#include <stdio.h>
#include <string.h>
#include <ctype.h>
#define MAX_PRODUCTS 50
#define MAX_RECORDS 50
// 商品库存结构体
struct ProductInventory {
char name[50]; //商品名称
int quantity; //商品库存数量
};
// 商品进销记录结构体
struct SalesRecord {
int product_id; //进销编号
char name[10]; //商品名称
char transaction_type[10]; // 进销类型,可以是进货或销售
float unit_price; //商品单价
int quantity; //商品数量
char date[12]; //进销日期
char note[20]; //备注
};
struct ProductInventory inventory[MAX_PRODUCTS]; //库存量数组
int inventory_count = 0; //库存数据条数
struct SalesRecord records[MAX_RECORDS]; //进销记录数组
int records_count = 0; //进销记录条数
//打印商品库存信息表格
void printInventoryTable() {
int i;
// 打印表头
printf("%-10s %-10s\n", "商品名称", "库存数量");
printf("-----------------------\n");
// 打印每条库存信息
for (i = 0; i < inventory_count; ++i) {
printf("%-10s %-10d\n", inventory[i].name, inventory[i].quantity);
}
}
// 辅助函数:检查商品编号是否重复
int isProductIDDuplicate(int product_id) {
int i;
for (i = 0; i < records_count; ++i) {
if (records[i].product_id == product_id) {
return 1; // 商品编号重复
}
}
return 0; // 商品编号不重复
}
// 辅助函数定义:检查日期格式是否正确
int isValidDateFormat(const char *date) {
int i;
if (strlen(date) != 10) {
return 0; // 日期长度不符合
}
// 检查每个字符是否符合日期格式要求
for (i = 0; i < 10; ++i) {
if (i == 4 || i == 7) {
if (date[i] != '-') {
return 0; // 位置为年份或月份分隔符不是 '-'
}
} else {
if (!isdigit(date[i])) {
return 0; // 不是数字
}
}
}
return 1; // 格式正确
}
// 辅助函数:根据商品名称查找库存信息索引
int findInventoryIndex(const char *name) {
int i;
for (i = 0; i < inventory_count; ++i) {
if (strcmp(inventory[i].name, name) == 0) {
return i; // 找到该商品名称,返回索引
}
}
return -1; // 没有找到该商品名称
}
// 添加进销记录到数组中
void addSalesRecord() {
int flag=0;
char answer[10];
do {
// 检查数组是否已满
if (records_count >= MAX_RECORDS) {
printf("错误: 进销记录数组已满,无法添加新记录。\n");
return;
}
struct SalesRecord newRecord;
// 用户输入新记录的信息
printf("请输入记录编号: ");
scanf("%d", &newRecord.product_id);
// 检查商品编号是否重复
if (isProductIDDuplicate(newRecord.product_id)) {
printf("错误: 商品编号 %d 已经存在,请输入唯一的商品编号。\n", newRecord.product_id);
return;
}
printf("请输入商品名称: ");
scanf("%s", newRecord.name);
int inventoryIndex = findInventoryIndex(newRecord.name);
// 输入进销类型,并检查是否为有效类型
printf("请输入进销类型 (进货/销售): ");
scanf("%s", newRecord.transaction_type);
// 若商品库存数组中不存在该商品,则无法销售
if (inventoryIndex == -1) {
printf("错误,商品库存不存在该商品,无法销售\n");
return;
}
// 检查进销类型是否为有效类型
if (strcmp(newRecord.transaction_type, "进货") != 0 && strcmp(newRecord.transaction_type, "销售") != 0) {
printf("错误: 进销类型只能是 '进货' 或 '销售',添加记录失败。\n");
return;
}
printf("请输入商品单价: ");
scanf("%f", &newRecord.unit_price);
printf("请输入商品数量: ");
scanf("%d", &newRecord.quantity);
// 销售操作,检查库存是否足够
