package com.example.weather;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.annotation.SuppressLint;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import org.jetbrains.annotations.NotNull;
import org.json.JSONArray;
import org.json.JSONObject;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.List;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
public static final int MSG_DOWN_OK=1;
public static final int MSG_DOWN_ERROR=2;
private EditText m_cityEdit;
private Button m_queryBtn;
private ListView m_listview;
private static String m_data="";
private List<Weather> m_datalist=null;//未来5天的数据
private Weather m_nowWeather;//实时数据
private DataAdapter listAdapter;
//uiHandler
private Handler uiHandler =new Handler(){
@Override
public void handleMessage(@NonNull Message msg) {
super.handleMessage(msg);
switch (msg.what){
case MSG_DOWN_OK:{
//更新ui
System.out.println("main thread get essage from down thread.");
displayDataToUI();
break;
}
case MSG_DOWN_ERROR:{
Toast.makeText(MainActivity.this,"下载天气预报失败!",Toast.LENGTH_LONG).show();
break;
}
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main_weather);
m_cityEdit=(EditText)findViewById(R.id.edit_city);
m_queryBtn=(Button)findViewById(R.id.btn_query);
m_listview=(ListView)findViewById(R.id.dataListview);
//设置背景透明度,需存在背景
View view_city=findViewById(R.id.layout_city);
//view_city.getBackground().setAlpha(100);
View view_now=findViewById(R.id.layout_nowweather);
view_now.getBackground().setAlpha(100);
View view_future=findViewById(R.id.layout_future);
view_future.getBackground().setAlpha(100);
View view_icon=findViewById(R.id.layout_icon);
view_icon.getBackground().setAlpha(100);
