package com.yadan.common.core.sms;
import com.alibaba.fastjson.JSONObject;
import com.aliyun.dysmsapi20170525.Client;
import com.aliyun.dysmsapi20170525.models.*;
import com.aliyun.tea.TeaException;
import com.aliyun.teaopenapi.models.Config;
import com.aliyun.teautil.Common;
import com.aliyun.teautil.models.RuntimeOptions;
import com.yadan.common.core.domain.SmsInfo;
import com.yadan.common.utils.CommonUtils;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
@Service
public class AliSms {
@Value("${sms.ali.accessKeyId}")
private String accessKeyId;
@Value("${sms.ali.accessKeySecret}")
private String accessKeySecret;
/**
* 使用AK&SK初始化账号Client
*/
public Client createClient() throws Exception {
Config config = new Config()
// 必填,您的 AccessKey ID
.setAccessKeyId(accessKeyId)
// 必填,您的 AccessKey Secret
.setAccessKeySecret(accessKeySecret);
// 访问的域名
config.endpoint = "dysmsapi.aliyuncs.com";
return new Client(config);
}
public String sendSms(SmsInfo<?> smsInfo) throws Exception {
if (CommonUtils.isTrue(smsInfo.getIsBatch())) {
return sendBatchSms(smsInfo);
}
// 工程代码泄露可能会导致AccessKey泄露,并威胁账号下所有资源的安全性。以下代码示例仅供参考,建议使用更安全的 STS 方式,更多鉴权访问方式请参见:https://help.aliyun.com/document_detail/378657.html
Client client = createClient();
SendSmsRequest sendSmsRequest = new SendSmsRequest()
.setPhoneNumbers(smsInfo.getPhoneNumber())
.setSignName(smsInfo.getSignName())
.setTemplateCode(smsInfo.getTemplateCode())
.setTemplateParam(JSONObject.toJSONString(smsInfo.getTemplateParam()));
RuntimeOptions runtime = new RuntimeOptions();
try {
// 复制代码运行请自行打印 API 的返回值
SendSmsResponse sendSmsResponse = client.sendSmsWithOptions(sendSmsRequest, runtime);
System.out.println(JSONObject.toJSONString(sendSmsResponse));
} catch (TeaException error) {
// 如有需要,请打印 error
Common.assertAsString(error.message);
} catch (Exception _error) {
TeaException error = new TeaException(_error.getMessage(), _error);
// 如有需要,请打印 error
Common.assertAsString(error.message);
}
return null;
}
public String sendBatchSms(SmsInfo<?> smsInfo) throws Exception {
// 工程代码泄露可能会导致AccessKey泄露,并威胁账号下所有资源的安全性。以下代码示例仅供参考,建议使用更安全的 STS 方式,更多鉴权访问方式请参见:https://help.aliyun.com/document_detail/378657.html
Client client = createClient();
SendBatchSmsRequest sendBatchSmsRequest = new SendBatchSmsRequest()
.setPhoneNumberJson(JSONObject.toJSONString(smsInfo.getPhoneNumberList()))
.setSignNameJson(JSONObject.toJSONString(smsInfo.getSignNameList()))
.setTemplateCode(smsInfo.getTemplateCode())
.setTemplateParamJson(JSONObject.toJSONString(smsInfo.getTemplateParamList()));
RuntimeOptions runtime = new RuntimeOptions();
try {
// 复制代码运行请自行打印 API 的返回值
SendBatchSmsResponse sendBatchSmsResponse = client.sendBatchSmsWithOptions(sendBatchSmsRequest, runtime);
System.out.println(JSONObject.toJSONString(sendBatchSmsResponse));
} catch (TeaException error) {
// 如有需要,请打印 error
Common.assertAsString(error.message);
} catch (Exception _error) {
TeaException error = new TeaException(_error.getMessage(), _error);
// 如有需要,请打印 error
Common.assertAsString(error.message);
}
return null;
}
public String querySmsSignList() throws Exception {
Client client = createClient();
QuerySmsSignListRequest querySmsSignListRequest = new QuerySmsSignListRequest();
RuntimeOptions runtime = new com.aliyun.teautil.models.RuntimeOptions();
Object querySmsSignListResponse = null;
try {
// 复制代码运行请自行打印 API 的返回值
querySmsSignListResponse = client.querySmsSignListWithOptions(querySmsSignListRequest, runtime);
System.out.println(JSONObject.toJSONString(querySmsSignListResponse));
} catch (TeaException error) {
// 如有需要,请打印 error
com.aliyun.teautil.Common.assertAsString(error.message);
} catch (Exception _error) {
TeaException error = new TeaException(_error.getMessage(), _error);
// 如有需要,请打印 error
com.aliyun.teautil.Common.assertAsString(error.message);
}
return querySmsSignListResponse == null ? "false" : JSONObject.toJSONString(querySmsSignListResponse);
}
}

林间6
- 粉丝: 1394
- 资源: 66
最新资源
- 使用Python Turtle库模拟3D动态圣诞树
- java毕业设计-基于springboot+vue+element-ui 办公自动化系统、前后端分离全部资料+详细文档+高分项目+源码.zip
- java毕业设计-基于选题系统全部资料+详细文档+高分项目+源码.zip
- java毕业设计-基于在线考试系统全部资料+详细文档+高分项目+源码.zip
- 本科毕设-基于 一个云笔记系统,全部资料+详细文档+高分项目+源码.zip
- 本科毕设-基于LabVIEW的过控实验系统全部资料+详细文档+高分项目+源码.zip
- 本科毕设-基于旅游景点推荐系统全部资料+详细文档+高分项目+源码.zip
- 毕业设计-基于B2B 在线招标系统全部资料+详细文档+高分项目+源码.zip
- 基于STM32单片机的双管正激式开关电源设计.zip
- 本科毕设-基于奖助学金管理系统全部资料+详细文档+高分项目+源码.zip
- 毕业设计-基于JAVA+MySQL超市供销存管理系统,超市管理系统,供销存管理系统,进销存全部资料+详细文档+高分项目+源码.zip
- 毕业设计-基于Java题库管理系统全部资料+详细文档+高分项目+源码.zip
- 毕业设计-基于javaEE心理咨询预约管理系统全部资料+详细文档+高分项目+源码.zip
- 毕业设计-基于SpringBoot + Vue美妆商城系统全部资料+详细文档+高分项目+源码.zip
- 毕业设计-基于Spring+SpringMVC+MyBatis+Mysql 销售管理系统全部资料+详细文档+高分项目+源码.zip
- MATLAB中绘制简单2D圣诞树的图形代码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


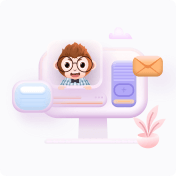