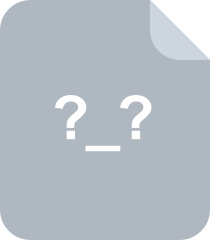
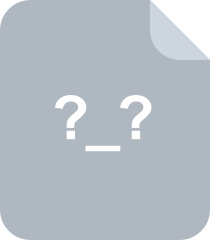
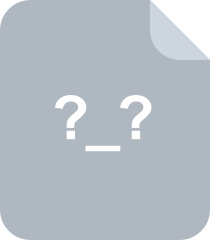
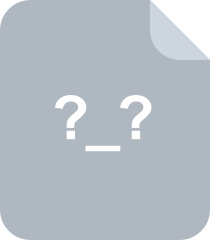
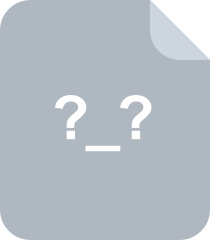
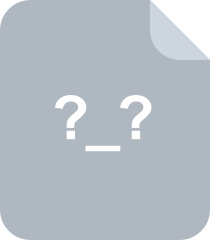
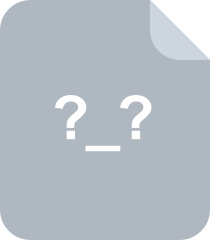
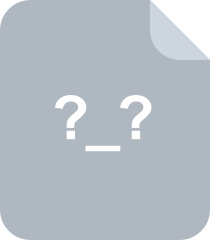
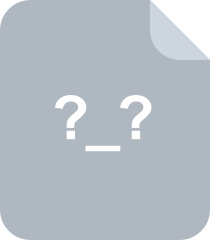
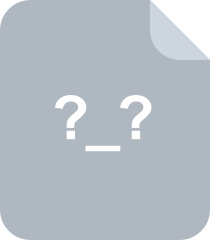
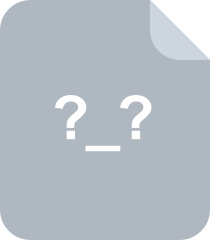
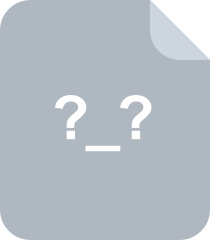
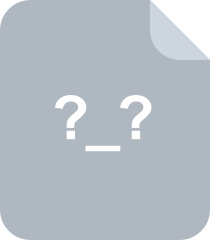
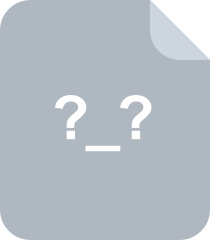
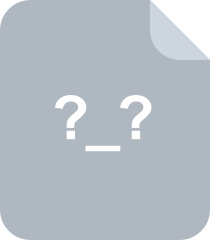
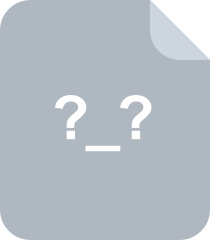
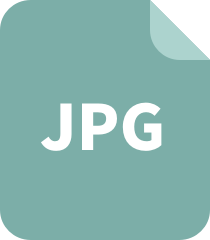
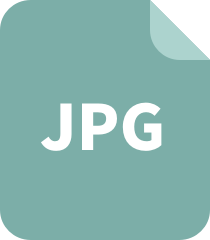
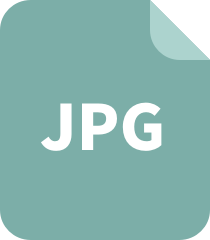
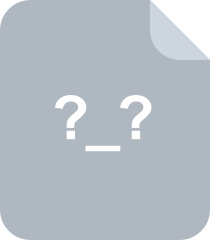
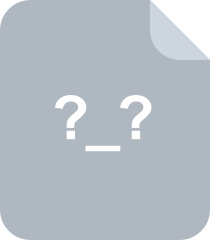
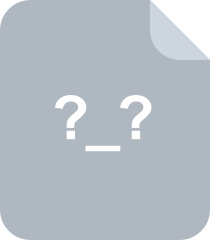
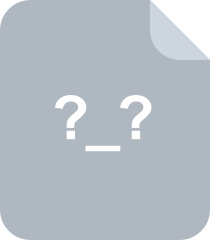
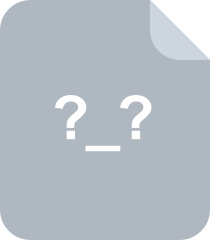
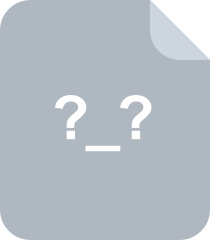
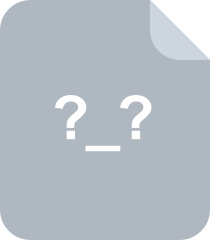
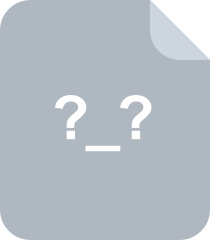
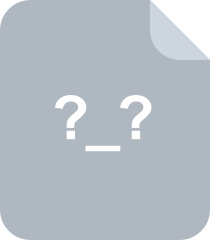
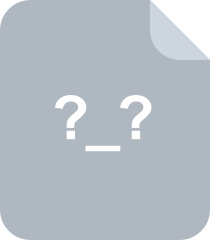
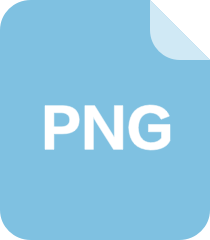
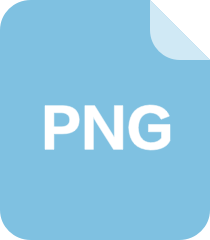
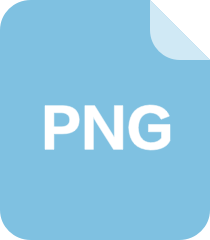
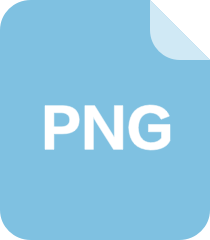
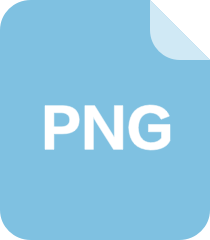
共 308 条
- 1
- 2
- 3
- 4
资源评论
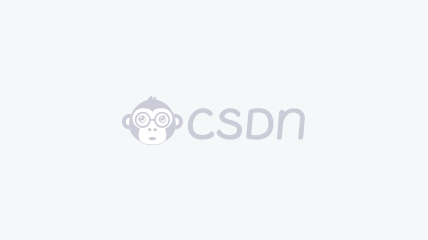

「已注销」
- 粉丝: 795
- 资源: 3612
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

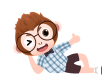
安全验证
文档复制为VIP权益,开通VIP直接复制