m_queryBtn.setOnClickListener(this);
//默认天气为北京
String defaultcity=m_cityEdit.getText().toString().trim();//默认为北京
//在子线程下载数据
DownThread mythread=new DownThread(defaultcity);
mythread.start();
}//end of onCreate
@Override
public void onClick(View v) {
try {
switch (v.getId()){
case R.id.btn_query:{
String cityname=m_cityEdit.getText().toString().trim();
//输入有效性
if(cityname.equals("")){
Toast.makeText(this,"请输入要查询的城市!",Toast.LENGTH_LONG).show();
return;
}
//String citynameutf8= URLEncoder.encode(cityname,"UTF-8");
//在子线程下载数据
DownThread mythread=new DownThread(cityname);
mythread.start();
break;
}
default:
break;
}
}catch (Exception e){
e.printStackTrace();
}
}//end of onClick
/*
显示数据到ui界面
*/
private void displayDataToUI(){
System.out.println("main:"+m_data);
if (parseJsonData()==false){
//提示
Toast.makeText(this,"下载的数据有错误!",Toast.LENGTH_LONG).show();
return;
}
displayNowWeather();
listAdapter=new DataAdapter(this,R.layout.list_weather,m_datalist,m_listview);
m_listview.setAdapter(listAdapter);
}//end of displayDataToUI
@SuppressLint("SetTextI18n")
private void displayNowWeather(){
ImageView image_nowweather=(ImageView)findViewById(R.id.image_nowweather);
TextView text_nowtemp=(TextView)findViewById(R.id.text_tempnow);//温度
TextView text_nowweather=(TextView)findViewById(R.id.text_situation);//天气情况
TextView text_nowdirection=(TextView)findViewById(R.id.text_direction);//风向
TextView text_nowpower=(TextView)findViewById(R.id.text_power);//风力
TextView text_nowquality=(TextView)findViewById(R.id.text_quality);//空气质量
TextView text_city=(TextView)findViewById(R.id.text_city);//当前城市
TextView text_humidity=(TextView)findViewById(R.id.text_humidity);//湿度
