package lms.code.action;
import java.util.Collection;
import java.util.LinkedList;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import org.apache.struts2.convention.annotation.Action;
import org.apache.struts2.convention.annotation.ParentPackage;
import org.apache.struts2.convention.annotation.Result;
import org.apache.struts2.convention.annotation.Results;
import org.dom4j.Element;
import com.opensymphony.xwork2.ModelDriven;
import dev.frame.util.StringUtil;
import dev.frame.xml.XMLUtility;
import lms.code.action.model.StaffActionModel;
import lms.code.action.returnconst.StaffActionConst;
import lms.code.beans.LMS_Roles;
import lms.code.beans.LMS_Staffs;
import lms.code.service.RoleService;
import lms.code.service.StaffService;
import lms.common.AbstractAction;
import lms.common.AuthorInfo;
import lms.common.BeanUtility;
import lms.common.SessionUser;
import lms.common.SiteConfig;
@ParentPackage("staffPackage")
@Action(value = "staffActions")
@Results({
@Result(name = StaffActionConst.StaffSignIn_Success,location = "/Main.jsp")
,@Result(name = StaffActionConst.StaffSignIn_Fialed, location = "/SignIn.jsp")
,@Result(name = StaffActionConst.StaffSignOut_Success,location = "/SignIn.jsp")
,@Result(name = StaffActionConst.StaffChangePassword_OldPwdError,location = "/manage/StaffManage/ChangePwd.jsp")
,@Result(name = StaffActionConst.StaffChangePassword_Success,location = "/manage/StaffManage/ChangePwd.jsp")
,@Result(name = StaffActionConst.InitialAddStaffInfo_Success,location = "/manage/StaffManage/AddStaff.jsp")
,@Result(name = StaffActionConst.AddStaffInfo_Success,location = "/manage/StaffManage/AddStaff.jsp")
,@Result(name = StaffActionConst.CompleteStaffInfo_Success,location = "/manage/StaffManage/StaffInfo.jsp")
,@Result(name = StaffActionConst.GetStaffInfoDetail_Success,location = "/manage/StaffManage/StaffInfo.jsp")