if (strcmp(newRecord.transaction_type, "销售") == 0&&inventory[inventoryIndex].quantity < newRecord.quantity) {
printf("错误: 商品 %s 库存不足,无法销售。\n", newRecord.name);
return;
}
// 输入进销日期,并检查格式是否正确
printf("请输入进销日期 (YYYY-MM-DD): ");
scanf("%s", newRecord.date);
// 检查日期格式是否正确
if (!isValidDateFormat(newRecord.date)) {
printf("错误: 日期格式不正确,请输入有效的日期格式 'YYYY-MM-DD'。\n");
return;
}
printf("请输入备注: ");
scanf(" %[^\n]", newRecord.note);
// 在添加到记录数组之前,处理库存数组
if (strcmp(newRecord.transaction_type, "进货") == 0) {
if (inventoryIndex == -1) {
// 商品库存数组中不存在该商品,将其添加到库存数组中
strcpy(inventory[inventory_count].name, newRecord.name);
inventory[inventory_count].quantity = newRecord.quantity;
inventory_count++;
}
// 进货操作,增加库存量
inventory[inventoryIndex].quantity += newRecord.quantity;
} else if (strcmp(newRecord.transaction_type, "销售") == 0) {
// 减少库存量
inventory[inventoryIndex].quantity -= newRecord.quantity;
}
// 将新记录添加到数组末尾
records[records_count] = newRecord;
// 记录条数加一
records_count++;
printf("添加成功,是否继续添加进销记录?(是/否): ");
scanf("%s", answer);
if (strcmp(answer, "是") == 0) {
flag = 1; // 用户输入不是“是”,设置标志为0,结束循环
} else {
flag=0;
}
} while(flag);
}
// 打印商品进销记录数组内容
void printSalesRecords() {
int i;
printf("%-10s %-10s %-10s %-10s %-10s %-12s %-20s\n",
"编号", "名称", "类型", "单价", "数量", "日期", "备注");
printf("--------------------------------------------------------------------------------\n");
for (i = 0; i < records_count; ++i) {
printf("%-10d %-10s %-10s %-10.2f %-10d %-12s %-20s\n",
records[i].product_id, records[i].name, records[i].transaction_type,
records[i].unit_price, records[i].quantity, records[i].date, records[i].note);
}
}
// 查询并打印指定编号的进销记录
void findAndPrintRecordById() {
int search_id,i;
int found = 0;
// 接收用户输入的记录编号
printf("请输入要查询的记录编号: ");
scanf("%d", &search_id);
// 查找并打印记录
for (i = 0; i < records_count; ++i) {
if (records[i].product_id == search_id) {
printf("\n找到记录:\n");
printf("%-10s %-10s %-10s %-10s %-10s %-12s %-20s\n",
"编号", "名称", "类型", "单价", "数量", "日期", "备注");
printf("--------------------------------------------------------------------------------\n");
printf("%-10d %-10s %-10s %-10.2f %-10d %-12s %-20s\n",
records[i].product_id, records[i].name, records[i].transaction_type,
records[i].unit_price, records[i].quantity, records[i].date, records[i].note);
found = 1;
break;
}
}
if (!found) {
printf("\n未找到编号为 %d 的记录。\n", search_id);
}
}
// 根据用户输入进行排序并打印
void sortAndPrintRecords() {
struct SalesRecord sortedRecords[MAX_RECORDS];
int sortedCount = 0;
int choice,i,j;
// 打印菜单并接收用户输入
printf("请选择操作编号进行排序:\n");
printf("1. 排序进货数据\n");
printf("2. 排序销售数据\n");
printf("请输入操作编号: ");
scanf("%d", &choice);
// 根据用户选择将对应的记录复制到新数组中
for (i = 0; i < records_count; ++i) {