image_nowweather.setImageResource(DataAdapter.getIcon(m_nowWeather.getWid()));
text_nowtemp.setText(m_nowWeather.getTemperature()+"\u2103");
text_nowweather.setText(m_nowWeather.getWeather());
text_nowdirection.setText(m_nowWeather.getDirct());
text_nowpower.setText(m_nowWeather.getPower());
text_nowquality.setText(m_nowWeather.getAqi());
text_city.setText(m_cityEdit.getText().toString().trim());
text_humidity.setText(m_nowWeather.getHumidity());
}//end of displayNowWeather
/*
解析数据
in:m_data
out:m_datalist,m_nowWeather
*/
private boolean parseJsonData(){
try {
JSONObject object=new JSONObject(m_data);
if (object.getInt("error_code")!=0){
System.out.println(object.get("error_code")+":"+object.get("reason"));
return false;
}
m_datalist=new ArrayList<Weather>();
//实时数据
m_nowWeather=new Weather();
JSONObject nowObject=object.getJSONObject("result").getJSONObject("realtime");
m_nowWeather.setTemperature(nowObject.getString("temperature"));
m_nowWeather.setWid(nowObject.getString("wid"));
m_nowWeather.setWeather(nowObject.getString("info"));
m_nowWeather.setPower(nowObject.getString("power"));
m_nowWeather.setAqi(nowObject.getString("aqi"));
m_nowWeather.setDirct(nowObject.getString("direct"));
m_nowWeather.setHumidity(nowObject.getString("humidity"));
//未来5天的天气
JSONArray futureArray=object.getJSONObject("result").getJSONArray("future");
for (int i=0;i<futureArray.length();i++){
Weather tempWeather=new Weather();
JSONObject weatherObject=futureArray.getJSONObject(i);
tempWeather.setDatastr(weatherObject.getString("date"));
tempWeather.setTemperature(weatherObject.getString("temperature"));
tempWeather.setWid(weatherObject.getJSONObject("wid").getString("day"));