,@Result(name = StaffActionConst.GetStaffList_Success,location = "/manage/StaffManage/StaffList.jsp")
,@Result(name = StaffActionConst.DeleteOneStaffInfo_Success,location = "/actions/staff/staffActions.action?method=getStaffInfoList" ,type="redirect")
,@Result(name = StaffActionConst.GetStaffByStaffID_Success,location = "/manage/StaffManage/EditStaff.jsp")
,@Result(name = StaffActionConst.EditStaffInfo_Success,location = "/manage/StaffManage/EditStaff.jsp")
,@Result(name = StaffActionConst.EditStaffInfo_NameIsExist,location = "/manage/StaffManage/EditStaff.jsp")
,@Result(name = StaffActionConst.ResetStaffPwd_Success,location = "/actions/staff/staffActions.action?method=getStaffInfoList&resetpwd=1" ,type="redirect")
,@Result(name = StaffActionConst.ResetStaffPwd_Fialed,location = "/actions/staff/staffActions.action?method=getStaffInfoList&resetpwd=0" ,type="redirect")
})
@SuppressWarnings("unchecked")
public class StaffAction extends AbstractAction implements
ModelDriven<StaffActionModel> {
private static final long serialVersionUID = -7604937715777424700L;
private StaffActionModel actionModel = new StaffActionModel();
@Resource
private StaffService staffService;
@Resource
private RoleService roleService;
private Collection<LMS_Roles> addStaffRoles;
private LMS_Staffs staffInfo;
private Collection<LMS_Staffs> staffList;
private Collection<AuthorInfo> staffAuthors;
public String staffSignIn() {
String userName = actionModel.getLoginName();
String passWord = actionModel.getPassWord();
LMS_Staffs loginStaff = staffService.staffLogin(userName, passWord);
if (loginStaff != null) {
staffAuthors = new LinkedList<AuthorInfo>();
String [] authors = loginStaff.getRole().getAuthors().split(";");
//XMLUtility xmlUtility = new XMLUtility(SiteConfig.AppPath+"/WEB-INF/xml/pop.xml");