if ((choice == 1 && strcmp(records[i].transaction_type, "进货") == 0) ||
(choice == 2 && strcmp(records[i].transaction_type, "销售") == 0)) {
sortedRecords[sortedCount++] = records[i];
}
}
// 对新数组按商品数量进行降序排序
for (i = 0; i < sortedCount - 1; ++i) {
for (j = 0; j < sortedCount - 1 - i; ++j) {
if (sortedRecords[j].quantity < sortedRecords[j + 1].quantity) {
struct SalesRecord temp = sortedRecords[j];
sortedRecords[j] = sortedRecords[j + 1];
sortedRecords[j + 1] = temp;
}
}
}
// 打印排序后的记录
printf("\n%-10s %-10s %-10s %-10s %-10s %-12s %-20s\n",
"编号", "名称", "类型", "单价", "数量", "日期", "备注");
printf("--------------------------------------------------------------------------------\n");
for (i = 0; i < sortedCount; ++i) {
printf("%-10d %-10s %-10s %-10.2f %-10d %-12s %-20s\n",
sortedRecords[i].product_id, sortedRecords[i].name, sortedRecords[i].transaction_type,
sortedRecords[i].unit_price, sortedRecords[i].quantity, sortedRecords[i].date, sortedRecords[i].note);
}
}
// 比较日期大小
int compareDate(const char *date1, const char *date2) {
return strcmp(date1, date2);
}
// 计算总和
void sortAndCalculate() {
char start_date[11

小辰代写
- 粉丝: 4743
- 资源: 109
最新资源
- MATLAB代码:基于储能电站服务的冷热电多微网系统双层优化配置 关键词:储能电站 共享储能电站 冷热电多微网 双层优化配置 参考文档:《基于储能电站服务的冷热电多微网系统双层优化配置》完全复
- CarSim or TruckSim与Simulink联合仿真,使用键盘控制车辆加速,减速,转向,包含前进与后 档位切,支持自定义按键功能,支持拓展 提供carsim参数配置文件,导入即可运行 提供s
- COMSOL:AlGaAs纳米天线倍频产生(二次谐波非线性效应SHG)三维模型
- 内容介绍:考虑风光不确定性的微电网优化,机会约束描述风光不确定性,采用概率序列处理不确定问题 代码来源:原作者,非倒卖 代码说明:该代码主要贡献为不确定性处理和优化,可供大家学习参考
- 电动汽车 双层优化 选址定容 输配协同 时空优化 MATLAB代码:基于双层优化的电动汽车优化调度研究 参考文档:考虑大规模电动汽车接入电网的双层优化调度策略 仿真平台:MATLAB+CPLEX
- 基于拉丁超立方抽样的风,光,负荷场景生成方法 风电功率场景生成 ,光伏功率场景生成,负荷场景生成 通过后向场景削减BR得到典型场景及其概率 提供参考文献,完美复现 语言:MATLAB
- 西门子博途热力发电厂汽轮机控制系统程序画面案例,采用西门子S7-1516cpu,画面采用经典WINCC7.5 文件内容包含工艺流程图,电仪设计EPLAN图,及控制逻辑等 博图版本V16
- 51单片机PID法设计的开关电源Proteus仿真 功能描述如下: 1、220交流电输入整流滤波得到18 V直流,通过7805得到5v直流电给单片机供电; 2、18 V直流电经过开关变电路(buck
- 通过node Red的OPC UA节点,使用OPC UA server节点建立服务,量NX MCD作为客户端实现数据的交互 node Red+NX MCD轻松实现可视化界面的开发,实现远程控制,数据
- IM即时通讯APP源码搭建语音视频聊天交友软件
- Carsim和simulink联合仿真车辆状态估计 估计的状态为:横摆角速度,质心侧偏角,纵向车速,侧向车速 先基于滑模观测器SMO估计轮胎的纵向力和侧向力 ,再基于无迹卡尔曼UKF和容积卡尔曼CKF
- 三菱Q系列程序 三菱Q06UDV程序,搭配三菱MR-JE伺服,QD77MS16运动控制模块,QD70P8运动模块控制24轴运动,QX42.QY42P等输入输出模块 全自动iPad撕胶贴二维
- 负荷需求响应,matlab 在基于价格的需求侧管理模型研究中,首要任务便是建立负荷对价格的响应模型 有的文献中建立了价格型需求响应功率对电价的响应模型,认为两者之间是简单的线性关系 也有文献忽略了
- BYVIN 比德文知名厂家电动四轮车控制器代码,包含PCB文件,pdf原理图,代码齐全,风格很好
- 质子交膜燃料电池pemfc热管理水管理进气控制 固体氧化物燃料电池sofc 模糊控制,pid控制,模糊pid控制 自抗扰控制 bp-pid rbf-pid ga-pid pso-pid matlab
- 利用最小支持向量机LSSVM做拟合预测建模,数据要求是多列输入单列输出做拟合预测建模,程序内注释详细,直接替数据就可以用
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


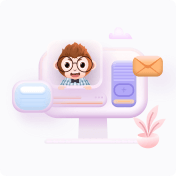