tempWeather.setWeather(weatherObject.getString("weather"));
tempWeather.setDirct(weatherObject.getString("direct"));
m_datalist.add(te
在Android开发领域,这些Demo是初学者和有经验开发者巩固基础、学习新技能的重要资源。以下将详细解析每个Demo的功能和涉及的关键知识点: 1. 身高计算器:这是一个简单的应用,用户可以输入身高数据(厘米或英寸),计算并显示对应的高度。涉及到的主要知识点包括: - Android布局设计:如XML布局文件,线性布局、相对布局等。 - 输入验证:检查用户输入的有效性,确保数据格式正确。 - 单位转换:实现厘米到英寸或反之的转换算法。 - UI更新:使用TextView动态显示计算结果。 2. 天气预报:这个Demo通常会展示未来几天的天气情况,包括温度、湿度、风力等。关键知识点: - 数据获取:可能通过网络API(如OpenWeatherMap)获取实时天气数据。 - JSON解析:处理从API获取的JSON数据,将其转化为可读格式。 - RecyclerView:显示多条天气预报信息,提高性能。 - 自定义Adapter和ViewHolder:适配RecyclerView,绑定数据到UI组件。 - 沙盒权限:请求互联网权限以获取数据。 3. 播放器:这个Demo可能包含音乐或视频播放功能。涉及知识点: - 媒体播放器API:使用MediaPlayer或ExoPlayer库进行音频/视频播放。 - 播放控制:实现播放、暂停、停止、快进、后退等功能。 - UI设计:包括播放进度条、音量调节、全屏切换等元素。 - 播放列表管理:处理多个媒体文件的播放顺序和切换。 4. 相机和传感器:这个Demo允许用户拍照或访问设备的各种传感器。关键点: - 摄像头API:使用Camera API或CameraX库进行拍照和录像。 - 图片处理:可能包括图片裁剪、旋转、保存到相册等操作。 - 传感器访问:通过SensorManager获取加速度计、陀螺仪等传感器数据。 - 实时数据可视化:将传感器数据展示在图表或UI上。 5. 联系人Demo:这个示例可能包含添加、查看、编辑联系人的功能。主要知识点: - 权限管理:请求读写联系人权限。 - ContentProvider:与系统联系人数据库交互。 - CursorLoader和LoaderManager:异步加载和更新联系人列表。 - Intent和IntentFilter:启动系统联系人选择器,共享数据。 - 数据库操作:可能涉及SQLite数据库的创建、查询、插入和更新。 这些Demo涵盖了Android开发中的基本组件、数据处理、网络通信和用户界面等多个方面,对理解Android应用开发的全貌非常有帮助。通过深入学习和实践这些代码,开发者可以增强对Android系统的理解和编程能力。

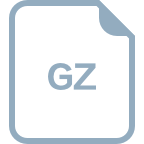

























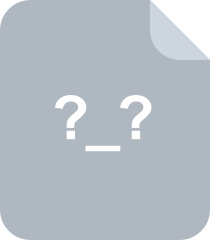
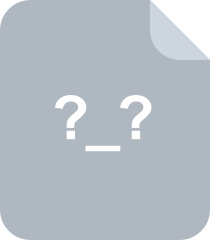
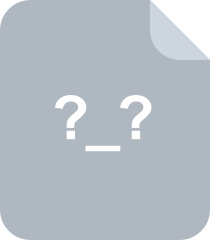
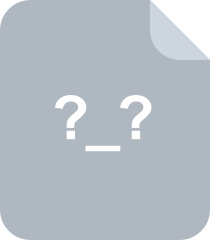
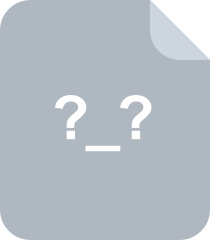
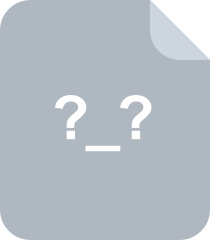
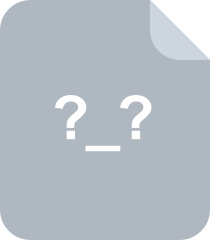
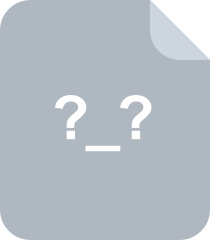