XMLUtility xmlUtility = new XMLUtility("D:\\xml\\pop.xml");
Collection<Element> authorElements = xmlUtility.getRootElement().elements();
for (String author : authors) {
for (Element element : authorElements) {
String nameValue = element.attribute("name").getValue();
String codeValue = element.attribute("code").getValue();
String urlValue = element.attribute("url").getValue();
String iconValue = element.attribute("icon").getValue();
AuthorInfo authorInfo = new AuthorInfo();
if (codeValue.equals(author)) {
authorInfo.setName(nameValue);
authorInfo.setCode(codeValue);
authorInfo.setUrl(urlValue);
authorInfo.setIcon(iconValue);
staffAuthors.add(authorInfo);
}
}
}
super.setSession(SiteConfig.SessionUserKey, new SessionUser(loginStaff));
logger.info("用户:"+loginStaff.getLoginName()+"已登录!");
return StaffActionConst.StaffSignIn_Success;
}
registerScript("fnPasswordError();",true);
return StaffActionConst.StaffSignIn_Fialed;
}
public String staffSignOut() {
SessionUser sessionUser = getSessionUser();
super.getSession().removeAttribute(SiteConfig.SessionUserKey);
logger.info("用户:"+sessionUser.getLoginName()+"已退出!");
return StaffActionConst.StaffSignOut_Success;
}
public String staffChangePassword(){
SessionUser sessionUser = getSessionUser();
int changeResult = staffService.changePassword(sessionUser.getLoginName(), actionModel.getPassWord(), actionModel.getNewPassWord());
if (changeResult == 0) {
registerScript("fnOldPasswordError();", true);
logger.error("用户:"+sessionUser.getLoginName()+"修改密码,旧密码出错!");
return StaffActionConst.StaffChangePassword_OldPwdError;
}
registerScript("fnChangePasswordSuccess();", true);
logger.info("用户:"+sessionUser.getLoginName()+"已修改新密码!");
return StaffActionConst.StaffChangePassword_Success;
}
public String initialAddStaffInfo(){
this.addStaffRoles = roleService.getAllRoles();
return StaffActionConst.InitialAddStaffInfo_Success;