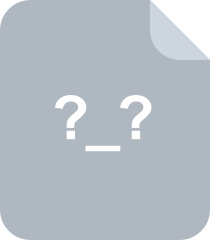
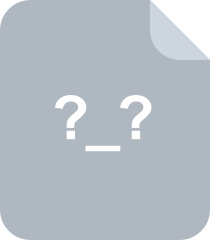
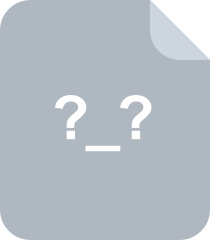
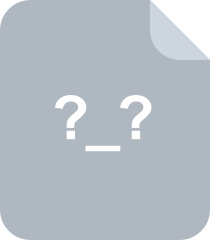
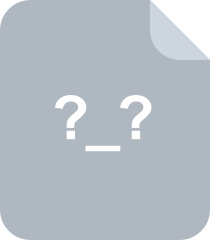
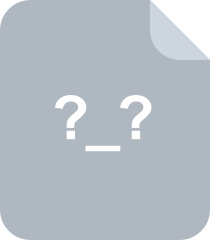
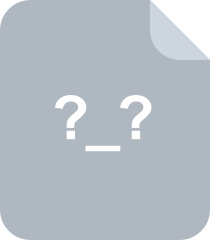
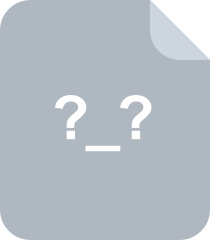
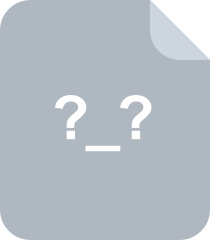
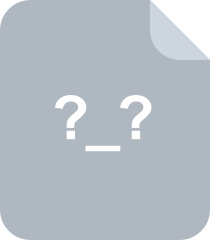
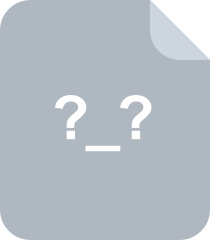
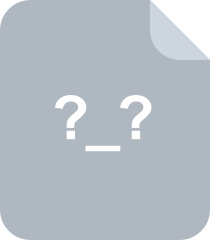
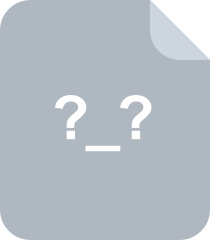
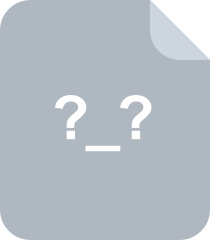
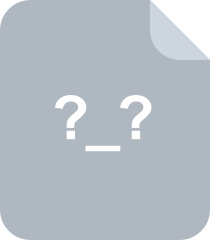
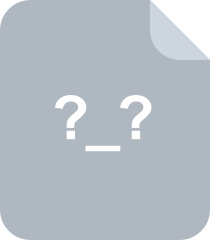
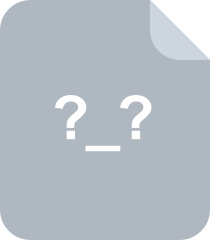
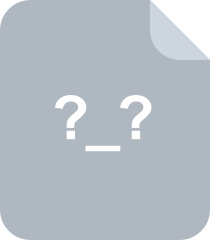
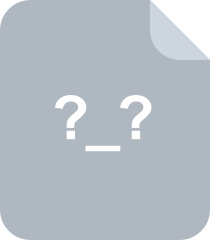
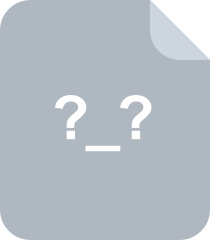
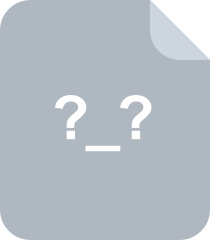
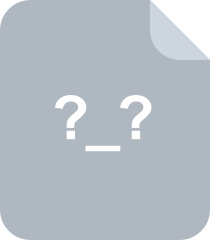
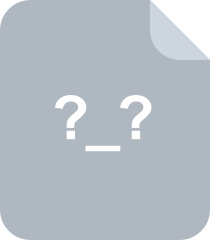
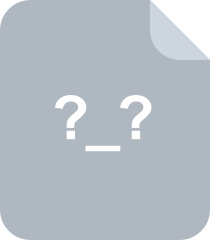
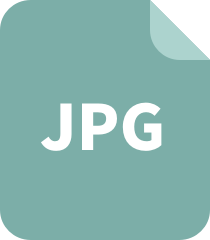
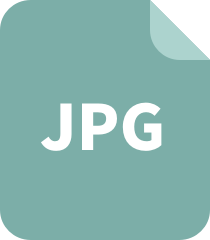
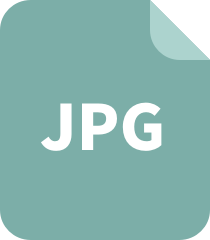
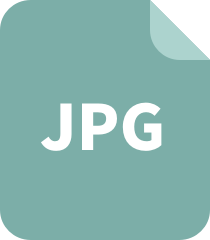
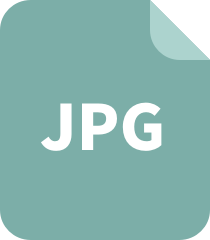
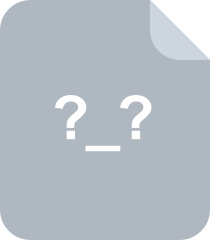
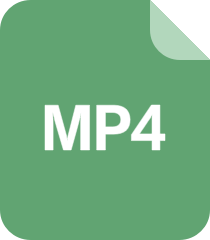
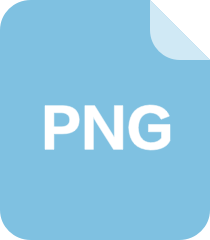
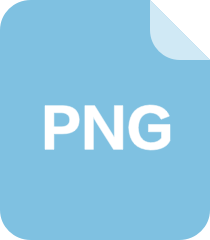
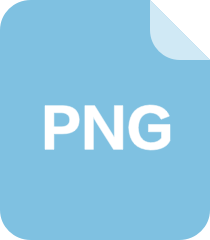
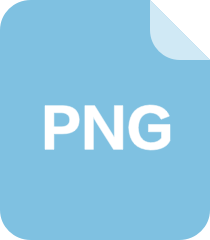
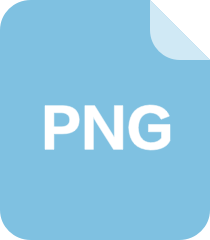
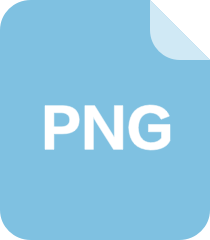
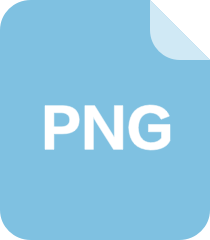
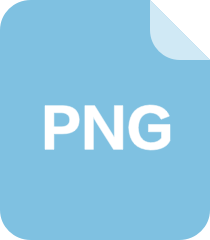
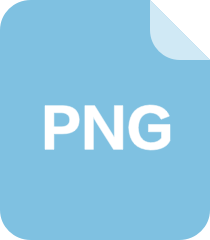
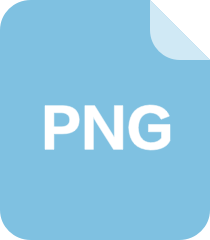
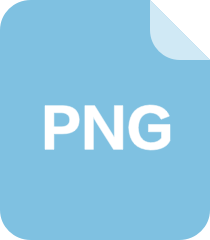
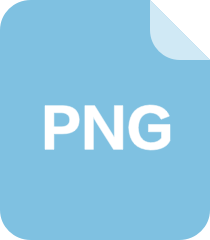
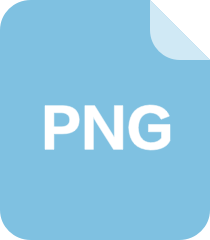
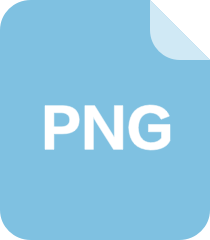
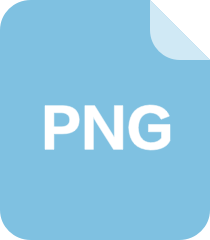
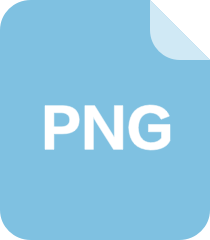
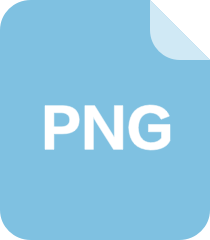
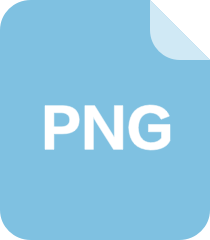
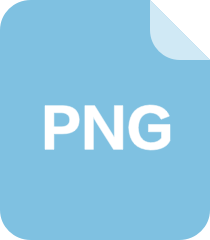
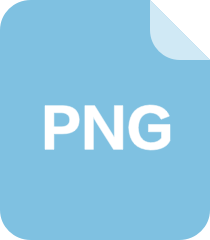
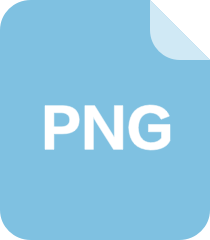
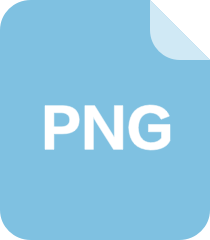
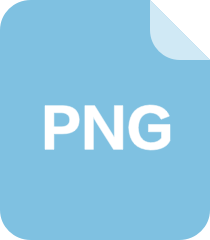
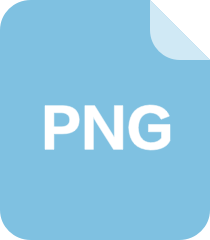
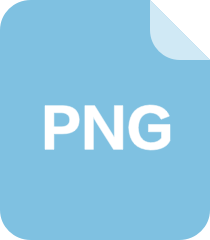
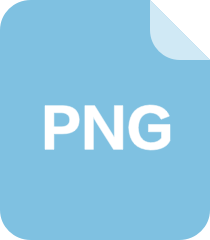
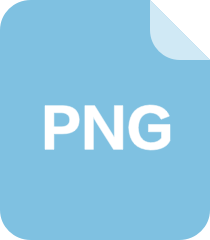
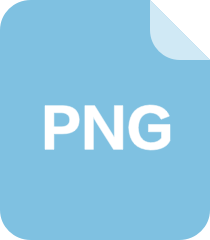
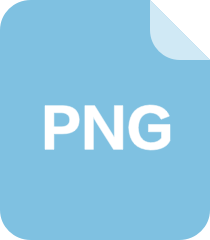
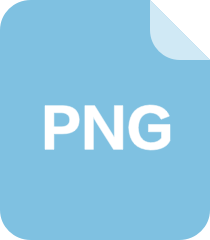
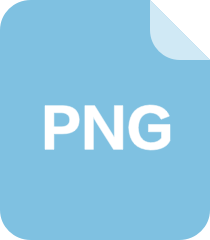
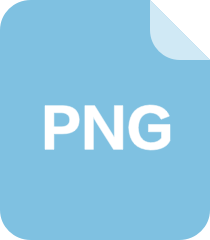
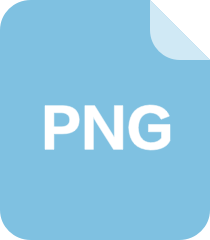
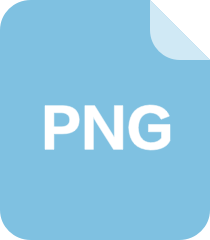
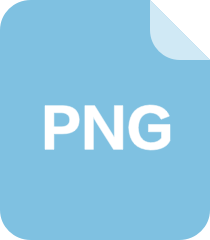
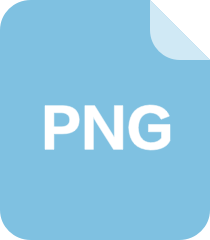
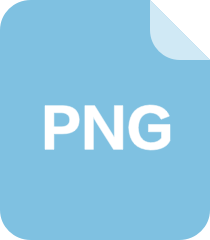
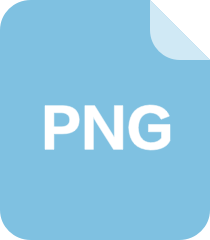
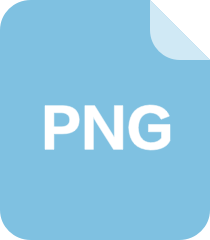
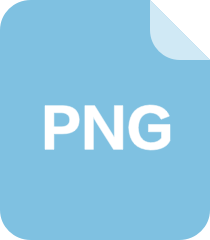
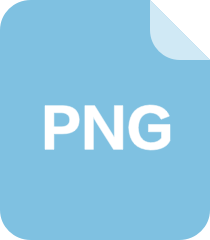
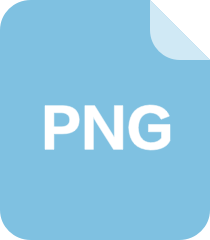
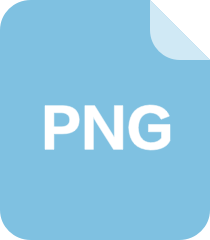
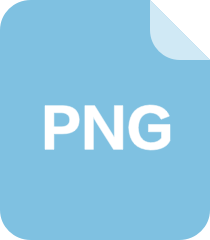
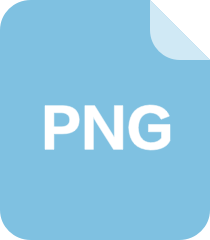
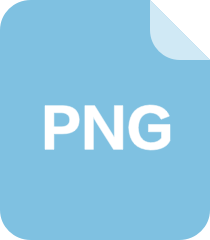
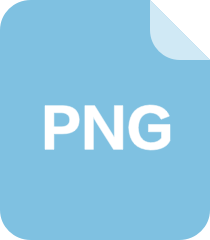
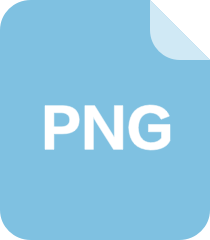
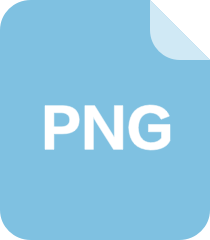
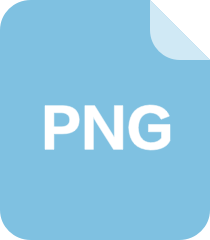