}
public String addStaffInfo(){
this.addStaffRoles = roleService.getAllRoles();
int excResult = this.staffService.addNewStaffInfo(this.actionModel.getStaffName(), this.actionModel.getStaffRole());
if(excResult == 1){
logger.info("添加新员工:"+this.actionModel.getStaffName()+"成功!");
registerScript("fnAddNewStaffSuccess();", true);
}else{
logger.error("添加新员工失败:"+this.actionModel.getStaffName()+"已存在!");
registerScript("fnStaffNameIsExist();", true);
}
return StaffActionConst.AddStaffInfo_Success;
}
public String editStaffInfo(){
this.addStaffRoles = roleService.getAllRoles();
LMS_Staffs staffInfo = staffService.getStaffByStaffID(actionModel.getStaffID());
staffInfo.setName(actionModel.getStaffName());
LMS_Roles roleInfo = roleService.getOneRole(actionModel.getStaffRole());
staffInfo.setRole(roleInfo);
int updateResult = staffService.updateStaff(staffInfo);
if (updateResult == 0) {
registerScript("fnEditStaffNameIsExist();", true);
return StaffActionConst.EditStaffInfo_NameIsExist;
}
registerScript("fnEditStaffSuccess();", true);
return StaffActionConst.EditStaffInfo_Success;
}
public String getOneStaffInfo(){
this.addStaffRoles = roleService.getAllRoles();
this.staffInfo = staffService.getStaffByStaffID(actionModel.getStaffID());
return StaffActionConst.GetStaffByStaffID_Success;
}
public String completeStaffInfo(){
SessionUser sessionUser = getSessionUser();
try {
LMS_Staffs staffInfo = staffService.getStaffByUserName(sessionUser.getLoginName());
BeanUtility.copyProperties(actionModel, staffInfo);
staffService.updateStaffInfo(staffInfo);
registerScript("fnCompleteStaffInfo();", true);
logger.info(sessionUser.getLoginName()+"已完善个人资料!");
} catch (Exception e) {
e.printStackTrace();
logger.error(sessionUser.getLoginName()+"完善资料出错:"+e.getMessage());
}
return
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