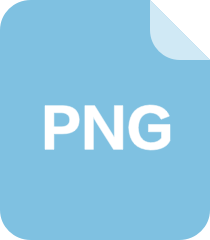
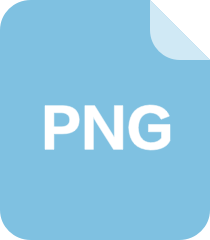
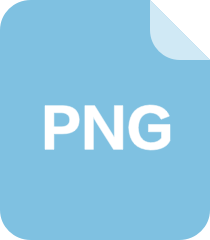
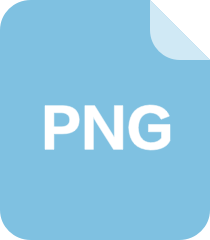
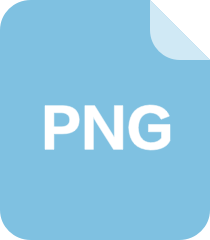
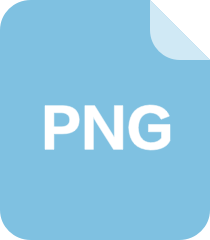
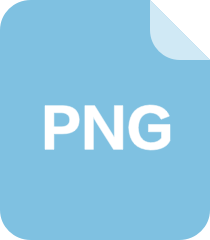
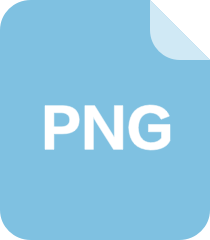
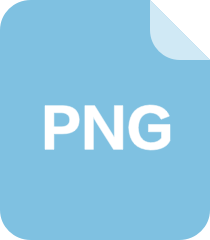
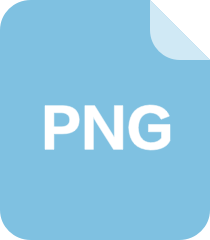
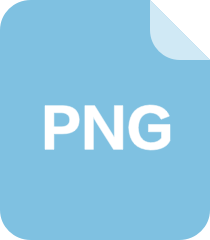
- 1
- 2
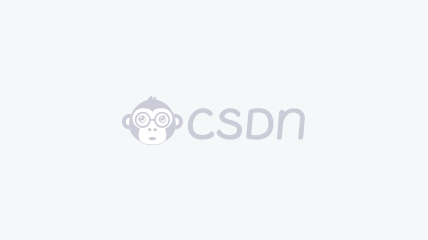
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

- 粉丝: 4915
- 资源: 3





我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

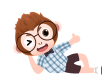
最新资源
- 【悬梁桥】基于matlab单段耦合脉动速度悬停桥频率域计算【含Matlab源码 12057期】.mp4
- 【相位平面】基于matlab GUI动态系统的相位平面【含Matlab源码 12047期】.mp4
- 【应力分析】基于matlab平面应力中薄板位移和应变有限元分析FEA【含Matlab源码 11118期】.mp4
- 【运动学】基于matlab汽车平顺性加权加速度评判标准【含Matlab源码 12039期】含使用说明.mp4
- 【运动学】基于matlab航天飞机旋翼在有限阻力下的运动的微分方程【含Matlab源码 11166期】.mp4
- 【运动学】基于matlab追踪法导弹打飞机仿真【含Matlab源码 11016期】.mp4
- 【重力仿真】基于matlab GUI水平圆柱体重力异常正演【含Matlab源码 11176期】.mp4
- 【轴承压力】基于matlab GUI止推轴承压力计算【含Matlab源码 12069期】.mp4
- 【轴向压缩能力】基于matlab GUI计算CFDST柱的轴向压缩能力【含Matlab源码 9990期】.mp4
- 096-FreeRTOS+LCD1602+ADS1015 application.rar
- log凑字数 12345678910
- 基于三菱PLC与组态王技术的恒温加热炉温度控制:梯形图程序、接线图与组态画面详解,基于三菱PLC与组态王技术的恒温加热炉精准温度控制解决方案,基于三菱PLC和组态王恒温控制系统的设计加热炉温度控制 带
- 基于PSO-XGBoost算法的交叉验证多变量时间序列预测模型优化研究,基于PSO-XGBoost算法的交叉验证多变量时间序列预测模型优化研究,基于粒子群优化算法优化XGBoost的(PSO-XGBo
- 【PID仿真】基于matlab PID模拟水泥行业稳料仓重流程仿真【含Matlab源码 12028期】.mp4
- 【控制成本】基于matlab集群网络的计算效率保证成本控制设计【含Matlab源码 9833期】.mp4
- 【控制器】基于matlab固定优先级调度和控制器共同设计【含Matlab源码 13040期】.mp4

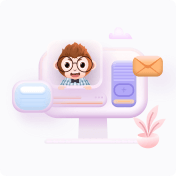