日志管理系统 功能:项目管理、日志管理、员工管理、角色管理、汇报管理 技术栈:jsp\B/s\spring\mysql\struts2\hibernate\tomcat
资源推荐
资源详情
资源评论
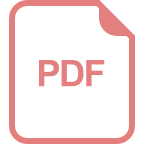
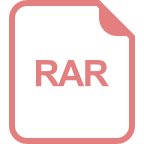
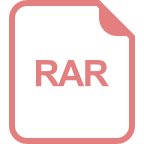
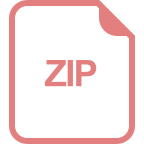
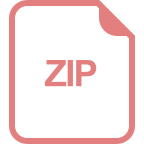
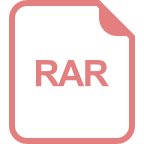
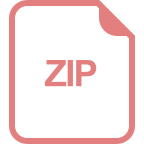
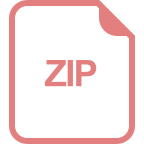
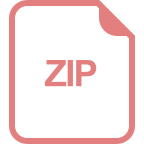
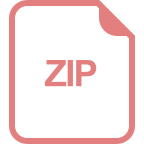
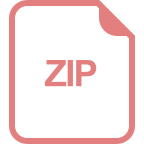
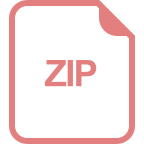
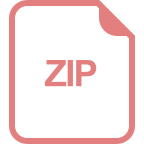
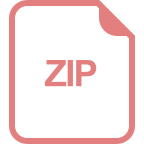
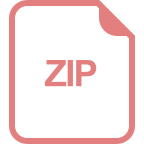
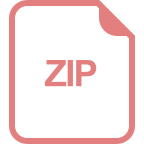
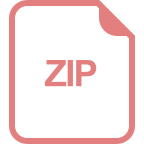
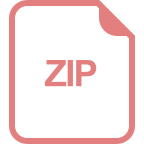
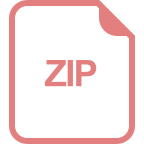
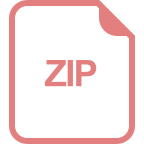
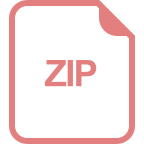
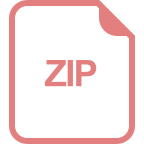
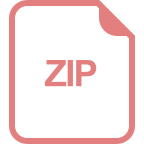
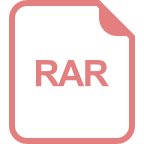
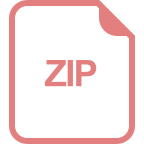
收起资源包目录

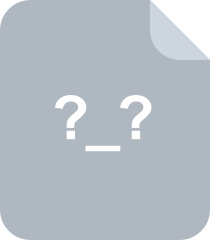
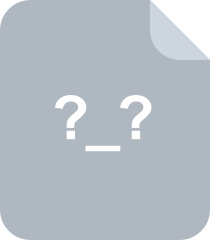
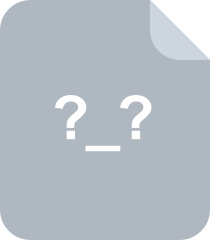
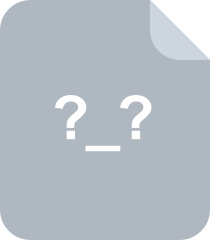
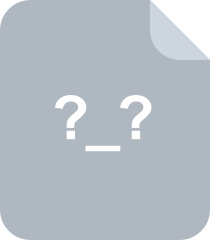
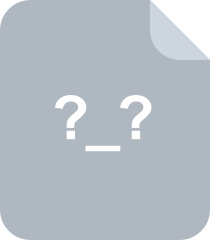
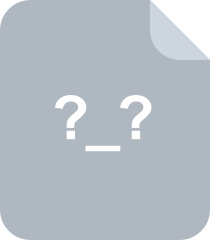
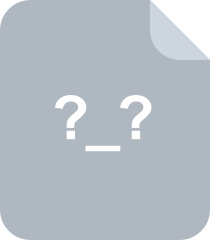
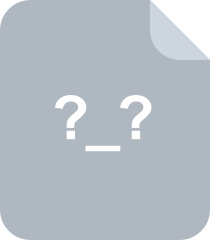
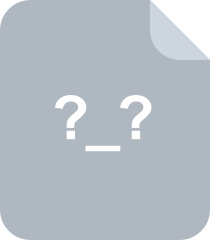
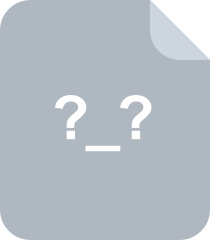
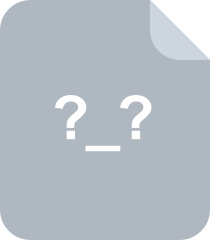
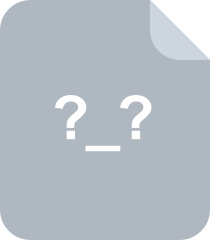
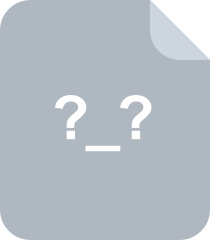
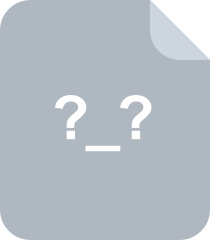
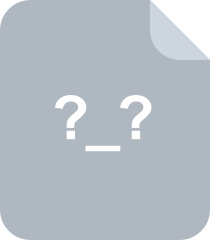
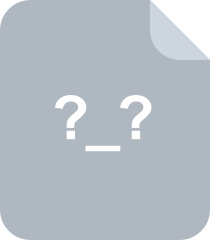
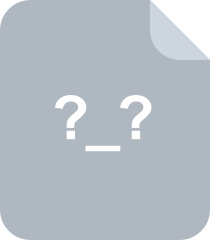
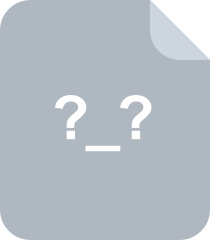
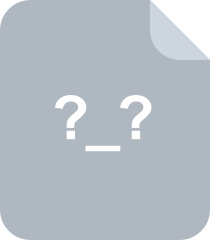
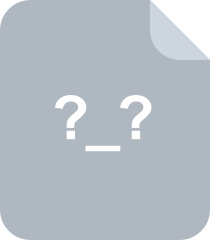
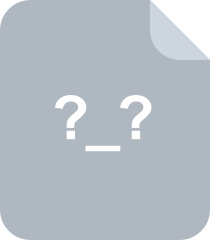
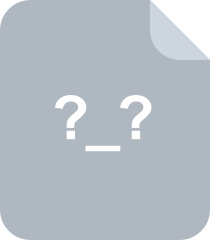
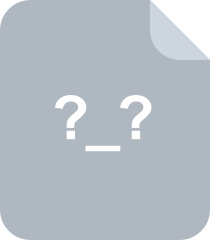
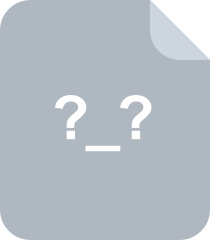
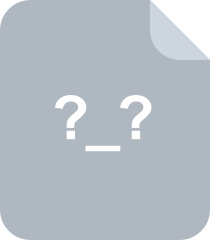
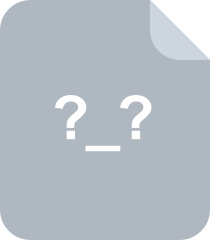
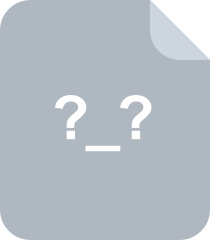
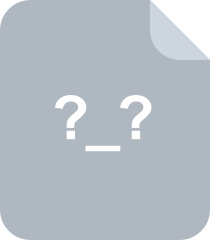
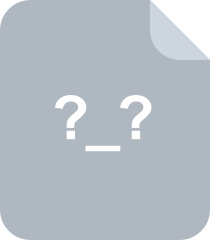
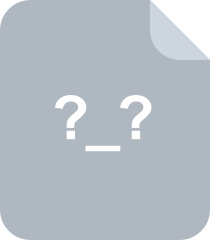
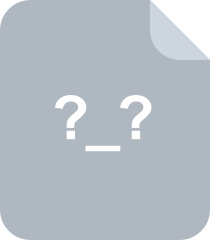
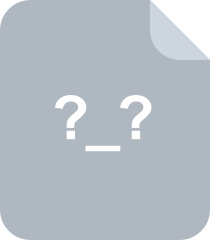
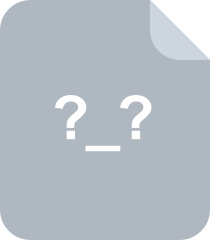
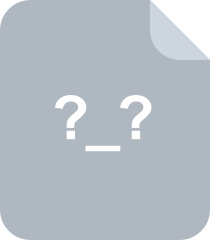
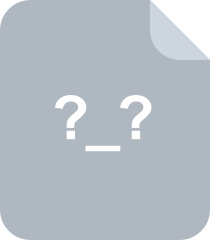
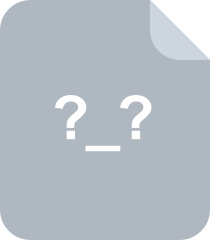
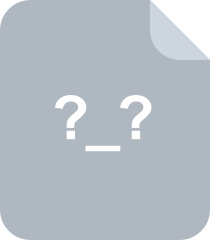
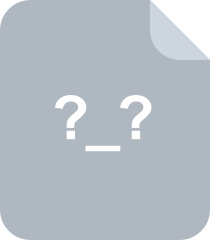
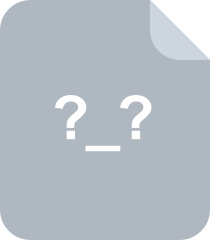
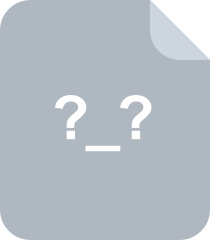
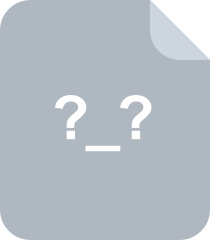
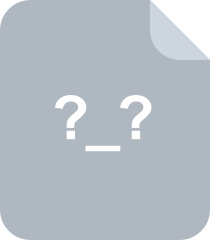
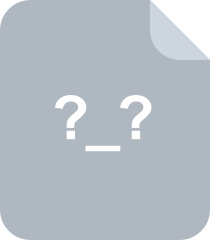
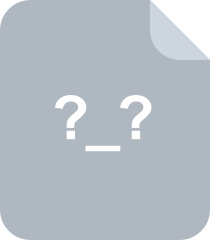
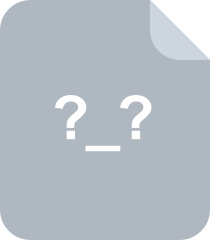
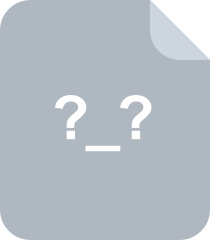
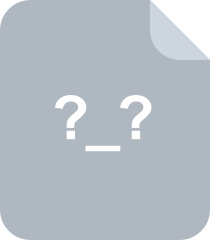
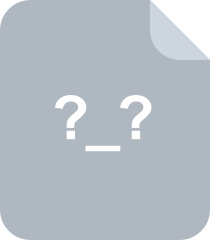
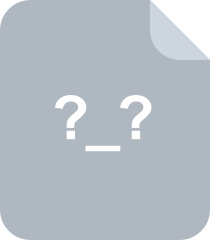
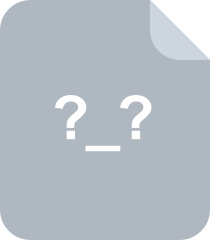
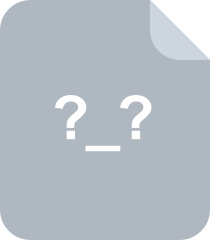
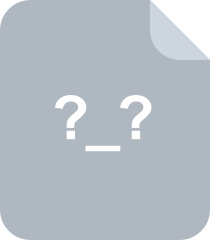
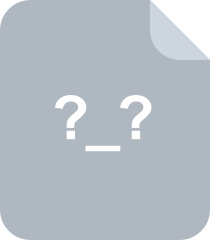
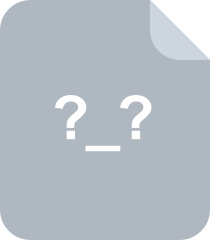
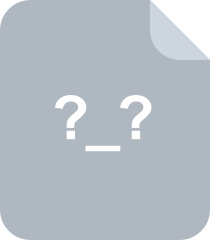
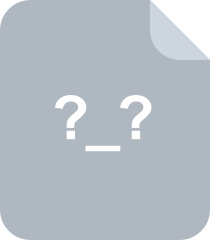
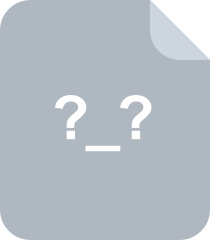
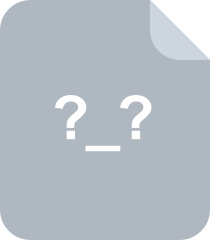
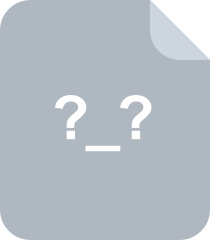
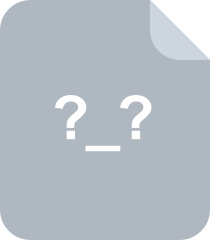
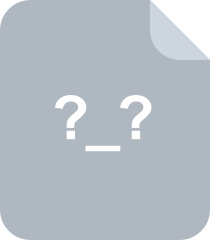
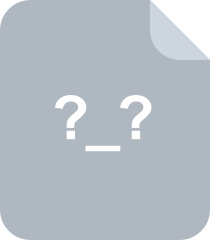
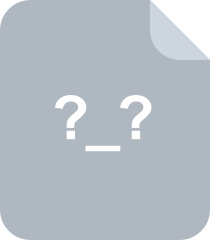
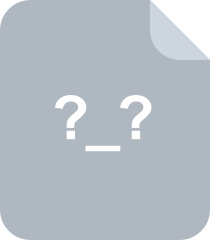
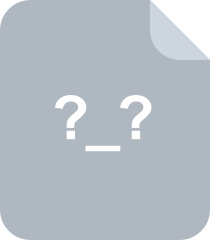
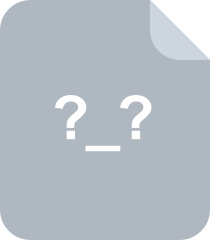
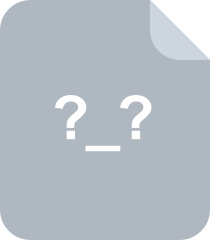
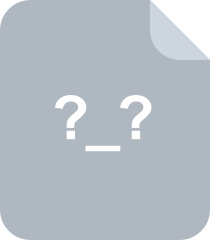
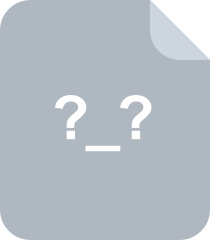
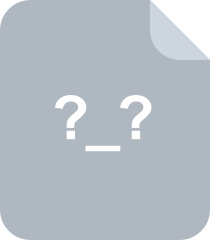
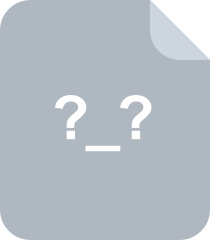
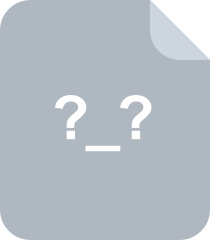
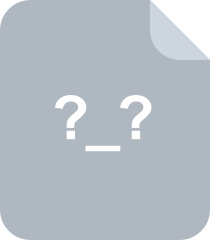
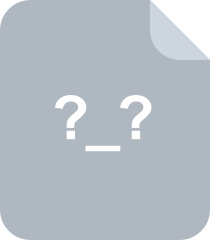
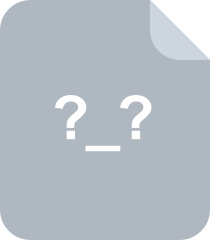
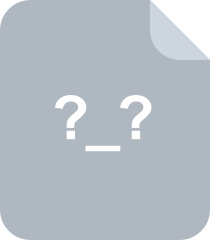
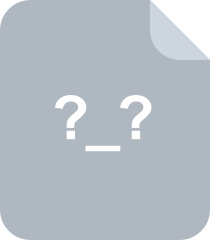
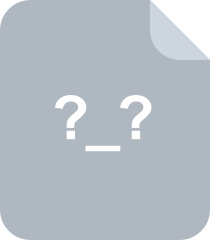
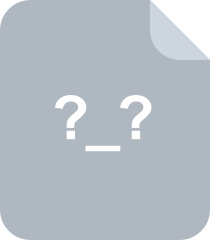
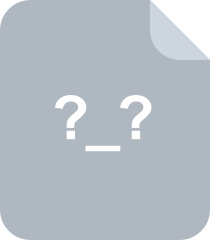
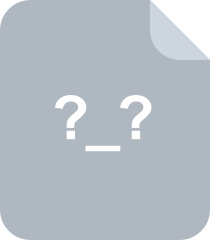
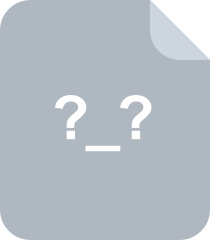
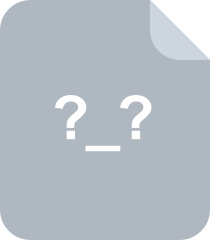
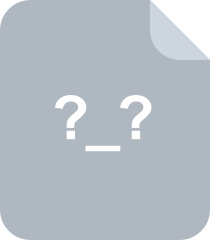
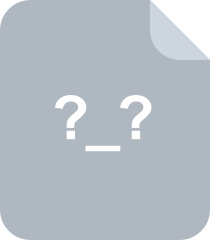
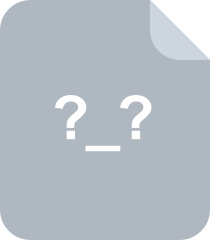
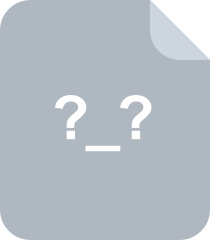
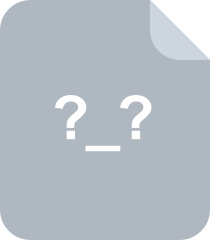
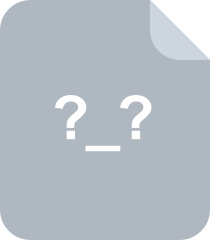
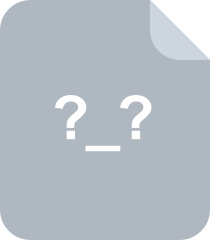
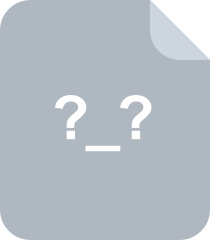
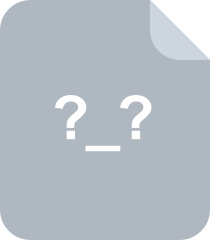
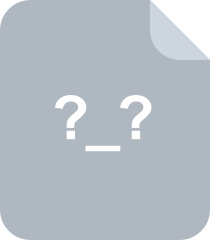
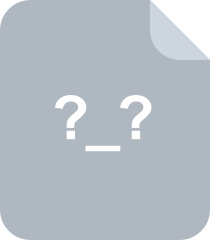
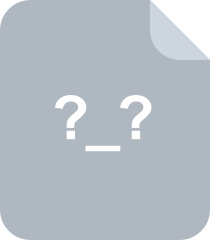
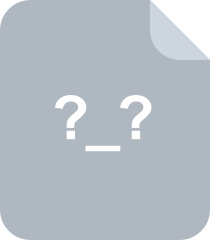
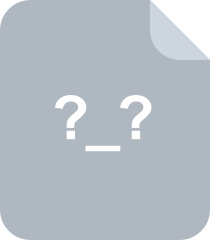
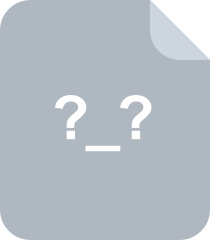
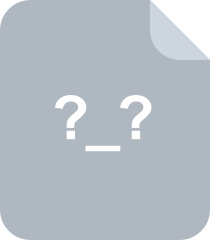
共 496 条
- 1
- 2
- 3
- 4
- 5
资源评论
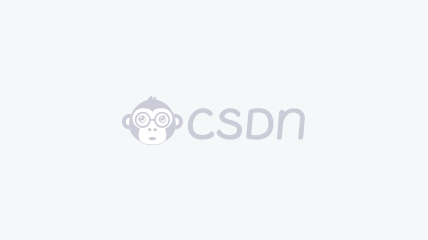
- BeHEll2023-04-23支持这个资源,内容详细,主要是能解决当下的问题,感谢大佬分享~

zy.gong
- 粉丝: 4
- 资源: 11
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

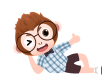
安全验证
文档复制为VIP权益,开通VIP直接复制
